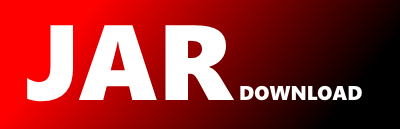
com.amazonaws.services.cloudfront.model.ViewerCertificate Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A complex type that specifies the following:
*
*
* -
*
* Whether you want viewers to use HTTP or HTTPS to request your objects.
*
*
* -
*
* If you want viewers to use HTTPS, whether you're using an alternate domain name such as example.com
or
* the CloudFront domain name for your distribution, such as d111111abcdef8.cloudfront.net
.
*
*
* -
*
* If you're using an alternate domain name, whether AWS Certificate Manager (ACM) provided the certificate, or you
* purchased a certificate from a third-party certificate authority and imported it into ACM or uploaded it to the IAM
* certificate store.
*
*
*
*
* Specify only one of the following values:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* For more information, see
* Using Alternate Domain Names and HTTPS in the Amazon CloudFront Developer Guide.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ViewerCertificate implements Serializable, Cloneable {
/**
*
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*
*/
private Boolean cloudFrontDefaultCertificate;
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if you purchased your
* certificate from a third-party certificate authority:
*
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to the IAM
* certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for SSLSupportMethod
.
*
*/
private String iAMCertificateId;
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if ACM provided your
* certificate:
*
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
where
* ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate that you want to
* use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for SSLSupportMethod
.
*
*/
private String aCMCertificateArn;
/**
*
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a method that
* works for browsers and clients released after 2010 or one that works for all clients.
*
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name Indication
* (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of the browsers that
* support SNI, see the Wikipedia entry Server Name
* Indication. To learn about options to explore if you have users with browsers that don't include SNI support,
* see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS requests from
* any viewer. However, there are additional monthly charges. For details, including specific pricing information,
* see Custom SSL options for Amazon CloudFront
* on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*/
private String sSLSupportMethod;
/**
*
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy determines
* two settings:
*
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices that do
* not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically sets the
* security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and ciphers
* that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the Amazon
* CloudFront Developer Guide.
*
*/
private String minimumProtocolVersion;
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*/
@Deprecated
private String certificate;
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*/
@Deprecated
private String certificateSource;
/**
*
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*
*
* @param cloudFrontDefaultCertificate
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*/
public void setCloudFrontDefaultCertificate(Boolean cloudFrontDefaultCertificate) {
this.cloudFrontDefaultCertificate = cloudFrontDefaultCertificate;
}
/**
*
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*
*
* @return If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*/
public Boolean getCloudFrontDefaultCertificate() {
return this.cloudFrontDefaultCertificate;
}
/**
*
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*
*
* @param cloudFrontDefaultCertificate
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ViewerCertificate withCloudFrontDefaultCertificate(Boolean cloudFrontDefaultCertificate) {
setCloudFrontDefaultCertificate(cloudFrontDefaultCertificate);
return this;
}
/**
*
* If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*
*
* @return If you're using the CloudFront domain name for your distribution, such as
* d111111abcdef8.cloudfront.net
, specify the following value:
*
* -
*
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
*
*
*/
public Boolean isCloudFrontDefaultCertificate() {
return this.cloudFrontDefaultCertificate;
}
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if you purchased your
* certificate from a third-party certificate authority:
*
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to the IAM
* certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for SSLSupportMethod
.
*
*
* @param iAMCertificateId
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you
* must choose the type of certificate that you want to use. Specify the following value if you purchased
* your certificate from a third-party certificate authority:
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to the
* IAM certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for
* SSLSupportMethod
.
*/
public void setIAMCertificateId(String iAMCertificateId) {
this.iAMCertificateId = iAMCertificateId;
}
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if you purchased your
* certificate from a third-party certificate authority:
*
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to the IAM
* certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for SSLSupportMethod
.
*
*
* @return If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you
* must choose the type of certificate that you want to use. Specify the following value if you purchased
* your certificate from a third-party certificate authority:
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to
* the IAM certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for
* SSLSupportMethod
.
*/
public String getIAMCertificateId() {
return this.iAMCertificateId;
}
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if you purchased your
* certificate from a third-party certificate authority:
*
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to the IAM
* certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for SSLSupportMethod
.
*
*
* @param iAMCertificateId
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you
* must choose the type of certificate that you want to use. Specify the following value if you purchased
* your certificate from a third-party certificate authority:
*
* -
*
* <IAMCertificateId>IAM certificate ID<IAMCertificateId>
where
* IAM certificate ID
is the ID that IAM returned when you added the certificate to the
* IAM certificate store.
*
*
*
*
* If you specify IAMCertificateId
, you must also specify a value for
* SSLSupportMethod
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ViewerCertificate withIAMCertificateId(String iAMCertificateId) {
setIAMCertificateId(iAMCertificateId);
return this;
}
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if ACM provided your
* certificate:
*
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
where
* ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate that you want to
* use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for SSLSupportMethod
.
*
*
* @param aCMCertificateArn
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you
* must choose the type of certificate that you want to use. Specify the following value if ACM provided your
* certificate:
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
* where ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate
* that you want to use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for
* SSLSupportMethod
.
*/
public void setACMCertificateArn(String aCMCertificateArn) {
this.aCMCertificateArn = aCMCertificateArn;
}
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if ACM provided your
* certificate:
*
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
where
* ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate that you want to
* use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for SSLSupportMethod
.
*
*
* @return If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you
* must choose the type of certificate that you want to use. Specify the following value if ACM provided
* your certificate:
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
* where ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate
* that you want to use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for
* SSLSupportMethod
.
*/
public String getACMCertificateArn() {
return this.aCMCertificateArn;
}
/**
*
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you must
* choose the type of certificate that you want to use. Specify the following value if ACM provided your
* certificate:
*
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
where
* ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate that you want to
* use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for SSLSupportMethod
.
*
*
* @param aCMCertificateArn
* If you want viewers to use HTTPS to request your objects and you're using an alternate domain name, you
* must choose the type of certificate that you want to use. Specify the following value if ACM provided your
* certificate:
*
* -
*
* <ACMCertificateArn>ARN for ACM SSL/TLS certificate<ACMCertificateArn>
* where ARN for ACM SSL/TLS certificate
is the ARN for the ACM SSL/TLS certificate
* that you want to use for this distribution.
*
*
*
*
* If you specify ACMCertificateArn
, you must also specify a value for
* SSLSupportMethod
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ViewerCertificate withACMCertificateArn(String aCMCertificateArn) {
setACMCertificateArn(aCMCertificateArn);
return this;
}
/**
*
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a method that
* works for browsers and clients released after 2010 or one that works for all clients.
*
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name Indication
* (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of the browsers that
* support SNI, see the Wikipedia entry Server Name
* Indication. To learn about options to explore if you have users with browsers that don't include SNI support,
* see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS requests from
* any viewer. However, there are additional monthly charges. For details, including specific pricing information,
* see Custom SSL options for Amazon CloudFront
* on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* @param sSLSupportMethod
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a
* method that works for browsers and clients released after 2010 or one that works for all clients.
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name
* Indication (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of
* the browsers that support SNI, see the Wikipedia entry Server Name Indication. To
* learn about options to explore if you have users with browsers that don't include SNI support, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS
* requests from any viewer. However, there are additional monthly charges. For details, including specific
* pricing information, see Custom SSL options
* for Amazon CloudFront on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
* @see SSLSupportMethod
*/
public void setSSLSupportMethod(String sSLSupportMethod) {
this.sSLSupportMethod = sSLSupportMethod;
}
/**
*
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a method that
* works for browsers and clients released after 2010 or one that works for all clients.
*
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name Indication
* (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of the browsers that
* support SNI, see the Wikipedia entry Server Name
* Indication. To learn about options to explore if you have users with browsers that don't include SNI support,
* see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS requests from
* any viewer. However, there are additional monthly charges. For details, including specific pricing information,
* see Custom SSL options for Amazon CloudFront
* on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* @return If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a
* method that works for browsers and clients released after 2010 or one that works for all clients.
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name
* Indication (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of
* the browsers that support SNI, see the Wikipedia entry Server Name Indication. To
* learn about options to explore if you have users with browsers that don't include SNI support, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS
* requests from any viewer. However, there are additional monthly charges. For details, including specific
* pricing information, see Custom SSL
* options for Amazon CloudFront on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
* @see SSLSupportMethod
*/
public String getSSLSupportMethod() {
return this.sSLSupportMethod;
}
/**
*
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a method that
* works for browsers and clients released after 2010 or one that works for all clients.
*
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name Indication
* (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of the browsers that
* support SNI, see the Wikipedia entry Server Name
* Indication. To learn about options to explore if you have users with browsers that don't include SNI support,
* see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS requests from
* any viewer. However, there are additional monthly charges. For details, including specific pricing information,
* see Custom SSL options for Amazon CloudFront
* on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* @param sSLSupportMethod
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a
* method that works for browsers and clients released after 2010 or one that works for all clients.
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name
* Indication (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of
* the browsers that support SNI, see the Wikipedia entry Server Name Indication. To
* learn about options to explore if you have users with browsers that don't include SNI support, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS
* requests from any viewer. However, there are additional monthly charges. For details, including specific
* pricing information, see Custom SSL options
* for Amazon CloudFront on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SSLSupportMethod
*/
public ViewerCertificate withSSLSupportMethod(String sSLSupportMethod) {
setSSLSupportMethod(sSLSupportMethod);
return this;
}
/**
*
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a method that
* works for browsers and clients released after 2010 or one that works for all clients.
*
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name Indication
* (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of the browsers that
* support SNI, see the Wikipedia entry Server Name
* Indication. To learn about options to explore if you have users with browsers that don't include SNI support,
* see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS requests from
* any viewer. However, there are additional monthly charges. For details, including specific pricing information,
* see Custom SSL options for Amazon CloudFront
* on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* @param sSLSupportMethod
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a
* method that works for browsers and clients released after 2010 or one that works for all clients.
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name
* Indication (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of
* the browsers that support SNI, see the Wikipedia entry Server Name Indication. To
* learn about options to explore if you have users with browsers that don't include SNI support, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS
* requests from any viewer. However, there are additional monthly charges. For details, including specific
* pricing information, see Custom SSL options
* for Amazon CloudFront on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
* @see SSLSupportMethod
*/
public void setSSLSupportMethod(SSLSupportMethod sSLSupportMethod) {
withSSLSupportMethod(sSLSupportMethod);
}
/**
*
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a method that
* works for browsers and clients released after 2010 or one that works for all clients.
*
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name Indication
* (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of the browsers that
* support SNI, see the Wikipedia entry Server Name
* Indication. To learn about options to explore if you have users with browsers that don't include SNI support,
* see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS requests from
* any viewer. However, there are additional monthly charges. For details, including specific pricing information,
* see Custom SSL options for Amazon CloudFront
* on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* @param sSLSupportMethod
* If you specify a value for ACMCertificateArn or for IAMCertificateId, you must also specify how you want CloudFront to serve HTTPS requests: using a
* method that works for browsers and clients released after 2010 or one that works for all clients.
*
* -
*
* sni-only
: CloudFront can respond to HTTPS requests from viewers that support Server Name
* Indication (SNI). All modern browsers support SNI, but there are a few that don't. For a current list of
* the browsers that support SNI, see the Wikipedia entry Server Name Indication. To
* learn about options to explore if you have users with browsers that don't include SNI support, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
*
*
* -
*
* vip
: CloudFront uses dedicated IP addresses for your content and can respond to HTTPS
* requests from any viewer. However, there are additional monthly charges. For details, including specific
* pricing information, see Custom SSL options
* for Amazon CloudFront on the AWS marketing site.
*
*
*
*
* Don't specify a value for SSLSupportMethod
if you specified
* <CloudFrontDefaultCertificate>true<CloudFrontDefaultCertificate>
.
*
*
* For more information, see Choosing How CloudFront Serves HTTPS Requests in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SSLSupportMethod
*/
public ViewerCertificate withSSLSupportMethod(SSLSupportMethod sSLSupportMethod) {
this.sSLSupportMethod = sSLSupportMethod.toString();
return this;
}
/**
*
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy determines
* two settings:
*
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices that do
* not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically sets the
* security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and ciphers
* that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the Amazon
* CloudFront Developer Guide.
*
*
* @param minimumProtocolVersion
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy
* determines two settings:
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices
* that do not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically
* sets the security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and
* ciphers that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the
* Amazon CloudFront Developer Guide.
* @see MinimumProtocolVersion
*/
public void setMinimumProtocolVersion(String minimumProtocolVersion) {
this.minimumProtocolVersion = minimumProtocolVersion;
}
/**
*
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy determines
* two settings:
*
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices that do
* not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically sets the
* security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and ciphers
* that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the Amazon
* CloudFront Developer Guide.
*
*
* @return Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy
* determines two settings:
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices
* that do not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security
* policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically
* sets the security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and
* ciphers that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the
* Amazon CloudFront Developer Guide.
* @see MinimumProtocolVersion
*/
public String getMinimumProtocolVersion() {
return this.minimumProtocolVersion;
}
/**
*
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy determines
* two settings:
*
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices that do
* not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically sets the
* security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and ciphers
* that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the Amazon
* CloudFront Developer Guide.
*
*
* @param minimumProtocolVersion
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy
* determines two settings:
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices
* that do not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically
* sets the security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and
* ciphers that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the
* Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MinimumProtocolVersion
*/
public ViewerCertificate withMinimumProtocolVersion(String minimumProtocolVersion) {
setMinimumProtocolVersion(minimumProtocolVersion);
return this;
}
/**
*
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy determines
* two settings:
*
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices that do
* not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically sets the
* security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and ciphers
* that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the Amazon
* CloudFront Developer Guide.
*
*
* @param minimumProtocolVersion
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy
* determines two settings:
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices
* that do not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically
* sets the security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and
* ciphers that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the
* Amazon CloudFront Developer Guide.
* @see MinimumProtocolVersion
*/
public void setMinimumProtocolVersion(MinimumProtocolVersion minimumProtocolVersion) {
withMinimumProtocolVersion(minimumProtocolVersion);
}
/**
*
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy determines
* two settings:
*
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices that do
* not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically sets the
* security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and ciphers
* that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the Amazon
* CloudFront Developer Guide.
*
*
* @param minimumProtocolVersion
* Specify the security policy that you want CloudFront to use for HTTPS connections. A security policy
* determines two settings:
*
* -
*
* The minimum SSL/TLS protocol that CloudFront uses to communicate with viewers
*
*
* -
*
* The cipher that CloudFront uses to encrypt the content that it returns to viewers
*
*
*
*
*
* On the CloudFront console, this setting is called Security policy.
*
*
*
* We recommend that you specify TLSv1.1_2016
unless your users are using browsers or devices
* that do not support TLSv1.1 or later.
*
*
* When both of the following are true, you must specify TLSv1
or later for the security policy:
*
*
* -
*
* You're using a custom certificate: you specified a value for ACMCertificateArn
or for
* IAMCertificateId
*
*
* -
*
* You're using SNI: you specified sni-only
for SSLSupportMethod
*
*
*
*
* If you specify true
for CloudFrontDefaultCertificate
, CloudFront automatically
* sets the security policy to TLSv1
regardless of the value that you specify for
* MinimumProtocolVersion
.
*
*
* For information about the relationship between the security policy that you choose and the protocols and
* ciphers that CloudFront uses to communicate with viewers, see Supported SSL/TLS Protocols and Ciphers for Communication Between Viewers and CloudFront in the
* Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MinimumProtocolVersion
*/
public ViewerCertificate withMinimumProtocolVersion(MinimumProtocolVersion minimumProtocolVersion) {
this.minimumProtocolVersion = minimumProtocolVersion.toString();
return this;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @param certificate
* This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*/
@Deprecated
public void setCertificate(String certificate) {
this.certificate = certificate;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @return This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*/
@Deprecated
public String getCertificate() {
return this.certificate;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @param certificate
* This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public ViewerCertificate withCertificate(String certificate) {
setCertificate(certificate);
return this;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @param certificateSource
* This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
* @see CertificateSource
*/
@Deprecated
public void setCertificateSource(String certificateSource) {
this.certificateSource = certificateSource;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @return This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
* @see CertificateSource
*/
@Deprecated
public String getCertificateSource() {
return this.certificateSource;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @param certificateSource
* This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CertificateSource
*/
@Deprecated
public ViewerCertificate withCertificateSource(String certificateSource) {
setCertificateSource(certificateSource);
return this;
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @param certificateSource
* This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
* @see CertificateSource
*/
@Deprecated
public void setCertificateSource(CertificateSource certificateSource) {
withCertificateSource(certificateSource);
}
/**
*
* This field is no longer used. Use one of the following fields instead:
*
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
*
*
* @param certificateSource
* This field is no longer used. Use one of the following fields instead:
*
* -
*
*
* -
*
* IAMCertificateId
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CertificateSource
*/
@Deprecated
public ViewerCertificate withCertificateSource(CertificateSource certificateSource) {
this.certificateSource = certificateSource.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCloudFrontDefaultCertificate() != null)
sb.append("CloudFrontDefaultCertificate: ").append(getCloudFrontDefaultCertificate()).append(",");
if (getIAMCertificateId() != null)
sb.append("IAMCertificateId: ").append(getIAMCertificateId()).append(",");
if (getACMCertificateArn() != null)
sb.append("ACMCertificateArn: ").append(getACMCertificateArn()).append(",");
if (getSSLSupportMethod() != null)
sb.append("SSLSupportMethod: ").append(getSSLSupportMethod()).append(",");
if (getMinimumProtocolVersion() != null)
sb.append("MinimumProtocolVersion: ").append(getMinimumProtocolVersion()).append(",");
if (getCertificate() != null)
sb.append("Certificate: ").append(getCertificate()).append(",");
if (getCertificateSource() != null)
sb.append("CertificateSource: ").append(getCertificateSource());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ViewerCertificate == false)
return false;
ViewerCertificate other = (ViewerCertificate) obj;
if (other.getCloudFrontDefaultCertificate() == null ^ this.getCloudFrontDefaultCertificate() == null)
return false;
if (other.getCloudFrontDefaultCertificate() != null && other.getCloudFrontDefaultCertificate().equals(this.getCloudFrontDefaultCertificate()) == false)
return false;
if (other.getIAMCertificateId() == null ^ this.getIAMCertificateId() == null)
return false;
if (other.getIAMCertificateId() != null && other.getIAMCertificateId().equals(this.getIAMCertificateId()) == false)
return false;
if (other.getACMCertificateArn() == null ^ this.getACMCertificateArn() == null)
return false;
if (other.getACMCertificateArn() != null && other.getACMCertificateArn().equals(this.getACMCertificateArn()) == false)
return false;
if (other.getSSLSupportMethod() == null ^ this.getSSLSupportMethod() == null)
return false;
if (other.getSSLSupportMethod() != null && other.getSSLSupportMethod().equals(this.getSSLSupportMethod()) == false)
return false;
if (other.getMinimumProtocolVersion() == null ^ this.getMinimumProtocolVersion() == null)
return false;
if (other.getMinimumProtocolVersion() != null && other.getMinimumProtocolVersion().equals(this.getMinimumProtocolVersion()) == false)
return false;
if (other.getCertificate() == null ^ this.getCertificate() == null)
return false;
if (other.getCertificate() != null && other.getCertificate().equals(this.getCertificate()) == false)
return false;
if (other.getCertificateSource() == null ^ this.getCertificateSource() == null)
return false;
if (other.getCertificateSource() != null && other.getCertificateSource().equals(this.getCertificateSource()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCloudFrontDefaultCertificate() == null) ? 0 : getCloudFrontDefaultCertificate().hashCode());
hashCode = prime * hashCode + ((getIAMCertificateId() == null) ? 0 : getIAMCertificateId().hashCode());
hashCode = prime * hashCode + ((getACMCertificateArn() == null) ? 0 : getACMCertificateArn().hashCode());
hashCode = prime * hashCode + ((getSSLSupportMethod() == null) ? 0 : getSSLSupportMethod().hashCode());
hashCode = prime * hashCode + ((getMinimumProtocolVersion() == null) ? 0 : getMinimumProtocolVersion().hashCode());
hashCode = prime * hashCode + ((getCertificate() == null) ? 0 : getCertificate().hashCode());
hashCode = prime * hashCode + ((getCertificateSource() == null) ? 0 : getCertificateSource().hashCode());
return hashCode;
}
@Override
public ViewerCertificate clone() {
try {
return (ViewerCertificate) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}