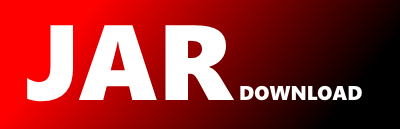
com.amazonaws.services.cloudfront.AmazonCloudFrontAsync Maven / Gradle / Ivy
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront;
import com.amazonaws.services.cloudfront.model.*;
/**
* Interface for accessing CloudFront asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudfront.AbstractAmazonCloudFrontAsync} instead.
*
*
* Amazon CloudFront
*
* This is the Amazon CloudFront API Reference. This guide is for developers who need detailed information about
* the CloudFront API actions, data types, and errors. For detailed information about CloudFront features and their
* associated API calls, see the Amazon CloudFront Developer Guide.
*
*/
public interface AmazonCloudFrontAsync extends AmazonCloudFront {
/**
*
* Creates a new origin access identity. If you're using Amazon S3 for your origin, you can use an origin access
* identity to require users to access your content using a CloudFront URL instead of the Amazon S3 URL. For more
* information about how to use origin access identities, see Serving Private
* Content through CloudFront in the Amazon CloudFront Developer Guide.
*
*
* @param createCloudFrontOriginAccessIdentityRequest
* The request to create a new origin access identity.
* @return A Java Future containing the result of the CreateCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFrontOriginAccessIdentityAsync(
CreateCloudFrontOriginAccessIdentityRequest createCloudFrontOriginAccessIdentityRequest);
/**
*
* Creates a new origin access identity. If you're using Amazon S3 for your origin, you can use an origin access
* identity to require users to access your content using a CloudFront URL instead of the Amazon S3 URL. For more
* information about how to use origin access identities, see Serving Private
* Content through CloudFront in the Amazon CloudFront Developer Guide.
*
*
* @param createCloudFrontOriginAccessIdentityRequest
* The request to create a new origin access identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFrontOriginAccessIdentityAsync(
CreateCloudFrontOriginAccessIdentityRequest createCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new web distribution. Send a GET
request to the
* /CloudFront API version/distribution
/distribution ID
resource.
*
*
* @param createDistributionRequest
* The request to create a new distribution.
* @return A Java Future containing the result of the CreateDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDistributionAsync(CreateDistributionRequest createDistributionRequest);
/**
*
* Creates a new web distribution. Send a GET
request to the
* /CloudFront API version/distribution
/distribution ID
resource.
*
*
* @param createDistributionRequest
* The request to create a new distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDistributionAsync(CreateDistributionRequest createDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new distribution with tags.
*
*
* @param createDistributionWithTagsRequest
* The request to create a new distribution with tags.
* @return A Java Future containing the result of the CreateDistributionWithTags operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createDistributionWithTagsAsync(
CreateDistributionWithTagsRequest createDistributionWithTagsRequest);
/**
*
* Create a new distribution with tags.
*
*
* @param createDistributionWithTagsRequest
* The request to create a new distribution with tags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDistributionWithTags operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createDistributionWithTagsAsync(
CreateDistributionWithTagsRequest createDistributionWithTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new invalidation.
*
*
* @param createInvalidationRequest
* The request to create an invalidation.
* @return A Java Future containing the result of the CreateInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateInvalidation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInvalidationAsync(CreateInvalidationRequest createInvalidationRequest);
/**
*
* Create a new invalidation.
*
*
* @param createInvalidationRequest
* The request to create an invalidation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateInvalidation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInvalidationAsync(CreateInvalidationRequest createInvalidationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new RMTP distribution. An RTMP distribution is similar to a web distribution, but an RTMP distribution
* streams media files using the Adobe Real-Time Messaging Protocol (RTMP) instead of serving files using HTTP.
*
*
* To create a new web distribution, submit a POST
request to the CloudFront API
* version/distribution resource. The request body must include a document with a
* StreamingDistributionConfig element. The response echoes the StreamingDistributionConfig
* element and returns other information about the RTMP distribution.
*
*
* To get the status of your request, use the GET StreamingDistribution API action. When the value of
* Enabled
is true
and the value of Status
is Deployed
, your
* distribution is ready. A distribution usually deploys in less than 15 minutes.
*
*
* For more information about web distributions, see Working with RTMP
* Distributions in the Amazon CloudFront Developer Guide.
*
*
*
* Beginning with the 2012-05-05 version of the CloudFront API, we made substantial changes to the format of the XML
* document that you include in the request body when you create or update a web distribution or an RTMP
* distribution, and when you invalidate objects. With previous versions of the API, we discovered that it was too
* easy to accidentally delete one or more values for an element that accepts multiple values, for example, CNAMEs
* and trusted signers. Our changes for the 2012-05-05 release are intended to prevent these accidental deletions
* and to notify you when there's a mismatch between the number of values you say you're specifying in the
* Quantity
element and the number of values specified.
*
*
*
* @param createStreamingDistributionRequest
* The request to create a new streaming distribution.
* @return A Java Future containing the result of the CreateStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionAsync(
CreateStreamingDistributionRequest createStreamingDistributionRequest);
/**
*
* Creates a new RMTP distribution. An RTMP distribution is similar to a web distribution, but an RTMP distribution
* streams media files using the Adobe Real-Time Messaging Protocol (RTMP) instead of serving files using HTTP.
*
*
* To create a new web distribution, submit a POST
request to the CloudFront API
* version/distribution resource. The request body must include a document with a
* StreamingDistributionConfig element. The response echoes the StreamingDistributionConfig
* element and returns other information about the RTMP distribution.
*
*
* To get the status of your request, use the GET StreamingDistribution API action. When the value of
* Enabled
is true
and the value of Status
is Deployed
, your
* distribution is ready. A distribution usually deploys in less than 15 minutes.
*
*
* For more information about web distributions, see Working with RTMP
* Distributions in the Amazon CloudFront Developer Guide.
*
*
*
* Beginning with the 2012-05-05 version of the CloudFront API, we made substantial changes to the format of the XML
* document that you include in the request body when you create or update a web distribution or an RTMP
* distribution, and when you invalidate objects. With previous versions of the API, we discovered that it was too
* easy to accidentally delete one or more values for an element that accepts multiple values, for example, CNAMEs
* and trusted signers. Our changes for the 2012-05-05 release are intended to prevent these accidental deletions
* and to notify you when there's a mismatch between the number of values you say you're specifying in the
* Quantity
element and the number of values specified.
*
*
*
* @param createStreamingDistributionRequest
* The request to create a new streaming distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionAsync(
CreateStreamingDistributionRequest createStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new streaming distribution with tags.
*
*
* @param createStreamingDistributionWithTagsRequest
* The request to create a new streaming distribution with tags.
* @return A Java Future containing the result of the CreateStreamingDistributionWithTags operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateStreamingDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionWithTagsAsync(
CreateStreamingDistributionWithTagsRequest createStreamingDistributionWithTagsRequest);
/**
*
* Create a new streaming distribution with tags.
*
*
* @param createStreamingDistributionWithTagsRequest
* The request to create a new streaming distribution with tags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStreamingDistributionWithTags operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateStreamingDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionWithTagsAsync(
CreateStreamingDistributionWithTagsRequest createStreamingDistributionWithTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an origin access identity.
*
*
* @param deleteCloudFrontOriginAccessIdentityRequest
* Deletes a origin access identity.
* @return A Java Future containing the result of the DeleteCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.DeleteCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCloudFrontOriginAccessIdentityAsync(
DeleteCloudFrontOriginAccessIdentityRequest deleteCloudFrontOriginAccessIdentityRequest);
/**
*
* Delete an origin access identity.
*
*
* @param deleteCloudFrontOriginAccessIdentityRequest
* Deletes a origin access identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.DeleteCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCloudFrontOriginAccessIdentityAsync(
DeleteCloudFrontOriginAccessIdentityRequest deleteCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a distribution.
*
*
* @param deleteDistributionRequest
* This action deletes a web distribution. To delete a web distribution using the CloudFront API, perform the
* following steps.
*
* To delete a web distribution using the CloudFront API:
*
*
* -
*
* Disable the web distribution
*
*
* -
*
* Submit a GET Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Distribution Config
request to update the configuration for your distribution.
* In the request body, include the XML document that you updated in Step 3. Set the value of the HTTP
* If-Match
header to the value of the ETag
header that CloudFront returned when
* you submitted the GET Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Distribution Config
request to confirm that the distribution
* was successfully disabled.
*
*
* -
*
* Submit a GET Distribution
request to confirm that your changes have propagated. When
* propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Distribution
request. Set the value of the HTTP If-Match
header
* to the value of the ETag
header that CloudFront returned when you submitted the
* GET Distribution Config
request in Step 6.
*
*
* -
*
* Review the response to your DELETE Distribution
request to confirm that the distribution was
* successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a Distribution in the Amazon CloudFront Developer Guide.
* @return A Java Future containing the result of the DeleteDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDistributionAsync(DeleteDistributionRequest deleteDistributionRequest);
/**
*
* Delete a distribution.
*
*
* @param deleteDistributionRequest
* This action deletes a web distribution. To delete a web distribution using the CloudFront API, perform the
* following steps.
*
* To delete a web distribution using the CloudFront API:
*
*
* -
*
* Disable the web distribution
*
*
* -
*
* Submit a GET Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Distribution Config
request to update the configuration for your distribution.
* In the request body, include the XML document that you updated in Step 3. Set the value of the HTTP
* If-Match
header to the value of the ETag
header that CloudFront returned when
* you submitted the GET Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Distribution Config
request to confirm that the distribution
* was successfully disabled.
*
*
* -
*
* Submit a GET Distribution
request to confirm that your changes have propagated. When
* propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Distribution
request. Set the value of the HTTP If-Match
header
* to the value of the ETag
header that CloudFront returned when you submitted the
* GET Distribution Config
request in Step 6.
*
*
* -
*
* Review the response to your DELETE Distribution
request to confirm that the distribution was
* successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a Distribution in the Amazon CloudFront Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDistributionAsync(DeleteDistributionRequest deleteDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a streaming distribution. To delete an RTMP distribution using the CloudFront API, perform the following
* steps.
*
*
* To delete an RTMP distribution using the CloudFront API:
*
*
* -
*
* Disable the RTMP distribution.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Streaming Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Streaming Distribution Config
request to update the configuration for your
* distribution. In the request body, include the XML document that you updated in Step 3. Then set the value of the
* HTTP If-Match
header to the value of the ETag
header that CloudFront returned when you
* submitted the GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Streaming Distribution Config
request to confirm that the
* distribution was successfully disabled.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to confirm that your changes have propagated.
* When propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Streaming Distribution
request. Set the value of the HTTP If-Match
* header to the value of the ETag
header that CloudFront returned when you submitted the
* GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to your DELETE Streaming Distribution
request to confirm that the distribution
* was successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a
* Distribution in the Amazon CloudFront Developer Guide.
*
*
* @param deleteStreamingDistributionRequest
* The request to delete a streaming distribution.
* @return A Java Future containing the result of the DeleteStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStreamingDistributionAsync(
DeleteStreamingDistributionRequest deleteStreamingDistributionRequest);
/**
*
* Delete a streaming distribution. To delete an RTMP distribution using the CloudFront API, perform the following
* steps.
*
*
* To delete an RTMP distribution using the CloudFront API:
*
*
* -
*
* Disable the RTMP distribution.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Streaming Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Streaming Distribution Config
request to update the configuration for your
* distribution. In the request body, include the XML document that you updated in Step 3. Then set the value of the
* HTTP If-Match
header to the value of the ETag
header that CloudFront returned when you
* submitted the GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Streaming Distribution Config
request to confirm that the
* distribution was successfully disabled.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to confirm that your changes have propagated.
* When propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Streaming Distribution
request. Set the value of the HTTP If-Match
* header to the value of the ETag
header that CloudFront returned when you submitted the
* GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to your DELETE Streaming Distribution
request to confirm that the distribution
* was successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a
* Distribution in the Amazon CloudFront Developer Guide.
*
*
* @param deleteStreamingDistributionRequest
* The request to delete a streaming distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStreamingDistributionAsync(
DeleteStreamingDistributionRequest deleteStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityRequest
* The request to get an origin access identity's information.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityAsync(
GetCloudFrontOriginAccessIdentityRequest getCloudFrontOriginAccessIdentityRequest);
/**
*
* Get the information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityRequest
* The request to get an origin access identity's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityAsync(
GetCloudFrontOriginAccessIdentityRequest getCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the configuration information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityConfigRequest
* The origin access identity's configuration information. For more information, see
* CloudFrontOriginAccessIdentityConfigComplexType.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentityConfig operation returned by
* the service.
* @sample AmazonCloudFrontAsync.GetCloudFrontOriginAccessIdentityConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityConfigAsync(
GetCloudFrontOriginAccessIdentityConfigRequest getCloudFrontOriginAccessIdentityConfigRequest);
/**
*
* Get the configuration information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityConfigRequest
* The origin access identity's configuration information. For more information, see
* CloudFrontOriginAccessIdentityConfigComplexType.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentityConfig operation returned by
* the service.
* @sample AmazonCloudFrontAsyncHandler.GetCloudFrontOriginAccessIdentityConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityConfigAsync(
GetCloudFrontOriginAccessIdentityConfigRequest getCloudFrontOriginAccessIdentityConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the information about a distribution.
*
*
* @param getDistributionRequest
* The request to get a distribution's information.
* @return A Java Future containing the result of the GetDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.GetDistribution
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDistributionAsync(GetDistributionRequest getDistributionRequest);
/**
*
* Get the information about a distribution.
*
*
* @param getDistributionRequest
* The request to get a distribution's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetDistribution
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDistributionAsync(GetDistributionRequest getDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the configuration information about a distribution.
*
*
* @param getDistributionConfigRequest
* The request to get a distribution configuration.
* @return A Java Future containing the result of the GetDistributionConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.GetDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getDistributionConfigAsync(GetDistributionConfigRequest getDistributionConfigRequest);
/**
*
* Get the configuration information about a distribution.
*
*
* @param getDistributionConfigRequest
* The request to get a distribution configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDistributionConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getDistributionConfigAsync(GetDistributionConfigRequest getDistributionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the information about an invalidation.
*
*
* @param getInvalidationRequest
* The request to get an invalidation's information.
* @return A Java Future containing the result of the GetInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsync.GetInvalidation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInvalidationAsync(GetInvalidationRequest getInvalidationRequest);
/**
*
* Get the information about an invalidation.
*
*
* @param getInvalidationRequest
* The request to get an invalidation's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetInvalidation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInvalidationAsync(GetInvalidationRequest getInvalidationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a specified RTMP distribution, including the distribution configuration.
*
*
* @param getStreamingDistributionRequest
* The request to get a streaming distribution's information.
* @return A Java Future containing the result of the GetStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.GetStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionAsync(GetStreamingDistributionRequest getStreamingDistributionRequest);
/**
*
* Gets information about a specified RTMP distribution, including the distribution configuration.
*
*
* @param getStreamingDistributionRequest
* The request to get a streaming distribution's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionAsync(GetStreamingDistributionRequest getStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the configuration information about a streaming distribution.
*
*
* @param getStreamingDistributionConfigRequest
* To request to get a streaming distribution configuration.
* @return A Java Future containing the result of the GetStreamingDistributionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetStreamingDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionConfigAsync(
GetStreamingDistributionConfigRequest getStreamingDistributionConfigRequest);
/**
*
* Get the configuration information about a streaming distribution.
*
*
* @param getStreamingDistributionConfigRequest
* To request to get a streaming distribution configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStreamingDistributionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetStreamingDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionConfigAsync(
GetStreamingDistributionConfigRequest getStreamingDistributionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists origin access identities.
*
*
* @param listCloudFrontOriginAccessIdentitiesRequest
* The request to list origin access identities.
* @return A Java Future containing the result of the ListCloudFrontOriginAccessIdentities operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListCloudFrontOriginAccessIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listCloudFrontOriginAccessIdentitiesAsync(
ListCloudFrontOriginAccessIdentitiesRequest listCloudFrontOriginAccessIdentitiesRequest);
/**
*
* Lists origin access identities.
*
*
* @param listCloudFrontOriginAccessIdentitiesRequest
* The request to list origin access identities.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCloudFrontOriginAccessIdentities operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListCloudFrontOriginAccessIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listCloudFrontOriginAccessIdentitiesAsync(
ListCloudFrontOriginAccessIdentitiesRequest listCloudFrontOriginAccessIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List distributions.
*
*
* @param listDistributionsRequest
* The request to list your distributions.
* @return A Java Future containing the result of the ListDistributions operation returned by the service.
* @sample AmazonCloudFrontAsync.ListDistributions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDistributionsAsync(ListDistributionsRequest listDistributionsRequest);
/**
*
* List distributions.
*
*
* @param listDistributionsRequest
* The request to list your distributions.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributions operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDistributionsAsync(ListDistributionsRequest listDistributionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the distributions that are associated with a specified AWS WAF web ACL.
*
*
* @param listDistributionsByWebACLIdRequest
* The request to list distributions that are associated with a specified AWS WAF web ACL.
* @return A Java Future containing the result of the ListDistributionsByWebACLId operation returned by the service.
* @sample AmazonCloudFrontAsync.ListDistributionsByWebACLId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByWebACLIdAsync(
ListDistributionsByWebACLIdRequest listDistributionsByWebACLIdRequest);
/**
*
* List the distributions that are associated with a specified AWS WAF web ACL.
*
*
* @param listDistributionsByWebACLIdRequest
* The request to list distributions that are associated with a specified AWS WAF web ACL.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByWebACLId operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByWebACLId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByWebACLIdAsync(
ListDistributionsByWebACLIdRequest listDistributionsByWebACLIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists invalidation batches.
*
*
* @param listInvalidationsRequest
* The request to list invalidations.
* @return A Java Future containing the result of the ListInvalidations operation returned by the service.
* @sample AmazonCloudFrontAsync.ListInvalidations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listInvalidationsAsync(ListInvalidationsRequest listInvalidationsRequest);
/**
*
* Lists invalidation batches.
*
*
* @param listInvalidationsRequest
* The request to list invalidations.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInvalidations operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListInvalidations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listInvalidationsAsync(ListInvalidationsRequest listInvalidationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List streaming distributions.
*
*
* @param listStreamingDistributionsRequest
* The request to list your streaming distributions.
* @return A Java Future containing the result of the ListStreamingDistributions operation returned by the service.
* @sample AmazonCloudFrontAsync.ListStreamingDistributions
* @see AWS API Documentation
*/
java.util.concurrent.Future listStreamingDistributionsAsync(
ListStreamingDistributionsRequest listStreamingDistributionsRequest);
/**
*
* List streaming distributions.
*
*
* @param listStreamingDistributionsRequest
* The request to list your streaming distributions.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStreamingDistributions operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListStreamingDistributions
* @see AWS API Documentation
*/
java.util.concurrent.Future listStreamingDistributionsAsync(
ListStreamingDistributionsRequest listStreamingDistributionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List tags for a CloudFront resource.
*
*
* @param listTagsForResourceRequest
* The request to list tags for a CloudFront resource.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonCloudFrontAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List tags for a CloudFront resource.
*
*
* @param listTagsForResourceRequest
* The request to list tags for a CloudFront resource.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add tags to a CloudFront resource.
*
*
* @param tagResourceRequest
* The request to add tags to a CloudFront resource.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonCloudFrontAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Add tags to a CloudFront resource.
*
*
* @param tagResourceRequest
* The request to add tags to a CloudFront resource.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove tags from a CloudFront resource.
*
*
* @param untagResourceRequest
* The request to remove tags from a CloudFront resource.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonCloudFrontAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove tags from a CloudFront resource.
*
*
* @param untagResourceRequest
* The request to remove tags from a CloudFront resource.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update an origin access identity.
*
*
* @param updateCloudFrontOriginAccessIdentityRequest
* The request to update an origin access identity.
* @return A Java Future containing the result of the UpdateCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.UpdateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCloudFrontOriginAccessIdentityAsync(
UpdateCloudFrontOriginAccessIdentityRequest updateCloudFrontOriginAccessIdentityRequest);
/**
*
* Update an origin access identity.
*
*
* @param updateCloudFrontOriginAccessIdentityRequest
* The request to update an origin access identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.UpdateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCloudFrontOriginAccessIdentityAsync(
UpdateCloudFrontOriginAccessIdentityRequest updateCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update a distribution.
*
*
* @param updateDistributionRequest
* The request to update a distribution.
* @return A Java Future containing the result of the UpdateDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.UpdateDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDistributionAsync(UpdateDistributionRequest updateDistributionRequest);
/**
*
* Update a distribution.
*
*
* @param updateDistributionRequest
* The request to update a distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.UpdateDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDistributionAsync(UpdateDistributionRequest updateDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update a streaming distribution.
*
*
* @param updateStreamingDistributionRequest
* The request to update a streaming distribution.
* @return A Java Future containing the result of the UpdateStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.UpdateStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future updateStreamingDistributionAsync(
UpdateStreamingDistributionRequest updateStreamingDistributionRequest);
/**
*
* Update a streaming distribution.
*
*
* @param updateStreamingDistributionRequest
* The request to update a streaming distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.UpdateStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future updateStreamingDistributionAsync(
UpdateStreamingDistributionRequest updateStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}