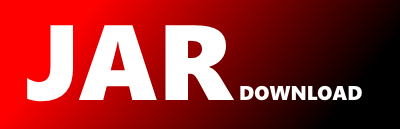
com.amazonaws.services.cloudfront.AmazonCloudFrontClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.cloudfront.AmazonCloudFrontClientBuilder;
import com.amazonaws.services.cloudfront.waiters.AmazonCloudFrontWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.cloudfront.model.*;
import com.amazonaws.services.cloudfront.model.transform.*;
/**
* Client for accessing CloudFront. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* Amazon CloudFront
*
* This is the Amazon CloudFront API Reference. This guide is for developers who need detailed information about
* CloudFront API actions, data types, and errors. For detailed information about CloudFront features, see the Amazon
* CloudFront Developer Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonCloudFrontClient extends AmazonWebServiceClient implements AmazonCloudFront {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonCloudFront.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "cloudfront";
private volatile AmazonCloudFrontWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
/**
* List of exception unmarshallers for all modeled exceptions
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on CloudFront. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonCloudFrontClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonCloudFrontClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudFront. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudFront (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonCloudFrontClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonCloudFrontClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonCloudFrontClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AmazonCloudFrontClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonCloudFrontClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudFront (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AmazonCloudFrontClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonCloudFrontClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonCloudFrontClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonCloudFrontClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonCloudFrontClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudFront (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AmazonCloudFrontClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonCloudFrontClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonCloudFrontClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudFront (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonCloudFrontClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonCloudFrontClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonCloudFrontClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonCloudFrontClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AmazonCloudFrontClientBuilder builder() {
return AmazonCloudFrontClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonCloudFrontClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on CloudFront using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonCloudFrontClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
exceptionUnmarshallers.add(new StreamingDistributionAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TrustedSignerDoesNotExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchInvalidationExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidWebACLIdExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchFieldLevelEncryptionConfigExceptionUnmarshaller());
exceptionUnmarshallers.add(new OriginRequestPolicyAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyHeadersInForwardedValuesExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidOriginReadTimeoutExceptionUnmarshaller());
exceptionUnmarshallers.add(new OriginRequestPolicyInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidMinimumProtocolVersionExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionCNAMEsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyInvalidationsInProgressExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyFieldLevelEncryptionConfigsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyPublicKeysExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidErrorCodeExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCacheBehaviorsExceptionUnmarshaller());
exceptionUnmarshallers.add(new CloudFrontOriginAccessIdentityInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new FieldLevelEncryptionProfileAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyFieldLevelEncryptionFieldPatternsExceptionUnmarshaller());
exceptionUnmarshallers.add(new MissingBodyExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyOriginsExceptionUnmarshaller());
exceptionUnmarshallers.add(new IllegalFieldLevelEncryptionConfigAssociationWithCacheBehaviorExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidTTLOrderExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidRequiredProtocolExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchOriginRequestPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchOriginExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyQueryStringsInOriginRequestPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyTrustedSignersExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionsWithSingleFunctionARNExceptionUnmarshaller());
exceptionUnmarshallers.add(new BatchTooLargeExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidResponseCodeExceptionUnmarshaller());
exceptionUnmarshallers.add(new FieldLevelEncryptionConfigAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidOriginKeepaliveTimeoutExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyStreamingDistributionsExceptionUnmarshaller());
exceptionUnmarshallers.add(new PublicKeyInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new StreamingDistributionNotDisabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new PreconditionFailedExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyQueryStringParametersExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyFieldLevelEncryptionEncryptionEntitiesExceptionUnmarshaller());
exceptionUnmarshallers.add(new FieldLevelEncryptionConfigInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new DistributionAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyStreamingDistributionCNAMEsExceptionUnmarshaller());
exceptionUnmarshallers.add(new CloudFrontOriginAccessIdentityAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new CachePolicyAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidProtocolSettingsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyFieldLevelEncryptionProfilesExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyOriginCustomHeadersExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidLocationCodeExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidForwardCookiesExceptionUnmarshaller());
exceptionUnmarshallers.add(new FieldLevelEncryptionProfileSizeExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCertificatesExceptionUnmarshaller());
exceptionUnmarshallers.add(new QueryArgProfileEmptyExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidOriginAccessIdentityExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidQueryStringParametersExceptionUnmarshaller());
exceptionUnmarshallers.add(new CNAMEAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new IllegalUpdateExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCookieNamesInWhiteListExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyHeadersInCachePolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchDistributionExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchFieldLevelEncryptionProfileExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchResourceExceptionUnmarshaller());
exceptionUnmarshallers.add(new InconsistentQuantitiesExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidLambdaFunctionAssociationExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyLambdaFunctionAssociationsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidViewerCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCachePoliciesExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyQueryStringsInCachePolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new DistributionNotDisabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyOriginGroupsPerDistributionExceptionUnmarshaller());
exceptionUnmarshallers.add(new CachePolicyInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDefaultRootObjectExceptionUnmarshaller());
exceptionUnmarshallers.add(new AccessDeniedExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCookiesInCachePolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidArgumentExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyOriginRequestPoliciesExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchCachePolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchStreamingDistributionExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCloudFrontOriginAccessIdentitiesExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchPublicKeyExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyFieldLevelEncryptionContentTypeProfilesExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidRelativePathExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionsAssociatedToFieldLevelEncryptionConfigExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionsWithLambdaAssociationsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionsAssociatedToCachePolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidHeadersForS3OriginExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyDistributionsAssociatedToOriginRequestPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new CannotChangeImmutablePublicKeyFieldsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyFieldLevelEncryptionQueryArgProfilesExceptionUnmarshaller());
exceptionUnmarshallers.add(new PublicKeyAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new FieldLevelEncryptionProfileInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyCookiesInOriginRequestPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new IllegalDeleteExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidIfMatchVersionExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidTaggingExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidOriginExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyHeadersInOriginRequestPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchCloudFrontOriginAccessIdentityExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidGeoRestrictionParameterExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.cloudfront.model.AmazonCloudFrontException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("https://cloudfront.amazonaws.com/");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/cloudfront/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/cloudfront/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a cache policy.
*
*
* After you create a cache policy, you can attach it to one or more cache behaviors. When it’s attached to a cache
* behavior, the cache policy determines the following:
*
*
* -
*
* The values that CloudFront includes in the cache key. These values can include HTTP headers, cookies, and
* URL query strings. CloudFront uses the cache key to find an object in its cache that it can return to the viewer.
*
*
* -
*
* The default, minimum, and maximum time to live (TTL) values that you want objects to stay in the CloudFront
* cache.
*
*
*
*
* The headers, cookies, and query strings that are included in the cache key are automatically included in requests
* that CloudFront sends to the origin. CloudFront sends a request when it can’t find an object in its cache that
* matches the request’s cache key. If you want to send values to the origin but not include them in the
* cache key, use CreateOriginRequestPolicy
.
*
*
* For more information about cache policies, see Controlling the cache key in the Amazon CloudFront Developer Guide.
*
*
* @param createCachePolicyRequest
* @return Result of the CreateCachePolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws CachePolicyAlreadyExistsException
* A cache policy with this name already exists. You must provide a unique name. To modify an existing cache
* policy, use UpdateCachePolicy
.
* @throws TooManyCachePoliciesException
* You have reached the maximum number of cache policies for this AWS account. For more information, see Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyHeadersInCachePolicyException
* The number of headers in the cache policy exceeds the maximum. For more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyCookiesInCachePolicyException
* The number of cookies in the cache policy exceeds the maximum. For more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyQueryStringsInCachePolicyException
* The number of query strings in the cache policy exceeds the maximum. For more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.CreateCachePolicy
* @see AWS
* API Documentation
*/
@Override
public CreateCachePolicyResult createCachePolicy(CreateCachePolicyRequest request) {
request = beforeClientExecution(request);
return executeCreateCachePolicy(request);
}
@SdkInternalApi
final CreateCachePolicyResult executeCreateCachePolicy(CreateCachePolicyRequest createCachePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createCachePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCachePolicyRequestMarshaller().marshall(super.beforeMarshalling(createCachePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCachePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateCachePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new origin access identity. If you're using Amazon S3 for your origin, you can use an origin access
* identity to require users to access your content using a CloudFront URL instead of the Amazon S3 URL. For more
* information about how to use origin access identities, see Serving Private
* Content through CloudFront in the Amazon CloudFront Developer Guide.
*
*
* @param createCloudFrontOriginAccessIdentityRequest
* The request to create a new origin access identity (OAI). An origin access identity is a special
* CloudFront user that you can associate with Amazon S3 origins, so that you can secure all or just some of
* your Amazon S3 content. For more information, see Restricting Access to Amazon S3 Content by Using an Origin Access Identity in the Amazon
* CloudFront Developer Guide.
* @return Result of the CreateCloudFrontOriginAccessIdentity operation returned by the service.
* @throws CloudFrontOriginAccessIdentityAlreadyExistsException
* If the CallerReference
is a value you already sent in a previous request to create an
* identity but the content of the CloudFrontOriginAccessIdentityConfig
is different from the
* original request, CloudFront returns a CloudFrontOriginAccessIdentityAlreadyExists
error.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws TooManyCloudFrontOriginAccessIdentitiesException
* Processing your request would cause you to exceed the maximum number of origin access identities allowed.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @sample AmazonCloudFront.CreateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
@Override
public CreateCloudFrontOriginAccessIdentityResult createCloudFrontOriginAccessIdentity(CreateCloudFrontOriginAccessIdentityRequest request) {
request = beforeClientExecution(request);
return executeCreateCloudFrontOriginAccessIdentity(request);
}
@SdkInternalApi
final CreateCloudFrontOriginAccessIdentityResult executeCreateCloudFrontOriginAccessIdentity(
CreateCloudFrontOriginAccessIdentityRequest createCloudFrontOriginAccessIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(createCloudFrontOriginAccessIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCloudFrontOriginAccessIdentityRequestMarshaller().marshall(super
.beforeMarshalling(createCloudFrontOriginAccessIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCloudFrontOriginAccessIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateCloudFrontOriginAccessIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new web distribution. You create a CloudFront distribution to tell CloudFront where you want content to
* be delivered from, and the details about how to track and manage content delivery. Send a POST
* request to the /CloudFront API version/distribution
/distribution ID
resource.
*
*
*
* When you update a distribution, there are more required fields than when you create a distribution. When you
* update your distribution by using UpdateDistribution, follow the steps included in the documentation to get the current configuration and then
* make your updates. This helps to make sure that you include all of the required fields. To view a summary, see Required Fields for Create Distribution and Update Distribution in the Amazon CloudFront Developer
* Guide.
*
*
*
* @param createDistributionRequest
* The request to create a new distribution.
* @return Result of the CreateDistribution operation returned by the service.
* @throws CNAMEAlreadyExistsException
* The CNAME specified is already defined for CloudFront.
* @throws DistributionAlreadyExistsException
* The caller reference you attempted to create the distribution with is associated with another
* distribution.
* @throws InvalidOriginException
* The Amazon S3 origin server specified does not refer to a valid Amazon S3 bucket.
* @throws InvalidOriginAccessIdentityException
* The origin access identity is not valid or doesn't exist.
* @throws AccessDeniedException
* Access denied.
* @throws TooManyTrustedSignersException
* Your request contains more trusted signers than are allowed per distribution.
* @throws TrustedSignerDoesNotExistException
* One or more of your trusted signers don't exist.
* @throws InvalidViewerCertificateException
* A viewer certificate specified is not valid.
* @throws InvalidMinimumProtocolVersionException
* The minimum protocol version specified is not valid.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws TooManyDistributionCNAMEsException
* Your request contains more CNAMEs than are allowed per distribution.
* @throws TooManyDistributionsException
* Processing your request would cause you to exceed the maximum number of distributions allowed.
* @throws InvalidDefaultRootObjectException
* The default root object file name is too big or contains an invalid character.
* @throws InvalidRelativePathException
* The relative path is too big, is not URL-encoded, or does not begin with a slash (/).
* @throws InvalidErrorCodeException
* An invalid error code was specified.
* @throws InvalidResponseCodeException
* A response code is not valid.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidRequiredProtocolException
* This operation requires the HTTPS protocol. Ensure that you specify the HTTPS protocol in your request,
* or omit the RequiredProtocols
element from your distribution configuration.
* @throws NoSuchOriginException
* No origin exists with the specified Origin Id
.
* @throws TooManyOriginsException
* You cannot create more origins for the distribution.
* @throws TooManyOriginGroupsPerDistributionException
* Processing your request would cause you to exceed the maximum number of origin groups allowed.
* @throws TooManyCacheBehaviorsException
* You cannot create more cache behaviors for the distribution.
* @throws TooManyCookieNamesInWhiteListException
* Your request contains more cookie names in the whitelist than are allowed per cache behavior.
* @throws InvalidForwardCookiesException
* Your request contains forward cookies option which doesn't match with the expectation for the
* whitelisted
list of cookie names. Either list of cookie names has been specified when not
* allowed or list of cookie names is missing when expected.
* @throws TooManyHeadersInForwardedValuesException
* Your request contains too many headers in forwarded values.
* @throws InvalidHeadersForS3OriginException
* The headers specified are not valid for an Amazon S3 origin.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws TooManyCertificatesException
* You cannot create anymore custom SSL/TLS certificates.
* @throws InvalidLocationCodeException
* The location code specified is not valid.
* @throws InvalidGeoRestrictionParameterException
* The specified geo restriction parameter is not valid.
* @throws InvalidProtocolSettingsException
* You cannot specify SSLv3 as the minimum protocol version if you only want to support only clients that
* support Server Name Indication (SNI).
* @throws InvalidTTLOrderException
* The TTL order specified is not valid.
* @throws InvalidWebACLIdException
* A web ACL ID specified is not valid. To specify a web ACL created using the latest version of AWS WAF,
* use the ACL ARN, for example
* arn:aws:wafv2:us-east-1:123456789012:global/webacl/ExampleWebACL/473e64fd-f30b-4765-81a0-62ad96dd167a
* . To specify a web ACL created using AWS WAF Classic, use the ACL ID, for example
* 473e64fd-f30b-4765-81a0-62ad96dd167a
.
* @throws TooManyOriginCustomHeadersException
* Your request contains too many origin custom headers.
* @throws TooManyQueryStringParametersException
* Your request contains too many query string parameters.
* @throws InvalidQueryStringParametersException
* The query string parameters specified are not valid.
* @throws TooManyDistributionsWithLambdaAssociationsException
* Processing your request would cause the maximum number of distributions with Lambda function associations
* per owner to be exceeded.
* @throws TooManyDistributionsWithSingleFunctionARNException
* The maximum number of distributions have been associated with the specified Lambda function.
* @throws TooManyLambdaFunctionAssociationsException
* Your request contains more Lambda function associations than are allowed per distribution.
* @throws InvalidLambdaFunctionAssociationException
* The specified Lambda function association is invalid.
* @throws InvalidOriginReadTimeoutException
* The read timeout specified for the origin is not valid.
* @throws InvalidOriginKeepaliveTimeoutException
* The keep alive timeout specified for the origin is not valid.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @throws IllegalFieldLevelEncryptionConfigAssociationWithCacheBehaviorException
* The specified configuration for field-level encryption can't be associated with the specified cache
* behavior.
* @throws TooManyDistributionsAssociatedToFieldLevelEncryptionConfigException
* The maximum number of distributions have been associated with the specified configuration for field-level
* encryption.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws TooManyDistributionsAssociatedToCachePolicyException
* The maximum number of distributions have been associated with the specified cache policy. For more
* information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws TooManyDistributionsAssociatedToOriginRequestPolicyException
* The maximum number of distributions have been associated with the specified origin request policy. For
* more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.CreateDistribution
* @see AWS
* API Documentation
*/
@Override
public CreateDistributionResult createDistribution(CreateDistributionRequest request) {
request = beforeClientExecution(request);
return executeCreateDistribution(request);
}
@SdkInternalApi
final CreateDistributionResult executeCreateDistribution(CreateDistributionRequest createDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(createDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDistributionRequestMarshaller().marshall(super.beforeMarshalling(createDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a new distribution with tags.
*
*
* @param createDistributionWithTagsRequest
* The request to create a new distribution with tags.
* @return Result of the CreateDistributionWithTags operation returned by the service.
* @throws CNAMEAlreadyExistsException
* The CNAME specified is already defined for CloudFront.
* @throws DistributionAlreadyExistsException
* The caller reference you attempted to create the distribution with is associated with another
* distribution.
* @throws InvalidOriginException
* The Amazon S3 origin server specified does not refer to a valid Amazon S3 bucket.
* @throws InvalidOriginAccessIdentityException
* The origin access identity is not valid or doesn't exist.
* @throws AccessDeniedException
* Access denied.
* @throws TooManyTrustedSignersException
* Your request contains more trusted signers than are allowed per distribution.
* @throws TrustedSignerDoesNotExistException
* One or more of your trusted signers don't exist.
* @throws InvalidViewerCertificateException
* A viewer certificate specified is not valid.
* @throws InvalidMinimumProtocolVersionException
* The minimum protocol version specified is not valid.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws TooManyDistributionCNAMEsException
* Your request contains more CNAMEs than are allowed per distribution.
* @throws TooManyDistributionsException
* Processing your request would cause you to exceed the maximum number of distributions allowed.
* @throws InvalidDefaultRootObjectException
* The default root object file name is too big or contains an invalid character.
* @throws InvalidRelativePathException
* The relative path is too big, is not URL-encoded, or does not begin with a slash (/).
* @throws InvalidErrorCodeException
* An invalid error code was specified.
* @throws InvalidResponseCodeException
* A response code is not valid.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidRequiredProtocolException
* This operation requires the HTTPS protocol. Ensure that you specify the HTTPS protocol in your request,
* or omit the RequiredProtocols
element from your distribution configuration.
* @throws NoSuchOriginException
* No origin exists with the specified Origin Id
.
* @throws TooManyOriginsException
* You cannot create more origins for the distribution.
* @throws TooManyOriginGroupsPerDistributionException
* Processing your request would cause you to exceed the maximum number of origin groups allowed.
* @throws TooManyCacheBehaviorsException
* You cannot create more cache behaviors for the distribution.
* @throws TooManyCookieNamesInWhiteListException
* Your request contains more cookie names in the whitelist than are allowed per cache behavior.
* @throws InvalidForwardCookiesException
* Your request contains forward cookies option which doesn't match with the expectation for the
* whitelisted
list of cookie names. Either list of cookie names has been specified when not
* allowed or list of cookie names is missing when expected.
* @throws TooManyHeadersInForwardedValuesException
* Your request contains too many headers in forwarded values.
* @throws InvalidHeadersForS3OriginException
* The headers specified are not valid for an Amazon S3 origin.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws TooManyCertificatesException
* You cannot create anymore custom SSL/TLS certificates.
* @throws InvalidLocationCodeException
* The location code specified is not valid.
* @throws InvalidGeoRestrictionParameterException
* The specified geo restriction parameter is not valid.
* @throws InvalidProtocolSettingsException
* You cannot specify SSLv3 as the minimum protocol version if you only want to support only clients that
* support Server Name Indication (SNI).
* @throws InvalidTTLOrderException
* The TTL order specified is not valid.
* @throws InvalidWebACLIdException
* A web ACL ID specified is not valid. To specify a web ACL created using the latest version of AWS WAF,
* use the ACL ARN, for example
* arn:aws:wafv2:us-east-1:123456789012:global/webacl/ExampleWebACL/473e64fd-f30b-4765-81a0-62ad96dd167a
* . To specify a web ACL created using AWS WAF Classic, use the ACL ID, for example
* 473e64fd-f30b-4765-81a0-62ad96dd167a
.
* @throws TooManyOriginCustomHeadersException
* Your request contains too many origin custom headers.
* @throws InvalidTaggingException
* The tagging specified is not valid.
* @throws TooManyQueryStringParametersException
* Your request contains too many query string parameters.
* @throws InvalidQueryStringParametersException
* The query string parameters specified are not valid.
* @throws TooManyDistributionsWithLambdaAssociationsException
* Processing your request would cause the maximum number of distributions with Lambda function associations
* per owner to be exceeded.
* @throws TooManyDistributionsWithSingleFunctionARNException
* The maximum number of distributions have been associated with the specified Lambda function.
* @throws TooManyLambdaFunctionAssociationsException
* Your request contains more Lambda function associations than are allowed per distribution.
* @throws InvalidLambdaFunctionAssociationException
* The specified Lambda function association is invalid.
* @throws InvalidOriginReadTimeoutException
* The read timeout specified for the origin is not valid.
* @throws InvalidOriginKeepaliveTimeoutException
* The keep alive timeout specified for the origin is not valid.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @throws IllegalFieldLevelEncryptionConfigAssociationWithCacheBehaviorException
* The specified configuration for field-level encryption can't be associated with the specified cache
* behavior.
* @throws TooManyDistributionsAssociatedToFieldLevelEncryptionConfigException
* The maximum number of distributions have been associated with the specified configuration for field-level
* encryption.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws TooManyDistributionsAssociatedToCachePolicyException
* The maximum number of distributions have been associated with the specified cache policy. For more
* information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws TooManyDistributionsAssociatedToOriginRequestPolicyException
* The maximum number of distributions have been associated with the specified origin request policy. For
* more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.CreateDistributionWithTags
* @see AWS API Documentation
*/
@Override
public CreateDistributionWithTagsResult createDistributionWithTags(CreateDistributionWithTagsRequest request) {
request = beforeClientExecution(request);
return executeCreateDistributionWithTags(request);
}
@SdkInternalApi
final CreateDistributionWithTagsResult executeCreateDistributionWithTags(CreateDistributionWithTagsRequest createDistributionWithTagsRequest) {
ExecutionContext executionContext = createExecutionContext(createDistributionWithTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDistributionWithTagsRequestMarshaller().marshall(super.beforeMarshalling(createDistributionWithTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDistributionWithTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateDistributionWithTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a new field-level encryption configuration.
*
*
* @param createFieldLevelEncryptionConfigRequest
* @return Result of the CreateFieldLevelEncryptionConfig operation returned by the service.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws NoSuchFieldLevelEncryptionProfileException
* The specified profile for field-level encryption doesn't exist.
* @throws FieldLevelEncryptionConfigAlreadyExistsException
* The specified configuration for field-level encryption already exists.
* @throws TooManyFieldLevelEncryptionConfigsException
* The maximum number of configurations for field-level encryption have been created.
* @throws TooManyFieldLevelEncryptionQueryArgProfilesException
* The maximum number of query arg profiles for field-level encryption have been created.
* @throws TooManyFieldLevelEncryptionContentTypeProfilesException
* The maximum number of content type profiles for field-level encryption have been created.
* @throws QueryArgProfileEmptyException
* No profile specified for the field-level encryption query argument.
* @sample AmazonCloudFront.CreateFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
@Override
public CreateFieldLevelEncryptionConfigResult createFieldLevelEncryptionConfig(CreateFieldLevelEncryptionConfigRequest request) {
request = beforeClientExecution(request);
return executeCreateFieldLevelEncryptionConfig(request);
}
@SdkInternalApi
final CreateFieldLevelEncryptionConfigResult executeCreateFieldLevelEncryptionConfig(
CreateFieldLevelEncryptionConfigRequest createFieldLevelEncryptionConfigRequest) {
ExecutionContext executionContext = createExecutionContext(createFieldLevelEncryptionConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFieldLevelEncryptionConfigRequestMarshaller().marshall(super.beforeMarshalling(createFieldLevelEncryptionConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFieldLevelEncryptionConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateFieldLevelEncryptionConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a field-level encryption profile.
*
*
* @param createFieldLevelEncryptionProfileRequest
* @return Result of the CreateFieldLevelEncryptionProfile operation returned by the service.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws NoSuchPublicKeyException
* The specified public key doesn't exist.
* @throws FieldLevelEncryptionProfileAlreadyExistsException
* The specified profile for field-level encryption already exists.
* @throws FieldLevelEncryptionProfileSizeExceededException
* The maximum size of a profile for field-level encryption was exceeded.
* @throws TooManyFieldLevelEncryptionProfilesException
* The maximum number of profiles for field-level encryption have been created.
* @throws TooManyFieldLevelEncryptionEncryptionEntitiesException
* The maximum number of encryption entities for field-level encryption have been created.
* @throws TooManyFieldLevelEncryptionFieldPatternsException
* The maximum number of field patterns for field-level encryption have been created.
* @sample AmazonCloudFront.CreateFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
@Override
public CreateFieldLevelEncryptionProfileResult createFieldLevelEncryptionProfile(CreateFieldLevelEncryptionProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateFieldLevelEncryptionProfile(request);
}
@SdkInternalApi
final CreateFieldLevelEncryptionProfileResult executeCreateFieldLevelEncryptionProfile(
CreateFieldLevelEncryptionProfileRequest createFieldLevelEncryptionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createFieldLevelEncryptionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFieldLevelEncryptionProfileRequestMarshaller().marshall(super.beforeMarshalling(createFieldLevelEncryptionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFieldLevelEncryptionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateFieldLevelEncryptionProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a new invalidation.
*
*
* @param createInvalidationRequest
* The request to create an invalidation.
* @return Result of the CreateInvalidation operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws BatchTooLargeException
* Invalidation batch specified is too large.
* @throws TooManyInvalidationsInProgressException
* You have exceeded the maximum number of allowable InProgress invalidation batch requests, or invalidation
* objects.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @sample AmazonCloudFront.CreateInvalidation
* @see AWS
* API Documentation
*/
@Override
public CreateInvalidationResult createInvalidation(CreateInvalidationRequest request) {
request = beforeClientExecution(request);
return executeCreateInvalidation(request);
}
@SdkInternalApi
final CreateInvalidationResult executeCreateInvalidation(CreateInvalidationRequest createInvalidationRequest) {
ExecutionContext executionContext = createExecutionContext(createInvalidationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateInvalidationRequestMarshaller().marshall(super.beforeMarshalling(createInvalidationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateInvalidation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateInvalidationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an origin request policy.
*
*
* After you create an origin request policy, you can attach it to one or more cache behaviors. When it’s attached
* to a cache behavior, the origin request policy determines the values that CloudFront includes in requests that it
* sends to the origin. Each request that CloudFront sends to the origin includes the following:
*
*
* -
*
* The request body and the URL path (without the domain name) from the viewer request.
*
*
* -
*
* The headers that CloudFront automatically includes in every origin request, including Host
,
* User-Agent
, and X-Amz-Cf-Id
.
*
*
* -
*
* All HTTP headers, cookies, and URL query strings that are specified in the cache policy or the origin request
* policy. These can include items from the viewer request and, in the case of headers, additional ones that are
* added by CloudFront.
*
*
*
*
* CloudFront sends a request when it can’t find a valid object in its cache that matches the request. If you want
* to send values to the origin and also include them in the cache key, use CreateCachePolicy
.
*
*
* For more information about origin request policies, see Controlling origin requests in the Amazon CloudFront Developer Guide.
*
*
* @param createOriginRequestPolicyRequest
* @return Result of the CreateOriginRequestPolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws OriginRequestPolicyAlreadyExistsException
* An origin request policy with this name already exists. You must provide a unique name. To modify an
* existing origin request policy, use UpdateOriginRequestPolicy
.
* @throws TooManyOriginRequestPoliciesException
* You have reached the maximum number of origin request policies for this AWS account. For more
* information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyHeadersInOriginRequestPolicyException
* The number of headers in the origin request policy exceeds the maximum. For more information, see Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyCookiesInOriginRequestPolicyException
* The number of cookies in the origin request policy exceeds the maximum. For more information, see Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyQueryStringsInOriginRequestPolicyException
* The number of query strings in the origin request policy exceeds the maximum. For more information, see
* Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.CreateOriginRequestPolicy
* @see AWS API Documentation
*/
@Override
public CreateOriginRequestPolicyResult createOriginRequestPolicy(CreateOriginRequestPolicyRequest request) {
request = beforeClientExecution(request);
return executeCreateOriginRequestPolicy(request);
}
@SdkInternalApi
final CreateOriginRequestPolicyResult executeCreateOriginRequestPolicy(CreateOriginRequestPolicyRequest createOriginRequestPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createOriginRequestPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateOriginRequestPolicyRequestMarshaller().marshall(super.beforeMarshalling(createOriginRequestPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateOriginRequestPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateOriginRequestPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Add a new public key to CloudFront to use, for example, for field-level encryption. You can add a maximum of 10
* public keys with one AWS account.
*
*
* @param createPublicKeyRequest
* @return Result of the CreatePublicKey operation returned by the service.
* @throws PublicKeyAlreadyExistsException
* The specified public key already exists.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws TooManyPublicKeysException
* The maximum number of public keys for field-level encryption have been created. To create a new public
* key, delete one of the existing keys.
* @sample AmazonCloudFront.CreatePublicKey
* @see AWS API
* Documentation
*/
@Override
public CreatePublicKeyResult createPublicKey(CreatePublicKeyRequest request) {
request = beforeClientExecution(request);
return executeCreatePublicKey(request);
}
@SdkInternalApi
final CreatePublicKeyResult executeCreatePublicKey(CreatePublicKeyRequest createPublicKeyRequest) {
ExecutionContext executionContext = createExecutionContext(createPublicKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePublicKeyRequestMarshaller().marshall(super.beforeMarshalling(createPublicKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePublicKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreatePublicKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new RTMP distribution. An RTMP distribution is similar to a web distribution, but an RTMP distribution
* streams media files using the Adobe Real-Time Messaging Protocol (RTMP) instead of serving files using HTTP.
*
*
* To create a new distribution, submit a POST
request to the CloudFront API
* version/distribution resource. The request body must include a document with a
* StreamingDistributionConfig element. The response echoes the StreamingDistributionConfig
* element and returns other information about the RTMP distribution.
*
*
* To get the status of your request, use the GET StreamingDistribution API action. When the value of
* Enabled
is true
and the value of Status
is Deployed
, your
* distribution is ready. A distribution usually deploys in less than 15 minutes.
*
*
* For more information about web distributions, see Working with
* RTMP Distributions in the Amazon CloudFront Developer Guide.
*
*
*
* Beginning with the 2012-05-05 version of the CloudFront API, we made substantial changes to the format of the XML
* document that you include in the request body when you create or update a web distribution or an RTMP
* distribution, and when you invalidate objects. With previous versions of the API, we discovered that it was too
* easy to accidentally delete one or more values for an element that accepts multiple values, for example, CNAMEs
* and trusted signers. Our changes for the 2012-05-05 release are intended to prevent these accidental deletions
* and to notify you when there's a mismatch between the number of values you say you're specifying in the
* Quantity
element and the number of values specified.
*
*
*
* @param createStreamingDistributionRequest
* The request to create a new streaming distribution.
* @return Result of the CreateStreamingDistribution operation returned by the service.
* @throws CNAMEAlreadyExistsException
* The CNAME specified is already defined for CloudFront.
* @throws StreamingDistributionAlreadyExistsException
* The caller reference you attempted to create the streaming distribution with is associated with another
* distribution
* @throws InvalidOriginException
* The Amazon S3 origin server specified does not refer to a valid Amazon S3 bucket.
* @throws InvalidOriginAccessIdentityException
* The origin access identity is not valid or doesn't exist.
* @throws AccessDeniedException
* Access denied.
* @throws TooManyTrustedSignersException
* Your request contains more trusted signers than are allowed per distribution.
* @throws TrustedSignerDoesNotExistException
* One or more of your trusted signers don't exist.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws TooManyStreamingDistributionCNAMEsException
* Your request contains more CNAMEs than are allowed per distribution.
* @throws TooManyStreamingDistributionsException
* Processing your request would cause you to exceed the maximum number of streaming distributions allowed.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @sample AmazonCloudFront.CreateStreamingDistribution
* @see AWS API Documentation
*/
@Override
public CreateStreamingDistributionResult createStreamingDistribution(CreateStreamingDistributionRequest request) {
request = beforeClientExecution(request);
return executeCreateStreamingDistribution(request);
}
@SdkInternalApi
final CreateStreamingDistributionResult executeCreateStreamingDistribution(CreateStreamingDistributionRequest createStreamingDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(createStreamingDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStreamingDistributionRequestMarshaller().marshall(super.beforeMarshalling(createStreamingDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStreamingDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateStreamingDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a new streaming distribution with tags.
*
*
* @param createStreamingDistributionWithTagsRequest
* The request to create a new streaming distribution with tags.
* @return Result of the CreateStreamingDistributionWithTags operation returned by the service.
* @throws CNAMEAlreadyExistsException
* The CNAME specified is already defined for CloudFront.
* @throws StreamingDistributionAlreadyExistsException
* The caller reference you attempted to create the streaming distribution with is associated with another
* distribution
* @throws InvalidOriginException
* The Amazon S3 origin server specified does not refer to a valid Amazon S3 bucket.
* @throws InvalidOriginAccessIdentityException
* The origin access identity is not valid or doesn't exist.
* @throws AccessDeniedException
* Access denied.
* @throws TooManyTrustedSignersException
* Your request contains more trusted signers than are allowed per distribution.
* @throws TrustedSignerDoesNotExistException
* One or more of your trusted signers don't exist.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws TooManyStreamingDistributionCNAMEsException
* Your request contains more CNAMEs than are allowed per distribution.
* @throws TooManyStreamingDistributionsException
* Processing your request would cause you to exceed the maximum number of streaming distributions allowed.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidTaggingException
* The tagging specified is not valid.
* @sample AmazonCloudFront.CreateStreamingDistributionWithTags
* @see AWS API Documentation
*/
@Override
public CreateStreamingDistributionWithTagsResult createStreamingDistributionWithTags(CreateStreamingDistributionWithTagsRequest request) {
request = beforeClientExecution(request);
return executeCreateStreamingDistributionWithTags(request);
}
@SdkInternalApi
final CreateStreamingDistributionWithTagsResult executeCreateStreamingDistributionWithTags(
CreateStreamingDistributionWithTagsRequest createStreamingDistributionWithTagsRequest) {
ExecutionContext executionContext = createExecutionContext(createStreamingDistributionWithTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStreamingDistributionWithTagsRequestMarshaller().marshall(super
.beforeMarshalling(createStreamingDistributionWithTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStreamingDistributionWithTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateStreamingDistributionWithTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a cache policy.
*
*
* You cannot delete a cache policy if it’s attached to a cache behavior. First update your distributions to remove
* the cache policy from all cache behaviors, then delete the cache policy.
*
*
* To delete a cache policy, you must provide the policy’s identifier and version. To get these values, you can use
* ListCachePolicies
or GetCachePolicy
.
*
*
* @param deleteCachePolicyRequest
* @return Result of the DeleteCachePolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws IllegalDeleteException
* You cannot delete a managed policy.
* @throws CachePolicyInUseException
* Cannot delete the cache policy because it is attached to one or more cache behaviors.
* @sample AmazonCloudFront.DeleteCachePolicy
* @see AWS
* API Documentation
*/
@Override
public DeleteCachePolicyResult deleteCachePolicy(DeleteCachePolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteCachePolicy(request);
}
@SdkInternalApi
final DeleteCachePolicyResult executeDeleteCachePolicy(DeleteCachePolicyRequest deleteCachePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCachePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCachePolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteCachePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCachePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteCachePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an origin access identity.
*
*
* @param deleteCloudFrontOriginAccessIdentityRequest
* Deletes a origin access identity.
* @return Result of the DeleteCloudFrontOriginAccessIdentity operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchCloudFrontOriginAccessIdentityException
* The specified origin access identity does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws CloudFrontOriginAccessIdentityInUseException
* The Origin Access Identity specified is already in use.
* @sample AmazonCloudFront.DeleteCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
@Override
public DeleteCloudFrontOriginAccessIdentityResult deleteCloudFrontOriginAccessIdentity(DeleteCloudFrontOriginAccessIdentityRequest request) {
request = beforeClientExecution(request);
return executeDeleteCloudFrontOriginAccessIdentity(request);
}
@SdkInternalApi
final DeleteCloudFrontOriginAccessIdentityResult executeDeleteCloudFrontOriginAccessIdentity(
DeleteCloudFrontOriginAccessIdentityRequest deleteCloudFrontOriginAccessIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCloudFrontOriginAccessIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCloudFrontOriginAccessIdentityRequestMarshaller().marshall(super
.beforeMarshalling(deleteCloudFrontOriginAccessIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCloudFrontOriginAccessIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteCloudFrontOriginAccessIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete a distribution.
*
*
* @param deleteDistributionRequest
* This action deletes a web distribution. To delete a web distribution using the CloudFront API, perform the
* following steps.
*
* To delete a web distribution using the CloudFront API:
*
*
* -
*
* Disable the web distribution
*
*
* -
*
* Submit a GET Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Distribution Config
request to update the configuration for your distribution.
* In the request body, include the XML document that you updated in Step 3. Set the value of the HTTP
* If-Match
header to the value of the ETag
header that CloudFront returned when
* you submitted the GET Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Distribution Config
request to confirm that the distribution
* was successfully disabled.
*
*
* -
*
* Submit a GET Distribution
request to confirm that your changes have propagated. When
* propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Distribution
request. Set the value of the HTTP If-Match
header
* to the value of the ETag
header that CloudFront returned when you submitted the
* GET Distribution Config
request in Step 6.
*
*
* -
*
* Review the response to your DELETE Distribution
request to confirm that the distribution was
* successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a Distribution in the Amazon CloudFront Developer Guide.
* @return Result of the DeleteDistribution operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws DistributionNotDisabledException
* The specified CloudFront distribution is not disabled. You must disable the distribution before you can
* delete it.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @sample AmazonCloudFront.DeleteDistribution
* @see AWS
* API Documentation
*/
@Override
public DeleteDistributionResult deleteDistribution(DeleteDistributionRequest request) {
request = beforeClientExecution(request);
return executeDeleteDistribution(request);
}
@SdkInternalApi
final DeleteDistributionResult executeDeleteDistribution(DeleteDistributionRequest deleteDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDistributionRequestMarshaller().marshall(super.beforeMarshalling(deleteDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove a field-level encryption configuration.
*
*
* @param deleteFieldLevelEncryptionConfigRequest
* @return Result of the DeleteFieldLevelEncryptionConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws FieldLevelEncryptionConfigInUseException
* The specified configuration for field-level encryption is in use.
* @sample AmazonCloudFront.DeleteFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
@Override
public DeleteFieldLevelEncryptionConfigResult deleteFieldLevelEncryptionConfig(DeleteFieldLevelEncryptionConfigRequest request) {
request = beforeClientExecution(request);
return executeDeleteFieldLevelEncryptionConfig(request);
}
@SdkInternalApi
final DeleteFieldLevelEncryptionConfigResult executeDeleteFieldLevelEncryptionConfig(
DeleteFieldLevelEncryptionConfigRequest deleteFieldLevelEncryptionConfigRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFieldLevelEncryptionConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFieldLevelEncryptionConfigRequestMarshaller().marshall(super.beforeMarshalling(deleteFieldLevelEncryptionConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFieldLevelEncryptionConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteFieldLevelEncryptionConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove a field-level encryption profile.
*
*
* @param deleteFieldLevelEncryptionProfileRequest
* @return Result of the DeleteFieldLevelEncryptionProfile operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchFieldLevelEncryptionProfileException
* The specified profile for field-level encryption doesn't exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws FieldLevelEncryptionProfileInUseException
* The specified profile for field-level encryption is in use.
* @sample AmazonCloudFront.DeleteFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
@Override
public DeleteFieldLevelEncryptionProfileResult deleteFieldLevelEncryptionProfile(DeleteFieldLevelEncryptionProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteFieldLevelEncryptionProfile(request);
}
@SdkInternalApi
final DeleteFieldLevelEncryptionProfileResult executeDeleteFieldLevelEncryptionProfile(
DeleteFieldLevelEncryptionProfileRequest deleteFieldLevelEncryptionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFieldLevelEncryptionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFieldLevelEncryptionProfileRequestMarshaller().marshall(super.beforeMarshalling(deleteFieldLevelEncryptionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFieldLevelEncryptionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteFieldLevelEncryptionProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an origin request policy.
*
*
* You cannot delete an origin request policy if it’s attached to any cache behaviors. First update your
* distributions to remove the origin request policy from all cache behaviors, then delete the origin request
* policy.
*
*
* To delete an origin request policy, you must provide the policy’s identifier and version. To get the identifier,
* you can use ListOriginRequestPolicies
or GetOriginRequestPolicy
.
*
*
* @param deleteOriginRequestPolicyRequest
* @return Result of the DeleteOriginRequestPolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws IllegalDeleteException
* You cannot delete a managed policy.
* @throws OriginRequestPolicyInUseException
* Cannot delete the origin request policy because it is attached to one or more cache behaviors.
* @sample AmazonCloudFront.DeleteOriginRequestPolicy
* @see AWS API Documentation
*/
@Override
public DeleteOriginRequestPolicyResult deleteOriginRequestPolicy(DeleteOriginRequestPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteOriginRequestPolicy(request);
}
@SdkInternalApi
final DeleteOriginRequestPolicyResult executeDeleteOriginRequestPolicy(DeleteOriginRequestPolicyRequest deleteOriginRequestPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteOriginRequestPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteOriginRequestPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteOriginRequestPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteOriginRequestPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteOriginRequestPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove a public key you previously added to CloudFront.
*
*
* @param deletePublicKeyRequest
* @return Result of the DeletePublicKey operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws PublicKeyInUseException
* The specified public key is in use.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchPublicKeyException
* The specified public key doesn't exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @sample AmazonCloudFront.DeletePublicKey
* @see AWS API
* Documentation
*/
@Override
public DeletePublicKeyResult deletePublicKey(DeletePublicKeyRequest request) {
request = beforeClientExecution(request);
return executeDeletePublicKey(request);
}
@SdkInternalApi
final DeletePublicKeyResult executeDeletePublicKey(DeletePublicKeyRequest deletePublicKeyRequest) {
ExecutionContext executionContext = createExecutionContext(deletePublicKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePublicKeyRequestMarshaller().marshall(super.beforeMarshalling(deletePublicKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePublicKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeletePublicKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete a streaming distribution. To delete an RTMP distribution using the CloudFront API, perform the following
* steps.
*
*
* To delete an RTMP distribution using the CloudFront API:
*
*
* -
*
* Disable the RTMP distribution.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Streaming Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Streaming Distribution Config
request to update the configuration for your
* distribution. In the request body, include the XML document that you updated in Step 3. Then set the value of the
* HTTP If-Match
header to the value of the ETag
header that CloudFront returned when you
* submitted the GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Streaming Distribution Config
request to confirm that the
* distribution was successfully disabled.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to confirm that your changes have propagated.
* When propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Streaming Distribution
request. Set the value of the HTTP If-Match
* header to the value of the ETag
header that CloudFront returned when you submitted the
* GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to your DELETE Streaming Distribution
request to confirm that the distribution
* was successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a
* Distribution in the Amazon CloudFront Developer Guide.
*
*
* @param deleteStreamingDistributionRequest
* The request to delete a streaming distribution.
* @return Result of the DeleteStreamingDistribution operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws StreamingDistributionNotDisabledException
* The specified CloudFront distribution is not disabled. You must disable the distribution before you can
* delete it.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchStreamingDistributionException
* The specified streaming distribution does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @sample AmazonCloudFront.DeleteStreamingDistribution
* @see AWS API Documentation
*/
@Override
public DeleteStreamingDistributionResult deleteStreamingDistribution(DeleteStreamingDistributionRequest request) {
request = beforeClientExecution(request);
return executeDeleteStreamingDistribution(request);
}
@SdkInternalApi
final DeleteStreamingDistributionResult executeDeleteStreamingDistribution(DeleteStreamingDistributionRequest deleteStreamingDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStreamingDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStreamingDistributionRequestMarshaller().marshall(super.beforeMarshalling(deleteStreamingDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStreamingDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteStreamingDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a cache policy, including the following metadata:
*
*
* -
*
* The policy’s identifier.
*
*
* -
*
* The date and time when the policy was last modified.
*
*
*
*
* To get a cache policy, you must provide the policy’s identifier. If the cache policy is attached to a
* distribution’s cache behavior, you can get the policy’s identifier using ListDistributions
or
* GetDistribution
. If the cache policy is not attached to a cache behavior, you can get the identifier
* using ListCachePolicies
.
*
*
* @param getCachePolicyRequest
* @return Result of the GetCachePolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @sample AmazonCloudFront.GetCachePolicy
* @see AWS API
* Documentation
*/
@Override
public GetCachePolicyResult getCachePolicy(GetCachePolicyRequest request) {
request = beforeClientExecution(request);
return executeGetCachePolicy(request);
}
@SdkInternalApi
final GetCachePolicyResult executeGetCachePolicy(GetCachePolicyRequest getCachePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getCachePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCachePolicyRequestMarshaller().marshall(super.beforeMarshalling(getCachePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCachePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetCachePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a cache policy configuration.
*
*
* To get a cache policy configuration, you must provide the policy’s identifier. If the cache policy is attached to
* a distribution’s cache behavior, you can get the policy’s identifier using ListDistributions
or
* GetDistribution
. If the cache policy is not attached to a cache behavior, you can get the identifier
* using ListCachePolicies
.
*
*
* @param getCachePolicyConfigRequest
* @return Result of the GetCachePolicyConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @sample AmazonCloudFront.GetCachePolicyConfig
* @see AWS API Documentation
*/
@Override
public GetCachePolicyConfigResult getCachePolicyConfig(GetCachePolicyConfigRequest request) {
request = beforeClientExecution(request);
return executeGetCachePolicyConfig(request);
}
@SdkInternalApi
final GetCachePolicyConfigResult executeGetCachePolicyConfig(GetCachePolicyConfigRequest getCachePolicyConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getCachePolicyConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCachePolicyConfigRequestMarshaller().marshall(super.beforeMarshalling(getCachePolicyConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCachePolicyConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetCachePolicyConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityRequest
* The request to get an origin access identity's information.
* @return Result of the GetCloudFrontOriginAccessIdentity operation returned by the service.
* @throws NoSuchCloudFrontOriginAccessIdentityException
* The specified origin access identity does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
@Override
public GetCloudFrontOriginAccessIdentityResult getCloudFrontOriginAccessIdentity(GetCloudFrontOriginAccessIdentityRequest request) {
request = beforeClientExecution(request);
return executeGetCloudFrontOriginAccessIdentity(request);
}
@SdkInternalApi
final GetCloudFrontOriginAccessIdentityResult executeGetCloudFrontOriginAccessIdentity(
GetCloudFrontOriginAccessIdentityRequest getCloudFrontOriginAccessIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(getCloudFrontOriginAccessIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCloudFrontOriginAccessIdentityRequestMarshaller().marshall(super.beforeMarshalling(getCloudFrontOriginAccessIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCloudFrontOriginAccessIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetCloudFrontOriginAccessIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the configuration information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityConfigRequest
* The origin access identity's configuration information. For more information, see CloudFrontOriginAccessIdentityConfig.
* @return Result of the GetCloudFrontOriginAccessIdentityConfig operation returned by the service.
* @throws NoSuchCloudFrontOriginAccessIdentityException
* The specified origin access identity does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetCloudFrontOriginAccessIdentityConfig
* @see AWS API Documentation
*/
@Override
public GetCloudFrontOriginAccessIdentityConfigResult getCloudFrontOriginAccessIdentityConfig(GetCloudFrontOriginAccessIdentityConfigRequest request) {
request = beforeClientExecution(request);
return executeGetCloudFrontOriginAccessIdentityConfig(request);
}
@SdkInternalApi
final GetCloudFrontOriginAccessIdentityConfigResult executeGetCloudFrontOriginAccessIdentityConfig(
GetCloudFrontOriginAccessIdentityConfigRequest getCloudFrontOriginAccessIdentityConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getCloudFrontOriginAccessIdentityConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCloudFrontOriginAccessIdentityConfigRequestMarshaller().marshall(super
.beforeMarshalling(getCloudFrontOriginAccessIdentityConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCloudFrontOriginAccessIdentityConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetCloudFrontOriginAccessIdentityConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the information about a distribution.
*
*
* @param getDistributionRequest
* The request to get a distribution's information.
* @return Result of the GetDistribution operation returned by the service.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetDistribution
* @see AWS API
* Documentation
*/
@Override
public GetDistributionResult getDistribution(GetDistributionRequest request) {
request = beforeClientExecution(request);
return executeGetDistribution(request);
}
@SdkInternalApi
final GetDistributionResult executeGetDistribution(GetDistributionRequest getDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(getDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDistributionRequestMarshaller().marshall(super.beforeMarshalling(getDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the configuration information about a distribution.
*
*
* @param getDistributionConfigRequest
* The request to get a distribution configuration.
* @return Result of the GetDistributionConfig operation returned by the service.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetDistributionConfig
* @see AWS API Documentation
*/
@Override
public GetDistributionConfigResult getDistributionConfig(GetDistributionConfigRequest request) {
request = beforeClientExecution(request);
return executeGetDistributionConfig(request);
}
@SdkInternalApi
final GetDistributionConfigResult executeGetDistributionConfig(GetDistributionConfigRequest getDistributionConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getDistributionConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDistributionConfigRequestMarshaller().marshall(super.beforeMarshalling(getDistributionConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDistributionConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetDistributionConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the field-level encryption configuration information.
*
*
* @param getFieldLevelEncryptionRequest
* @return Result of the GetFieldLevelEncryption operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @sample AmazonCloudFront.GetFieldLevelEncryption
* @see AWS API Documentation
*/
@Override
public GetFieldLevelEncryptionResult getFieldLevelEncryption(GetFieldLevelEncryptionRequest request) {
request = beforeClientExecution(request);
return executeGetFieldLevelEncryption(request);
}
@SdkInternalApi
final GetFieldLevelEncryptionResult executeGetFieldLevelEncryption(GetFieldLevelEncryptionRequest getFieldLevelEncryptionRequest) {
ExecutionContext executionContext = createExecutionContext(getFieldLevelEncryptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFieldLevelEncryptionRequestMarshaller().marshall(super.beforeMarshalling(getFieldLevelEncryptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFieldLevelEncryption");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetFieldLevelEncryptionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the field-level encryption configuration information.
*
*
* @param getFieldLevelEncryptionConfigRequest
* @return Result of the GetFieldLevelEncryptionConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @sample AmazonCloudFront.GetFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
@Override
public GetFieldLevelEncryptionConfigResult getFieldLevelEncryptionConfig(GetFieldLevelEncryptionConfigRequest request) {
request = beforeClientExecution(request);
return executeGetFieldLevelEncryptionConfig(request);
}
@SdkInternalApi
final GetFieldLevelEncryptionConfigResult executeGetFieldLevelEncryptionConfig(GetFieldLevelEncryptionConfigRequest getFieldLevelEncryptionConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getFieldLevelEncryptionConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFieldLevelEncryptionConfigRequestMarshaller().marshall(super.beforeMarshalling(getFieldLevelEncryptionConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFieldLevelEncryptionConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetFieldLevelEncryptionConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the field-level encryption profile information.
*
*
* @param getFieldLevelEncryptionProfileRequest
* @return Result of the GetFieldLevelEncryptionProfile operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchFieldLevelEncryptionProfileException
* The specified profile for field-level encryption doesn't exist.
* @sample AmazonCloudFront.GetFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
@Override
public GetFieldLevelEncryptionProfileResult getFieldLevelEncryptionProfile(GetFieldLevelEncryptionProfileRequest request) {
request = beforeClientExecution(request);
return executeGetFieldLevelEncryptionProfile(request);
}
@SdkInternalApi
final GetFieldLevelEncryptionProfileResult executeGetFieldLevelEncryptionProfile(GetFieldLevelEncryptionProfileRequest getFieldLevelEncryptionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getFieldLevelEncryptionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFieldLevelEncryptionProfileRequestMarshaller().marshall(super.beforeMarshalling(getFieldLevelEncryptionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFieldLevelEncryptionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetFieldLevelEncryptionProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the field-level encryption profile configuration information.
*
*
* @param getFieldLevelEncryptionProfileConfigRequest
* @return Result of the GetFieldLevelEncryptionProfileConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchFieldLevelEncryptionProfileException
* The specified profile for field-level encryption doesn't exist.
* @sample AmazonCloudFront.GetFieldLevelEncryptionProfileConfig
* @see AWS API Documentation
*/
@Override
public GetFieldLevelEncryptionProfileConfigResult getFieldLevelEncryptionProfileConfig(GetFieldLevelEncryptionProfileConfigRequest request) {
request = beforeClientExecution(request);
return executeGetFieldLevelEncryptionProfileConfig(request);
}
@SdkInternalApi
final GetFieldLevelEncryptionProfileConfigResult executeGetFieldLevelEncryptionProfileConfig(
GetFieldLevelEncryptionProfileConfigRequest getFieldLevelEncryptionProfileConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getFieldLevelEncryptionProfileConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFieldLevelEncryptionProfileConfigRequestMarshaller().marshall(super
.beforeMarshalling(getFieldLevelEncryptionProfileConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFieldLevelEncryptionProfileConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetFieldLevelEncryptionProfileConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the information about an invalidation.
*
*
* @param getInvalidationRequest
* The request to get an invalidation's information.
* @return Result of the GetInvalidation operation returned by the service.
* @throws NoSuchInvalidationException
* The specified invalidation does not exist.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetInvalidation
* @see AWS API
* Documentation
*/
@Override
public GetInvalidationResult getInvalidation(GetInvalidationRequest request) {
request = beforeClientExecution(request);
return executeGetInvalidation(request);
}
@SdkInternalApi
final GetInvalidationResult executeGetInvalidation(GetInvalidationRequest getInvalidationRequest) {
ExecutionContext executionContext = createExecutionContext(getInvalidationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetInvalidationRequestMarshaller().marshall(super.beforeMarshalling(getInvalidationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetInvalidation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetInvalidationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an origin request policy, including the following metadata:
*
*
* -
*
* The policy’s identifier.
*
*
* -
*
* The date and time when the policy was last modified.
*
*
*
*
* To get an origin request policy, you must provide the policy’s identifier. If the origin request policy is
* attached to a distribution’s cache behavior, you can get the policy’s identifier using
* ListDistributions
or GetDistribution
. If the origin request policy is not attached to a
* cache behavior, you can get the identifier using ListOriginRequestPolicies
.
*
*
* @param getOriginRequestPolicyRequest
* @return Result of the GetOriginRequestPolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @sample AmazonCloudFront.GetOriginRequestPolicy
* @see AWS API Documentation
*/
@Override
public GetOriginRequestPolicyResult getOriginRequestPolicy(GetOriginRequestPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetOriginRequestPolicy(request);
}
@SdkInternalApi
final GetOriginRequestPolicyResult executeGetOriginRequestPolicy(GetOriginRequestPolicyRequest getOriginRequestPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getOriginRequestPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetOriginRequestPolicyRequestMarshaller().marshall(super.beforeMarshalling(getOriginRequestPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetOriginRequestPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetOriginRequestPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an origin request policy configuration.
*
*
* To get an origin request policy configuration, you must provide the policy’s identifier. If the origin request
* policy is attached to a distribution’s cache behavior, you can get the policy’s identifier using
* ListDistributions
or GetDistribution
. If the origin request policy is not attached to a
* cache behavior, you can get the identifier using ListOriginRequestPolicies
.
*
*
* @param getOriginRequestPolicyConfigRequest
* @return Result of the GetOriginRequestPolicyConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @sample AmazonCloudFront.GetOriginRequestPolicyConfig
* @see AWS API Documentation
*/
@Override
public GetOriginRequestPolicyConfigResult getOriginRequestPolicyConfig(GetOriginRequestPolicyConfigRequest request) {
request = beforeClientExecution(request);
return executeGetOriginRequestPolicyConfig(request);
}
@SdkInternalApi
final GetOriginRequestPolicyConfigResult executeGetOriginRequestPolicyConfig(GetOriginRequestPolicyConfigRequest getOriginRequestPolicyConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getOriginRequestPolicyConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetOriginRequestPolicyConfigRequestMarshaller().marshall(super.beforeMarshalling(getOriginRequestPolicyConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetOriginRequestPolicyConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetOriginRequestPolicyConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the public key information.
*
*
* @param getPublicKeyRequest
* @return Result of the GetPublicKey operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchPublicKeyException
* The specified public key doesn't exist.
* @sample AmazonCloudFront.GetPublicKey
* @see AWS API
* Documentation
*/
@Override
public GetPublicKeyResult getPublicKey(GetPublicKeyRequest request) {
request = beforeClientExecution(request);
return executeGetPublicKey(request);
}
@SdkInternalApi
final GetPublicKeyResult executeGetPublicKey(GetPublicKeyRequest getPublicKeyRequest) {
ExecutionContext executionContext = createExecutionContext(getPublicKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPublicKeyRequestMarshaller().marshall(super.beforeMarshalling(getPublicKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPublicKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetPublicKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return public key configuration informaation
*
*
* @param getPublicKeyConfigRequest
* @return Result of the GetPublicKeyConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchPublicKeyException
* The specified public key doesn't exist.
* @sample AmazonCloudFront.GetPublicKeyConfig
* @see AWS
* API Documentation
*/
@Override
public GetPublicKeyConfigResult getPublicKeyConfig(GetPublicKeyConfigRequest request) {
request = beforeClientExecution(request);
return executeGetPublicKeyConfig(request);
}
@SdkInternalApi
final GetPublicKeyConfigResult executeGetPublicKeyConfig(GetPublicKeyConfigRequest getPublicKeyConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getPublicKeyConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPublicKeyConfigRequestMarshaller().marshall(super.beforeMarshalling(getPublicKeyConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPublicKeyConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetPublicKeyConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a specified RTMP distribution, including the distribution configuration.
*
*
* @param getStreamingDistributionRequest
* The request to get a streaming distribution's information.
* @return Result of the GetStreamingDistribution operation returned by the service.
* @throws NoSuchStreamingDistributionException
* The specified streaming distribution does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetStreamingDistribution
* @see AWS API Documentation
*/
@Override
public GetStreamingDistributionResult getStreamingDistribution(GetStreamingDistributionRequest request) {
request = beforeClientExecution(request);
return executeGetStreamingDistribution(request);
}
@SdkInternalApi
final GetStreamingDistributionResult executeGetStreamingDistribution(GetStreamingDistributionRequest getStreamingDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(getStreamingDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetStreamingDistributionRequestMarshaller().marshall(super.beforeMarshalling(getStreamingDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetStreamingDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetStreamingDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the configuration information about a streaming distribution.
*
*
* @param getStreamingDistributionConfigRequest
* To request to get a streaming distribution configuration.
* @return Result of the GetStreamingDistributionConfig operation returned by the service.
* @throws NoSuchStreamingDistributionException
* The specified streaming distribution does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.GetStreamingDistributionConfig
* @see AWS API Documentation
*/
@Override
public GetStreamingDistributionConfigResult getStreamingDistributionConfig(GetStreamingDistributionConfigRequest request) {
request = beforeClientExecution(request);
return executeGetStreamingDistributionConfig(request);
}
@SdkInternalApi
final GetStreamingDistributionConfigResult executeGetStreamingDistributionConfig(GetStreamingDistributionConfigRequest getStreamingDistributionConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getStreamingDistributionConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetStreamingDistributionConfigRequestMarshaller().marshall(super.beforeMarshalling(getStreamingDistributionConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetStreamingDistributionConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetStreamingDistributionConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of cache policies.
*
*
* You can optionally apply a filter to return only the managed policies created by AWS, or only the custom policies
* created in your AWS account.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listCachePoliciesRequest
* @return Result of the ListCachePolicies operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListCachePolicies
* @see AWS
* API Documentation
*/
@Override
public ListCachePoliciesResult listCachePolicies(ListCachePoliciesRequest request) {
request = beforeClientExecution(request);
return executeListCachePolicies(request);
}
@SdkInternalApi
final ListCachePoliciesResult executeListCachePolicies(ListCachePoliciesRequest listCachePoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listCachePoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCachePoliciesRequestMarshaller().marshall(super.beforeMarshalling(listCachePoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCachePolicies");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListCachePoliciesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists origin access identities.
*
*
* @param listCloudFrontOriginAccessIdentitiesRequest
* The request to list origin access identities.
* @return Result of the ListCloudFrontOriginAccessIdentities operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListCloudFrontOriginAccessIdentities
* @see AWS API Documentation
*/
@Override
public ListCloudFrontOriginAccessIdentitiesResult listCloudFrontOriginAccessIdentities(ListCloudFrontOriginAccessIdentitiesRequest request) {
request = beforeClientExecution(request);
return executeListCloudFrontOriginAccessIdentities(request);
}
@SdkInternalApi
final ListCloudFrontOriginAccessIdentitiesResult executeListCloudFrontOriginAccessIdentities(
ListCloudFrontOriginAccessIdentitiesRequest listCloudFrontOriginAccessIdentitiesRequest) {
ExecutionContext executionContext = createExecutionContext(listCloudFrontOriginAccessIdentitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCloudFrontOriginAccessIdentitiesRequestMarshaller().marshall(super
.beforeMarshalling(listCloudFrontOriginAccessIdentitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCloudFrontOriginAccessIdentities");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListCloudFrontOriginAccessIdentitiesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List CloudFront distributions.
*
*
* @param listDistributionsRequest
* The request to list your distributions.
* @return Result of the ListDistributions operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListDistributions
* @see AWS
* API Documentation
*/
@Override
public ListDistributionsResult listDistributions(ListDistributionsRequest request) {
request = beforeClientExecution(request);
return executeListDistributions(request);
}
@SdkInternalApi
final ListDistributionsResult executeListDistributions(ListDistributionsRequest listDistributionsRequest) {
ExecutionContext executionContext = createExecutionContext(listDistributionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDistributionsRequestMarshaller().marshall(super.beforeMarshalling(listDistributionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDistributions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListDistributionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that’s associated with the specified
* cache policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByCachePolicyIdRequest
* @return Result of the ListDistributionsByCachePolicyId operation returned by the service.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.ListDistributionsByCachePolicyId
* @see AWS API Documentation
*/
@Override
public ListDistributionsByCachePolicyIdResult listDistributionsByCachePolicyId(ListDistributionsByCachePolicyIdRequest request) {
request = beforeClientExecution(request);
return executeListDistributionsByCachePolicyId(request);
}
@SdkInternalApi
final ListDistributionsByCachePolicyIdResult executeListDistributionsByCachePolicyId(
ListDistributionsByCachePolicyIdRequest listDistributionsByCachePolicyIdRequest) {
ExecutionContext executionContext = createExecutionContext(listDistributionsByCachePolicyIdRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDistributionsByCachePolicyIdRequestMarshaller().marshall(super.beforeMarshalling(listDistributionsByCachePolicyIdRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDistributionsByCachePolicyId");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListDistributionsByCachePolicyIdResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that’s associated with the specified
* origin request policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByOriginRequestPolicyIdRequest
* @return Result of the ListDistributionsByOriginRequestPolicyId operation returned by the service.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.ListDistributionsByOriginRequestPolicyId
* @see AWS API Documentation
*/
@Override
public ListDistributionsByOriginRequestPolicyIdResult listDistributionsByOriginRequestPolicyId(ListDistributionsByOriginRequestPolicyIdRequest request) {
request = beforeClientExecution(request);
return executeListDistributionsByOriginRequestPolicyId(request);
}
@SdkInternalApi
final ListDistributionsByOriginRequestPolicyIdResult executeListDistributionsByOriginRequestPolicyId(
ListDistributionsByOriginRequestPolicyIdRequest listDistributionsByOriginRequestPolicyIdRequest) {
ExecutionContext executionContext = createExecutionContext(listDistributionsByOriginRequestPolicyIdRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDistributionsByOriginRequestPolicyIdRequestMarshaller().marshall(super
.beforeMarshalling(listDistributionsByOriginRequestPolicyIdRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDistributionsByOriginRequestPolicyId");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListDistributionsByOriginRequestPolicyIdResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List the distributions that are associated with a specified AWS WAF web ACL.
*
*
* @param listDistributionsByWebACLIdRequest
* The request to list distributions that are associated with a specified AWS WAF web ACL.
* @return Result of the ListDistributionsByWebACLId operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidWebACLIdException
* A web ACL ID specified is not valid. To specify a web ACL created using the latest version of AWS WAF,
* use the ACL ARN, for example
* arn:aws:wafv2:us-east-1:123456789012:global/webacl/ExampleWebACL/473e64fd-f30b-4765-81a0-62ad96dd167a
* . To specify a web ACL created using AWS WAF Classic, use the ACL ID, for example
* 473e64fd-f30b-4765-81a0-62ad96dd167a
.
* @sample AmazonCloudFront.ListDistributionsByWebACLId
* @see AWS API Documentation
*/
@Override
public ListDistributionsByWebACLIdResult listDistributionsByWebACLId(ListDistributionsByWebACLIdRequest request) {
request = beforeClientExecution(request);
return executeListDistributionsByWebACLId(request);
}
@SdkInternalApi
final ListDistributionsByWebACLIdResult executeListDistributionsByWebACLId(ListDistributionsByWebACLIdRequest listDistributionsByWebACLIdRequest) {
ExecutionContext executionContext = createExecutionContext(listDistributionsByWebACLIdRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDistributionsByWebACLIdRequestMarshaller().marshall(super.beforeMarshalling(listDistributionsByWebACLIdRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDistributionsByWebACLId");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListDistributionsByWebACLIdResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all field-level encryption configurations that have been created in CloudFront for this account.
*
*
* @param listFieldLevelEncryptionConfigsRequest
* @return Result of the ListFieldLevelEncryptionConfigs operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListFieldLevelEncryptionConfigs
* @see AWS API Documentation
*/
@Override
public ListFieldLevelEncryptionConfigsResult listFieldLevelEncryptionConfigs(ListFieldLevelEncryptionConfigsRequest request) {
request = beforeClientExecution(request);
return executeListFieldLevelEncryptionConfigs(request);
}
@SdkInternalApi
final ListFieldLevelEncryptionConfigsResult executeListFieldLevelEncryptionConfigs(
ListFieldLevelEncryptionConfigsRequest listFieldLevelEncryptionConfigsRequest) {
ExecutionContext executionContext = createExecutionContext(listFieldLevelEncryptionConfigsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFieldLevelEncryptionConfigsRequestMarshaller().marshall(super.beforeMarshalling(listFieldLevelEncryptionConfigsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFieldLevelEncryptionConfigs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListFieldLevelEncryptionConfigsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Request a list of field-level encryption profiles that have been created in CloudFront for this account.
*
*
* @param listFieldLevelEncryptionProfilesRequest
* @return Result of the ListFieldLevelEncryptionProfiles operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListFieldLevelEncryptionProfiles
* @see AWS API Documentation
*/
@Override
public ListFieldLevelEncryptionProfilesResult listFieldLevelEncryptionProfiles(ListFieldLevelEncryptionProfilesRequest request) {
request = beforeClientExecution(request);
return executeListFieldLevelEncryptionProfiles(request);
}
@SdkInternalApi
final ListFieldLevelEncryptionProfilesResult executeListFieldLevelEncryptionProfiles(
ListFieldLevelEncryptionProfilesRequest listFieldLevelEncryptionProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listFieldLevelEncryptionProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFieldLevelEncryptionProfilesRequestMarshaller().marshall(super.beforeMarshalling(listFieldLevelEncryptionProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFieldLevelEncryptionProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListFieldLevelEncryptionProfilesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists invalidation batches.
*
*
* @param listInvalidationsRequest
* The request to list invalidations.
* @return Result of the ListInvalidations operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws AccessDeniedException
* Access denied.
* @sample AmazonCloudFront.ListInvalidations
* @see AWS
* API Documentation
*/
@Override
public ListInvalidationsResult listInvalidations(ListInvalidationsRequest request) {
request = beforeClientExecution(request);
return executeListInvalidations(request);
}
@SdkInternalApi
final ListInvalidationsResult executeListInvalidations(ListInvalidationsRequest listInvalidationsRequest) {
ExecutionContext executionContext = createExecutionContext(listInvalidationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListInvalidationsRequestMarshaller().marshall(super.beforeMarshalling(listInvalidationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListInvalidations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListInvalidationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of origin request policies.
*
*
* You can optionally apply a filter to return only the managed policies created by AWS, or only the custom policies
* created in your AWS account.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listOriginRequestPoliciesRequest
* @return Result of the ListOriginRequestPolicies operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListOriginRequestPolicies
* @see AWS API Documentation
*/
@Override
public ListOriginRequestPoliciesResult listOriginRequestPolicies(ListOriginRequestPoliciesRequest request) {
request = beforeClientExecution(request);
return executeListOriginRequestPolicies(request);
}
@SdkInternalApi
final ListOriginRequestPoliciesResult executeListOriginRequestPolicies(ListOriginRequestPoliciesRequest listOriginRequestPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listOriginRequestPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListOriginRequestPoliciesRequestMarshaller().marshall(super.beforeMarshalling(listOriginRequestPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListOriginRequestPolicies");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListOriginRequestPoliciesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all public keys that have been added to CloudFront for this account.
*
*
* @param listPublicKeysRequest
* @return Result of the ListPublicKeys operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListPublicKeys
* @see AWS API
* Documentation
*/
@Override
public ListPublicKeysResult listPublicKeys(ListPublicKeysRequest request) {
request = beforeClientExecution(request);
return executeListPublicKeys(request);
}
@SdkInternalApi
final ListPublicKeysResult executeListPublicKeys(ListPublicKeysRequest listPublicKeysRequest) {
ExecutionContext executionContext = createExecutionContext(listPublicKeysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPublicKeysRequestMarshaller().marshall(super.beforeMarshalling(listPublicKeysRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPublicKeys");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListPublicKeysResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List streaming distributions.
*
*
* @param listStreamingDistributionsRequest
* The request to list your streaming distributions.
* @return Result of the ListStreamingDistributions operation returned by the service.
* @throws InvalidArgumentException
* An argument is invalid.
* @sample AmazonCloudFront.ListStreamingDistributions
* @see AWS API Documentation
*/
@Override
public ListStreamingDistributionsResult listStreamingDistributions(ListStreamingDistributionsRequest request) {
request = beforeClientExecution(request);
return executeListStreamingDistributions(request);
}
@SdkInternalApi
final ListStreamingDistributionsResult executeListStreamingDistributions(ListStreamingDistributionsRequest listStreamingDistributionsRequest) {
ExecutionContext executionContext = createExecutionContext(listStreamingDistributionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStreamingDistributionsRequestMarshaller().marshall(super.beforeMarshalling(listStreamingDistributionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStreamingDistributions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStreamingDistributionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List tags for a CloudFront resource.
*
*
* @param listTagsForResourceRequest
* The request to list tags for a CloudFront resource.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidTaggingException
* The tagging specified is not valid.
* @throws NoSuchResourceException
* A resource that was specified is not valid.
* @sample AmazonCloudFront.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestMarshaller().marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListTagsForResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Add tags to a CloudFront resource.
*
*
* @param tagResourceRequest
* The request to add tags to a CloudFront resource.
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidTaggingException
* The tagging specified is not valid.
* @throws NoSuchResourceException
* A resource that was specified is not valid.
* @sample AmazonCloudFront.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestMarshaller().marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new TagResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove tags from a CloudFront resource.
*
*
* @param untagResourceRequest
* The request to remove tags from a CloudFront resource.
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidTaggingException
* The tagging specified is not valid.
* @throws NoSuchResourceException
* A resource that was specified is not valid.
* @sample AmazonCloudFront.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestMarshaller().marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new UntagResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a cache policy configuration.
*
*
* When you update a cache policy configuration, all the fields are updated with the values provided in the request.
* You cannot update some fields independent of others. To update a cache policy configuration:
*
*
* -
*
* Use GetCachePolicyConfig
to get the current configuration.
*
*
* -
*
* Locally modify the fields in the cache policy configuration that you want to update.
*
*
* -
*
* Call UpdateCachePolicy
by providing the entire cache policy configuration, including the fields that
* you modified and those that you didn’t.
*
*
*
*
* @param updateCachePolicyRequest
* @return Result of the UpdateCachePolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws CachePolicyAlreadyExistsException
* A cache policy with this name already exists. You must provide a unique name. To modify an existing cache
* policy, use UpdateCachePolicy
.
* @throws TooManyHeadersInCachePolicyException
* The number of headers in the cache policy exceeds the maximum. For more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyCookiesInCachePolicyException
* The number of cookies in the cache policy exceeds the maximum. For more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyQueryStringsInCachePolicyException
* The number of query strings in the cache policy exceeds the maximum. For more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.UpdateCachePolicy
* @see AWS
* API Documentation
*/
@Override
public UpdateCachePolicyResult updateCachePolicy(UpdateCachePolicyRequest request) {
request = beforeClientExecution(request);
return executeUpdateCachePolicy(request);
}
@SdkInternalApi
final UpdateCachePolicyResult executeUpdateCachePolicy(UpdateCachePolicyRequest updateCachePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(updateCachePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateCachePolicyRequestMarshaller().marshall(super.beforeMarshalling(updateCachePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateCachePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateCachePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update an origin access identity.
*
*
* @param updateCloudFrontOriginAccessIdentityRequest
* The request to update an origin access identity.
* @return Result of the UpdateCloudFrontOriginAccessIdentity operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws NoSuchCloudFrontOriginAccessIdentityException
* The specified origin access identity does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @sample AmazonCloudFront.UpdateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
@Override
public UpdateCloudFrontOriginAccessIdentityResult updateCloudFrontOriginAccessIdentity(UpdateCloudFrontOriginAccessIdentityRequest request) {
request = beforeClientExecution(request);
return executeUpdateCloudFrontOriginAccessIdentity(request);
}
@SdkInternalApi
final UpdateCloudFrontOriginAccessIdentityResult executeUpdateCloudFrontOriginAccessIdentity(
UpdateCloudFrontOriginAccessIdentityRequest updateCloudFrontOriginAccessIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(updateCloudFrontOriginAccessIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateCloudFrontOriginAccessIdentityRequestMarshaller().marshall(super
.beforeMarshalling(updateCloudFrontOriginAccessIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateCloudFrontOriginAccessIdentity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateCloudFrontOriginAccessIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the configuration for a web distribution.
*
*
*
* When you update a distribution, there are more required fields than when you create a distribution. When you
* update your distribution by using this API action, follow the steps here to get the current configuration and
* then make your updates, to make sure that you include all of the required fields. To view a summary, see Required Fields for Create Distribution and Update Distribution in the Amazon CloudFront Developer
* Guide.
*
*
*
* The update process includes getting the current distribution configuration, updating the XML document that is
* returned to make your changes, and then submitting an UpdateDistribution
request to make the
* updates.
*
*
* For information about updating a distribution using the CloudFront console instead, see Creating a Distribution in the Amazon CloudFront Developer Guide.
*
*
* To update a web distribution using the CloudFront API
*
*
* -
*
* Submit a
* GetDistributionConfig request to get the current configuration and an Etag
header for the
* distribution.
*
*
*
* If you update the distribution again, you must get a new Etag
header.
*
*
* -
*
* Update the XML document that was returned in the response to your GetDistributionConfig
request to
* include your changes.
*
*
*
* When you edit the XML file, be aware of the following:
*
*
* -
*
* You must strip out the ETag parameter that is returned.
*
*
* -
*
* Additional fields are required when you update a distribution. There may be fields included in the XML file for
* features that you haven't configured for your distribution. This is expected and required to successfully update
* the distribution.
*
*
* -
*
* You can't change the value of CallerReference
. If you try to change this value, CloudFront returns
* an IllegalUpdate
error.
*
*
* -
*
* The new configuration replaces the existing configuration; the values that you specify in an
* UpdateDistribution
request are not merged into your existing configuration. When you add, delete, or
* replace values in an element that allows multiple values (for example, CNAME
), you must specify all
* of the values that you want to appear in the updated distribution. In addition, you must update the corresponding
* Quantity
element.
*
*
*
*
* -
*
* Submit an UpdateDistribution
request to update the configuration for your distribution:
*
*
* -
*
* In the request body, include the XML document that you updated in Step 2. The request body must include an XML
* document with a DistributionConfig
element.
*
*
* -
*
* Set the value of the HTTP If-Match
header to the value of the ETag
header that
* CloudFront returned when you submitted the GetDistributionConfig
request in Step 1.
*
*
*
*
* -
*
* Review the response to the UpdateDistribution
request to confirm that the configuration was
* successfully updated.
*
*
* -
*
* Optional: Submit a GetDistribution
* request to confirm that your changes have propagated. When propagation is complete, the value of
* Status
is Deployed
.
*
*
*
*
* @param updateDistributionRequest
* The request to update a distribution.
* @return Result of the UpdateDistribution operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws CNAMEAlreadyExistsException
* The CNAME specified is already defined for CloudFront.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws NoSuchDistributionException
* The specified distribution does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws TooManyDistributionCNAMEsException
* Your request contains more CNAMEs than are allowed per distribution.
* @throws InvalidDefaultRootObjectException
* The default root object file name is too big or contains an invalid character.
* @throws InvalidRelativePathException
* The relative path is too big, is not URL-encoded, or does not begin with a slash (/).
* @throws InvalidErrorCodeException
* An invalid error code was specified.
* @throws InvalidResponseCodeException
* A response code is not valid.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidOriginAccessIdentityException
* The origin access identity is not valid or doesn't exist.
* @throws TooManyTrustedSignersException
* Your request contains more trusted signers than are allowed per distribution.
* @throws TrustedSignerDoesNotExistException
* One or more of your trusted signers don't exist.
* @throws InvalidViewerCertificateException
* A viewer certificate specified is not valid.
* @throws InvalidMinimumProtocolVersionException
* The minimum protocol version specified is not valid.
* @throws InvalidRequiredProtocolException
* This operation requires the HTTPS protocol. Ensure that you specify the HTTPS protocol in your request,
* or omit the RequiredProtocols
element from your distribution configuration.
* @throws NoSuchOriginException
* No origin exists with the specified Origin Id
.
* @throws TooManyOriginsException
* You cannot create more origins for the distribution.
* @throws TooManyOriginGroupsPerDistributionException
* Processing your request would cause you to exceed the maximum number of origin groups allowed.
* @throws TooManyCacheBehaviorsException
* You cannot create more cache behaviors for the distribution.
* @throws TooManyCookieNamesInWhiteListException
* Your request contains more cookie names in the whitelist than are allowed per cache behavior.
* @throws InvalidForwardCookiesException
* Your request contains forward cookies option which doesn't match with the expectation for the
* whitelisted
list of cookie names. Either list of cookie names has been specified when not
* allowed or list of cookie names is missing when expected.
* @throws TooManyHeadersInForwardedValuesException
* Your request contains too many headers in forwarded values.
* @throws InvalidHeadersForS3OriginException
* The headers specified are not valid for an Amazon S3 origin.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws TooManyCertificatesException
* You cannot create anymore custom SSL/TLS certificates.
* @throws InvalidLocationCodeException
* The location code specified is not valid.
* @throws InvalidGeoRestrictionParameterException
* The specified geo restriction parameter is not valid.
* @throws InvalidTTLOrderException
* The TTL order specified is not valid.
* @throws InvalidWebACLIdException
* A web ACL ID specified is not valid. To specify a web ACL created using the latest version of AWS WAF,
* use the ACL ARN, for example
* arn:aws:wafv2:us-east-1:123456789012:global/webacl/ExampleWebACL/473e64fd-f30b-4765-81a0-62ad96dd167a
* . To specify a web ACL created using AWS WAF Classic, use the ACL ID, for example
* 473e64fd-f30b-4765-81a0-62ad96dd167a
.
* @throws TooManyOriginCustomHeadersException
* Your request contains too many origin custom headers.
* @throws TooManyQueryStringParametersException
* Your request contains too many query string parameters.
* @throws InvalidQueryStringParametersException
* The query string parameters specified are not valid.
* @throws TooManyDistributionsWithLambdaAssociationsException
* Processing your request would cause the maximum number of distributions with Lambda function associations
* per owner to be exceeded.
* @throws TooManyDistributionsWithSingleFunctionARNException
* The maximum number of distributions have been associated with the specified Lambda function.
* @throws TooManyLambdaFunctionAssociationsException
* Your request contains more Lambda function associations than are allowed per distribution.
* @throws InvalidLambdaFunctionAssociationException
* The specified Lambda function association is invalid.
* @throws InvalidOriginReadTimeoutException
* The read timeout specified for the origin is not valid.
* @throws InvalidOriginKeepaliveTimeoutException
* The keep alive timeout specified for the origin is not valid.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @throws IllegalFieldLevelEncryptionConfigAssociationWithCacheBehaviorException
* The specified configuration for field-level encryption can't be associated with the specified cache
* behavior.
* @throws TooManyDistributionsAssociatedToFieldLevelEncryptionConfigException
* The maximum number of distributions have been associated with the specified configuration for field-level
* encryption.
* @throws NoSuchCachePolicyException
* The cache policy does not exist.
* @throws TooManyDistributionsAssociatedToCachePolicyException
* The maximum number of distributions have been associated with the specified cache policy. For more
* information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws TooManyDistributionsAssociatedToOriginRequestPolicyException
* The maximum number of distributions have been associated with the specified origin request policy. For
* more information, see Quotas (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.UpdateDistribution
* @see AWS
* API Documentation
*/
@Override
public UpdateDistributionResult updateDistribution(UpdateDistributionRequest request) {
request = beforeClientExecution(request);
return executeUpdateDistribution(request);
}
@SdkInternalApi
final UpdateDistributionResult executeUpdateDistribution(UpdateDistributionRequest updateDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(updateDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDistributionRequestMarshaller().marshall(super.beforeMarshalling(updateDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update a field-level encryption configuration.
*
*
* @param updateFieldLevelEncryptionConfigRequest
* @return Result of the UpdateFieldLevelEncryptionConfig operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchFieldLevelEncryptionProfileException
* The specified profile for field-level encryption doesn't exist.
* @throws NoSuchFieldLevelEncryptionConfigException
* The specified configuration for field-level encryption doesn't exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws TooManyFieldLevelEncryptionQueryArgProfilesException
* The maximum number of query arg profiles for field-level encryption have been created.
* @throws TooManyFieldLevelEncryptionContentTypeProfilesException
* The maximum number of content type profiles for field-level encryption have been created.
* @throws QueryArgProfileEmptyException
* No profile specified for the field-level encryption query argument.
* @sample AmazonCloudFront.UpdateFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
@Override
public UpdateFieldLevelEncryptionConfigResult updateFieldLevelEncryptionConfig(UpdateFieldLevelEncryptionConfigRequest request) {
request = beforeClientExecution(request);
return executeUpdateFieldLevelEncryptionConfig(request);
}
@SdkInternalApi
final UpdateFieldLevelEncryptionConfigResult executeUpdateFieldLevelEncryptionConfig(
UpdateFieldLevelEncryptionConfigRequest updateFieldLevelEncryptionConfigRequest) {
ExecutionContext executionContext = createExecutionContext(updateFieldLevelEncryptionConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFieldLevelEncryptionConfigRequestMarshaller().marshall(super.beforeMarshalling(updateFieldLevelEncryptionConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFieldLevelEncryptionConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateFieldLevelEncryptionConfigResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update a field-level encryption profile.
*
*
* @param updateFieldLevelEncryptionProfileRequest
* @return Result of the UpdateFieldLevelEncryptionProfile operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws FieldLevelEncryptionProfileAlreadyExistsException
* The specified profile for field-level encryption already exists.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchPublicKeyException
* The specified public key doesn't exist.
* @throws NoSuchFieldLevelEncryptionProfileException
* The specified profile for field-level encryption doesn't exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws FieldLevelEncryptionProfileSizeExceededException
* The maximum size of a profile for field-level encryption was exceeded.
* @throws TooManyFieldLevelEncryptionEncryptionEntitiesException
* The maximum number of encryption entities for field-level encryption have been created.
* @throws TooManyFieldLevelEncryptionFieldPatternsException
* The maximum number of field patterns for field-level encryption have been created.
* @sample AmazonCloudFront.UpdateFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
@Override
public UpdateFieldLevelEncryptionProfileResult updateFieldLevelEncryptionProfile(UpdateFieldLevelEncryptionProfileRequest request) {
request = beforeClientExecution(request);
return executeUpdateFieldLevelEncryptionProfile(request);
}
@SdkInternalApi
final UpdateFieldLevelEncryptionProfileResult executeUpdateFieldLevelEncryptionProfile(
UpdateFieldLevelEncryptionProfileRequest updateFieldLevelEncryptionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(updateFieldLevelEncryptionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFieldLevelEncryptionProfileRequestMarshaller().marshall(super.beforeMarshalling(updateFieldLevelEncryptionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFieldLevelEncryptionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateFieldLevelEncryptionProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an origin request policy configuration.
*
*
* When you update an origin request policy configuration, all the fields are updated with the values provided in
* the request. You cannot update some fields independent of others. To update an origin request policy
* configuration:
*
*
* -
*
* Use GetOriginRequestPolicyConfig
to get the current configuration.
*
*
* -
*
* Locally modify the fields in the origin request policy configuration that you want to update.
*
*
* -
*
* Call UpdateOriginRequestPolicy
by providing the entire origin request policy configuration,
* including the fields that you modified and those that you didn’t.
*
*
*
*
* @param updateOriginRequestPolicyRequest
* @return Result of the UpdateOriginRequestPolicy operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws NoSuchOriginRequestPolicyException
* The origin request policy does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws OriginRequestPolicyAlreadyExistsException
* An origin request policy with this name already exists. You must provide a unique name. To modify an
* existing origin request policy, use UpdateOriginRequestPolicy
.
* @throws TooManyHeadersInOriginRequestPolicyException
* The number of headers in the origin request policy exceeds the maximum. For more information, see Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyCookiesInOriginRequestPolicyException
* The number of cookies in the origin request policy exceeds the maximum. For more information, see Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @throws TooManyQueryStringsInOriginRequestPolicyException
* The number of query strings in the origin request policy exceeds the maximum. For more information, see
* Quotas
* (formerly known as limits) in the Amazon CloudFront Developer Guide.
* @sample AmazonCloudFront.UpdateOriginRequestPolicy
* @see AWS API Documentation
*/
@Override
public UpdateOriginRequestPolicyResult updateOriginRequestPolicy(UpdateOriginRequestPolicyRequest request) {
request = beforeClientExecution(request);
return executeUpdateOriginRequestPolicy(request);
}
@SdkInternalApi
final UpdateOriginRequestPolicyResult executeUpdateOriginRequestPolicy(UpdateOriginRequestPolicyRequest updateOriginRequestPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(updateOriginRequestPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateOriginRequestPolicyRequestMarshaller().marshall(super.beforeMarshalling(updateOriginRequestPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateOriginRequestPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateOriginRequestPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update public key information. Note that the only value you can change is the comment.
*
*
* @param updatePublicKeyRequest
* @return Result of the UpdatePublicKey operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws CannotChangeImmutablePublicKeyFieldsException
* You can't change the value of a public key.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws NoSuchPublicKeyException
* The specified public key doesn't exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @sample AmazonCloudFront.UpdatePublicKey
* @see AWS API
* Documentation
*/
@Override
public UpdatePublicKeyResult updatePublicKey(UpdatePublicKeyRequest request) {
request = beforeClientExecution(request);
return executeUpdatePublicKey(request);
}
@SdkInternalApi
final UpdatePublicKeyResult executeUpdatePublicKey(UpdatePublicKeyRequest updatePublicKeyRequest) {
ExecutionContext executionContext = createExecutionContext(updatePublicKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePublicKeyRequestMarshaller().marshall(super.beforeMarshalling(updatePublicKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePublicKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdatePublicKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update a streaming distribution.
*
*
* @param updateStreamingDistributionRequest
* The request to update a streaming distribution.
* @return Result of the UpdateStreamingDistribution operation returned by the service.
* @throws AccessDeniedException
* Access denied.
* @throws CNAMEAlreadyExistsException
* The CNAME specified is already defined for CloudFront.
* @throws IllegalUpdateException
* The update contains modifications that are not allowed.
* @throws InvalidIfMatchVersionException
* The If-Match
version is missing or not valid.
* @throws MissingBodyException
* This operation requires a body. Ensure that the body is present and the Content-Type
header
* is set.
* @throws NoSuchStreamingDistributionException
* The specified streaming distribution does not exist.
* @throws PreconditionFailedException
* The precondition given in one or more of the request header fields evaluated to false
.
* @throws TooManyStreamingDistributionCNAMEsException
* Your request contains more CNAMEs than are allowed per distribution.
* @throws InvalidArgumentException
* An argument is invalid.
* @throws InvalidOriginAccessIdentityException
* The origin access identity is not valid or doesn't exist.
* @throws TooManyTrustedSignersException
* Your request contains more trusted signers than are allowed per distribution.
* @throws TrustedSignerDoesNotExistException
* One or more of your trusted signers don't exist.
* @throws InconsistentQuantitiesException
* The value of Quantity
and the size of Items
don't match.
* @sample AmazonCloudFront.UpdateStreamingDistribution
* @see AWS API Documentation
*/
@Override
public UpdateStreamingDistributionResult updateStreamingDistribution(UpdateStreamingDistributionRequest request) {
request = beforeClientExecution(request);
return executeUpdateStreamingDistribution(request);
}
@SdkInternalApi
final UpdateStreamingDistributionResult executeUpdateStreamingDistribution(UpdateStreamingDistributionRequest updateStreamingDistributionRequest) {
ExecutionContext executionContext = createExecutionContext(updateStreamingDistributionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStreamingDistributionRequestMarshaller().marshall(super.beforeMarshalling(updateStreamingDistributionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFront");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateStreamingDistribution");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateStreamingDistributionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request