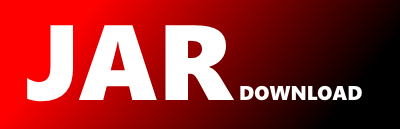
com.amazonaws.services.cloudfront.model.DefaultCacheBehavior Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A complex type that describes the default cache behavior if you don’t specify a CacheBehavior
element or
* if request URLs don’t match any of the values of PathPattern
in CacheBehavior
elements. You
* must create exactly one default cache behavior.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DefaultCacheBehavior implements Serializable, Cloneable {
/**
*
* The value of ID
for the origin that you want CloudFront to route requests to when they use the
* default cache behavior.
*
*/
private String targetOriginId;
/**
*
*
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or signed
* cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a CloudFront
* key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains information about
* which public key CloudFront should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*/
private TrustedSigners trustedSigners;
/**
*
* A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with a private key whose corresponding
* public key is in the key group. The signed URL or cookie contains information about which public key CloudFront
* should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*/
private TrustedKeyGroups trustedKeyGroups;
/**
*
* The protocol that viewers can use to access the files in the origin specified by TargetOriginId
when
* a request matches the path pattern in PathPattern
. You can specify the following options:
*
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status code of
* 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the request using the
* new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is never
* to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we recommend that
* you clear your objects’ cache because cached objects are protocol agnostic. That means that an edge location will
* return an object from the cache regardless of whether the current request protocol matches the protocol used
* previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
*
*/
private String viewerProtocolPolicy;
private AllowedMethods allowedMethods;
/**
*
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the origin
* that is associated with this cache behavior. If so, specify true
; if not, specify false
* . If you specify true
for SmoothStreaming
, you can still distribute other content using
* this cache behavior if the content matches the value of PathPattern
.
*
*/
private Boolean smoothStreaming;
/**
*
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so, specify
* true
; if not, specify false
. For more information, see Serving
* Compressed Files in the Amazon CloudFront Developer Guide.
*
*/
private Boolean compress;
/**
*
* A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
*
*/
private LambdaFunctionAssociations lambdaFunctionAssociations;
/**
*
* A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
*
*/
private FunctionAssociations functionAssociations;
/**
*
* The value of ID
for the field-level encryption configuration that you want CloudFront to use for
* encrypting specific fields of data for the default cache behavior.
*
*/
private String fieldLevelEncryptionId;
/**
*
* The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache behavior. For
* more information, see Real-time logs
* in the Amazon CloudFront Developer Guide.
*
*/
private String realtimeLogConfigArn;
/**
*
* The unique identifier of the cache policy that is attached to the default cache behavior. For more information,
* see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*/
private String cachePolicyId;
/**
*
* The unique identifier of the origin request policy that is attached to the default cache behavior. For more
* information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*/
private String originRequestPolicyId;
/**
*
* The identifier for a response headers policy.
*
*/
private String responseHeadersPolicyId;
/**
*
* This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of this
* field. For more information, see Working with
* policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request policy. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
*
*/
@Deprecated
private ForwardedValues forwardedValues;
/**
*
* This field is deprecated. We recommend that you use the MinTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all headers to
* your origin (under Headers
, if you specify 1
for Quantity
and
* *
for Name
).
*
*/
@Deprecated
private Long minTTL;
/**
*
* This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy instead
* of this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin does not add HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*/
@Deprecated
private Long defaultTTL;
/**
*
* This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*/
@Deprecated
private Long maxTTL;
/**
*
* The value of ID
for the origin that you want CloudFront to route requests to when they use the
* default cache behavior.
*
*
* @param targetOriginId
* The value of ID
for the origin that you want CloudFront to route requests to when they use
* the default cache behavior.
*/
public void setTargetOriginId(String targetOriginId) {
this.targetOriginId = targetOriginId;
}
/**
*
* The value of ID
for the origin that you want CloudFront to route requests to when they use the
* default cache behavior.
*
*
* @return The value of ID
for the origin that you want CloudFront to route requests to when they use
* the default cache behavior.
*/
public String getTargetOriginId() {
return this.targetOriginId;
}
/**
*
* The value of ID
for the origin that you want CloudFront to route requests to when they use the
* default cache behavior.
*
*
* @param targetOriginId
* The value of ID
for the origin that you want CloudFront to route requests to when they use
* the default cache behavior.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withTargetOriginId(String targetOriginId) {
setTargetOriginId(targetOriginId);
return this;
}
/**
*
*
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or signed
* cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a CloudFront
* key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains information about
* which public key CloudFront should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @param trustedSigners
*
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or
* signed cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a
* CloudFront key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains
* information about which public key CloudFront should use to verify the signature. For more information,
* see Serving
* private content in the Amazon CloudFront Developer Guide.
*/
public void setTrustedSigners(TrustedSigners trustedSigners) {
this.trustedSigners = trustedSigners;
}
/**
*
*
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or signed
* cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a CloudFront
* key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains information about
* which public key CloudFront should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @return
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or
* signed cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a
* CloudFront key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains
* information about which public key CloudFront should use to verify the signature. For more information,
* see Serving
* private content in the Amazon CloudFront Developer Guide.
*/
public TrustedSigners getTrustedSigners() {
return this.trustedSigners;
}
/**
*
*
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or signed
* cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a CloudFront
* key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains information about
* which public key CloudFront should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @param trustedSigners
*
* We recommend using TrustedKeyGroups
instead of TrustedSigners
.
*
*
*
* A list of Amazon Web Services account IDs whose public keys CloudFront can use to validate signed URLs or
* signed cookies.
*
*
* When a cache behavior contains trusted signers, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with the private key of a
* CloudFront key pair in a trusted signer’s Amazon Web Services account. The signed URL or cookie contains
* information about which public key CloudFront should use to verify the signature. For more information,
* see Serving
* private content in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withTrustedSigners(TrustedSigners trustedSigners) {
setTrustedSigners(trustedSigners);
return this;
}
/**
*
* A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with a private key whose corresponding
* public key is in the key group. The signed URL or cookie contains information about which public key CloudFront
* should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @param trustedKeyGroups
* A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for
* all requests that match the cache behavior. The URLs or cookies must be signed with a private key whose
* corresponding public key is in the key group. The signed URL or cookie contains information about which
* public key CloudFront should use to verify the signature. For more information, see Serving
* private content in the Amazon CloudFront Developer Guide.
*/
public void setTrustedKeyGroups(TrustedKeyGroups trustedKeyGroups) {
this.trustedKeyGroups = trustedKeyGroups;
}
/**
*
* A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with a private key whose corresponding
* public key is in the key group. The signed URL or cookie contains information about which public key CloudFront
* should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @return A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for
* all requests that match the cache behavior. The URLs or cookies must be signed with a private key whose
* corresponding public key is in the key group. The signed URL or cookie contains information about which
* public key CloudFront should use to verify the signature. For more information, see Serving
* private content in the Amazon CloudFront Developer Guide.
*/
public TrustedKeyGroups getTrustedKeyGroups() {
return this.trustedKeyGroups;
}
/**
*
* A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for all
* requests that match the cache behavior. The URLs or cookies must be signed with a private key whose corresponding
* public key is in the key group. The signed URL or cookie contains information about which public key CloudFront
* should use to verify the signature. For more information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @param trustedKeyGroups
* A list of key groups that CloudFront can use to validate signed URLs or signed cookies.
*
* When a cache behavior contains trusted key groups, CloudFront requires signed URLs or signed cookies for
* all requests that match the cache behavior. The URLs or cookies must be signed with a private key whose
* corresponding public key is in the key group. The signed URL or cookie contains information about which
* public key CloudFront should use to verify the signature. For more information, see Serving
* private content in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withTrustedKeyGroups(TrustedKeyGroups trustedKeyGroups) {
setTrustedKeyGroups(trustedKeyGroups);
return this;
}
/**
*
* The protocol that viewers can use to access the files in the origin specified by TargetOriginId
when
* a request matches the path pattern in PathPattern
. You can specify the following options:
*
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status code of
* 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the request using the
* new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is never
* to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we recommend that
* you clear your objects’ cache because cached objects are protocol agnostic. That means that an edge location will
* return an object from the cache regardless of whether the current request protocol matches the protocol used
* previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
*
*
* @param viewerProtocolPolicy
* The protocol that viewers can use to access the files in the origin specified by
* TargetOriginId
when a request matches the path pattern in PathPattern
. You can
* specify the following options:
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status
* code of 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the
* request using the new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is
* never to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we
* recommend that you clear your objects’ cache because cached objects are protocol agnostic. That means that
* an edge location will return an object from the cache regardless of whether the current request protocol
* matches the protocol used previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
* @see ViewerProtocolPolicy
*/
public void setViewerProtocolPolicy(String viewerProtocolPolicy) {
this.viewerProtocolPolicy = viewerProtocolPolicy;
}
/**
*
* The protocol that viewers can use to access the files in the origin specified by TargetOriginId
when
* a request matches the path pattern in PathPattern
. You can specify the following options:
*
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status code of
* 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the request using the
* new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is never
* to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we recommend that
* you clear your objects’ cache because cached objects are protocol agnostic. That means that an edge location will
* return an object from the cache regardless of whether the current request protocol matches the protocol used
* previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
*
*
* @return The protocol that viewers can use to access the files in the origin specified by
* TargetOriginId
when a request matches the path pattern in PathPattern
. You can
* specify the following options:
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status
* code of 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the
* request using the new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is
* never to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we
* recommend that you clear your objects’ cache because cached objects are protocol agnostic. That means
* that an edge location will return an object from the cache regardless of whether the current request
* protocol matches the protocol used previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
* @see ViewerProtocolPolicy
*/
public String getViewerProtocolPolicy() {
return this.viewerProtocolPolicy;
}
/**
*
* The protocol that viewers can use to access the files in the origin specified by TargetOriginId
when
* a request matches the path pattern in PathPattern
. You can specify the following options:
*
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status code of
* 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the request using the
* new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is never
* to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we recommend that
* you clear your objects’ cache because cached objects are protocol agnostic. That means that an edge location will
* return an object from the cache regardless of whether the current request protocol matches the protocol used
* previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
*
*
* @param viewerProtocolPolicy
* The protocol that viewers can use to access the files in the origin specified by
* TargetOriginId
when a request matches the path pattern in PathPattern
. You can
* specify the following options:
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status
* code of 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the
* request using the new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is
* never to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we
* recommend that you clear your objects’ cache because cached objects are protocol agnostic. That means that
* an edge location will return an object from the cache regardless of whether the current request protocol
* matches the protocol used previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ViewerProtocolPolicy
*/
public DefaultCacheBehavior withViewerProtocolPolicy(String viewerProtocolPolicy) {
setViewerProtocolPolicy(viewerProtocolPolicy);
return this;
}
/**
*
* The protocol that viewers can use to access the files in the origin specified by TargetOriginId
when
* a request matches the path pattern in PathPattern
. You can specify the following options:
*
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status code of
* 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the request using the
* new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is never
* to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we recommend that
* you clear your objects’ cache because cached objects are protocol agnostic. That means that an edge location will
* return an object from the cache regardless of whether the current request protocol matches the protocol used
* previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
*
*
* @param viewerProtocolPolicy
* The protocol that viewers can use to access the files in the origin specified by
* TargetOriginId
when a request matches the path pattern in PathPattern
. You can
* specify the following options:
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status
* code of 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the
* request using the new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is
* never to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we
* recommend that you clear your objects’ cache because cached objects are protocol agnostic. That means that
* an edge location will return an object from the cache regardless of whether the current request protocol
* matches the protocol used previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
* @see ViewerProtocolPolicy
*/
public void setViewerProtocolPolicy(ViewerProtocolPolicy viewerProtocolPolicy) {
withViewerProtocolPolicy(viewerProtocolPolicy);
}
/**
*
* The protocol that viewers can use to access the files in the origin specified by TargetOriginId
when
* a request matches the path pattern in PathPattern
. You can specify the following options:
*
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status code of
* 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the request using the
* new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is never
* to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we recommend that
* you clear your objects’ cache because cached objects are protocol agnostic. That means that an edge location will
* return an object from the cache regardless of whether the current request protocol matches the protocol used
* previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
*
*
* @param viewerProtocolPolicy
* The protocol that viewers can use to access the files in the origin specified by
* TargetOriginId
when a request matches the path pattern in PathPattern
. You can
* specify the following options:
*
* -
*
* allow-all
: Viewers can use HTTP or HTTPS.
*
*
* -
*
* redirect-to-https
: If a viewer submits an HTTP request, CloudFront returns an HTTP status
* code of 301 (Moved Permanently) to the viewer along with the HTTPS URL. The viewer then resubmits the
* request using the new URL.
*
*
* -
*
* https-only
: If a viewer sends an HTTP request, CloudFront returns an HTTP status code of 403
* (Forbidden).
*
*
*
*
* For more information about requiring the HTTPS protocol, see Requiring HTTPS Between Viewers and CloudFront in the Amazon CloudFront Developer Guide.
*
*
*
* The only way to guarantee that viewers retrieve an object that was fetched from the origin using HTTPS is
* never to use any other protocol to fetch the object. If you have recently changed from HTTP to HTTPS, we
* recommend that you clear your objects’ cache because cached objects are protocol agnostic. That means that
* an edge location will return an object from the cache regardless of whether the current request protocol
* matches the protocol used previously. For more information, see Managing Cache
* Expiration in the Amazon CloudFront Developer Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ViewerProtocolPolicy
*/
public DefaultCacheBehavior withViewerProtocolPolicy(ViewerProtocolPolicy viewerProtocolPolicy) {
this.viewerProtocolPolicy = viewerProtocolPolicy.toString();
return this;
}
/**
* @param allowedMethods
*/
public void setAllowedMethods(AllowedMethods allowedMethods) {
this.allowedMethods = allowedMethods;
}
/**
* @return
*/
public AllowedMethods getAllowedMethods() {
return this.allowedMethods;
}
/**
* @param allowedMethods
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withAllowedMethods(AllowedMethods allowedMethods) {
setAllowedMethods(allowedMethods);
return this;
}
/**
*
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the origin
* that is associated with this cache behavior. If so, specify true
; if not, specify false
* . If you specify true
for SmoothStreaming
, you can still distribute other content using
* this cache behavior if the content matches the value of PathPattern
.
*
*
* @param smoothStreaming
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the
* origin that is associated with this cache behavior. If so, specify true
; if not, specify
* false
. If you specify true
for SmoothStreaming
, you can still
* distribute other content using this cache behavior if the content matches the value of
* PathPattern
.
*/
public void setSmoothStreaming(Boolean smoothStreaming) {
this.smoothStreaming = smoothStreaming;
}
/**
*
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the origin
* that is associated with this cache behavior. If so, specify true
; if not, specify false
* . If you specify true
for SmoothStreaming
, you can still distribute other content using
* this cache behavior if the content matches the value of PathPattern
.
*
*
* @return Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the
* origin that is associated with this cache behavior. If so, specify true
; if not, specify
* false
. If you specify true
for SmoothStreaming
, you can still
* distribute other content using this cache behavior if the content matches the value of
* PathPattern
.
*/
public Boolean getSmoothStreaming() {
return this.smoothStreaming;
}
/**
*
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the origin
* that is associated with this cache behavior. If so, specify true
; if not, specify false
* . If you specify true
for SmoothStreaming
, you can still distribute other content using
* this cache behavior if the content matches the value of PathPattern
.
*
*
* @param smoothStreaming
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the
* origin that is associated with this cache behavior. If so, specify true
; if not, specify
* false
. If you specify true
for SmoothStreaming
, you can still
* distribute other content using this cache behavior if the content matches the value of
* PathPattern
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withSmoothStreaming(Boolean smoothStreaming) {
setSmoothStreaming(smoothStreaming);
return this;
}
/**
*
* Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the origin
* that is associated with this cache behavior. If so, specify true
; if not, specify false
* . If you specify true
for SmoothStreaming
, you can still distribute other content using
* this cache behavior if the content matches the value of PathPattern
.
*
*
* @return Indicates whether you want to distribute media files in the Microsoft Smooth Streaming format using the
* origin that is associated with this cache behavior. If so, specify true
; if not, specify
* false
. If you specify true
for SmoothStreaming
, you can still
* distribute other content using this cache behavior if the content matches the value of
* PathPattern
.
*/
public Boolean isSmoothStreaming() {
return this.smoothStreaming;
}
/**
*
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so, specify
* true
; if not, specify false
. For more information, see Serving
* Compressed Files in the Amazon CloudFront Developer Guide.
*
*
* @param compress
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so,
* specify true
; if not, specify false
. For more information, see Serving Compressed Files in the Amazon CloudFront Developer Guide.
*/
public void setCompress(Boolean compress) {
this.compress = compress;
}
/**
*
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so, specify
* true
; if not, specify false
. For more information, see Serving
* Compressed Files in the Amazon CloudFront Developer Guide.
*
*
* @return Whether you want CloudFront to automatically compress certain files for this cache behavior. If so,
* specify true
; if not, specify false
. For more information, see Serving Compressed Files in the Amazon CloudFront Developer Guide.
*/
public Boolean getCompress() {
return this.compress;
}
/**
*
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so, specify
* true
; if not, specify false
. For more information, see Serving
* Compressed Files in the Amazon CloudFront Developer Guide.
*
*
* @param compress
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so,
* specify true
; if not, specify false
. For more information, see Serving Compressed Files in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withCompress(Boolean compress) {
setCompress(compress);
return this;
}
/**
*
* Whether you want CloudFront to automatically compress certain files for this cache behavior. If so, specify
* true
; if not, specify false
. For more information, see Serving
* Compressed Files in the Amazon CloudFront Developer Guide.
*
*
* @return Whether you want CloudFront to automatically compress certain files for this cache behavior. If so,
* specify true
; if not, specify false
. For more information, see Serving Compressed Files in the Amazon CloudFront Developer Guide.
*/
public Boolean isCompress() {
return this.compress;
}
/**
*
* A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
*
*
* @param lambdaFunctionAssociations
* A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
*/
public void setLambdaFunctionAssociations(LambdaFunctionAssociations lambdaFunctionAssociations) {
this.lambdaFunctionAssociations = lambdaFunctionAssociations;
}
/**
*
* A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
*
*
* @return A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
*/
public LambdaFunctionAssociations getLambdaFunctionAssociations() {
return this.lambdaFunctionAssociations;
}
/**
*
* A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
*
*
* @param lambdaFunctionAssociations
* A complex type that contains zero or more Lambda@Edge function associations for a cache behavior.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withLambdaFunctionAssociations(LambdaFunctionAssociations lambdaFunctionAssociations) {
setLambdaFunctionAssociations(lambdaFunctionAssociations);
return this;
}
/**
*
* A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
*
*
* @param functionAssociations
* A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
*/
public void setFunctionAssociations(FunctionAssociations functionAssociations) {
this.functionAssociations = functionAssociations;
}
/**
*
* A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
*
*
* @return A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
*/
public FunctionAssociations getFunctionAssociations() {
return this.functionAssociations;
}
/**
*
* A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
*
*
* @param functionAssociations
* A list of CloudFront functions that are associated with this cache behavior. CloudFront functions must be
* published to the LIVE
stage to associate them with a cache behavior.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withFunctionAssociations(FunctionAssociations functionAssociations) {
setFunctionAssociations(functionAssociations);
return this;
}
/**
*
* The value of ID
for the field-level encryption configuration that you want CloudFront to use for
* encrypting specific fields of data for the default cache behavior.
*
*
* @param fieldLevelEncryptionId
* The value of ID
for the field-level encryption configuration that you want CloudFront to use
* for encrypting specific fields of data for the default cache behavior.
*/
public void setFieldLevelEncryptionId(String fieldLevelEncryptionId) {
this.fieldLevelEncryptionId = fieldLevelEncryptionId;
}
/**
*
* The value of ID
for the field-level encryption configuration that you want CloudFront to use for
* encrypting specific fields of data for the default cache behavior.
*
*
* @return The value of ID
for the field-level encryption configuration that you want CloudFront to use
* for encrypting specific fields of data for the default cache behavior.
*/
public String getFieldLevelEncryptionId() {
return this.fieldLevelEncryptionId;
}
/**
*
* The value of ID
for the field-level encryption configuration that you want CloudFront to use for
* encrypting specific fields of data for the default cache behavior.
*
*
* @param fieldLevelEncryptionId
* The value of ID
for the field-level encryption configuration that you want CloudFront to use
* for encrypting specific fields of data for the default cache behavior.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withFieldLevelEncryptionId(String fieldLevelEncryptionId) {
setFieldLevelEncryptionId(fieldLevelEncryptionId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache behavior. For
* more information, see Real-time logs
* in the Amazon CloudFront Developer Guide.
*
*
* @param realtimeLogConfigArn
* The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache behavior.
* For more information, see Real-time
* logs in the Amazon CloudFront Developer Guide.
*/
public void setRealtimeLogConfigArn(String realtimeLogConfigArn) {
this.realtimeLogConfigArn = realtimeLogConfigArn;
}
/**
*
* The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache behavior. For
* more information, see Real-time logs
* in the Amazon CloudFront Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache
* behavior. For more information, see Real-time
* logs in the Amazon CloudFront Developer Guide.
*/
public String getRealtimeLogConfigArn() {
return this.realtimeLogConfigArn;
}
/**
*
* The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache behavior. For
* more information, see Real-time logs
* in the Amazon CloudFront Developer Guide.
*
*
* @param realtimeLogConfigArn
* The Amazon Resource Name (ARN) of the real-time log configuration that is attached to this cache behavior.
* For more information, see Real-time
* logs in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withRealtimeLogConfigArn(String realtimeLogConfigArn) {
setRealtimeLogConfigArn(realtimeLogConfigArn);
return this;
}
/**
*
* The unique identifier of the cache policy that is attached to the default cache behavior. For more information,
* see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* @param cachePolicyId
* The unique identifier of the cache policy that is attached to the default cache behavior. For more
* information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*/
public void setCachePolicyId(String cachePolicyId) {
this.cachePolicyId = cachePolicyId;
}
/**
*
* The unique identifier of the cache policy that is attached to the default cache behavior. For more information,
* see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* @return The unique identifier of the cache policy that is attached to the default cache behavior. For more
* information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*/
public String getCachePolicyId() {
return this.cachePolicyId;
}
/**
*
* The unique identifier of the cache policy that is attached to the default cache behavior. For more information,
* see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* @param cachePolicyId
* The unique identifier of the cache policy that is attached to the default cache behavior. For more
* information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withCachePolicyId(String cachePolicyId) {
setCachePolicyId(cachePolicyId);
return this;
}
/**
*
* The unique identifier of the origin request policy that is attached to the default cache behavior. For more
* information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* @param originRequestPolicyId
* The unique identifier of the origin request policy that is attached to the default cache behavior. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*/
public void setOriginRequestPolicyId(String originRequestPolicyId) {
this.originRequestPolicyId = originRequestPolicyId;
}
/**
*
* The unique identifier of the origin request policy that is attached to the default cache behavior. For more
* information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* @return The unique identifier of the origin request policy that is attached to the default cache behavior. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*/
public String getOriginRequestPolicyId() {
return this.originRequestPolicyId;
}
/**
*
* The unique identifier of the origin request policy that is attached to the default cache behavior. For more
* information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* @param originRequestPolicyId
* The unique identifier of the origin request policy that is attached to the default cache behavior. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withOriginRequestPolicyId(String originRequestPolicyId) {
setOriginRequestPolicyId(originRequestPolicyId);
return this;
}
/**
*
* The identifier for a response headers policy.
*
*
* @param responseHeadersPolicyId
* The identifier for a response headers policy.
*/
public void setResponseHeadersPolicyId(String responseHeadersPolicyId) {
this.responseHeadersPolicyId = responseHeadersPolicyId;
}
/**
*
* The identifier for a response headers policy.
*
*
* @return The identifier for a response headers policy.
*/
public String getResponseHeadersPolicyId() {
return this.responseHeadersPolicyId;
}
/**
*
* The identifier for a response headers policy.
*
*
* @param responseHeadersPolicyId
* The identifier for a response headers policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DefaultCacheBehavior withResponseHeadersPolicyId(String responseHeadersPolicyId) {
setResponseHeadersPolicyId(responseHeadersPolicyId);
return this;
}
/**
*
* This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of this
* field. For more information, see Working with
* policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request policy. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
*
*
* @param forwardedValues
* This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of
* this field. For more information, see Working with policies in the Amazon CloudFront Developer Guide.
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request
* policy. For more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
*/
@Deprecated
public void setForwardedValues(ForwardedValues forwardedValues) {
this.forwardedValues = forwardedValues;
}
/**
*
* This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of this
* field. For more information, see Working with
* policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request policy. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
*
*
* @return This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of
* this field. For more information, see Working with policies in the Amazon CloudFront Developer Guide.
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request
* policy. For more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
*/
@Deprecated
public ForwardedValues getForwardedValues() {
return this.forwardedValues;
}
/**
*
* This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of this
* field. For more information, see Working with
* policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request policy. For
* more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
*
*
* @param forwardedValues
* This field is deprecated. We recommend that you use a cache policy or an origin request policy instead of
* this field. For more information, see Working with policies in the Amazon CloudFront Developer Guide.
*
* If you want to include values in the cache key, use a cache policy. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* If you want to send values to the origin but not include them in the cache key, use an origin request
* policy. For more information, see Creating origin request policies or Using the managed origin request policies in the Amazon CloudFront Developer Guide.
*
*
* A DefaultCacheBehavior
must include either a CachePolicyId
or
* ForwardedValues
. We recommend that you use a CachePolicyId
.
*
*
* A complex type that specifies how CloudFront handles query strings, cookies, and HTTP headers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public DefaultCacheBehavior withForwardedValues(ForwardedValues forwardedValues) {
setForwardedValues(forwardedValues);
return this;
}
/**
*
* This field is deprecated. We recommend that you use the MinTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all headers to
* your origin (under Headers
, if you specify 1
for Quantity
and
* *
for Name
).
*
*
* @param minTTL
* This field is deprecated. We recommend that you use the MinTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. For more information, see
* Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all headers
* to your origin (under Headers
, if you specify 1
for Quantity
and
* *
for Name
).
*/
@Deprecated
public void setMinTTL(Long minTTL) {
this.minTTL = minTTL;
}
/**
*
* This field is deprecated. We recommend that you use the MinTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all headers to
* your origin (under Headers
, if you specify 1
for Quantity
and
* *
for Name
).
*
*
* @return This field is deprecated. We recommend that you use the MinTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. For more information,
* see Managing
* How Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all
* headers to your origin (under Headers
, if you specify 1
for
* Quantity
and *
for Name
).
*/
@Deprecated
public Long getMinTTL() {
return this.minTTL;
}
/**
*
* This field is deprecated. We recommend that you use the MinTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all headers to
* your origin (under Headers
, if you specify 1
for Quantity
and
* *
for Name
).
*
*
* @param minTTL
* This field is deprecated. We recommend that you use the MinTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The minimum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. For more information, see
* Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* You must specify 0
for MinTTL
if you configure CloudFront to forward all headers
* to your origin (under Headers
, if you specify 1
for Quantity
and
* *
for Name
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public DefaultCacheBehavior withMinTTL(Long minTTL) {
setMinTTL(minTTL);
return this;
}
/**
*
* This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy instead
* of this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin does not add HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @param defaultTTL
* This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. The value that you
* specify applies only when your origin does not add HTTP headers such as Cache-Control max-age
, Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*/
@Deprecated
public void setDefaultTTL(Long defaultTTL) {
this.defaultTTL = defaultTTL;
}
/**
*
* This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy instead
* of this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin does not add HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @return This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. The value that you
* specify applies only when your origin does not add HTTP headers such as
* Cache-Control max-age
, Cache-Control s-maxage
, and Expires
to
* objects. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*/
@Deprecated
public Long getDefaultTTL() {
return this.defaultTTL;
}
/**
*
* This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy instead
* of this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin does not add HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @param defaultTTL
* This field is deprecated. We recommend that you use the DefaultTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The default amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. The value that you
* specify applies only when your origin does not add HTTP headers such as Cache-Control max-age
, Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public DefaultCacheBehavior withDefaultTTL(Long defaultTTL) {
setDefaultTTL(defaultTTL);
return this;
}
/**
*
* This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @param maxTTL
* This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. The value that you
* specify applies only when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*/
@Deprecated
public void setMaxTTL(Long maxTTL) {
this.maxTTL = maxTTL;
}
/**
*
* This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @return This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. The value that you
* specify applies only when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*/
@Deprecated
public Long getMaxTTL() {
return this.maxTTL;
}
/**
*
* This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy instead of
* this field. For more information, see Creating cache policies or Using
* the managed cache policies in the Amazon CloudFront Developer Guide.
*
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards another
* request to your origin to determine whether the object has been updated. The value that you specify applies only
* when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @param maxTTL
* This field is deprecated. We recommend that you use the MaxTTL
field in a cache policy
* instead of this field. For more information, see Creating cache policies or Using the managed cache policies in the Amazon CloudFront Developer Guide.
*
* The maximum amount of time that you want objects to stay in CloudFront caches before CloudFront forwards
* another request to your origin to determine whether the object has been updated. The value that you
* specify applies only when your origin adds HTTP headers such as Cache-Control max-age
,
* Cache-Control s-maxage
, and Expires
to objects. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public DefaultCacheBehavior withMaxTTL(Long maxTTL) {
setMaxTTL(maxTTL);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTargetOriginId() != null)
sb.append("TargetOriginId: ").append(getTargetOriginId()).append(",");
if (getTrustedSigners() != null)
sb.append("TrustedSigners: ").append(getTrustedSigners()).append(",");
if (getTrustedKeyGroups() != null)
sb.append("TrustedKeyGroups: ").append(getTrustedKeyGroups()).append(",");
if (getViewerProtocolPolicy() != null)
sb.append("ViewerProtocolPolicy: ").append(getViewerProtocolPolicy()).append(",");
if (getAllowedMethods() != null)
sb.append("AllowedMethods: ").append(getAllowedMethods()).append(",");
if (getSmoothStreaming() != null)
sb.append("SmoothStreaming: ").append(getSmoothStreaming()).append(",");
if (getCompress() != null)
sb.append("Compress: ").append(getCompress()).append(",");
if (getLambdaFunctionAssociations() != null)
sb.append("LambdaFunctionAssociations: ").append(getLambdaFunctionAssociations()).append(",");
if (getFunctionAssociations() != null)
sb.append("FunctionAssociations: ").append(getFunctionAssociations()).append(",");
if (getFieldLevelEncryptionId() != null)
sb.append("FieldLevelEncryptionId: ").append(getFieldLevelEncryptionId()).append(",");
if (getRealtimeLogConfigArn() != null)
sb.append("RealtimeLogConfigArn: ").append(getRealtimeLogConfigArn()).append(",");
if (getCachePolicyId() != null)
sb.append("CachePolicyId: ").append(getCachePolicyId()).append(",");
if (getOriginRequestPolicyId() != null)
sb.append("OriginRequestPolicyId: ").append(getOriginRequestPolicyId()).append(",");
if (getResponseHeadersPolicyId() != null)
sb.append("ResponseHeadersPolicyId: ").append(getResponseHeadersPolicyId()).append(",");
if (getForwardedValues() != null)
sb.append("ForwardedValues: ").append(getForwardedValues()).append(",");
if (getMinTTL() != null)
sb.append("MinTTL: ").append(getMinTTL()).append(",");
if (getDefaultTTL() != null)
sb.append("DefaultTTL: ").append(getDefaultTTL()).append(",");
if (getMaxTTL() != null)
sb.append("MaxTTL: ").append(getMaxTTL());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DefaultCacheBehavior == false)
return false;
DefaultCacheBehavior other = (DefaultCacheBehavior) obj;
if (other.getTargetOriginId() == null ^ this.getTargetOriginId() == null)
return false;
if (other.getTargetOriginId() != null && other.getTargetOriginId().equals(this.getTargetOriginId()) == false)
return false;
if (other.getTrustedSigners() == null ^ this.getTrustedSigners() == null)
return false;
if (other.getTrustedSigners() != null && other.getTrustedSigners().equals(this.getTrustedSigners()) == false)
return false;
if (other.getTrustedKeyGroups() == null ^ this.getTrustedKeyGroups() == null)
return false;
if (other.getTrustedKeyGroups() != null && other.getTrustedKeyGroups().equals(this.getTrustedKeyGroups()) == false)
return false;
if (other.getViewerProtocolPolicy() == null ^ this.getViewerProtocolPolicy() == null)
return false;
if (other.getViewerProtocolPolicy() != null && other.getViewerProtocolPolicy().equals(this.getViewerProtocolPolicy()) == false)
return false;
if (other.getAllowedMethods() == null ^ this.getAllowedMethods() == null)
return false;
if (other.getAllowedMethods() != null && other.getAllowedMethods().equals(this.getAllowedMethods()) == false)
return false;
if (other.getSmoothStreaming() == null ^ this.getSmoothStreaming() == null)
return false;
if (other.getSmoothStreaming() != null && other.getSmoothStreaming().equals(this.getSmoothStreaming()) == false)
return false;
if (other.getCompress() == null ^ this.getCompress() == null)
return false;
if (other.getCompress() != null && other.getCompress().equals(this.getCompress()) == false)
return false;
if (other.getLambdaFunctionAssociations() == null ^ this.getLambdaFunctionAssociations() == null)
return false;
if (other.getLambdaFunctionAssociations() != null && other.getLambdaFunctionAssociations().equals(this.getLambdaFunctionAssociations()) == false)
return false;
if (other.getFunctionAssociations() == null ^ this.getFunctionAssociations() == null)
return false;
if (other.getFunctionAssociations() != null && other.getFunctionAssociations().equals(this.getFunctionAssociations()) == false)
return false;
if (other.getFieldLevelEncryptionId() == null ^ this.getFieldLevelEncryptionId() == null)
return false;
if (other.getFieldLevelEncryptionId() != null && other.getFieldLevelEncryptionId().equals(this.getFieldLevelEncryptionId()) == false)
return false;
if (other.getRealtimeLogConfigArn() == null ^ this.getRealtimeLogConfigArn() == null)
return false;
if (other.getRealtimeLogConfigArn() != null && other.getRealtimeLogConfigArn().equals(this.getRealtimeLogConfigArn()) == false)
return false;
if (other.getCachePolicyId() == null ^ this.getCachePolicyId() == null)
return false;
if (other.getCachePolicyId() != null && other.getCachePolicyId().equals(this.getCachePolicyId()) == false)
return false;
if (other.getOriginRequestPolicyId() == null ^ this.getOriginRequestPolicyId() == null)
return false;
if (other.getOriginRequestPolicyId() != null && other.getOriginRequestPolicyId().equals(this.getOriginRequestPolicyId()) == false)
return false;
if (other.getResponseHeadersPolicyId() == null ^ this.getResponseHeadersPolicyId() == null)
return false;
if (other.getResponseHeadersPolicyId() != null && other.getResponseHeadersPolicyId().equals(this.getResponseHeadersPolicyId()) == false)
return false;
if (other.getForwardedValues() == null ^ this.getForwardedValues() == null)
return false;
if (other.getForwardedValues() != null && other.getForwardedValues().equals(this.getForwardedValues()) == false)
return false;
if (other.getMinTTL() == null ^ this.getMinTTL() == null)
return false;
if (other.getMinTTL() != null && other.getMinTTL().equals(this.getMinTTL()) == false)
return false;
if (other.getDefaultTTL() == null ^ this.getDefaultTTL() == null)
return false;
if (other.getDefaultTTL() != null && other.getDefaultTTL().equals(this.getDefaultTTL()) == false)
return false;
if (other.getMaxTTL() == null ^ this.getMaxTTL() == null)
return false;
if (other.getMaxTTL() != null && other.getMaxTTL().equals(this.getMaxTTL()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTargetOriginId() == null) ? 0 : getTargetOriginId().hashCode());
hashCode = prime * hashCode + ((getTrustedSigners() == null) ? 0 : getTrustedSigners().hashCode());
hashCode = prime * hashCode + ((getTrustedKeyGroups() == null) ? 0 : getTrustedKeyGroups().hashCode());
hashCode = prime * hashCode + ((getViewerProtocolPolicy() == null) ? 0 : getViewerProtocolPolicy().hashCode());
hashCode = prime * hashCode + ((getAllowedMethods() == null) ? 0 : getAllowedMethods().hashCode());
hashCode = prime * hashCode + ((getSmoothStreaming() == null) ? 0 : getSmoothStreaming().hashCode());
hashCode = prime * hashCode + ((getCompress() == null) ? 0 : getCompress().hashCode());
hashCode = prime * hashCode + ((getLambdaFunctionAssociations() == null) ? 0 : getLambdaFunctionAssociations().hashCode());
hashCode = prime * hashCode + ((getFunctionAssociations() == null) ? 0 : getFunctionAssociations().hashCode());
hashCode = prime * hashCode + ((getFieldLevelEncryptionId() == null) ? 0 : getFieldLevelEncryptionId().hashCode());
hashCode = prime * hashCode + ((getRealtimeLogConfigArn() == null) ? 0 : getRealtimeLogConfigArn().hashCode());
hashCode = prime * hashCode + ((getCachePolicyId() == null) ? 0 : getCachePolicyId().hashCode());
hashCode = prime * hashCode + ((getOriginRequestPolicyId() == null) ? 0 : getOriginRequestPolicyId().hashCode());
hashCode = prime * hashCode + ((getResponseHeadersPolicyId() == null) ? 0 : getResponseHeadersPolicyId().hashCode());
hashCode = prime * hashCode + ((getForwardedValues() == null) ? 0 : getForwardedValues().hashCode());
hashCode = prime * hashCode + ((getMinTTL() == null) ? 0 : getMinTTL().hashCode());
hashCode = prime * hashCode + ((getDefaultTTL() == null) ? 0 : getDefaultTTL().hashCode());
hashCode = prime * hashCode + ((getMaxTTL() == null) ? 0 : getMaxTTL().hashCode());
return hashCode;
}
@Override
public DefaultCacheBehavior clone() {
try {
return (DefaultCacheBehavior) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}