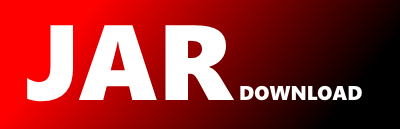
com.amazonaws.services.cloudfront.model.OriginAccessControlConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A CloudFront origin access control configuration.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OriginAccessControlConfig implements Serializable, Cloneable {
/**
*
* A name to identify the origin access control.
*
*/
private String name;
/**
*
* A description of the origin access control.
*
*/
private String description;
/**
*
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
*
*/
private String signingProtocol;
/**
*
* Specifies which requests CloudFront signs (adds authentication information to). Specify always
for
* the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
header
* from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access control for
* all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header, then
* CloudFront signs the origin request. If the viewer request contains the Authorization
header, then
* CloudFront doesn't sign the origin request and instead passes along the Authorization
header from
* the viewer request. WARNING: To pass along the Authorization
header from the viewer request, you
* must add the Authorization
header to a cache
* policy for all cache behaviors that use origins associated with this origin access control.
*
*
*
*/
private String signingBehavior;
/**
*
* The type of origin that this origin access control is for. The only valid value is s3
.
*
*/
private String originAccessControlOriginType;
/**
*
* A name to identify the origin access control.
*
*
* @param name
* A name to identify the origin access control.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A name to identify the origin access control.
*
*
* @return A name to identify the origin access control.
*/
public String getName() {
return this.name;
}
/**
*
* A name to identify the origin access control.
*
*
* @param name
* A name to identify the origin access control.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginAccessControlConfig withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the origin access control.
*
*
* @param description
* A description of the origin access control.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the origin access control.
*
*
* @return A description of the origin access control.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the origin access control.
*
*
* @param description
* A description of the origin access control.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginAccessControlConfig withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
*
*
* @param signingProtocol
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
* @see OriginAccessControlSigningProtocols
*/
public void setSigningProtocol(String signingProtocol) {
this.signingProtocol = signingProtocol;
}
/**
*
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
*
*
* @return The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
* @see OriginAccessControlSigningProtocols
*/
public String getSigningProtocol() {
return this.signingProtocol;
}
/**
*
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
*
*
* @param signingProtocol
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginAccessControlSigningProtocols
*/
public OriginAccessControlConfig withSigningProtocol(String signingProtocol) {
setSigningProtocol(signingProtocol);
return this;
}
/**
*
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
*
*
* @param signingProtocol
* The signing protocol of the origin access control, which determines how CloudFront signs (authenticates)
* requests. The only valid value is sigv4
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginAccessControlSigningProtocols
*/
public OriginAccessControlConfig withSigningProtocol(OriginAccessControlSigningProtocols signingProtocol) {
this.signingProtocol = signingProtocol.toString();
return this;
}
/**
*
* Specifies which requests CloudFront signs (adds authentication information to). Specify always
for
* the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
header
* from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access control for
* all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header, then
* CloudFront signs the origin request. If the viewer request contains the Authorization
header, then
* CloudFront doesn't sign the origin request and instead passes along the Authorization
header from
* the viewer request. WARNING: To pass along the Authorization
header from the viewer request, you
* must add the Authorization
header to a cache
* policy for all cache behaviors that use origins associated with this origin access control.
*
*
*
*
* @param signingBehavior
* Specifies which requests CloudFront signs (adds authentication information to). Specify
* always
for the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
* header from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access
* control for all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header,
* then CloudFront signs the origin request. If the viewer request contains the Authorization
* header, then CloudFront doesn't sign the origin request and instead passes along the
* Authorization
header from the viewer request. WARNING: To pass along the
* Authorization
header from the viewer request, you must add the
* Authorization
header to a cache policy for all cache behaviors that use origins associated with this origin access control.
*
*
* @see OriginAccessControlSigningBehaviors
*/
public void setSigningBehavior(String signingBehavior) {
this.signingBehavior = signingBehavior;
}
/**
*
* Specifies which requests CloudFront signs (adds authentication information to). Specify always
for
* the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
header
* from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access control for
* all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header, then
* CloudFront signs the origin request. If the viewer request contains the Authorization
header, then
* CloudFront doesn't sign the origin request and instead passes along the Authorization
header from
* the viewer request. WARNING: To pass along the Authorization
header from the viewer request, you
* must add the Authorization
header to a cache
* policy for all cache behaviors that use origins associated with this origin access control.
*
*
*
*
* @return Specifies which requests CloudFront signs (adds authentication information to). Specify
* always
for the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
* header from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access
* control for all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header,
* then CloudFront signs the origin request. If the viewer request contains the Authorization
* header, then CloudFront doesn't sign the origin request and instead passes along the
* Authorization
header from the viewer request. WARNING: To pass along the
* Authorization
header from the viewer request, you must add the
* Authorization
header to a cache policy for all cache behaviors that use origins associated with this origin access
* control.
*
*
* @see OriginAccessControlSigningBehaviors
*/
public String getSigningBehavior() {
return this.signingBehavior;
}
/**
*
* Specifies which requests CloudFront signs (adds authentication information to). Specify always
for
* the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
header
* from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access control for
* all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header, then
* CloudFront signs the origin request. If the viewer request contains the Authorization
header, then
* CloudFront doesn't sign the origin request and instead passes along the Authorization
header from
* the viewer request. WARNING: To pass along the Authorization
header from the viewer request, you
* must add the Authorization
header to a cache
* policy for all cache behaviors that use origins associated with this origin access control.
*
*
*
*
* @param signingBehavior
* Specifies which requests CloudFront signs (adds authentication information to). Specify
* always
for the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
* header from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access
* control for all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header,
* then CloudFront signs the origin request. If the viewer request contains the Authorization
* header, then CloudFront doesn't sign the origin request and instead passes along the
* Authorization
header from the viewer request. WARNING: To pass along the
* Authorization
header from the viewer request, you must add the
* Authorization
header to a cache policy for all cache behaviors that use origins associated with this origin access control.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginAccessControlSigningBehaviors
*/
public OriginAccessControlConfig withSigningBehavior(String signingBehavior) {
setSigningBehavior(signingBehavior);
return this;
}
/**
*
* Specifies which requests CloudFront signs (adds authentication information to). Specify always
for
* the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
header
* from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access control for
* all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header, then
* CloudFront signs the origin request. If the viewer request contains the Authorization
header, then
* CloudFront doesn't sign the origin request and instead passes along the Authorization
header from
* the viewer request. WARNING: To pass along the Authorization
header from the viewer request, you
* must add the Authorization
header to a cache
* policy for all cache behaviors that use origins associated with this origin access control.
*
*
*
*
* @param signingBehavior
* Specifies which requests CloudFront signs (adds authentication information to). Specify
* always
for the most common use case. For more information, see origin access control advanced settings in the Amazon CloudFront Developer Guide.
*
* This field can have one of the following values:
*
*
* -
*
* always
– CloudFront signs all origin requests, overwriting the Authorization
* header from the viewer request if one exists.
*
*
* -
*
* never
– CloudFront doesn't sign any origin requests. This value turns off origin access
* control for all origins in all distributions that use this origin access control.
*
*
* -
*
* no-override
– If the viewer request doesn't contain the Authorization
header,
* then CloudFront signs the origin request. If the viewer request contains the Authorization
* header, then CloudFront doesn't sign the origin request and instead passes along the
* Authorization
header from the viewer request. WARNING: To pass along the
* Authorization
header from the viewer request, you must add the
* Authorization
header to a cache policy for all cache behaviors that use origins associated with this origin access control.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginAccessControlSigningBehaviors
*/
public OriginAccessControlConfig withSigningBehavior(OriginAccessControlSigningBehaviors signingBehavior) {
this.signingBehavior = signingBehavior.toString();
return this;
}
/**
*
* The type of origin that this origin access control is for. The only valid value is s3
.
*
*
* @param originAccessControlOriginType
* The type of origin that this origin access control is for. The only valid value is s3
.
* @see OriginAccessControlOriginTypes
*/
public void setOriginAccessControlOriginType(String originAccessControlOriginType) {
this.originAccessControlOriginType = originAccessControlOriginType;
}
/**
*
* The type of origin that this origin access control is for. The only valid value is s3
.
*
*
* @return The type of origin that this origin access control is for. The only valid value is s3
.
* @see OriginAccessControlOriginTypes
*/
public String getOriginAccessControlOriginType() {
return this.originAccessControlOriginType;
}
/**
*
* The type of origin that this origin access control is for. The only valid value is s3
.
*
*
* @param originAccessControlOriginType
* The type of origin that this origin access control is for. The only valid value is s3
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginAccessControlOriginTypes
*/
public OriginAccessControlConfig withOriginAccessControlOriginType(String originAccessControlOriginType) {
setOriginAccessControlOriginType(originAccessControlOriginType);
return this;
}
/**
*
* The type of origin that this origin access control is for. The only valid value is s3
.
*
*
* @param originAccessControlOriginType
* The type of origin that this origin access control is for. The only valid value is s3
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginAccessControlOriginTypes
*/
public OriginAccessControlConfig withOriginAccessControlOriginType(OriginAccessControlOriginTypes originAccessControlOriginType) {
this.originAccessControlOriginType = originAccessControlOriginType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSigningProtocol() != null)
sb.append("SigningProtocol: ").append(getSigningProtocol()).append(",");
if (getSigningBehavior() != null)
sb.append("SigningBehavior: ").append(getSigningBehavior()).append(",");
if (getOriginAccessControlOriginType() != null)
sb.append("OriginAccessControlOriginType: ").append(getOriginAccessControlOriginType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OriginAccessControlConfig == false)
return false;
OriginAccessControlConfig other = (OriginAccessControlConfig) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSigningProtocol() == null ^ this.getSigningProtocol() == null)
return false;
if (other.getSigningProtocol() != null && other.getSigningProtocol().equals(this.getSigningProtocol()) == false)
return false;
if (other.getSigningBehavior() == null ^ this.getSigningBehavior() == null)
return false;
if (other.getSigningBehavior() != null && other.getSigningBehavior().equals(this.getSigningBehavior()) == false)
return false;
if (other.getOriginAccessControlOriginType() == null ^ this.getOriginAccessControlOriginType() == null)
return false;
if (other.getOriginAccessControlOriginType() != null
&& other.getOriginAccessControlOriginType().equals(this.getOriginAccessControlOriginType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSigningProtocol() == null) ? 0 : getSigningProtocol().hashCode());
hashCode = prime * hashCode + ((getSigningBehavior() == null) ? 0 : getSigningBehavior().hashCode());
hashCode = prime * hashCode + ((getOriginAccessControlOriginType() == null) ? 0 : getOriginAccessControlOriginType().hashCode());
return hashCode;
}
@Override
public OriginAccessControlConfig clone() {
try {
return (OriginAccessControlConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}