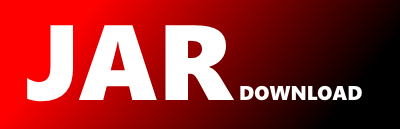
com.amazonaws.services.cloudfront.AmazonCloudFrontAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront;
import javax.annotation.Generated;
import com.amazonaws.services.cloudfront.model.*;
/**
* Interface for accessing CloudFront asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudfront.AbstractAmazonCloudFrontAsync} instead.
*
*
* Amazon CloudFront
*
* This is the Amazon CloudFront API Reference. This guide is for developers who need detailed information about
* CloudFront API actions, data types, and errors. For detailed information about CloudFront features, see the Amazon CloudFront
* Developer Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCloudFrontAsync extends AmazonCloudFront {
/**
*
* Associates an alias (also known as a CNAME or an alternate domain name) with a CloudFront distribution.
*
*
* With this operation you can move an alias that's already in use on a CloudFront distribution to a different
* distribution in one step. This prevents the downtime that could occur if you first remove the alias from one
* distribution and then separately add the alias to another distribution.
*
*
* To use this operation to associate an alias with a distribution, you provide the alias and the ID of the target
* distribution for the alias. For more information, including how to set up the target distribution, prerequisites
* that you must complete, and other restrictions, see Moving an alternate domain name to a different distribution in the Amazon CloudFront Developer Guide.
*
*
* @param associateAliasRequest
* @return A Java Future containing the result of the AssociateAlias operation returned by the service.
* @sample AmazonCloudFrontAsync.AssociateAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAliasAsync(AssociateAliasRequest associateAliasRequest);
/**
*
* Associates an alias (also known as a CNAME or an alternate domain name) with a CloudFront distribution.
*
*
* With this operation you can move an alias that's already in use on a CloudFront distribution to a different
* distribution in one step. This prevents the downtime that could occur if you first remove the alias from one
* distribution and then separately add the alias to another distribution.
*
*
* To use this operation to associate an alias with a distribution, you provide the alias and the ID of the target
* distribution for the alias. For more information, including how to set up the target distribution, prerequisites
* that you must complete, and other restrictions, see Moving an alternate domain name to a different distribution in the Amazon CloudFront Developer Guide.
*
*
* @param associateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAlias operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.AssociateAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAliasAsync(AssociateAliasRequest associateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a staging distribution using the configuration of the provided primary distribution. A staging
* distribution is a copy of an existing distribution (called the primary distribution) that you can use in a
* continuous deployment workflow.
*
*
* After you create a staging distribution, you can use UpdateDistribution
to modify the staging
* distribution's configuration. Then you can use CreateContinuousDeploymentPolicy
to incrementally
* move traffic to the staging distribution.
*
*
* This API operation requires the following IAM permissions:
*
*
* -
*
* GetDistribution
*
*
* -
*
*
* -
*
* CopyDistribution
*
*
*
*
* @param copyDistributionRequest
* @return A Java Future containing the result of the CopyDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.CopyDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyDistributionAsync(CopyDistributionRequest copyDistributionRequest);
/**
*
* Creates a staging distribution using the configuration of the provided primary distribution. A staging
* distribution is a copy of an existing distribution (called the primary distribution) that you can use in a
* continuous deployment workflow.
*
*
* After you create a staging distribution, you can use UpdateDistribution
to modify the staging
* distribution's configuration. Then you can use CreateContinuousDeploymentPolicy
to incrementally
* move traffic to the staging distribution.
*
*
* This API operation requires the following IAM permissions:
*
*
* -
*
* GetDistribution
*
*
* -
*
*
* -
*
* CopyDistribution
*
*
*
*
* @param copyDistributionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CopyDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyDistributionAsync(CopyDistributionRequest copyDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a cache policy.
*
*
* After you create a cache policy, you can attach it to one or more cache behaviors. When it's attached to a cache
* behavior, the cache policy determines the following:
*
*
* -
*
* The values that CloudFront includes in the cache key. These values can include HTTP headers, cookies, and
* URL query strings. CloudFront uses the cache key to find an object in its cache that it can return to the viewer.
*
*
* -
*
* The default, minimum, and maximum time to live (TTL) values that you want objects to stay in the CloudFront
* cache.
*
*
*
*
* The headers, cookies, and query strings that are included in the cache key are also included in requests that
* CloudFront sends to the origin. CloudFront sends a request when it can't find an object in its cache that matches
* the request's cache key. If you want to send values to the origin but not include them in the cache key,
* use OriginRequestPolicy
.
*
*
* For more information about cache policies, see Controlling the cache key in the Amazon CloudFront Developer Guide.
*
*
* @param createCachePolicyRequest
* @return A Java Future containing the result of the CreateCachePolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateCachePolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createCachePolicyAsync(CreateCachePolicyRequest createCachePolicyRequest);
/**
*
* Creates a cache policy.
*
*
* After you create a cache policy, you can attach it to one or more cache behaviors. When it's attached to a cache
* behavior, the cache policy determines the following:
*
*
* -
*
* The values that CloudFront includes in the cache key. These values can include HTTP headers, cookies, and
* URL query strings. CloudFront uses the cache key to find an object in its cache that it can return to the viewer.
*
*
* -
*
* The default, minimum, and maximum time to live (TTL) values that you want objects to stay in the CloudFront
* cache.
*
*
*
*
* The headers, cookies, and query strings that are included in the cache key are also included in requests that
* CloudFront sends to the origin. CloudFront sends a request when it can't find an object in its cache that matches
* the request's cache key. If you want to send values to the origin but not include them in the cache key,
* use OriginRequestPolicy
.
*
*
* For more information about cache policies, see Controlling the cache key in the Amazon CloudFront Developer Guide.
*
*
* @param createCachePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCachePolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateCachePolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createCachePolicyAsync(CreateCachePolicyRequest createCachePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new origin access identity. If you're using Amazon S3 for your origin, you can use an origin access
* identity to require users to access your content using a CloudFront URL instead of the Amazon S3 URL. For more
* information about how to use origin access identities, see Serving Private
* Content through CloudFront in the Amazon CloudFront Developer Guide.
*
*
* @param createCloudFrontOriginAccessIdentityRequest
* The request to create a new origin access identity (OAI). An origin access identity is a special
* CloudFront user that you can associate with Amazon S3 origins, so that you can secure all or just some of
* your Amazon S3 content. For more information, see Restricting Access to Amazon S3 Content by Using an Origin Access Identity in the Amazon
* CloudFront Developer Guide.
* @return A Java Future containing the result of the CreateCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFrontOriginAccessIdentityAsync(
CreateCloudFrontOriginAccessIdentityRequest createCloudFrontOriginAccessIdentityRequest);
/**
*
* Creates a new origin access identity. If you're using Amazon S3 for your origin, you can use an origin access
* identity to require users to access your content using a CloudFront URL instead of the Amazon S3 URL. For more
* information about how to use origin access identities, see Serving Private
* Content through CloudFront in the Amazon CloudFront Developer Guide.
*
*
* @param createCloudFrontOriginAccessIdentityRequest
* The request to create a new origin access identity (OAI). An origin access identity is a special
* CloudFront user that you can associate with Amazon S3 origins, so that you can secure all or just some of
* your Amazon S3 content. For more information, see Restricting Access to Amazon S3 Content by Using an Origin Access Identity in the Amazon
* CloudFront Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFrontOriginAccessIdentityAsync(
CreateCloudFrontOriginAccessIdentityRequest createCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a continuous deployment policy that distributes traffic for a custom domain name to two different
* CloudFront distributions.
*
*
* To use a continuous deployment policy, first use CopyDistribution
to create a staging distribution,
* then use UpdateDistribution
to modify the staging distribution's configuration.
*
*
* After you create and update a staging distribution, you can use a continuous deployment policy to incrementally
* move traffic to the staging distribution. This workflow enables you to test changes to a distribution's
* configuration before moving all of your domain's production traffic to the new configuration.
*
*
* @param createContinuousDeploymentPolicyRequest
* @return A Java Future containing the result of the CreateContinuousDeploymentPolicy operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateContinuousDeploymentPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createContinuousDeploymentPolicyAsync(
CreateContinuousDeploymentPolicyRequest createContinuousDeploymentPolicyRequest);
/**
*
* Creates a continuous deployment policy that distributes traffic for a custom domain name to two different
* CloudFront distributions.
*
*
* To use a continuous deployment policy, first use CopyDistribution
to create a staging distribution,
* then use UpdateDistribution
to modify the staging distribution's configuration.
*
*
* After you create and update a staging distribution, you can use a continuous deployment policy to incrementally
* move traffic to the staging distribution. This workflow enables you to test changes to a distribution's
* configuration before moving all of your domain's production traffic to the new configuration.
*
*
* @param createContinuousDeploymentPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateContinuousDeploymentPolicy operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateContinuousDeploymentPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createContinuousDeploymentPolicyAsync(
CreateContinuousDeploymentPolicyRequest createContinuousDeploymentPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a CloudFront distribution.
*
*
* @param createDistributionRequest
* The request to create a new distribution.
* @return A Java Future containing the result of the CreateDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDistributionAsync(CreateDistributionRequest createDistributionRequest);
/**
*
* Creates a CloudFront distribution.
*
*
* @param createDistributionRequest
* The request to create a new distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDistributionAsync(CreateDistributionRequest createDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new distribution with tags. This API operation requires the following IAM permissions:
*
*
* -
*
*
* -
*
* TagResource
*
*
*
*
* @param createDistributionWithTagsRequest
* The request to create a new distribution with tags.
* @return A Java Future containing the result of the CreateDistributionWithTags operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createDistributionWithTagsAsync(
CreateDistributionWithTagsRequest createDistributionWithTagsRequest);
/**
*
* Create a new distribution with tags. This API operation requires the following IAM permissions:
*
*
* -
*
*
* -
*
* TagResource
*
*
*
*
* @param createDistributionWithTagsRequest
* The request to create a new distribution with tags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDistributionWithTags operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createDistributionWithTagsAsync(
CreateDistributionWithTagsRequest createDistributionWithTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new field-level encryption configuration.
*
*
* @param createFieldLevelEncryptionConfigRequest
* @return A Java Future containing the result of the CreateFieldLevelEncryptionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createFieldLevelEncryptionConfigAsync(
CreateFieldLevelEncryptionConfigRequest createFieldLevelEncryptionConfigRequest);
/**
*
* Create a new field-level encryption configuration.
*
*
* @param createFieldLevelEncryptionConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFieldLevelEncryptionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createFieldLevelEncryptionConfigAsync(
CreateFieldLevelEncryptionConfigRequest createFieldLevelEncryptionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a field-level encryption profile.
*
*
* @param createFieldLevelEncryptionProfileRequest
* @return A Java Future containing the result of the CreateFieldLevelEncryptionProfile operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createFieldLevelEncryptionProfileAsync(
CreateFieldLevelEncryptionProfileRequest createFieldLevelEncryptionProfileRequest);
/**
*
* Create a field-level encryption profile.
*
*
* @param createFieldLevelEncryptionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFieldLevelEncryptionProfile operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createFieldLevelEncryptionProfileAsync(
CreateFieldLevelEncryptionProfileRequest createFieldLevelEncryptionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a CloudFront function.
*
*
* To create a function, you provide the function code and some configuration information about the function. The
* response contains an Amazon Resource Name (ARN) that uniquely identifies the function.
*
*
* When you create a function, it's in the DEVELOPMENT
stage. In this stage, you can test the function
* with TestFunction
, and update it with UpdateFunction
.
*
*
* When you're ready to use your function with a CloudFront distribution, use PublishFunction
to copy
* the function from the DEVELOPMENT
stage to LIVE
. When it's live, you can attach the
* function to a distribution's cache behavior, using the function's ARN.
*
*
* @param createFunctionRequest
* @return A Java Future containing the result of the CreateFunction operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFunctionAsync(CreateFunctionRequest createFunctionRequest);
/**
*
* Creates a CloudFront function.
*
*
* To create a function, you provide the function code and some configuration information about the function. The
* response contains an Amazon Resource Name (ARN) that uniquely identifies the function.
*
*
* When you create a function, it's in the DEVELOPMENT
stage. In this stage, you can test the function
* with TestFunction
, and update it with UpdateFunction
.
*
*
* When you're ready to use your function with a CloudFront distribution, use PublishFunction
to copy
* the function from the DEVELOPMENT
stage to LIVE
. When it's live, you can attach the
* function to a distribution's cache behavior, using the function's ARN.
*
*
* @param createFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFunction operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFunctionAsync(CreateFunctionRequest createFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new invalidation. For more information, see Invalidating
* files in the Amazon CloudFront Developer Guide.
*
*
* @param createInvalidationRequest
* The request to create an invalidation.
* @return A Java Future containing the result of the CreateInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateInvalidation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInvalidationAsync(CreateInvalidationRequest createInvalidationRequest);
/**
*
* Create a new invalidation. For more information, see Invalidating
* files in the Amazon CloudFront Developer Guide.
*
*
* @param createInvalidationRequest
* The request to create an invalidation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateInvalidation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInvalidationAsync(CreateInvalidationRequest createInvalidationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a key group that you can use with CloudFront signed
* URLs and signed cookies.
*
*
* To create a key group, you must specify at least one public key for the key group. After you create a key group,
* you can reference it from one or more cache behaviors. When you reference a key group in a cache behavior,
* CloudFront requires signed URLs or signed cookies for all requests that match the cache behavior. The URLs or
* cookies must be signed with a private key whose corresponding public key is in the key group. The signed URL or
* cookie contains information about which public key CloudFront should use to verify the signature. For more
* information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @param createKeyGroupRequest
* @return A Java Future containing the result of the CreateKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateKeyGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyGroupAsync(CreateKeyGroupRequest createKeyGroupRequest);
/**
*
* Creates a key group that you can use with CloudFront signed
* URLs and signed cookies.
*
*
* To create a key group, you must specify at least one public key for the key group. After you create a key group,
* you can reference it from one or more cache behaviors. When you reference a key group in a cache behavior,
* CloudFront requires signed URLs or signed cookies for all requests that match the cache behavior. The URLs or
* cookies must be signed with a private key whose corresponding public key is in the key group. The signed URL or
* cookie contains information about which public key CloudFront should use to verify the signature. For more
* information, see Serving private
* content in the Amazon CloudFront Developer Guide.
*
*
* @param createKeyGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateKeyGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyGroupAsync(CreateKeyGroupRequest createKeyGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies the key value store resource to add to your account. In your account, the key value store names must be
* unique. You can also import key value store data in JSON format from an S3 bucket by providing a valid
* ImportSource
that you own.
*
*
* @param createKeyValueStoreRequest
* @return A Java Future containing the result of the CreateKeyValueStore operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateKeyValueStore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createKeyValueStoreAsync(CreateKeyValueStoreRequest createKeyValueStoreRequest);
/**
*
* Specifies the key value store resource to add to your account. In your account, the key value store names must be
* unique. You can also import key value store data in JSON format from an S3 bucket by providing a valid
* ImportSource
that you own.
*
*
* @param createKeyValueStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateKeyValueStore operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateKeyValueStore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createKeyValueStoreAsync(CreateKeyValueStoreRequest createKeyValueStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables additional CloudWatch metrics for the specified CloudFront distribution. The additional metrics incur an
* additional cost.
*
*
* For more information, see Viewing additional CloudFront distribution metrics in the Amazon CloudFront Developer Guide.
*
*
* @param createMonitoringSubscriptionRequest
* @return A Java Future containing the result of the CreateMonitoringSubscription operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateMonitoringSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createMonitoringSubscriptionAsync(
CreateMonitoringSubscriptionRequest createMonitoringSubscriptionRequest);
/**
*
* Enables additional CloudWatch metrics for the specified CloudFront distribution. The additional metrics incur an
* additional cost.
*
*
* For more information, see Viewing additional CloudFront distribution metrics in the Amazon CloudFront Developer Guide.
*
*
* @param createMonitoringSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMonitoringSubscription operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateMonitoringSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createMonitoringSubscriptionAsync(
CreateMonitoringSubscriptionRequest createMonitoringSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new origin access control in CloudFront. After you create an origin access control, you can add it to
* an origin in a CloudFront distribution so that CloudFront sends authenticated (signed) requests to the origin.
*
*
* This makes it possible to block public access to the origin, allowing viewers (users) to access the origin's
* content only through CloudFront.
*
*
* For more information about using a CloudFront origin access control, see Restricting access to an Amazon Web Services origin in the Amazon CloudFront Developer Guide.
*
*
* @param createOriginAccessControlRequest
* @return A Java Future containing the result of the CreateOriginAccessControl operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateOriginAccessControl
* @see AWS API Documentation
*/
java.util.concurrent.Future createOriginAccessControlAsync(
CreateOriginAccessControlRequest createOriginAccessControlRequest);
/**
*
* Creates a new origin access control in CloudFront. After you create an origin access control, you can add it to
* an origin in a CloudFront distribution so that CloudFront sends authenticated (signed) requests to the origin.
*
*
* This makes it possible to block public access to the origin, allowing viewers (users) to access the origin's
* content only through CloudFront.
*
*
* For more information about using a CloudFront origin access control, see Restricting access to an Amazon Web Services origin in the Amazon CloudFront Developer Guide.
*
*
* @param createOriginAccessControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOriginAccessControl operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateOriginAccessControl
* @see AWS API Documentation
*/
java.util.concurrent.Future createOriginAccessControlAsync(
CreateOriginAccessControlRequest createOriginAccessControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an origin request policy.
*
*
* After you create an origin request policy, you can attach it to one or more cache behaviors. When it's attached
* to a cache behavior, the origin request policy determines the values that CloudFront includes in requests that it
* sends to the origin. Each request that CloudFront sends to the origin includes the following:
*
*
* -
*
* The request body and the URL path (without the domain name) from the viewer request.
*
*
* -
*
* The headers that CloudFront automatically includes in every origin request, including Host
,
* User-Agent
, and X-Amz-Cf-Id
.
*
*
* -
*
* All HTTP headers, cookies, and URL query strings that are specified in the cache policy or the origin request
* policy. These can include items from the viewer request and, in the case of headers, additional ones that are
* added by CloudFront.
*
*
*
*
* CloudFront sends a request when it can't find a valid object in its cache that matches the request. If you want
* to send values to the origin and also include them in the cache key, use CachePolicy
.
*
*
* For more information about origin request policies, see Controlling origin requests in the Amazon CloudFront Developer Guide.
*
*
* @param createOriginRequestPolicyRequest
* @return A Java Future containing the result of the CreateOriginRequestPolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateOriginRequestPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createOriginRequestPolicyAsync(
CreateOriginRequestPolicyRequest createOriginRequestPolicyRequest);
/**
*
* Creates an origin request policy.
*
*
* After you create an origin request policy, you can attach it to one or more cache behaviors. When it's attached
* to a cache behavior, the origin request policy determines the values that CloudFront includes in requests that it
* sends to the origin. Each request that CloudFront sends to the origin includes the following:
*
*
* -
*
* The request body and the URL path (without the domain name) from the viewer request.
*
*
* -
*
* The headers that CloudFront automatically includes in every origin request, including Host
,
* User-Agent
, and X-Amz-Cf-Id
.
*
*
* -
*
* All HTTP headers, cookies, and URL query strings that are specified in the cache policy or the origin request
* policy. These can include items from the viewer request and, in the case of headers, additional ones that are
* added by CloudFront.
*
*
*
*
* CloudFront sends a request when it can't find a valid object in its cache that matches the request. If you want
* to send values to the origin and also include them in the cache key, use CachePolicy
.
*
*
* For more information about origin request policies, see Controlling origin requests in the Amazon CloudFront Developer Guide.
*
*
* @param createOriginRequestPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOriginRequestPolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateOriginRequestPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createOriginRequestPolicyAsync(
CreateOriginRequestPolicyRequest createOriginRequestPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads a public key to CloudFront that you can use with signed URLs and
* signed cookies, or with field-level
* encryption.
*
*
* @param createPublicKeyRequest
* @return A Java Future containing the result of the CreatePublicKey operation returned by the service.
* @sample AmazonCloudFrontAsync.CreatePublicKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPublicKeyAsync(CreatePublicKeyRequest createPublicKeyRequest);
/**
*
* Uploads a public key to CloudFront that you can use with signed URLs and
* signed cookies, or with field-level
* encryption.
*
*
* @param createPublicKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePublicKey operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreatePublicKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPublicKeyAsync(CreatePublicKeyRequest createPublicKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a real-time log configuration.
*
*
* After you create a real-time log configuration, you can attach it to one or more cache behaviors to send
* real-time log data to the specified Amazon Kinesis data stream.
*
*
* For more information about real-time log configurations, see Real-time logs
* in the Amazon CloudFront Developer Guide.
*
*
* @param createRealtimeLogConfigRequest
* @return A Java Future containing the result of the CreateRealtimeLogConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createRealtimeLogConfigAsync(CreateRealtimeLogConfigRequest createRealtimeLogConfigRequest);
/**
*
* Creates a real-time log configuration.
*
*
* After you create a real-time log configuration, you can attach it to one or more cache behaviors to send
* real-time log data to the specified Amazon Kinesis data stream.
*
*
* For more information about real-time log configurations, see Real-time logs
* in the Amazon CloudFront Developer Guide.
*
*
* @param createRealtimeLogConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRealtimeLogConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createRealtimeLogConfigAsync(CreateRealtimeLogConfigRequest createRealtimeLogConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a response headers policy.
*
*
* A response headers policy contains information about a set of HTTP headers. To create a response headers policy,
* you provide some metadata about the policy and a set of configurations that specify the headers.
*
*
* After you create a response headers policy, you can use its ID to attach it to one or more cache behaviors in a
* CloudFront distribution. When it's attached to a cache behavior, the response headers policy affects the HTTP
* headers that CloudFront includes in HTTP responses to requests that match the cache behavior. CloudFront adds or
* removes response headers according to the configuration of the response headers policy.
*
*
* For more information, see Adding
* or removing HTTP headers in CloudFront responses in the Amazon CloudFront Developer Guide.
*
*
* @param createResponseHeadersPolicyRequest
* @return A Java Future containing the result of the CreateResponseHeadersPolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateResponseHeadersPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createResponseHeadersPolicyAsync(
CreateResponseHeadersPolicyRequest createResponseHeadersPolicyRequest);
/**
*
* Creates a response headers policy.
*
*
* A response headers policy contains information about a set of HTTP headers. To create a response headers policy,
* you provide some metadata about the policy and a set of configurations that specify the headers.
*
*
* After you create a response headers policy, you can use its ID to attach it to one or more cache behaviors in a
* CloudFront distribution. When it's attached to a cache behavior, the response headers policy affects the HTTP
* headers that CloudFront includes in HTTP responses to requests that match the cache behavior. CloudFront adds or
* removes response headers according to the configuration of the response headers policy.
*
*
* For more information, see Adding
* or removing HTTP headers in CloudFront responses in the Amazon CloudFront Developer Guide.
*
*
* @param createResponseHeadersPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateResponseHeadersPolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateResponseHeadersPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createResponseHeadersPolicyAsync(
CreateResponseHeadersPolicyRequest createResponseHeadersPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This API is deprecated. Amazon CloudFront is deprecating real-time messaging protocol (RTMP) distributions on
* December 31, 2020. For more information, read the
* announcement on the Amazon CloudFront discussion forum.
*
*
* @param createStreamingDistributionRequest
* The request to create a new streaming distribution.
* @return A Java Future containing the result of the CreateStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.CreateStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionAsync(
CreateStreamingDistributionRequest createStreamingDistributionRequest);
/**
*
* This API is deprecated. Amazon CloudFront is deprecating real-time messaging protocol (RTMP) distributions on
* December 31, 2020. For more information, read the
* announcement on the Amazon CloudFront discussion forum.
*
*
* @param createStreamingDistributionRequest
* The request to create a new streaming distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.CreateStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionAsync(
CreateStreamingDistributionRequest createStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This API is deprecated. Amazon CloudFront is deprecating real-time messaging protocol (RTMP) distributions on
* December 31, 2020. For more information, read the
* announcement on the Amazon CloudFront discussion forum.
*
*
* @param createStreamingDistributionWithTagsRequest
* The request to create a new streaming distribution with tags.
* @return A Java Future containing the result of the CreateStreamingDistributionWithTags operation returned by the
* service.
* @sample AmazonCloudFrontAsync.CreateStreamingDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionWithTagsAsync(
CreateStreamingDistributionWithTagsRequest createStreamingDistributionWithTagsRequest);
/**
*
* This API is deprecated. Amazon CloudFront is deprecating real-time messaging protocol (RTMP) distributions on
* December 31, 2020. For more information, read the
* announcement on the Amazon CloudFront discussion forum.
*
*
* @param createStreamingDistributionWithTagsRequest
* The request to create a new streaming distribution with tags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStreamingDistributionWithTags operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.CreateStreamingDistributionWithTags
* @see AWS API Documentation
*/
java.util.concurrent.Future createStreamingDistributionWithTagsAsync(
CreateStreamingDistributionWithTagsRequest createStreamingDistributionWithTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a cache policy.
*
*
* You cannot delete a cache policy if it's attached to a cache behavior. First update your distributions to remove
* the cache policy from all cache behaviors, then delete the cache policy.
*
*
* To delete a cache policy, you must provide the policy's identifier and version. To get these values, you can use
* ListCachePolicies
or GetCachePolicy
.
*
*
* @param deleteCachePolicyRequest
* @return A Java Future containing the result of the DeleteCachePolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteCachePolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteCachePolicyAsync(DeleteCachePolicyRequest deleteCachePolicyRequest);
/**
*
* Deletes a cache policy.
*
*
* You cannot delete a cache policy if it's attached to a cache behavior. First update your distributions to remove
* the cache policy from all cache behaviors, then delete the cache policy.
*
*
* To delete a cache policy, you must provide the policy's identifier and version. To get these values, you can use
* ListCachePolicies
or GetCachePolicy
.
*
*
* @param deleteCachePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCachePolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteCachePolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteCachePolicyAsync(DeleteCachePolicyRequest deleteCachePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an origin access identity.
*
*
* @param deleteCloudFrontOriginAccessIdentityRequest
* Deletes a origin access identity.
* @return A Java Future containing the result of the DeleteCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.DeleteCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCloudFrontOriginAccessIdentityAsync(
DeleteCloudFrontOriginAccessIdentityRequest deleteCloudFrontOriginAccessIdentityRequest);
/**
*
* Delete an origin access identity.
*
*
* @param deleteCloudFrontOriginAccessIdentityRequest
* Deletes a origin access identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.DeleteCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCloudFrontOriginAccessIdentityAsync(
DeleteCloudFrontOriginAccessIdentityRequest deleteCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a continuous deployment policy.
*
*
* You cannot delete a continuous deployment policy that's attached to a primary distribution. First update your
* distribution to remove the continuous deployment policy, then you can delete the policy.
*
*
* @param deleteContinuousDeploymentPolicyRequest
* @return A Java Future containing the result of the DeleteContinuousDeploymentPolicy operation returned by the
* service.
* @sample AmazonCloudFrontAsync.DeleteContinuousDeploymentPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteContinuousDeploymentPolicyAsync(
DeleteContinuousDeploymentPolicyRequest deleteContinuousDeploymentPolicyRequest);
/**
*
* Deletes a continuous deployment policy.
*
*
* You cannot delete a continuous deployment policy that's attached to a primary distribution. First update your
* distribution to remove the continuous deployment policy, then you can delete the policy.
*
*
* @param deleteContinuousDeploymentPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContinuousDeploymentPolicy operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.DeleteContinuousDeploymentPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteContinuousDeploymentPolicyAsync(
DeleteContinuousDeploymentPolicyRequest deleteContinuousDeploymentPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a distribution.
*
*
* @param deleteDistributionRequest
* This action deletes a web distribution. To delete a web distribution using the CloudFront API, perform the
* following steps.
*
* To delete a web distribution using the CloudFront API:
*
*
* -
*
* Disable the web distribution
*
*
* -
*
* Submit a GET Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Distribution Config
request to update the configuration for your distribution.
* In the request body, include the XML document that you updated in Step 3. Set the value of the HTTP
* If-Match
header to the value of the ETag
header that CloudFront returned when
* you submitted the GET Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Distribution Config
request to confirm that the distribution
* was successfully disabled.
*
*
* -
*
* Submit a GET Distribution
request to confirm that your changes have propagated. When
* propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Distribution
request. Set the value of the HTTP If-Match
header
* to the value of the ETag
header that CloudFront returned when you submitted the
* GET Distribution Config
request in Step 6.
*
*
* -
*
* Review the response to your DELETE Distribution
request to confirm that the distribution was
* successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a Distribution in the Amazon CloudFront Developer Guide.
* @return A Java Future containing the result of the DeleteDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDistributionAsync(DeleteDistributionRequest deleteDistributionRequest);
/**
*
* Delete a distribution.
*
*
* @param deleteDistributionRequest
* This action deletes a web distribution. To delete a web distribution using the CloudFront API, perform the
* following steps.
*
* To delete a web distribution using the CloudFront API:
*
*
* -
*
* Disable the web distribution
*
*
* -
*
* Submit a GET Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Distribution Config
request to update the configuration for your distribution.
* In the request body, include the XML document that you updated in Step 3. Set the value of the HTTP
* If-Match
header to the value of the ETag
header that CloudFront returned when
* you submitted the GET Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Distribution Config
request to confirm that the distribution
* was successfully disabled.
*
*
* -
*
* Submit a GET Distribution
request to confirm that your changes have propagated. When
* propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Distribution
request. Set the value of the HTTP If-Match
header
* to the value of the ETag
header that CloudFront returned when you submitted the
* GET Distribution Config
request in Step 6.
*
*
* -
*
* Review the response to your DELETE Distribution
request to confirm that the distribution was
* successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a Distribution in the Amazon CloudFront Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteDistribution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDistributionAsync(DeleteDistributionRequest deleteDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove a field-level encryption configuration.
*
*
* @param deleteFieldLevelEncryptionConfigRequest
* @return A Java Future containing the result of the DeleteFieldLevelEncryptionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.DeleteFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFieldLevelEncryptionConfigAsync(
DeleteFieldLevelEncryptionConfigRequest deleteFieldLevelEncryptionConfigRequest);
/**
*
* Remove a field-level encryption configuration.
*
*
* @param deleteFieldLevelEncryptionConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFieldLevelEncryptionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.DeleteFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFieldLevelEncryptionConfigAsync(
DeleteFieldLevelEncryptionConfigRequest deleteFieldLevelEncryptionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove a field-level encryption profile.
*
*
* @param deleteFieldLevelEncryptionProfileRequest
* @return A Java Future containing the result of the DeleteFieldLevelEncryptionProfile operation returned by the
* service.
* @sample AmazonCloudFrontAsync.DeleteFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFieldLevelEncryptionProfileAsync(
DeleteFieldLevelEncryptionProfileRequest deleteFieldLevelEncryptionProfileRequest);
/**
*
* Remove a field-level encryption profile.
*
*
* @param deleteFieldLevelEncryptionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFieldLevelEncryptionProfile operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.DeleteFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFieldLevelEncryptionProfileAsync(
DeleteFieldLevelEncryptionProfileRequest deleteFieldLevelEncryptionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a CloudFront function.
*
*
* You cannot delete a function if it's associated with a cache behavior. First, update your distributions to remove
* the function association from all cache behaviors, then delete the function.
*
*
* To delete a function, you must provide the function's name and version (ETag
value). To get these
* values, you can use ListFunctions
and DescribeFunction
.
*
*
* @param deleteFunctionRequest
* @return A Java Future containing the result of the DeleteFunction operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFunctionAsync(DeleteFunctionRequest deleteFunctionRequest);
/**
*
* Deletes a CloudFront function.
*
*
* You cannot delete a function if it's associated with a cache behavior. First, update your distributions to remove
* the function association from all cache behaviors, then delete the function.
*
*
* To delete a function, you must provide the function's name and version (ETag
value). To get these
* values, you can use ListFunctions
and DescribeFunction
.
*
*
* @param deleteFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFunction operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFunctionAsync(DeleteFunctionRequest deleteFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a key group.
*
*
* You cannot delete a key group that is referenced in a cache behavior. First update your distributions to remove
* the key group from all cache behaviors, then delete the key group.
*
*
* To delete a key group, you must provide the key group's identifier and version. To get these values, use
* ListKeyGroups
followed by GetKeyGroup
or GetKeyGroupConfig
.
*
*
* @param deleteKeyGroupRequest
* @return A Java Future containing the result of the DeleteKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteKeyGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteKeyGroupAsync(DeleteKeyGroupRequest deleteKeyGroupRequest);
/**
*
* Deletes a key group.
*
*
* You cannot delete a key group that is referenced in a cache behavior. First update your distributions to remove
* the key group from all cache behaviors, then delete the key group.
*
*
* To delete a key group, you must provide the key group's identifier and version. To get these values, use
* ListKeyGroups
followed by GetKeyGroup
or GetKeyGroupConfig
.
*
*
* @param deleteKeyGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteKeyGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteKeyGroupAsync(DeleteKeyGroupRequest deleteKeyGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies the key value store to delete.
*
*
* @param deleteKeyValueStoreRequest
* @return A Java Future containing the result of the DeleteKeyValueStore operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteKeyValueStore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteKeyValueStoreAsync(DeleteKeyValueStoreRequest deleteKeyValueStoreRequest);
/**
*
* Specifies the key value store to delete.
*
*
* @param deleteKeyValueStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteKeyValueStore operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteKeyValueStore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteKeyValueStoreAsync(DeleteKeyValueStoreRequest deleteKeyValueStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables additional CloudWatch metrics for the specified CloudFront distribution.
*
*
* @param deleteMonitoringSubscriptionRequest
* @return A Java Future containing the result of the DeleteMonitoringSubscription operation returned by the
* service.
* @sample AmazonCloudFrontAsync.DeleteMonitoringSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMonitoringSubscriptionAsync(
DeleteMonitoringSubscriptionRequest deleteMonitoringSubscriptionRequest);
/**
*
* Disables additional CloudWatch metrics for the specified CloudFront distribution.
*
*
* @param deleteMonitoringSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMonitoringSubscription operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.DeleteMonitoringSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMonitoringSubscriptionAsync(
DeleteMonitoringSubscriptionRequest deleteMonitoringSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a CloudFront origin access control.
*
*
* You cannot delete an origin access control if it's in use. First, update all distributions to remove the origin
* access control from all origins, then delete the origin access control.
*
*
* @param deleteOriginAccessControlRequest
* @return A Java Future containing the result of the DeleteOriginAccessControl operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteOriginAccessControl
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOriginAccessControlAsync(
DeleteOriginAccessControlRequest deleteOriginAccessControlRequest);
/**
*
* Deletes a CloudFront origin access control.
*
*
* You cannot delete an origin access control if it's in use. First, update all distributions to remove the origin
* access control from all origins, then delete the origin access control.
*
*
* @param deleteOriginAccessControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOriginAccessControl operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteOriginAccessControl
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOriginAccessControlAsync(
DeleteOriginAccessControlRequest deleteOriginAccessControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an origin request policy.
*
*
* You cannot delete an origin request policy if it's attached to any cache behaviors. First update your
* distributions to remove the origin request policy from all cache behaviors, then delete the origin request
* policy.
*
*
* To delete an origin request policy, you must provide the policy's identifier and version. To get the identifier,
* you can use ListOriginRequestPolicies
or GetOriginRequestPolicy
.
*
*
* @param deleteOriginRequestPolicyRequest
* @return A Java Future containing the result of the DeleteOriginRequestPolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteOriginRequestPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOriginRequestPolicyAsync(
DeleteOriginRequestPolicyRequest deleteOriginRequestPolicyRequest);
/**
*
* Deletes an origin request policy.
*
*
* You cannot delete an origin request policy if it's attached to any cache behaviors. First update your
* distributions to remove the origin request policy from all cache behaviors, then delete the origin request
* policy.
*
*
* To delete an origin request policy, you must provide the policy's identifier and version. To get the identifier,
* you can use ListOriginRequestPolicies
or GetOriginRequestPolicy
.
*
*
* @param deleteOriginRequestPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOriginRequestPolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteOriginRequestPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOriginRequestPolicyAsync(
DeleteOriginRequestPolicyRequest deleteOriginRequestPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove a public key you previously added to CloudFront.
*
*
* @param deletePublicKeyRequest
* @return A Java Future containing the result of the DeletePublicKey operation returned by the service.
* @sample AmazonCloudFrontAsync.DeletePublicKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePublicKeyAsync(DeletePublicKeyRequest deletePublicKeyRequest);
/**
*
* Remove a public key you previously added to CloudFront.
*
*
* @param deletePublicKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePublicKey operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeletePublicKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePublicKeyAsync(DeletePublicKeyRequest deletePublicKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a real-time log configuration.
*
*
* You cannot delete a real-time log configuration if it's attached to a cache behavior. First update your
* distributions to remove the real-time log configuration from all cache behaviors, then delete the real-time log
* configuration.
*
*
* To delete a real-time log configuration, you can provide the configuration's name or its Amazon Resource Name
* (ARN). You must provide at least one. If you provide both, CloudFront uses the name to identify the real-time log
* configuration to delete.
*
*
* @param deleteRealtimeLogConfigRequest
* @return A Java Future containing the result of the DeleteRealtimeLogConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRealtimeLogConfigAsync(DeleteRealtimeLogConfigRequest deleteRealtimeLogConfigRequest);
/**
*
* Deletes a real-time log configuration.
*
*
* You cannot delete a real-time log configuration if it's attached to a cache behavior. First update your
* distributions to remove the real-time log configuration from all cache behaviors, then delete the real-time log
* configuration.
*
*
* To delete a real-time log configuration, you can provide the configuration's name or its Amazon Resource Name
* (ARN). You must provide at least one. If you provide both, CloudFront uses the name to identify the real-time log
* configuration to delete.
*
*
* @param deleteRealtimeLogConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRealtimeLogConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRealtimeLogConfigAsync(DeleteRealtimeLogConfigRequest deleteRealtimeLogConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a response headers policy.
*
*
* You cannot delete a response headers policy if it's attached to a cache behavior. First update your distributions
* to remove the response headers policy from all cache behaviors, then delete the response headers policy.
*
*
* To delete a response headers policy, you must provide the policy's identifier and version. To get these values,
* you can use ListResponseHeadersPolicies
or GetResponseHeadersPolicy
.
*
*
* @param deleteResponseHeadersPolicyRequest
* @return A Java Future containing the result of the DeleteResponseHeadersPolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteResponseHeadersPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResponseHeadersPolicyAsync(
DeleteResponseHeadersPolicyRequest deleteResponseHeadersPolicyRequest);
/**
*
* Deletes a response headers policy.
*
*
* You cannot delete a response headers policy if it's attached to a cache behavior. First update your distributions
* to remove the response headers policy from all cache behaviors, then delete the response headers policy.
*
*
* To delete a response headers policy, you must provide the policy's identifier and version. To get these values,
* you can use ListResponseHeadersPolicies
or GetResponseHeadersPolicy
.
*
*
* @param deleteResponseHeadersPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResponseHeadersPolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteResponseHeadersPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResponseHeadersPolicyAsync(
DeleteResponseHeadersPolicyRequest deleteResponseHeadersPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a streaming distribution. To delete an RTMP distribution using the CloudFront API, perform the following
* steps.
*
*
* To delete an RTMP distribution using the CloudFront API:
*
*
* -
*
* Disable the RTMP distribution.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Streaming Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Streaming Distribution Config
request to update the configuration for your
* distribution. In the request body, include the XML document that you updated in Step 3. Then set the value of the
* HTTP If-Match
header to the value of the ETag
header that CloudFront returned when you
* submitted the GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Streaming Distribution Config
request to confirm that the
* distribution was successfully disabled.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to confirm that your changes have propagated.
* When propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Streaming Distribution
request. Set the value of the HTTP If-Match
* header to the value of the ETag
header that CloudFront returned when you submitted the
* GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to your DELETE Streaming Distribution
request to confirm that the distribution
* was successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a
* Distribution in the Amazon CloudFront Developer Guide.
*
*
* @param deleteStreamingDistributionRequest
* The request to delete a streaming distribution.
* @return A Java Future containing the result of the DeleteStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.DeleteStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStreamingDistributionAsync(
DeleteStreamingDistributionRequest deleteStreamingDistributionRequest);
/**
*
* Delete a streaming distribution. To delete an RTMP distribution using the CloudFront API, perform the following
* steps.
*
*
* To delete an RTMP distribution using the CloudFront API:
*
*
* -
*
* Disable the RTMP distribution.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to get the current configuration and the
* Etag
header for the distribution.
*
*
* -
*
* Update the XML document that was returned in the response to your GET Streaming Distribution Config
* request to change the value of Enabled
to false
.
*
*
* -
*
* Submit a PUT Streaming Distribution Config
request to update the configuration for your
* distribution. In the request body, include the XML document that you updated in Step 3. Then set the value of the
* HTTP If-Match
header to the value of the ETag
header that CloudFront returned when you
* submitted the GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to the PUT Streaming Distribution Config
request to confirm that the
* distribution was successfully disabled.
*
*
* -
*
* Submit a GET Streaming Distribution Config
request to confirm that your changes have propagated.
* When propagation is complete, the value of Status
is Deployed
.
*
*
* -
*
* Submit a DELETE Streaming Distribution
request. Set the value of the HTTP If-Match
* header to the value of the ETag
header that CloudFront returned when you submitted the
* GET Streaming Distribution Config
request in Step 2.
*
*
* -
*
* Review the response to your DELETE Streaming Distribution
request to confirm that the distribution
* was successfully deleted.
*
*
*
*
* For information about deleting a distribution using the CloudFront console, see Deleting a
* Distribution in the Amazon CloudFront Developer Guide.
*
*
* @param deleteStreamingDistributionRequest
* The request to delete a streaming distribution.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DeleteStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStreamingDistributionAsync(
DeleteStreamingDistributionRequest deleteStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets configuration information and metadata about a CloudFront function, but not the function's code. To get a
* function's code, use GetFunction
.
*
*
* To get configuration information and metadata about a function, you must provide the function's name and stage.
* To get these values, you can use ListFunctions
.
*
*
* @param describeFunctionRequest
* @return A Java Future containing the result of the DescribeFunction operation returned by the service.
* @sample AmazonCloudFrontAsync.DescribeFunction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeFunctionAsync(DescribeFunctionRequest describeFunctionRequest);
/**
*
* Gets configuration information and metadata about a CloudFront function, but not the function's code. To get a
* function's code, use GetFunction
.
*
*
* To get configuration information and metadata about a function, you must provide the function's name and stage.
* To get these values, you can use ListFunctions
.
*
*
* @param describeFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFunction operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DescribeFunction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeFunctionAsync(DescribeFunctionRequest describeFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies the key value store and its configuration.
*
*
* @param describeKeyValueStoreRequest
* @return A Java Future containing the result of the DescribeKeyValueStore operation returned by the service.
* @sample AmazonCloudFrontAsync.DescribeKeyValueStore
* @see AWS API Documentation
*/
java.util.concurrent.Future describeKeyValueStoreAsync(DescribeKeyValueStoreRequest describeKeyValueStoreRequest);
/**
*
* Specifies the key value store and its configuration.
*
*
* @param describeKeyValueStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeKeyValueStore operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.DescribeKeyValueStore
* @see AWS API Documentation
*/
java.util.concurrent.Future describeKeyValueStoreAsync(DescribeKeyValueStoreRequest describeKeyValueStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a cache policy, including the following metadata:
*
*
* -
*
* The policy's identifier.
*
*
* -
*
* The date and time when the policy was last modified.
*
*
*
*
* To get a cache policy, you must provide the policy's identifier. If the cache policy is attached to a
* distribution's cache behavior, you can get the policy's identifier using ListDistributions
or
* GetDistribution
. If the cache policy is not attached to a cache behavior, you can get the identifier
* using ListCachePolicies
.
*
*
* @param getCachePolicyRequest
* @return A Java Future containing the result of the GetCachePolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.GetCachePolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCachePolicyAsync(GetCachePolicyRequest getCachePolicyRequest);
/**
*
* Gets a cache policy, including the following metadata:
*
*
* -
*
* The policy's identifier.
*
*
* -
*
* The date and time when the policy was last modified.
*
*
*
*
* To get a cache policy, you must provide the policy's identifier. If the cache policy is attached to a
* distribution's cache behavior, you can get the policy's identifier using ListDistributions
or
* GetDistribution
. If the cache policy is not attached to a cache behavior, you can get the identifier
* using ListCachePolicies
.
*
*
* @param getCachePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCachePolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetCachePolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCachePolicyAsync(GetCachePolicyRequest getCachePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a cache policy configuration.
*
*
* To get a cache policy configuration, you must provide the policy's identifier. If the cache policy is attached to
* a distribution's cache behavior, you can get the policy's identifier using ListDistributions
or
* GetDistribution
. If the cache policy is not attached to a cache behavior, you can get the identifier
* using ListCachePolicies
.
*
*
* @param getCachePolicyConfigRequest
* @return A Java Future containing the result of the GetCachePolicyConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.GetCachePolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getCachePolicyConfigAsync(GetCachePolicyConfigRequest getCachePolicyConfigRequest);
/**
*
* Gets a cache policy configuration.
*
*
* To get a cache policy configuration, you must provide the policy's identifier. If the cache policy is attached to
* a distribution's cache behavior, you can get the policy's identifier using ListDistributions
or
* GetDistribution
. If the cache policy is not attached to a cache behavior, you can get the identifier
* using ListCachePolicies
.
*
*
* @param getCachePolicyConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCachePolicyConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetCachePolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getCachePolicyConfigAsync(GetCachePolicyConfigRequest getCachePolicyConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityRequest
* The request to get an origin access identity's information.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityAsync(
GetCloudFrontOriginAccessIdentityRequest getCloudFrontOriginAccessIdentityRequest);
/**
*
* Get the information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityRequest
* The request to get an origin access identity's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentity operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetCloudFrontOriginAccessIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityAsync(
GetCloudFrontOriginAccessIdentityRequest getCloudFrontOriginAccessIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the configuration information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityConfigRequest
* The origin access identity's configuration information. For more information, see CloudFrontOriginAccessIdentityConfig.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentityConfig operation returned by
* the service.
* @sample AmazonCloudFrontAsync.GetCloudFrontOriginAccessIdentityConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityConfigAsync(
GetCloudFrontOriginAccessIdentityConfigRequest getCloudFrontOriginAccessIdentityConfigRequest);
/**
*
* Get the configuration information about an origin access identity.
*
*
* @param getCloudFrontOriginAccessIdentityConfigRequest
* The origin access identity's configuration information. For more information, see CloudFrontOriginAccessIdentityConfig.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCloudFrontOriginAccessIdentityConfig operation returned by
* the service.
* @sample AmazonCloudFrontAsyncHandler.GetCloudFrontOriginAccessIdentityConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFrontOriginAccessIdentityConfigAsync(
GetCloudFrontOriginAccessIdentityConfigRequest getCloudFrontOriginAccessIdentityConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a continuous deployment policy, including metadata (the policy's identifier and the date and time when the
* policy was last modified).
*
*
* @param getContinuousDeploymentPolicyRequest
* @return A Java Future containing the result of the GetContinuousDeploymentPolicy operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetContinuousDeploymentPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getContinuousDeploymentPolicyAsync(
GetContinuousDeploymentPolicyRequest getContinuousDeploymentPolicyRequest);
/**
*
* Gets a continuous deployment policy, including metadata (the policy's identifier and the date and time when the
* policy was last modified).
*
*
* @param getContinuousDeploymentPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContinuousDeploymentPolicy operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetContinuousDeploymentPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getContinuousDeploymentPolicyAsync(
GetContinuousDeploymentPolicyRequest getContinuousDeploymentPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets configuration information about a continuous deployment policy.
*
*
* @param getContinuousDeploymentPolicyConfigRequest
* @return A Java Future containing the result of the GetContinuousDeploymentPolicyConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetContinuousDeploymentPolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getContinuousDeploymentPolicyConfigAsync(
GetContinuousDeploymentPolicyConfigRequest getContinuousDeploymentPolicyConfigRequest);
/**
*
* Gets configuration information about a continuous deployment policy.
*
*
* @param getContinuousDeploymentPolicyConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContinuousDeploymentPolicyConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetContinuousDeploymentPolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getContinuousDeploymentPolicyConfigAsync(
GetContinuousDeploymentPolicyConfigRequest getContinuousDeploymentPolicyConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the information about a distribution.
*
*
* @param getDistributionRequest
* The request to get a distribution's information.
* @return A Java Future containing the result of the GetDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.GetDistribution
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDistributionAsync(GetDistributionRequest getDistributionRequest);
/**
*
* Get the information about a distribution.
*
*
* @param getDistributionRequest
* The request to get a distribution's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetDistribution
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDistributionAsync(GetDistributionRequest getDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the configuration information about a distribution.
*
*
* @param getDistributionConfigRequest
* The request to get a distribution configuration.
* @return A Java Future containing the result of the GetDistributionConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.GetDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getDistributionConfigAsync(GetDistributionConfigRequest getDistributionConfigRequest);
/**
*
* Get the configuration information about a distribution.
*
*
* @param getDistributionConfigRequest
* The request to get a distribution configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDistributionConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getDistributionConfigAsync(GetDistributionConfigRequest getDistributionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the field-level encryption configuration information.
*
*
* @param getFieldLevelEncryptionRequest
* @return A Java Future containing the result of the GetFieldLevelEncryption operation returned by the service.
* @sample AmazonCloudFrontAsync.GetFieldLevelEncryption
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionAsync(GetFieldLevelEncryptionRequest getFieldLevelEncryptionRequest);
/**
*
* Get the field-level encryption configuration information.
*
*
* @param getFieldLevelEncryptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFieldLevelEncryption operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetFieldLevelEncryption
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionAsync(GetFieldLevelEncryptionRequest getFieldLevelEncryptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the field-level encryption configuration information.
*
*
* @param getFieldLevelEncryptionConfigRequest
* @return A Java Future containing the result of the GetFieldLevelEncryptionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionConfigAsync(
GetFieldLevelEncryptionConfigRequest getFieldLevelEncryptionConfigRequest);
/**
*
* Get the field-level encryption configuration information.
*
*
* @param getFieldLevelEncryptionConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFieldLevelEncryptionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetFieldLevelEncryptionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionConfigAsync(
GetFieldLevelEncryptionConfigRequest getFieldLevelEncryptionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the field-level encryption profile information.
*
*
* @param getFieldLevelEncryptionProfileRequest
* @return A Java Future containing the result of the GetFieldLevelEncryptionProfile operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionProfileAsync(
GetFieldLevelEncryptionProfileRequest getFieldLevelEncryptionProfileRequest);
/**
*
* Get the field-level encryption profile information.
*
*
* @param getFieldLevelEncryptionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFieldLevelEncryptionProfile operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetFieldLevelEncryptionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionProfileAsync(
GetFieldLevelEncryptionProfileRequest getFieldLevelEncryptionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the field-level encryption profile configuration information.
*
*
* @param getFieldLevelEncryptionProfileConfigRequest
* @return A Java Future containing the result of the GetFieldLevelEncryptionProfileConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetFieldLevelEncryptionProfileConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionProfileConfigAsync(
GetFieldLevelEncryptionProfileConfigRequest getFieldLevelEncryptionProfileConfigRequest);
/**
*
* Get the field-level encryption profile configuration information.
*
*
* @param getFieldLevelEncryptionProfileConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFieldLevelEncryptionProfileConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetFieldLevelEncryptionProfileConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getFieldLevelEncryptionProfileConfigAsync(
GetFieldLevelEncryptionProfileConfigRequest getFieldLevelEncryptionProfileConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the code of a CloudFront function. To get configuration information and metadata about a function, use
* DescribeFunction
.
*
*
* To get a function's code, you must provide the function's name and stage. To get these values, you can use
* ListFunctions
.
*
*
* @param getFunctionRequest
* @return A Java Future containing the result of the GetFunction operation returned by the service.
* @sample AmazonCloudFrontAsync.GetFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFunctionAsync(GetFunctionRequest getFunctionRequest);
/**
*
* Gets the code of a CloudFront function. To get configuration information and metadata about a function, use
* DescribeFunction
.
*
*
* To get a function's code, you must provide the function's name and stage. To get these values, you can use
* ListFunctions
.
*
*
* @param getFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFunction operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFunctionAsync(GetFunctionRequest getFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the information about an invalidation.
*
*
* @param getInvalidationRequest
* The request to get an invalidation's information.
* @return A Java Future containing the result of the GetInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsync.GetInvalidation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInvalidationAsync(GetInvalidationRequest getInvalidationRequest);
/**
*
* Get the information about an invalidation.
*
*
* @param getInvalidationRequest
* The request to get an invalidation's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInvalidation operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetInvalidation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInvalidationAsync(GetInvalidationRequest getInvalidationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a key group, including the date and time when the key group was last modified.
*
*
* To get a key group, you must provide the key group's identifier. If the key group is referenced in a
* distribution's cache behavior, you can get the key group's identifier using ListDistributions
or
* GetDistribution
. If the key group is not referenced in a cache behavior, you can get the identifier
* using ListKeyGroups
.
*
*
* @param getKeyGroupRequest
* @return A Java Future containing the result of the GetKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsync.GetKeyGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getKeyGroupAsync(GetKeyGroupRequest getKeyGroupRequest);
/**
*
* Gets a key group, including the date and time when the key group was last modified.
*
*
* To get a key group, you must provide the key group's identifier. If the key group is referenced in a
* distribution's cache behavior, you can get the key group's identifier using ListDistributions
or
* GetDistribution
. If the key group is not referenced in a cache behavior, you can get the identifier
* using ListKeyGroups
.
*
*
* @param getKeyGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetKeyGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getKeyGroupAsync(GetKeyGroupRequest getKeyGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a key group configuration.
*
*
* To get a key group configuration, you must provide the key group's identifier. If the key group is referenced in
* a distribution's cache behavior, you can get the key group's identifier using ListDistributions
or
* GetDistribution
. If the key group is not referenced in a cache behavior, you can get the identifier
* using ListKeyGroups
.
*
*
* @param getKeyGroupConfigRequest
* @return A Java Future containing the result of the GetKeyGroupConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.GetKeyGroupConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getKeyGroupConfigAsync(GetKeyGroupConfigRequest getKeyGroupConfigRequest);
/**
*
* Gets a key group configuration.
*
*
* To get a key group configuration, you must provide the key group's identifier. If the key group is referenced in
* a distribution's cache behavior, you can get the key group's identifier using ListDistributions
or
* GetDistribution
. If the key group is not referenced in a cache behavior, you can get the identifier
* using ListKeyGroups
.
*
*
* @param getKeyGroupConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetKeyGroupConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetKeyGroupConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getKeyGroupConfigAsync(GetKeyGroupConfigRequest getKeyGroupConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about whether additional CloudWatch metrics are enabled for the specified CloudFront
* distribution.
*
*
* @param getMonitoringSubscriptionRequest
* @return A Java Future containing the result of the GetMonitoringSubscription operation returned by the service.
* @sample AmazonCloudFrontAsync.GetMonitoringSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future getMonitoringSubscriptionAsync(
GetMonitoringSubscriptionRequest getMonitoringSubscriptionRequest);
/**
*
* Gets information about whether additional CloudWatch metrics are enabled for the specified CloudFront
* distribution.
*
*
* @param getMonitoringSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMonitoringSubscription operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetMonitoringSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future getMonitoringSubscriptionAsync(
GetMonitoringSubscriptionRequest getMonitoringSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a CloudFront origin access control, including its unique identifier.
*
*
* @param getOriginAccessControlRequest
* @return A Java Future containing the result of the GetOriginAccessControl operation returned by the service.
* @sample AmazonCloudFrontAsync.GetOriginAccessControl
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginAccessControlAsync(GetOriginAccessControlRequest getOriginAccessControlRequest);
/**
*
* Gets a CloudFront origin access control, including its unique identifier.
*
*
* @param getOriginAccessControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOriginAccessControl operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetOriginAccessControl
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginAccessControlAsync(GetOriginAccessControlRequest getOriginAccessControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a CloudFront origin access control configuration.
*
*
* @param getOriginAccessControlConfigRequest
* @return A Java Future containing the result of the GetOriginAccessControlConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetOriginAccessControlConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginAccessControlConfigAsync(
GetOriginAccessControlConfigRequest getOriginAccessControlConfigRequest);
/**
*
* Gets a CloudFront origin access control configuration.
*
*
* @param getOriginAccessControlConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOriginAccessControlConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetOriginAccessControlConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginAccessControlConfigAsync(
GetOriginAccessControlConfigRequest getOriginAccessControlConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an origin request policy, including the following metadata:
*
*
* -
*
* The policy's identifier.
*
*
* -
*
* The date and time when the policy was last modified.
*
*
*
*
* To get an origin request policy, you must provide the policy's identifier. If the origin request policy is
* attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the origin request policy is not attached to a
* cache behavior, you can get the identifier using ListOriginRequestPolicies
.
*
*
* @param getOriginRequestPolicyRequest
* @return A Java Future containing the result of the GetOriginRequestPolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.GetOriginRequestPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginRequestPolicyAsync(GetOriginRequestPolicyRequest getOriginRequestPolicyRequest);
/**
*
* Gets an origin request policy, including the following metadata:
*
*
* -
*
* The policy's identifier.
*
*
* -
*
* The date and time when the policy was last modified.
*
*
*
*
* To get an origin request policy, you must provide the policy's identifier. If the origin request policy is
* attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the origin request policy is not attached to a
* cache behavior, you can get the identifier using ListOriginRequestPolicies
.
*
*
* @param getOriginRequestPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOriginRequestPolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetOriginRequestPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginRequestPolicyAsync(GetOriginRequestPolicyRequest getOriginRequestPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an origin request policy configuration.
*
*
* To get an origin request policy configuration, you must provide the policy's identifier. If the origin request
* policy is attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the origin request policy is not attached to a
* cache behavior, you can get the identifier using ListOriginRequestPolicies
.
*
*
* @param getOriginRequestPolicyConfigRequest
* @return A Java Future containing the result of the GetOriginRequestPolicyConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetOriginRequestPolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginRequestPolicyConfigAsync(
GetOriginRequestPolicyConfigRequest getOriginRequestPolicyConfigRequest);
/**
*
* Gets an origin request policy configuration.
*
*
* To get an origin request policy configuration, you must provide the policy's identifier. If the origin request
* policy is attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the origin request policy is not attached to a
* cache behavior, you can get the identifier using ListOriginRequestPolicies
.
*
*
* @param getOriginRequestPolicyConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOriginRequestPolicyConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetOriginRequestPolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getOriginRequestPolicyConfigAsync(
GetOriginRequestPolicyConfigRequest getOriginRequestPolicyConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a public key.
*
*
* @param getPublicKeyRequest
* @return A Java Future containing the result of the GetPublicKey operation returned by the service.
* @sample AmazonCloudFrontAsync.GetPublicKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPublicKeyAsync(GetPublicKeyRequest getPublicKeyRequest);
/**
*
* Gets a public key.
*
*
* @param getPublicKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPublicKey operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetPublicKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPublicKeyAsync(GetPublicKeyRequest getPublicKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a public key configuration.
*
*
* @param getPublicKeyConfigRequest
* @return A Java Future containing the result of the GetPublicKeyConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.GetPublicKeyConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getPublicKeyConfigAsync(GetPublicKeyConfigRequest getPublicKeyConfigRequest);
/**
*
* Gets a public key configuration.
*
*
* @param getPublicKeyConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPublicKeyConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetPublicKeyConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getPublicKeyConfigAsync(GetPublicKeyConfigRequest getPublicKeyConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a real-time log configuration.
*
*
* To get a real-time log configuration, you can provide the configuration's name or its Amazon Resource Name (ARN).
* You must provide at least one. If you provide both, CloudFront uses the name to identify the real-time log
* configuration to get.
*
*
* @param getRealtimeLogConfigRequest
* @return A Java Future containing the result of the GetRealtimeLogConfig operation returned by the service.
* @sample AmazonCloudFrontAsync.GetRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getRealtimeLogConfigAsync(GetRealtimeLogConfigRequest getRealtimeLogConfigRequest);
/**
*
* Gets a real-time log configuration.
*
*
* To get a real-time log configuration, you can provide the configuration's name or its Amazon Resource Name (ARN).
* You must provide at least one. If you provide both, CloudFront uses the name to identify the real-time log
* configuration to get.
*
*
* @param getRealtimeLogConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRealtimeLogConfig operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getRealtimeLogConfigAsync(GetRealtimeLogConfigRequest getRealtimeLogConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a response headers policy, including metadata (the policy's identifier and the date and time when the policy
* was last modified).
*
*
* To get a response headers policy, you must provide the policy's identifier. If the response headers policy is
* attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the response headers policy is not attached to
* a cache behavior, you can get the identifier using ListResponseHeadersPolicies
.
*
*
* @param getResponseHeadersPolicyRequest
* @return A Java Future containing the result of the GetResponseHeadersPolicy operation returned by the service.
* @sample AmazonCloudFrontAsync.GetResponseHeadersPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getResponseHeadersPolicyAsync(GetResponseHeadersPolicyRequest getResponseHeadersPolicyRequest);
/**
*
* Gets a response headers policy, including metadata (the policy's identifier and the date and time when the policy
* was last modified).
*
*
* To get a response headers policy, you must provide the policy's identifier. If the response headers policy is
* attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the response headers policy is not attached to
* a cache behavior, you can get the identifier using ListResponseHeadersPolicies
.
*
*
* @param getResponseHeadersPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResponseHeadersPolicy operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetResponseHeadersPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getResponseHeadersPolicyAsync(GetResponseHeadersPolicyRequest getResponseHeadersPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a response headers policy configuration.
*
*
* To get a response headers policy configuration, you must provide the policy's identifier. If the response headers
* policy is attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the response headers policy is not attached to
* a cache behavior, you can get the identifier using ListResponseHeadersPolicies
.
*
*
* @param getResponseHeadersPolicyConfigRequest
* @return A Java Future containing the result of the GetResponseHeadersPolicyConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetResponseHeadersPolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getResponseHeadersPolicyConfigAsync(
GetResponseHeadersPolicyConfigRequest getResponseHeadersPolicyConfigRequest);
/**
*
* Gets a response headers policy configuration.
*
*
* To get a response headers policy configuration, you must provide the policy's identifier. If the response headers
* policy is attached to a distribution's cache behavior, you can get the policy's identifier using
* ListDistributions
or GetDistribution
. If the response headers policy is not attached to
* a cache behavior, you can get the identifier using ListResponseHeadersPolicies
.
*
*
* @param getResponseHeadersPolicyConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResponseHeadersPolicyConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetResponseHeadersPolicyConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getResponseHeadersPolicyConfigAsync(
GetResponseHeadersPolicyConfigRequest getResponseHeadersPolicyConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a specified RTMP distribution, including the distribution configuration.
*
*
* @param getStreamingDistributionRequest
* The request to get a streaming distribution's information.
* @return A Java Future containing the result of the GetStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsync.GetStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionAsync(GetStreamingDistributionRequest getStreamingDistributionRequest);
/**
*
* Gets information about a specified RTMP distribution, including the distribution configuration.
*
*
* @param getStreamingDistributionRequest
* The request to get a streaming distribution's information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStreamingDistribution operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.GetStreamingDistribution
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionAsync(GetStreamingDistributionRequest getStreamingDistributionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the configuration information about a streaming distribution.
*
*
* @param getStreamingDistributionConfigRequest
* To request to get a streaming distribution configuration.
* @return A Java Future containing the result of the GetStreamingDistributionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.GetStreamingDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionConfigAsync(
GetStreamingDistributionConfigRequest getStreamingDistributionConfigRequest);
/**
*
* Get the configuration information about a streaming distribution.
*
*
* @param getStreamingDistributionConfigRequest
* To request to get a streaming distribution configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStreamingDistributionConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.GetStreamingDistributionConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future getStreamingDistributionConfigAsync(
GetStreamingDistributionConfigRequest getStreamingDistributionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of cache policies.
*
*
* You can optionally apply a filter to return only the managed policies created by Amazon Web Services, or only the
* custom policies created in your Amazon Web Services account.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listCachePoliciesRequest
* @return A Java Future containing the result of the ListCachePolicies operation returned by the service.
* @sample AmazonCloudFrontAsync.ListCachePolicies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCachePoliciesAsync(ListCachePoliciesRequest listCachePoliciesRequest);
/**
*
* Gets a list of cache policies.
*
*
* You can optionally apply a filter to return only the managed policies created by Amazon Web Services, or only the
* custom policies created in your Amazon Web Services account.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listCachePoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCachePolicies operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListCachePolicies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCachePoliciesAsync(ListCachePoliciesRequest listCachePoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists origin access identities.
*
*
* @param listCloudFrontOriginAccessIdentitiesRequest
* The request to list origin access identities.
* @return A Java Future containing the result of the ListCloudFrontOriginAccessIdentities operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListCloudFrontOriginAccessIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listCloudFrontOriginAccessIdentitiesAsync(
ListCloudFrontOriginAccessIdentitiesRequest listCloudFrontOriginAccessIdentitiesRequest);
/**
*
* Lists origin access identities.
*
*
* @param listCloudFrontOriginAccessIdentitiesRequest
* The request to list origin access identities.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCloudFrontOriginAccessIdentities operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListCloudFrontOriginAccessIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listCloudFrontOriginAccessIdentitiesAsync(
ListCloudFrontOriginAccessIdentitiesRequest listCloudFrontOriginAccessIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of aliases (also called CNAMEs or alternate domain names) that conflict or overlap with the provided
* alias, and the associated CloudFront distributions and Amazon Web Services accounts for each conflicting alias.
* In the returned list, the distribution and account IDs are partially hidden, which allows you to identify the
* distributions and accounts that you own, but helps to protect the information of ones that you don't own.
*
*
* Use this operation to find aliases that are in use in CloudFront that conflict or overlap with the provided
* alias. For example, if you provide www.example.com
as input, the returned list can include
* www.example.com
and the overlapping wildcard alternate domain name (*.example.com
), if
* they exist. If you provide *.example.com
as input, the returned list can include
* *.example.com
and any alternate domain names covered by that wildcard (for example,
* www.example.com
, test.example.com
, dev.example.com
, and so on), if they
* exist.
*
*
* To list conflicting aliases, you provide the alias to search and the ID of a distribution in your account that
* has an attached SSL/TLS certificate that includes the provided alias. For more information, including how to set
* up the distribution and certificate, see Moving an alternate domain name to a different distribution in the Amazon CloudFront Developer Guide.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listConflictingAliasesRequest
* @return A Java Future containing the result of the ListConflictingAliases operation returned by the service.
* @sample AmazonCloudFrontAsync.ListConflictingAliases
* @see AWS API Documentation
*/
java.util.concurrent.Future listConflictingAliasesAsync(ListConflictingAliasesRequest listConflictingAliasesRequest);
/**
*
* Gets a list of aliases (also called CNAMEs or alternate domain names) that conflict or overlap with the provided
* alias, and the associated CloudFront distributions and Amazon Web Services accounts for each conflicting alias.
* In the returned list, the distribution and account IDs are partially hidden, which allows you to identify the
* distributions and accounts that you own, but helps to protect the information of ones that you don't own.
*
*
* Use this operation to find aliases that are in use in CloudFront that conflict or overlap with the provided
* alias. For example, if you provide www.example.com
as input, the returned list can include
* www.example.com
and the overlapping wildcard alternate domain name (*.example.com
), if
* they exist. If you provide *.example.com
as input, the returned list can include
* *.example.com
and any alternate domain names covered by that wildcard (for example,
* www.example.com
, test.example.com
, dev.example.com
, and so on), if they
* exist.
*
*
* To list conflicting aliases, you provide the alias to search and the ID of a distribution in your account that
* has an attached SSL/TLS certificate that includes the provided alias. For more information, including how to set
* up the distribution and certificate, see Moving an alternate domain name to a different distribution in the Amazon CloudFront Developer Guide.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listConflictingAliasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConflictingAliases operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListConflictingAliases
* @see AWS API Documentation
*/
java.util.concurrent.Future listConflictingAliasesAsync(ListConflictingAliasesRequest listConflictingAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of the continuous deployment policies in your Amazon Web Services account.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listContinuousDeploymentPoliciesRequest
* @return A Java Future containing the result of the ListContinuousDeploymentPolicies operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListContinuousDeploymentPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listContinuousDeploymentPoliciesAsync(
ListContinuousDeploymentPoliciesRequest listContinuousDeploymentPoliciesRequest);
/**
*
* Gets a list of the continuous deployment policies in your Amazon Web Services account.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listContinuousDeploymentPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContinuousDeploymentPolicies operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListContinuousDeploymentPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listContinuousDeploymentPoliciesAsync(
ListContinuousDeploymentPoliciesRequest listContinuousDeploymentPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List CloudFront distributions.
*
*
* @param listDistributionsRequest
* The request to list your distributions.
* @return A Java Future containing the result of the ListDistributions operation returned by the service.
* @sample AmazonCloudFrontAsync.ListDistributions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDistributionsAsync(ListDistributionsRequest listDistributionsRequest);
/**
*
* List CloudFront distributions.
*
*
* @param listDistributionsRequest
* The request to list your distributions.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributions operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDistributionsAsync(ListDistributionsRequest listDistributionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that's associated with the specified
* cache policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByCachePolicyIdRequest
* @return A Java Future containing the result of the ListDistributionsByCachePolicyId operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListDistributionsByCachePolicyId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByCachePolicyIdAsync(
ListDistributionsByCachePolicyIdRequest listDistributionsByCachePolicyIdRequest);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that's associated with the specified
* cache policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByCachePolicyIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByCachePolicyId operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByCachePolicyId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByCachePolicyIdAsync(
ListDistributionsByCachePolicyIdRequest listDistributionsByCachePolicyIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that references the specified key
* group.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByKeyGroupRequest
* @return A Java Future containing the result of the ListDistributionsByKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsync.ListDistributionsByKeyGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByKeyGroupAsync(
ListDistributionsByKeyGroupRequest listDistributionsByKeyGroupRequest);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that references the specified key
* group.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByKeyGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByKeyGroup operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByKeyGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByKeyGroupAsync(
ListDistributionsByKeyGroupRequest listDistributionsByKeyGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that's associated with the specified
* origin request policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByOriginRequestPolicyIdRequest
* @return A Java Future containing the result of the ListDistributionsByOriginRequestPolicyId operation returned by
* the service.
* @sample AmazonCloudFrontAsync.ListDistributionsByOriginRequestPolicyId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByOriginRequestPolicyIdAsync(
ListDistributionsByOriginRequestPolicyIdRequest listDistributionsByOriginRequestPolicyIdRequest);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that's associated with the specified
* origin request policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByOriginRequestPolicyIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByOriginRequestPolicyId operation returned by
* the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByOriginRequestPolicyId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByOriginRequestPolicyIdAsync(
ListDistributionsByOriginRequestPolicyIdRequest listDistributionsByOriginRequestPolicyIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of distributions that have a cache behavior that's associated with the specified real-time log
* configuration.
*
*
* You can specify the real-time log configuration by its name or its Amazon Resource Name (ARN). You must provide
* at least one. If you provide both, CloudFront uses the name to identify the real-time log configuration to list
* distributions for.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByRealtimeLogConfigRequest
* @return A Java Future containing the result of the ListDistributionsByRealtimeLogConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListDistributionsByRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByRealtimeLogConfigAsync(
ListDistributionsByRealtimeLogConfigRequest listDistributionsByRealtimeLogConfigRequest);
/**
*
* Gets a list of distributions that have a cache behavior that's associated with the specified real-time log
* configuration.
*
*
* You can specify the real-time log configuration by its name or its Amazon Resource Name (ARN). You must provide
* at least one. If you provide both, CloudFront uses the name to identify the real-time log configuration to list
* distributions for.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByRealtimeLogConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByRealtimeLogConfig operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByRealtimeLogConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByRealtimeLogConfigAsync(
ListDistributionsByRealtimeLogConfigRequest listDistributionsByRealtimeLogConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that's associated with the specified
* response headers policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByResponseHeadersPolicyIdRequest
* @return A Java Future containing the result of the ListDistributionsByResponseHeadersPolicyId operation returned
* by the service.
* @sample AmazonCloudFrontAsync.ListDistributionsByResponseHeadersPolicyId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByResponseHeadersPolicyIdAsync(
ListDistributionsByResponseHeadersPolicyIdRequest listDistributionsByResponseHeadersPolicyIdRequest);
/**
*
* Gets a list of distribution IDs for distributions that have a cache behavior that's associated with the specified
* response headers policy.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listDistributionsByResponseHeadersPolicyIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByResponseHeadersPolicyId operation returned
* by the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByResponseHeadersPolicyId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByResponseHeadersPolicyIdAsync(
ListDistributionsByResponseHeadersPolicyIdRequest listDistributionsByResponseHeadersPolicyIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the distributions that are associated with a specified WAF web ACL.
*
*
* @param listDistributionsByWebACLIdRequest
* The request to list distributions that are associated with a specified WAF web ACL.
* @return A Java Future containing the result of the ListDistributionsByWebACLId operation returned by the service.
* @sample AmazonCloudFrontAsync.ListDistributionsByWebACLId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByWebACLIdAsync(
ListDistributionsByWebACLIdRequest listDistributionsByWebACLIdRequest);
/**
*
* List the distributions that are associated with a specified WAF web ACL.
*
*
* @param listDistributionsByWebACLIdRequest
* The request to list distributions that are associated with a specified WAF web ACL.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDistributionsByWebACLId operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListDistributionsByWebACLId
* @see AWS API Documentation
*/
java.util.concurrent.Future listDistributionsByWebACLIdAsync(
ListDistributionsByWebACLIdRequest listDistributionsByWebACLIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all field-level encryption configurations that have been created in CloudFront for this account.
*
*
* @param listFieldLevelEncryptionConfigsRequest
* @return A Java Future containing the result of the ListFieldLevelEncryptionConfigs operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListFieldLevelEncryptionConfigs
* @see AWS API Documentation
*/
java.util.concurrent.Future listFieldLevelEncryptionConfigsAsync(
ListFieldLevelEncryptionConfigsRequest listFieldLevelEncryptionConfigsRequest);
/**
*
* List all field-level encryption configurations that have been created in CloudFront for this account.
*
*
* @param listFieldLevelEncryptionConfigsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFieldLevelEncryptionConfigs operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListFieldLevelEncryptionConfigs
* @see AWS API Documentation
*/
java.util.concurrent.Future listFieldLevelEncryptionConfigsAsync(
ListFieldLevelEncryptionConfigsRequest listFieldLevelEncryptionConfigsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Request a list of field-level encryption profiles that have been created in CloudFront for this account.
*
*
* @param listFieldLevelEncryptionProfilesRequest
* @return A Java Future containing the result of the ListFieldLevelEncryptionProfiles operation returned by the
* service.
* @sample AmazonCloudFrontAsync.ListFieldLevelEncryptionProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listFieldLevelEncryptionProfilesAsync(
ListFieldLevelEncryptionProfilesRequest listFieldLevelEncryptionProfilesRequest);
/**
*
* Request a list of field-level encryption profiles that have been created in CloudFront for this account.
*
*
* @param listFieldLevelEncryptionProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFieldLevelEncryptionProfiles operation returned by the
* service.
* @sample AmazonCloudFrontAsyncHandler.ListFieldLevelEncryptionProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listFieldLevelEncryptionProfilesAsync(
ListFieldLevelEncryptionProfilesRequest listFieldLevelEncryptionProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of all CloudFront functions in your Amazon Web Services account.
*
*
* You can optionally apply a filter to return only the functions that are in the specified stage, either
* DEVELOPMENT
or LIVE
.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listFunctionsRequest
* @return A Java Future containing the result of the ListFunctions operation returned by the service.
* @sample AmazonCloudFrontAsync.ListFunctions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFunctionsAsync(ListFunctionsRequest listFunctionsRequest);
/**
*
* Gets a list of all CloudFront functions in your Amazon Web Services account.
*
*
* You can optionally apply a filter to return only the functions that are in the specified stage, either
* DEVELOPMENT
or LIVE
.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listFunctionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFunctions operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListFunctions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFunctionsAsync(ListFunctionsRequest listFunctionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists invalidation batches.
*
*
* @param listInvalidationsRequest
* The request to list invalidations.
* @return A Java Future containing the result of the ListInvalidations operation returned by the service.
* @sample AmazonCloudFrontAsync.ListInvalidations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listInvalidationsAsync(ListInvalidationsRequest listInvalidationsRequest);
/**
*
* Lists invalidation batches.
*
*
* @param listInvalidationsRequest
* The request to list invalidations.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInvalidations operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListInvalidations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listInvalidationsAsync(ListInvalidationsRequest listInvalidationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of key groups.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listKeyGroupsRequest
* @return A Java Future containing the result of the ListKeyGroups operation returned by the service.
* @sample AmazonCloudFrontAsync.ListKeyGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listKeyGroupsAsync(ListKeyGroupsRequest listKeyGroupsRequest);
/**
*
* Gets a list of key groups.
*
*
* You can optionally specify the maximum number of items to receive in the response. If the total number of items
* in the list exceeds the maximum that you specify, or the default maximum, the response is paginated. To get the
* next page of items, send a subsequent request that specifies the NextMarker
value from the current
* response as the Marker
value in the subsequent request.
*
*
* @param listKeyGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListKeyGroups operation returned by the service.
* @sample AmazonCloudFrontAsyncHandler.ListKeyGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listKeyGroupsAsync(ListKeyGroupsRequest listKeyGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies the key value stores to list.
*
*
* @param listKeyValueStoresRequest
* @return A Java Future containing the result of the ListKeyValueStores operation returned by the service.
* @sample AmazonCloudFrontAsync.ListKeyValueStores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listKeyValueStoresAsync(ListKeyValueStoresRequest listKeyValueStoresRequest);
/**
*