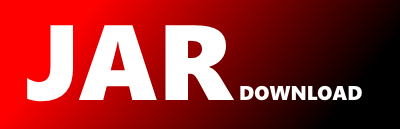
com.amazonaws.services.cloudfront.model.CustomOriginConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A custom origin. A custom origin is any origin that is not an Amazon S3 bucket, with one exception. An Amazon
* S3 bucket that is configured with
* static website hosting is a custom origin.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CustomOriginConfig implements Serializable, Cloneable {
/**
*
* The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin listens on.
*
*/
private Integer hTTPPort;
/**
*
* The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin listens on.
*
*/
private Integer hTTPSPort;
/**
*
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values are:
*
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used to
* connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
*
*/
private String originProtocolPolicy;
/**
*
* Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS. Valid
* values include SSLv3
, TLSv1
, TLSv1.1
, and TLSv1.2
.
*
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
*
*/
private OriginSslProtocols originSslProtocols;
/**
*
* Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as the
* origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the default (if
* you don't specify otherwise) is 30 seconds.
*
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
*
*/
private Integer originReadTimeout;
/**
*
* Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is 1
* second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
*
*/
private Integer originKeepaliveTimeout;
/**
*
* The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin listens on.
*
*
* @param hTTPPort
* The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin listens
* on.
*/
public void setHTTPPort(Integer hTTPPort) {
this.hTTPPort = hTTPPort;
}
/**
*
* The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin listens on.
*
*
* @return The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin
* listens on.
*/
public Integer getHTTPPort() {
return this.hTTPPort;
}
/**
*
* The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin listens on.
*
*
* @param hTTPPort
* The HTTP port that CloudFront uses to connect to the origin. Specify the HTTP port that the origin listens
* on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomOriginConfig withHTTPPort(Integer hTTPPort) {
setHTTPPort(hTTPPort);
return this;
}
/**
*
* The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin listens on.
*
*
* @param hTTPSPort
* The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin
* listens on.
*/
public void setHTTPSPort(Integer hTTPSPort) {
this.hTTPSPort = hTTPSPort;
}
/**
*
* The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin listens on.
*
*
* @return The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin
* listens on.
*/
public Integer getHTTPSPort() {
return this.hTTPSPort;
}
/**
*
* The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin listens on.
*
*
* @param hTTPSPort
* The HTTPS port that CloudFront uses to connect to the origin. Specify the HTTPS port that the origin
* listens on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomOriginConfig withHTTPSPort(Integer hTTPSPort) {
setHTTPSPort(hTTPSPort);
return this;
}
/**
*
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values are:
*
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used to
* connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
*
*
* @param originProtocolPolicy
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values
* are:
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used
* to connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
* @see OriginProtocolPolicy
*/
public void setOriginProtocolPolicy(String originProtocolPolicy) {
this.originProtocolPolicy = originProtocolPolicy;
}
/**
*
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values are:
*
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used to
* connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
*
*
* @return Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values
* are:
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer
* used to connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
* @see OriginProtocolPolicy
*/
public String getOriginProtocolPolicy() {
return this.originProtocolPolicy;
}
/**
*
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values are:
*
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used to
* connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
*
*
* @param originProtocolPolicy
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values
* are:
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used
* to connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginProtocolPolicy
*/
public CustomOriginConfig withOriginProtocolPolicy(String originProtocolPolicy) {
setOriginProtocolPolicy(originProtocolPolicy);
return this;
}
/**
*
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values are:
*
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used to
* connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
*
*
* @param originProtocolPolicy
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values
* are:
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used
* to connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
* @see OriginProtocolPolicy
*/
public void setOriginProtocolPolicy(OriginProtocolPolicy originProtocolPolicy) {
withOriginProtocolPolicy(originProtocolPolicy);
}
/**
*
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values are:
*
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used to
* connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
*
*
* @param originProtocolPolicy
* Specifies the protocol (HTTP or HTTPS) that CloudFront uses to connect to the origin. Valid values
* are:
*
* -
*
* http-only
– CloudFront always uses HTTP to connect to the origin.
*
*
* -
*
* match-viewer
– CloudFront connects to the origin using the same protocol that the viewer used
* to connect to CloudFront.
*
*
* -
*
* https-only
– CloudFront always uses HTTPS to connect to the origin.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginProtocolPolicy
*/
public CustomOriginConfig withOriginProtocolPolicy(OriginProtocolPolicy originProtocolPolicy) {
this.originProtocolPolicy = originProtocolPolicy.toString();
return this;
}
/**
*
* Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS. Valid
* values include SSLv3
, TLSv1
, TLSv1.1
, and TLSv1.2
.
*
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
*
*
* @param originSslProtocols
* Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS.
* Valid values include SSLv3
, TLSv1
, TLSv1.1
, and
* TLSv1.2
.
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
*/
public void setOriginSslProtocols(OriginSslProtocols originSslProtocols) {
this.originSslProtocols = originSslProtocols;
}
/**
*
* Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS. Valid
* values include SSLv3
, TLSv1
, TLSv1.1
, and TLSv1.2
.
*
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
*
*
* @return Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS.
* Valid values include SSLv3
, TLSv1
, TLSv1.1
, and
* TLSv1.2
.
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
*/
public OriginSslProtocols getOriginSslProtocols() {
return this.originSslProtocols;
}
/**
*
* Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS. Valid
* values include SSLv3
, TLSv1
, TLSv1.1
, and TLSv1.2
.
*
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
*
*
* @param originSslProtocols
* Specifies the minimum SSL/TLS protocol that CloudFront uses when connecting to your origin over HTTPS.
* Valid values include SSLv3
, TLSv1
, TLSv1.1
, and
* TLSv1.2
.
*
* For more information, see Minimum Origin SSL Protocol in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomOriginConfig withOriginSslProtocols(OriginSslProtocols originSslProtocols) {
setOriginSslProtocols(originSslProtocols);
return this;
}
/**
*
* Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as the
* origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the default (if
* you don't specify otherwise) is 30 seconds.
*
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
*
*
* @param originReadTimeout
* Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as the
* origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the
* default (if you don't specify otherwise) is 30 seconds.
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
*/
public void setOriginReadTimeout(Integer originReadTimeout) {
this.originReadTimeout = originReadTimeout;
}
/**
*
* Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as the
* origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the default (if
* you don't specify otherwise) is 30 seconds.
*
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
*
*
* @return Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as
* the origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the
* default (if you don't specify otherwise) is 30 seconds.
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
*/
public Integer getOriginReadTimeout() {
return this.originReadTimeout;
}
/**
*
* Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as the
* origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the default (if
* you don't specify otherwise) is 30 seconds.
*
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
*
*
* @param originReadTimeout
* Specifies how long, in seconds, CloudFront waits for a response from the origin. This is also known as the
* origin response timeout. The minimum timeout is 1 second, the maximum is 60 seconds, and the
* default (if you don't specify otherwise) is 30 seconds.
*
* For more information, see Origin Response Timeout in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomOriginConfig withOriginReadTimeout(Integer originReadTimeout) {
setOriginReadTimeout(originReadTimeout);
return this;
}
/**
*
* Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is 1
* second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
*
*
* @param originKeepaliveTimeout
* Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is 1
* second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
*/
public void setOriginKeepaliveTimeout(Integer originKeepaliveTimeout) {
this.originKeepaliveTimeout = originKeepaliveTimeout;
}
/**
*
* Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is 1
* second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
*
*
* @return Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is
* 1 second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
*/
public Integer getOriginKeepaliveTimeout() {
return this.originKeepaliveTimeout;
}
/**
*
* Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is 1
* second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
*
*
* @param originKeepaliveTimeout
* Specifies how long, in seconds, CloudFront persists its connection to the origin. The minimum timeout is 1
* second, the maximum is 60 seconds, and the default (if you don't specify otherwise) is 5 seconds.
*
* For more information, see Origin Keep-alive Timeout in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomOriginConfig withOriginKeepaliveTimeout(Integer originKeepaliveTimeout) {
setOriginKeepaliveTimeout(originKeepaliveTimeout);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getHTTPPort() != null)
sb.append("HTTPPort: ").append(getHTTPPort()).append(",");
if (getHTTPSPort() != null)
sb.append("HTTPSPort: ").append(getHTTPSPort()).append(",");
if (getOriginProtocolPolicy() != null)
sb.append("OriginProtocolPolicy: ").append(getOriginProtocolPolicy()).append(",");
if (getOriginSslProtocols() != null)
sb.append("OriginSslProtocols: ").append(getOriginSslProtocols()).append(",");
if (getOriginReadTimeout() != null)
sb.append("OriginReadTimeout: ").append(getOriginReadTimeout()).append(",");
if (getOriginKeepaliveTimeout() != null)
sb.append("OriginKeepaliveTimeout: ").append(getOriginKeepaliveTimeout());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CustomOriginConfig == false)
return false;
CustomOriginConfig other = (CustomOriginConfig) obj;
if (other.getHTTPPort() == null ^ this.getHTTPPort() == null)
return false;
if (other.getHTTPPort() != null && other.getHTTPPort().equals(this.getHTTPPort()) == false)
return false;
if (other.getHTTPSPort() == null ^ this.getHTTPSPort() == null)
return false;
if (other.getHTTPSPort() != null && other.getHTTPSPort().equals(this.getHTTPSPort()) == false)
return false;
if (other.getOriginProtocolPolicy() == null ^ this.getOriginProtocolPolicy() == null)
return false;
if (other.getOriginProtocolPolicy() != null && other.getOriginProtocolPolicy().equals(this.getOriginProtocolPolicy()) == false)
return false;
if (other.getOriginSslProtocols() == null ^ this.getOriginSslProtocols() == null)
return false;
if (other.getOriginSslProtocols() != null && other.getOriginSslProtocols().equals(this.getOriginSslProtocols()) == false)
return false;
if (other.getOriginReadTimeout() == null ^ this.getOriginReadTimeout() == null)
return false;
if (other.getOriginReadTimeout() != null && other.getOriginReadTimeout().equals(this.getOriginReadTimeout()) == false)
return false;
if (other.getOriginKeepaliveTimeout() == null ^ this.getOriginKeepaliveTimeout() == null)
return false;
if (other.getOriginKeepaliveTimeout() != null && other.getOriginKeepaliveTimeout().equals(this.getOriginKeepaliveTimeout()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getHTTPPort() == null) ? 0 : getHTTPPort().hashCode());
hashCode = prime * hashCode + ((getHTTPSPort() == null) ? 0 : getHTTPSPort().hashCode());
hashCode = prime * hashCode + ((getOriginProtocolPolicy() == null) ? 0 : getOriginProtocolPolicy().hashCode());
hashCode = prime * hashCode + ((getOriginSslProtocols() == null) ? 0 : getOriginSslProtocols().hashCode());
hashCode = prime * hashCode + ((getOriginReadTimeout() == null) ? 0 : getOriginReadTimeout().hashCode());
hashCode = prime * hashCode + ((getOriginKeepaliveTimeout() == null) ? 0 : getOriginKeepaliveTimeout().hashCode());
return hashCode;
}
@Override
public CustomOriginConfig clone() {
try {
return (CustomOriginConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}