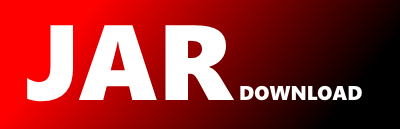
com.amazonaws.services.cloudfront.model.OriginRequestPolicyCookiesConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* An object that determines whether any cookies in viewer requests (and if so, which cookies) are included in requests
* that CloudFront sends to the origin.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OriginRequestPolicyCookiesConfig implements Serializable, Cloneable {
/**
*
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the origin. Valid
* values are:
*
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the origin.
* Even when this field is set to none
, any cookies that are listed in a CachePolicy
* are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the CookieNames
type
* are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin, except for those listed in the CookieNames
type, which are not included.
*
*
*
*/
private String cookieBehavior;
private CookieNames cookies;
/**
*
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the origin. Valid
* values are:
*
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the origin.
* Even when this field is set to none
, any cookies that are listed in a CachePolicy
* are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the CookieNames
type
* are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin, except for those listed in the CookieNames
type, which are not included.
*
*
*
*
* @param cookieBehavior
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Valid values are:
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Even when this field is set to none
, any cookies that are listed in a
* CachePolicy
are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the
* CookieNames
type are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to
* the origin, except for those listed in the CookieNames
type, which are not
* included.
*
*
* @see OriginRequestPolicyCookieBehavior
*/
public void setCookieBehavior(String cookieBehavior) {
this.cookieBehavior = cookieBehavior;
}
/**
*
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the origin. Valid
* values are:
*
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the origin.
* Even when this field is set to none
, any cookies that are listed in a CachePolicy
* are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the CookieNames
type
* are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin, except for those listed in the CookieNames
type, which are not included.
*
*
*
*
* @return Determines whether cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Valid values are:
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Even when this field is set to none
, any cookies that are listed in a
* CachePolicy
are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the
* CookieNames
type are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to
* the origin, except for those listed in the CookieNames
type, which are not
* included.
*
*
* @see OriginRequestPolicyCookieBehavior
*/
public String getCookieBehavior() {
return this.cookieBehavior;
}
/**
*
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the origin. Valid
* values are:
*
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the origin.
* Even when this field is set to none
, any cookies that are listed in a CachePolicy
* are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the CookieNames
type
* are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin, except for those listed in the CookieNames
type, which are not included.
*
*
*
*
* @param cookieBehavior
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Valid values are:
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Even when this field is set to none
, any cookies that are listed in a
* CachePolicy
are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the
* CookieNames
type are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to
* the origin, except for those listed in the CookieNames
type, which are not
* included.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginRequestPolicyCookieBehavior
*/
public OriginRequestPolicyCookiesConfig withCookieBehavior(String cookieBehavior) {
setCookieBehavior(cookieBehavior);
return this;
}
/**
*
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the origin. Valid
* values are:
*
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the origin.
* Even when this field is set to none
, any cookies that are listed in a CachePolicy
* are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the CookieNames
type
* are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin, except for those listed in the CookieNames
type, which are not included.
*
*
*
*
* @param cookieBehavior
* Determines whether cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Valid values are:
*
* -
*
* none
– No cookies in viewer requests are included in requests that CloudFront sends to the
* origin. Even when this field is set to none
, any cookies that are listed in a
* CachePolicy
are included in origin requests.
*
*
* -
*
* whitelist
– Only the cookies in viewer requests that are listed in the
* CookieNames
type are included in requests that CloudFront sends to the origin.
*
*
* -
*
* all
– All cookies in viewer requests are included in requests that CloudFront sends to the
* origin.
*
*
* -
*
* allExcept
– All cookies in viewer requests are included in requests that CloudFront sends to
* the origin, except for those listed in the CookieNames
type, which are not
* included.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginRequestPolicyCookieBehavior
*/
public OriginRequestPolicyCookiesConfig withCookieBehavior(OriginRequestPolicyCookieBehavior cookieBehavior) {
this.cookieBehavior = cookieBehavior.toString();
return this;
}
/**
* @param cookies
*/
public void setCookies(CookieNames cookies) {
this.cookies = cookies;
}
/**
* @return
*/
public CookieNames getCookies() {
return this.cookies;
}
/**
* @param cookies
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginRequestPolicyCookiesConfig withCookies(CookieNames cookies) {
setCookies(cookies);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCookieBehavior() != null)
sb.append("CookieBehavior: ").append(getCookieBehavior()).append(",");
if (getCookies() != null)
sb.append("Cookies: ").append(getCookies());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OriginRequestPolicyCookiesConfig == false)
return false;
OriginRequestPolicyCookiesConfig other = (OriginRequestPolicyCookiesConfig) obj;
if (other.getCookieBehavior() == null ^ this.getCookieBehavior() == null)
return false;
if (other.getCookieBehavior() != null && other.getCookieBehavior().equals(this.getCookieBehavior()) == false)
return false;
if (other.getCookies() == null ^ this.getCookies() == null)
return false;
if (other.getCookies() != null && other.getCookies().equals(this.getCookies()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCookieBehavior() == null) ? 0 : getCookieBehavior().hashCode());
hashCode = prime * hashCode + ((getCookies() == null) ? 0 : getCookies().hashCode());
return hashCode;
}
@Override
public OriginRequestPolicyCookiesConfig clone() {
try {
return (OriginRequestPolicyCookiesConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}