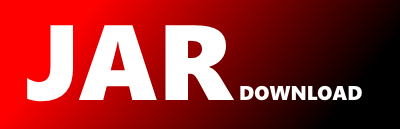
com.amazonaws.services.cloudfront.model.ResponseHeadersPolicyCorsConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A configuration for a set of HTTP response headers that are used for cross-origin resource sharing (CORS). CloudFront
* adds these headers to HTTP responses that it sends for CORS requests that match a cache behavior associated with this
* response headers policy.
*
*
* For more information about CORS, see Cross-Origin
* Resource Sharing (CORS) in the MDN Web Docs.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResponseHeadersPolicyCorsConfig implements Serializable, Cloneable {
/**
*
* A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control-Allow-Origin in the MDN Web Docs.
*
*/
private ResponseHeadersPolicyAccessControlAllowOrigins accessControlAllowOrigins;
/**
*
* A list of HTTP header names that CloudFront includes as values for the Access-Control-Allow-Headers
* HTTP response header.
*
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control-Allow-Headers in the MDN Web Docs.
*
*/
private ResponseHeadersPolicyAccessControlAllowHeaders accessControlAllowHeaders;
/**
*
* A list of HTTP methods that CloudFront includes as values for the Access-Control-Allow-Methods
HTTP
* response header.
*
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control-Allow-Methods in the MDN Web Docs.
*
*/
private ResponseHeadersPolicyAccessControlAllowMethods accessControlAllowMethods;
/**
*
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP response
* header.
*
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-Control-Allow-Credentials in the MDN Web Docs.
*
*/
private Boolean accessControlAllowCredentials;
/**
*
* A list of HTTP headers that CloudFront includes as values for the Access-Control-Expose-Headers
HTTP
* response header.
*
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-Control-Expose-Headers in the MDN Web Docs.
*
*/
private ResponseHeadersPolicyAccessControlExposeHeaders accessControlExposeHeaders;
/**
*
* A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response header.
*
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
*
*/
private Integer accessControlMaxAgeSec;
/**
*
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with the
* ones specified in this response headers policy.
*
*/
private Boolean originOverride;
/**
*
* A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control-Allow-Origin in the MDN Web Docs.
*
*
* @param accessControlAllowOrigins
* A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control-Allow-Origin in the MDN Web Docs.
*/
public void setAccessControlAllowOrigins(ResponseHeadersPolicyAccessControlAllowOrigins accessControlAllowOrigins) {
this.accessControlAllowOrigins = accessControlAllowOrigins;
}
/**
*
* A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control-Allow-Origin in the MDN Web Docs.
*
*
* @return A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control
* -Allow-Origin in the MDN Web Docs.
*/
public ResponseHeadersPolicyAccessControlAllowOrigins getAccessControlAllowOrigins() {
return this.accessControlAllowOrigins;
}
/**
*
* A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control-Allow-Origin in the MDN Web Docs.
*
*
* @param accessControlAllowOrigins
* A list of origins (domain names) that CloudFront can use as the value for the
* Access-Control-Allow-Origin
HTTP response header.
*
* For more information about the Access-Control-Allow-Origin
HTTP response header, see Access-Control-Allow-Origin in the MDN Web Docs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withAccessControlAllowOrigins(ResponseHeadersPolicyAccessControlAllowOrigins accessControlAllowOrigins) {
setAccessControlAllowOrigins(accessControlAllowOrigins);
return this;
}
/**
*
* A list of HTTP header names that CloudFront includes as values for the Access-Control-Allow-Headers
* HTTP response header.
*
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control-Allow-Headers in the MDN Web Docs.
*
*
* @param accessControlAllowHeaders
* A list of HTTP header names that CloudFront includes as values for the
* Access-Control-Allow-Headers
HTTP response header.
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control
* -Allow-Headers in the MDN Web Docs.
*/
public void setAccessControlAllowHeaders(ResponseHeadersPolicyAccessControlAllowHeaders accessControlAllowHeaders) {
this.accessControlAllowHeaders = accessControlAllowHeaders;
}
/**
*
* A list of HTTP header names that CloudFront includes as values for the Access-Control-Allow-Headers
* HTTP response header.
*
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control-Allow-Headers in the MDN Web Docs.
*
*
* @return A list of HTTP header names that CloudFront includes as values for the
* Access-Control-Allow-Headers
HTTP response header.
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control
* -Allow-Headers in the MDN Web Docs.
*/
public ResponseHeadersPolicyAccessControlAllowHeaders getAccessControlAllowHeaders() {
return this.accessControlAllowHeaders;
}
/**
*
* A list of HTTP header names that CloudFront includes as values for the Access-Control-Allow-Headers
* HTTP response header.
*
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control-Allow-Headers in the MDN Web Docs.
*
*
* @param accessControlAllowHeaders
* A list of HTTP header names that CloudFront includes as values for the
* Access-Control-Allow-Headers
HTTP response header.
*
* For more information about the Access-Control-Allow-Headers
HTTP response header, see Access-Control
* -Allow-Headers in the MDN Web Docs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withAccessControlAllowHeaders(ResponseHeadersPolicyAccessControlAllowHeaders accessControlAllowHeaders) {
setAccessControlAllowHeaders(accessControlAllowHeaders);
return this;
}
/**
*
* A list of HTTP methods that CloudFront includes as values for the Access-Control-Allow-Methods
HTTP
* response header.
*
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control-Allow-Methods in the MDN Web Docs.
*
*
* @param accessControlAllowMethods
* A list of HTTP methods that CloudFront includes as values for the
* Access-Control-Allow-Methods
HTTP response header.
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control
* -Allow-Methods in the MDN Web Docs.
*/
public void setAccessControlAllowMethods(ResponseHeadersPolicyAccessControlAllowMethods accessControlAllowMethods) {
this.accessControlAllowMethods = accessControlAllowMethods;
}
/**
*
* A list of HTTP methods that CloudFront includes as values for the Access-Control-Allow-Methods
HTTP
* response header.
*
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control-Allow-Methods in the MDN Web Docs.
*
*
* @return A list of HTTP methods that CloudFront includes as values for the
* Access-Control-Allow-Methods
HTTP response header.
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control
* -Allow-Methods in the MDN Web Docs.
*/
public ResponseHeadersPolicyAccessControlAllowMethods getAccessControlAllowMethods() {
return this.accessControlAllowMethods;
}
/**
*
* A list of HTTP methods that CloudFront includes as values for the Access-Control-Allow-Methods
HTTP
* response header.
*
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control-Allow-Methods in the MDN Web Docs.
*
*
* @param accessControlAllowMethods
* A list of HTTP methods that CloudFront includes as values for the
* Access-Control-Allow-Methods
HTTP response header.
*
* For more information about the Access-Control-Allow-Methods
HTTP response header, see Access-Control
* -Allow-Methods in the MDN Web Docs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withAccessControlAllowMethods(ResponseHeadersPolicyAccessControlAllowMethods accessControlAllowMethods) {
setAccessControlAllowMethods(accessControlAllowMethods);
return this;
}
/**
*
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP response
* header.
*
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-Control-Allow-Credentials in the MDN Web Docs.
*
*
* @param accessControlAllowCredentials
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP
* response header.
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-
* Control-Allow-Credentials in the MDN Web Docs.
*/
public void setAccessControlAllowCredentials(Boolean accessControlAllowCredentials) {
this.accessControlAllowCredentials = accessControlAllowCredentials;
}
/**
*
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP response
* header.
*
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-Control-Allow-Credentials in the MDN Web Docs.
*
*
* @return A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP
* response header.
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-
* Control-Allow-Credentials in the MDN Web Docs.
*/
public Boolean getAccessControlAllowCredentials() {
return this.accessControlAllowCredentials;
}
/**
*
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP response
* header.
*
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-Control-Allow-Credentials in the MDN Web Docs.
*
*
* @param accessControlAllowCredentials
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP
* response header.
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-
* Control-Allow-Credentials in the MDN Web Docs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withAccessControlAllowCredentials(Boolean accessControlAllowCredentials) {
setAccessControlAllowCredentials(accessControlAllowCredentials);
return this;
}
/**
*
* A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP response
* header.
*
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-Control-Allow-Credentials in the MDN Web Docs.
*
*
* @return A Boolean that CloudFront uses as the value for the Access-Control-Allow-Credentials
HTTP
* response header.
*
* For more information about the Access-Control-Allow-Credentials
HTTP response header, see Access-
* Control-Allow-Credentials in the MDN Web Docs.
*/
public Boolean isAccessControlAllowCredentials() {
return this.accessControlAllowCredentials;
}
/**
*
* A list of HTTP headers that CloudFront includes as values for the Access-Control-Expose-Headers
HTTP
* response header.
*
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-Control-Expose-Headers in the MDN Web Docs.
*
*
* @param accessControlExposeHeaders
* A list of HTTP headers that CloudFront includes as values for the
* Access-Control-Expose-Headers
HTTP response header.
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-Control
* -Expose-Headers in the MDN Web Docs.
*/
public void setAccessControlExposeHeaders(ResponseHeadersPolicyAccessControlExposeHeaders accessControlExposeHeaders) {
this.accessControlExposeHeaders = accessControlExposeHeaders;
}
/**
*
* A list of HTTP headers that CloudFront includes as values for the Access-Control-Expose-Headers
HTTP
* response header.
*
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-Control-Expose-Headers in the MDN Web Docs.
*
*
* @return A list of HTTP headers that CloudFront includes as values for the
* Access-Control-Expose-Headers
HTTP response header.
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-
* Control-Expose-Headers in the MDN Web Docs.
*/
public ResponseHeadersPolicyAccessControlExposeHeaders getAccessControlExposeHeaders() {
return this.accessControlExposeHeaders;
}
/**
*
* A list of HTTP headers that CloudFront includes as values for the Access-Control-Expose-Headers
HTTP
* response header.
*
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-Control-Expose-Headers in the MDN Web Docs.
*
*
* @param accessControlExposeHeaders
* A list of HTTP headers that CloudFront includes as values for the
* Access-Control-Expose-Headers
HTTP response header.
*
* For more information about the Access-Control-Expose-Headers
HTTP response header, see Access-Control
* -Expose-Headers in the MDN Web Docs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withAccessControlExposeHeaders(ResponseHeadersPolicyAccessControlExposeHeaders accessControlExposeHeaders) {
setAccessControlExposeHeaders(accessControlExposeHeaders);
return this;
}
/**
*
* A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response header.
*
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
*
*
* @param accessControlMaxAgeSec
* A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response
* header.
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
*/
public void setAccessControlMaxAgeSec(Integer accessControlMaxAgeSec) {
this.accessControlMaxAgeSec = accessControlMaxAgeSec;
}
/**
*
* A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response header.
*
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
*
*
* @return A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response
* header.
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
*/
public Integer getAccessControlMaxAgeSec() {
return this.accessControlMaxAgeSec;
}
/**
*
* A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response header.
*
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
*
*
* @param accessControlMaxAgeSec
* A number that CloudFront uses as the value for the Access-Control-Max-Age
HTTP response
* header.
*
* For more information about the Access-Control-Max-Age
HTTP response header, see Access-Control-Max-Age in the MDN Web Docs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withAccessControlMaxAgeSec(Integer accessControlMaxAgeSec) {
setAccessControlMaxAgeSec(accessControlMaxAgeSec);
return this;
}
/**
*
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with the
* ones specified in this response headers policy.
*
*
* @param originOverride
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with
* the ones specified in this response headers policy.
*/
public void setOriginOverride(Boolean originOverride) {
this.originOverride = originOverride;
}
/**
*
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with the
* ones specified in this response headers policy.
*
*
* @return A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin
* with the ones specified in this response headers policy.
*/
public Boolean getOriginOverride() {
return this.originOverride;
}
/**
*
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with the
* ones specified in this response headers policy.
*
*
* @param originOverride
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with
* the ones specified in this response headers policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResponseHeadersPolicyCorsConfig withOriginOverride(Boolean originOverride) {
setOriginOverride(originOverride);
return this;
}
/**
*
* A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin with the
* ones specified in this response headers policy.
*
*
* @return A Boolean that determines whether CloudFront overrides HTTP response headers received from the origin
* with the ones specified in this response headers policy.
*/
public Boolean isOriginOverride() {
return this.originOverride;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccessControlAllowOrigins() != null)
sb.append("AccessControlAllowOrigins: ").append(getAccessControlAllowOrigins()).append(",");
if (getAccessControlAllowHeaders() != null)
sb.append("AccessControlAllowHeaders: ").append(getAccessControlAllowHeaders()).append(",");
if (getAccessControlAllowMethods() != null)
sb.append("AccessControlAllowMethods: ").append(getAccessControlAllowMethods()).append(",");
if (getAccessControlAllowCredentials() != null)
sb.append("AccessControlAllowCredentials: ").append(getAccessControlAllowCredentials()).append(",");
if (getAccessControlExposeHeaders() != null)
sb.append("AccessControlExposeHeaders: ").append(getAccessControlExposeHeaders()).append(",");
if (getAccessControlMaxAgeSec() != null)
sb.append("AccessControlMaxAgeSec: ").append(getAccessControlMaxAgeSec()).append(",");
if (getOriginOverride() != null)
sb.append("OriginOverride: ").append(getOriginOverride());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResponseHeadersPolicyCorsConfig == false)
return false;
ResponseHeadersPolicyCorsConfig other = (ResponseHeadersPolicyCorsConfig) obj;
if (other.getAccessControlAllowOrigins() == null ^ this.getAccessControlAllowOrigins() == null)
return false;
if (other.getAccessControlAllowOrigins() != null && other.getAccessControlAllowOrigins().equals(this.getAccessControlAllowOrigins()) == false)
return false;
if (other.getAccessControlAllowHeaders() == null ^ this.getAccessControlAllowHeaders() == null)
return false;
if (other.getAccessControlAllowHeaders() != null && other.getAccessControlAllowHeaders().equals(this.getAccessControlAllowHeaders()) == false)
return false;
if (other.getAccessControlAllowMethods() == null ^ this.getAccessControlAllowMethods() == null)
return false;
if (other.getAccessControlAllowMethods() != null && other.getAccessControlAllowMethods().equals(this.getAccessControlAllowMethods()) == false)
return false;
if (other.getAccessControlAllowCredentials() == null ^ this.getAccessControlAllowCredentials() == null)
return false;
if (other.getAccessControlAllowCredentials() != null
&& other.getAccessControlAllowCredentials().equals(this.getAccessControlAllowCredentials()) == false)
return false;
if (other.getAccessControlExposeHeaders() == null ^ this.getAccessControlExposeHeaders() == null)
return false;
if (other.getAccessControlExposeHeaders() != null && other.getAccessControlExposeHeaders().equals(this.getAccessControlExposeHeaders()) == false)
return false;
if (other.getAccessControlMaxAgeSec() == null ^ this.getAccessControlMaxAgeSec() == null)
return false;
if (other.getAccessControlMaxAgeSec() != null && other.getAccessControlMaxAgeSec().equals(this.getAccessControlMaxAgeSec()) == false)
return false;
if (other.getOriginOverride() == null ^ this.getOriginOverride() == null)
return false;
if (other.getOriginOverride() != null && other.getOriginOverride().equals(this.getOriginOverride()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccessControlAllowOrigins() == null) ? 0 : getAccessControlAllowOrigins().hashCode());
hashCode = prime * hashCode + ((getAccessControlAllowHeaders() == null) ? 0 : getAccessControlAllowHeaders().hashCode());
hashCode = prime * hashCode + ((getAccessControlAllowMethods() == null) ? 0 : getAccessControlAllowMethods().hashCode());
hashCode = prime * hashCode + ((getAccessControlAllowCredentials() == null) ? 0 : getAccessControlAllowCredentials().hashCode());
hashCode = prime * hashCode + ((getAccessControlExposeHeaders() == null) ? 0 : getAccessControlExposeHeaders().hashCode());
hashCode = prime * hashCode + ((getAccessControlMaxAgeSec() == null) ? 0 : getAccessControlMaxAgeSec().hashCode());
hashCode = prime * hashCode + ((getOriginOverride() == null) ? 0 : getOriginOverride().hashCode());
return hashCode;
}
@Override
public ResponseHeadersPolicyCorsConfig clone() {
try {
return (ResponseHeadersPolicyCorsConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}