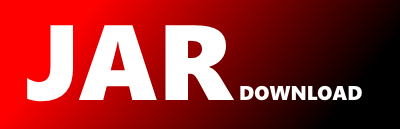
com.amazonaws.services.cloudfront.model.CachePolicyConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudfront Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A cache policy configuration.
*
*
* This configuration determines the following:
*
*
* -
*
* The values that CloudFront includes in the cache key. These values can include HTTP headers, cookies, and URL query
* strings. CloudFront uses the cache key to find an object in its cache that it can return to the viewer.
*
*
* -
*
* The default, minimum, and maximum time to live (TTL) values that you want objects to stay in the CloudFront cache.
*
*
*
*
* The headers, cookies, and query strings that are included in the cache key are automatically included in requests
* that CloudFront sends to the origin. CloudFront sends a request when it can’t find a valid object in its cache that
* matches the request’s cache key. If you want to send values to the origin but not include them in the cache
* key, use OriginRequestPolicy
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CachePolicyConfig implements Serializable, Cloneable {
/**
*
* A comment to describe the cache policy. The comment cannot be longer than 128 characters.
*
*/
private String comment;
/**
*
* A unique name to identify the cache policy.
*
*/
private String name;
/**
*
* The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. CloudFront uses this value as the
* object’s time to live (TTL) only when the origin does not send Cache-Control
or
* Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more than
* 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
*
*/
private Long defaultTTL;
/**
*
* The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends another
* request to the origin to see if the object has been updated. CloudFront uses this value only when the origin
* sends Cache-Control
or Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same as the
* value of DefaultTTL
.
*
*/
private Long maxTTL;
/**
*
* The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*/
private Long minTTL;
/**
*
* The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the cache
* key are automatically included in requests that CloudFront sends to the origin.
*
*/
private ParametersInCacheKeyAndForwardedToOrigin parametersInCacheKeyAndForwardedToOrigin;
/**
*
* A comment to describe the cache policy. The comment cannot be longer than 128 characters.
*
*
* @param comment
* A comment to describe the cache policy. The comment cannot be longer than 128 characters.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* A comment to describe the cache policy. The comment cannot be longer than 128 characters.
*
*
* @return A comment to describe the cache policy. The comment cannot be longer than 128 characters.
*/
public String getComment() {
return this.comment;
}
/**
*
* A comment to describe the cache policy. The comment cannot be longer than 128 characters.
*
*
* @param comment
* A comment to describe the cache policy. The comment cannot be longer than 128 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachePolicyConfig withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* A unique name to identify the cache policy.
*
*
* @param name
* A unique name to identify the cache policy.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A unique name to identify the cache policy.
*
*
* @return A unique name to identify the cache policy.
*/
public String getName() {
return this.name;
}
/**
*
* A unique name to identify the cache policy.
*
*
* @param name
* A unique name to identify the cache policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachePolicyConfig withName(String name) {
setName(name);
return this;
}
/**
*
* The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. CloudFront uses this value as the
* object’s time to live (TTL) only when the origin does not send Cache-Control
or
* Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more than
* 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
*
*
* @param defaultTTL
* The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before
* CloudFront sends another request to the origin to see if the object has been updated. CloudFront uses this
* value as the object’s time to live (TTL) only when the origin does not send
* Cache-Control
or Expires
headers with the object. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more
* than 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
*/
public void setDefaultTTL(Long defaultTTL) {
this.defaultTTL = defaultTTL;
}
/**
*
* The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. CloudFront uses this value as the
* object’s time to live (TTL) only when the origin does not send Cache-Control
or
* Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more than
* 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
*
*
* @return The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before
* CloudFront sends another request to the origin to see if the object has been updated. CloudFront uses
* this value as the object’s time to live (TTL) only when the origin does not send
* Cache-Control
or Expires
headers with the object. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more
* than 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
*/
public Long getDefaultTTL() {
return this.defaultTTL;
}
/**
*
* The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. CloudFront uses this value as the
* object’s time to live (TTL) only when the origin does not send Cache-Control
or
* Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more than
* 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
*
*
* @param defaultTTL
* The default amount of time, in seconds, that you want objects to stay in the CloudFront cache before
* CloudFront sends another request to the origin to see if the object has been updated. CloudFront uses this
* value as the object’s time to live (TTL) only when the origin does not send
* Cache-Control
or Expires
headers with the object. For more information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
* The default value for this field is 86400 seconds (one day). If the value of MinTTL
is more
* than 86400 seconds, then the default value for this field is the same as the value of MinTTL
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachePolicyConfig withDefaultTTL(Long defaultTTL) {
setDefaultTTL(defaultTTL);
return this;
}
/**
*
* The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends another
* request to the origin to see if the object has been updated. CloudFront uses this value only when the origin
* sends Cache-Control
or Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same as the
* value of DefaultTTL
.
*
*
* @param maxTTL
* The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends
* another request to the origin to see if the object has been updated. CloudFront uses this value only when
* the origin sends Cache-Control
or Expires
headers with the object. For more
* information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same
* as the value of DefaultTTL
.
*/
public void setMaxTTL(Long maxTTL) {
this.maxTTL = maxTTL;
}
/**
*
* The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends another
* request to the origin to see if the object has been updated. CloudFront uses this value only when the origin
* sends Cache-Control
or Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same as the
* value of DefaultTTL
.
*
*
* @return The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends
* another request to the origin to see if the object has been updated. CloudFront uses this value only when
* the origin sends Cache-Control
or Expires
headers with the object. For more
* information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same
* as the value of DefaultTTL
.
*/
public Long getMaxTTL() {
return this.maxTTL;
}
/**
*
* The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends another
* request to the origin to see if the object has been updated. CloudFront uses this value only when the origin
* sends Cache-Control
or Expires
headers with the object. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same as the
* value of DefaultTTL
.
*
*
* @param maxTTL
* The maximum amount of time, in seconds, that objects stay in the CloudFront cache before CloudFront sends
* another request to the origin to see if the object has been updated. CloudFront uses this value only when
* the origin sends Cache-Control
or Expires
headers with the object. For more
* information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
* The default value for this field is 31536000 seconds (one year). If the value of MinTTL
or
* DefaultTTL
is more than 31536000 seconds, then the default value for this field is the same
* as the value of DefaultTTL
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachePolicyConfig withMaxTTL(Long maxTTL) {
setMaxTTL(maxTTL);
return this;
}
/**
*
* The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @param minTTL
* The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before
* CloudFront sends another request to the origin to see if the object has been updated. For more
* information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*/
public void setMinTTL(Long minTTL) {
this.minTTL = minTTL;
}
/**
*
* The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @return The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before
* CloudFront sends another request to the origin to see if the object has been updated. For more
* information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*/
public Long getMinTTL() {
return this.minTTL;
}
/**
*
* The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before CloudFront
* sends another request to the origin to see if the object has been updated. For more information, see Managing How Long
* Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
*
*
* @param minTTL
* The minimum amount of time, in seconds, that you want objects to stay in the CloudFront cache before
* CloudFront sends another request to the origin to see if the object has been updated. For more
* information, see Managing How
* Long Content Stays in an Edge Cache (Expiration) in the Amazon CloudFront Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachePolicyConfig withMinTTL(Long minTTL) {
setMinTTL(minTTL);
return this;
}
/**
*
* The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the cache
* key are automatically included in requests that CloudFront sends to the origin.
*
*
* @param parametersInCacheKeyAndForwardedToOrigin
* The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the
* cache key are automatically included in requests that CloudFront sends to the origin.
*/
public void setParametersInCacheKeyAndForwardedToOrigin(ParametersInCacheKeyAndForwardedToOrigin parametersInCacheKeyAndForwardedToOrigin) {
this.parametersInCacheKeyAndForwardedToOrigin = parametersInCacheKeyAndForwardedToOrigin;
}
/**
*
* The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the cache
* key are automatically included in requests that CloudFront sends to the origin.
*
*
* @return The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the
* cache key are automatically included in requests that CloudFront sends to the origin.
*/
public ParametersInCacheKeyAndForwardedToOrigin getParametersInCacheKeyAndForwardedToOrigin() {
return this.parametersInCacheKeyAndForwardedToOrigin;
}
/**
*
* The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the cache
* key are automatically included in requests that CloudFront sends to the origin.
*
*
* @param parametersInCacheKeyAndForwardedToOrigin
* The HTTP headers, cookies, and URL query strings to include in the cache key. The values included in the
* cache key are automatically included in requests that CloudFront sends to the origin.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachePolicyConfig withParametersInCacheKeyAndForwardedToOrigin(ParametersInCacheKeyAndForwardedToOrigin parametersInCacheKeyAndForwardedToOrigin) {
setParametersInCacheKeyAndForwardedToOrigin(parametersInCacheKeyAndForwardedToOrigin);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDefaultTTL() != null)
sb.append("DefaultTTL: ").append(getDefaultTTL()).append(",");
if (getMaxTTL() != null)
sb.append("MaxTTL: ").append(getMaxTTL()).append(",");
if (getMinTTL() != null)
sb.append("MinTTL: ").append(getMinTTL()).append(",");
if (getParametersInCacheKeyAndForwardedToOrigin() != null)
sb.append("ParametersInCacheKeyAndForwardedToOrigin: ").append(getParametersInCacheKeyAndForwardedToOrigin());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CachePolicyConfig == false)
return false;
CachePolicyConfig other = (CachePolicyConfig) obj;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDefaultTTL() == null ^ this.getDefaultTTL() == null)
return false;
if (other.getDefaultTTL() != null && other.getDefaultTTL().equals(this.getDefaultTTL()) == false)
return false;
if (other.getMaxTTL() == null ^ this.getMaxTTL() == null)
return false;
if (other.getMaxTTL() != null && other.getMaxTTL().equals(this.getMaxTTL()) == false)
return false;
if (other.getMinTTL() == null ^ this.getMinTTL() == null)
return false;
if (other.getMinTTL() != null && other.getMinTTL().equals(this.getMinTTL()) == false)
return false;
if (other.getParametersInCacheKeyAndForwardedToOrigin() == null ^ this.getParametersInCacheKeyAndForwardedToOrigin() == null)
return false;
if (other.getParametersInCacheKeyAndForwardedToOrigin() != null
&& other.getParametersInCacheKeyAndForwardedToOrigin().equals(this.getParametersInCacheKeyAndForwardedToOrigin()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDefaultTTL() == null) ? 0 : getDefaultTTL().hashCode());
hashCode = prime * hashCode + ((getMaxTTL() == null) ? 0 : getMaxTTL().hashCode());
hashCode = prime * hashCode + ((getMinTTL() == null) ? 0 : getMinTTL().hashCode());
hashCode = prime * hashCode + ((getParametersInCacheKeyAndForwardedToOrigin() == null) ? 0 : getParametersInCacheKeyAndForwardedToOrigin().hashCode());
return hashCode;
}
@Override
public CachePolicyConfig clone() {
try {
return (CachePolicyConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}