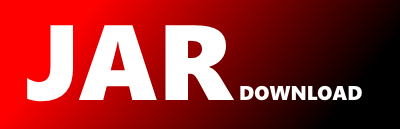
com.amazonaws.services.cloudsearch.AmazonCloudSearchAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudsearch Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudsearch;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.cloudsearch.model.*;
/**
* Interface for accessing AmazonCloudSearch asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
* Amazon CloudSearch Configuration Service
* You use the configuration service to create, configure, and manage search domains. Configuration service requests are submitted using the AWS Query
* protocol. AWS Query requests are HTTP or HTTPS requests submitted via HTTP GET or POST with a query parameter named Action.
*
*
* The endpoint for configuration service requests is region-specific: cloudsearch. region .amazonaws.com. For example,
* cloudsearch.us-east-1.amazonaws.com. For a current list of supported regions and endpoints, see Regions and Endpoints .
*
* @deprecated This code uses the older version of Amazon Cloud Search API (2011-02-01) and
* hence deprecated. The code in the package
* {@link com.amazonaws.services.cloudsearchv2} uses the latest
* version of Amazon Cloud Search API.Use
* {@link com.amazonaws.services.cloudsearchv2.AmazonCloudSearch}
* instead.
*/
@Deprecated
public interface AmazonCloudSearchAsync extends AmazonCloudSearch {
/**
*
* Configures the default search field for the search domain. The default
* search field is the text field that is searched when a search request
* does not specify which fields to search. By default, it is configured
* to include the contents of all of the domain's text fields.
*
*
* @param updateDefaultSearchFieldRequest Container for the necessary
* parameters to execute the UpdateDefaultSearchField operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* UpdateDefaultSearchField service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateDefaultSearchFieldAsync(UpdateDefaultSearchFieldRequest updateDefaultSearchFieldRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures the default search field for the search domain. The default
* search field is the text field that is searched when a search request
* does not specify which fields to search. By default, it is configured
* to include the contents of all of the domain's text fields.
*
*
* @param updateDefaultSearchFieldRequest Container for the necessary
* parameters to execute the UpdateDefaultSearchField operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateDefaultSearchField service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateDefaultSearchFieldAsync(UpdateDefaultSearchFieldRequest updateDefaultSearchFieldRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes a RankExpression
from the search domain.
*
*
* @param deleteRankExpressionRequest Container for the necessary
* parameters to execute the DeleteRankExpression operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DeleteRankExpression service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteRankExpressionAsync(DeleteRankExpressionRequest deleteRankExpressionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes a RankExpression
from the search domain.
*
*
* @param deleteRankExpressionRequest Container for the necessary
* parameters to execute the DeleteRankExpression operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteRankExpression service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteRankExpressionAsync(DeleteRankExpressionRequest deleteRankExpressionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the rank expressions configured for the search domain. Can be
* limited to specific rank expressions by name. Shows all rank
* expressions by default.
*
*
* @param describeRankExpressionsRequest Container for the necessary
* parameters to execute the DescribeRankExpressions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeRankExpressions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeRankExpressionsAsync(DescribeRankExpressionsRequest describeRankExpressionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the rank expressions configured for the search domain. Can be
* limited to specific rank expressions by name. Shows all rank
* expressions by default.
*
*
* @param describeRankExpressionsRequest Container for the necessary
* parameters to execute the DescribeRankExpressions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeRankExpressions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeRankExpressionsAsync(DescribeRankExpressionsRequest describeRankExpressionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new search domain.
*
*
* @param createDomainRequest Container for the necessary parameters to
* execute the CreateDomain operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* CreateDomain service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDomainAsync(CreateDomainRequest createDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new search domain.
*
*
* @param createDomainRequest Container for the necessary parameters to
* execute the CreateDomain operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateDomain service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDomainAsync(CreateDomainRequest createDomainRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures the policies that control access to the domain's document
* and search services. The maximum size of an access policy document is
* 100 KB.
*
*
* @param updateServiceAccessPoliciesRequest Container for the necessary
* parameters to execute the UpdateServiceAccessPolicies operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* UpdateServiceAccessPolicies service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateServiceAccessPoliciesAsync(UpdateServiceAccessPoliciesRequest updateServiceAccessPoliciesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures the policies that control access to the domain's document
* and search services. The maximum size of an access policy document is
* 100 KB.
*
*
* @param updateServiceAccessPoliciesRequest Container for the necessary
* parameters to execute the UpdateServiceAccessPolicies operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateServiceAccessPolicies service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateServiceAccessPoliciesAsync(UpdateServiceAccessPoliciesRequest updateServiceAccessPoliciesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures an IndexField
for the search domain. Used to
* create new fields and modify existing ones. If the field exists, the
* new configuration replaces the old one. You can configure a maximum of
* 200 index fields.
*
*
* @param defineIndexFieldRequest Container for the necessary parameters
* to execute the DefineIndexField operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DefineIndexField service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future defineIndexFieldAsync(DefineIndexFieldRequest defineIndexFieldRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures an IndexField
for the search domain. Used to
* create new fields and modify existing ones. If the field exists, the
* new configuration replaces the old one. You can configure a maximum of
* 200 index fields.
*
*
* @param defineIndexFieldRequest Container for the necessary parameters
* to execute the DefineIndexField operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DefineIndexField service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future defineIndexFieldAsync(DefineIndexFieldRequest defineIndexFieldRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures a RankExpression
for the search domain. Used
* to create new rank expressions and modify existing ones. If the
* expression exists, the new configuration replaces the old one. You can
* configure a maximum of 50 rank expressions.
*
*
* @param defineRankExpressionRequest Container for the necessary
* parameters to execute the DefineRankExpression operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DefineRankExpression service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future defineRankExpressionAsync(DefineRankExpressionRequest defineRankExpressionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures a RankExpression
for the search domain. Used
* to create new rank expressions and modify existing ones. If the
* expression exists, the new configuration replaces the old one. You can
* configure a maximum of 50 rank expressions.
*
*
* @param defineRankExpressionRequest Container for the necessary
* parameters to execute the DefineRankExpression operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DefineRankExpression service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future defineRankExpressionAsync(DefineRankExpressionRequest defineRankExpressionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the synonym dictionary configured for the search domain.
*
*
* @param describeSynonymOptionsRequest Container for the necessary
* parameters to execute the DescribeSynonymOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeSynonymOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSynonymOptionsAsync(DescribeSynonymOptionsRequest describeSynonymOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the synonym dictionary configured for the search domain.
*
*
* @param describeSynonymOptionsRequest Container for the necessary
* parameters to execute the DescribeSynonymOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeSynonymOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSynonymOptionsAsync(DescribeSynonymOptionsRequest describeSynonymOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the stopwords configured for the search domain.
*
*
* @param describeStopwordOptionsRequest Container for the necessary
* parameters to execute the DescribeStopwordOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeStopwordOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStopwordOptionsAsync(DescribeStopwordOptionsRequest describeStopwordOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the stopwords configured for the search domain.
*
*
* @param describeStopwordOptionsRequest Container for the necessary
* parameters to execute the DescribeStopwordOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStopwordOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStopwordOptionsAsync(DescribeStopwordOptionsRequest describeStopwordOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Permanently deletes a search domain and all of its data.
*
*
* @param deleteDomainRequest Container for the necessary parameters to
* execute the DeleteDomain operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DeleteDomain service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Permanently deletes a search domain and all of its data.
*
*
* @param deleteDomainRequest Container for the necessary parameters to
* execute the DeleteDomain operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteDomain service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets information about the resource-based policies that control access
* to the domain's document and search services.
*
*
* @param describeServiceAccessPoliciesRequest Container for the
* necessary parameters to execute the DescribeServiceAccessPolicies
* operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeServiceAccessPolicies service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeServiceAccessPoliciesAsync(DescribeServiceAccessPoliciesRequest describeServiceAccessPoliciesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets information about the resource-based policies that control access
* to the domain's document and search services.
*
*
* @param describeServiceAccessPoliciesRequest Container for the
* necessary parameters to execute the DescribeServiceAccessPolicies
* operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeServiceAccessPolicies service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeServiceAccessPoliciesAsync(DescribeServiceAccessPoliciesRequest describeServiceAccessPoliciesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures the availability options for a domain. Enabling the
* Multi-AZ option expands an Amazon CloudSearch domain to an additional
* Availability Zone in the same Region to increase fault tolerance in
* the event of a service disruption. Changes to the Multi-AZ option can
* take about half an hour to become active. For more information, see
* Configuring Availability Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param updateAvailabilityOptionsRequest Container for the necessary
* parameters to execute the UpdateAvailabilityOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* UpdateAvailabilityOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateAvailabilityOptionsAsync(UpdateAvailabilityOptionsRequest updateAvailabilityOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures the availability options for a domain. Enabling the
* Multi-AZ option expands an Amazon CloudSearch domain to an additional
* Availability Zone in the same Region to increase fault tolerance in
* the event of a service disruption. Changes to the Multi-AZ option can
* take about half an hour to become active. For more information, see
* Configuring Availability Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param updateAvailabilityOptionsRequest Container for the necessary
* parameters to execute the UpdateAvailabilityOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateAvailabilityOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateAvailabilityOptionsAsync(UpdateAvailabilityOptionsRequest updateAvailabilityOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the default search field configured for the search domain.
*
*
* @param describeDefaultSearchFieldRequest Container for the necessary
* parameters to execute the DescribeDefaultSearchField operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeDefaultSearchField service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDefaultSearchFieldAsync(DescribeDefaultSearchFieldRequest describeDefaultSearchFieldRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the default search field configured for the search domain.
*
*
* @param describeDefaultSearchFieldRequest Container for the necessary
* parameters to execute the DescribeDefaultSearchField operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeDefaultSearchField service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDefaultSearchFieldAsync(DescribeDefaultSearchFieldRequest describeDefaultSearchFieldRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures stopwords for the search domain. Stopwords are used during
* indexing and when processing search requests. The maximum size of the
* stopwords dictionary is 10 KB.
*
*
* @param updateStopwordOptionsRequest Container for the necessary
* parameters to execute the UpdateStopwordOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* UpdateStopwordOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStopwordOptionsAsync(UpdateStopwordOptionsRequest updateStopwordOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures stopwords for the search domain. Stopwords are used during
* indexing and when processing search requests. The maximum size of the
* stopwords dictionary is 10 KB.
*
*
* @param updateStopwordOptionsRequest Container for the necessary
* parameters to execute the UpdateStopwordOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateStopwordOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStopwordOptionsAsync(UpdateStopwordOptionsRequest updateStopwordOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures a synonym dictionary for the search domain. The synonym
* dictionary is used during indexing to configure mappings for terms
* that occur in text fields. The maximum size of the synonym dictionary
* is 100 KB.
*
*
* @param updateSynonymOptionsRequest Container for the necessary
* parameters to execute the UpdateSynonymOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* UpdateSynonymOptions service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateSynonymOptionsAsync(UpdateSynonymOptionsRequest updateSynonymOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures a synonym dictionary for the search domain. The synonym
* dictionary is used during indexing to configure mappings for terms
* that occur in text fields. The maximum size of the synonym dictionary
* is 100 KB.
*
*
* @param updateSynonymOptionsRequest Container for the necessary
* parameters to execute the UpdateSynonymOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateSynonymOptions service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateSynonymOptionsAsync(UpdateSynonymOptionsRequest updateSynonymOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures a stemming dictionary for the search domain. The stemming
* dictionary is used during indexing and when processing search
* requests. The maximum size of the stemming dictionary is 500 KB.
*
*
* @param updateStemmingOptionsRequest Container for the necessary
* parameters to execute the UpdateStemmingOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* UpdateStemmingOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStemmingOptionsAsync(UpdateStemmingOptionsRequest updateStemmingOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Configures a stemming dictionary for the search domain. The stemming
* dictionary is used during indexing and when processing search
* requests. The maximum size of the stemming dictionary is 500 KB.
*
*
* @param updateStemmingOptionsRequest Container for the necessary
* parameters to execute the UpdateStemmingOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateStemmingOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStemmingOptionsAsync(UpdateStemmingOptionsRequest updateStemmingOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the stemming dictionary configured for the search domain.
*
*
* @param describeStemmingOptionsRequest Container for the necessary
* parameters to execute the DescribeStemmingOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeStemmingOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStemmingOptionsAsync(DescribeStemmingOptionsRequest describeStemmingOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the stemming dictionary configured for the search domain.
*
*
* @param describeStemmingOptionsRequest Container for the necessary
* parameters to execute the DescribeStemmingOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStemmingOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStemmingOptionsAsync(DescribeStemmingOptionsRequest describeStemmingOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets information about the search domains owned by this account. Can
* be limited to specific domains. Shows all domains by default.
*
*
* @param describeDomainsRequest Container for the necessary parameters
* to execute the DescribeDomains operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeDomains service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDomainsAsync(DescribeDomainsRequest describeDomainsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets information about the search domains owned by this account. Can
* be limited to specific domains. Shows all domains by default.
*
*
* @param describeDomainsRequest Container for the necessary parameters
* to execute the DescribeDomains operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeDomains service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDomainsAsync(DescribeDomainsRequest describeDomainsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Tells the search domain to start indexing its documents using the
* latest text processing options and IndexFields
. This
* operation must be invoked to make options whose OptionStatus has
* OptionState
of RequiresIndexDocuments
* visible in search results.
*
*
* @param indexDocumentsRequest Container for the necessary parameters to
* execute the IndexDocuments operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* IndexDocuments service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future indexDocumentsAsync(IndexDocumentsRequest indexDocumentsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Tells the search domain to start indexing its documents using the
* latest text processing options and IndexFields
. This
* operation must be invoked to make options whose OptionStatus has
* OptionState
of RequiresIndexDocuments
* visible in search results.
*
*
* @param indexDocumentsRequest Container for the necessary parameters to
* execute the IndexDocuments operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* IndexDocuments service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future indexDocumentsAsync(IndexDocumentsRequest indexDocumentsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the availability options configured for a domain. By default,
* shows the configuration with any pending changes. Set the
* Deployed
option to true
to show the active
* configuration and exclude pending changes. For more information, see
* Configuring Availability Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeAvailabilityOptionsRequest Container for the necessary
* parameters to execute the DescribeAvailabilityOptions operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeAvailabilityOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeAvailabilityOptionsAsync(DescribeAvailabilityOptionsRequest describeAvailabilityOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets the availability options configured for a domain. By default,
* shows the configuration with any pending changes. Set the
* Deployed
option to true
to show the active
* configuration and exclude pending changes. For more information, see
* Configuring Availability Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeAvailabilityOptionsRequest Container for the necessary
* parameters to execute the DescribeAvailabilityOptions operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeAvailabilityOptions service method, as returned by
* AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeAvailabilityOptionsAsync(DescribeAvailabilityOptionsRequest describeAvailabilityOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets information about the index fields configured for the search
* domain. Can be limited to specific fields by name. Shows all fields by
* default.
*
*
* @param describeIndexFieldsRequest Container for the necessary
* parameters to execute the DescribeIndexFields operation on
* AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DescribeIndexFields service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeIndexFieldsAsync(DescribeIndexFieldsRequest describeIndexFieldsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets information about the index fields configured for the search
* domain. Can be limited to specific fields by name. Shows all fields by
* default.
*
*
* @param describeIndexFieldsRequest Container for the necessary
* parameters to execute the DescribeIndexFields operation on
* AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeIndexFields service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeIndexFieldsAsync(DescribeIndexFieldsRequest describeIndexFieldsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes an IndexField
from the search domain.
*
*
* @param deleteIndexFieldRequest Container for the necessary parameters
* to execute the DeleteIndexField operation on AmazonCloudSearch.
*
* @return A Java Future object containing the response from the
* DeleteIndexField service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteIndexFieldAsync(DeleteIndexFieldRequest deleteIndexFieldRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes an IndexField
from the search domain.
*
*
* @param deleteIndexFieldRequest Container for the necessary parameters
* to execute the DeleteIndexField operation on AmazonCloudSearch.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteIndexField service method, as returned by AmazonCloudSearch.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearch indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteIndexFieldAsync(DeleteIndexFieldRequest deleteIndexFieldRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}