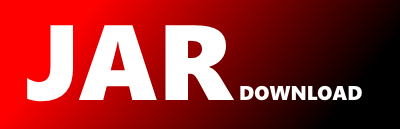
com.amazonaws.services.cloudsearchv2.model.DomainStatus Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudsearch Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudsearchv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The current status of the search domain.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DomainStatus implements Serializable, Cloneable {
private String domainId;
private String domainName;
private String aRN;
/**
*
* True if the search domain is created. It can take several minutes to initialize a domain when CreateDomain
* is called. Newly created search domains are returned from DescribeDomains with a false value for Created
* until domain creation is complete.
*
*/
private Boolean created;
/**
*
* True if the search domain has been deleted. The system must clean up resources dedicated to the search domain
* when DeleteDomain is called. Newly deleted search domains are returned from DescribeDomains with a
* true value for IsDeleted for several minutes until resource cleanup is complete.
*
*/
private Boolean deleted;
/**
*
* The service endpoint for updating documents in a search domain.
*
*/
private ServiceEndpoint docService;
/**
*
* The service endpoint for requesting search results from a search domain.
*
*/
private ServiceEndpoint searchService;
/**
*
* True if IndexDocuments needs to be called to activate the current domain configuration.
*
*/
private Boolean requiresIndexDocuments;
/**
*
* True if processing is being done to activate the current domain configuration.
*
*/
private Boolean processing;
/**
*
* The instance type that is being used to process search requests.
*
*/
private String searchInstanceType;
/**
*
* The number of partitions across which the search index is spread.
*
*/
private Integer searchPartitionCount;
/**
*
* The number of search instances that are available to process search requests.
*
*/
private Integer searchInstanceCount;
private Limits limits;
/**
* @param domainId
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
* @return
*/
public String getDomainId() {
return this.domainId;
}
/**
* @param domainId
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
* @param domainName
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
* @return
*/
public String getDomainName() {
return this.domainName;
}
/**
* @param domainName
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
* @param aRN
*/
public void setARN(String aRN) {
this.aRN = aRN;
}
/**
* @return
*/
public String getARN() {
return this.aRN;
}
/**
* @param aRN
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withARN(String aRN) {
setARN(aRN);
return this;
}
/**
*
* True if the search domain is created. It can take several minutes to initialize a domain when CreateDomain
* is called. Newly created search domains are returned from DescribeDomains with a false value for Created
* until domain creation is complete.
*
*
* @param created
* True if the search domain is created. It can take several minutes to initialize a domain when
* CreateDomain is called. Newly created search domains are returned from DescribeDomains with
* a false value for Created until domain creation is complete.
*/
public void setCreated(Boolean created) {
this.created = created;
}
/**
*
* True if the search domain is created. It can take several minutes to initialize a domain when CreateDomain
* is called. Newly created search domains are returned from DescribeDomains with a false value for Created
* until domain creation is complete.
*
*
* @return True if the search domain is created. It can take several minutes to initialize a domain when
* CreateDomain is called. Newly created search domains are returned from DescribeDomains with
* a false value for Created until domain creation is complete.
*/
public Boolean getCreated() {
return this.created;
}
/**
*
* True if the search domain is created. It can take several minutes to initialize a domain when CreateDomain
* is called. Newly created search domains are returned from DescribeDomains with a false value for Created
* until domain creation is complete.
*
*
* @param created
* True if the search domain is created. It can take several minutes to initialize a domain when
* CreateDomain is called. Newly created search domains are returned from DescribeDomains with
* a false value for Created until domain creation is complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withCreated(Boolean created) {
setCreated(created);
return this;
}
/**
*
* True if the search domain is created. It can take several minutes to initialize a domain when CreateDomain
* is called. Newly created search domains are returned from DescribeDomains with a false value for Created
* until domain creation is complete.
*
*
* @return True if the search domain is created. It can take several minutes to initialize a domain when
* CreateDomain is called. Newly created search domains are returned from DescribeDomains with
* a false value for Created until domain creation is complete.
*/
public Boolean isCreated() {
return this.created;
}
/**
*
* True if the search domain has been deleted. The system must clean up resources dedicated to the search domain
* when DeleteDomain is called. Newly deleted search domains are returned from DescribeDomains with a
* true value for IsDeleted for several minutes until resource cleanup is complete.
*
*
* @param deleted
* True if the search domain has been deleted. The system must clean up resources dedicated to the search
* domain when DeleteDomain is called. Newly deleted search domains are returned from
* DescribeDomains with a true value for IsDeleted for several minutes until resource cleanup is
* complete.
*/
public void setDeleted(Boolean deleted) {
this.deleted = deleted;
}
/**
*
* True if the search domain has been deleted. The system must clean up resources dedicated to the search domain
* when DeleteDomain is called. Newly deleted search domains are returned from DescribeDomains with a
* true value for IsDeleted for several minutes until resource cleanup is complete.
*
*
* @return True if the search domain has been deleted. The system must clean up resources dedicated to the search
* domain when DeleteDomain is called. Newly deleted search domains are returned from
* DescribeDomains with a true value for IsDeleted for several minutes until resource cleanup is
* complete.
*/
public Boolean getDeleted() {
return this.deleted;
}
/**
*
* True if the search domain has been deleted. The system must clean up resources dedicated to the search domain
* when DeleteDomain is called. Newly deleted search domains are returned from DescribeDomains with a
* true value for IsDeleted for several minutes until resource cleanup is complete.
*
*
* @param deleted
* True if the search domain has been deleted. The system must clean up resources dedicated to the search
* domain when DeleteDomain is called. Newly deleted search domains are returned from
* DescribeDomains with a true value for IsDeleted for several minutes until resource cleanup is
* complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withDeleted(Boolean deleted) {
setDeleted(deleted);
return this;
}
/**
*
* True if the search domain has been deleted. The system must clean up resources dedicated to the search domain
* when DeleteDomain is called. Newly deleted search domains are returned from DescribeDomains with a
* true value for IsDeleted for several minutes until resource cleanup is complete.
*
*
* @return True if the search domain has been deleted. The system must clean up resources dedicated to the search
* domain when DeleteDomain is called. Newly deleted search domains are returned from
* DescribeDomains with a true value for IsDeleted for several minutes until resource cleanup is
* complete.
*/
public Boolean isDeleted() {
return this.deleted;
}
/**
*
* The service endpoint for updating documents in a search domain.
*
*
* @param docService
* The service endpoint for updating documents in a search domain.
*/
public void setDocService(ServiceEndpoint docService) {
this.docService = docService;
}
/**
*
* The service endpoint for updating documents in a search domain.
*
*
* @return The service endpoint for updating documents in a search domain.
*/
public ServiceEndpoint getDocService() {
return this.docService;
}
/**
*
* The service endpoint for updating documents in a search domain.
*
*
* @param docService
* The service endpoint for updating documents in a search domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withDocService(ServiceEndpoint docService) {
setDocService(docService);
return this;
}
/**
*
* The service endpoint for requesting search results from a search domain.
*
*
* @param searchService
* The service endpoint for requesting search results from a search domain.
*/
public void setSearchService(ServiceEndpoint searchService) {
this.searchService = searchService;
}
/**
*
* The service endpoint for requesting search results from a search domain.
*
*
* @return The service endpoint for requesting search results from a search domain.
*/
public ServiceEndpoint getSearchService() {
return this.searchService;
}
/**
*
* The service endpoint for requesting search results from a search domain.
*
*
* @param searchService
* The service endpoint for requesting search results from a search domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withSearchService(ServiceEndpoint searchService) {
setSearchService(searchService);
return this;
}
/**
*
* True if IndexDocuments needs to be called to activate the current domain configuration.
*
*
* @param requiresIndexDocuments
* True if IndexDocuments needs to be called to activate the current domain configuration.
*/
public void setRequiresIndexDocuments(Boolean requiresIndexDocuments) {
this.requiresIndexDocuments = requiresIndexDocuments;
}
/**
*
* True if IndexDocuments needs to be called to activate the current domain configuration.
*
*
* @return True if IndexDocuments needs to be called to activate the current domain configuration.
*/
public Boolean getRequiresIndexDocuments() {
return this.requiresIndexDocuments;
}
/**
*
* True if IndexDocuments needs to be called to activate the current domain configuration.
*
*
* @param requiresIndexDocuments
* True if IndexDocuments needs to be called to activate the current domain configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withRequiresIndexDocuments(Boolean requiresIndexDocuments) {
setRequiresIndexDocuments(requiresIndexDocuments);
return this;
}
/**
*
* True if IndexDocuments needs to be called to activate the current domain configuration.
*
*
* @return True if IndexDocuments needs to be called to activate the current domain configuration.
*/
public Boolean isRequiresIndexDocuments() {
return this.requiresIndexDocuments;
}
/**
*
* True if processing is being done to activate the current domain configuration.
*
*
* @param processing
* True if processing is being done to activate the current domain configuration.
*/
public void setProcessing(Boolean processing) {
this.processing = processing;
}
/**
*
* True if processing is being done to activate the current domain configuration.
*
*
* @return True if processing is being done to activate the current domain configuration.
*/
public Boolean getProcessing() {
return this.processing;
}
/**
*
* True if processing is being done to activate the current domain configuration.
*
*
* @param processing
* True if processing is being done to activate the current domain configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withProcessing(Boolean processing) {
setProcessing(processing);
return this;
}
/**
*
* True if processing is being done to activate the current domain configuration.
*
*
* @return True if processing is being done to activate the current domain configuration.
*/
public Boolean isProcessing() {
return this.processing;
}
/**
*
* The instance type that is being used to process search requests.
*
*
* @param searchInstanceType
* The instance type that is being used to process search requests.
*/
public void setSearchInstanceType(String searchInstanceType) {
this.searchInstanceType = searchInstanceType;
}
/**
*
* The instance type that is being used to process search requests.
*
*
* @return The instance type that is being used to process search requests.
*/
public String getSearchInstanceType() {
return this.searchInstanceType;
}
/**
*
* The instance type that is being used to process search requests.
*
*
* @param searchInstanceType
* The instance type that is being used to process search requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withSearchInstanceType(String searchInstanceType) {
setSearchInstanceType(searchInstanceType);
return this;
}
/**
*
* The number of partitions across which the search index is spread.
*
*
* @param searchPartitionCount
* The number of partitions across which the search index is spread.
*/
public void setSearchPartitionCount(Integer searchPartitionCount) {
this.searchPartitionCount = searchPartitionCount;
}
/**
*
* The number of partitions across which the search index is spread.
*
*
* @return The number of partitions across which the search index is spread.
*/
public Integer getSearchPartitionCount() {
return this.searchPartitionCount;
}
/**
*
* The number of partitions across which the search index is spread.
*
*
* @param searchPartitionCount
* The number of partitions across which the search index is spread.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withSearchPartitionCount(Integer searchPartitionCount) {
setSearchPartitionCount(searchPartitionCount);
return this;
}
/**
*
* The number of search instances that are available to process search requests.
*
*
* @param searchInstanceCount
* The number of search instances that are available to process search requests.
*/
public void setSearchInstanceCount(Integer searchInstanceCount) {
this.searchInstanceCount = searchInstanceCount;
}
/**
*
* The number of search instances that are available to process search requests.
*
*
* @return The number of search instances that are available to process search requests.
*/
public Integer getSearchInstanceCount() {
return this.searchInstanceCount;
}
/**
*
* The number of search instances that are available to process search requests.
*
*
* @param searchInstanceCount
* The number of search instances that are available to process search requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withSearchInstanceCount(Integer searchInstanceCount) {
setSearchInstanceCount(searchInstanceCount);
return this;
}
/**
* @param limits
*/
public void setLimits(Limits limits) {
this.limits = limits;
}
/**
* @return
*/
public Limits getLimits() {
return this.limits;
}
/**
* @param limits
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DomainStatus withLimits(Limits limits) {
setLimits(limits);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getARN() != null)
sb.append("ARN: ").append(getARN()).append(",");
if (getCreated() != null)
sb.append("Created: ").append(getCreated()).append(",");
if (getDeleted() != null)
sb.append("Deleted: ").append(getDeleted()).append(",");
if (getDocService() != null)
sb.append("DocService: ").append(getDocService()).append(",");
if (getSearchService() != null)
sb.append("SearchService: ").append(getSearchService()).append(",");
if (getRequiresIndexDocuments() != null)
sb.append("RequiresIndexDocuments: ").append(getRequiresIndexDocuments()).append(",");
if (getProcessing() != null)
sb.append("Processing: ").append(getProcessing()).append(",");
if (getSearchInstanceType() != null)
sb.append("SearchInstanceType: ").append(getSearchInstanceType()).append(",");
if (getSearchPartitionCount() != null)
sb.append("SearchPartitionCount: ").append(getSearchPartitionCount()).append(",");
if (getSearchInstanceCount() != null)
sb.append("SearchInstanceCount: ").append(getSearchInstanceCount()).append(",");
if (getLimits() != null)
sb.append("Limits: ").append(getLimits());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DomainStatus == false)
return false;
DomainStatus other = (DomainStatus) obj;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getARN() == null ^ this.getARN() == null)
return false;
if (other.getARN() != null && other.getARN().equals(this.getARN()) == false)
return false;
if (other.getCreated() == null ^ this.getCreated() == null)
return false;
if (other.getCreated() != null && other.getCreated().equals(this.getCreated()) == false)
return false;
if (other.getDeleted() == null ^ this.getDeleted() == null)
return false;
if (other.getDeleted() != null && other.getDeleted().equals(this.getDeleted()) == false)
return false;
if (other.getDocService() == null ^ this.getDocService() == null)
return false;
if (other.getDocService() != null && other.getDocService().equals(this.getDocService()) == false)
return false;
if (other.getSearchService() == null ^ this.getSearchService() == null)
return false;
if (other.getSearchService() != null && other.getSearchService().equals(this.getSearchService()) == false)
return false;
if (other.getRequiresIndexDocuments() == null ^ this.getRequiresIndexDocuments() == null)
return false;
if (other.getRequiresIndexDocuments() != null && other.getRequiresIndexDocuments().equals(this.getRequiresIndexDocuments()) == false)
return false;
if (other.getProcessing() == null ^ this.getProcessing() == null)
return false;
if (other.getProcessing() != null && other.getProcessing().equals(this.getProcessing()) == false)
return false;
if (other.getSearchInstanceType() == null ^ this.getSearchInstanceType() == null)
return false;
if (other.getSearchInstanceType() != null && other.getSearchInstanceType().equals(this.getSearchInstanceType()) == false)
return false;
if (other.getSearchPartitionCount() == null ^ this.getSearchPartitionCount() == null)
return false;
if (other.getSearchPartitionCount() != null && other.getSearchPartitionCount().equals(this.getSearchPartitionCount()) == false)
return false;
if (other.getSearchInstanceCount() == null ^ this.getSearchInstanceCount() == null)
return false;
if (other.getSearchInstanceCount() != null && other.getSearchInstanceCount().equals(this.getSearchInstanceCount()) == false)
return false;
if (other.getLimits() == null ^ this.getLimits() == null)
return false;
if (other.getLimits() != null && other.getLimits().equals(this.getLimits()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getARN() == null) ? 0 : getARN().hashCode());
hashCode = prime * hashCode + ((getCreated() == null) ? 0 : getCreated().hashCode());
hashCode = prime * hashCode + ((getDeleted() == null) ? 0 : getDeleted().hashCode());
hashCode = prime * hashCode + ((getDocService() == null) ? 0 : getDocService().hashCode());
hashCode = prime * hashCode + ((getSearchService() == null) ? 0 : getSearchService().hashCode());
hashCode = prime * hashCode + ((getRequiresIndexDocuments() == null) ? 0 : getRequiresIndexDocuments().hashCode());
hashCode = prime * hashCode + ((getProcessing() == null) ? 0 : getProcessing().hashCode());
hashCode = prime * hashCode + ((getSearchInstanceType() == null) ? 0 : getSearchInstanceType().hashCode());
hashCode = prime * hashCode + ((getSearchPartitionCount() == null) ? 0 : getSearchPartitionCount().hashCode());
hashCode = prime * hashCode + ((getSearchInstanceCount() == null) ? 0 : getSearchInstanceCount().hashCode());
hashCode = prime * hashCode + ((getLimits() == null) ? 0 : getLimits().hashCode());
return hashCode;
}
@Override
public DomainStatus clone() {
try {
return (DomainStatus) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}