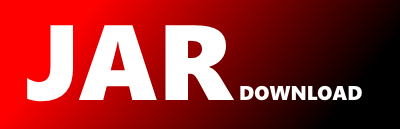
com.amazonaws.services.cloudsearchdomain.model.SearchRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudsearch Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudsearchdomain.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Container for the parameters to the Search
request.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SearchRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Retrieves a cursor value you can use to page through large result sets. Use the size
parameter to
* control the number of hits to include in each response. You can specify either the cursor
or
* start
parameter in a request; they are mutually exclusive. To get the first cursor, set the cursor
* value to initial
. In subsequent requests, specify the cursor value returned in the hits section of
* the response.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*/
private String cursor;
/**
*
* Defines one or more numeric expressions that can be used to sort results or specify search or filter criteria.
* You can also specify expressions as return fields.
*
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can define
* and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
*
*/
private String expr;
/**
*
* Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The fields and
* options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified using the
* same syntax that you use to search for a range of values. For more information, see Searching for a Range
* of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order they are
* specified in the request. The sort
and size
options are not valid if you specify
* buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify the
* sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value (in
* descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted by facet
* count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following request
* uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets the
* sort
option to count
to sort the facet values by facet count, with the facet values
* that have the most matching documents listed first. Setting the size
option to 3 returns only the
* top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets the
* sort
option to bucket
to sort the facet values numerically by year, with earliest year
* listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
*
*/
private String facet;
/**
*
* Specifies a structured query that filters the results of a search without affecting how the results are scored
* and sorted. You use filterQuery
in conjunction with the query
parameter to filter the
* documents that match the constraints specified in the query
parameter. Specifying a filter controls
* only which matching documents are included in the results, it has no effect on how they are scored and sorted.
* The filterQuery
parameter supports the full structured query syntax.
*
*
* For more information about using filters, see Filtering Matching
* Documents in the Amazon CloudSearch Developer Guide.
*
*/
private String filterQuery;
/**
*
* Retrieves highlights for matches in the specified text
or text-array
fields. Each
* specified field must be highlight enabled in the domain configuration. The fields and options are specified in
* JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The default is
* html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want to
* highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default for HTML
* highlights is <em>
. The default for text highlights is *
.
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default for HTML
* highlights is </em>
. The default for text highlights is *
.
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the first match
* is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and title
* fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
*
*/
private String highlight;
/**
*
* Enables partial results to be returned if one or more index partitions are unavailable. When your search index is
* partitioned across multiple search instances, by default Amazon CloudSearch only returns results if every
* partition can be queried. This means that the failure of a single search instance can result in 5xx (internal
* server) errors. When you enable partial results, Amazon CloudSearch returns whatever results are available and
* includes the percentage of documents searched in the search results (percent-searched). This enables you to more
* gracefully degrade your users' search experience. For example, rather than displaying no results, you could
* display the partial results and a message indicating that the results might be incomplete due to a temporary
* system outage.
*
*/
private Boolean partial;
/**
*
* Specifies the search criteria for the request. How you specify the search criteria depends on the query parser
* used for the request and the parser options specified in the queryOptions
parameter. By default, the
* simple
query parser is used to process requests. To use the structured
,
* lucene
, or dismax
query parser, you must also specify the queryParser
* parameter.
*
*
* For more information about specifying search criteria, see Searching Your Data in the
* Amazon CloudSearch Developer Guide.
*
*/
private String query;
/**
*
* Configures options for the query parser specified in the queryParser
parameter. You specify the
* options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search string. For
* example: defaultOperator: 'or'
. For the dismax
parser, you specify a percentage that
* represents the percentage of terms in the search string (rounded down) that must match, rather than a default
* operator. A value of 0%
is the equivalent to OR, and a value of 100%
is equivalent to
* AND. The percentage must be specified as a value in the range 0-100 followed by the percent (%) symbol. For
* example, defaultOperator: 50%
. Valid values: and
, or
, a percentage in the
* range 0%-100% (dismax
). Default: and
(simple
, structured
,
* lucene
) or 100
(dismax
). Valid for: simple
,
* structured
, lucene
, and dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no fields
* are specified in a search and this option is not specified, all text and text-array fields are searched. You can
* specify a weight for each field to control the relative importance of each field when Amazon CloudSearch
* calculates relevance scores. To specify a field weight, append a caret (^
) symbol and the weight to
* the field name. For example, to boost the importance of the title
field over the
* description
field you could specify: "fields":["title^5","description"]
. Valid values:
* The name of any configured field and an optional numeric value greater than zero. Default: All text
* and text-array
fields. Valid for: simple
, structured
, lucene
,
* and dismax
.
* operators
: An array of the operators or special characters you want to disable for the simple
* query parser. If you disable the and
, or
, or not
operators, the
* corresponding operators (+
, |
, -
) have no special meaning and are dropped
* from the search string. Similarly, disabling prefix
disables the wildcard operator (*
)
* and disabling phrase
disables the ability to search for phrases by enclosing phrases in double
* quotes. Disabling precedence disables the ability to control order of precedence using parentheses. Disabling
* near
disables the ability to use the ~ operator to perform a sloppy phrase search. Disabling the
* fuzzy
operator disables the ability to use the ~ operator to perform a fuzzy search.
* escape
disables the ability to use a backslash (\
) to escape special characters within
* the search string. Disabling whitespace is an advanced option that prevents the parser from tokenizing on
* whitespace, which can be useful for Vietnamese. (It prevents Vietnamese words from being split incorrectly.) For
* example, you could disable all operators other than the phrase operator to support just simple term and phrase
* queries: "operators":["and","not","or", "prefix"]
. Valid values: and
,
* escape
, fuzzy
, near
, not
, or
,
* phrase
, precedence
, prefix
, whitespace
. Default: All
* operators and special characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you want to
* use for phrase searches. When the terms in the search string appear in close proximity within a field, the field
* scores higher. You can specify a weight for each field to boost that score. The phraseSlop
option
* controls how much the matches can deviate from the search string and still be boosted. To specify a field weight,
* append a caret (^
) symbol and the weight to the field name. For example, to boost phrase matches in
* the title
field over the abstract
field, you could specify:
* "phraseFields":["title^3", "plot"]
Valid values: The name of any text
or
* text-array
field and an optional numeric value greater than zero. Default: No fields. If you don't
* specify any fields with phraseFields
, proximity scoring is disabled even if phraseSlop
* is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search phrase
* and still be boosted according to the weights specified in the phraseFields
option; for example,
* phraseSlop: 2
. You must also specify phraseFields
to enable proximity scoring. Valid
* values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from the search
* phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed this proximity
* distance are not considered a match.) For example, to specify a slop of three for dismax phrase queries, you
* would specify "explicitPhraseSlop":3
. Valid values: positive integers. Default: 0. Valid for:
* dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If the term
* occurs in multiple fields within a document, by default only the highest scoring field contributes to the
* document's overall score. You can specify a tieBreaker
value to enable the matches in lower-scoring
* fields to contribute to the document's score. That way, if two documents have the same max field score for a
* particular term, the score for the document that has matches in more fields will be higher. The formula for
* calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
. Set
* tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum): "tieBreaker":1
.
* Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
*
*/
private String queryOptions;
/**
*
* Specifies which query parser to use to process the request. If queryParser
is not specified, Amazon
* CloudSearch uses the simple
query parser.
*
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields. By
* default, the simple
query parser searches all text
and text-array
fields.
* You can specify which fields to search by with the queryOptions
parameter. If you prefix a search
* term with a plus sign (+) documents must contain the term to be considered a match. (This is the default, unless
* you configure the default operator with the queryOptions
parameter.) You can use the -
* (NOT), |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than individual
* terms, enclose the phrase in double quotes. For more information, see Searching for Text in
* the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the search
* criteria. You can also search within particular fields, search for values and ranges of values, and use advanced
* options such as term boosting, matchall
, and near
. For more information, see Constructing
* Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax defined by
* the DisMax query parser. For more information, see DisMax Query Parser Syntax.
*
*/
private String queryParser;
/**
*
* Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
*
*/
private String returnValue;
/**
*
* Specifies the maximum number of search hits to include in the response.
*
*/
private Long size;
/**
*
* Specifies the fields or custom expressions to use to sort the search results. Multiple fields or expressions are
* specified as a comma-separated list. You must specify the sort direction (asc
or desc
)
* for each field; for example, year desc,title asc
. To use a field to sort results, the field must be
* sort-enabled in the domain configuration. Array type fields cannot be used for sorting. If no sort
* parameter is specified, results are sorted by their default relevance scores in descending order:
* _score desc
. You can also sort by document ID (_id asc
) and version (
* _version desc
).
*
*
* For more information, see Sorting Results in
* the Amazon CloudSearch Developer Guide.
*
*/
private String sort;
/**
*
* Specifies the offset of the first search hit you want to return. Note that the result set is zero-based; the
* first result is at index 0. You can specify either the start
or cursor
parameter in a
* request, they are mutually exclusive.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*/
private Long start;
/**
*
* Specifies one or more fields for which to get statistics information. Each specified field must be facet-enabled
* in the domain configuration. The fields are specified in JSON using the form:
*
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
*
*/
private String stats;
/**
*
* Retrieves a cursor value you can use to page through large result sets. Use the size
parameter to
* control the number of hits to include in each response. You can specify either the cursor
or
* start
parameter in a request; they are mutually exclusive. To get the first cursor, set the cursor
* value to initial
. In subsequent requests, specify the cursor value returned in the hits section of
* the response.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*
* @param cursor
* Retrieves a cursor value you can use to page through large result sets. Use the size
* parameter to control the number of hits to include in each response. You can specify either the
* cursor
or start
parameter in a request; they are mutually exclusive. To get the
* first cursor, set the cursor value to initial
. In subsequent requests, specify the cursor
* value returned in the hits section of the response.
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*/
public void setCursor(String cursor) {
this.cursor = cursor;
}
/**
*
* Retrieves a cursor value you can use to page through large result sets. Use the size
parameter to
* control the number of hits to include in each response. You can specify either the cursor
or
* start
parameter in a request; they are mutually exclusive. To get the first cursor, set the cursor
* value to initial
. In subsequent requests, specify the cursor value returned in the hits section of
* the response.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*
* @return Retrieves a cursor value you can use to page through large result sets. Use the size
* parameter to control the number of hits to include in each response. You can specify either the
* cursor
or start
parameter in a request; they are mutually exclusive. To get the
* first cursor, set the cursor value to initial
. In subsequent requests, specify the cursor
* value returned in the hits section of the response.
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*/
public String getCursor() {
return this.cursor;
}
/**
*
* Retrieves a cursor value you can use to page through large result sets. Use the size
parameter to
* control the number of hits to include in each response. You can specify either the cursor
or
* start
parameter in a request; they are mutually exclusive. To get the first cursor, set the cursor
* value to initial
. In subsequent requests, specify the cursor value returned in the hits section of
* the response.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*
* @param cursor
* Retrieves a cursor value you can use to page through large result sets. Use the size
* parameter to control the number of hits to include in each response. You can specify either the
* cursor
or start
parameter in a request; they are mutually exclusive. To get the
* first cursor, set the cursor value to initial
. In subsequent requests, specify the cursor
* value returned in the hits section of the response.
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withCursor(String cursor) {
setCursor(cursor);
return this;
}
/**
*
* Defines one or more numeric expressions that can be used to sort results or specify search or filter criteria.
* You can also specify expressions as return fields.
*
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can define
* and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param expr
* Defines one or more numeric expressions that can be used to sort results or specify search or filter
* criteria. You can also specify expressions as return fields.
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can
* define and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
*/
public void setExpr(String expr) {
this.expr = expr;
}
/**
*
* Defines one or more numeric expressions that can be used to sort results or specify search or filter criteria.
* You can also specify expressions as return fields.
*
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can define
* and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @return Defines one or more numeric expressions that can be used to sort results or specify search or filter
* criteria. You can also specify expressions as return fields.
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can
* define and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
*/
public String getExpr() {
return this.expr;
}
/**
*
* Defines one or more numeric expressions that can be used to sort results or specify search or filter criteria.
* You can also specify expressions as return fields.
*
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can define
* and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param expr
* Defines one or more numeric expressions that can be used to sort results or specify search or filter
* criteria. You can also specify expressions as return fields.
*
* You specify the expressions in JSON using the form {"EXPRESSIONNAME":"EXPRESSION"}
. You can
* define and use multiple expressions in a search request. For example:
*
*
* {"expression1":"_score*rating", "expression2":"(1/rank)*year"}
*
*
* For information about the variables, operators, and functions you can use in expressions, see Writing Expressions in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withExpr(String expr) {
setExpr(expr);
return this;
}
/**
*
* Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The fields and
* options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified using the
* same syntax that you use to search for a range of values. For more information, see Searching for a Range
* of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order they are
* specified in the request. The sort
and size
options are not valid if you specify
* buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify the
* sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value (in
* descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted by facet
* count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following request
* uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets the
* sort
option to count
to sort the facet values by facet count, with the facet values
* that have the most matching documents listed first. Setting the size
option to 3 returns only the
* top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets the
* sort
option to bucket
to sort the facet values numerically by year, with earliest year
* listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
*
*
* @param facet
* Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The
* fields and options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified using
* the same syntax that you use to search for a range of values. For more information, see Searching for a
* Range of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order
* they are specified in the request. The sort
and size
options are not valid if
* you specify buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify
* the sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value (in
* descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted
* by facet count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following
* request uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets
* the sort
option to count
to sort the facet values by facet count, with the facet
* values that have the most matching documents listed first. Setting the size
option to 3
* returns only the top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets
* the sort
option to bucket
to sort the facet values numerically by year, with
* earliest year listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
*/
public void setFacet(String facet) {
this.facet = facet;
}
/**
*
* Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The fields and
* options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified using the
* same syntax that you use to search for a range of values. For more information, see Searching for a Range
* of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order they are
* specified in the request. The sort
and size
options are not valid if you specify
* buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify the
* sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value (in
* descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted by facet
* count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following request
* uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets the
* sort
option to count
to sort the facet values by facet count, with the facet values
* that have the most matching documents listed first. Setting the size
option to 3 returns only the
* top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets the
* sort
option to bucket
to sort the facet values numerically by year, with earliest year
* listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
*
*
* @return Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The
* fields and options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified
* using the same syntax that you use to search for a range of values. For more information, see Searching for
* a Range of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order
* they are specified in the request. The sort
and size
options are not valid if
* you specify buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify
* the sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value
* (in descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted
* by facet count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following
* request uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets
* the sort
option to count
to sort the facet values by facet count, with the
* facet values that have the most matching documents listed first. Setting the size
option to
* 3 returns only the top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets
* the sort
option to bucket
to sort the facet values numerically by year, with
* earliest year listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
*/
public String getFacet() {
return this.facet;
}
/**
*
* Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The fields and
* options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified using the
* same syntax that you use to search for a range of values. For more information, see Searching for a Range
* of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order they are
* specified in the request. The sort
and size
options are not valid if you specify
* buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify the
* sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value (in
* descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted by facet
* count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following request
* uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets the
* sort
option to count
to sort the facet values by facet count, with the facet values
* that have the most matching documents listed first. Setting the size
option to 3 returns only the
* top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets the
* sort
option to bucket
to sort the facet values numerically by year, with earliest year
* listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
*
*
* @param facet
* Specifies one or more fields for which to get facet information, and options that control how the facet
* information is returned. Each specified field must be facet-enabled in the domain configuration. The
* fields and options are specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
* You can specify the following faceting options:
*
*
* -
*
* buckets
specifies an array of the facet values or ranges to count. Ranges are specified using
* the same syntax that you use to search for a range of values. For more information, see Searching for a
* Range of Values in the Amazon CloudSearch Developer Guide. Buckets are returned in the order
* they are specified in the request. The sort
and size
options are not valid if
* you specify buckets
.
*
*
* -
*
* size
specifies the maximum number of facets to include in the results. By default, Amazon
* CloudSearch returns counts for the top 10. The size
parameter is only valid when you specify
* the sort
option; it cannot be used in conjunction with buckets
.
*
*
* -
*
* sort
specifies how you want to sort the facets in the results: bucket
or
* count
. Specify bucket
to sort alphabetically or numerically by facet value (in
* ascending order). Specify count
to sort by the facet counts computed for each facet value (in
* descending order). To retrieve facet counts for particular values or ranges of values, use the
* buckets
option instead of sort
.
*
*
*
*
* If no facet options are specified, facet counts are computed for all field values, the facets are sorted
* by facet count, and the top 10 facets are returned in the results.
*
*
* To count particular buckets of values, use the buckets
option. For example, the following
* request uses the buckets
option to calculate and return facet counts by decade.
*
*
* {"year":{"buckets":["[1970,1979]","[1980,1989]","[1990,1999]","[2000,2009]","[2010,}"]}}
*
*
* To sort facets by facet count, use the count
option. For example, the following request sets
* the sort
option to count
to sort the facet values by facet count, with the facet
* values that have the most matching documents listed first. Setting the size
option to 3
* returns only the top three facet values.
*
*
* {"year":{"sort":"count","size":3}}
*
*
* To sort the facets by value, use the bucket
option. For example, the following request sets
* the sort
option to bucket
to sort the facet values numerically by year, with
* earliest year listed first.
*
*
* {"year":{"sort":"bucket"}}
*
*
* For more information, see Getting and Using Facet
* Information in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withFacet(String facet) {
setFacet(facet);
return this;
}
/**
*
* Specifies a structured query that filters the results of a search without affecting how the results are scored
* and sorted. You use filterQuery
in conjunction with the query
parameter to filter the
* documents that match the constraints specified in the query
parameter. Specifying a filter controls
* only which matching documents are included in the results, it has no effect on how they are scored and sorted.
* The filterQuery
parameter supports the full structured query syntax.
*
*
* For more information about using filters, see Filtering Matching
* Documents in the Amazon CloudSearch Developer Guide.
*
*
* @param filterQuery
* Specifies a structured query that filters the results of a search without affecting how the results are
* scored and sorted. You use filterQuery
in conjunction with the query
parameter
* to filter the documents that match the constraints specified in the query
parameter.
* Specifying a filter controls only which matching documents are included in the results, it has no effect
* on how they are scored and sorted. The filterQuery
parameter supports the full structured
* query syntax.
*
* For more information about using filters, see Filtering
* Matching Documents in the Amazon CloudSearch Developer Guide.
*/
public void setFilterQuery(String filterQuery) {
this.filterQuery = filterQuery;
}
/**
*
* Specifies a structured query that filters the results of a search without affecting how the results are scored
* and sorted. You use filterQuery
in conjunction with the query
parameter to filter the
* documents that match the constraints specified in the query
parameter. Specifying a filter controls
* only which matching documents are included in the results, it has no effect on how they are scored and sorted.
* The filterQuery
parameter supports the full structured query syntax.
*
*
* For more information about using filters, see Filtering Matching
* Documents in the Amazon CloudSearch Developer Guide.
*
*
* @return Specifies a structured query that filters the results of a search without affecting how the results are
* scored and sorted. You use filterQuery
in conjunction with the query
parameter
* to filter the documents that match the constraints specified in the query
parameter.
* Specifying a filter controls only which matching documents are included in the results, it has no effect
* on how they are scored and sorted. The filterQuery
parameter supports the full structured
* query syntax.
*
* For more information about using filters, see Filtering
* Matching Documents in the Amazon CloudSearch Developer Guide.
*/
public String getFilterQuery() {
return this.filterQuery;
}
/**
*
* Specifies a structured query that filters the results of a search without affecting how the results are scored
* and sorted. You use filterQuery
in conjunction with the query
parameter to filter the
* documents that match the constraints specified in the query
parameter. Specifying a filter controls
* only which matching documents are included in the results, it has no effect on how they are scored and sorted.
* The filterQuery
parameter supports the full structured query syntax.
*
*
* For more information about using filters, see Filtering Matching
* Documents in the Amazon CloudSearch Developer Guide.
*
*
* @param filterQuery
* Specifies a structured query that filters the results of a search without affecting how the results are
* scored and sorted. You use filterQuery
in conjunction with the query
parameter
* to filter the documents that match the constraints specified in the query
parameter.
* Specifying a filter controls only which matching documents are included in the results, it has no effect
* on how they are scored and sorted. The filterQuery
parameter supports the full structured
* query syntax.
*
* For more information about using filters, see Filtering
* Matching Documents in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withFilterQuery(String filterQuery) {
setFilterQuery(filterQuery);
return this;
}
/**
*
* Retrieves highlights for matches in the specified text
or text-array
fields. Each
* specified field must be highlight enabled in the domain configuration. The fields and options are specified in
* JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The default is
* html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want to
* highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default for HTML
* highlights is <em>
. The default for text highlights is *
.
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default for HTML
* highlights is </em>
. The default for text highlights is *
.
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the first match
* is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and title
* fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
*
*
* @param highlight
* Retrieves highlights for matches in the specified text
or text-array
fields.
* Each specified field must be highlight enabled in the domain configuration. The fields and options are
* specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The default
* is html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want
* to highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default
* for HTML highlights is <em>
. The default for text highlights is *
.
*
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default
* for HTML highlights is </em>
. The default for text highlights is *
* .
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the
* first match is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and title
* fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
*/
public void setHighlight(String highlight) {
this.highlight = highlight;
}
/**
*
* Retrieves highlights for matches in the specified text
or text-array
fields. Each
* specified field must be highlight enabled in the domain configuration. The fields and options are specified in
* JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The default is
* html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want to
* highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default for HTML
* highlights is <em>
. The default for text highlights is *
.
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default for HTML
* highlights is </em>
. The default for text highlights is *
.
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the first match
* is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and title
* fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
*
*
* @return Retrieves highlights for matches in the specified text
or text-array
fields.
* Each specified field must be highlight enabled in the domain configuration. The fields and options are
* specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The
* default is html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want
* to highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default
* for HTML highlights is <em>
. The default for text highlights is *
* .
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default
* for HTML highlights is </em>
. The default for text highlights is
* *
.
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the
* first match is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and
* title
fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
*/
public String getHighlight() {
return this.highlight;
}
/**
*
* Retrieves highlights for matches in the specified text
or text-array
fields. Each
* specified field must be highlight enabled in the domain configuration. The fields and options are specified in
* JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The default is
* html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want to
* highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default for HTML
* highlights is <em>
. The default for text highlights is *
.
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default for HTML
* highlights is </em>
. The default for text highlights is *
.
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the first match
* is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and title
* fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
*
*
* @param highlight
* Retrieves highlights for matches in the specified text
or text-array
fields.
* Each specified field must be highlight enabled in the domain configuration. The fields and options are
* specified in JSON using the form
* {"FIELD":{"OPTION":VALUE,"OPTION:"STRING"},"FIELD":{"OPTION":VALUE,"OPTION":"STRING"}}
.
*
* You can specify the following highlight options:
*
*
* -
format
: specifies the format of the data in the text field: text
or
* html
. When data is returned as HTML, all non-alphanumeric characters are encoded. The default
* is html
.
* -
max_phrases
: specifies the maximum number of occurrences of the search term(s) you want
* to highlight. By default, the first occurrence is highlighted.
* -
pre_tag
: specifies the string to prepend to an occurrence of a search term. The default
* for HTML highlights is <em>
. The default for text highlights is *
.
*
* -
post_tag
: specifies the string to append to an occurrence of a search term. The default
* for HTML highlights is </em>
. The default for text highlights is *
* .
*
*
* If no highlight options are specified for a field, the returned field text is treated as HTML and the
* first match is highlighted with emphasis tags: <em>search-term</em>
.
*
*
* For example, the following request retrieves highlights for the actors
and title
* fields.
*
*
* { "actors": {}, "title": {"format": "text","max_phrases": 2,"pre_tag": "","post_tag": ""} }
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withHighlight(String highlight) {
setHighlight(highlight);
return this;
}
/**
*
* Enables partial results to be returned if one or more index partitions are unavailable. When your search index is
* partitioned across multiple search instances, by default Amazon CloudSearch only returns results if every
* partition can be queried. This means that the failure of a single search instance can result in 5xx (internal
* server) errors. When you enable partial results, Amazon CloudSearch returns whatever results are available and
* includes the percentage of documents searched in the search results (percent-searched). This enables you to more
* gracefully degrade your users' search experience. For example, rather than displaying no results, you could
* display the partial results and a message indicating that the results might be incomplete due to a temporary
* system outage.
*
*
* @param partial
* Enables partial results to be returned if one or more index partitions are unavailable. When your search
* index is partitioned across multiple search instances, by default Amazon CloudSearch only returns results
* if every partition can be queried. This means that the failure of a single search instance can result in
* 5xx (internal server) errors. When you enable partial results, Amazon CloudSearch returns whatever results
* are available and includes the percentage of documents searched in the search results (percent-searched).
* This enables you to more gracefully degrade your users' search experience. For example, rather than
* displaying no results, you could display the partial results and a message indicating that the results
* might be incomplete due to a temporary system outage.
*/
public void setPartial(Boolean partial) {
this.partial = partial;
}
/**
*
* Enables partial results to be returned if one or more index partitions are unavailable. When your search index is
* partitioned across multiple search instances, by default Amazon CloudSearch only returns results if every
* partition can be queried. This means that the failure of a single search instance can result in 5xx (internal
* server) errors. When you enable partial results, Amazon CloudSearch returns whatever results are available and
* includes the percentage of documents searched in the search results (percent-searched). This enables you to more
* gracefully degrade your users' search experience. For example, rather than displaying no results, you could
* display the partial results and a message indicating that the results might be incomplete due to a temporary
* system outage.
*
*
* @return Enables partial results to be returned if one or more index partitions are unavailable. When your search
* index is partitioned across multiple search instances, by default Amazon CloudSearch only returns results
* if every partition can be queried. This means that the failure of a single search instance can result in
* 5xx (internal server) errors. When you enable partial results, Amazon CloudSearch returns whatever
* results are available and includes the percentage of documents searched in the search results
* (percent-searched). This enables you to more gracefully degrade your users' search experience. For
* example, rather than displaying no results, you could display the partial results and a message
* indicating that the results might be incomplete due to a temporary system outage.
*/
public Boolean getPartial() {
return this.partial;
}
/**
*
* Enables partial results to be returned if one or more index partitions are unavailable. When your search index is
* partitioned across multiple search instances, by default Amazon CloudSearch only returns results if every
* partition can be queried. This means that the failure of a single search instance can result in 5xx (internal
* server) errors. When you enable partial results, Amazon CloudSearch returns whatever results are available and
* includes the percentage of documents searched in the search results (percent-searched). This enables you to more
* gracefully degrade your users' search experience. For example, rather than displaying no results, you could
* display the partial results and a message indicating that the results might be incomplete due to a temporary
* system outage.
*
*
* @param partial
* Enables partial results to be returned if one or more index partitions are unavailable. When your search
* index is partitioned across multiple search instances, by default Amazon CloudSearch only returns results
* if every partition can be queried. This means that the failure of a single search instance can result in
* 5xx (internal server) errors. When you enable partial results, Amazon CloudSearch returns whatever results
* are available and includes the percentage of documents searched in the search results (percent-searched).
* This enables you to more gracefully degrade your users' search experience. For example, rather than
* displaying no results, you could display the partial results and a message indicating that the results
* might be incomplete due to a temporary system outage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withPartial(Boolean partial) {
setPartial(partial);
return this;
}
/**
*
* Enables partial results to be returned if one or more index partitions are unavailable. When your search index is
* partitioned across multiple search instances, by default Amazon CloudSearch only returns results if every
* partition can be queried. This means that the failure of a single search instance can result in 5xx (internal
* server) errors. When you enable partial results, Amazon CloudSearch returns whatever results are available and
* includes the percentage of documents searched in the search results (percent-searched). This enables you to more
* gracefully degrade your users' search experience. For example, rather than displaying no results, you could
* display the partial results and a message indicating that the results might be incomplete due to a temporary
* system outage.
*
*
* @return Enables partial results to be returned if one or more index partitions are unavailable. When your search
* index is partitioned across multiple search instances, by default Amazon CloudSearch only returns results
* if every partition can be queried. This means that the failure of a single search instance can result in
* 5xx (internal server) errors. When you enable partial results, Amazon CloudSearch returns whatever
* results are available and includes the percentage of documents searched in the search results
* (percent-searched). This enables you to more gracefully degrade your users' search experience. For
* example, rather than displaying no results, you could display the partial results and a message
* indicating that the results might be incomplete due to a temporary system outage.
*/
public Boolean isPartial() {
return this.partial;
}
/**
*
* Specifies the search criteria for the request. How you specify the search criteria depends on the query parser
* used for the request and the parser options specified in the queryOptions
parameter. By default, the
* simple
query parser is used to process requests. To use the structured
,
* lucene
, or dismax
query parser, you must also specify the queryParser
* parameter.
*
*
* For more information about specifying search criteria, see Searching Your Data in the
* Amazon CloudSearch Developer Guide.
*
*
* @param query
* Specifies the search criteria for the request. How you specify the search criteria depends on the query
* parser used for the request and the parser options specified in the queryOptions
parameter.
* By default, the simple
query parser is used to process requests. To use the
* structured
, lucene
, or dismax
query parser, you must also specify
* the queryParser
parameter.
*
* For more information about specifying search criteria, see Searching Your Data
* in the Amazon CloudSearch Developer Guide.
*/
public void setQuery(String query) {
this.query = query;
}
/**
*
* Specifies the search criteria for the request. How you specify the search criteria depends on the query parser
* used for the request and the parser options specified in the queryOptions
parameter. By default, the
* simple
query parser is used to process requests. To use the structured
,
* lucene
, or dismax
query parser, you must also specify the queryParser
* parameter.
*
*
* For more information about specifying search criteria, see Searching Your Data in the
* Amazon CloudSearch Developer Guide.
*
*
* @return Specifies the search criteria for the request. How you specify the search criteria depends on the query
* parser used for the request and the parser options specified in the queryOptions
parameter.
* By default, the simple
query parser is used to process requests. To use the
* structured
, lucene
, or dismax
query parser, you must also specify
* the queryParser
parameter.
*
* For more information about specifying search criteria, see Searching Your
* Data in the Amazon CloudSearch Developer Guide.
*/
public String getQuery() {
return this.query;
}
/**
*
* Specifies the search criteria for the request. How you specify the search criteria depends on the query parser
* used for the request and the parser options specified in the queryOptions
parameter. By default, the
* simple
query parser is used to process requests. To use the structured
,
* lucene
, or dismax
query parser, you must also specify the queryParser
* parameter.
*
*
* For more information about specifying search criteria, see Searching Your Data in the
* Amazon CloudSearch Developer Guide.
*
*
* @param query
* Specifies the search criteria for the request. How you specify the search criteria depends on the query
* parser used for the request and the parser options specified in the queryOptions
parameter.
* By default, the simple
query parser is used to process requests. To use the
* structured
, lucene
, or dismax
query parser, you must also specify
* the queryParser
parameter.
*
* For more information about specifying search criteria, see Searching Your Data
* in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withQuery(String query) {
setQuery(query);
return this;
}
/**
*
* Configures options for the query parser specified in the queryParser
parameter. You specify the
* options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search string. For
* example: defaultOperator: 'or'
. For the dismax
parser, you specify a percentage that
* represents the percentage of terms in the search string (rounded down) that must match, rather than a default
* operator. A value of 0%
is the equivalent to OR, and a value of 100%
is equivalent to
* AND. The percentage must be specified as a value in the range 0-100 followed by the percent (%) symbol. For
* example, defaultOperator: 50%
. Valid values: and
, or
, a percentage in the
* range 0%-100% (dismax
). Default: and
(simple
, structured
,
* lucene
) or 100
(dismax
). Valid for: simple
,
* structured
, lucene
, and dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no fields
* are specified in a search and this option is not specified, all text and text-array fields are searched. You can
* specify a weight for each field to control the relative importance of each field when Amazon CloudSearch
* calculates relevance scores. To specify a field weight, append a caret (^
) symbol and the weight to
* the field name. For example, to boost the importance of the title
field over the
* description
field you could specify: "fields":["title^5","description"]
. Valid values:
* The name of any configured field and an optional numeric value greater than zero. Default: All text
* and text-array
fields. Valid for: simple
, structured
, lucene
,
* and dismax
.
* operators
: An array of the operators or special characters you want to disable for the simple
* query parser. If you disable the and
, or
, or not
operators, the
* corresponding operators (+
, |
, -
) have no special meaning and are dropped
* from the search string. Similarly, disabling prefix
disables the wildcard operator (*
)
* and disabling phrase
disables the ability to search for phrases by enclosing phrases in double
* quotes. Disabling precedence disables the ability to control order of precedence using parentheses. Disabling
* near
disables the ability to use the ~ operator to perform a sloppy phrase search. Disabling the
* fuzzy
operator disables the ability to use the ~ operator to perform a fuzzy search.
* escape
disables the ability to use a backslash (\
) to escape special characters within
* the search string. Disabling whitespace is an advanced option that prevents the parser from tokenizing on
* whitespace, which can be useful for Vietnamese. (It prevents Vietnamese words from being split incorrectly.) For
* example, you could disable all operators other than the phrase operator to support just simple term and phrase
* queries: "operators":["and","not","or", "prefix"]
. Valid values: and
,
* escape
, fuzzy
, near
, not
, or
,
* phrase
, precedence
, prefix
, whitespace
. Default: All
* operators and special characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you want to
* use for phrase searches. When the terms in the search string appear in close proximity within a field, the field
* scores higher. You can specify a weight for each field to boost that score. The phraseSlop
option
* controls how much the matches can deviate from the search string and still be boosted. To specify a field weight,
* append a caret (^
) symbol and the weight to the field name. For example, to boost phrase matches in
* the title
field over the abstract
field, you could specify:
* "phraseFields":["title^3", "plot"]
Valid values: The name of any text
or
* text-array
field and an optional numeric value greater than zero. Default: No fields. If you don't
* specify any fields with phraseFields
, proximity scoring is disabled even if phraseSlop
* is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search phrase
* and still be boosted according to the weights specified in the phraseFields
option; for example,
* phraseSlop: 2
. You must also specify phraseFields
to enable proximity scoring. Valid
* values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from the search
* phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed this proximity
* distance are not considered a match.) For example, to specify a slop of three for dismax phrase queries, you
* would specify "explicitPhraseSlop":3
. Valid values: positive integers. Default: 0. Valid for:
* dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If the term
* occurs in multiple fields within a document, by default only the highest scoring field contributes to the
* document's overall score. You can specify a tieBreaker
value to enable the matches in lower-scoring
* fields to contribute to the document's score. That way, if two documents have the same max field score for a
* particular term, the score for the document that has matches in more fields will be higher. The formula for
* calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
. Set
* tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum): "tieBreaker":1
.
* Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
*
*
* @param queryOptions
* Configures options for the query parser specified in the queryParser
parameter. You specify
* the options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search
* string. For example: defaultOperator: 'or'
. For the dismax
parser, you specify a
* percentage that represents the percentage of terms in the search string (rounded down) that must match,
* rather than a default operator. A value of 0%
is the equivalent to OR, and a value of
* 100%
is equivalent to AND. The percentage must be specified as a value in the range 0-100
* followed by the percent (%) symbol. For example, defaultOperator: 50%
. Valid values:
* and
, or
, a percentage in the range 0%-100% (dismax
). Default:
* and
(simple
, structured
, lucene
) or 100
(
* dismax
). Valid for: simple
, structured
, lucene
, and
* dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no
* fields are specified in a search and this option is not specified, all text and text-array fields are
* searched. You can specify a weight for each field to control the relative importance of each field when
* Amazon CloudSearch calculates relevance scores. To specify a field weight, append a caret (^
)
* symbol and the weight to the field name. For example, to boost the importance of the title
* field over the description
field you could specify:
* "fields":["title^5","description"]
. Valid values: The name of any configured field and an
* optional numeric value greater than zero. Default: All text
and text-array
* fields. Valid for: simple
, structured
, lucene
, and
* dismax
.
* operators
: An array of the operators or special characters you want to disable for the
* simple query parser. If you disable the and
, or
, or not
operators,
* the corresponding operators (+
, |
, -
) have no special meaning and
* are dropped from the search string. Similarly, disabling prefix
disables the wildcard
* operator (*
) and disabling phrase
disables the ability to search for phrases by
* enclosing phrases in double quotes. Disabling precedence disables the ability to control order of
* precedence using parentheses. Disabling near
disables the ability to use the ~ operator to
* perform a sloppy phrase search. Disabling the fuzzy
operator disables the ability to use the
* ~ operator to perform a fuzzy search. escape
disables the ability to use a backslash (
* \
) to escape special characters within the search string. Disabling whitespace is an advanced
* option that prevents the parser from tokenizing on whitespace, which can be useful for Vietnamese. (It
* prevents Vietnamese words from being split incorrectly.) For example, you could disable all operators
* other than the phrase operator to support just simple term and phrase queries:
* "operators":["and","not","or", "prefix"]
. Valid values: and
, escape
, fuzzy
, near
, not
, or
, phrase
,
* precedence
, prefix
, whitespace
. Default: All operators and special
* characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you
* want to use for phrase searches. When the terms in the search string appear in close proximity within a
* field, the field scores higher. You can specify a weight for each field to boost that score. The
* phraseSlop
option controls how much the matches can deviate from the search string and still
* be boosted. To specify a field weight, append a caret (^
) symbol and the weight to the field
* name. For example, to boost phrase matches in the title
field over the abstract
* field, you could specify: "phraseFields":["title^3", "plot"]
Valid values: The name of any
* text
or text-array
field and an optional numeric value greater than zero.
* Default: No fields. If you don't specify any fields with phraseFields
, proximity scoring is
* disabled even if phraseSlop
is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search
* phrase and still be boosted according to the weights specified in the phraseFields
option;
* for example, phraseSlop: 2
. You must also specify phraseFields
to enable
* proximity scoring. Valid values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from the
* search phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed this
* proximity distance are not considered a match.) For example, to specify a slop of three for dismax phrase
* queries, you would specify "explicitPhraseSlop":3
. Valid values: positive integers. Default:
* 0. Valid for: dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If
* the term occurs in multiple fields within a document, by default only the highest scoring field
* contributes to the document's overall score. You can specify a tieBreaker
value to enable the
* matches in lower-scoring fields to contribute to the document's score. That way, if two documents have the
* same max field score for a particular term, the score for the document that has matches in more fields
* will be higher. The formula for calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
.
* Set tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum):
* "tieBreaker":1
. Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
*/
public void setQueryOptions(String queryOptions) {
this.queryOptions = queryOptions;
}
/**
*
* Configures options for the query parser specified in the queryParser
parameter. You specify the
* options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search string. For
* example: defaultOperator: 'or'
. For the dismax
parser, you specify a percentage that
* represents the percentage of terms in the search string (rounded down) that must match, rather than a default
* operator. A value of 0%
is the equivalent to OR, and a value of 100%
is equivalent to
* AND. The percentage must be specified as a value in the range 0-100 followed by the percent (%) symbol. For
* example, defaultOperator: 50%
. Valid values: and
, or
, a percentage in the
* range 0%-100% (dismax
). Default: and
(simple
, structured
,
* lucene
) or 100
(dismax
). Valid for: simple
,
* structured
, lucene
, and dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no fields
* are specified in a search and this option is not specified, all text and text-array fields are searched. You can
* specify a weight for each field to control the relative importance of each field when Amazon CloudSearch
* calculates relevance scores. To specify a field weight, append a caret (^
) symbol and the weight to
* the field name. For example, to boost the importance of the title
field over the
* description
field you could specify: "fields":["title^5","description"]
. Valid values:
* The name of any configured field and an optional numeric value greater than zero. Default: All text
* and text-array
fields. Valid for: simple
, structured
, lucene
,
* and dismax
.
* operators
: An array of the operators or special characters you want to disable for the simple
* query parser. If you disable the and
, or
, or not
operators, the
* corresponding operators (+
, |
, -
) have no special meaning and are dropped
* from the search string. Similarly, disabling prefix
disables the wildcard operator (*
)
* and disabling phrase
disables the ability to search for phrases by enclosing phrases in double
* quotes. Disabling precedence disables the ability to control order of precedence using parentheses. Disabling
* near
disables the ability to use the ~ operator to perform a sloppy phrase search. Disabling the
* fuzzy
operator disables the ability to use the ~ operator to perform a fuzzy search.
* escape
disables the ability to use a backslash (\
) to escape special characters within
* the search string. Disabling whitespace is an advanced option that prevents the parser from tokenizing on
* whitespace, which can be useful for Vietnamese. (It prevents Vietnamese words from being split incorrectly.) For
* example, you could disable all operators other than the phrase operator to support just simple term and phrase
* queries: "operators":["and","not","or", "prefix"]
. Valid values: and
,
* escape
, fuzzy
, near
, not
, or
,
* phrase
, precedence
, prefix
, whitespace
. Default: All
* operators and special characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you want to
* use for phrase searches. When the terms in the search string appear in close proximity within a field, the field
* scores higher. You can specify a weight for each field to boost that score. The phraseSlop
option
* controls how much the matches can deviate from the search string and still be boosted. To specify a field weight,
* append a caret (^
) symbol and the weight to the field name. For example, to boost phrase matches in
* the title
field over the abstract
field, you could specify:
* "phraseFields":["title^3", "plot"]
Valid values: The name of any text
or
* text-array
field and an optional numeric value greater than zero. Default: No fields. If you don't
* specify any fields with phraseFields
, proximity scoring is disabled even if phraseSlop
* is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search phrase
* and still be boosted according to the weights specified in the phraseFields
option; for example,
* phraseSlop: 2
. You must also specify phraseFields
to enable proximity scoring. Valid
* values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from the search
* phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed this proximity
* distance are not considered a match.) For example, to specify a slop of three for dismax phrase queries, you
* would specify "explicitPhraseSlop":3
. Valid values: positive integers. Default: 0. Valid for:
* dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If the term
* occurs in multiple fields within a document, by default only the highest scoring field contributes to the
* document's overall score. You can specify a tieBreaker
value to enable the matches in lower-scoring
* fields to contribute to the document's score. That way, if two documents have the same max field score for a
* particular term, the score for the document that has matches in more fields will be higher. The formula for
* calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
. Set
* tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum): "tieBreaker":1
.
* Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
*
*
* @return Configures options for the query parser specified in the queryParser
parameter. You specify
* the options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search
* string. For example: defaultOperator: 'or'
. For the dismax
parser, you specify
* a percentage that represents the percentage of terms in the search string (rounded down) that must match,
* rather than a default operator. A value of 0%
is the equivalent to OR, and a value of
* 100%
is equivalent to AND. The percentage must be specified as a value in the range 0-100
* followed by the percent (%) symbol. For example, defaultOperator: 50%
. Valid values:
* and
, or
, a percentage in the range 0%-100% (dismax
). Default:
* and
(simple
, structured
, lucene
) or 100
* (dismax
). Valid for: simple
, structured
, lucene
, and
* dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no
* fields are specified in a search and this option is not specified, all text and text-array fields are
* searched. You can specify a weight for each field to control the relative importance of each field when
* Amazon CloudSearch calculates relevance scores. To specify a field weight, append a caret (^
* ) symbol and the weight to the field name. For example, to boost the importance of the title
* field over the description
field you could specify:
* "fields":["title^5","description"]
. Valid values: The name of any configured field and an
* optional numeric value greater than zero. Default: All text
and text-array
* fields. Valid for: simple
, structured
, lucene
, and
* dismax
.
* operators
: An array of the operators or special characters you want to disable for the
* simple query parser. If you disable the and
, or
, or not
operators,
* the corresponding operators (+
, |
, -
) have no special meaning and
* are dropped from the search string. Similarly, disabling prefix
disables the wildcard
* operator (*
) and disabling phrase
disables the ability to search for phrases by
* enclosing phrases in double quotes. Disabling precedence disables the ability to control order of
* precedence using parentheses. Disabling near
disables the ability to use the ~ operator to
* perform a sloppy phrase search. Disabling the fuzzy
operator disables the ability to use the
* ~ operator to perform a fuzzy search. escape
disables the ability to use a backslash (
* \
) to escape special characters within the search string. Disabling whitespace is an
* advanced option that prevents the parser from tokenizing on whitespace, which can be useful for
* Vietnamese. (It prevents Vietnamese words from being split incorrectly.) For example, you could disable
* all operators other than the phrase operator to support just simple term and phrase queries:
* "operators":["and","not","or", "prefix"]
. Valid values: and
,
* escape
, fuzzy
, near
, not
, or
,
* phrase
, precedence
, prefix
, whitespace
. Default: All
* operators and special characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you
* want to use for phrase searches. When the terms in the search string appear in close proximity within a
* field, the field scores higher. You can specify a weight for each field to boost that score. The
* phraseSlop
option controls how much the matches can deviate from the search string and still
* be boosted. To specify a field weight, append a caret (^
) symbol and the weight to the field
* name. For example, to boost phrase matches in the title
field over the abstract
* field, you could specify: "phraseFields":["title^3", "plot"]
Valid values: The name of any
* text
or text-array
field and an optional numeric value greater than zero.
* Default: No fields. If you don't specify any fields with phraseFields
, proximity scoring is
* disabled even if phraseSlop
is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search
* phrase and still be boosted according to the weights specified in the phraseFields
option;
* for example, phraseSlop: 2
. You must also specify phraseFields
to enable
* proximity scoring. Valid values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from
* the search phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed
* this proximity distance are not considered a match.) For example, to specify a slop of three for dismax
* phrase queries, you would specify "explicitPhraseSlop":3
. Valid values: positive integers.
* Default: 0. Valid for: dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If
* the term occurs in multiple fields within a document, by default only the highest scoring field
* contributes to the document's overall score. You can specify a tieBreaker
value to enable
* the matches in lower-scoring fields to contribute to the document's score. That way, if two documents
* have the same max field score for a particular term, the score for the document that has matches in more
* fields will be higher. The formula for calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
.
* Set tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum):
* "tieBreaker":1
. Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
*/
public String getQueryOptions() {
return this.queryOptions;
}
/**
*
* Configures options for the query parser specified in the queryParser
parameter. You specify the
* options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search string. For
* example: defaultOperator: 'or'
. For the dismax
parser, you specify a percentage that
* represents the percentage of terms in the search string (rounded down) that must match, rather than a default
* operator. A value of 0%
is the equivalent to OR, and a value of 100%
is equivalent to
* AND. The percentage must be specified as a value in the range 0-100 followed by the percent (%) symbol. For
* example, defaultOperator: 50%
. Valid values: and
, or
, a percentage in the
* range 0%-100% (dismax
). Default: and
(simple
, structured
,
* lucene
) or 100
(dismax
). Valid for: simple
,
* structured
, lucene
, and dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no fields
* are specified in a search and this option is not specified, all text and text-array fields are searched. You can
* specify a weight for each field to control the relative importance of each field when Amazon CloudSearch
* calculates relevance scores. To specify a field weight, append a caret (^
) symbol and the weight to
* the field name. For example, to boost the importance of the title
field over the
* description
field you could specify: "fields":["title^5","description"]
. Valid values:
* The name of any configured field and an optional numeric value greater than zero. Default: All text
* and text-array
fields. Valid for: simple
, structured
, lucene
,
* and dismax
.
* operators
: An array of the operators or special characters you want to disable for the simple
* query parser. If you disable the and
, or
, or not
operators, the
* corresponding operators (+
, |
, -
) have no special meaning and are dropped
* from the search string. Similarly, disabling prefix
disables the wildcard operator (*
)
* and disabling phrase
disables the ability to search for phrases by enclosing phrases in double
* quotes. Disabling precedence disables the ability to control order of precedence using parentheses. Disabling
* near
disables the ability to use the ~ operator to perform a sloppy phrase search. Disabling the
* fuzzy
operator disables the ability to use the ~ operator to perform a fuzzy search.
* escape
disables the ability to use a backslash (\
) to escape special characters within
* the search string. Disabling whitespace is an advanced option that prevents the parser from tokenizing on
* whitespace, which can be useful for Vietnamese. (It prevents Vietnamese words from being split incorrectly.) For
* example, you could disable all operators other than the phrase operator to support just simple term and phrase
* queries: "operators":["and","not","or", "prefix"]
. Valid values: and
,
* escape
, fuzzy
, near
, not
, or
,
* phrase
, precedence
, prefix
, whitespace
. Default: All
* operators and special characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you want to
* use for phrase searches. When the terms in the search string appear in close proximity within a field, the field
* scores higher. You can specify a weight for each field to boost that score. The phraseSlop
option
* controls how much the matches can deviate from the search string and still be boosted. To specify a field weight,
* append a caret (^
) symbol and the weight to the field name. For example, to boost phrase matches in
* the title
field over the abstract
field, you could specify:
* "phraseFields":["title^3", "plot"]
Valid values: The name of any text
or
* text-array
field and an optional numeric value greater than zero. Default: No fields. If you don't
* specify any fields with phraseFields
, proximity scoring is disabled even if phraseSlop
* is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search phrase
* and still be boosted according to the weights specified in the phraseFields
option; for example,
* phraseSlop: 2
. You must also specify phraseFields
to enable proximity scoring. Valid
* values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from the search
* phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed this proximity
* distance are not considered a match.) For example, to specify a slop of three for dismax phrase queries, you
* would specify "explicitPhraseSlop":3
. Valid values: positive integers. Default: 0. Valid for:
* dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If the term
* occurs in multiple fields within a document, by default only the highest scoring field contributes to the
* document's overall score. You can specify a tieBreaker
value to enable the matches in lower-scoring
* fields to contribute to the document's score. That way, if two documents have the same max field score for a
* particular term, the score for the document that has matches in more fields will be higher. The formula for
* calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
. Set
* tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum): "tieBreaker":1
.
* Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
*
*
* @param queryOptions
* Configures options for the query parser specified in the queryParser
parameter. You specify
* the options in JSON using the following form
* {"OPTION1":"VALUE1","OPTION2":VALUE2"..."OPTIONN":"VALUEN"}.
*
* The options you can configure vary according to which parser you use:
*
*
* defaultOperator
: The default operator used to combine individual terms in the search
* string. For example: defaultOperator: 'or'
. For the dismax
parser, you specify a
* percentage that represents the percentage of terms in the search string (rounded down) that must match,
* rather than a default operator. A value of 0%
is the equivalent to OR, and a value of
* 100%
is equivalent to AND. The percentage must be specified as a value in the range 0-100
* followed by the percent (%) symbol. For example, defaultOperator: 50%
. Valid values:
* and
, or
, a percentage in the range 0%-100% (dismax
). Default:
* and
(simple
, structured
, lucene
) or 100
(
* dismax
). Valid for: simple
, structured
, lucene
, and
* dismax
.
* fields
: An array of the fields to search when no fields are specified in a search. If no
* fields are specified in a search and this option is not specified, all text and text-array fields are
* searched. You can specify a weight for each field to control the relative importance of each field when
* Amazon CloudSearch calculates relevance scores. To specify a field weight, append a caret (^
)
* symbol and the weight to the field name. For example, to boost the importance of the title
* field over the description
field you could specify:
* "fields":["title^5","description"]
. Valid values: The name of any configured field and an
* optional numeric value greater than zero. Default: All text
and text-array
* fields. Valid for: simple
, structured
, lucene
, and
* dismax
.
* operators
: An array of the operators or special characters you want to disable for the
* simple query parser. If you disable the and
, or
, or not
operators,
* the corresponding operators (+
, |
, -
) have no special meaning and
* are dropped from the search string. Similarly, disabling prefix
disables the wildcard
* operator (*
) and disabling phrase
disables the ability to search for phrases by
* enclosing phrases in double quotes. Disabling precedence disables the ability to control order of
* precedence using parentheses. Disabling near
disables the ability to use the ~ operator to
* perform a sloppy phrase search. Disabling the fuzzy
operator disables the ability to use the
* ~ operator to perform a fuzzy search. escape
disables the ability to use a backslash (
* \
) to escape special characters within the search string. Disabling whitespace is an advanced
* option that prevents the parser from tokenizing on whitespace, which can be useful for Vietnamese. (It
* prevents Vietnamese words from being split incorrectly.) For example, you could disable all operators
* other than the phrase operator to support just simple term and phrase queries:
* "operators":["and","not","or", "prefix"]
. Valid values: and
, escape
, fuzzy
, near
, not
, or
, phrase
,
* precedence
, prefix
, whitespace
. Default: All operators and special
* characters are enabled. Valid for: simple
.
* phraseFields
: An array of the text
or text-array
fields you
* want to use for phrase searches. When the terms in the search string appear in close proximity within a
* field, the field scores higher. You can specify a weight for each field to boost that score. The
* phraseSlop
option controls how much the matches can deviate from the search string and still
* be boosted. To specify a field weight, append a caret (^
) symbol and the weight to the field
* name. For example, to boost phrase matches in the title
field over the abstract
* field, you could specify: "phraseFields":["title^3", "plot"]
Valid values: The name of any
* text
or text-array
field and an optional numeric value greater than zero.
* Default: No fields. If you don't specify any fields with phraseFields
, proximity scoring is
* disabled even if phraseSlop
is specified. Valid for: dismax
.
* phraseSlop
: An integer value that specifies how much matches can deviate from the search
* phrase and still be boosted according to the weights specified in the phraseFields
option;
* for example, phraseSlop: 2
. You must also specify phraseFields
to enable
* proximity scoring. Valid values: positive integers. Default: 0. Valid for: dismax
.
* explicitPhraseSlop
: An integer value that specifies how much a match can deviate from the
* search phrase when the phrase is enclosed in double quotes in the search string. (Phrases that exceed this
* proximity distance are not considered a match.) For example, to specify a slop of three for dismax phrase
* queries, you would specify "explicitPhraseSlop":3
. Valid values: positive integers. Default:
* 0. Valid for: dismax
.
* tieBreaker
: When a term in the search string is found in a document's field, a score is
* calculated for that field based on how common the word is in that field compared to other documents. If
* the term occurs in multiple fields within a document, by default only the highest scoring field
* contributes to the document's overall score. You can specify a tieBreaker
value to enable the
* matches in lower-scoring fields to contribute to the document's score. That way, if two documents have the
* same max field score for a particular term, the score for the document that has matches in more fields
* will be higher. The formula for calculating the score with a tieBreaker is
* (max field score) + (tieBreaker) * (sum of the scores for the rest of the matching fields)
.
* Set tieBreaker
to 0 to disregard all but the highest scoring field (pure max):
* "tieBreaker":0
. Set to 1 to sum the scores from all fields (pure sum):
* "tieBreaker":1
. Valid values: 0.0 to 1.0. Default: 0.0. Valid for: dismax
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withQueryOptions(String queryOptions) {
setQueryOptions(queryOptions);
return this;
}
/**
*
* Specifies which query parser to use to process the request. If queryParser
is not specified, Amazon
* CloudSearch uses the simple
query parser.
*
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields. By
* default, the simple
query parser searches all text
and text-array
fields.
* You can specify which fields to search by with the queryOptions
parameter. If you prefix a search
* term with a plus sign (+) documents must contain the term to be considered a match. (This is the default, unless
* you configure the default operator with the queryOptions
parameter.) You can use the -
* (NOT), |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than individual
* terms, enclose the phrase in double quotes. For more information, see Searching for Text in
* the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the search
* criteria. You can also search within particular fields, search for values and ranges of values, and use advanced
* options such as term boosting, matchall
, and near
. For more information, see Constructing
* Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax defined by
* the DisMax query parser. For more information, see DisMax Query Parser Syntax.
*
*
* @param queryParser
* Specifies which query parser to use to process the request. If queryParser
is not specified,
* Amazon CloudSearch uses the simple
query parser.
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields.
* By default, the simple
query parser searches all text
and
* text-array
fields. You can specify which fields to search by with the
* queryOptions
parameter. If you prefix a search term with a plus sign (+) documents must
* contain the term to be considered a match. (This is the default, unless you configure the default operator
* with the queryOptions
parameter.) You can use the -
(NOT), |
(OR),
* and *
(wildcard) operators to exclude particular terms, find results that match any of the
* specified terms, or search for a prefix. To search for a phrase rather than individual terms, enclose the
* phrase in double quotes. For more information, see Searching for
* Text in the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the
* search criteria. You can also search within particular fields, search for values and ranges of values, and
* use advanced options such as term boosting, matchall
, and near
. For more
* information, see Constructing Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax
* defined by the DisMax query parser. For more information, see DisMax Query Parser Syntax.
* @see QueryParser
*/
public void setQueryParser(String queryParser) {
this.queryParser = queryParser;
}
/**
*
* Specifies which query parser to use to process the request. If queryParser
is not specified, Amazon
* CloudSearch uses the simple
query parser.
*
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields. By
* default, the simple
query parser searches all text
and text-array
fields.
* You can specify which fields to search by with the queryOptions
parameter. If you prefix a search
* term with a plus sign (+) documents must contain the term to be considered a match. (This is the default, unless
* you configure the default operator with the queryOptions
parameter.) You can use the -
* (NOT), |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than individual
* terms, enclose the phrase in double quotes. For more information, see Searching for Text in
* the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the search
* criteria. You can also search within particular fields, search for values and ranges of values, and use advanced
* options such as term boosting, matchall
, and near
. For more information, see Constructing
* Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax defined by
* the DisMax query parser. For more information, see DisMax Query Parser Syntax.
*
*
* @return Specifies which query parser to use to process the request. If queryParser
is not specified,
* Amazon CloudSearch uses the simple
query parser.
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields.
* By default, the simple
query parser searches all text
and
* text-array
fields. You can specify which fields to search by with the
* queryOptions
parameter. If you prefix a search term with a plus sign (+) documents must
* contain the term to be considered a match. (This is the default, unless you configure the default
* operator with the queryOptions
parameter.) You can use the -
(NOT),
* |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than
* individual terms, enclose the phrase in double quotes. For more information, see Searching for
* Text in the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the
* search criteria. You can also search within particular fields, search for values and ranges of values,
* and use advanced options such as term boosting, matchall
, and near
. For more
* information, see Constructing Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax
* defined by the DisMax query parser. For more information, see DisMax Query Parser Syntax.
* @see QueryParser
*/
public String getQueryParser() {
return this.queryParser;
}
/**
*
* Specifies which query parser to use to process the request. If queryParser
is not specified, Amazon
* CloudSearch uses the simple
query parser.
*
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields. By
* default, the simple
query parser searches all text
and text-array
fields.
* You can specify which fields to search by with the queryOptions
parameter. If you prefix a search
* term with a plus sign (+) documents must contain the term to be considered a match. (This is the default, unless
* you configure the default operator with the queryOptions
parameter.) You can use the -
* (NOT), |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than individual
* terms, enclose the phrase in double quotes. For more information, see Searching for Text in
* the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the search
* criteria. You can also search within particular fields, search for values and ranges of values, and use advanced
* options such as term boosting, matchall
, and near
. For more information, see Constructing
* Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax defined by
* the DisMax query parser. For more information, see DisMax Query Parser Syntax.
*
*
* @param queryParser
* Specifies which query parser to use to process the request. If queryParser
is not specified,
* Amazon CloudSearch uses the simple
query parser.
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields.
* By default, the simple
query parser searches all text
and
* text-array
fields. You can specify which fields to search by with the
* queryOptions
parameter. If you prefix a search term with a plus sign (+) documents must
* contain the term to be considered a match. (This is the default, unless you configure the default operator
* with the queryOptions
parameter.) You can use the -
(NOT), |
(OR),
* and *
(wildcard) operators to exclude particular terms, find results that match any of the
* specified terms, or search for a prefix. To search for a phrase rather than individual terms, enclose the
* phrase in double quotes. For more information, see Searching for
* Text in the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the
* search criteria. You can also search within particular fields, search for values and ranges of values, and
* use advanced options such as term boosting, matchall
, and near
. For more
* information, see Constructing Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax
* defined by the DisMax query parser. For more information, see DisMax Query Parser Syntax.
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryParser
*/
public SearchRequest withQueryParser(String queryParser) {
setQueryParser(queryParser);
return this;
}
/**
*
* Specifies which query parser to use to process the request. If queryParser
is not specified, Amazon
* CloudSearch uses the simple
query parser.
*
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields. By
* default, the simple
query parser searches all text
and text-array
fields.
* You can specify which fields to search by with the queryOptions
parameter. If you prefix a search
* term with a plus sign (+) documents must contain the term to be considered a match. (This is the default, unless
* you configure the default operator with the queryOptions
parameter.) You can use the -
* (NOT), |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than individual
* terms, enclose the phrase in double quotes. For more information, see Searching for Text in
* the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the search
* criteria. You can also search within particular fields, search for values and ranges of values, and use advanced
* options such as term boosting, matchall
, and near
. For more information, see Constructing
* Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax defined by
* the DisMax query parser. For more information, see DisMax Query Parser Syntax.
*
*
* @param queryParser
* Specifies which query parser to use to process the request. If queryParser
is not specified,
* Amazon CloudSearch uses the simple
query parser.
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields.
* By default, the simple
query parser searches all text
and
* text-array
fields. You can specify which fields to search by with the
* queryOptions
parameter. If you prefix a search term with a plus sign (+) documents must
* contain the term to be considered a match. (This is the default, unless you configure the default operator
* with the queryOptions
parameter.) You can use the -
(NOT), |
(OR),
* and *
(wildcard) operators to exclude particular terms, find results that match any of the
* specified terms, or search for a prefix. To search for a phrase rather than individual terms, enclose the
* phrase in double quotes. For more information, see Searching for
* Text in the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the
* search criteria. You can also search within particular fields, search for values and ranges of values, and
* use advanced options such as term boosting, matchall
, and near
. For more
* information, see Constructing Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax
* defined by the DisMax query parser. For more information, see DisMax Query Parser Syntax.
* @see QueryParser
*/
public void setQueryParser(QueryParser queryParser) {
withQueryParser(queryParser);
}
/**
*
* Specifies which query parser to use to process the request. If queryParser
is not specified, Amazon
* CloudSearch uses the simple
query parser.
*
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields. By
* default, the simple
query parser searches all text
and text-array
fields.
* You can specify which fields to search by with the queryOptions
parameter. If you prefix a search
* term with a plus sign (+) documents must contain the term to be considered a match. (This is the default, unless
* you configure the default operator with the queryOptions
parameter.) You can use the -
* (NOT), |
(OR), and *
(wildcard) operators to exclude particular terms, find results
* that match any of the specified terms, or search for a prefix. To search for a phrase rather than individual
* terms, enclose the phrase in double quotes. For more information, see Searching for Text in
* the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the search
* criteria. You can also search within particular fields, search for values and ranges of values, and use advanced
* options such as term boosting, matchall
, and near
. For more information, see Constructing
* Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax defined by
* the DisMax query parser. For more information, see DisMax Query Parser Syntax.
*
*
* @param queryParser
* Specifies which query parser to use to process the request. If queryParser
is not specified,
* Amazon CloudSearch uses the simple
query parser.
*
* Amazon CloudSearch supports four query parsers:
*
*
* -
simple
: perform simple searches of text
and text-array
fields.
* By default, the simple
query parser searches all text
and
* text-array
fields. You can specify which fields to search by with the
* queryOptions
parameter. If you prefix a search term with a plus sign (+) documents must
* contain the term to be considered a match. (This is the default, unless you configure the default operator
* with the queryOptions
parameter.) You can use the -
(NOT), |
(OR),
* and *
(wildcard) operators to exclude particular terms, find results that match any of the
* specified terms, or search for a prefix. To search for a phrase rather than individual terms, enclose the
* phrase in double quotes. For more information, see Searching for
* Text in the Amazon CloudSearch Developer Guide.
* -
structured
: perform advanced searches by combining multiple expressions to define the
* search criteria. You can also search within particular fields, search for values and ranges of values, and
* use advanced options such as term boosting, matchall
, and near
. For more
* information, see Constructing Compound Queries in the Amazon CloudSearch Developer Guide.
* -
lucene
: search using the Apache Lucene query parser syntax. For more information, see Apache Lucene Query Parser Syntax.
* -
dismax
: search using the simplified subset of the Apache Lucene query parser syntax
* defined by the DisMax query parser. For more information, see DisMax Query Parser Syntax.
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryParser
*/
public SearchRequest withQueryParser(QueryParser queryParser) {
this.queryParser = queryParser.toString();
return this;
}
/**
*
* Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
*
*
* @param returnValue
* Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
*/
public void setReturn(String returnValue) {
this.returnValue = returnValue;
}
/**
*
* Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
*
*
* @return Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
*/
public String getReturn() {
return this.returnValue;
}
/**
*
* Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
*
*
* @param returnValue
* Specifies the field and expression values to include in the response. Multiple fields or expressions are
* specified as a comma-separated list. By default, a search response includes all return enabled fields (
* _all_fields
). To return only the document IDs for the matching documents, specify
* _no_fields
. To retrieve the relevance score calculated for each document, specify
* _score
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withReturn(String returnValue) {
setReturn(returnValue);
return this;
}
/**
*
* Specifies the maximum number of search hits to include in the response.
*
*
* @param size
* Specifies the maximum number of search hits to include in the response.
*/
public void setSize(Long size) {
this.size = size;
}
/**
*
* Specifies the maximum number of search hits to include in the response.
*
*
* @return Specifies the maximum number of search hits to include in the response.
*/
public Long getSize() {
return this.size;
}
/**
*
* Specifies the maximum number of search hits to include in the response.
*
*
* @param size
* Specifies the maximum number of search hits to include in the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withSize(Long size) {
setSize(size);
return this;
}
/**
*
* Specifies the fields or custom expressions to use to sort the search results. Multiple fields or expressions are
* specified as a comma-separated list. You must specify the sort direction (asc
or desc
)
* for each field; for example, year desc,title asc
. To use a field to sort results, the field must be
* sort-enabled in the domain configuration. Array type fields cannot be used for sorting. If no sort
* parameter is specified, results are sorted by their default relevance scores in descending order:
* _score desc
. You can also sort by document ID (_id asc
) and version (
* _version desc
).
*
*
* For more information, see Sorting Results in
* the Amazon CloudSearch Developer Guide.
*
*
* @param sort
* Specifies the fields or custom expressions to use to sort the search results. Multiple fields or
* expressions are specified as a comma-separated list. You must specify the sort direction (asc
* or desc
) for each field; for example, year desc,title asc
. To use a field to
* sort results, the field must be sort-enabled in the domain configuration. Array type fields cannot be used
* for sorting. If no sort
parameter is specified, results are sorted by their default relevance
* scores in descending order: _score desc
. You can also sort by document ID (
* _id asc
) and version (_version desc
).
*
* For more information, see Sorting
* Results in the Amazon CloudSearch Developer Guide.
*/
public void setSort(String sort) {
this.sort = sort;
}
/**
*
* Specifies the fields or custom expressions to use to sort the search results. Multiple fields or expressions are
* specified as a comma-separated list. You must specify the sort direction (asc
or desc
)
* for each field; for example, year desc,title asc
. To use a field to sort results, the field must be
* sort-enabled in the domain configuration. Array type fields cannot be used for sorting. If no sort
* parameter is specified, results are sorted by their default relevance scores in descending order:
* _score desc
. You can also sort by document ID (_id asc
) and version (
* _version desc
).
*
*
* For more information, see Sorting Results in
* the Amazon CloudSearch Developer Guide.
*
*
* @return Specifies the fields or custom expressions to use to sort the search results. Multiple fields or
* expressions are specified as a comma-separated list. You must specify the sort direction (
* asc
or desc
) for each field; for example, year desc,title asc
. To
* use a field to sort results, the field must be sort-enabled in the domain configuration. Array type
* fields cannot be used for sorting. If no sort
parameter is specified, results are sorted by
* their default relevance scores in descending order: _score desc
. You can also sort by
* document ID (_id asc
) and version (_version desc
).
*
* For more information, see Sorting
* Results in the Amazon CloudSearch Developer Guide.
*/
public String getSort() {
return this.sort;
}
/**
*
* Specifies the fields or custom expressions to use to sort the search results. Multiple fields or expressions are
* specified as a comma-separated list. You must specify the sort direction (asc
or desc
)
* for each field; for example, year desc,title asc
. To use a field to sort results, the field must be
* sort-enabled in the domain configuration. Array type fields cannot be used for sorting. If no sort
* parameter is specified, results are sorted by their default relevance scores in descending order:
* _score desc
. You can also sort by document ID (_id asc
) and version (
* _version desc
).
*
*
* For more information, see Sorting Results in
* the Amazon CloudSearch Developer Guide.
*
*
* @param sort
* Specifies the fields or custom expressions to use to sort the search results. Multiple fields or
* expressions are specified as a comma-separated list. You must specify the sort direction (asc
* or desc
) for each field; for example, year desc,title asc
. To use a field to
* sort results, the field must be sort-enabled in the domain configuration. Array type fields cannot be used
* for sorting. If no sort
parameter is specified, results are sorted by their default relevance
* scores in descending order: _score desc
. You can also sort by document ID (
* _id asc
) and version (_version desc
).
*
* For more information, see Sorting
* Results in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withSort(String sort) {
setSort(sort);
return this;
}
/**
*
* Specifies the offset of the first search hit you want to return. Note that the result set is zero-based; the
* first result is at index 0. You can specify either the start
or cursor
parameter in a
* request, they are mutually exclusive.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*
* @param start
* Specifies the offset of the first search hit you want to return. Note that the result set is zero-based;
* the first result is at index 0. You can specify either the start
or cursor
* parameter in a request, they are mutually exclusive.
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*/
public void setStart(Long start) {
this.start = start;
}
/**
*
* Specifies the offset of the first search hit you want to return. Note that the result set is zero-based; the
* first result is at index 0. You can specify either the start
or cursor
parameter in a
* request, they are mutually exclusive.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*
* @return Specifies the offset of the first search hit you want to return. Note that the result set is zero-based;
* the first result is at index 0. You can specify either the start
or cursor
* parameter in a request, they are mutually exclusive.
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*/
public Long getStart() {
return this.start;
}
/**
*
* Specifies the offset of the first search hit you want to return. Note that the result set is zero-based; the
* first result is at index 0. You can specify either the start
or cursor
parameter in a
* request, they are mutually exclusive.
*
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
*
*
* @param start
* Specifies the offset of the first search hit you want to return. Note that the result set is zero-based;
* the first result is at index 0. You can specify either the start
or cursor
* parameter in a request, they are mutually exclusive.
*
* For more information, see Paginating
* Results in the Amazon CloudSearch Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withStart(Long start) {
setStart(start);
return this;
}
/**
*
* Specifies one or more fields for which to get statistics information. Each specified field must be facet-enabled
* in the domain configuration. The fields are specified in JSON using the form:
*
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
*
*
* @param stats
* Specifies one or more fields for which to get statistics information. Each specified field must be
* facet-enabled in the domain configuration. The fields are specified in JSON using the form:
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
*/
public void setStats(String stats) {
this.stats = stats;
}
/**
*
* Specifies one or more fields for which to get statistics information. Each specified field must be facet-enabled
* in the domain configuration. The fields are specified in JSON using the form:
*
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
*
*
* @return Specifies one or more fields for which to get statistics information. Each specified field must be
* facet-enabled in the domain configuration. The fields are specified in JSON using the form:
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
*/
public String getStats() {
return this.stats;
}
/**
*
* Specifies one or more fields for which to get statistics information. Each specified field must be facet-enabled
* in the domain configuration. The fields are specified in JSON using the form:
*
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
*
*
* @param stats
* Specifies one or more fields for which to get statistics information. Each specified field must be
* facet-enabled in the domain configuration. The fields are specified in JSON using the form:
* {"FIELD-A":{},"FIELD-B":{}}
*
* There are currently no options supported for statistics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchRequest withStats(String stats) {
setStats(stats);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCursor() != null)
sb.append("Cursor: ").append(getCursor()).append(",");
if (getExpr() != null)
sb.append("Expr: ").append(getExpr()).append(",");
if (getFacet() != null)
sb.append("Facet: ").append(getFacet()).append(",");
if (getFilterQuery() != null)
sb.append("FilterQuery: ").append(getFilterQuery()).append(",");
if (getHighlight() != null)
sb.append("Highlight: ").append(getHighlight()).append(",");
if (getPartial() != null)
sb.append("Partial: ").append(getPartial()).append(",");
if (getQuery() != null)
sb.append("Query: ").append(getQuery()).append(",");
if (getQueryOptions() != null)
sb.append("QueryOptions: ").append(getQueryOptions()).append(",");
if (getQueryParser() != null)
sb.append("QueryParser: ").append(getQueryParser()).append(",");
if (getReturn() != null)
sb.append("Return: ").append(getReturn()).append(",");
if (getSize() != null)
sb.append("Size: ").append(getSize()).append(",");
if (getSort() != null)
sb.append("Sort: ").append(getSort()).append(",");
if (getStart() != null)
sb.append("Start: ").append(getStart()).append(",");
if (getStats() != null)
sb.append("Stats: ").append(getStats());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SearchRequest == false)
return false;
SearchRequest other = (SearchRequest) obj;
if (other.getCursor() == null ^ this.getCursor() == null)
return false;
if (other.getCursor() != null && other.getCursor().equals(this.getCursor()) == false)
return false;
if (other.getExpr() == null ^ this.getExpr() == null)
return false;
if (other.getExpr() != null && other.getExpr().equals(this.getExpr()) == false)
return false;
if (other.getFacet() == null ^ this.getFacet() == null)
return false;
if (other.getFacet() != null && other.getFacet().equals(this.getFacet()) == false)
return false;
if (other.getFilterQuery() == null ^ this.getFilterQuery() == null)
return false;
if (other.getFilterQuery() != null && other.getFilterQuery().equals(this.getFilterQuery()) == false)
return false;
if (other.getHighlight() == null ^ this.getHighlight() == null)
return false;
if (other.getHighlight() != null && other.getHighlight().equals(this.getHighlight()) == false)
return false;
if (other.getPartial() == null ^ this.getPartial() == null)
return false;
if (other.getPartial() != null && other.getPartial().equals(this.getPartial()) == false)
return false;
if (other.getQuery() == null ^ this.getQuery() == null)
return false;
if (other.getQuery() != null && other.getQuery().equals(this.getQuery()) == false)
return false;
if (other.getQueryOptions() == null ^ this.getQueryOptions() == null)
return false;
if (other.getQueryOptions() != null && other.getQueryOptions().equals(this.getQueryOptions()) == false)
return false;
if (other.getQueryParser() == null ^ this.getQueryParser() == null)
return false;
if (other.getQueryParser() != null && other.getQueryParser().equals(this.getQueryParser()) == false)
return false;
if (other.getReturn() == null ^ this.getReturn() == null)
return false;
if (other.getReturn() != null && other.getReturn().equals(this.getReturn()) == false)
return false;
if (other.getSize() == null ^ this.getSize() == null)
return false;
if (other.getSize() != null && other.getSize().equals(this.getSize()) == false)
return false;
if (other.getSort() == null ^ this.getSort() == null)
return false;
if (other.getSort() != null && other.getSort().equals(this.getSort()) == false)
return false;
if (other.getStart() == null ^ this.getStart() == null)
return false;
if (other.getStart() != null && other.getStart().equals(this.getStart()) == false)
return false;
if (other.getStats() == null ^ this.getStats() == null)
return false;
if (other.getStats() != null && other.getStats().equals(this.getStats()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCursor() == null) ? 0 : getCursor().hashCode());
hashCode = prime * hashCode + ((getExpr() == null) ? 0 : getExpr().hashCode());
hashCode = prime * hashCode + ((getFacet() == null) ? 0 : getFacet().hashCode());
hashCode = prime * hashCode + ((getFilterQuery() == null) ? 0 : getFilterQuery().hashCode());
hashCode = prime * hashCode + ((getHighlight() == null) ? 0 : getHighlight().hashCode());
hashCode = prime * hashCode + ((getPartial() == null) ? 0 : getPartial().hashCode());
hashCode = prime * hashCode + ((getQuery() == null) ? 0 : getQuery().hashCode());
hashCode = prime * hashCode + ((getQueryOptions() == null) ? 0 : getQueryOptions().hashCode());
hashCode = prime * hashCode + ((getQueryParser() == null) ? 0 : getQueryParser().hashCode());
hashCode = prime * hashCode + ((getReturn() == null) ? 0 : getReturn().hashCode());
hashCode = prime * hashCode + ((getSize() == null) ? 0 : getSize().hashCode());
hashCode = prime * hashCode + ((getSort() == null) ? 0 : getSort().hashCode());
hashCode = prime * hashCode + ((getStart() == null) ? 0 : getStart().hashCode());
hashCode = prime * hashCode + ((getStats() == null) ? 0 : getStats().hashCode());
return hashCode;
}
@Override
public SearchRequest clone() {
return (SearchRequest) super.clone();
}
}