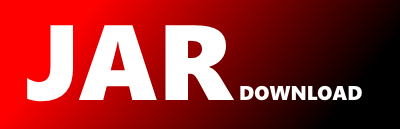
com.amazonaws.services.cloudsearchv2.AmazonCloudSearchAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudsearch Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudsearchv2;
import javax.annotation.Generated;
import com.amazonaws.services.cloudsearchv2.model.*;
/**
* Interface for accessing Amazon CloudSearch asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudsearchv2.AbstractAmazonCloudSearchAsync} instead.
*
*
* Amazon CloudSearch Configuration Service
*
* You use the Amazon CloudSearch configuration service to create, configure, and manage search domains. Configuration
* service requests are submitted using the AWS Query protocol. AWS Query requests are HTTP or HTTPS requests submitted
* via HTTP GET or POST with a query parameter named Action.
*
*
* The endpoint for configuration service requests is region-specific: cloudsearch.region.amazonaws.com. For
* example, cloudsearch.us-east-1.amazonaws.com. For a current list of supported regions and endpoints, see Regions and
* Endpoints.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCloudSearchAsync extends AmazonCloudSearch {
/**
*
* Indexes the search suggestions. For more information, see Configuring Suggesters in the Amazon CloudSearch Developer Guide.
*
*
* @param buildSuggestersRequest
* Container for the parameters to the BuildSuggester
operation. Specifies the name of
* the domain you want to update.
* @return A Java Future containing the result of the BuildSuggesters operation returned by the service.
* @sample AmazonCloudSearchAsync.BuildSuggesters
*/
java.util.concurrent.Future buildSuggestersAsync(BuildSuggestersRequest buildSuggestersRequest);
/**
*
* Indexes the search suggestions. For more information, see Configuring Suggesters in the Amazon CloudSearch Developer Guide.
*
*
* @param buildSuggestersRequest
* Container for the parameters to the BuildSuggester
operation. Specifies the name of
* the domain you want to update.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BuildSuggesters operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.BuildSuggesters
*/
java.util.concurrent.Future buildSuggestersAsync(BuildSuggestersRequest buildSuggestersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new search domain. For more information, see Creating a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param createDomainRequest
* Container for the parameters to the CreateDomain
operation. Specifies a name for the
* new search domain.
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonCloudSearchAsync.CreateDomain
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest);
/**
*
* Creates a new search domain. For more information, see Creating a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param createDomainRequest
* Container for the parameters to the CreateDomain
operation. Specifies a name for the
* new search domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.CreateDomain
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures an analysis scheme that can be applied to a text
or text-array
field to
* define language-specific text processing options. For more information, see Configuring Analysis Schemes in the Amazon CloudSearch Developer Guide.
*
*
* @param defineAnalysisSchemeRequest
* Container for the parameters to the DefineAnalysisScheme
operation. Specifies the name
* of the domain you want to update and the analysis scheme configuration.
* @return A Java Future containing the result of the DefineAnalysisScheme operation returned by the service.
* @sample AmazonCloudSearchAsync.DefineAnalysisScheme
*/
java.util.concurrent.Future defineAnalysisSchemeAsync(DefineAnalysisSchemeRequest defineAnalysisSchemeRequest);
/**
*
* Configures an analysis scheme that can be applied to a text
or text-array
field to
* define language-specific text processing options. For more information, see Configuring Analysis Schemes in the Amazon CloudSearch Developer Guide.
*
*
* @param defineAnalysisSchemeRequest
* Container for the parameters to the DefineAnalysisScheme
operation. Specifies the name
* of the domain you want to update and the analysis scheme configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DefineAnalysisScheme operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DefineAnalysisScheme
*/
java.util.concurrent.Future defineAnalysisSchemeAsync(DefineAnalysisSchemeRequest defineAnalysisSchemeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures an Expression
for the search domain. Used to create new expressions and modify
* existing ones. If the expression exists, the new configuration replaces the old one. For more information, see Configuring Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param defineExpressionRequest
* Container for the parameters to the DefineExpression
operation. Specifies the name of
* the domain you want to update and the expression you want to configure.
* @return A Java Future containing the result of the DefineExpression operation returned by the service.
* @sample AmazonCloudSearchAsync.DefineExpression
*/
java.util.concurrent.Future defineExpressionAsync(DefineExpressionRequest defineExpressionRequest);
/**
*
* Configures an Expression
for the search domain. Used to create new expressions and modify
* existing ones. If the expression exists, the new configuration replaces the old one. For more information, see Configuring Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param defineExpressionRequest
* Container for the parameters to the DefineExpression
operation. Specifies the name of
* the domain you want to update and the expression you want to configure.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DefineExpression operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DefineExpression
*/
java.util.concurrent.Future defineExpressionAsync(DefineExpressionRequest defineExpressionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures an IndexField
for the search domain. Used to create new fields and modify existing
* ones. You must specify the name of the domain you are configuring and an index field configuration. The index
* field configuration specifies a unique name, the index field type, and the options you want to configure for the
* field. The options you can specify depend on the IndexFieldType
. If the field exists, the new
* configuration replaces the old one. For more information, see Configuring Index Fields in the Amazon CloudSearch Developer Guide.
*
*
* @param defineIndexFieldRequest
* Container for the parameters to the DefineIndexField
operation. Specifies the name of
* the domain you want to update and the index field configuration.
* @return A Java Future containing the result of the DefineIndexField operation returned by the service.
* @sample AmazonCloudSearchAsync.DefineIndexField
*/
java.util.concurrent.Future defineIndexFieldAsync(DefineIndexFieldRequest defineIndexFieldRequest);
/**
*
* Configures an IndexField
for the search domain. Used to create new fields and modify existing
* ones. You must specify the name of the domain you are configuring and an index field configuration. The index
* field configuration specifies a unique name, the index field type, and the options you want to configure for the
* field. The options you can specify depend on the IndexFieldType
. If the field exists, the new
* configuration replaces the old one. For more information, see Configuring Index Fields in the Amazon CloudSearch Developer Guide.
*
*
* @param defineIndexFieldRequest
* Container for the parameters to the DefineIndexField
operation. Specifies the name of
* the domain you want to update and the index field configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DefineIndexField operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DefineIndexField
*/
java.util.concurrent.Future defineIndexFieldAsync(DefineIndexFieldRequest defineIndexFieldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures a suggester for a domain. A suggester enables you to display possible matches before users finish
* typing their queries. When you configure a suggester, you must specify the name of the text field you want to
* search for possible matches and a unique name for the suggester. For more information, see Getting Search Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* @param defineSuggesterRequest
* Container for the parameters to the DefineSuggester
operation. Specifies the name of
* the domain you want to update and the suggester configuration.
* @return A Java Future containing the result of the DefineSuggester operation returned by the service.
* @sample AmazonCloudSearchAsync.DefineSuggester
*/
java.util.concurrent.Future defineSuggesterAsync(DefineSuggesterRequest defineSuggesterRequest);
/**
*
* Configures a suggester for a domain. A suggester enables you to display possible matches before users finish
* typing their queries. When you configure a suggester, you must specify the name of the text field you want to
* search for possible matches and a unique name for the suggester. For more information, see Getting Search Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* @param defineSuggesterRequest
* Container for the parameters to the DefineSuggester
operation. Specifies the name of
* the domain you want to update and the suggester configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DefineSuggester operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DefineSuggester
*/
java.util.concurrent.Future defineSuggesterAsync(DefineSuggesterRequest defineSuggesterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an analysis scheme. For more information, see Configuring Analysis Schemes in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteAnalysisSchemeRequest
* Container for the parameters to the DeleteAnalysisScheme
operation. Specifies the name
* of the domain you want to update and the analysis scheme you want to delete.
* @return A Java Future containing the result of the DeleteAnalysisScheme operation returned by the service.
* @sample AmazonCloudSearchAsync.DeleteAnalysisScheme
*/
java.util.concurrent.Future deleteAnalysisSchemeAsync(DeleteAnalysisSchemeRequest deleteAnalysisSchemeRequest);
/**
*
* Deletes an analysis scheme. For more information, see Configuring Analysis Schemes in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteAnalysisSchemeRequest
* Container for the parameters to the DeleteAnalysisScheme
operation. Specifies the name
* of the domain you want to update and the analysis scheme you want to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAnalysisScheme operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DeleteAnalysisScheme
*/
java.util.concurrent.Future deleteAnalysisSchemeAsync(DeleteAnalysisSchemeRequest deleteAnalysisSchemeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently deletes a search domain and all of its data. Once a domain has been deleted, it cannot be recovered.
* For more information, see Deleting a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteDomainRequest
* Container for the parameters to the DeleteDomain
operation. Specifies the name of the
* domain you want to delete.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonCloudSearchAsync.DeleteDomain
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest);
/**
*
* Permanently deletes a search domain and all of its data. Once a domain has been deleted, it cannot be recovered.
* For more information, see Deleting a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteDomainRequest
* Container for the parameters to the DeleteDomain
operation. Specifies the name of the
* domain you want to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DeleteDomain
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an Expression
from the search domain. For more information, see Configuring Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteExpressionRequest
* Container for the parameters to the DeleteExpression
operation. Specifies the name of
* the domain you want to update and the name of the expression you want to delete.
* @return A Java Future containing the result of the DeleteExpression operation returned by the service.
* @sample AmazonCloudSearchAsync.DeleteExpression
*/
java.util.concurrent.Future deleteExpressionAsync(DeleteExpressionRequest deleteExpressionRequest);
/**
*
* Removes an Expression
from the search domain. For more information, see Configuring Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteExpressionRequest
* Container for the parameters to the DeleteExpression
operation. Specifies the name of
* the domain you want to update and the name of the expression you want to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteExpression operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DeleteExpression
*/
java.util.concurrent.Future deleteExpressionAsync(DeleteExpressionRequest deleteExpressionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an IndexField
from the search domain. For more information, see Configuring Index Fields in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteIndexFieldRequest
* Container for the parameters to the DeleteIndexField
operation. Specifies the name of
* the domain you want to update and the name of the index field you want to delete.
* @return A Java Future containing the result of the DeleteIndexField operation returned by the service.
* @sample AmazonCloudSearchAsync.DeleteIndexField
*/
java.util.concurrent.Future deleteIndexFieldAsync(DeleteIndexFieldRequest deleteIndexFieldRequest);
/**
*
* Removes an IndexField
from the search domain. For more information, see Configuring Index Fields in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteIndexFieldRequest
* Container for the parameters to the DeleteIndexField
operation. Specifies the name of
* the domain you want to update and the name of the index field you want to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIndexField operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DeleteIndexField
*/
java.util.concurrent.Future deleteIndexFieldAsync(DeleteIndexFieldRequest deleteIndexFieldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a suggester. For more information, see Getting Search Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteSuggesterRequest
* Container for the parameters to the DeleteSuggester
operation. Specifies the name of
* the domain you want to update and name of the suggester you want to delete.
* @return A Java Future containing the result of the DeleteSuggester operation returned by the service.
* @sample AmazonCloudSearchAsync.DeleteSuggester
*/
java.util.concurrent.Future deleteSuggesterAsync(DeleteSuggesterRequest deleteSuggesterRequest);
/**
*
* Deletes a suggester. For more information, see Getting Search Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* @param deleteSuggesterRequest
* Container for the parameters to the DeleteSuggester
operation. Specifies the name of
* the domain you want to update and name of the suggester you want to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSuggester operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DeleteSuggester
*/
java.util.concurrent.Future deleteSuggesterAsync(DeleteSuggesterRequest deleteSuggesterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the analysis schemes configured for a domain. An analysis scheme defines language-specific text processing
* options for a text
field. Can be limited to specific analysis schemes by name. By default, shows all
* analysis schemes and includes any pending changes to the configuration. Set the Deployed
option to
* true
to show the active configuration and exclude pending changes. For more information, see Configuring Analysis Schemes in the Amazon CloudSearch Developer Guide.
*
*
* @param describeAnalysisSchemesRequest
* Container for the parameters to the DescribeAnalysisSchemes
operation. Specifies the
* name of the domain you want to describe. To limit the response to particular analysis schemes, specify the
* names of the analysis schemes you want to describe. To show the active configuration and exclude any
* pending changes, set the Deployed
option to true
.
* @return A Java Future containing the result of the DescribeAnalysisSchemes operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeAnalysisSchemes
*/
java.util.concurrent.Future describeAnalysisSchemesAsync(DescribeAnalysisSchemesRequest describeAnalysisSchemesRequest);
/**
*
* Gets the analysis schemes configured for a domain. An analysis scheme defines language-specific text processing
* options for a text
field. Can be limited to specific analysis schemes by name. By default, shows all
* analysis schemes and includes any pending changes to the configuration. Set the Deployed
option to
* true
to show the active configuration and exclude pending changes. For more information, see Configuring Analysis Schemes in the Amazon CloudSearch Developer Guide.
*
*
* @param describeAnalysisSchemesRequest
* Container for the parameters to the DescribeAnalysisSchemes
operation. Specifies the
* name of the domain you want to describe. To limit the response to particular analysis schemes, specify the
* names of the analysis schemes you want to describe. To show the active configuration and exclude any
* pending changes, set the Deployed
option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAnalysisSchemes operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeAnalysisSchemes
*/
java.util.concurrent.Future describeAnalysisSchemesAsync(DescribeAnalysisSchemesRequest describeAnalysisSchemesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the availability options configured for a domain. By default, shows the configuration with any pending
* changes. Set the Deployed
option to true
to show the active configuration and exclude
* pending changes. For more information, see Configuring Availability Options in the Amazon CloudSearch Developer Guide.
*
*
* @param describeAvailabilityOptionsRequest
* Container for the parameters to the DescribeAvailabilityOptions
operation. Specifies
* the name of the domain you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed option to true
.
* @return A Java Future containing the result of the DescribeAvailabilityOptions operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeAvailabilityOptions
*/
java.util.concurrent.Future describeAvailabilityOptionsAsync(
DescribeAvailabilityOptionsRequest describeAvailabilityOptionsRequest);
/**
*
* Gets the availability options configured for a domain. By default, shows the configuration with any pending
* changes. Set the Deployed
option to true
to show the active configuration and exclude
* pending changes. For more information, see Configuring Availability Options in the Amazon CloudSearch Developer Guide.
*
*
* @param describeAvailabilityOptionsRequest
* Container for the parameters to the DescribeAvailabilityOptions
operation. Specifies
* the name of the domain you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAvailabilityOptions operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeAvailabilityOptions
*/
java.util.concurrent.Future describeAvailabilityOptionsAsync(
DescribeAvailabilityOptionsRequest describeAvailabilityOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the domain's endpoint options, specifically whether all requests to the domain must arrive over HTTPS.
* For more information, see Configuring Domain Endpoint Options in the Amazon CloudSearch Developer Guide.
*
*
* @param describeDomainEndpointOptionsRequest
* Container for the parameters to the DescribeDomainEndpointOptions
operation. Specify
* the name of the domain you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed option to true
.
* @return A Java Future containing the result of the DescribeDomainEndpointOptions operation returned by the
* service.
* @sample AmazonCloudSearchAsync.DescribeDomainEndpointOptions
*/
java.util.concurrent.Future describeDomainEndpointOptionsAsync(
DescribeDomainEndpointOptionsRequest describeDomainEndpointOptionsRequest);
/**
*
* Returns the domain's endpoint options, specifically whether all requests to the domain must arrive over HTTPS.
* For more information, see Configuring Domain Endpoint Options in the Amazon CloudSearch Developer Guide.
*
*
* @param describeDomainEndpointOptionsRequest
* Container for the parameters to the DescribeDomainEndpointOptions
operation. Specify
* the name of the domain you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDomainEndpointOptions operation returned by the
* service.
* @sample AmazonCloudSearchAsyncHandler.DescribeDomainEndpointOptions
*/
java.util.concurrent.Future describeDomainEndpointOptionsAsync(
DescribeDomainEndpointOptionsRequest describeDomainEndpointOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the search domains owned by this account. Can be limited to specific domains. Shows all
* domains by default. To get the number of searchable documents in a domain, use the console or submit a
* matchall
request to your domain's search endpoint:
* q=matchall&q.parser=structured&size=0
. For more information, see Getting Information about a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param describeDomainsRequest
* Container for the parameters to the DescribeDomains
operation. By default shows the
* status of all domains. To restrict the response to particular domains, specify the names of the domains
* you want to describe.
* @return A Java Future containing the result of the DescribeDomains operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeDomains
*/
java.util.concurrent.Future describeDomainsAsync(DescribeDomainsRequest describeDomainsRequest);
/**
*
* Gets information about the search domains owned by this account. Can be limited to specific domains. Shows all
* domains by default. To get the number of searchable documents in a domain, use the console or submit a
* matchall
request to your domain's search endpoint:
* q=matchall&q.parser=structured&size=0
. For more information, see Getting Information about a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param describeDomainsRequest
* Container for the parameters to the DescribeDomains
operation. By default shows the
* status of all domains. To restrict the response to particular domains, specify the names of the domains
* you want to describe.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDomains operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeDomains
*/
java.util.concurrent.Future describeDomainsAsync(DescribeDomainsRequest describeDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDomains operation.
*
* @see #describeDomainsAsync(DescribeDomainsRequest)
*/
java.util.concurrent.Future describeDomainsAsync();
/**
* Simplified method form for invoking the DescribeDomains operation with an AsyncHandler.
*
* @see #describeDomainsAsync(DescribeDomainsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDomainsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the expressions configured for the search domain. Can be limited to specific expressions by name. By
* default, shows all expressions and includes any pending changes to the configuration. Set the
* Deployed
option to true
to show the active configuration and exclude pending changes.
* For more information, see Configuring Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param describeExpressionsRequest
* Container for the parameters to the DescribeDomains
operation. Specifies the name of
* the domain you want to describe. To restrict the response to particular expressions, specify the names of
* the expressions you want to describe. To show the active configuration and exclude any pending changes,
* set the Deployed
option to true
.
* @return A Java Future containing the result of the DescribeExpressions operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeExpressions
*/
java.util.concurrent.Future describeExpressionsAsync(DescribeExpressionsRequest describeExpressionsRequest);
/**
*
* Gets the expressions configured for the search domain. Can be limited to specific expressions by name. By
* default, shows all expressions and includes any pending changes to the configuration. Set the
* Deployed
option to true
to show the active configuration and exclude pending changes.
* For more information, see Configuring Expressions in the Amazon CloudSearch Developer Guide.
*
*
* @param describeExpressionsRequest
* Container for the parameters to the DescribeDomains
operation. Specifies the name of
* the domain you want to describe. To restrict the response to particular expressions, specify the names of
* the expressions you want to describe. To show the active configuration and exclude any pending changes,
* set the Deployed
option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeExpressions operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeExpressions
*/
java.util.concurrent.Future describeExpressionsAsync(DescribeExpressionsRequest describeExpressionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the index fields configured for the search domain. Can be limited to specific fields by
* name. By default, shows all fields and includes any pending changes to the configuration. Set the
* Deployed
option to true
to show the active configuration and exclude pending changes.
* For more information, see Getting Domain Information in the Amazon CloudSearch Developer Guide.
*
*
* @param describeIndexFieldsRequest
* Container for the parameters to the DescribeIndexFields
operation. Specifies the name
* of the domain you want to describe. To restrict the response to particular index fields, specify the names
* of the index fields you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed
option to true
.
* @return A Java Future containing the result of the DescribeIndexFields operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeIndexFields
*/
java.util.concurrent.Future describeIndexFieldsAsync(DescribeIndexFieldsRequest describeIndexFieldsRequest);
/**
*
* Gets information about the index fields configured for the search domain. Can be limited to specific fields by
* name. By default, shows all fields and includes any pending changes to the configuration. Set the
* Deployed
option to true
to show the active configuration and exclude pending changes.
* For more information, see Getting Domain Information in the Amazon CloudSearch Developer Guide.
*
*
* @param describeIndexFieldsRequest
* Container for the parameters to the DescribeIndexFields
operation. Specifies the name
* of the domain you want to describe. To restrict the response to particular index fields, specify the names
* of the index fields you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed
option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIndexFields operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeIndexFields
*/
java.util.concurrent.Future describeIndexFieldsAsync(DescribeIndexFieldsRequest describeIndexFieldsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the scaling parameters configured for a domain. A domain's scaling parameters specify the desired search
* instance type and replication count. For more information, see Configuring Scaling Options in the Amazon CloudSearch Developer Guide.
*
*
* @param describeScalingParametersRequest
* Container for the parameters to the DescribeScalingParameters
operation. Specifies the
* name of the domain you want to describe.
* @return A Java Future containing the result of the DescribeScalingParameters operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeScalingParameters
*/
java.util.concurrent.Future describeScalingParametersAsync(
DescribeScalingParametersRequest describeScalingParametersRequest);
/**
*
* Gets the scaling parameters configured for a domain. A domain's scaling parameters specify the desired search
* instance type and replication count. For more information, see Configuring Scaling Options in the Amazon CloudSearch Developer Guide.
*
*
* @param describeScalingParametersRequest
* Container for the parameters to the DescribeScalingParameters
operation. Specifies the
* name of the domain you want to describe.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeScalingParameters operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeScalingParameters
*/
java.util.concurrent.Future describeScalingParametersAsync(
DescribeScalingParametersRequest describeScalingParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the access policies that control access to the domain's document and search endpoints. By
* default, shows the configuration with any pending changes. Set the Deployed
option to
* true
to show the active configuration and exclude pending changes. For more information, see Configuring Access for a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param describeServiceAccessPoliciesRequest
* Container for the parameters to the DescribeServiceAccessPolicies
operation. Specifies
* the name of the domain you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed
option to true
.
* @return A Java Future containing the result of the DescribeServiceAccessPolicies operation returned by the
* service.
* @sample AmazonCloudSearchAsync.DescribeServiceAccessPolicies
*/
java.util.concurrent.Future describeServiceAccessPoliciesAsync(
DescribeServiceAccessPoliciesRequest describeServiceAccessPoliciesRequest);
/**
*
* Gets information about the access policies that control access to the domain's document and search endpoints. By
* default, shows the configuration with any pending changes. Set the Deployed
option to
* true
to show the active configuration and exclude pending changes. For more information, see Configuring Access for a Search Domain in the Amazon CloudSearch Developer Guide.
*
*
* @param describeServiceAccessPoliciesRequest
* Container for the parameters to the DescribeServiceAccessPolicies
operation. Specifies
* the name of the domain you want to describe. To show the active configuration and exclude any pending
* changes, set the Deployed
option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServiceAccessPolicies operation returned by the
* service.
* @sample AmazonCloudSearchAsyncHandler.DescribeServiceAccessPolicies
*/
java.util.concurrent.Future describeServiceAccessPoliciesAsync(
DescribeServiceAccessPoliciesRequest describeServiceAccessPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the suggesters configured for a domain. A suggester enables you to display possible matches before users
* finish typing their queries. Can be limited to specific suggesters by name. By default, shows all suggesters and
* includes any pending changes to the configuration. Set the Deployed
option to true
to
* show the active configuration and exclude pending changes. For more information, see Getting Search Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* @param describeSuggestersRequest
* Container for the parameters to the DescribeSuggester
operation. Specifies the name of
* the domain you want to describe. To restrict the response to particular suggesters, specify the names of
* the suggesters you want to describe. To show the active configuration and exclude any pending changes, set
* the Deployed
option to true
.
* @return A Java Future containing the result of the DescribeSuggesters operation returned by the service.
* @sample AmazonCloudSearchAsync.DescribeSuggesters
*/
java.util.concurrent.Future describeSuggestersAsync(DescribeSuggestersRequest describeSuggestersRequest);
/**
*
* Gets the suggesters configured for a domain. A suggester enables you to display possible matches before users
* finish typing their queries. Can be limited to specific suggesters by name. By default, shows all suggesters and
* includes any pending changes to the configuration. Set the Deployed
option to true
to
* show the active configuration and exclude pending changes. For more information, see Getting Search Suggestions in the Amazon CloudSearch Developer Guide.
*
*
* @param describeSuggestersRequest
* Container for the parameters to the DescribeSuggester
operation. Specifies the name of
* the domain you want to describe. To restrict the response to particular suggesters, specify the names of
* the suggesters you want to describe. To show the active configuration and exclude any pending changes, set
* the Deployed
option to true
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSuggesters operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.DescribeSuggesters
*/
java.util.concurrent.Future describeSuggestersAsync(DescribeSuggestersRequest describeSuggestersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tells the search domain to start indexing its documents using the latest indexing options. This operation must be
* invoked to activate options whose OptionStatus is RequiresIndexDocuments
.
*
*
* @param indexDocumentsRequest
* Container for the parameters to the IndexDocuments
operation. Specifies the name of
* the domain you want to re-index.
* @return A Java Future containing the result of the IndexDocuments operation returned by the service.
* @sample AmazonCloudSearchAsync.IndexDocuments
*/
java.util.concurrent.Future indexDocumentsAsync(IndexDocumentsRequest indexDocumentsRequest);
/**
*
* Tells the search domain to start indexing its documents using the latest indexing options. This operation must be
* invoked to activate options whose OptionStatus is RequiresIndexDocuments
.
*
*
* @param indexDocumentsRequest
* Container for the parameters to the IndexDocuments
operation. Specifies the name of
* the domain you want to re-index.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the IndexDocuments operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.IndexDocuments
*/
java.util.concurrent.Future indexDocumentsAsync(IndexDocumentsRequest indexDocumentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all search domains owned by an account.
*
*
* @param listDomainNamesRequest
* @return A Java Future containing the result of the ListDomainNames operation returned by the service.
* @sample AmazonCloudSearchAsync.ListDomainNames
*/
java.util.concurrent.Future listDomainNamesAsync(ListDomainNamesRequest listDomainNamesRequest);
/**
*
* Lists all search domains owned by an account.
*
*
* @param listDomainNamesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomainNames operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.ListDomainNames
*/
java.util.concurrent.Future listDomainNamesAsync(ListDomainNamesRequest listDomainNamesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListDomainNames operation.
*
* @see #listDomainNamesAsync(ListDomainNamesRequest)
*/
java.util.concurrent.Future listDomainNamesAsync();
/**
* Simplified method form for invoking the ListDomainNames operation with an AsyncHandler.
*
* @see #listDomainNamesAsync(ListDomainNamesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listDomainNamesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures the availability options for a domain. Enabling the Multi-AZ option expands an Amazon CloudSearch
* domain to an additional Availability Zone in the same Region to increase fault tolerance in the event of a
* service disruption. Changes to the Multi-AZ option can take about half an hour to become active. For more
* information, see Configuring Availability Options in the Amazon CloudSearch Developer Guide.
*
*
* @param updateAvailabilityOptionsRequest
* Container for the parameters to the UpdateAvailabilityOptions
operation. Specifies the
* name of the domain you want to update and the Multi-AZ availability option.
* @return A Java Future containing the result of the UpdateAvailabilityOptions operation returned by the service.
* @sample AmazonCloudSearchAsync.UpdateAvailabilityOptions
*/
java.util.concurrent.Future updateAvailabilityOptionsAsync(
UpdateAvailabilityOptionsRequest updateAvailabilityOptionsRequest);
/**
*
* Configures the availability options for a domain. Enabling the Multi-AZ option expands an Amazon CloudSearch
* domain to an additional Availability Zone in the same Region to increase fault tolerance in the event of a
* service disruption. Changes to the Multi-AZ option can take about half an hour to become active. For more
* information, see Configuring Availability Options in the Amazon CloudSearch Developer Guide.
*
*
* @param updateAvailabilityOptionsRequest
* Container for the parameters to the UpdateAvailabilityOptions
operation. Specifies the
* name of the domain you want to update and the Multi-AZ availability option.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAvailabilityOptions operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.UpdateAvailabilityOptions
*/
java.util.concurrent.Future updateAvailabilityOptionsAsync(
UpdateAvailabilityOptionsRequest updateAvailabilityOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the domain's endpoint options, specifically whether all requests to the domain must arrive over HTTPS.
* For more information, see Configuring Domain Endpoint Options in the Amazon CloudSearch Developer Guide.
*
*
* @param updateDomainEndpointOptionsRequest
* Container for the parameters to the UpdateDomainEndpointOptions
operation. Specifies
* the name of the domain you want to update and the domain endpoint options.
* @return A Java Future containing the result of the UpdateDomainEndpointOptions operation returned by the service.
* @sample AmazonCloudSearchAsync.UpdateDomainEndpointOptions
*/
java.util.concurrent.Future updateDomainEndpointOptionsAsync(
UpdateDomainEndpointOptionsRequest updateDomainEndpointOptionsRequest);
/**
*
* Updates the domain's endpoint options, specifically whether all requests to the domain must arrive over HTTPS.
* For more information, see Configuring Domain Endpoint Options in the Amazon CloudSearch Developer Guide.
*
*
* @param updateDomainEndpointOptionsRequest
* Container for the parameters to the UpdateDomainEndpointOptions
operation. Specifies
* the name of the domain you want to update and the domain endpoint options.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDomainEndpointOptions operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.UpdateDomainEndpointOptions
*/
java.util.concurrent.Future updateDomainEndpointOptionsAsync(
UpdateDomainEndpointOptionsRequest updateDomainEndpointOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures scaling parameters for a domain. A domain's scaling parameters specify the desired search instance
* type and replication count. Amazon CloudSearch will still automatically scale your domain based on the volume of
* data and traffic, but not below the desired instance type and replication count. If the Multi-AZ option is
* enabled, these values control the resources used per Availability Zone. For more information, see Configuring Scaling Options in the Amazon CloudSearch Developer Guide.
*
*
* @param updateScalingParametersRequest
* Container for the parameters to the UpdateScalingParameters
operation. Specifies the
* name of the domain you want to update and the scaling parameters you want to configure.
* @return A Java Future containing the result of the UpdateScalingParameters operation returned by the service.
* @sample AmazonCloudSearchAsync.UpdateScalingParameters
*/
java.util.concurrent.Future updateScalingParametersAsync(UpdateScalingParametersRequest updateScalingParametersRequest);
/**
*
* Configures scaling parameters for a domain. A domain's scaling parameters specify the desired search instance
* type and replication count. Amazon CloudSearch will still automatically scale your domain based on the volume of
* data and traffic, but not below the desired instance type and replication count. If the Multi-AZ option is
* enabled, these values control the resources used per Availability Zone. For more information, see Configuring Scaling Options in the Amazon CloudSearch Developer Guide.
*
*
* @param updateScalingParametersRequest
* Container for the parameters to the UpdateScalingParameters
operation. Specifies the
* name of the domain you want to update and the scaling parameters you want to configure.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateScalingParameters operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.UpdateScalingParameters
*/
java.util.concurrent.Future updateScalingParametersAsync(UpdateScalingParametersRequest updateScalingParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures the access rules that control access to the domain's document and search endpoints. For more
* information, see Configuring Access for an Amazon CloudSearch Domain.
*
*
* @param updateServiceAccessPoliciesRequest
* Container for the parameters to the UpdateServiceAccessPolicies
operation. Specifies
* the name of the domain you want to update and the access rules you want to configure.
* @return A Java Future containing the result of the UpdateServiceAccessPolicies operation returned by the service.
* @sample AmazonCloudSearchAsync.UpdateServiceAccessPolicies
*/
java.util.concurrent.Future updateServiceAccessPoliciesAsync(
UpdateServiceAccessPoliciesRequest updateServiceAccessPoliciesRequest);
/**
*
* Configures the access rules that control access to the domain's document and search endpoints. For more
* information, see Configuring Access for an Amazon CloudSearch Domain.
*
*
* @param updateServiceAccessPoliciesRequest
* Container for the parameters to the UpdateServiceAccessPolicies
operation. Specifies
* the name of the domain you want to update and the access rules you want to configure.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateServiceAccessPolicies operation returned by the service.
* @sample AmazonCloudSearchAsyncHandler.UpdateServiceAccessPolicies
*/
java.util.concurrent.Future updateServiceAccessPoliciesAsync(
UpdateServiceAccessPoliciesRequest updateServiceAccessPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}