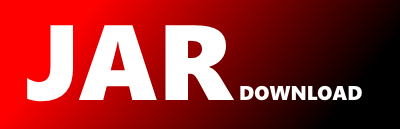
com.amazonaws.services.cloudtrail.AWSCloudTrailAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail;
import javax.annotation.Generated;
import com.amazonaws.services.cloudtrail.model.*;
/**
* Interface for accessing CloudTrail asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudtrail.AbstractAWSCloudTrailAsync} instead.
*
*
* AWS CloudTrail
*
* This is the CloudTrail API Reference. It provides descriptions of actions, data types, common parameters, and common
* errors for CloudTrail.
*
*
* CloudTrail is a web service that records AWS API calls for your AWS account and delivers log files to an Amazon S3
* bucket. The recorded information includes the identity of the user, the start time of the AWS API call, the source IP
* address, the request parameters, and the response elements returned by the service.
*
*
*
* As an alternative to the API, you can use one of the AWS SDKs, which consist of libraries and sample code for various
* programming languages and platforms (Java, Ruby, .NET, iOS, Android, etc.). The SDKs provide a convenient way to
* create programmatic access to AWSCloudTrail. For example, the SDKs take care of cryptographically signing requests,
* managing errors, and retrying requests automatically. For information about the AWS SDKs, including how to download
* and install them, see the Tools for Amazon Web Services page.
*
*
*
* See the AWS
* CloudTrail User Guide for information about the data that is included with each AWS API call listed in the log
* files.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSCloudTrailAsync extends AWSCloudTrail {
/**
*
* Adds one or more tags to a trail, up to a limit of 50. Overwrites an existing tag's value when a new value is
* specified for an existing tag key. Tag key names must be unique for a trail; you cannot have two keys with the
* same name but different values. If you specify a key without a value, the tag will be created with the specified
* key and a value of null. You can tag a trail that applies to all AWS Regions only from the Region in which the
* trail was created (also known as its home region).
*
*
* @param addTagsRequest
* Specifies the tags to add to a trail.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* @sample AWSCloudTrailAsync.AddTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsAsync(AddTagsRequest addTagsRequest);
/**
*
* Adds one or more tags to a trail, up to a limit of 50. Overwrites an existing tag's value when a new value is
* specified for an existing tag key. Tag key names must be unique for a trail; you cannot have two keys with the
* same name but different values. If you specify a key without a value, the tag will be created with the specified
* key and a value of null. You can tag a trail that applies to all AWS Regions only from the Region in which the
* trail was created (also known as its home region).
*
*
* @param addTagsRequest
* Specifies the tags to add to a trail.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.AddTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsAsync(AddTagsRequest addTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a trail that specifies the settings for delivery of log data to an Amazon S3 bucket.
*
*
* @param createTrailRequest
* Specifies the settings for each trail.
* @return A Java Future containing the result of the CreateTrail operation returned by the service.
* @sample AWSCloudTrailAsync.CreateTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTrailAsync(CreateTrailRequest createTrailRequest);
/**
*
* Creates a trail that specifies the settings for delivery of log data to an Amazon S3 bucket.
*
*
* @param createTrailRequest
* Specifies the settings for each trail.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrail operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.CreateTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTrailAsync(CreateTrailRequest createTrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a trail. This operation must be called from the region in which the trail was created.
* DeleteTrail
cannot be called on the shadow trails (replicated trails in other regions) of a trail
* that is enabled in all regions.
*
*
* @param deleteTrailRequest
* The request that specifies the name of a trail to delete.
* @return A Java Future containing the result of the DeleteTrail operation returned by the service.
* @sample AWSCloudTrailAsync.DeleteTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTrailAsync(DeleteTrailRequest deleteTrailRequest);
/**
*
* Deletes a trail. This operation must be called from the region in which the trail was created.
* DeleteTrail
cannot be called on the shadow trails (replicated trails in other regions) of a trail
* that is enabled in all regions.
*
*
* @param deleteTrailRequest
* The request that specifies the name of a trail to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTrail operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.DeleteTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTrailAsync(DeleteTrailRequest deleteTrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves settings for one or more trails associated with the current region for your account.
*
*
* @param describeTrailsRequest
* Returns information about the trail.
* @return A Java Future containing the result of the DescribeTrails operation returned by the service.
* @sample AWSCloudTrailAsync.DescribeTrails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTrailsAsync(DescribeTrailsRequest describeTrailsRequest);
/**
*
* Retrieves settings for one or more trails associated with the current region for your account.
*
*
* @param describeTrailsRequest
* Returns information about the trail.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTrails operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.DescribeTrails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTrailsAsync(DescribeTrailsRequest describeTrailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeTrails operation.
*
* @see #describeTrailsAsync(DescribeTrailsRequest)
*/
java.util.concurrent.Future describeTrailsAsync();
/**
* Simplified method form for invoking the DescribeTrails operation with an AsyncHandler.
*
* @see #describeTrailsAsync(DescribeTrailsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeTrailsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the settings for the event selectors that you configured for your trail. The information returned for
* your event selectors includes the following:
*
*
* -
*
* If your event selector includes read-only events, write-only events, or all events. This applies to both
* management events and data events.
*
*
* -
*
* If your event selector includes management events.
*
*
* -
*
* If your event selector includes data events, the Amazon S3 objects or AWS Lambda functions that you are logging
* for data events.
*
*
*
*
* For more information, see Logging Data and Management Events for Trails in the AWS CloudTrail User Guide.
*
*
* @param getEventSelectorsRequest
* @return A Java Future containing the result of the GetEventSelectors operation returned by the service.
* @sample AWSCloudTrailAsync.GetEventSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEventSelectorsAsync(GetEventSelectorsRequest getEventSelectorsRequest);
/**
*
* Describes the settings for the event selectors that you configured for your trail. The information returned for
* your event selectors includes the following:
*
*
* -
*
* If your event selector includes read-only events, write-only events, or all events. This applies to both
* management events and data events.
*
*
* -
*
* If your event selector includes management events.
*
*
* -
*
* If your event selector includes data events, the Amazon S3 objects or AWS Lambda functions that you are logging
* for data events.
*
*
*
*
* For more information, see Logging Data and Management Events for Trails in the AWS CloudTrail User Guide.
*
*
* @param getEventSelectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEventSelectors operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.GetEventSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEventSelectorsAsync(GetEventSelectorsRequest getEventSelectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the settings for the Insights event selectors that you configured for your trail.
* GetInsightSelectors
shows if CloudTrail Insights event logging is enabled on the trail, and if it
* is, which insight types are enabled. If you run GetInsightSelectors
on a trail that does not have
* Insights events enabled, the operation throws the exception InsightNotEnabledException
*
*
* For more information, see Logging CloudTrail Insights Events for Trails in the AWS CloudTrail User Guide.
*
*
* @param getInsightSelectorsRequest
* @return A Java Future containing the result of the GetInsightSelectors operation returned by the service.
* @sample AWSCloudTrailAsync.GetInsightSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getInsightSelectorsAsync(GetInsightSelectorsRequest getInsightSelectorsRequest);
/**
*
* Describes the settings for the Insights event selectors that you configured for your trail.
* GetInsightSelectors
shows if CloudTrail Insights event logging is enabled on the trail, and if it
* is, which insight types are enabled. If you run GetInsightSelectors
on a trail that does not have
* Insights events enabled, the operation throws the exception InsightNotEnabledException
*
*
* For more information, see Logging CloudTrail Insights Events for Trails in the AWS CloudTrail User Guide.
*
*
* @param getInsightSelectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInsightSelectors operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.GetInsightSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getInsightSelectorsAsync(GetInsightSelectorsRequest getInsightSelectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns settings information for a specified trail.
*
*
* @param getTrailRequest
* @return A Java Future containing the result of the GetTrail operation returned by the service.
* @sample AWSCloudTrailAsync.GetTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTrailAsync(GetTrailRequest getTrailRequest);
/**
*
* Returns settings information for a specified trail.
*
*
* @param getTrailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTrail operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.GetTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTrailAsync(GetTrailRequest getTrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a JSON-formatted list of information about the specified trail. Fields include information on delivery
* errors, Amazon SNS and Amazon S3 errors, and start and stop logging times for each trail. This operation returns
* trail status from a single region. To return trail status from all regions, you must call the operation on each
* region.
*
*
* @param getTrailStatusRequest
* The name of a trail about which you want the current status.
* @return A Java Future containing the result of the GetTrailStatus operation returned by the service.
* @sample AWSCloudTrailAsync.GetTrailStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTrailStatusAsync(GetTrailStatusRequest getTrailStatusRequest);
/**
*
* Returns a JSON-formatted list of information about the specified trail. Fields include information on delivery
* errors, Amazon SNS and Amazon S3 errors, and start and stop logging times for each trail. This operation returns
* trail status from a single region. To return trail status from all regions, you must call the operation on each
* region.
*
*
* @param getTrailStatusRequest
* The name of a trail about which you want the current status.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTrailStatus operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.GetTrailStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTrailStatusAsync(GetTrailStatusRequest getTrailStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all public keys whose private keys were used to sign the digest files within the specified time range.
* The public key is needed to validate digest files that were signed with its corresponding private key.
*
*
*
* CloudTrail uses different private/public key pairs per region. Each digest file is signed with a private key
* unique to its region. Therefore, when you validate a digest file from a particular region, you must look in the
* same region for its corresponding public key.
*
*
*
* @param listPublicKeysRequest
* Requests the public keys for a specified time range.
* @return A Java Future containing the result of the ListPublicKeys operation returned by the service.
* @sample AWSCloudTrailAsync.ListPublicKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPublicKeysAsync(ListPublicKeysRequest listPublicKeysRequest);
/**
*
* Returns all public keys whose private keys were used to sign the digest files within the specified time range.
* The public key is needed to validate digest files that were signed with its corresponding private key.
*
*
*
* CloudTrail uses different private/public key pairs per region. Each digest file is signed with a private key
* unique to its region. Therefore, when you validate a digest file from a particular region, you must look in the
* same region for its corresponding public key.
*
*
*
* @param listPublicKeysRequest
* Requests the public keys for a specified time range.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPublicKeys operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.ListPublicKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPublicKeysAsync(ListPublicKeysRequest listPublicKeysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListPublicKeys operation.
*
* @see #listPublicKeysAsync(ListPublicKeysRequest)
*/
java.util.concurrent.Future listPublicKeysAsync();
/**
* Simplified method form for invoking the ListPublicKeys operation with an AsyncHandler.
*
* @see #listPublicKeysAsync(ListPublicKeysRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listPublicKeysAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags for the trail in the current region.
*
*
* @param listTagsRequest
* Specifies a list of trail tags to return.
* @return A Java Future containing the result of the ListTags operation returned by the service.
* @sample AWSCloudTrailAsync.ListTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsAsync(ListTagsRequest listTagsRequest);
/**
*
* Lists the tags for the trail in the current region.
*
*
* @param listTagsRequest
* Specifies a list of trail tags to return.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTags operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.ListTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsAsync(ListTagsRequest listTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists trails that are in the current account.
*
*
* @param listTrailsRequest
* @return A Java Future containing the result of the ListTrails operation returned by the service.
* @sample AWSCloudTrailAsync.ListTrails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTrailsAsync(ListTrailsRequest listTrailsRequest);
/**
*
* Lists trails that are in the current account.
*
*
* @param listTrailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTrails operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.ListTrails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTrailsAsync(ListTrailsRequest listTrailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Looks up management events or CloudTrail Insights events that are captured by CloudTrail. You can look up events that occurred in a region
* within the last 90 days. Lookup supports the following attributes for management events:
*
*
* -
*
* AWS access key
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
* -
*
* Read only
*
*
* -
*
* Resource name
*
*
* -
*
* Resource type
*
*
* -
*
* User name
*
*
*
*
* Lookup supports the following attributes for Insights events:
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
*
*
* All attributes are optional. The default number of results returned is 50, with a maximum of 50 possible. The
* response includes a token that you can use to get the next page of results.
*
*
*
* The rate of lookup requests is limited to two per second, per account, per region. If this limit is exceeded, a
* throttling error occurs.
*
*
*
* @param lookupEventsRequest
* Contains a request for LookupEvents.
* @return A Java Future containing the result of the LookupEvents operation returned by the service.
* @sample AWSCloudTrailAsync.LookupEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future lookupEventsAsync(LookupEventsRequest lookupEventsRequest);
/**
*
* Looks up management events or CloudTrail Insights events that are captured by CloudTrail. You can look up events that occurred in a region
* within the last 90 days. Lookup supports the following attributes for management events:
*
*
* -
*
* AWS access key
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
* -
*
* Read only
*
*
* -
*
* Resource name
*
*
* -
*
* Resource type
*
*
* -
*
* User name
*
*
*
*
* Lookup supports the following attributes for Insights events:
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
*
*
* All attributes are optional. The default number of results returned is 50, with a maximum of 50 possible. The
* response includes a token that you can use to get the next page of results.
*
*
*
* The rate of lookup requests is limited to two per second, per account, per region. If this limit is exceeded, a
* throttling error occurs.
*
*
*
* @param lookupEventsRequest
* Contains a request for LookupEvents.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the LookupEvents operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.LookupEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future lookupEventsAsync(LookupEventsRequest lookupEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the LookupEvents operation.
*
* @see #lookupEventsAsync(LookupEventsRequest)
*/
java.util.concurrent.Future lookupEventsAsync();
/**
* Simplified method form for invoking the LookupEvents operation with an AsyncHandler.
*
* @see #lookupEventsAsync(LookupEventsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future lookupEventsAsync(com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures an event selector or advanced event selectors for your trail. Use event selectors or advanced event
* selectors to specify management and data event settings for your trail. By default, trails created without
* specific event selectors are configured to log all read and write management events, and no data events.
*
*
* When an event occurs in your account, CloudTrail evaluates the event selectors or advanced event selectors in all
* trails. For each trail, if the event matches any event selector, the trail processes and logs the event. If the
* event doesn't match any event selector, the trail doesn't log the event.
*
*
* Example
*
*
* -
*
* You create an event selector for a trail and specify that you want write-only events.
*
*
* -
*
* The EC2 GetConsoleOutput
and RunInstances
API operations occur in your account.
*
*
* -
*
* CloudTrail evaluates whether the events match your event selectors.
*
*
* -
*
* The RunInstances
is a write-only event and it matches your event selector. The trail logs the event.
*
*
* -
*
* The GetConsoleOutput
is a read-only event that doesn't match your event selector. The trail doesn't
* log the event.
*
*
*
*
* The PutEventSelectors
operation must be called from the region in which the trail was created;
* otherwise, an InvalidHomeRegionException
exception is thrown.
*
*
* You can configure up to five event selectors for each trail. For more information, see Logging data and management events for trails and Quotas in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*
* You can add advanced event selectors, and conditions for your advanced event selectors, up to a maximum of 500
* values for all conditions and selectors on a trail. You can use either AdvancedEventSelectors
or
* EventSelectors
, but not both. If you apply AdvancedEventSelectors
to a trail, any
* existing EventSelectors
are overwritten. For more information about advanced event selectors, see Logging
* data events for trails in the AWS CloudTrail User Guide.
*
*
* @param putEventSelectorsRequest
* @return A Java Future containing the result of the PutEventSelectors operation returned by the service.
* @sample AWSCloudTrailAsync.PutEventSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putEventSelectorsAsync(PutEventSelectorsRequest putEventSelectorsRequest);
/**
*
* Configures an event selector or advanced event selectors for your trail. Use event selectors or advanced event
* selectors to specify management and data event settings for your trail. By default, trails created without
* specific event selectors are configured to log all read and write management events, and no data events.
*
*
* When an event occurs in your account, CloudTrail evaluates the event selectors or advanced event selectors in all
* trails. For each trail, if the event matches any event selector, the trail processes and logs the event. If the
* event doesn't match any event selector, the trail doesn't log the event.
*
*
* Example
*
*
* -
*
* You create an event selector for a trail and specify that you want write-only events.
*
*
* -
*
* The EC2 GetConsoleOutput
and RunInstances
API operations occur in your account.
*
*
* -
*
* CloudTrail evaluates whether the events match your event selectors.
*
*
* -
*
* The RunInstances
is a write-only event and it matches your event selector. The trail logs the event.
*
*
* -
*
* The GetConsoleOutput
is a read-only event that doesn't match your event selector. The trail doesn't
* log the event.
*
*
*
*
* The PutEventSelectors
operation must be called from the region in which the trail was created;
* otherwise, an InvalidHomeRegionException
exception is thrown.
*
*
* You can configure up to five event selectors for each trail. For more information, see Logging data and management events for trails and Quotas in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*
* You can add advanced event selectors, and conditions for your advanced event selectors, up to a maximum of 500
* values for all conditions and selectors on a trail. You can use either AdvancedEventSelectors
or
* EventSelectors
, but not both. If you apply AdvancedEventSelectors
to a trail, any
* existing EventSelectors
are overwritten. For more information about advanced event selectors, see Logging
* data events for trails in the AWS CloudTrail User Guide.
*
*
* @param putEventSelectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEventSelectors operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.PutEventSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putEventSelectorsAsync(PutEventSelectorsRequest putEventSelectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lets you enable Insights event logging by specifying the Insights selectors that you want to enable on an
* existing trail. You also use PutInsightSelectors
to turn off Insights event logging, by passing an
* empty list of insight types. In this release, only ApiCallRateInsight
is supported as an Insights
* selector.
*
*
* @param putInsightSelectorsRequest
* @return A Java Future containing the result of the PutInsightSelectors operation returned by the service.
* @sample AWSCloudTrailAsync.PutInsightSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putInsightSelectorsAsync(PutInsightSelectorsRequest putInsightSelectorsRequest);
/**
*
* Lets you enable Insights event logging by specifying the Insights selectors that you want to enable on an
* existing trail. You also use PutInsightSelectors
to turn off Insights event logging, by passing an
* empty list of insight types. In this release, only ApiCallRateInsight
is supported as an Insights
* selector.
*
*
* @param putInsightSelectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutInsightSelectors operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.PutInsightSelectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putInsightSelectorsAsync(PutInsightSelectorsRequest putInsightSelectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from a trail.
*
*
* @param removeTagsRequest
* Specifies the tags to remove from a trail.
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* @sample AWSCloudTrailAsync.RemoveTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTagsAsync(RemoveTagsRequest removeTagsRequest);
/**
*
* Removes the specified tags from a trail.
*
*
* @param removeTagsRequest
* Specifies the tags to remove from a trail.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.RemoveTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTagsAsync(RemoveTagsRequest removeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the recording of AWS API calls and log file delivery for a trail. For a trail that is enabled in all
* regions, this operation must be called from the region in which the trail was created. This operation cannot be
* called on the shadow trails (replicated trails in other regions) of a trail that is enabled in all regions.
*
*
* @param startLoggingRequest
* The request to CloudTrail to start logging AWS API calls for an account.
* @return A Java Future containing the result of the StartLogging operation returned by the service.
* @sample AWSCloudTrailAsync.StartLogging
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startLoggingAsync(StartLoggingRequest startLoggingRequest);
/**
*
* Starts the recording of AWS API calls and log file delivery for a trail. For a trail that is enabled in all
* regions, this operation must be called from the region in which the trail was created. This operation cannot be
* called on the shadow trails (replicated trails in other regions) of a trail that is enabled in all regions.
*
*
* @param startLoggingRequest
* The request to CloudTrail to start logging AWS API calls for an account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartLogging operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.StartLogging
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startLoggingAsync(StartLoggingRequest startLoggingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Suspends the recording of AWS API calls and log file delivery for the specified trail. Under most circumstances,
* there is no need to use this action. You can update a trail without stopping it first. This action is the only
* way to stop recording. For a trail enabled in all regions, this operation must be called from the region in which
* the trail was created, or an InvalidHomeRegionException
will occur. This operation cannot be called
* on the shadow trails (replicated trails in other regions) of a trail enabled in all regions.
*
*
* @param stopLoggingRequest
* Passes the request to CloudTrail to stop logging AWS API calls for the specified account.
* @return A Java Future containing the result of the StopLogging operation returned by the service.
* @sample AWSCloudTrailAsync.StopLogging
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopLoggingAsync(StopLoggingRequest stopLoggingRequest);
/**
*
* Suspends the recording of AWS API calls and log file delivery for the specified trail. Under most circumstances,
* there is no need to use this action. You can update a trail without stopping it first. This action is the only
* way to stop recording. For a trail enabled in all regions, this operation must be called from the region in which
* the trail was created, or an InvalidHomeRegionException
will occur. This operation cannot be called
* on the shadow trails (replicated trails in other regions) of a trail enabled in all regions.
*
*
* @param stopLoggingRequest
* Passes the request to CloudTrail to stop logging AWS API calls for the specified account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopLogging operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.StopLogging
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopLoggingAsync(StopLoggingRequest stopLoggingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings that specify delivery of log files. Changes to a trail do not require stopping the
* CloudTrail service. Use this action to designate an existing bucket for log delivery. If the existing bucket has
* previously been a target for CloudTrail log files, an IAM policy exists for the bucket. UpdateTrail
* must be called from the region in which the trail was created; otherwise, an
* InvalidHomeRegionException
is thrown.
*
*
* @param updateTrailRequest
* Specifies settings to update for the trail.
* @return A Java Future containing the result of the UpdateTrail operation returned by the service.
* @sample AWSCloudTrailAsync.UpdateTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTrailAsync(UpdateTrailRequest updateTrailRequest);
/**
*
* Updates the settings that specify delivery of log files. Changes to a trail do not require stopping the
* CloudTrail service. Use this action to designate an existing bucket for log delivery. If the existing bucket has
* previously been a target for CloudTrail log files, an IAM policy exists for the bucket. UpdateTrail
* must be called from the region in which the trail was created; otherwise, an
* InvalidHomeRegionException
is thrown.
*
*
* @param updateTrailRequest
* Specifies settings to update for the trail.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTrail operation returned by the service.
* @sample AWSCloudTrailAsyncHandler.UpdateTrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTrailAsync(UpdateTrailRequest updateTrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}