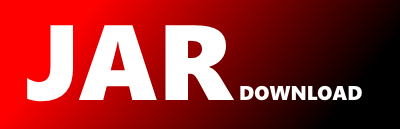
com.amazonaws.services.cloudtrail.model.CreateTrailRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Specifies the settings for each trail.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateTrailRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies the name of the trail. The name must meet the following requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
*
*/
private String name;
/**
*
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon S3
* Bucket Naming Requirements.
*
*/
private String s3BucketName;
/**
*
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log file
* delivery. For more information, see Finding Your
* CloudTrail Log Files. The maximum length is 200 characters.
*
*/
private String s3KeyPrefix;
/**
*
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum length is
* 256 characters.
*
*/
private String snsTopicName;
/**
*
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*
*/
private Boolean includeGlobalServiceEvents;
/**
*
* Specifies whether the trail is created in the current region or in all regions. The default is false, which
* creates a trail only in the region where you are signed in. As a best practice, consider creating trails that log
* events in all regions.
*
*/
private Boolean isMultiRegionTrail;
/**
*
* Specifies whether log file integrity validation is enabled. The default is false.
*
*
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour. CloudTrail
* will not create digest files for log files that were delivered during a period in which log file integrity
* validation was disabled. For example, if you enable log file integrity validation at noon on January 1, disable
* it at noon on January 2, and re-enable it at noon on January 10, digest files will not be created for the log
* files delivered from noon on January 2 to noon on January 10. The same applies whenever you stop CloudTrail
* logging or delete a trail.
*
*
*/
private Boolean enableLogFileValidation;
/**
*
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the log group
* to which CloudTrail logs will be delivered. Not required unless you specify CloudWatchLogsRoleArn.
*
*/
private String cloudWatchLogsLogGroupArn;
/**
*
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*
*/
private String cloudWatchLogsRoleArn;
/**
*
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias name
* prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a globally unique
* identifier.
*
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
*
*/
private String kmsKeyId;
/**
*
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only for the
* current AWS account. The default is false, and cannot be true unless the call is made on behalf of an AWS account
* that is the master account for an organization in AWS Organizations.
*
*/
private Boolean isOrganizationTrail;
private com.amazonaws.internal.SdkInternalList tagsList;
/**
*
* Specifies the name of the trail. The name must meet the following requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
*
*
* @param name
* Specifies the name of the trail. The name must meet the following requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
*/
public void setName(String name) {
this.name = name;
}
/**
*
* Specifies the name of the trail. The name must meet the following requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
*
*
* @return Specifies the name of the trail. The name must meet the following requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
*/
public String getName() {
return this.name;
}
/**
*
* Specifies the name of the trail. The name must meet the following requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
*
*
* @param name
* Specifies the name of the trail. The name must meet the following requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon S3
* Bucket Naming Requirements.
*
*
* @param s3BucketName
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon
* S3 Bucket Naming Requirements.
*/
public void setS3BucketName(String s3BucketName) {
this.s3BucketName = s3BucketName;
}
/**
*
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon S3
* Bucket Naming Requirements.
*
*
* @return Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon
* S3 Bucket Naming Requirements.
*/
public String getS3BucketName() {
return this.s3BucketName;
}
/**
*
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon S3
* Bucket Naming Requirements.
*
*
* @param s3BucketName
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon
* S3 Bucket Naming Requirements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withS3BucketName(String s3BucketName) {
setS3BucketName(s3BucketName);
return this;
}
/**
*
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log file
* delivery. For more information, see Finding Your
* CloudTrail Log Files. The maximum length is 200 characters.
*
*
* @param s3KeyPrefix
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log
* file delivery. For more information, see Finding
* Your CloudTrail Log Files. The maximum length is 200 characters.
*/
public void setS3KeyPrefix(String s3KeyPrefix) {
this.s3KeyPrefix = s3KeyPrefix;
}
/**
*
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log file
* delivery. For more information, see Finding Your
* CloudTrail Log Files. The maximum length is 200 characters.
*
*
* @return Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log
* file delivery. For more information, see Finding
* Your CloudTrail Log Files. The maximum length is 200 characters.
*/
public String getS3KeyPrefix() {
return this.s3KeyPrefix;
}
/**
*
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log file
* delivery. For more information, see Finding Your
* CloudTrail Log Files. The maximum length is 200 characters.
*
*
* @param s3KeyPrefix
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log
* file delivery. For more information, see Finding
* Your CloudTrail Log Files. The maximum length is 200 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withS3KeyPrefix(String s3KeyPrefix) {
setS3KeyPrefix(s3KeyPrefix);
return this;
}
/**
*
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum length is
* 256 characters.
*
*
* @param snsTopicName
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum
* length is 256 characters.
*/
public void setSnsTopicName(String snsTopicName) {
this.snsTopicName = snsTopicName;
}
/**
*
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum length is
* 256 characters.
*
*
* @return Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum
* length is 256 characters.
*/
public String getSnsTopicName() {
return this.snsTopicName;
}
/**
*
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum length is
* 256 characters.
*
*
* @param snsTopicName
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum
* length is 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withSnsTopicName(String snsTopicName) {
setSnsTopicName(snsTopicName);
return this;
}
/**
*
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*
*
* @param includeGlobalServiceEvents
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*/
public void setIncludeGlobalServiceEvents(Boolean includeGlobalServiceEvents) {
this.includeGlobalServiceEvents = includeGlobalServiceEvents;
}
/**
*
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*
*
* @return Specifies whether the trail is publishing events from global services such as IAM to the log files.
*/
public Boolean getIncludeGlobalServiceEvents() {
return this.includeGlobalServiceEvents;
}
/**
*
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*
*
* @param includeGlobalServiceEvents
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withIncludeGlobalServiceEvents(Boolean includeGlobalServiceEvents) {
setIncludeGlobalServiceEvents(includeGlobalServiceEvents);
return this;
}
/**
*
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*
*
* @return Specifies whether the trail is publishing events from global services such as IAM to the log files.
*/
public Boolean isIncludeGlobalServiceEvents() {
return this.includeGlobalServiceEvents;
}
/**
*
* Specifies whether the trail is created in the current region or in all regions. The default is false, which
* creates a trail only in the region where you are signed in. As a best practice, consider creating trails that log
* events in all regions.
*
*
* @param isMultiRegionTrail
* Specifies whether the trail is created in the current region or in all regions. The default is false,
* which creates a trail only in the region where you are signed in. As a best practice, consider creating
* trails that log events in all regions.
*/
public void setIsMultiRegionTrail(Boolean isMultiRegionTrail) {
this.isMultiRegionTrail = isMultiRegionTrail;
}
/**
*
* Specifies whether the trail is created in the current region or in all regions. The default is false, which
* creates a trail only in the region where you are signed in. As a best practice, consider creating trails that log
* events in all regions.
*
*
* @return Specifies whether the trail is created in the current region or in all regions. The default is false,
* which creates a trail only in the region where you are signed in. As a best practice, consider creating
* trails that log events in all regions.
*/
public Boolean getIsMultiRegionTrail() {
return this.isMultiRegionTrail;
}
/**
*
* Specifies whether the trail is created in the current region or in all regions. The default is false, which
* creates a trail only in the region where you are signed in. As a best practice, consider creating trails that log
* events in all regions.
*
*
* @param isMultiRegionTrail
* Specifies whether the trail is created in the current region or in all regions. The default is false,
* which creates a trail only in the region where you are signed in. As a best practice, consider creating
* trails that log events in all regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withIsMultiRegionTrail(Boolean isMultiRegionTrail) {
setIsMultiRegionTrail(isMultiRegionTrail);
return this;
}
/**
*
* Specifies whether the trail is created in the current region or in all regions. The default is false, which
* creates a trail only in the region where you are signed in. As a best practice, consider creating trails that log
* events in all regions.
*
*
* @return Specifies whether the trail is created in the current region or in all regions. The default is false,
* which creates a trail only in the region where you are signed in. As a best practice, consider creating
* trails that log events in all regions.
*/
public Boolean isMultiRegionTrail() {
return this.isMultiRegionTrail;
}
/**
*
* Specifies whether log file integrity validation is enabled. The default is false.
*
*
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour. CloudTrail
* will not create digest files for log files that were delivered during a period in which log file integrity
* validation was disabled. For example, if you enable log file integrity validation at noon on January 1, disable
* it at noon on January 2, and re-enable it at noon on January 10, digest files will not be created for the log
* files delivered from noon on January 2 to noon on January 10. The same applies whenever you stop CloudTrail
* logging or delete a trail.
*
*
*
* @param enableLogFileValidation
* Specifies whether log file integrity validation is enabled. The default is false.
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour.
* CloudTrail will not create digest files for log files that were delivered during a period in which log
* file integrity validation was disabled. For example, if you enable log file integrity validation at noon
* on January 1, disable it at noon on January 2, and re-enable it at noon on January 10, digest files will
* not be created for the log files delivered from noon on January 2 to noon on January 10. The same applies
* whenever you stop CloudTrail logging or delete a trail.
*
*/
public void setEnableLogFileValidation(Boolean enableLogFileValidation) {
this.enableLogFileValidation = enableLogFileValidation;
}
/**
*
* Specifies whether log file integrity validation is enabled. The default is false.
*
*
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour. CloudTrail
* will not create digest files for log files that were delivered during a period in which log file integrity
* validation was disabled. For example, if you enable log file integrity validation at noon on January 1, disable
* it at noon on January 2, and re-enable it at noon on January 10, digest files will not be created for the log
* files delivered from noon on January 2 to noon on January 10. The same applies whenever you stop CloudTrail
* logging or delete a trail.
*
*
*
* @return Specifies whether log file integrity validation is enabled. The default is false.
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour.
* CloudTrail will not create digest files for log files that were delivered during a period in which log
* file integrity validation was disabled. For example, if you enable log file integrity validation at noon
* on January 1, disable it at noon on January 2, and re-enable it at noon on January 10, digest files will
* not be created for the log files delivered from noon on January 2 to noon on January 10. The same applies
* whenever you stop CloudTrail logging or delete a trail.
*
*/
public Boolean getEnableLogFileValidation() {
return this.enableLogFileValidation;
}
/**
*
* Specifies whether log file integrity validation is enabled. The default is false.
*
*
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour. CloudTrail
* will not create digest files for log files that were delivered during a period in which log file integrity
* validation was disabled. For example, if you enable log file integrity validation at noon on January 1, disable
* it at noon on January 2, and re-enable it at noon on January 10, digest files will not be created for the log
* files delivered from noon on January 2 to noon on January 10. The same applies whenever you stop CloudTrail
* logging or delete a trail.
*
*
*
* @param enableLogFileValidation
* Specifies whether log file integrity validation is enabled. The default is false.
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour.
* CloudTrail will not create digest files for log files that were delivered during a period in which log
* file integrity validation was disabled. For example, if you enable log file integrity validation at noon
* on January 1, disable it at noon on January 2, and re-enable it at noon on January 10, digest files will
* not be created for the log files delivered from noon on January 2 to noon on January 10. The same applies
* whenever you stop CloudTrail logging or delete a trail.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withEnableLogFileValidation(Boolean enableLogFileValidation) {
setEnableLogFileValidation(enableLogFileValidation);
return this;
}
/**
*
* Specifies whether log file integrity validation is enabled. The default is false.
*
*
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour. CloudTrail
* will not create digest files for log files that were delivered during a period in which log file integrity
* validation was disabled. For example, if you enable log file integrity validation at noon on January 1, disable
* it at noon on January 2, and re-enable it at noon on January 10, digest files will not be created for the log
* files delivered from noon on January 2 to noon on January 10. The same applies whenever you stop CloudTrail
* logging or delete a trail.
*
*
*
* @return Specifies whether log file integrity validation is enabled. The default is false.
*
* When you disable log file integrity validation, the chain of digest files is broken after one hour.
* CloudTrail will not create digest files for log files that were delivered during a period in which log
* file integrity validation was disabled. For example, if you enable log file integrity validation at noon
* on January 1, disable it at noon on January 2, and re-enable it at noon on January 10, digest files will
* not be created for the log files delivered from noon on January 2 to noon on January 10. The same applies
* whenever you stop CloudTrail logging or delete a trail.
*
*/
public Boolean isEnableLogFileValidation() {
return this.enableLogFileValidation;
}
/**
*
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the log group
* to which CloudTrail logs will be delivered. Not required unless you specify CloudWatchLogsRoleArn.
*
*
* @param cloudWatchLogsLogGroupArn
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the
* log group to which CloudTrail logs will be delivered. Not required unless you specify
* CloudWatchLogsRoleArn.
*/
public void setCloudWatchLogsLogGroupArn(String cloudWatchLogsLogGroupArn) {
this.cloudWatchLogsLogGroupArn = cloudWatchLogsLogGroupArn;
}
/**
*
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the log group
* to which CloudTrail logs will be delivered. Not required unless you specify CloudWatchLogsRoleArn.
*
*
* @return Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the
* log group to which CloudTrail logs will be delivered. Not required unless you specify
* CloudWatchLogsRoleArn.
*/
public String getCloudWatchLogsLogGroupArn() {
return this.cloudWatchLogsLogGroupArn;
}
/**
*
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the log group
* to which CloudTrail logs will be delivered. Not required unless you specify CloudWatchLogsRoleArn.
*
*
* @param cloudWatchLogsLogGroupArn
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the
* log group to which CloudTrail logs will be delivered. Not required unless you specify
* CloudWatchLogsRoleArn.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withCloudWatchLogsLogGroupArn(String cloudWatchLogsLogGroupArn) {
setCloudWatchLogsLogGroupArn(cloudWatchLogsLogGroupArn);
return this;
}
/**
*
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*
*
* @param cloudWatchLogsRoleArn
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*/
public void setCloudWatchLogsRoleArn(String cloudWatchLogsRoleArn) {
this.cloudWatchLogsRoleArn = cloudWatchLogsRoleArn;
}
/**
*
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*
*
* @return Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*/
public String getCloudWatchLogsRoleArn() {
return this.cloudWatchLogsRoleArn;
}
/**
*
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*
*
* @param cloudWatchLogsRoleArn
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withCloudWatchLogsRoleArn(String cloudWatchLogsRoleArn) {
setCloudWatchLogsRoleArn(cloudWatchLogsRoleArn);
return this;
}
/**
*
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias name
* prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a globally unique
* identifier.
*
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
*
*
* @param kmsKeyId
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias
* name prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a
* globally unique identifier.
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias name
* prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a globally unique
* identifier.
*
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
*
*
* @return Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias
* name prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a
* globally unique identifier.
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias name
* prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a globally unique
* identifier.
*
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
*
*
* @param kmsKeyId
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias
* name prefixed by "alias/", a fully specified ARN to an alias, a fully specified ARN to a key, or a
* globally unique identifier.
*
* Examples:
*
*
* -
*
* alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:alias/MyAliasName
*
*
* -
*
* arn:aws:kms:us-east-2:123456789012:key/12345678-1234-1234-1234-123456789012
*
*
* -
*
* 12345678-1234-1234-1234-123456789012
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only for the
* current AWS account. The default is false, and cannot be true unless the call is made on behalf of an AWS account
* that is the master account for an organization in AWS Organizations.
*
*
* @param isOrganizationTrail
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only
* for the current AWS account. The default is false, and cannot be true unless the call is made on behalf of
* an AWS account that is the master account for an organization in AWS Organizations.
*/
public void setIsOrganizationTrail(Boolean isOrganizationTrail) {
this.isOrganizationTrail = isOrganizationTrail;
}
/**
*
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only for the
* current AWS account. The default is false, and cannot be true unless the call is made on behalf of an AWS account
* that is the master account for an organization in AWS Organizations.
*
*
* @return Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only
* for the current AWS account. The default is false, and cannot be true unless the call is made on behalf
* of an AWS account that is the master account for an organization in AWS Organizations.
*/
public Boolean getIsOrganizationTrail() {
return this.isOrganizationTrail;
}
/**
*
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only for the
* current AWS account. The default is false, and cannot be true unless the call is made on behalf of an AWS account
* that is the master account for an organization in AWS Organizations.
*
*
* @param isOrganizationTrail
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only
* for the current AWS account. The default is false, and cannot be true unless the call is made on behalf of
* an AWS account that is the master account for an organization in AWS Organizations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withIsOrganizationTrail(Boolean isOrganizationTrail) {
setIsOrganizationTrail(isOrganizationTrail);
return this;
}
/**
*
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only for the
* current AWS account. The default is false, and cannot be true unless the call is made on behalf of an AWS account
* that is the master account for an organization in AWS Organizations.
*
*
* @return Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only
* for the current AWS account. The default is false, and cannot be true unless the call is made on behalf
* of an AWS account that is the master account for an organization in AWS Organizations.
*/
public Boolean isOrganizationTrail() {
return this.isOrganizationTrail;
}
/**
* @return
*/
public java.util.List getTagsList() {
if (tagsList == null) {
tagsList = new com.amazonaws.internal.SdkInternalList();
}
return tagsList;
}
/**
* @param tagsList
*/
public void setTagsList(java.util.Collection tagsList) {
if (tagsList == null) {
this.tagsList = null;
return;
}
this.tagsList = new com.amazonaws.internal.SdkInternalList(tagsList);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagsList(java.util.Collection)} or {@link #withTagsList(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param tagsList
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withTagsList(Tag... tagsList) {
if (this.tagsList == null) {
setTagsList(new com.amazonaws.internal.SdkInternalList(tagsList.length));
}
for (Tag ele : tagsList) {
this.tagsList.add(ele);
}
return this;
}
/**
* @param tagsList
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrailRequest withTagsList(java.util.Collection tagsList) {
setTagsList(tagsList);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getS3BucketName() != null)
sb.append("S3BucketName: ").append(getS3BucketName()).append(",");
if (getS3KeyPrefix() != null)
sb.append("S3KeyPrefix: ").append(getS3KeyPrefix()).append(",");
if (getSnsTopicName() != null)
sb.append("SnsTopicName: ").append(getSnsTopicName()).append(",");
if (getIncludeGlobalServiceEvents() != null)
sb.append("IncludeGlobalServiceEvents: ").append(getIncludeGlobalServiceEvents()).append(",");
if (getIsMultiRegionTrail() != null)
sb.append("IsMultiRegionTrail: ").append(getIsMultiRegionTrail()).append(",");
if (getEnableLogFileValidation() != null)
sb.append("EnableLogFileValidation: ").append(getEnableLogFileValidation()).append(",");
if (getCloudWatchLogsLogGroupArn() != null)
sb.append("CloudWatchLogsLogGroupArn: ").append(getCloudWatchLogsLogGroupArn()).append(",");
if (getCloudWatchLogsRoleArn() != null)
sb.append("CloudWatchLogsRoleArn: ").append(getCloudWatchLogsRoleArn()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getIsOrganizationTrail() != null)
sb.append("IsOrganizationTrail: ").append(getIsOrganizationTrail()).append(",");
if (getTagsList() != null)
sb.append("TagsList: ").append(getTagsList());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateTrailRequest == false)
return false;
CreateTrailRequest other = (CreateTrailRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getS3BucketName() == null ^ this.getS3BucketName() == null)
return false;
if (other.getS3BucketName() != null && other.getS3BucketName().equals(this.getS3BucketName()) == false)
return false;
if (other.getS3KeyPrefix() == null ^ this.getS3KeyPrefix() == null)
return false;
if (other.getS3KeyPrefix() != null && other.getS3KeyPrefix().equals(this.getS3KeyPrefix()) == false)
return false;
if (other.getSnsTopicName() == null ^ this.getSnsTopicName() == null)
return false;
if (other.getSnsTopicName() != null && other.getSnsTopicName().equals(this.getSnsTopicName()) == false)
return false;
if (other.getIncludeGlobalServiceEvents() == null ^ this.getIncludeGlobalServiceEvents() == null)
return false;
if (other.getIncludeGlobalServiceEvents() != null && other.getIncludeGlobalServiceEvents().equals(this.getIncludeGlobalServiceEvents()) == false)
return false;
if (other.getIsMultiRegionTrail() == null ^ this.getIsMultiRegionTrail() == null)
return false;
if (other.getIsMultiRegionTrail() != null && other.getIsMultiRegionTrail().equals(this.getIsMultiRegionTrail()) == false)
return false;
if (other.getEnableLogFileValidation() == null ^ this.getEnableLogFileValidation() == null)
return false;
if (other.getEnableLogFileValidation() != null && other.getEnableLogFileValidation().equals(this.getEnableLogFileValidation()) == false)
return false;
if (other.getCloudWatchLogsLogGroupArn() == null ^ this.getCloudWatchLogsLogGroupArn() == null)
return false;
if (other.getCloudWatchLogsLogGroupArn() != null && other.getCloudWatchLogsLogGroupArn().equals(this.getCloudWatchLogsLogGroupArn()) == false)
return false;
if (other.getCloudWatchLogsRoleArn() == null ^ this.getCloudWatchLogsRoleArn() == null)
return false;
if (other.getCloudWatchLogsRoleArn() != null && other.getCloudWatchLogsRoleArn().equals(this.getCloudWatchLogsRoleArn()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getIsOrganizationTrail() == null ^ this.getIsOrganizationTrail() == null)
return false;
if (other.getIsOrganizationTrail() != null && other.getIsOrganizationTrail().equals(this.getIsOrganizationTrail()) == false)
return false;
if (other.getTagsList() == null ^ this.getTagsList() == null)
return false;
if (other.getTagsList() != null && other.getTagsList().equals(this.getTagsList()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getS3BucketName() == null) ? 0 : getS3BucketName().hashCode());
hashCode = prime * hashCode + ((getS3KeyPrefix() == null) ? 0 : getS3KeyPrefix().hashCode());
hashCode = prime * hashCode + ((getSnsTopicName() == null) ? 0 : getSnsTopicName().hashCode());
hashCode = prime * hashCode + ((getIncludeGlobalServiceEvents() == null) ? 0 : getIncludeGlobalServiceEvents().hashCode());
hashCode = prime * hashCode + ((getIsMultiRegionTrail() == null) ? 0 : getIsMultiRegionTrail().hashCode());
hashCode = prime * hashCode + ((getEnableLogFileValidation() == null) ? 0 : getEnableLogFileValidation().hashCode());
hashCode = prime * hashCode + ((getCloudWatchLogsLogGroupArn() == null) ? 0 : getCloudWatchLogsLogGroupArn().hashCode());
hashCode = prime * hashCode + ((getCloudWatchLogsRoleArn() == null) ? 0 : getCloudWatchLogsRoleArn().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getIsOrganizationTrail() == null) ? 0 : getIsOrganizationTrail().hashCode());
hashCode = prime * hashCode + ((getTagsList() == null) ? 0 : getTagsList().hashCode());
return hashCode;
}
@Override
public CreateTrailRequest clone() {
return (CreateTrailRequest) super.clone();
}
}