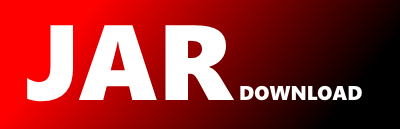
com.amazonaws.services.cloudtrail.model.DataResource Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The Amazon S3 buckets or AWS Lambda functions that you specify in your event selectors for your trail to log data
* events. Data events provide information about the resource operations performed on or within a resource itself. These
* are also known as data plane operations. You can specify up to 250 data resources for a trail.
*
*
*
* The total number of allowed data resources is 250. This number can be distributed between 1 and 5 event selectors,
* but the total cannot exceed 250 across all selectors.
*
*
* If you are using advanced event selectors, the maximum total number of values for all conditions, across all advanced
* event selectors for the trail, is 500.
*
*
*
* The following example demonstrates how logging works when you configure logging of all data events for an S3 bucket
* named bucket-1
. In this example, the CloudTrail user specified an empty prefix, and the option to log
* both Read
and Write
data events.
*
*
* -
*
* A user uploads an image file to bucket-1
.
*
*
* -
*
* The PutObject
API operation is an Amazon S3 object-level API. It is recorded as a data event in
* CloudTrail. Because the CloudTrail user specified an S3 bucket with an empty prefix, events that occur on any object
* in that bucket are logged. The trail processes and logs the event.
*
*
* -
*
* A user uploads an object to an Amazon S3 bucket named arn:aws:s3:::bucket-2
.
*
*
* -
*
* The PutObject
API operation occurred for an object in an S3 bucket that the CloudTrail user didn't
* specify for the trail. The trail doesn’t log the event.
*
*
*
*
* The following example demonstrates how logging works when you configure logging of AWS Lambda data events for a
* Lambda function named MyLambdaFunction, but not for all AWS Lambda functions.
*
*
* -
*
* A user runs a script that includes a call to the MyLambdaFunction function and the
* MyOtherLambdaFunction function.
*
*
* -
*
* The Invoke
API operation on MyLambdaFunction is an AWS Lambda API. It is recorded as a data event
* in CloudTrail. Because the CloudTrail user specified logging data events for MyLambdaFunction, any invocations
* of that function are logged. The trail processes and logs the event.
*
*
* -
*
* The Invoke
API operation on MyOtherLambdaFunction is an AWS Lambda API. Because the CloudTrail
* user did not specify logging data events for all Lambda functions, the Invoke
operation for
* MyOtherLambdaFunction does not match the function specified for the trail. The trail doesn’t log the event.
*
*
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataResource implements Serializable, Cloneable, StructuredPojo {
/**
*
* The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data events
* on this resource type, use advanced event selectors.
*
*/
private String type;
/**
*
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account, even if
* that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3 bucket that
* match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS account,
* even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList values;
/**
*
* The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data events
* on this resource type, use advanced event selectors.
*
*
* @param type
* The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data
* events on this resource type, use advanced event selectors.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data events
* on this resource type, use advanced event selectors.
*
*
* @return The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data
* events on this resource type, use advanced event selectors.
*/
public String getType() {
return this.type;
}
/**
*
* The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data events
* on this resource type, use advanced event selectors.
*
*
* @param type
* The resource type in which you want to log data events. You can specify AWS::S3::Object
or
* AWS::Lambda::Function
resources.
*
* The AWS::S3Outposts::Object
resource type is not valid in basic event selectors. To log data
* events on this resource type, use advanced event selectors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataResource withType(String type) {
setType(type);
return this;
}
/**
*
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account, even if
* that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3 bucket that
* match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS account,
* even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*
*
* @return An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account,
* even if that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3
* bucket that match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as
* arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS
* account, even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*/
public java.util.List getValues() {
if (values == null) {
values = new com.amazonaws.internal.SdkInternalList();
}
return values;
}
/**
*
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account, even if
* that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3 bucket that
* match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS account,
* even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*
*
* @param values
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account,
* even if that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3
* bucket that match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as
* arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS
* account, even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*/
public void setValues(java.util.Collection values) {
if (values == null) {
this.values = null;
return;
}
this.values = new com.amazonaws.internal.SdkInternalList(values);
}
/**
*
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account, even if
* that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3 bucket that
* match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS account,
* even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValues(java.util.Collection)} or {@link #withValues(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param values
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account,
* even if that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3
* bucket that match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as
* arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS
* account, even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataResource withValues(String... values) {
if (this.values == null) {
setValues(new com.amazonaws.internal.SdkInternalList(values.length));
}
for (String ele : values) {
this.values.add(ele);
}
return this;
}
/**
*
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account, even if
* that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3 bucket that
* match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS account,
* even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
*
*
* @param values
* An array of Amazon Resource Name (ARN) strings or partial ARN strings for the specified objects.
*
* -
*
* To log data events for all objects in all S3 buckets in your AWS account, specify the prefix as
* arn:aws:s3:::
.
*
*
*
* This will also enable logging of data event activity performed by any user or role in your AWS account,
* even if that activity is performed on a bucket that belongs to another AWS account.
*
*
* -
*
* To log data events for all objects in an S3 bucket, specify the bucket and an empty object prefix such as
* arn:aws:s3:::bucket-1/
. The trail logs data events for all objects in this S3 bucket.
*
*
* -
*
* To log data events for specific objects, specify the S3 bucket and object prefix such as
* arn:aws:s3:::bucket-1/example-images
. The trail logs data events for objects in this S3
* bucket that match the prefix.
*
*
* -
*
* To log data events for all functions in your AWS account, specify the prefix as
* arn:aws:lambda
.
*
*
*
* This will also enable logging of Invoke
activity performed by any user or role in your AWS
* account, even if that activity is performed on a function that belongs to another AWS account.
*
*
* -
*
* To log data events for a specific Lambda function, specify the function ARN.
*
*
*
* Lambda function ARNs are exact. For example, if you specify a function ARN
* arn:aws:lambda:us-west-2:111111111111:function:helloworld, data events will only be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld. They will not be logged for
* arn:aws:lambda:us-west-2:111111111111:function:helloworld2.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataResource withValues(java.util.Collection values) {
setValues(values);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getValues() != null)
sb.append("Values: ").append(getValues());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataResource == false)
return false;
DataResource other = (DataResource) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getValues() == null ^ this.getValues() == null)
return false;
if (other.getValues() != null && other.getValues().equals(this.getValues()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getValues() == null) ? 0 : getValues().hashCode());
return hashCode;
}
@Override
public DataResource clone() {
try {
return (DataResource) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.cloudtrail.model.transform.DataResourceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}