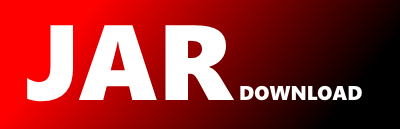
com.amazonaws.services.cloudtrail.model.EventSelector Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Use event selectors to further specify the management and data event settings for your trail. By default, trails
* created without specific event selectors will be configured to log all read and write management events, and no data
* events. When an event occurs in your account, CloudTrail evaluates the event selector for all trails. For each trail,
* if the event matches any event selector, the trail processes and logs the event. If the event doesn't match any event
* selector, the trail doesn't log the event.
*
*
* You can configure up to five event selectors for a trail.
*
*
* You cannot apply both event selectors and advanced event selectors to a trail.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EventSelector implements Serializable, Cloneable, StructuredPojo {
/**
*
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only API
* operation.
*
*
* By default, the value is All
.
*
*/
private String readWriteType;
/**
*
* Specify if you want your event selector to include management events for your trail.
*
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events that you
* are logging on any subsequent trail in the same region. For more information about CloudTrail pricing, see AWS CloudTrail Pricing.
*
*/
private Boolean includeManagementEvents;
/**
*
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up to 250
* resources for an individual event selector, but the total number of data resources cannot exceed 250 across all
* event selectors in a trail. This limit does not apply if you configure resource logging for all data events.
*
*
* For more information, see Data Events and Limits in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList dataResources;
/**
*
* An optional list of service event sources from which you do not want management events to be logged on your
* trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key Management
* Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are logged to
* your trail.
*
*/
private com.amazonaws.internal.SdkInternalList excludeManagementEventSources;
/**
*
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only API
* operation.
*
*
* By default, the value is All
.
*
*
* @param readWriteType
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only
* API operation.
*
* By default, the value is All
.
* @see ReadWriteType
*/
public void setReadWriteType(String readWriteType) {
this.readWriteType = readWriteType;
}
/**
*
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only API
* operation.
*
*
* By default, the value is All
.
*
*
* @return Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only
* API operation.
*
* By default, the value is All
.
* @see ReadWriteType
*/
public String getReadWriteType() {
return this.readWriteType;
}
/**
*
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only API
* operation.
*
*
* By default, the value is All
.
*
*
* @param readWriteType
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only
* API operation.
*
* By default, the value is All
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReadWriteType
*/
public EventSelector withReadWriteType(String readWriteType) {
setReadWriteType(readWriteType);
return this;
}
/**
*
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only API
* operation.
*
*
* By default, the value is All
.
*
*
* @param readWriteType
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only
* API operation.
*
* By default, the value is All
.
* @see ReadWriteType
*/
public void setReadWriteType(ReadWriteType readWriteType) {
withReadWriteType(readWriteType);
}
/**
*
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only API
* operation.
*
*
* By default, the value is All
.
*
*
* @param readWriteType
* Specify if you want your trail to log read-only events, write-only events, or all. For example, the EC2
* GetConsoleOutput
is a read-only API operation and RunInstances
is a write-only
* API operation.
*
* By default, the value is All
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReadWriteType
*/
public EventSelector withReadWriteType(ReadWriteType readWriteType) {
this.readWriteType = readWriteType.toString();
return this;
}
/**
*
* Specify if you want your event selector to include management events for your trail.
*
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events that you
* are logging on any subsequent trail in the same region. For more information about CloudTrail pricing, see AWS CloudTrail Pricing.
*
*
* @param includeManagementEvents
* Specify if you want your event selector to include management events for your trail.
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events
* that you are logging on any subsequent trail in the same region. For more information about CloudTrail
* pricing, see AWS CloudTrail Pricing.
*/
public void setIncludeManagementEvents(Boolean includeManagementEvents) {
this.includeManagementEvents = includeManagementEvents;
}
/**
*
* Specify if you want your event selector to include management events for your trail.
*
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events that you
* are logging on any subsequent trail in the same region. For more information about CloudTrail pricing, see AWS CloudTrail Pricing.
*
*
* @return Specify if you want your event selector to include management events for your trail.
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events
* that you are logging on any subsequent trail in the same region. For more information about CloudTrail
* pricing, see AWS CloudTrail Pricing.
*/
public Boolean getIncludeManagementEvents() {
return this.includeManagementEvents;
}
/**
*
* Specify if you want your event selector to include management events for your trail.
*
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events that you
* are logging on any subsequent trail in the same region. For more information about CloudTrail pricing, see AWS CloudTrail Pricing.
*
*
* @param includeManagementEvents
* Specify if you want your event selector to include management events for your trail.
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events
* that you are logging on any subsequent trail in the same region. For more information about CloudTrail
* pricing, see AWS CloudTrail Pricing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EventSelector withIncludeManagementEvents(Boolean includeManagementEvents) {
setIncludeManagementEvents(includeManagementEvents);
return this;
}
/**
*
* Specify if you want your event selector to include management events for your trail.
*
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events that you
* are logging on any subsequent trail in the same region. For more information about CloudTrail pricing, see AWS CloudTrail Pricing.
*
*
* @return Specify if you want your event selector to include management events for your trail.
*
* For more information, see Management Events in the AWS CloudTrail User Guide.
*
*
* By default, the value is true
.
*
*
* The first copy of management events is free. You are charged for additional copies of management events
* that you are logging on any subsequent trail in the same region. For more information about CloudTrail
* pricing, see AWS CloudTrail Pricing.
*/
public Boolean isIncludeManagementEvents() {
return this.includeManagementEvents;
}
/**
*
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up to 250
* resources for an individual event selector, but the total number of data resources cannot exceed 250 across all
* event selectors in a trail. This limit does not apply if you configure resource logging for all data events.
*
*
* For more information, see Data Events and Limits in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*
* @return CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up
* to 250 resources for an individual event selector, but the total number of data resources cannot exceed
* 250 across all event selectors in a trail. This limit does not apply if you configure resource logging
* for all data events.
*
* For more information, see Data Events and Limits in
* AWS CloudTrail in the AWS CloudTrail User Guide.
*/
public java.util.List getDataResources() {
if (dataResources == null) {
dataResources = new com.amazonaws.internal.SdkInternalList();
}
return dataResources;
}
/**
*
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up to 250
* resources for an individual event selector, but the total number of data resources cannot exceed 250 across all
* event selectors in a trail. This limit does not apply if you configure resource logging for all data events.
*
*
* For more information, see Data Events and Limits in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*
* @param dataResources
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up
* to 250 resources for an individual event selector, but the total number of data resources cannot exceed
* 250 across all event selectors in a trail. This limit does not apply if you configure resource logging for
* all data events.
*
* For more information, see Data Events and Limits in
* AWS CloudTrail in the AWS CloudTrail User Guide.
*/
public void setDataResources(java.util.Collection dataResources) {
if (dataResources == null) {
this.dataResources = null;
return;
}
this.dataResources = new com.amazonaws.internal.SdkInternalList(dataResources);
}
/**
*
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up to 250
* resources for an individual event selector, but the total number of data resources cannot exceed 250 across all
* event selectors in a trail. This limit does not apply if you configure resource logging for all data events.
*
*
* For more information, see Data Events and Limits in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDataResources(java.util.Collection)} or {@link #withDataResources(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param dataResources
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up
* to 250 resources for an individual event selector, but the total number of data resources cannot exceed
* 250 across all event selectors in a trail. This limit does not apply if you configure resource logging for
* all data events.
*
* For more information, see Data Events and Limits in
* AWS CloudTrail in the AWS CloudTrail User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EventSelector withDataResources(DataResource... dataResources) {
if (this.dataResources == null) {
setDataResources(new com.amazonaws.internal.SdkInternalList(dataResources.length));
}
for (DataResource ele : dataResources) {
this.dataResources.add(ele);
}
return this;
}
/**
*
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up to 250
* resources for an individual event selector, but the total number of data resources cannot exceed 250 across all
* event selectors in a trail. This limit does not apply if you configure resource logging for all data events.
*
*
* For more information, see Data Events and Limits in AWS
* CloudTrail in the AWS CloudTrail User Guide.
*
*
* @param dataResources
* CloudTrail supports data event logging for Amazon S3 objects and AWS Lambda functions. You can specify up
* to 250 resources for an individual event selector, but the total number of data resources cannot exceed
* 250 across all event selectors in a trail. This limit does not apply if you configure resource logging for
* all data events.
*
* For more information, see Data Events and Limits in
* AWS CloudTrail in the AWS CloudTrail User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EventSelector withDataResources(java.util.Collection dataResources) {
setDataResources(dataResources);
return this;
}
/**
*
* An optional list of service event sources from which you do not want management events to be logged on your
* trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key Management
* Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are logged to
* your trail.
*
*
* @return An optional list of service event sources from which you do not want management events to be logged on
* your trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key
* Management Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are
* logged to your trail.
*/
public java.util.List getExcludeManagementEventSources() {
if (excludeManagementEventSources == null) {
excludeManagementEventSources = new com.amazonaws.internal.SdkInternalList();
}
return excludeManagementEventSources;
}
/**
*
* An optional list of service event sources from which you do not want management events to be logged on your
* trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key Management
* Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are logged to
* your trail.
*
*
* @param excludeManagementEventSources
* An optional list of service event sources from which you do not want management events to be logged on
* your trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key
* Management Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are
* logged to your trail.
*/
public void setExcludeManagementEventSources(java.util.Collection excludeManagementEventSources) {
if (excludeManagementEventSources == null) {
this.excludeManagementEventSources = null;
return;
}
this.excludeManagementEventSources = new com.amazonaws.internal.SdkInternalList(excludeManagementEventSources);
}
/**
*
* An optional list of service event sources from which you do not want management events to be logged on your
* trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key Management
* Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are logged to
* your trail.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExcludeManagementEventSources(java.util.Collection)} or
* {@link #withExcludeManagementEventSources(java.util.Collection)} if you want to override the existing values.
*
*
* @param excludeManagementEventSources
* An optional list of service event sources from which you do not want management events to be logged on
* your trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key
* Management Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are
* logged to your trail.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EventSelector withExcludeManagementEventSources(String... excludeManagementEventSources) {
if (this.excludeManagementEventSources == null) {
setExcludeManagementEventSources(new com.amazonaws.internal.SdkInternalList(excludeManagementEventSources.length));
}
for (String ele : excludeManagementEventSources) {
this.excludeManagementEventSources.add(ele);
}
return this;
}
/**
*
* An optional list of service event sources from which you do not want management events to be logged on your
* trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key Management
* Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are logged to
* your trail.
*
*
* @param excludeManagementEventSources
* An optional list of service event sources from which you do not want management events to be logged on
* your trail. In this release, the list can be empty (disables the filter), or it can filter out AWS Key
* Management Service events by containing "kms.amazonaws.com"
. By default,
* ExcludeManagementEventSources
is empty, and AWS KMS events are included in events that are
* logged to your trail.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EventSelector withExcludeManagementEventSources(java.util.Collection excludeManagementEventSources) {
setExcludeManagementEventSources(excludeManagementEventSources);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReadWriteType() != null)
sb.append("ReadWriteType: ").append(getReadWriteType()).append(",");
if (getIncludeManagementEvents() != null)
sb.append("IncludeManagementEvents: ").append(getIncludeManagementEvents()).append(",");
if (getDataResources() != null)
sb.append("DataResources: ").append(getDataResources()).append(",");
if (getExcludeManagementEventSources() != null)
sb.append("ExcludeManagementEventSources: ").append(getExcludeManagementEventSources());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EventSelector == false)
return false;
EventSelector other = (EventSelector) obj;
if (other.getReadWriteType() == null ^ this.getReadWriteType() == null)
return false;
if (other.getReadWriteType() != null && other.getReadWriteType().equals(this.getReadWriteType()) == false)
return false;
if (other.getIncludeManagementEvents() == null ^ this.getIncludeManagementEvents() == null)
return false;
if (other.getIncludeManagementEvents() != null && other.getIncludeManagementEvents().equals(this.getIncludeManagementEvents()) == false)
return false;
if (other.getDataResources() == null ^ this.getDataResources() == null)
return false;
if (other.getDataResources() != null && other.getDataResources().equals(this.getDataResources()) == false)
return false;
if (other.getExcludeManagementEventSources() == null ^ this.getExcludeManagementEventSources() == null)
return false;
if (other.getExcludeManagementEventSources() != null
&& other.getExcludeManagementEventSources().equals(this.getExcludeManagementEventSources()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReadWriteType() == null) ? 0 : getReadWriteType().hashCode());
hashCode = prime * hashCode + ((getIncludeManagementEvents() == null) ? 0 : getIncludeManagementEvents().hashCode());
hashCode = prime * hashCode + ((getDataResources() == null) ? 0 : getDataResources().hashCode());
hashCode = prime * hashCode + ((getExcludeManagementEventSources() == null) ? 0 : getExcludeManagementEventSources().hashCode());
return hashCode;
}
@Override
public EventSelector clone() {
try {
return (EventSelector) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.cloudtrail.model.transform.EventSelectorMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}