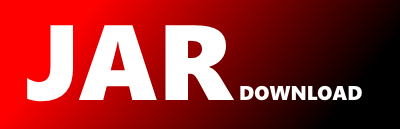
com.amazonaws.services.cloudtrail.model.GetTrailStatusResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Returns the objects or data listed below if successful. Otherwise, returns an error.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetTrailStatusResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* Whether the CloudTrail is currently logging AWS API calls.
*
*/
private Boolean isLogging;
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the designated
* bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*/
private String latestDeliveryError;
/**
*
* Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For more
* information about Amazon SNS errors, see the Amazon SNS Developer Guide.
*
*/
private String latestNotificationError;
/**
*
* Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
*
*/
private java.util.Date latestDeliveryTime;
/**
*
* Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new log file
* to an account's Amazon S3 bucket.
*
*/
private java.util.Date latestNotificationTime;
/**
*
* Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
*
*/
private java.util.Date startLoggingTime;
/**
*
* Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
*
*/
private java.util.Date stopLoggingTime;
/**
*
* Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to CloudWatch
* Logs.
*
*/
private String latestCloudWatchLogsDeliveryError;
/**
*
* Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
*
*/
private java.util.Date latestCloudWatchLogsDeliveryTime;
/**
*
* Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3 bucket.
*
*/
private java.util.Date latestDigestDeliveryTime;
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*/
private String latestDigestDeliveryError;
/**
*
* This field is no longer in use.
*
*/
private String latestDeliveryAttemptTime;
/**
*
* This field is no longer in use.
*
*/
private String latestNotificationAttemptTime;
/**
*
* This field is no longer in use.
*
*/
private String latestNotificationAttemptSucceeded;
/**
*
* This field is no longer in use.
*
*/
private String latestDeliveryAttemptSucceeded;
/**
*
* This field is no longer in use.
*
*/
private String timeLoggingStarted;
/**
*
* This field is no longer in use.
*
*/
private String timeLoggingStopped;
/**
*
* Whether the CloudTrail is currently logging AWS API calls.
*
*
* @param isLogging
* Whether the CloudTrail is currently logging AWS API calls.
*/
public void setIsLogging(Boolean isLogging) {
this.isLogging = isLogging;
}
/**
*
* Whether the CloudTrail is currently logging AWS API calls.
*
*
* @return Whether the CloudTrail is currently logging AWS API calls.
*/
public Boolean getIsLogging() {
return this.isLogging;
}
/**
*
* Whether the CloudTrail is currently logging AWS API calls.
*
*
* @param isLogging
* Whether the CloudTrail is currently logging AWS API calls.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withIsLogging(Boolean isLogging) {
setIsLogging(isLogging);
return this;
}
/**
*
* Whether the CloudTrail is currently logging AWS API calls.
*
*
* @return Whether the CloudTrail is currently logging AWS API calls.
*/
public Boolean isLogging() {
return this.isLogging;
}
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the designated
* bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*
* @param latestDeliveryError
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the
* designated bucket. For more information see the topic Error Responses in the
* Amazon S3 API Reference.
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for
* timeouts. To resolve the issue, create a new bucket and call UpdateTrail
to specify the new
* bucket, or fix the existing objects so that CloudTrail can again write to the bucket.
*
*/
public void setLatestDeliveryError(String latestDeliveryError) {
this.latestDeliveryError = latestDeliveryError;
}
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the designated
* bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*
* @return Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the
* designated bucket. For more information see the topic Error Responses in the
* Amazon S3 API Reference.
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for
* timeouts. To resolve the issue, create a new bucket and call UpdateTrail
to specify the new
* bucket, or fix the existing objects so that CloudTrail can again write to the bucket.
*
*/
public String getLatestDeliveryError() {
return this.latestDeliveryError;
}
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the designated
* bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*
* @param latestDeliveryError
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver log files to the
* designated bucket. For more information see the topic Error Responses in the
* Amazon S3 API Reference.
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for
* timeouts. To resolve the issue, create a new bucket and call UpdateTrail
to specify the new
* bucket, or fix the existing objects so that CloudTrail can again write to the bucket.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestDeliveryError(String latestDeliveryError) {
setLatestDeliveryError(latestDeliveryError);
return this;
}
/**
*
* Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For more
* information about Amazon SNS errors, see the Amazon SNS Developer Guide.
*
*
* @param latestNotificationError
* Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For more
* information about Amazon SNS errors, see the Amazon SNS Developer Guide.
*/
public void setLatestNotificationError(String latestNotificationError) {
this.latestNotificationError = latestNotificationError;
}
/**
*
* Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For more
* information about Amazon SNS errors, see the Amazon SNS Developer Guide.
*
*
* @return Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For
* more information about Amazon SNS errors, see the Amazon SNS Developer Guide.
*/
public String getLatestNotificationError() {
return this.latestNotificationError;
}
/**
*
* Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For more
* information about Amazon SNS errors, see the Amazon SNS Developer Guide.
*
*
* @param latestNotificationError
* Displays any Amazon SNS error that CloudTrail encountered when attempting to send a notification. For more
* information about Amazon SNS errors, see the Amazon SNS Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestNotificationError(String latestNotificationError) {
setLatestNotificationError(latestNotificationError);
return this;
}
/**
*
* Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
*
*
* @param latestDeliveryTime
* Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
*/
public void setLatestDeliveryTime(java.util.Date latestDeliveryTime) {
this.latestDeliveryTime = latestDeliveryTime;
}
/**
*
* Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
*
*
* @return Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
*/
public java.util.Date getLatestDeliveryTime() {
return this.latestDeliveryTime;
}
/**
*
* Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
*
*
* @param latestDeliveryTime
* Specifies the date and time that CloudTrail last delivered log files to an account's Amazon S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestDeliveryTime(java.util.Date latestDeliveryTime) {
setLatestDeliveryTime(latestDeliveryTime);
return this;
}
/**
*
* Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new log file
* to an account's Amazon S3 bucket.
*
*
* @param latestNotificationTime
* Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new
* log file to an account's Amazon S3 bucket.
*/
public void setLatestNotificationTime(java.util.Date latestNotificationTime) {
this.latestNotificationTime = latestNotificationTime;
}
/**
*
* Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new log file
* to an account's Amazon S3 bucket.
*
*
* @return Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new
* log file to an account's Amazon S3 bucket.
*/
public java.util.Date getLatestNotificationTime() {
return this.latestNotificationTime;
}
/**
*
* Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new log file
* to an account's Amazon S3 bucket.
*
*
* @param latestNotificationTime
* Specifies the date and time of the most recent Amazon SNS notification that CloudTrail has written a new
* log file to an account's Amazon S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestNotificationTime(java.util.Date latestNotificationTime) {
setLatestNotificationTime(latestNotificationTime);
return this;
}
/**
*
* Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
*
*
* @param startLoggingTime
* Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
*/
public void setStartLoggingTime(java.util.Date startLoggingTime) {
this.startLoggingTime = startLoggingTime;
}
/**
*
* Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
*
*
* @return Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
*/
public java.util.Date getStartLoggingTime() {
return this.startLoggingTime;
}
/**
*
* Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
*
*
* @param startLoggingTime
* Specifies the most recent date and time when CloudTrail started recording API calls for an AWS account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withStartLoggingTime(java.util.Date startLoggingTime) {
setStartLoggingTime(startLoggingTime);
return this;
}
/**
*
* Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
*
*
* @param stopLoggingTime
* Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
*/
public void setStopLoggingTime(java.util.Date stopLoggingTime) {
this.stopLoggingTime = stopLoggingTime;
}
/**
*
* Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
*
*
* @return Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
*/
public java.util.Date getStopLoggingTime() {
return this.stopLoggingTime;
}
/**
*
* Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
*
*
* @param stopLoggingTime
* Specifies the most recent date and time when CloudTrail stopped recording API calls for an AWS account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withStopLoggingTime(java.util.Date stopLoggingTime) {
setStopLoggingTime(stopLoggingTime);
return this;
}
/**
*
* Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to CloudWatch
* Logs.
*
*
* @param latestCloudWatchLogsDeliveryError
* Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to
* CloudWatch Logs.
*/
public void setLatestCloudWatchLogsDeliveryError(String latestCloudWatchLogsDeliveryError) {
this.latestCloudWatchLogsDeliveryError = latestCloudWatchLogsDeliveryError;
}
/**
*
* Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to CloudWatch
* Logs.
*
*
* @return Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to
* CloudWatch Logs.
*/
public String getLatestCloudWatchLogsDeliveryError() {
return this.latestCloudWatchLogsDeliveryError;
}
/**
*
* Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to CloudWatch
* Logs.
*
*
* @param latestCloudWatchLogsDeliveryError
* Displays any CloudWatch Logs error that CloudTrail encountered when attempting to deliver logs to
* CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestCloudWatchLogsDeliveryError(String latestCloudWatchLogsDeliveryError) {
setLatestCloudWatchLogsDeliveryError(latestCloudWatchLogsDeliveryError);
return this;
}
/**
*
* Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
*
*
* @param latestCloudWatchLogsDeliveryTime
* Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
*/
public void setLatestCloudWatchLogsDeliveryTime(java.util.Date latestCloudWatchLogsDeliveryTime) {
this.latestCloudWatchLogsDeliveryTime = latestCloudWatchLogsDeliveryTime;
}
/**
*
* Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
*
*
* @return Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
*/
public java.util.Date getLatestCloudWatchLogsDeliveryTime() {
return this.latestCloudWatchLogsDeliveryTime;
}
/**
*
* Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
*
*
* @param latestCloudWatchLogsDeliveryTime
* Displays the most recent date and time when CloudTrail delivered logs to CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestCloudWatchLogsDeliveryTime(java.util.Date latestCloudWatchLogsDeliveryTime) {
setLatestCloudWatchLogsDeliveryTime(latestCloudWatchLogsDeliveryTime);
return this;
}
/**
*
* Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3 bucket.
*
*
* @param latestDigestDeliveryTime
* Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3 bucket.
*/
public void setLatestDigestDeliveryTime(java.util.Date latestDigestDeliveryTime) {
this.latestDigestDeliveryTime = latestDigestDeliveryTime;
}
/**
*
* Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3 bucket.
*
*
* @return Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3
* bucket.
*/
public java.util.Date getLatestDigestDeliveryTime() {
return this.latestDigestDeliveryTime;
}
/**
*
* Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3 bucket.
*
*
* @param latestDigestDeliveryTime
* Specifies the date and time that CloudTrail last delivered a digest file to an account's Amazon S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestDigestDeliveryTime(java.util.Date latestDigestDeliveryTime) {
setLatestDigestDeliveryTime(latestDigestDeliveryTime);
return this;
}
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*
* @param latestDigestDeliveryError
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the
* Amazon S3 API Reference.
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for
* timeouts. To resolve the issue, create a new bucket and call UpdateTrail
to specify the new
* bucket, or fix the existing objects so that CloudTrail can again write to the bucket.
*
*/
public void setLatestDigestDeliveryError(String latestDigestDeliveryError) {
this.latestDigestDeliveryError = latestDigestDeliveryError;
}
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*
* @return Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the
* Amazon S3 API Reference.
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for
* timeouts. To resolve the issue, create a new bucket and call UpdateTrail
to specify the new
* bucket, or fix the existing objects so that CloudTrail can again write to the bucket.
*
*/
public String getLatestDigestDeliveryError() {
return this.latestDigestDeliveryError;
}
/**
*
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the Amazon S3
* API Reference.
*
*
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for timeouts. To
* resolve the issue, create a new bucket and call UpdateTrail
to specify the new bucket, or fix the
* existing objects so that CloudTrail can again write to the bucket.
*
*
*
* @param latestDigestDeliveryError
* Displays any Amazon S3 error that CloudTrail encountered when attempting to deliver a digest file to the
* designated bucket. For more information see the topic Error Responses in the
* Amazon S3 API Reference.
*
* This error occurs only when there is a problem with the destination S3 bucket and will not occur for
* timeouts. To resolve the issue, create a new bucket and call UpdateTrail
to specify the new
* bucket, or fix the existing objects so that CloudTrail can again write to the bucket.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestDigestDeliveryError(String latestDigestDeliveryError) {
setLatestDigestDeliveryError(latestDigestDeliveryError);
return this;
}
/**
*
* This field is no longer in use.
*
*
* @param latestDeliveryAttemptTime
* This field is no longer in use.
*/
public void setLatestDeliveryAttemptTime(String latestDeliveryAttemptTime) {
this.latestDeliveryAttemptTime = latestDeliveryAttemptTime;
}
/**
*
* This field is no longer in use.
*
*
* @return This field is no longer in use.
*/
public String getLatestDeliveryAttemptTime() {
return this.latestDeliveryAttemptTime;
}
/**
*
* This field is no longer in use.
*
*
* @param latestDeliveryAttemptTime
* This field is no longer in use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestDeliveryAttemptTime(String latestDeliveryAttemptTime) {
setLatestDeliveryAttemptTime(latestDeliveryAttemptTime);
return this;
}
/**
*
* This field is no longer in use.
*
*
* @param latestNotificationAttemptTime
* This field is no longer in use.
*/
public void setLatestNotificationAttemptTime(String latestNotificationAttemptTime) {
this.latestNotificationAttemptTime = latestNotificationAttemptTime;
}
/**
*
* This field is no longer in use.
*
*
* @return This field is no longer in use.
*/
public String getLatestNotificationAttemptTime() {
return this.latestNotificationAttemptTime;
}
/**
*
* This field is no longer in use.
*
*
* @param latestNotificationAttemptTime
* This field is no longer in use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestNotificationAttemptTime(String latestNotificationAttemptTime) {
setLatestNotificationAttemptTime(latestNotificationAttemptTime);
return this;
}
/**
*
* This field is no longer in use.
*
*
* @param latestNotificationAttemptSucceeded
* This field is no longer in use.
*/
public void setLatestNotificationAttemptSucceeded(String latestNotificationAttemptSucceeded) {
this.latestNotificationAttemptSucceeded = latestNotificationAttemptSucceeded;
}
/**
*
* This field is no longer in use.
*
*
* @return This field is no longer in use.
*/
public String getLatestNotificationAttemptSucceeded() {
return this.latestNotificationAttemptSucceeded;
}
/**
*
* This field is no longer in use.
*
*
* @param latestNotificationAttemptSucceeded
* This field is no longer in use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestNotificationAttemptSucceeded(String latestNotificationAttemptSucceeded) {
setLatestNotificationAttemptSucceeded(latestNotificationAttemptSucceeded);
return this;
}
/**
*
* This field is no longer in use.
*
*
* @param latestDeliveryAttemptSucceeded
* This field is no longer in use.
*/
public void setLatestDeliveryAttemptSucceeded(String latestDeliveryAttemptSucceeded) {
this.latestDeliveryAttemptSucceeded = latestDeliveryAttemptSucceeded;
}
/**
*
* This field is no longer in use.
*
*
* @return This field is no longer in use.
*/
public String getLatestDeliveryAttemptSucceeded() {
return this.latestDeliveryAttemptSucceeded;
}
/**
*
* This field is no longer in use.
*
*
* @param latestDeliveryAttemptSucceeded
* This field is no longer in use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withLatestDeliveryAttemptSucceeded(String latestDeliveryAttemptSucceeded) {
setLatestDeliveryAttemptSucceeded(latestDeliveryAttemptSucceeded);
return this;
}
/**
*
* This field is no longer in use.
*
*
* @param timeLoggingStarted
* This field is no longer in use.
*/
public void setTimeLoggingStarted(String timeLoggingStarted) {
this.timeLoggingStarted = timeLoggingStarted;
}
/**
*
* This field is no longer in use.
*
*
* @return This field is no longer in use.
*/
public String getTimeLoggingStarted() {
return this.timeLoggingStarted;
}
/**
*
* This field is no longer in use.
*
*
* @param timeLoggingStarted
* This field is no longer in use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withTimeLoggingStarted(String timeLoggingStarted) {
setTimeLoggingStarted(timeLoggingStarted);
return this;
}
/**
*
* This field is no longer in use.
*
*
* @param timeLoggingStopped
* This field is no longer in use.
*/
public void setTimeLoggingStopped(String timeLoggingStopped) {
this.timeLoggingStopped = timeLoggingStopped;
}
/**
*
* This field is no longer in use.
*
*
* @return This field is no longer in use.
*/
public String getTimeLoggingStopped() {
return this.timeLoggingStopped;
}
/**
*
* This field is no longer in use.
*
*
* @param timeLoggingStopped
* This field is no longer in use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetTrailStatusResult withTimeLoggingStopped(String timeLoggingStopped) {
setTimeLoggingStopped(timeLoggingStopped);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIsLogging() != null)
sb.append("IsLogging: ").append(getIsLogging()).append(",");
if (getLatestDeliveryError() != null)
sb.append("LatestDeliveryError: ").append(getLatestDeliveryError()).append(",");
if (getLatestNotificationError() != null)
sb.append("LatestNotificationError: ").append(getLatestNotificationError()).append(",");
if (getLatestDeliveryTime() != null)
sb.append("LatestDeliveryTime: ").append(getLatestDeliveryTime()).append(",");
if (getLatestNotificationTime() != null)
sb.append("LatestNotificationTime: ").append(getLatestNotificationTime()).append(",");
if (getStartLoggingTime() != null)
sb.append("StartLoggingTime: ").append(getStartLoggingTime()).append(",");
if (getStopLoggingTime() != null)
sb.append("StopLoggingTime: ").append(getStopLoggingTime()).append(",");
if (getLatestCloudWatchLogsDeliveryError() != null)
sb.append("LatestCloudWatchLogsDeliveryError: ").append(getLatestCloudWatchLogsDeliveryError()).append(",");
if (getLatestCloudWatchLogsDeliveryTime() != null)
sb.append("LatestCloudWatchLogsDeliveryTime: ").append(getLatestCloudWatchLogsDeliveryTime()).append(",");
if (getLatestDigestDeliveryTime() != null)
sb.append("LatestDigestDeliveryTime: ").append(getLatestDigestDeliveryTime()).append(",");
if (getLatestDigestDeliveryError() != null)
sb.append("LatestDigestDeliveryError: ").append(getLatestDigestDeliveryError()).append(",");
if (getLatestDeliveryAttemptTime() != null)
sb.append("LatestDeliveryAttemptTime: ").append(getLatestDeliveryAttemptTime()).append(",");
if (getLatestNotificationAttemptTime() != null)
sb.append("LatestNotificationAttemptTime: ").append(getLatestNotificationAttemptTime()).append(",");
if (getLatestNotificationAttemptSucceeded() != null)
sb.append("LatestNotificationAttemptSucceeded: ").append(getLatestNotificationAttemptSucceeded()).append(",");
if (getLatestDeliveryAttemptSucceeded() != null)
sb.append("LatestDeliveryAttemptSucceeded: ").append(getLatestDeliveryAttemptSucceeded()).append(",");
if (getTimeLoggingStarted() != null)
sb.append("TimeLoggingStarted: ").append(getTimeLoggingStarted()).append(",");
if (getTimeLoggingStopped() != null)
sb.append("TimeLoggingStopped: ").append(getTimeLoggingStopped());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetTrailStatusResult == false)
return false;
GetTrailStatusResult other = (GetTrailStatusResult) obj;
if (other.getIsLogging() == null ^ this.getIsLogging() == null)
return false;
if (other.getIsLogging() != null && other.getIsLogging().equals(this.getIsLogging()) == false)
return false;
if (other.getLatestDeliveryError() == null ^ this.getLatestDeliveryError() == null)
return false;
if (other.getLatestDeliveryError() != null && other.getLatestDeliveryError().equals(this.getLatestDeliveryError()) == false)
return false;
if (other.getLatestNotificationError() == null ^ this.getLatestNotificationError() == null)
return false;
if (other.getLatestNotificationError() != null && other.getLatestNotificationError().equals(this.getLatestNotificationError()) == false)
return false;
if (other.getLatestDeliveryTime() == null ^ this.getLatestDeliveryTime() == null)
return false;
if (other.getLatestDeliveryTime() != null && other.getLatestDeliveryTime().equals(this.getLatestDeliveryTime()) == false)
return false;
if (other.getLatestNotificationTime() == null ^ this.getLatestNotificationTime() == null)
return false;
if (other.getLatestNotificationTime() != null && other.getLatestNotificationTime().equals(this.getLatestNotificationTime()) == false)
return false;
if (other.getStartLoggingTime() == null ^ this.getStartLoggingTime() == null)
return false;
if (other.getStartLoggingTime() != null && other.getStartLoggingTime().equals(this.getStartLoggingTime()) == false)
return false;
if (other.getStopLoggingTime() == null ^ this.getStopLoggingTime() == null)
return false;
if (other.getStopLoggingTime() != null && other.getStopLoggingTime().equals(this.getStopLoggingTime()) == false)
return false;
if (other.getLatestCloudWatchLogsDeliveryError() == null ^ this.getLatestCloudWatchLogsDeliveryError() == null)
return false;
if (other.getLatestCloudWatchLogsDeliveryError() != null
&& other.getLatestCloudWatchLogsDeliveryError().equals(this.getLatestCloudWatchLogsDeliveryError()) == false)
return false;
if (other.getLatestCloudWatchLogsDeliveryTime() == null ^ this.getLatestCloudWatchLogsDeliveryTime() == null)
return false;
if (other.getLatestCloudWatchLogsDeliveryTime() != null
&& other.getLatestCloudWatchLogsDeliveryTime().equals(this.getLatestCloudWatchLogsDeliveryTime()) == false)
return false;
if (other.getLatestDigestDeliveryTime() == null ^ this.getLatestDigestDeliveryTime() == null)
return false;
if (other.getLatestDigestDeliveryTime() != null && other.getLatestDigestDeliveryTime().equals(this.getLatestDigestDeliveryTime()) == false)
return false;
if (other.getLatestDigestDeliveryError() == null ^ this.getLatestDigestDeliveryError() == null)
return false;
if (other.getLatestDigestDeliveryError() != null && other.getLatestDigestDeliveryError().equals(this.getLatestDigestDeliveryError()) == false)
return false;
if (other.getLatestDeliveryAttemptTime() == null ^ this.getLatestDeliveryAttemptTime() == null)
return false;
if (other.getLatestDeliveryAttemptTime() != null && other.getLatestDeliveryAttemptTime().equals(this.getLatestDeliveryAttemptTime()) == false)
return false;
if (other.getLatestNotificationAttemptTime() == null ^ this.getLatestNotificationAttemptTime() == null)
return false;
if (other.getLatestNotificationAttemptTime() != null
&& other.getLatestNotificationAttemptTime().equals(this.getLatestNotificationAttemptTime()) == false)
return false;
if (other.getLatestNotificationAttemptSucceeded() == null ^ this.getLatestNotificationAttemptSucceeded() == null)
return false;
if (other.getLatestNotificationAttemptSucceeded() != null
&& other.getLatestNotificationAttemptSucceeded().equals(this.getLatestNotificationAttemptSucceeded()) == false)
return false;
if (other.getLatestDeliveryAttemptSucceeded() == null ^ this.getLatestDeliveryAttemptSucceeded() == null)
return false;
if (other.getLatestDeliveryAttemptSucceeded() != null
&& other.getLatestDeliveryAttemptSucceeded().equals(this.getLatestDeliveryAttemptSucceeded()) == false)
return false;
if (other.getTimeLoggingStarted() == null ^ this.getTimeLoggingStarted() == null)
return false;
if (other.getTimeLoggingStarted() != null && other.getTimeLoggingStarted().equals(this.getTimeLoggingStarted()) == false)
return false;
if (other.getTimeLoggingStopped() == null ^ this.getTimeLoggingStopped() == null)
return false;
if (other.getTimeLoggingStopped() != null && other.getTimeLoggingStopped().equals(this.getTimeLoggingStopped()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIsLogging() == null) ? 0 : getIsLogging().hashCode());
hashCode = prime * hashCode + ((getLatestDeliveryError() == null) ? 0 : getLatestDeliveryError().hashCode());
hashCode = prime * hashCode + ((getLatestNotificationError() == null) ? 0 : getLatestNotificationError().hashCode());
hashCode = prime * hashCode + ((getLatestDeliveryTime() == null) ? 0 : getLatestDeliveryTime().hashCode());
hashCode = prime * hashCode + ((getLatestNotificationTime() == null) ? 0 : getLatestNotificationTime().hashCode());
hashCode = prime * hashCode + ((getStartLoggingTime() == null) ? 0 : getStartLoggingTime().hashCode());
hashCode = prime * hashCode + ((getStopLoggingTime() == null) ? 0 : getStopLoggingTime().hashCode());
hashCode = prime * hashCode + ((getLatestCloudWatchLogsDeliveryError() == null) ? 0 : getLatestCloudWatchLogsDeliveryError().hashCode());
hashCode = prime * hashCode + ((getLatestCloudWatchLogsDeliveryTime() == null) ? 0 : getLatestCloudWatchLogsDeliveryTime().hashCode());
hashCode = prime * hashCode + ((getLatestDigestDeliveryTime() == null) ? 0 : getLatestDigestDeliveryTime().hashCode());
hashCode = prime * hashCode + ((getLatestDigestDeliveryError() == null) ? 0 : getLatestDigestDeliveryError().hashCode());
hashCode = prime * hashCode + ((getLatestDeliveryAttemptTime() == null) ? 0 : getLatestDeliveryAttemptTime().hashCode());
hashCode = prime * hashCode + ((getLatestNotificationAttemptTime() == null) ? 0 : getLatestNotificationAttemptTime().hashCode());
hashCode = prime * hashCode + ((getLatestNotificationAttemptSucceeded() == null) ? 0 : getLatestNotificationAttemptSucceeded().hashCode());
hashCode = prime * hashCode + ((getLatestDeliveryAttemptSucceeded() == null) ? 0 : getLatestDeliveryAttemptSucceeded().hashCode());
hashCode = prime * hashCode + ((getTimeLoggingStarted() == null) ? 0 : getTimeLoggingStarted().hashCode());
hashCode = prime * hashCode + ((getTimeLoggingStopped() == null) ? 0 : getTimeLoggingStopped().hashCode());
return hashCode;
}
@Override
public GetTrailStatusResult clone() {
try {
return (GetTrailStatusResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}