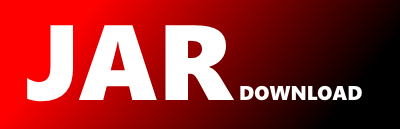
com.amazonaws.services.cloudtrail.AWSCloudTrailClient Maven / Gradle / Ivy
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.services.cloudtrail.AWSCloudTrailClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.cloudtrail.model.*;
import com.amazonaws.services.cloudtrail.model.transform.*;
/**
* Client for accessing CloudTrail. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* AWS CloudTrail
*
* This is the CloudTrail API Reference. It provides descriptions of actions, data types, common parameters, and common
* errors for CloudTrail.
*
*
* CloudTrail is a web service that records AWS API calls for your AWS account and delivers log files to an Amazon S3
* bucket. The recorded information includes the identity of the user, the start time of the AWS API call, the source IP
* address, the request parameters, and the response elements returned by the service.
*
*
*
* As an alternative to the API, you can use one of the AWS SDKs, which consist of libraries and sample code for various
* programming languages and platforms (Java, Ruby, .NET, iOS, Android, etc.). The SDKs provide a convenient way to
* create programmatic access to AWSCloudTrail. For example, the SDKs take care of cryptographically signing requests,
* managing errors, and retrying requests automatically. For information about the AWS SDKs, including how to download
* and install them, see the Tools for Amazon Web Services page.
*
*
*
* See the AWS CloudTrail
* User Guide for information about the data that is included with each AWS API call listed in the log files.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSCloudTrailClient extends AmazonWebServiceClient implements AWSCloudTrail {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSCloudTrail.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "cloudtrail";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTokenException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidTokenException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CloudTrailARNInvalidException").withModeledClass(
com.amazonaws.services.cloudtrail.model.CloudTrailARNInvalidException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidCloudWatchLogsRoleArnException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidCloudWatchLogsRoleArnException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidKmsKeyIdException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidKmsKeyIdException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withModeledClass(
com.amazonaws.services.cloudtrail.model.ResourceNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTimeRangeException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidTimeRangeException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidEventSelectorsException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidEventSelectorsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedOperationException").withModeledClass(
com.amazonaws.services.cloudtrail.model.UnsupportedOperationException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TrailNotProvidedException").withModeledClass(
com.amazonaws.services.cloudtrail.model.TrailNotProvidedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidCloudWatchLogsLogGroupArnException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidCloudWatchLogsLogGroupArnException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("KmsException").withModeledClass(
com.amazonaws.services.cloudtrail.model.KmsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("S3BucketDoesNotExistException").withModeledClass(
com.amazonaws.services.cloudtrail.model.S3BucketDoesNotExistException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterCombinationException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidParameterCombinationException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidMaxResultsException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidMaxResultsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TagsLimitExceededException").withModeledClass(
com.amazonaws.services.cloudtrail.model.TagsLimitExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidS3BucketNameException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidS3BucketNameException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CloudWatchLogsDeliveryUnavailableException").withModeledClass(
com.amazonaws.services.cloudtrail.model.CloudWatchLogsDeliveryUnavailableException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTrailNameException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidTrailNameException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidSnsTopicNameException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidSnsTopicNameException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientEncryptionPolicyException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InsufficientEncryptionPolicyException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTagParameterException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidTagParameterException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("KmsKeyNotFoundException").withModeledClass(
com.amazonaws.services.cloudtrail.model.KmsKeyNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OperationNotPermittedException").withModeledClass(
com.amazonaws.services.cloudtrail.model.OperationNotPermittedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TrailNotFoundException").withModeledClass(
com.amazonaws.services.cloudtrail.model.TrailNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidLookupAttributesException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidLookupAttributesException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MaximumNumberOfTrailsExceededException").withModeledClass(
com.amazonaws.services.cloudtrail.model.MaximumNumberOfTrailsExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidS3PrefixException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidS3PrefixException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientSnsTopicPolicyException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InsufficientSnsTopicPolicyException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidNextTokenException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidNextTokenException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientS3BucketPolicyException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InsufficientS3BucketPolicyException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidHomeRegionException").withModeledClass(
com.amazonaws.services.cloudtrail.model.InvalidHomeRegionException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceTypeNotSupportedException").withModeledClass(
com.amazonaws.services.cloudtrail.model.ResourceTypeNotSupportedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TrailAlreadyExistsException").withModeledClass(
com.amazonaws.services.cloudtrail.model.TrailAlreadyExistsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("KmsKeyDisabledException").withModeledClass(
com.amazonaws.services.cloudtrail.model.KmsKeyDisabledException.class))
.withBaseServiceExceptionClass(com.amazonaws.services.cloudtrail.model.AWSCloudTrailException.class));
/**
* Constructs a new client to invoke service methods on CloudTrail. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSCloudTrailClientBuilder#defaultClient()}
*/
@Deprecated
public AWSCloudTrailClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudTrail. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSCloudTrailClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSCloudTrailClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSCloudTrailClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
public static AWSCloudTrailClientBuilder builder() {
return AWSCloudTrailClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSCloudTrailClient(AwsSyncClientParams clientParams) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("cloudtrail.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/cloudtrail/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/cloudtrail/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Adds one or more tags to a trail, up to a limit of 50. Tags must be unique per trail. Overwrites an existing
* tag's value when a new value is specified for an existing tag key. If you specify a key without a value, the tag
* will be created with the specified key and a value of null. You can tag a trail that applies to all regions only
* from the region in which the trail was created (that is, from its home region).
*
*
* @param addTagsRequest
* Specifies the tags to add to a trail.
* @return Result of the AddTags operation returned by the service.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an invalid trail ARN. The format of a trail ARN
* is:
*
* arn:aws:cloudtrail:us-east-1:123456789012:trail/MyTrail
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws TagsLimitExceededException
* The number of tags per trail has exceeded the permitted amount. Currently, the limit is 50.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidTagParameterException
* This exception is thrown when the key or value specified for the tag does not match the regular
* expression ^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$
.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.AddTags
* @see AWS API
* Documentation
*/
@Override
public AddTagsResult addTags(AddTagsRequest request) {
request = beforeClientExecution(request);
return executeAddTags(request);
}
@SdkInternalApi
final AddTagsResult executeAddTags(AddTagsRequest addTagsRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(addTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new AddTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a trail that specifies the settings for delivery of log data to an Amazon S3 bucket. A maximum of five
* trails can exist in a region, irrespective of the region in which they were created.
*
*
* @param createTrailRequest
* Specifies the settings for each trail.
* @return Result of the CreateTrail operation returned by the service.
* @throws MaximumNumberOfTrailsExceededException
* This exception is thrown when the maximum number of trails is reached.
* @throws TrailAlreadyExistsException
* This exception is thrown when the specified trail already exists.
* @throws S3BucketDoesNotExistException
* This exception is thrown when the specified S3 bucket does not exist.
* @throws InsufficientS3BucketPolicyException
* This exception is thrown when the policy on the S3 bucket is not sufficient.
* @throws InsufficientSnsTopicPolicyException
* This exception is thrown when the policy on the SNS topic is not sufficient.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key is not sufficient.
* @throws InvalidS3BucketNameException
* This exception is thrown when the provided S3 bucket name is not valid.
* @throws InvalidS3PrefixException
* This exception is thrown when the provided S3 prefix is not valid.
* @throws InvalidSnsTopicNameException
* This exception is thrown when the provided SNS topic name is not valid.
* @throws InvalidKmsKeyIdException
* This exception is thrown when the KMS key ARN is invalid.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws TrailNotProvidedException
* This exception is deprecated.
* @throws InvalidParameterCombinationException
* This exception is thrown when the combination of parameters provided is not valid.
* @throws KmsKeyNotFoundException
* This exception is thrown when the KMS key does not exist, or when the S3 bucket and the KMS key are not
* in the same region.
* @throws KmsKeyDisabledException
* This exception is deprecated.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail can’t be
* updated.
* @throws InvalidCloudWatchLogsLogGroupArnException
* This exception is thrown when the provided CloudWatch log group is not valid.
* @throws InvalidCloudWatchLogsRoleArnException
* This exception is thrown when the provided role is not valid.
* @throws CloudWatchLogsDeliveryUnavailableException
* Cannot set a CloudWatch Logs delivery for this region.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.CreateTrail
* @see AWS API
* Documentation
*/
@Override
public CreateTrailResult createTrail(CreateTrailRequest request) {
request = beforeClientExecution(request);
return executeCreateTrail(request);
}
@SdkInternalApi
final CreateTrailResult executeCreateTrail(CreateTrailRequest createTrailRequest) {
ExecutionContext executionContext = createExecutionContext(createTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a trail. This operation must be called from the region in which the trail was created.
* DeleteTrail
cannot be called on the shadow trails (replicated trails in other regions) of a trail
* that is enabled in all regions.
*
*
* @param deleteTrailRequest
* The request that specifies the name of a trail to delete.
* @return Result of the DeleteTrail operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a region other than the region in
* which the trail was created.
* @sample AWSCloudTrail.DeleteTrail
* @see AWS API
* Documentation
*/
@Override
public DeleteTrailResult deleteTrail(DeleteTrailRequest request) {
request = beforeClientExecution(request);
return executeDeleteTrail(request);
}
@SdkInternalApi
final DeleteTrailResult executeDeleteTrail(DeleteTrailRequest deleteTrailRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves settings for the trail associated with the current region for your account.
*
*
* @param describeTrailsRequest
* Returns information about the trail.
* @return Result of the DescribeTrails operation returned by the service.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.DescribeTrails
* @see AWS API
* Documentation
*/
@Override
public DescribeTrailsResult describeTrails(DescribeTrailsRequest request) {
request = beforeClientExecution(request);
return executeDescribeTrails(request);
}
@SdkInternalApi
final DescribeTrailsResult executeDescribeTrails(DescribeTrailsRequest describeTrailsRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrailsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeTrailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeTrailsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeTrailsResult describeTrails() {
return describeTrails(new DescribeTrailsRequest());
}
/**
*
* Describes the settings for the event selectors that you configured for your trail. The information returned for
* your event selectors includes the following:
*
*
* -
*
* The S3 objects that you are logging for data events.
*
*
* -
*
* If your event selector includes management events.
*
*
* -
*
* If your event selector includes read-only events, write-only events, or all.
*
*
*
*
* For more information, see Logging Data and Management Events for Trails in the AWS CloudTrail User Guide.
*
*
* @param getEventSelectorsRequest
* @return Result of the GetEventSelectors operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.GetEventSelectors
* @see AWS
* API Documentation
*/
@Override
public GetEventSelectorsResult getEventSelectors(GetEventSelectorsRequest request) {
request = beforeClientExecution(request);
return executeGetEventSelectors(request);
}
@SdkInternalApi
final GetEventSelectorsResult executeGetEventSelectors(GetEventSelectorsRequest getEventSelectorsRequest) {
ExecutionContext executionContext = createExecutionContext(getEventSelectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEventSelectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEventSelectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEventSelectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a JSON-formatted list of information about the specified trail. Fields include information on delivery
* errors, Amazon SNS and Amazon S3 errors, and start and stop logging times for each trail. This operation returns
* trail status from a single region. To return trail status from all regions, you must call the operation on each
* region.
*
*
* @param getTrailStatusRequest
* The name of a trail about which you want the current status.
* @return Result of the GetTrailStatus operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @sample AWSCloudTrail.GetTrailStatus
* @see AWS API
* Documentation
*/
@Override
public GetTrailStatusResult getTrailStatus(GetTrailStatusRequest request) {
request = beforeClientExecution(request);
return executeGetTrailStatus(request);
}
@SdkInternalApi
final GetTrailStatusResult executeGetTrailStatus(GetTrailStatusRequest getTrailStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getTrailStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTrailStatusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getTrailStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetTrailStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns all public keys whose private keys were used to sign the digest files within the specified time range.
* The public key is needed to validate digest files that were signed with its corresponding private key.
*
*
*
* CloudTrail uses different private/public key pairs per region. Each digest file is signed with a private key
* unique to its region. Therefore, when you validate a digest file from a particular region, you must look in the
* same region for its corresponding public key.
*
*
*
* @param listPublicKeysRequest
* Requests the public keys for a specified time range.
* @return Result of the ListPublicKeys operation returned by the service.
* @throws InvalidTimeRangeException
* Occurs if the timestamp values are invalid. Either the start time occurs after the end time or the time
* range is outside the range of possible values.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws InvalidTokenException
* Reserved for future use.
* @sample AWSCloudTrail.ListPublicKeys
* @see AWS API
* Documentation
*/
@Override
public ListPublicKeysResult listPublicKeys(ListPublicKeysRequest request) {
request = beforeClientExecution(request);
return executeListPublicKeys(request);
}
@SdkInternalApi
final ListPublicKeysResult executeListPublicKeys(ListPublicKeysRequest listPublicKeysRequest) {
ExecutionContext executionContext = createExecutionContext(listPublicKeysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPublicKeysRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPublicKeysRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPublicKeysResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListPublicKeysResult listPublicKeys() {
return listPublicKeys(new ListPublicKeysRequest());
}
/**
*
* Lists the tags for the trail in the current region.
*
*
* @param listTagsRequest
* Specifies a list of trail tags to return.
* @return Result of the ListTags operation returned by the service.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an invalid trail ARN. The format of a trail ARN
* is:
*
* arn:aws:cloudtrail:us-east-1:123456789012:trail/MyTrail
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws InvalidTokenException
* Reserved for future use.
* @sample AWSCloudTrail.ListTags
* @see AWS API
* Documentation
*/
@Override
public ListTagsResult listTags(ListTagsRequest request) {
request = beforeClientExecution(request);
return executeListTags(request);
}
@SdkInternalApi
final ListTagsResult executeListTags(ListTagsRequest listTagsRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Looks up API activity events captured by CloudTrail that create, update, or delete resources in your account.
* Events for a region can be looked up for the times in which you had CloudTrail turned on in that region during
* the last seven days. Lookup supports the following attributes:
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
* -
*
* Resource name
*
*
* -
*
* Resource type
*
*
* -
*
* User name
*
*
*
*
* All attributes are optional. The default number of results returned is 10, with a maximum of 50 possible. The
* response includes a token that you can use to get the next page of results.
*
*
*
* The rate of lookup requests is limited to one per second per account. If this limit is exceeded, a throttling
* error occurs.
*
*
*
* Events that occurred during the selected time range will not be available for lookup if CloudTrail logging was
* not enabled when the events occurred.
*
*
*
* @param lookupEventsRequest
* Contains a request for LookupEvents.
* @return Result of the LookupEvents operation returned by the service.
* @throws InvalidLookupAttributesException
* Occurs when an invalid lookup attribute is specified.
* @throws InvalidTimeRangeException
* Occurs if the timestamp values are invalid. Either the start time occurs after the end time or the time
* range is outside the range of possible values.
* @throws InvalidMaxResultsException
* This exception is thrown if the limit specified is invalid.
* @throws InvalidNextTokenException
* Invalid token or token that was previously used in a request with different parameters. This exception is
* thrown if the token is invalid.
* @sample AWSCloudTrail.LookupEvents
* @see AWS API
* Documentation
*/
@Override
public LookupEventsResult lookupEvents(LookupEventsRequest request) {
request = beforeClientExecution(request);
return executeLookupEvents(request);
}
@SdkInternalApi
final LookupEventsResult executeLookupEvents(LookupEventsRequest lookupEventsRequest) {
ExecutionContext executionContext = createExecutionContext(lookupEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new LookupEventsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(lookupEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new LookupEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public LookupEventsResult lookupEvents() {
return lookupEvents(new LookupEventsRequest());
}
/**
*
* Configures an event selector for your trail. Use event selectors to specify whether you want your trail to log
* management and/or data events. When an event occurs in your account, CloudTrail evaluates the event selectors in
* all trails. For each trail, if the event matches any event selector, the trail processes and logs the event. If
* the event doesn't match any event selector, the trail doesn't log the event.
*
*
* Example
*
*
* -
*
* You create an event selector for a trail and specify that you want write-only events.
*
*
* -
*
* The EC2 GetConsoleOutput
and RunInstances
API operations occur in your account.
*
*
* -
*
* CloudTrail evaluates whether the events match your event selectors.
*
*
* -
*
* The RunInstances
is a write-only event and it matches your event selector. The trail logs the event.
*
*
* -
*
* The GetConsoleOutput
is a read-only event but it doesn't match your event selector. The trail
* doesn't log the event.
*
*
*
*
* The PutEventSelectors
operation must be called from the region in which the trail was created;
* otherwise, an InvalidHomeRegionException
is thrown.
*
*
* You can configure up to five event selectors for each trail. For more information, see Logging Data and Management Events for Trails in the AWS CloudTrail User Guide.
*
*
* @param putEventSelectorsRequest
* @return Result of the PutEventSelectors operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a region other than the region in
* which the trail was created.
* @throws InvalidEventSelectorsException
* This exception is thrown when the PutEventSelectors
operation is called with an invalid
* number of event selectors, data resources, or an invalid value for a parameter:
*
* -
*
* Specify a valid number of event selectors (1 to 5) for a trail.
*
*
* -
*
* Specify a valid number of data resources (1 to 250) for an event selector.
*
*
* -
*
* Specify a valid value for a parameter. For example, specifying the ReadWriteType
parameter
* with a value of read-only
is invalid.
*
*
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.PutEventSelectors
* @see AWS
* API Documentation
*/
@Override
public PutEventSelectorsResult putEventSelectors(PutEventSelectorsRequest request) {
request = beforeClientExecution(request);
return executePutEventSelectors(request);
}
@SdkInternalApi
final PutEventSelectorsResult executePutEventSelectors(PutEventSelectorsRequest putEventSelectorsRequest) {
ExecutionContext executionContext = createExecutionContext(putEventSelectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutEventSelectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putEventSelectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutEventSelectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified tags from a trail.
*
*
* @param removeTagsRequest
* Specifies the tags to remove from a trail.
* @return Result of the RemoveTags operation returned by the service.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an invalid trail ARN. The format of a trail ARN
* is:
*
* arn:aws:cloudtrail:us-east-1:123456789012:trail/MyTrail
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidTagParameterException
* This exception is thrown when the key or value specified for the tag does not match the regular
* expression ^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$
.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.RemoveTags
* @see AWS API
* Documentation
*/
@Override
public RemoveTagsResult removeTags(RemoveTagsRequest request) {
request = beforeClientExecution(request);
return executeRemoveTags(request);
}
@SdkInternalApi
final RemoveTagsResult executeRemoveTags(RemoveTagsRequest removeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(removeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemoveTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts the recording of AWS API calls and log file delivery for a trail. For a trail that is enabled in all
* regions, this operation must be called from the region in which the trail was created. This operation cannot be
* called on the shadow trails (replicated trails in other regions) of a trail that is enabled in all regions.
*
*
* @param startLoggingRequest
* The request to CloudTrail to start logging AWS API calls for an account.
* @return Result of the StartLogging operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a region other than the region in
* which the trail was created.
* @sample AWSCloudTrail.StartLogging
* @see AWS API
* Documentation
*/
@Override
public StartLoggingResult startLogging(StartLoggingRequest request) {
request = beforeClientExecution(request);
return executeStartLogging(request);
}
@SdkInternalApi
final StartLoggingResult executeStartLogging(StartLoggingRequest startLoggingRequest) {
ExecutionContext executionContext = createExecutionContext(startLoggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartLoggingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startLoggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartLoggingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Suspends the recording of AWS API calls and log file delivery for the specified trail. Under most circumstances,
* there is no need to use this action. You can update a trail without stopping it first. This action is the only
* way to stop recording. For a trail enabled in all regions, this operation must be called from the region in which
* the trail was created, or an InvalidHomeRegionException
will occur. This operation cannot be called
* on the shadow trails (replicated trails in other regions) of a trail enabled in all regions.
*
*
* @param stopLoggingRequest
* Passes the request to CloudTrail to stop logging AWS API calls for the specified account.
* @return Result of the StopLogging operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a region other than the region in
* which the trail was created.
* @sample AWSCloudTrail.StopLogging
* @see AWS API
* Documentation
*/
@Override
public StopLoggingResult stopLogging(StopLoggingRequest request) {
request = beforeClientExecution(request);
return executeStopLogging(request);
}
@SdkInternalApi
final StopLoggingResult executeStopLogging(StopLoggingRequest stopLoggingRequest) {
ExecutionContext executionContext = createExecutionContext(stopLoggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopLoggingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopLoggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopLoggingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the settings that specify delivery of log files. Changes to a trail do not require stopping the
* CloudTrail service. Use this action to designate an existing bucket for log delivery. If the existing bucket has
* previously been a target for CloudTrail log files, an IAM policy exists for the bucket. UpdateTrail
* must be called from the region in which the trail was created; otherwise, an
* InvalidHomeRegionException
is thrown.
*
*
* @param updateTrailRequest
* Specifies settings to update for the trail.
* @return Result of the UpdateTrail operation returned by the service.
* @throws S3BucketDoesNotExistException
* This exception is thrown when the specified S3 bucket does not exist.
* @throws InsufficientS3BucketPolicyException
* This exception is thrown when the policy on the S3 bucket is not sufficient.
* @throws InsufficientSnsTopicPolicyException
* This exception is thrown when the policy on the SNS topic is not sufficient.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key is not sufficient.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidS3BucketNameException
* This exception is thrown when the provided S3 bucket name is not valid.
* @throws InvalidS3PrefixException
* This exception is thrown when the provided S3 prefix is not valid.
* @throws InvalidSnsTopicNameException
* This exception is thrown when the provided SNS topic name is not valid.
* @throws InvalidKmsKeyIdException
* This exception is thrown when the KMS key ARN is invalid.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are invalid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws TrailNotProvidedException
* This exception is deprecated.
* @throws InvalidParameterCombinationException
* This exception is thrown when the combination of parameters provided is not valid.
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a region other than the region in
* which the trail was created.
* @throws KmsKeyNotFoundException
* This exception is thrown when the KMS key does not exist, or when the S3 bucket and the KMS key are not
* in the same region.
* @throws KmsKeyDisabledException
* This exception is deprecated.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail can’t be
* updated.
* @throws InvalidCloudWatchLogsLogGroupArnException
* This exception is thrown when the provided CloudWatch log group is not valid.
* @throws InvalidCloudWatchLogsRoleArnException
* This exception is thrown when the provided role is not valid.
* @throws CloudWatchLogsDeliveryUnavailableException
* Cannot set a CloudWatch Logs delivery for this region.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.UpdateTrail
* @see AWS API
* Documentation
*/
@Override
public UpdateTrailResult updateTrail(UpdateTrailRequest request) {
request = beforeClientExecution(request);
return executeUpdateTrail(request);
}
@SdkInternalApi
final UpdateTrailResult executeUpdateTrail(UpdateTrailRequest updateTrailRequest) {
ExecutionContext executionContext = createExecutionContext(updateTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}