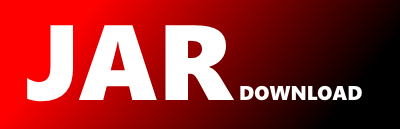
com.amazonaws.services.cloudtrail.AWSCloudTrailClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.cloudtrail.AWSCloudTrailClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.cloudtrail.model.*;
import com.amazonaws.services.cloudtrail.model.transform.*;
/**
* Client for accessing CloudTrail. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* CloudTrail
*
* This is the CloudTrail API Reference. It provides descriptions of actions, data types, common parameters, and common
* errors for CloudTrail.
*
*
* CloudTrail is a web service that records Amazon Web Services API calls for your Amazon Web Services account and
* delivers log files to an Amazon S3 bucket. The recorded information includes the identity of the user, the start time
* of the Amazon Web Services API call, the source IP address, the request parameters, and the response elements
* returned by the service.
*
*
*
* As an alternative to the API, you can use one of the Amazon Web Services SDKs, which consist of libraries and sample
* code for various programming languages and platforms (Java, Ruby, .NET, iOS, Android, etc.). The SDKs provide
* programmatic access to CloudTrail. For example, the SDKs handle cryptographically signing requests, managing errors,
* and retrying requests automatically. For more information about the Amazon Web Services SDKs, including how to
* download and install them, see Tools to Build on Amazon Web Services.
*
*
*
* See the CloudTrail
* User Guide for information about the data that is included with each Amazon Web Services API call listed in the
* log files.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSCloudTrailClient extends AmazonWebServiceClient implements AWSCloudTrail {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSCloudTrail.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "cloudtrail";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConcurrentModificationException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ConcurrentModificationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreMaxLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreMaxLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreTerminationProtectedException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreTerminationProtectedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourcePolicyNotValidException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ResourcePolicyNotValidExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidKmsKeyIdException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidKmsKeyIdExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidCloudWatchLogsLogGroupArnException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidCloudWatchLogsLogGroupArnExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidEventDataStoreCategoryException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidEventDataStoreCategoryExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidSourceException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidSourceExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotOrganizationMasterAccountException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.NotOrganizationMasterAccountExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidLookupAttributesException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidLookupAttributesExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CloudTrailInvalidClientTokenIdException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.CloudTrailInvalidClientTokenIdExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OrganizationsNotInUseException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.OrganizationsNotInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidNextTokenException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidNextTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidEventSelectorsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidEventSelectorsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ChannelARNInvalidException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ChannelARNInvalidExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedOperationException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.UnsupportedOperationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidEventDataStoreStatusException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidEventDataStoreStatusExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccountNotRegisteredException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.AccountNotRegisteredExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreFederationEnabledException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreFederationEnabledExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CloudTrailAccessNotEnabledException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.CloudTrailAccessNotEnabledExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTrailNameException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidTrailNameExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidImportSourceException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidImportSourceExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientEncryptionPolicyException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InsufficientEncryptionPolicyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourcePolicyNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ResourcePolicyNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DelegatedAdminAccountLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.DelegatedAdminAccountLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidS3PrefixException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidS3PrefixExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientSnsTopicPolicyException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InsufficientSnsTopicPolicyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidHomeRegionException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidHomeRegionExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientDependencyServiceAccessPermissionException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InsufficientDependencyServiceAccessPermissionExceptionUnmarshaller
.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ChannelAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ChannelAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidParameterExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreARNInvalidException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreARNInvalidExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("QueryIdNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.QueryIdNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InactiveEventDataStoreException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InactiveEventDataStoreExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TrailNotProvidedException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.TrailNotProvidedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidQueryStatusException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidQueryStatusExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidEventCategoryException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidEventCategoryExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("KmsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.KmsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OrganizationNotInAllFeaturesModeException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.OrganizationNotInAllFeaturesModeExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("S3BucketDoesNotExistException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.S3BucketDoesNotExistExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NoManagementAccountSLRExistsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.NoManagementAccountSLRExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CannotDelegateManagementAccountException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.CannotDelegateManagementAccountExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TagsLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.TagsLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidSnsTopicNameException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidSnsTopicNameExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("KmsKeyNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.KmsKeyNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccountRegisteredException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.AccountRegisteredExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OperationNotPermittedException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.OperationNotPermittedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ChannelExistsForEDSException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ChannelExistsForEDSExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccountHasOngoingImportException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.AccountHasOngoingImportExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceTypeNotSupportedException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ResourceTypeNotSupportedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("KmsKeyDisabledException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.KmsKeyDisabledExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTokenException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CloudTrailARNInvalidException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.CloudTrailARNInvalidExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidCloudWatchLogsRoleArnException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidCloudWatchLogsRoleArnExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidQueryStatementException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidQueryStatementExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceARNNotValidException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ResourceARNNotValidExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTimeRangeException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidTimeRangeExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ImportNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ImportNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccountNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.AccountNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterCombinationException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidParameterCombinationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidMaxResultsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidMaxResultsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidS3BucketNameException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidS3BucketNameExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CloudWatchLogsDeliveryUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.CloudWatchLogsDeliveryUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InactiveQueryException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InactiveQueryExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ChannelMaxLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ChannelMaxLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTagParameterException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidTagParameterExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TrailNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.TrailNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MaximumNumberOfTrailsExceededException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.MaximumNumberOfTrailsExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EventDataStoreHasOngoingImportException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.EventDataStoreHasOngoingImportExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidInsightSelectorsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidInsightSelectorsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidDateRangeException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InvalidDateRangeExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotOrganizationManagementAccountException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.NotOrganizationManagementAccountExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ChannelNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.ChannelNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MaxConcurrentQueriesException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.MaxConcurrentQueriesExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientS3BucketPolicyException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InsufficientS3BucketPolicyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TrailAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.TrailAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsightNotEnabledException").withExceptionUnmarshaller(
com.amazonaws.services.cloudtrail.model.transform.InsightNotEnabledExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.cloudtrail.model.AWSCloudTrailException.class));
/**
* Constructs a new client to invoke service methods on CloudTrail. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSCloudTrailClientBuilder#defaultClient()}
*/
@Deprecated
public AWSCloudTrailClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudTrail. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSCloudTrailClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSCloudTrailClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to CloudTrail (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSCloudTrailClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSCloudTrailClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSCloudTrailClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSCloudTrailClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AWSCloudTrailClientBuilder builder() {
return AWSCloudTrailClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSCloudTrailClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on CloudTrail using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSCloudTrailClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("cloudtrail.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/cloudtrail/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/cloudtrail/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Adds one or more tags to a trail, event data store, or channel, up to a limit of 50. Overwrites an existing tag's
* value when a new value is specified for an existing tag key. Tag key names must be unique; you cannot have two
* keys with the same name but different values. If you specify a key without a value, the tag will be created with
* the specified key and a value of null. You can tag a trail or event data store that applies to all Amazon Web
* Services Regions only from the Region in which the trail or event data store was created (also known as its home
* Region).
*
*
* @param addTagsRequest
* Specifies the tags to add to a trail, event data store, or channel.
* @return Result of the AddTags operation returned by the service.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws ChannelARNInvalidException
* This exception is thrown when the specified value of ChannelARN
is not valid.
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws TagsLimitExceededException
* The number of tags per trail, event data store, or channel has exceeded the permitted amount. Currently,
* the limit is 50.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidTagParameterException
* This exception is thrown when the specified tag key or values are not valid. It can also occur if there
* are duplicate tags or too many tags on the resource.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws ChannelNotFoundException
* This exception is thrown when CloudTrail cannot find the specified channel.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @sample AWSCloudTrail.AddTags
* @see AWS API
* Documentation
*/
@Override
public AddTagsResult addTags(AddTagsRequest request) {
request = beforeClientExecution(request);
return executeAddTags(request);
}
@SdkInternalApi
final AddTagsResult executeAddTags(AddTagsRequest addTagsRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(addTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new AddTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels a query if the query is not in a terminated state, such as CANCELLED
, FAILED
,
* TIMED_OUT
, or FINISHED
. You must specify an ARN value for EventDataStore
.
* The ID of the query that you want to cancel is also required. When you run CancelQuery
, the query
* status might show as CANCELLED
even if the operation is not yet finished.
*
*
* @param cancelQueryRequest
* @return Result of the CancelQuery operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InactiveQueryException
* The specified query cannot be canceled because it is in the FINISHED
, FAILED
,
* TIMED_OUT
, or CANCELLED
state.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws QueryIdNotFoundException
* The query ID does not exist or does not map to a query.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @sample AWSCloudTrail.CancelQuery
* @see AWS API
* Documentation
*/
@Override
public CancelQueryResult cancelQuery(CancelQueryRequest request) {
request = beforeClientExecution(request);
return executeCancelQuery(request);
}
@SdkInternalApi
final CancelQueryResult executeCancelQuery(CancelQueryRequest cancelQueryRequest) {
ExecutionContext executionContext = createExecutionContext(cancelQueryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelQueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(cancelQueryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelQuery");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CancelQueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a channel for CloudTrail to ingest events from a partner or external source. After you create a channel,
* a CloudTrail Lake event data store can log events from the partner or source that you specify.
*
*
* @param createChannelRequest
* @return Result of the CreateChannel operation returned by the service.
* @throws ChannelMaxLimitExceededException
* This exception is thrown when the maximum number of channels limit is exceeded.
* @throws InvalidSourceException
* This exception is thrown when the specified value of Source
is not valid.
* @throws ChannelAlreadyExistsException
* This exception is thrown when the provided channel already exists.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidEventDataStoreCategoryException
* This exception is thrown when event categories of specified event data stores are not valid.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidTagParameterException
* This exception is thrown when the specified tag key or values are not valid. It can also occur if there
* are duplicate tags or too many tags on the resource.
* @throws TagsLimitExceededException
* The number of tags per trail, event data store, or channel has exceeded the permitted amount. Currently,
* the limit is 50.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.CreateChannel
* @see AWS API
* Documentation
*/
@Override
public CreateChannelResult createChannel(CreateChannelRequest request) {
request = beforeClientExecution(request);
return executeCreateChannel(request);
}
@SdkInternalApi
final CreateChannelResult executeCreateChannel(CreateChannelRequest createChannelRequest) {
ExecutionContext executionContext = createExecutionContext(createChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new event data store.
*
*
* @param createEventDataStoreRequest
* @return Result of the CreateEventDataStore operation returned by the service.
* @throws EventDataStoreAlreadyExistsException
* An event data store with that name already exists.
* @throws EventDataStoreMaxLimitExceededException
* Your account has used the maximum number of event data stores.
* @throws InvalidEventSelectorsException
* This exception is thrown when the PutEventSelectors
operation is called with a number of
* event selectors, advanced event selectors, or data resources that is not valid. The combination of event
* selectors or advanced event selectors and data resources is not valid. A trail can have up to 5 event
* selectors. If a trail uses advanced event selectors, a maximum of 500 total values for all conditions in
* all advanced event selectors is allowed. A trail is limited to 250 data resources. These data resources
* can be distributed across event selectors, but the overall total cannot exceed 250.
*
* You can:
*
*
* -
*
* Specify a valid number of event selectors (1 to 5) for a trail.
*
*
* -
*
* Specify a valid number of data resources (1 to 250) for an event selector. The limit of number of
* resources on an individual event selector is configurable up to 250. However, this upper limit is allowed
* only if the total number of data resources does not exceed 250 across all event selectors for a trail.
*
*
* -
*
* Specify up to 500 values for all conditions in all advanced event selectors for a trail.
*
*
* -
*
* Specify a valid value for a parameter. For example, specifying the ReadWriteType
parameter
* with a value of read-only
is not valid.
*
*
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidTagParameterException
* This exception is thrown when the specified tag key or values are not valid. It can also occur if there
* are duplicate tags or too many tags on the resource.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws InvalidKmsKeyIdException
* This exception is thrown when the KMS key ARN is not valid.
* @throws KmsKeyNotFoundException
* This exception is thrown when the KMS key does not exist, when the S3 bucket and the KMS key are not in
* the same Region, or when the KMS key associated with the Amazon SNS topic either does not exist or is not
* in the same Region.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail or event data
* store can't be updated.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.CreateEventDataStore
* @see AWS API Documentation
*/
@Override
public CreateEventDataStoreResult createEventDataStore(CreateEventDataStoreRequest request) {
request = beforeClientExecution(request);
return executeCreateEventDataStore(request);
}
@SdkInternalApi
final CreateEventDataStoreResult executeCreateEventDataStore(CreateEventDataStoreRequest createEventDataStoreRequest) {
ExecutionContext executionContext = createExecutionContext(createEventDataStoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEventDataStoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEventDataStoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEventDataStore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEventDataStoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a trail that specifies the settings for delivery of log data to an Amazon S3 bucket.
*
*
* @param createTrailRequest
* Specifies the settings for each trail.
* @return Result of the CreateTrail operation returned by the service.
* @throws MaximumNumberOfTrailsExceededException
* This exception is thrown when the maximum number of trails is reached.
* @throws TrailAlreadyExistsException
* This exception is thrown when the specified trail already exists.
* @throws S3BucketDoesNotExistException
* This exception is thrown when the specified S3 bucket does not exist.
* @throws InsufficientS3BucketPolicyException
* This exception is thrown when the policy on the S3 bucket is not sufficient.
* @throws InsufficientSnsTopicPolicyException
* This exception is thrown when the policy on the Amazon SNS topic is not sufficient.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws InvalidS3BucketNameException
* This exception is thrown when the provided S3 bucket name is not valid.
* @throws InvalidS3PrefixException
* This exception is thrown when the provided S3 prefix is not valid.
* @throws InvalidSnsTopicNameException
* This exception is thrown when the provided SNS topic name is not valid.
* @throws InvalidKmsKeyIdException
* This exception is thrown when the KMS key ARN is not valid.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws TrailNotProvidedException
* This exception is no longer in use.
* @throws TagsLimitExceededException
* The number of tags per trail, event data store, or channel has exceeded the permitted amount. Currently,
* the limit is 50.
* @throws InvalidParameterCombinationException
* This exception is thrown when the combination of parameters provided is not valid.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws KmsKeyNotFoundException
* This exception is thrown when the KMS key does not exist, when the S3 bucket and the KMS key are not in
* the same Region, or when the KMS key associated with the Amazon SNS topic either does not exist or is not
* in the same Region.
* @throws KmsKeyDisabledException
* This exception is no longer in use.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail or event data
* store can't be updated.
* @throws InvalidCloudWatchLogsLogGroupArnException
* This exception is thrown when the provided CloudWatch Logs log group is not valid.
* @throws InvalidCloudWatchLogsRoleArnException
* This exception is thrown when the provided role is not valid.
* @throws CloudWatchLogsDeliveryUnavailableException
* Cannot set a CloudWatch Logs delivery for this Region.
* @throws InvalidTagParameterException
* This exception is thrown when the specified tag key or values are not valid. It can also occur if there
* are duplicate tags or too many tags on the resource.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws CloudTrailInvalidClientTokenIdException
* This exception is thrown when a call results in the InvalidClientTokenId
error code. This
* can occur when you are creating or updating a trail to send notifications to an Amazon SNS topic that is
* in a suspended Amazon Web Services account.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @sample AWSCloudTrail.CreateTrail
* @see AWS API
* Documentation
*/
@Override
public CreateTrailResult createTrail(CreateTrailRequest request) {
request = beforeClientExecution(request);
return executeCreateTrail(request);
}
@SdkInternalApi
final CreateTrailResult executeCreateTrail(CreateTrailRequest createTrailRequest) {
ExecutionContext executionContext = createExecutionContext(createTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTrail");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a channel.
*
*
* @param deleteChannelRequest
* @return Result of the DeleteChannel operation returned by the service.
* @throws ChannelARNInvalidException
* This exception is thrown when the specified value of ChannelARN
is not valid.
* @throws ChannelNotFoundException
* This exception is thrown when CloudTrail cannot find the specified channel.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.DeleteChannel
* @see AWS API
* Documentation
*/
@Override
public DeleteChannelResult deleteChannel(DeleteChannelRequest request) {
request = beforeClientExecution(request);
return executeDeleteChannel(request);
}
@SdkInternalApi
final DeleteChannelResult executeDeleteChannel(DeleteChannelRequest deleteChannelRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables the event data store specified by EventDataStore
, which accepts an event data store ARN.
* After you run DeleteEventDataStore
, the event data store enters a PENDING_DELETION
* state, and is automatically deleted after a wait period of seven days. TerminationProtectionEnabled
* must be set to False
on the event data store and the FederationStatus
must be
* DISABLED
. You cannot delete an event data store if TerminationProtectionEnabled
is
* True
or the FederationStatus
is ENABLED
.
*
*
* After you run DeleteEventDataStore
on an event data store, you cannot run ListQueries
,
* DescribeQuery
, or GetQueryResults
on queries that are using an event data store in a
* PENDING_DELETION
state. An event data store in the PENDING_DELETION
state does not
* incur costs.
*
*
* @param deleteEventDataStoreRequest
* @return Result of the DeleteEventDataStore operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws EventDataStoreTerminationProtectedException
* The event data store cannot be deleted because termination protection is enabled for it.
* @throws EventDataStoreHasOngoingImportException
* This exception is thrown when you try to update or delete an event data store that currently has an
* import in progress.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws ChannelExistsForEDSException
* This exception is thrown when the specified event data store cannot yet be deleted because it is in use
* by a channel.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws EventDataStoreFederationEnabledException
* You cannot delete the event data store because Lake query federation is enabled. To delete the event data
* store, run the DisableFederation
operation to disable Lake query federation on the event
* data store.
* @sample AWSCloudTrail.DeleteEventDataStore
* @see AWS API Documentation
*/
@Override
public DeleteEventDataStoreResult deleteEventDataStore(DeleteEventDataStoreRequest request) {
request = beforeClientExecution(request);
return executeDeleteEventDataStore(request);
}
@SdkInternalApi
final DeleteEventDataStoreResult executeDeleteEventDataStore(DeleteEventDataStoreRequest deleteEventDataStoreRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventDataStoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventDataStoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEventDataStoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEventDataStore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEventDataStoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the resource-based policy attached to the CloudTrail channel.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ResourceARNNotValidException
* This exception is thrown when the provided resource does not exist, or the ARN format of the resource is
* not valid. The following is the valid format for a resource ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/MyChannel
.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws ResourcePolicyNotFoundException
* This exception is thrown when the specified resource policy is not found.
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.DeleteResourcePolicy
* @see AWS API Documentation
*/
@Override
public DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteResourcePolicy(request);
}
@SdkInternalApi
final DeleteResourcePolicyResult executeDeleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a trail. This operation must be called from the Region in which the trail was created.
* DeleteTrail
cannot be called on the shadow trails (replicated trails in other Regions) of a trail
* that is enabled in all Regions.
*
*
* @param deleteTrailRequest
* The request that specifies the name of a trail to delete.
* @return Result of the DeleteTrail operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a Region other than the Region in
* which the trail was created.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @sample AWSCloudTrail.DeleteTrail
* @see AWS API
* Documentation
*/
@Override
public DeleteTrailResult deleteTrail(DeleteTrailRequest request) {
request = beforeClientExecution(request);
return executeDeleteTrail(request);
}
@SdkInternalApi
final DeleteTrailResult executeDeleteTrail(DeleteTrailRequest deleteTrailRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTrail");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes CloudTrail delegated administrator permissions from a member account in an organization.
*
*
* @param deregisterOrganizationDelegatedAdminRequest
* Removes CloudTrail delegated administrator permissions from a specified member account in an organization
* that is currently designated as a delegated administrator.
* @return Result of the DeregisterOrganizationDelegatedAdmin operation returned by the service.
* @throws AccountNotFoundException
* This exception is thrown when the specified account is not found or not part of an organization.
* @throws AccountNotRegisteredException
* This exception is thrown when the specified account is not registered as the CloudTrail delegated
* administrator.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws NotOrganizationManagementAccountException
* This exception is thrown when the account making the request is not the organization's management
* account.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.DeregisterOrganizationDelegatedAdmin
* @see AWS API Documentation
*/
@Override
public DeregisterOrganizationDelegatedAdminResult deregisterOrganizationDelegatedAdmin(DeregisterOrganizationDelegatedAdminRequest request) {
request = beforeClientExecution(request);
return executeDeregisterOrganizationDelegatedAdmin(request);
}
@SdkInternalApi
final DeregisterOrganizationDelegatedAdminResult executeDeregisterOrganizationDelegatedAdmin(
DeregisterOrganizationDelegatedAdminRequest deregisterOrganizationDelegatedAdminRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterOrganizationDelegatedAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterOrganizationDelegatedAdminRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deregisterOrganizationDelegatedAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterOrganizationDelegatedAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeregisterOrganizationDelegatedAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata about a query, including query run time in milliseconds, number of events scanned and matched,
* and query status. If the query results were delivered to an S3 bucket, the response also provides the S3 URI and
* the delivery status.
*
*
* You must specify either a QueryID
or a QueryAlias
. Specifying the
* QueryAlias
parameter returns information about the last query run for the alias.
*
*
* @param describeQueryRequest
* @return Result of the DescribeQuery operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws QueryIdNotFoundException
* The query ID does not exist or does not map to a query.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.DescribeQuery
* @see AWS API
* Documentation
*/
@Override
public DescribeQueryResult describeQuery(DescribeQueryRequest request) {
request = beforeClientExecution(request);
return executeDescribeQuery(request);
}
@SdkInternalApi
final DescribeQueryResult executeDescribeQuery(DescribeQueryRequest describeQueryRequest) {
ExecutionContext executionContext = createExecutionContext(describeQueryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeQueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeQueryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeQuery");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeQueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves settings for one or more trails associated with the current Region for your account.
*
*
* @param describeTrailsRequest
* Returns information about the trail.
* @return Result of the DescribeTrails operation returned by the service.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.DescribeTrails
* @see AWS API
* Documentation
*/
@Override
public DescribeTrailsResult describeTrails(DescribeTrailsRequest request) {
request = beforeClientExecution(request);
return executeDescribeTrails(request);
}
@SdkInternalApi
final DescribeTrailsResult executeDescribeTrails(DescribeTrailsRequest describeTrailsRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrailsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeTrailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeTrails");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeTrailsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeTrailsResult describeTrails() {
return describeTrails(new DescribeTrailsRequest());
}
/**
*
* Disables Lake query federation on the specified event data store. When you disable federation, CloudTrail
* disables the integration with Glue, Lake Formation, and Amazon Athena. After disabling Lake query federation, you
* can no longer query your event data in Amazon Athena.
*
*
* No CloudTrail Lake data is deleted when you disable federation and you can continue to run queries in CloudTrail
* Lake.
*
*
* @param disableFederationRequest
* @return Result of the DisableFederation operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws ConcurrentModificationException
* You are trying to update a resource when another request is in progress. Allow sufficient wait time for
* the previous request to complete, then retry your request.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSCloudTrail.DisableFederation
* @see AWS
* API Documentation
*/
@Override
public DisableFederationResult disableFederation(DisableFederationRequest request) {
request = beforeClientExecution(request);
return executeDisableFederation(request);
}
@SdkInternalApi
final DisableFederationResult executeDisableFederation(DisableFederationRequest disableFederationRequest) {
ExecutionContext executionContext = createExecutionContext(disableFederationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableFederationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableFederationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableFederation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableFederationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables Lake query federation on the specified event data store. Federating an event data store lets you view the
* metadata associated with the event data store in the Glue Data Catalog
* and run SQL queries against your event data using Amazon Athena. The table metadata stored in the Glue Data
* Catalog lets the Athena query engine know how to find, read, and process the data that you want to query.
*
*
* When you enable Lake query federation, CloudTrail creates a managed database named aws:cloudtrail
* (if the database doesn't already exist) and a managed federated table in the Glue Data Catalog. The event data
* store ID is used for the table name. CloudTrail registers the role ARN and event data store in Lake
* Formation, the service responsible for allowing fine-grained access control of the federated resources in the
* Glue Data Catalog.
*
*
* For more information about Lake query federation, see Federate an event data
* store.
*
*
* @param enableFederationRequest
* @return Result of the EnableFederation operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws ConcurrentModificationException
* You are trying to update a resource when another request is in progress. Allow sufficient wait time for
* the previous request to complete, then retry your request.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws EventDataStoreFederationEnabledException
* You cannot delete the event data store because Lake query federation is enabled. To delete the event data
* store, run the DisableFederation
operation to disable Lake query federation on the event
* data store.
* @sample AWSCloudTrail.EnableFederation
* @see AWS
* API Documentation
*/
@Override
public EnableFederationResult enableFederation(EnableFederationRequest request) {
request = beforeClientExecution(request);
return executeEnableFederation(request);
}
@SdkInternalApi
final EnableFederationResult executeEnableFederation(EnableFederationRequest enableFederationRequest) {
ExecutionContext executionContext = createExecutionContext(enableFederationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableFederationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableFederationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableFederation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableFederationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a specific channel.
*
*
* @param getChannelRequest
* @return Result of the GetChannel operation returned by the service.
* @throws ChannelARNInvalidException
* This exception is thrown when the specified value of ChannelARN
is not valid.
* @throws ChannelNotFoundException
* This exception is thrown when CloudTrail cannot find the specified channel.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.GetChannel
* @see AWS API
* Documentation
*/
@Override
public GetChannelResult getChannel(GetChannelRequest request) {
request = beforeClientExecution(request);
return executeGetChannel(request);
}
@SdkInternalApi
final GetChannelResult executeGetChannel(GetChannelRequest getChannelRequest) {
ExecutionContext executionContext = createExecutionContext(getChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about an event data store specified as either an ARN or the ID portion of the ARN.
*
*
* @param getEventDataStoreRequest
* @return Result of the GetEventDataStore operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.GetEventDataStore
* @see AWS
* API Documentation
*/
@Override
public GetEventDataStoreResult getEventDataStore(GetEventDataStoreRequest request) {
request = beforeClientExecution(request);
return executeGetEventDataStore(request);
}
@SdkInternalApi
final GetEventDataStoreResult executeGetEventDataStore(GetEventDataStoreRequest getEventDataStoreRequest) {
ExecutionContext executionContext = createExecutionContext(getEventDataStoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEventDataStoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEventDataStoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEventDataStore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEventDataStoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the settings for the event selectors that you configured for your trail. The information returned for
* your event selectors includes the following:
*
*
* -
*
* If your event selector includes read-only events, write-only events, or all events. This applies to both
* management events and data events.
*
*
* -
*
* If your event selector includes management events.
*
*
* -
*
* If your event selector includes data events, the resources on which you are logging data events.
*
*
*
*
* For more information about logging management and data events, see the following topics in the CloudTrail User
* Guide:
*
*
* -
*
*
* -
*
*
*
*
* @param getEventSelectorsRequest
* @return Result of the GetEventSelectors operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.GetEventSelectors
* @see AWS
* API Documentation
*/
@Override
public GetEventSelectorsResult getEventSelectors(GetEventSelectorsRequest request) {
request = beforeClientExecution(request);
return executeGetEventSelectors(request);
}
@SdkInternalApi
final GetEventSelectorsResult executeGetEventSelectors(GetEventSelectorsRequest getEventSelectorsRequest) {
ExecutionContext executionContext = createExecutionContext(getEventSelectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEventSelectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEventSelectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEventSelectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEventSelectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a specific import.
*
*
* @param getImportRequest
* @return Result of the GetImport operation returned by the service.
* @throws ImportNotFoundException
* The specified import was not found.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.GetImport
* @see AWS API
* Documentation
*/
@Override
public GetImportResult getImport(GetImportRequest request) {
request = beforeClientExecution(request);
return executeGetImport(request);
}
@SdkInternalApi
final GetImportResult executeGetImport(GetImportRequest getImportRequest) {
ExecutionContext executionContext = createExecutionContext(getImportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetImportRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getImportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetImport");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetImportResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the settings for the Insights event selectors that you configured for your trail or event data store.
* GetInsightSelectors
shows if CloudTrail Insights event logging is enabled on the trail or event data
* store, and if it is, which Insights types are enabled. If you run GetInsightSelectors
on a trail or
* event data store that does not have Insights events enabled, the operation throws the exception
* InsightNotEnabledException
*
*
* Specify either the EventDataStore
parameter to get Insights event selectors for an event data store,
* or the TrailName
parameter to the get Insights event selectors for a trail. You cannot specify these
* parameters together.
*
*
* For more information, see Logging CloudTrail Insights events in the CloudTrail User Guide.
*
*
* @param getInsightSelectorsRequest
* @return Result of the GetInsightSelectors operation returned by the service.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidParameterCombinationException
* This exception is thrown when the combination of parameters provided is not valid.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws InsightNotEnabledException
* If you run GetInsightSelectors
on a trail or event data store that does not have Insights
* events enabled, the operation throws the exception InsightNotEnabledException
.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @sample AWSCloudTrail.GetInsightSelectors
* @see AWS
* API Documentation
*/
@Override
public GetInsightSelectorsResult getInsightSelectors(GetInsightSelectorsRequest request) {
request = beforeClientExecution(request);
return executeGetInsightSelectors(request);
}
@SdkInternalApi
final GetInsightSelectorsResult executeGetInsightSelectors(GetInsightSelectorsRequest getInsightSelectorsRequest) {
ExecutionContext executionContext = createExecutionContext(getInsightSelectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetInsightSelectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getInsightSelectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetInsightSelectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetInsightSelectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets event data results of a query. You must specify the QueryID
value returned by the
* StartQuery
operation.
*
*
* @param getQueryResultsRequest
* @return Result of the GetQueryResults operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidMaxResultsException
* This exception is thrown if the limit specified is not valid.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws QueryIdNotFoundException
* The query ID does not exist or does not map to a query.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.GetQueryResults
* @see AWS API
* Documentation
*/
@Override
public GetQueryResultsResult getQueryResults(GetQueryResultsRequest request) {
request = beforeClientExecution(request);
return executeGetQueryResults(request);
}
@SdkInternalApi
final GetQueryResultsResult executeGetQueryResults(GetQueryResultsRequest getQueryResultsRequest) {
ExecutionContext executionContext = createExecutionContext(getQueryResultsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetQueryResultsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getQueryResultsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetQueryResults");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetQueryResultsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the JSON text of the resource-based policy document attached to the CloudTrail channel.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceARNNotValidException
* This exception is thrown when the provided resource does not exist, or the ARN format of the resource is
* not valid. The following is the valid format for a resource ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/MyChannel
.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws ResourcePolicyNotFoundException
* This exception is thrown when the specified resource policy is not found.
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.GetResourcePolicy
* @see AWS
* API Documentation
*/
@Override
public GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeGetResourcePolicy(request);
}
@SdkInternalApi
final GetResourcePolicyResult executeGetResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns settings information for a specified trail.
*
*
* @param getTrailRequest
* @return Result of the GetTrail operation returned by the service.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.GetTrail
* @see AWS API
* Documentation
*/
@Override
public GetTrailResult getTrail(GetTrailRequest request) {
request = beforeClientExecution(request);
return executeGetTrail(request);
}
@SdkInternalApi
final GetTrailResult executeGetTrail(GetTrailRequest getTrailRequest) {
ExecutionContext executionContext = createExecutionContext(getTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTrail");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a JSON-formatted list of information about the specified trail. Fields include information on delivery
* errors, Amazon SNS and Amazon S3 errors, and start and stop logging times for each trail. This operation returns
* trail status from a single Region. To return trail status from all Regions, you must call the operation on each
* Region.
*
*
* @param getTrailStatusRequest
* The name of a trail about which you want the current status.
* @return Result of the GetTrailStatus operation returned by the service.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.GetTrailStatus
* @see AWS API
* Documentation
*/
@Override
public GetTrailStatusResult getTrailStatus(GetTrailStatusRequest request) {
request = beforeClientExecution(request);
return executeGetTrailStatus(request);
}
@SdkInternalApi
final GetTrailStatusResult executeGetTrailStatus(GetTrailStatusRequest getTrailStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getTrailStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTrailStatusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getTrailStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTrailStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetTrailStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the channels in the current account, and their source names.
*
*
* @param listChannelsRequest
* @return Result of the ListChannels operation returned by the service.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.ListChannels
* @see AWS API
* Documentation
*/
@Override
public ListChannelsResult listChannels(ListChannelsRequest request) {
request = beforeClientExecution(request);
return executeListChannels(request);
}
@SdkInternalApi
final ListChannelsResult executeListChannels(ListChannelsRequest listChannelsRequest) {
ExecutionContext executionContext = createExecutionContext(listChannelsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListChannelsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listChannelsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListChannels");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListChannelsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about all event data stores in the account, in the current Region.
*
*
* @param listEventDataStoresRequest
* @return Result of the ListEventDataStores operation returned by the service.
* @throws InvalidMaxResultsException
* This exception is thrown if the limit specified is not valid.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.ListEventDataStores
* @see AWS
* API Documentation
*/
@Override
public ListEventDataStoresResult listEventDataStores(ListEventDataStoresRequest request) {
request = beforeClientExecution(request);
return executeListEventDataStores(request);
}
@SdkInternalApi
final ListEventDataStoresResult executeListEventDataStores(ListEventDataStoresRequest listEventDataStoresRequest) {
ExecutionContext executionContext = createExecutionContext(listEventDataStoresRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEventDataStoresRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEventDataStoresRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEventDataStores");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEventDataStoresResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of failures for the specified import.
*
*
* @param listImportFailuresRequest
* @return Result of the ListImportFailures operation returned by the service.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @sample AWSCloudTrail.ListImportFailures
* @see AWS
* API Documentation
*/
@Override
public ListImportFailuresResult listImportFailures(ListImportFailuresRequest request) {
request = beforeClientExecution(request);
return executeListImportFailures(request);
}
@SdkInternalApi
final ListImportFailuresResult executeListImportFailures(ListImportFailuresRequest listImportFailuresRequest) {
ExecutionContext executionContext = createExecutionContext(listImportFailuresRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListImportFailuresRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listImportFailuresRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListImportFailures");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListImportFailuresResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information on all imports, or a select set of imports by ImportStatus
or
* Destination
.
*
*
* @param listImportsRequest
* @return Result of the ListImports operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.ListImports
* @see AWS API
* Documentation
*/
@Override
public ListImportsResult listImports(ListImportsRequest request) {
request = beforeClientExecution(request);
return executeListImports(request);
}
@SdkInternalApi
final ListImportsResult executeListImports(ListImportsRequest listImportsRequest) {
ExecutionContext executionContext = createExecutionContext(listImportsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListImportsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listImportsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListImports");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListImportsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns Insights metrics data for trails that have enabled Insights. The request must include the
* EventSource
, EventName
, and InsightType
parameters.
*
*
* If the InsightType
is set to ApiErrorRateInsight
, the request must also include the
* ErrorCode
parameter.
*
*
* The following are the available time periods for ListInsightsMetricData
. Each cutoff is inclusive.
*
*
* -
*
* Data points with a period of 60 seconds (1-minute) are available for 15 days.
*
*
* -
*
* Data points with a period of 300 seconds (5-minute) are available for 63 days.
*
*
* -
*
* Data points with a period of 3600 seconds (1 hour) are available for 90 days.
*
*
*
*
* Access to the ListInsightsMetricData
API operation is linked to the
* cloudtrail:LookupEvents
action. To use this operation, you must have permissions to perform the
* cloudtrail:LookupEvents
action.
*
*
* @param listInsightsMetricDataRequest
* @return Result of the ListInsightsMetricData operation returned by the service.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.ListInsightsMetricData
* @see AWS API Documentation
*/
@Override
public ListInsightsMetricDataResult listInsightsMetricData(ListInsightsMetricDataRequest request) {
request = beforeClientExecution(request);
return executeListInsightsMetricData(request);
}
@SdkInternalApi
final ListInsightsMetricDataResult executeListInsightsMetricData(ListInsightsMetricDataRequest listInsightsMetricDataRequest) {
ExecutionContext executionContext = createExecutionContext(listInsightsMetricDataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListInsightsMetricDataRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listInsightsMetricDataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListInsightsMetricData");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListInsightsMetricDataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns all public keys whose private keys were used to sign the digest files within the specified time range.
* The public key is needed to validate digest files that were signed with its corresponding private key.
*
*
*
* CloudTrail uses different private and public key pairs per Region. Each digest file is signed with a private key
* unique to its Region. When you validate a digest file from a specific Region, you must look in the same Region
* for its corresponding public key.
*
*
*
* @param listPublicKeysRequest
* Requests the public keys for a specified time range.
* @return Result of the ListPublicKeys operation returned by the service.
* @throws InvalidTimeRangeException
* Occurs if the timestamp values are not valid. Either the start time occurs after the end time, or the
* time range is outside the range of possible values.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws InvalidTokenException
* Reserved for future use.
* @sample AWSCloudTrail.ListPublicKeys
* @see AWS API
* Documentation
*/
@Override
public ListPublicKeysResult listPublicKeys(ListPublicKeysRequest request) {
request = beforeClientExecution(request);
return executeListPublicKeys(request);
}
@SdkInternalApi
final ListPublicKeysResult executeListPublicKeys(ListPublicKeysRequest listPublicKeysRequest) {
ExecutionContext executionContext = createExecutionContext(listPublicKeysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPublicKeysRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPublicKeysRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPublicKeys");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPublicKeysResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListPublicKeysResult listPublicKeys() {
return listPublicKeys(new ListPublicKeysRequest());
}
/**
*
* Returns a list of queries and query statuses for the past seven days. You must specify an ARN value for
* EventDataStore
. Optionally, to shorten the list of results, you can specify a time range, formatted
* as timestamps, by adding StartTime
and EndTime
parameters, and a
* QueryStatus
value. Valid values for QueryStatus
include QUEUED
,
* RUNNING
, FINISHED
, FAILED
, TIMED_OUT
, or
* CANCELLED
.
*
*
* @param listQueriesRequest
* @return Result of the ListQueries operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidDateRangeException
* A date range for the query was specified that is not valid. Be sure that the start time is
* chronologically before the end time. For more information about writing a query, see Create or
* edit a query in the CloudTrail User Guide.
* @throws InvalidMaxResultsException
* This exception is thrown if the limit specified is not valid.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidQueryStatusException
* The query status is not valid for the operation.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.ListQueries
* @see AWS API
* Documentation
*/
@Override
public ListQueriesResult listQueries(ListQueriesRequest request) {
request = beforeClientExecution(request);
return executeListQueries(request);
}
@SdkInternalApi
final ListQueriesResult executeListQueries(ListQueriesRequest listQueriesRequest) {
ExecutionContext executionContext = createExecutionContext(listQueriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListQueriesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listQueriesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListQueries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListQueriesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags for the specified trails, event data stores, or channels in the current Region.
*
*
* @param listTagsRequest
* Specifies a list of tags to return.
* @return Result of the ListTags operation returned by the service.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws ChannelARNInvalidException
* This exception is thrown when the specified value of ChannelARN
is not valid.
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws InvalidTokenException
* Reserved for future use.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.ListTags
* @see AWS API
* Documentation
*/
@Override
public ListTagsResult listTags(ListTagsRequest request) {
request = beforeClientExecution(request);
return executeListTags(request);
}
@SdkInternalApi
final ListTagsResult executeListTags(ListTagsRequest listTagsRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists trails that are in the current account.
*
*
* @param listTrailsRequest
* @return Result of the ListTrails operation returned by the service.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.ListTrails
* @see AWS API
* Documentation
*/
@Override
public ListTrailsResult listTrails(ListTrailsRequest request) {
request = beforeClientExecution(request);
return executeListTrails(request);
}
@SdkInternalApi
final ListTrailsResult executeListTrails(ListTrailsRequest listTrailsRequest) {
ExecutionContext executionContext = createExecutionContext(listTrailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTrailsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTrailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTrails");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTrailsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Looks up management events or CloudTrail Insights events that are captured by CloudTrail. You can look up events that occurred in a Region
* within the last 90 days.
*
*
*
* LookupEvents
returns recent Insights events for trails that enable Insights. To view Insights events
* for an event data store, you can run queries on your Insights event data store, and you can also view the Lake
* dashboard for Insights.
*
*
*
* Lookup supports the following attributes for management events:
*
*
* -
*
* Amazon Web Services access key
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
* -
*
* Read only
*
*
* -
*
* Resource name
*
*
* -
*
* Resource type
*
*
* -
*
* User name
*
*
*
*
* Lookup supports the following attributes for Insights events:
*
*
* -
*
* Event ID
*
*
* -
*
* Event name
*
*
* -
*
* Event source
*
*
*
*
* All attributes are optional. The default number of results returned is 50, with a maximum of 50 possible. The
* response includes a token that you can use to get the next page of results.
*
*
*
* The rate of lookup requests is limited to two per second, per account, per Region. If this limit is exceeded, a
* throttling error occurs.
*
*
*
* @param lookupEventsRequest
* Contains a request for LookupEvents.
* @return Result of the LookupEvents operation returned by the service.
* @throws InvalidLookupAttributesException
* Occurs when a lookup attribute is specified that is not valid.
* @throws InvalidTimeRangeException
* Occurs if the timestamp values are not valid. Either the start time occurs after the end time, or the
* time range is outside the range of possible values.
* @throws InvalidMaxResultsException
* This exception is thrown if the limit specified is not valid.
* @throws InvalidNextTokenException
* A token that is not valid, or a token that was previously used in a request with different parameters.
* This exception is thrown if the token is not valid.
* @throws InvalidEventCategoryException
* Occurs if an event category that is not valid is specified as a value of EventCategory
.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.LookupEvents
* @see AWS API
* Documentation
*/
@Override
public LookupEventsResult lookupEvents(LookupEventsRequest request) {
request = beforeClientExecution(request);
return executeLookupEvents(request);
}
@SdkInternalApi
final LookupEventsResult executeLookupEvents(LookupEventsRequest lookupEventsRequest) {
ExecutionContext executionContext = createExecutionContext(lookupEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new LookupEventsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(lookupEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "LookupEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new LookupEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public LookupEventsResult lookupEvents() {
return lookupEvents(new LookupEventsRequest());
}
/**
*
* Configures an event selector or advanced event selectors for your trail. Use event selectors or advanced event
* selectors to specify management and data event settings for your trail. If you want your trail to log Insights
* events, be sure the event selector enables logging of the Insights event types you want configured for your
* trail. For more information about logging Insights events, see Logging Insights events in the CloudTrail User Guide. By default, trails created without specific
* event selectors are configured to log all read and write management events, and no data events.
*
*
* When an event occurs in your account, CloudTrail evaluates the event selectors or advanced event selectors in all
* trails. For each trail, if the event matches any event selector, the trail processes and logs the event. If the
* event doesn't match any event selector, the trail doesn't log the event.
*
*
* Example
*
*
* -
*
* You create an event selector for a trail and specify that you want write-only events.
*
*
* -
*
* The EC2 GetConsoleOutput
and RunInstances
API operations occur in your account.
*
*
* -
*
* CloudTrail evaluates whether the events match your event selectors.
*
*
* -
*
* The RunInstances
is a write-only event and it matches your event selector. The trail logs the event.
*
*
* -
*
* The GetConsoleOutput
is a read-only event that doesn't match your event selector. The trail doesn't
* log the event.
*
*
*
*
* The PutEventSelectors
operation must be called from the Region in which the trail was created;
* otherwise, an InvalidHomeRegionException
exception is thrown.
*
*
* You can configure up to five event selectors for each trail. For more information, see Logging management events, Logging data events, and Quotas in
* CloudTrail in the CloudTrail User Guide.
*
*
* You can add advanced event selectors, and conditions for your advanced event selectors, up to a maximum of 500
* values for all conditions and selectors on a trail. You can use either AdvancedEventSelectors
or
* EventSelectors
, but not both. If you apply AdvancedEventSelectors
to a trail, any
* existing EventSelectors
are overwritten. For more information about advanced event selectors, see Logging
* data events in the CloudTrail User Guide.
*
*
* @param putEventSelectorsRequest
* @return Result of the PutEventSelectors operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a Region other than the Region in
* which the trail was created.
* @throws InvalidEventSelectorsException
* This exception is thrown when the PutEventSelectors
operation is called with a number of
* event selectors, advanced event selectors, or data resources that is not valid. The combination of event
* selectors or advanced event selectors and data resources is not valid. A trail can have up to 5 event
* selectors. If a trail uses advanced event selectors, a maximum of 500 total values for all conditions in
* all advanced event selectors is allowed. A trail is limited to 250 data resources. These data resources
* can be distributed across event selectors, but the overall total cannot exceed 250.
*
*
* You can:
*
*
* -
*
* Specify a valid number of event selectors (1 to 5) for a trail.
*
*
* -
*
* Specify a valid number of data resources (1 to 250) for an event selector. The limit of number of
* resources on an individual event selector is configurable up to 250. However, this upper limit is allowed
* only if the total number of data resources does not exceed 250 across all event selectors for a trail.
*
*
* -
*
* Specify up to 500 values for all conditions in all advanced event selectors for a trail.
*
*
* -
*
* Specify a valid value for a parameter. For example, specifying the ReadWriteType
parameter
* with a value of read-only
is not valid.
*
*
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @sample AWSCloudTrail.PutEventSelectors
* @see AWS
* API Documentation
*/
@Override
public PutEventSelectorsResult putEventSelectors(PutEventSelectorsRequest request) {
request = beforeClientExecution(request);
return executePutEventSelectors(request);
}
@SdkInternalApi
final PutEventSelectorsResult executePutEventSelectors(PutEventSelectorsRequest putEventSelectorsRequest) {
ExecutionContext executionContext = createExecutionContext(putEventSelectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutEventSelectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putEventSelectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutEventSelectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutEventSelectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lets you enable Insights event logging by specifying the Insights selectors that you want to enable on an
* existing trail or event data store. You also use PutInsightSelectors
to turn off Insights event
* logging, by passing an empty list of Insights types. The valid Insights event types are
* ApiErrorRateInsight
and ApiCallRateInsight
.
*
*
* To enable Insights on an event data store, you must specify the ARNs (or ID suffix of the ARNs) for the source
* event data store (EventDataStore
) and the destination event data store (
* InsightsDestination
). The source event data store logs management events and enables Insights. The
* destination event data store logs Insights events based upon the management event activity of the source event
* data store. The source and destination event data stores must belong to the same Amazon Web Services account.
*
*
* To log Insights events for a trail, you must specify the name (TrailName
) of the CloudTrail trail
* for which you want to change or add Insights selectors.
*
*
* To log CloudTrail Insights events on API call volume, the trail or event data store must log write
* management events. To log CloudTrail Insights events on API error rate, the trail or event data store must log
* read
or write
management events. You can call GetEventSelectors
on a trail
* to check whether the trail logs management events. You can call GetEventDataStore
on an event data
* store to check whether the event data store logs management events.
*
*
* For more information, see Logging CloudTrail Insights events in the CloudTrail User Guide.
*
*
* @param putInsightSelectorsRequest
* @return Result of the PutInsightSelectors operation returned by the service.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidParameterCombinationException
* This exception is thrown when the combination of parameters provided is not valid.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a Region other than the Region in
* which the trail was created.
* @throws InvalidInsightSelectorsException
* For PutInsightSelectors
, this exception is thrown when the formatting or syntax of the
* InsightSelectors
JSON statement is not valid, or the specified InsightType
in
* the InsightSelectors
statement is not valid. Valid values for InsightType
are
* ApiCallRateInsight
and ApiErrorRateInsight
. To enable Insights on an event data
* store, the destination event data store specified by the InsightsDestination
parameter must
* log Insights events and the source event data store specified by the EventDataStore
* parameter must log management events.
*
*
* For UpdateEventDataStore
, this exception is thrown if Insights are enabled on the event data
* store and the updated advanced event selectors are not compatible with the configured
* InsightSelectors
. If the InsightSelectors
includes an InsightType
of
* ApiCallRateInsight
, the source event data store must log write
management
* events. If the InsightSelectors
includes an InsightType
of
* ApiErrorRateInsight
, the source event data store must log management events.
* @throws InsufficientS3BucketPolicyException
* This exception is thrown when the policy on the S3 bucket is not sufficient.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws S3BucketDoesNotExistException
* This exception is thrown when the specified S3 bucket does not exist.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail or event data
* store can't be updated.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @sample AWSCloudTrail.PutInsightSelectors
* @see AWS
* API Documentation
*/
@Override
public PutInsightSelectorsResult putInsightSelectors(PutInsightSelectorsRequest request) {
request = beforeClientExecution(request);
return executePutInsightSelectors(request);
}
@SdkInternalApi
final PutInsightSelectorsResult executePutInsightSelectors(PutInsightSelectorsRequest putInsightSelectorsRequest) {
ExecutionContext executionContext = createExecutionContext(putInsightSelectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutInsightSelectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putInsightSelectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutInsightSelectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutInsightSelectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches a resource-based permission policy to a CloudTrail channel that is used for an integration with an event
* source outside of Amazon Web Services. For more information about resource-based policies, see CloudTrail resource-based policy examples in the CloudTrail User Guide.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ResourceARNNotValidException
* This exception is thrown when the provided resource does not exist, or the ARN format of the resource is
* not valid. The following is the valid format for a resource ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/MyChannel
.
* @throws ResourcePolicyNotValidException
* This exception is thrown when the resouce-based policy has syntax errors, or contains a principal that is
* not valid.
*
* The following are requirements for the resource policy:
*
*
* -
*
* Contains only one action: cloudtrail-data:PutAuditEvents
*
*
* -
*
* Contains at least one statement. The policy can have a maximum of 20 statements.
*
*
* -
*
* Each statement contains at least one principal. A statement can have a maximum of 50 principals.
*
*
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.PutResourcePolicy
* @see AWS
* API Documentation
*/
@Override
public PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executePutResourcePolicy(request);
}
@SdkInternalApi
final PutResourcePolicyResult executePutResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers an organization’s member account as the CloudTrail delegated administrator.
*
*
* @param registerOrganizationDelegatedAdminRequest
* Specifies an organization member account ID as a CloudTrail delegated administrator.
* @return Result of the RegisterOrganizationDelegatedAdmin operation returned by the service.
* @throws AccountRegisteredException
* This exception is thrown when the account is already registered as the CloudTrail delegated
* administrator.
* @throws AccountNotFoundException
* This exception is thrown when the specified account is not found or not part of an organization.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws CannotDelegateManagementAccountException
* This exception is thrown when the management account of an organization is registered as the CloudTrail
* delegated administrator.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws DelegatedAdminAccountLimitExceededException
* This exception is thrown when the maximum number of CloudTrail delegated administrators is reached.
* @throws NotOrganizationManagementAccountException
* This exception is thrown when the account making the request is not the organization's management
* account.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @sample AWSCloudTrail.RegisterOrganizationDelegatedAdmin
* @see AWS API Documentation
*/
@Override
public RegisterOrganizationDelegatedAdminResult registerOrganizationDelegatedAdmin(RegisterOrganizationDelegatedAdminRequest request) {
request = beforeClientExecution(request);
return executeRegisterOrganizationDelegatedAdmin(request);
}
@SdkInternalApi
final RegisterOrganizationDelegatedAdminResult executeRegisterOrganizationDelegatedAdmin(
RegisterOrganizationDelegatedAdminRequest registerOrganizationDelegatedAdminRequest) {
ExecutionContext executionContext = createExecutionContext(registerOrganizationDelegatedAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterOrganizationDelegatedAdminRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(registerOrganizationDelegatedAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterOrganizationDelegatedAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RegisterOrganizationDelegatedAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified tags from a trail, event data store, or channel.
*
*
* @param removeTagsRequest
* Specifies the tags to remove from a trail, event data store, or channel.
* @return Result of the RemoveTags operation returned by the service.
* @throws ResourceNotFoundException
* This exception is thrown when the specified resource is not found.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws ChannelARNInvalidException
* This exception is thrown when the specified value of ChannelARN
is not valid.
* @throws ResourceTypeNotSupportedException
* This exception is thrown when the specified resource type is not supported by CloudTrail.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidTagParameterException
* This exception is thrown when the specified tag key or values are not valid. It can also occur if there
* are duplicate tags or too many tags on the resource.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws ChannelNotFoundException
* This exception is thrown when CloudTrail cannot find the specified channel.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.RemoveTags
* @see AWS API
* Documentation
*/
@Override
public RemoveTagsResult removeTags(RemoveTagsRequest request) {
request = beforeClientExecution(request);
return executeRemoveTags(request);
}
@SdkInternalApi
final RemoveTagsResult executeRemoveTags(RemoveTagsRequest removeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(removeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemoveTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Restores a deleted event data store specified by EventDataStore
, which accepts an event data store
* ARN. You can only restore a deleted event data store within the seven-day wait period after deletion. Restoring
* an event data store can take several minutes, depending on the size of the event data store.
*
*
* @param restoreEventDataStoreRequest
* @return Result of the RestoreEventDataStore operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws EventDataStoreMaxLimitExceededException
* Your account has used the maximum number of event data stores.
* @throws InvalidEventDataStoreStatusException
* The event data store is not in a status that supports the operation.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @sample AWSCloudTrail.RestoreEventDataStore
* @see AWS API Documentation
*/
@Override
public RestoreEventDataStoreResult restoreEventDataStore(RestoreEventDataStoreRequest request) {
request = beforeClientExecution(request);
return executeRestoreEventDataStore(request);
}
@SdkInternalApi
final RestoreEventDataStoreResult executeRestoreEventDataStore(RestoreEventDataStoreRequest restoreEventDataStoreRequest) {
ExecutionContext executionContext = createExecutionContext(restoreEventDataStoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RestoreEventDataStoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(restoreEventDataStoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RestoreEventDataStore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RestoreEventDataStoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts the ingestion of live events on an event data store specified as either an ARN or the ID portion of the
* ARN. To start ingestion, the event data store Status
must be STOPPED_INGESTION
and the
* eventCategory
must be Management
, Data
, or ConfigurationItem
.
*
*
* @param startEventDataStoreIngestionRequest
* @return Result of the StartEventDataStoreIngestion operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidEventDataStoreStatusException
* The event data store is not in a status that supports the operation.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidEventDataStoreCategoryException
* This exception is thrown when event categories of specified event data stores are not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @sample AWSCloudTrail.StartEventDataStoreIngestion
* @see AWS API Documentation
*/
@Override
public StartEventDataStoreIngestionResult startEventDataStoreIngestion(StartEventDataStoreIngestionRequest request) {
request = beforeClientExecution(request);
return executeStartEventDataStoreIngestion(request);
}
@SdkInternalApi
final StartEventDataStoreIngestionResult executeStartEventDataStoreIngestion(StartEventDataStoreIngestionRequest startEventDataStoreIngestionRequest) {
ExecutionContext executionContext = createExecutionContext(startEventDataStoreIngestionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartEventDataStoreIngestionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startEventDataStoreIngestionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartEventDataStoreIngestion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartEventDataStoreIngestionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts an import of logged trail events from a source S3 bucket to a destination event data store. By default,
* CloudTrail only imports events contained in the S3 bucket's CloudTrail
prefix and the prefixes
* inside the CloudTrail
prefix, and does not check prefixes for other Amazon Web Services services. If
* you want to import CloudTrail events contained in another prefix, you must include the prefix in the
* S3LocationUri
. For more considerations about importing trail events, see Considerations for copying trail events in the CloudTrail User Guide.
*
*
* When you start a new import, the Destinations
and ImportSource
parameters are required.
* Before starting a new import, disable any access control lists (ACLs) attached to the source S3 bucket. For more
* information about disabling ACLs, see Controlling ownership of
* objects and disabling ACLs for your bucket.
*
*
* When you retry an import, the ImportID
parameter is required.
*
*
*
* If the destination event data store is for an organization, you must use the management account to import trail
* events. You cannot use the delegated administrator account for the organization.
*
*
*
* @param startImportRequest
* @return Result of the StartImport operation returned by the service.
* @throws AccountHasOngoingImportException
* This exception is thrown when you start a new import and a previous import is still in progress.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidEventDataStoreStatusException
* The event data store is not in a status that supports the operation.
* @throws InvalidEventDataStoreCategoryException
* This exception is thrown when event categories of specified event data stores are not valid.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidImportSourceException
* This exception is thrown when the provided source S3 bucket is not valid for import.
* @throws ImportNotFoundException
* The specified import was not found.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.StartImport
* @see AWS API
* Documentation
*/
@Override
public StartImportResult startImport(StartImportRequest request) {
request = beforeClientExecution(request);
return executeStartImport(request);
}
@SdkInternalApi
final StartImportResult executeStartImport(StartImportRequest startImportRequest) {
ExecutionContext executionContext = createExecutionContext(startImportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartImportRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startImportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartImport");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartImportResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts the recording of Amazon Web Services API calls and log file delivery for a trail. For a trail that is
* enabled in all Regions, this operation must be called from the Region in which the trail was created. This
* operation cannot be called on the shadow trails (replicated trails in other Regions) of a trail that is enabled
* in all Regions.
*
*
* @param startLoggingRequest
* The request to CloudTrail to start logging Amazon Web Services API calls for an account.
* @return Result of the StartLogging operation returned by the service.
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a Region other than the Region in
* which the trail was created.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @sample AWSCloudTrail.StartLogging
* @see AWS API
* Documentation
*/
@Override
public StartLoggingResult startLogging(StartLoggingRequest request) {
request = beforeClientExecution(request);
return executeStartLogging(request);
}
@SdkInternalApi
final StartLoggingResult executeStartLogging(StartLoggingRequest startLoggingRequest) {
ExecutionContext executionContext = createExecutionContext(startLoggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartLoggingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startLoggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartLogging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartLoggingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a CloudTrail Lake query. Use the QueryStatement
parameter to provide your SQL query, enclosed
* in single quotation marks. Use the optional DeliveryS3Uri
parameter to deliver the query results to
* an S3 bucket.
*
*
* StartQuery
requires you specify either the QueryStatement
parameter, or a
* QueryAlias
and any QueryParameters
. In the current release, the QueryAlias
* and QueryParameters
parameters are used only for the queries that populate the CloudTrail Lake
* dashboards.
*
*
* @param startQueryRequest
* @return Result of the StartQuery operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidQueryStatementException
* The query that was submitted has validation errors, or uses incorrect syntax or unsupported keywords. For
* more information about writing a query, see Create or
* edit a query in the CloudTrail User Guide.
* @throws MaxConcurrentQueriesException
* You are already running the maximum number of concurrent queries. The maximum number of concurrent
* queries is 10. Wait a minute for some queries to finish, and then run the query again.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws InvalidS3PrefixException
* This exception is thrown when the provided S3 prefix is not valid.
* @throws InvalidS3BucketNameException
* This exception is thrown when the provided S3 bucket name is not valid.
* @throws InsufficientS3BucketPolicyException
* This exception is thrown when the policy on the S3 bucket is not sufficient.
* @throws S3BucketDoesNotExistException
* This exception is thrown when the specified S3 bucket does not exist.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @sample AWSCloudTrail.StartQuery
* @see AWS API
* Documentation
*/
@Override
public StartQueryResult startQuery(StartQueryRequest request) {
request = beforeClientExecution(request);
return executeStartQuery(request);
}
@SdkInternalApi
final StartQueryResult executeStartQuery(StartQueryRequest startQueryRequest) {
ExecutionContext executionContext = createExecutionContext(startQueryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartQueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startQueryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartQuery");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartQueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops the ingestion of live events on an event data store specified as either an ARN or the ID portion of the
* ARN. To stop ingestion, the event data store Status
must be ENABLED
and the
* eventCategory
must be Management
, Data
, or ConfigurationItem
.
*
*
* @param stopEventDataStoreIngestionRequest
* @return Result of the StopEventDataStoreIngestion operation returned by the service.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidEventDataStoreStatusException
* The event data store is not in a status that supports the operation.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws InvalidEventDataStoreCategoryException
* This exception is thrown when event categories of specified event data stores are not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @sample AWSCloudTrail.StopEventDataStoreIngestion
* @see AWS API Documentation
*/
@Override
public StopEventDataStoreIngestionResult stopEventDataStoreIngestion(StopEventDataStoreIngestionRequest request) {
request = beforeClientExecution(request);
return executeStopEventDataStoreIngestion(request);
}
@SdkInternalApi
final StopEventDataStoreIngestionResult executeStopEventDataStoreIngestion(StopEventDataStoreIngestionRequest stopEventDataStoreIngestionRequest) {
ExecutionContext executionContext = createExecutionContext(stopEventDataStoreIngestionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopEventDataStoreIngestionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(stopEventDataStoreIngestionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopEventDataStoreIngestion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopEventDataStoreIngestionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a specified import.
*
*
* @param stopImportRequest
* @return Result of the StopImport operation returned by the service.
* @throws ImportNotFoundException
* The specified import was not found.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.StopImport
* @see AWS API
* Documentation
*/
@Override
public StopImportResult stopImport(StopImportRequest request) {
request = beforeClientExecution(request);
return executeStopImport(request);
}
@SdkInternalApi
final StopImportResult executeStopImport(StopImportRequest stopImportRequest) {
ExecutionContext executionContext = createExecutionContext(stopImportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopImportRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopImportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopImport");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopImportResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Suspends the recording of Amazon Web Services API calls and log file delivery for the specified trail. Under most
* circumstances, there is no need to use this action. You can update a trail without stopping it first. This action
* is the only way to stop recording. For a trail enabled in all Regions, this operation must be called from the
* Region in which the trail was created, or an InvalidHomeRegionException
will occur. This operation
* cannot be called on the shadow trails (replicated trails in other Regions) of a trail enabled in all Regions.
*
*
* @param stopLoggingRequest
* Passes the request to CloudTrail to stop logging Amazon Web Services API calls for the specified account.
* @return Result of the StopLogging operation returned by the service.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a Region other than the Region in
* which the trail was created.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @sample AWSCloudTrail.StopLogging
* @see AWS API
* Documentation
*/
@Override
public StopLoggingResult stopLogging(StopLoggingRequest request) {
request = beforeClientExecution(request);
return executeStopLogging(request);
}
@SdkInternalApi
final StopLoggingResult executeStopLogging(StopLoggingRequest stopLoggingRequest) {
ExecutionContext executionContext = createExecutionContext(stopLoggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopLoggingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopLoggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopLogging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopLoggingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a channel specified by a required channel ARN or UUID.
*
*
* @param updateChannelRequest
* @return Result of the UpdateChannel operation returned by the service.
* @throws ChannelARNInvalidException
* This exception is thrown when the specified value of ChannelARN
is not valid.
* @throws ChannelNotFoundException
* This exception is thrown when CloudTrail cannot find the specified channel.
* @throws ChannelAlreadyExistsException
* This exception is thrown when the provided channel already exists.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidEventDataStoreCategoryException
* This exception is thrown when event categories of specified event data stores are not valid.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @sample AWSCloudTrail.UpdateChannel
* @see AWS API
* Documentation
*/
@Override
public UpdateChannelResult updateChannel(UpdateChannelRequest request) {
request = beforeClientExecution(request);
return executeUpdateChannel(request);
}
@SdkInternalApi
final UpdateChannelResult executeUpdateChannel(UpdateChannelRequest updateChannelRequest) {
ExecutionContext executionContext = createExecutionContext(updateChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an event data store. The required EventDataStore
value is an ARN or the ID portion of the
* ARN. Other parameters are optional, but at least one optional parameter must be specified, or CloudTrail throws
* an error. RetentionPeriod
is in days, and valid values are integers between 7 and 3653 if the
* BillingMode
is set to EXTENDABLE_RETENTION_PRICING
, or between 7 and 2557 if
* BillingMode
is set to FIXED_RETENTION_PRICING
. By default,
* TerminationProtection
is enabled.
*
*
* For event data stores for CloudTrail events, AdvancedEventSelectors
includes or excludes management
* or data events in your event data store. For more information about AdvancedEventSelectors
, see
* AdvancedEventSelectors.
*
*
* For event data stores for CloudTrail Insights events, Config configuration items, Audit Manager evidence, or
* non-Amazon Web Services events, AdvancedEventSelectors
includes events of that type in your event
* data store.
*
*
* @param updateEventDataStoreRequest
* @return Result of the UpdateEventDataStore operation returned by the service.
* @throws EventDataStoreAlreadyExistsException
* An event data store with that name already exists.
* @throws EventDataStoreARNInvalidException
* The specified event data store ARN is not valid or does not map to an event data store in your account.
* @throws EventDataStoreNotFoundException
* The specified event data store was not found.
* @throws InvalidEventSelectorsException
* This exception is thrown when the PutEventSelectors
operation is called with a number of
* event selectors, advanced event selectors, or data resources that is not valid. The combination of event
* selectors or advanced event selectors and data resources is not valid. A trail can have up to 5 event
* selectors. If a trail uses advanced event selectors, a maximum of 500 total values for all conditions in
* all advanced event selectors is allowed. A trail is limited to 250 data resources. These data resources
* can be distributed across event selectors, but the overall total cannot exceed 250.
*
* You can:
*
*
* -
*
* Specify a valid number of event selectors (1 to 5) for a trail.
*
*
* -
*
* Specify a valid number of data resources (1 to 250) for an event selector. The limit of number of
* resources on an individual event selector is configurable up to 250. However, this upper limit is allowed
* only if the total number of data resources does not exceed 250 across all event selectors for a trail.
*
*
* -
*
* Specify up to 500 values for all conditions in all advanced event selectors for a trail.
*
*
* -
*
* Specify a valid value for a parameter. For example, specifying the ReadWriteType
parameter
* with a value of read-only
is not valid.
*
*
* @throws InvalidInsightSelectorsException
* For PutInsightSelectors
, this exception is thrown when the formatting or syntax of the
* InsightSelectors
JSON statement is not valid, or the specified InsightType
in
* the InsightSelectors
statement is not valid. Valid values for InsightType
are
* ApiCallRateInsight
and ApiErrorRateInsight
. To enable Insights on an event data
* store, the destination event data store specified by the InsightsDestination
parameter must
* log Insights events and the source event data store specified by the EventDataStore
* parameter must log management events.
*
* For UpdateEventDataStore
, this exception is thrown if Insights are enabled on the event data
* store and the updated advanced event selectors are not compatible with the configured
* InsightSelectors
. If the InsightSelectors
includes an InsightType
of
* ApiCallRateInsight
, the source event data store must log write
management
* events. If the InsightSelectors
includes an InsightType
of
* ApiErrorRateInsight
, the source event data store must log management events.
* @throws EventDataStoreHasOngoingImportException
* This exception is thrown when you try to update or delete an event data store that currently has an
* import in progress.
* @throws InactiveEventDataStoreException
* The event data store is inactive.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws InvalidKmsKeyIdException
* This exception is thrown when the KMS key ARN is not valid.
* @throws KmsKeyNotFoundException
* This exception is thrown when the KMS key does not exist, when the S3 bucket and the KMS key are not in
* the same Region, or when the KMS key associated with the Amazon SNS topic either does not exist or is not
* in the same Region.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail or event data
* store can't be updated.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @sample AWSCloudTrail.UpdateEventDataStore
* @see AWS API Documentation
*/
@Override
public UpdateEventDataStoreResult updateEventDataStore(UpdateEventDataStoreRequest request) {
request = beforeClientExecution(request);
return executeUpdateEventDataStore(request);
}
@SdkInternalApi
final UpdateEventDataStoreResult executeUpdateEventDataStore(UpdateEventDataStoreRequest updateEventDataStoreRequest) {
ExecutionContext executionContext = createExecutionContext(updateEventDataStoreRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateEventDataStoreRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateEventDataStoreRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateEventDataStore");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateEventDataStoreResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates trail settings that control what events you are logging, and how to handle log files. Changes to a trail
* do not require stopping the CloudTrail service. Use this action to designate an existing bucket for log delivery.
* If the existing bucket has previously been a target for CloudTrail log files, an IAM policy exists for the
* bucket. UpdateTrail
must be called from the Region in which the trail was created; otherwise, an
* InvalidHomeRegionException
is thrown.
*
*
* @param updateTrailRequest
* Specifies settings to update for the trail.
* @return Result of the UpdateTrail operation returned by the service.
* @throws S3BucketDoesNotExistException
* This exception is thrown when the specified S3 bucket does not exist.
* @throws InsufficientS3BucketPolicyException
* This exception is thrown when the policy on the S3 bucket is not sufficient.
* @throws InsufficientSnsTopicPolicyException
* This exception is thrown when the policy on the Amazon SNS topic is not sufficient.
* @throws InsufficientEncryptionPolicyException
* This exception is thrown when the policy on the S3 bucket or KMS key does not have sufficient permissions
* for the operation.
* @throws TrailNotFoundException
* This exception is thrown when the trail with the given name is not found.
* @throws InvalidS3BucketNameException
* This exception is thrown when the provided S3 bucket name is not valid.
* @throws InvalidS3PrefixException
* This exception is thrown when the provided S3 prefix is not valid.
* @throws InvalidSnsTopicNameException
* This exception is thrown when the provided SNS topic name is not valid.
* @throws InvalidKmsKeyIdException
* This exception is thrown when the KMS key ARN is not valid.
* @throws InvalidTrailNameException
* This exception is thrown when the provided trail name is not valid. Trail names must meet the following
* requirements:
*
* -
*
* Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
*
*
* -
*
* Start with a letter or number, and end with a letter or number
*
*
* -
*
* Be between 3 and 128 characters
*
*
* -
*
* Have no adjacent periods, underscores or dashes. Names like my-_namespace
and
* my--namespace
are not valid.
*
*
* -
*
* Not be in IP address format (for example, 192.168.5.4)
*
*
* @throws TrailNotProvidedException
* This exception is no longer in use.
* @throws InvalidEventSelectorsException
* This exception is thrown when the PutEventSelectors
operation is called with a number of
* event selectors, advanced event selectors, or data resources that is not valid. The combination of event
* selectors or advanced event selectors and data resources is not valid. A trail can have up to 5 event
* selectors. If a trail uses advanced event selectors, a maximum of 500 total values for all conditions in
* all advanced event selectors is allowed. A trail is limited to 250 data resources. These data resources
* can be distributed across event selectors, but the overall total cannot exceed 250.
*
* You can:
*
*
* -
*
* Specify a valid number of event selectors (1 to 5) for a trail.
*
*
* -
*
* Specify a valid number of data resources (1 to 250) for an event selector. The limit of number of
* resources on an individual event selector is configurable up to 250. However, this upper limit is allowed
* only if the total number of data resources does not exceed 250 across all event selectors for a trail.
*
*
* -
*
* Specify up to 500 values for all conditions in all advanced event selectors for a trail.
*
*
* -
*
* Specify a valid value for a parameter. For example, specifying the ReadWriteType
parameter
* with a value of read-only
is not valid.
*
*
* @throws CloudTrailARNInvalidException
* This exception is thrown when an operation is called with an ARN that is not valid.
*
* The following is the format of a trail ARN: arn:aws:cloudtrail:us-east-2:123456789012:trail/MyTrail
*
*
*
* The following is the format of an event data store ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:eventdatastore/EXAMPLE-f852-4e8f-8bd1-bcf6cEXAMPLE
*
*
* The following is the format of a channel ARN:
* arn:aws:cloudtrail:us-east-2:123456789012:channel/01234567890
* @throws ConflictException
* This exception is thrown when the specified resource is not ready for an operation. This can occur when
* you try to run an operation on a resource before CloudTrail has time to fully load the resource, or
* because another operation is modifying the resource. If this exception occurs, wait a few minutes, and
* then try the operation again.
* @throws ThrottlingException
* This exception is thrown when the request rate exceeds the limit.
* @throws InvalidParameterCombinationException
* This exception is thrown when the combination of parameters provided is not valid.
* @throws InvalidHomeRegionException
* This exception is thrown when an operation is called on a trail from a Region other than the Region in
* which the trail was created.
* @throws KmsKeyNotFoundException
* This exception is thrown when the KMS key does not exist, when the S3 bucket and the KMS key are not in
* the same Region, or when the KMS key associated with the Amazon SNS topic either does not exist or is not
* in the same Region.
* @throws KmsKeyDisabledException
* This exception is no longer in use.
* @throws KmsException
* This exception is thrown when there is an issue with the specified KMS key and the trail or event data
* store can't be updated.
* @throws InvalidCloudWatchLogsLogGroupArnException
* This exception is thrown when the provided CloudWatch Logs log group is not valid.
* @throws InvalidCloudWatchLogsRoleArnException
* This exception is thrown when the provided role is not valid.
* @throws CloudWatchLogsDeliveryUnavailableException
* Cannot set a CloudWatch Logs delivery for this Region.
* @throws UnsupportedOperationException
* This exception is thrown when the requested operation is not supported.
* @throws OperationNotPermittedException
* This exception is thrown when the requested operation is not permitted.
* @throws CloudTrailAccessNotEnabledException
* This exception is thrown when trusted access has not been enabled between CloudTrail and Organizations.
* For more information, see How to enable or disable trusted access in the Organizations User Guide and Prepare For Creating a Trail For Your Organization in the CloudTrail User Guide.
* @throws InsufficientDependencyServiceAccessPermissionException
* This exception is thrown when the IAM identity that is used to create the organization resource lacks one
* or more required permissions for creating an organization resource in a required service.
* @throws OrganizationsNotInUseException
* This exception is thrown when the request is made from an Amazon Web Services account that is not a
* member of an organization. To make this request, sign in using the credentials of an account that belongs
* to an organization.
* @throws NotOrganizationMasterAccountException
* This exception is thrown when the Amazon Web Services account making the request to create or update an
* organization trail or event data store is not the management account for an organization in
* Organizations. For more information, see Prepare For Creating a Trail For Your Organization or Organization event data stores.
* @throws OrganizationNotInAllFeaturesModeException
* This exception is thrown when Organizations is not configured to support all features. All features must
* be enabled in Organizations to support creating an organization trail or event data store.
* @throws NoManagementAccountSLRExistsException
* This exception is thrown when the management account does not have a service-linked role.
* @throws CloudTrailInvalidClientTokenIdException
* This exception is thrown when a call results in the InvalidClientTokenId
error code. This
* can occur when you are creating or updating a trail to send notifications to an Amazon SNS topic that is
* in a suspended Amazon Web Services account.
* @throws InvalidParameterException
* The request includes a parameter that is not valid.
* @sample AWSCloudTrail.UpdateTrail
* @see AWS API
* Documentation
*/
@Override
public UpdateTrailResult updateTrail(UpdateTrailRequest request) {
request = beforeClientExecution(request);
return executeUpdateTrail(request);
}
@SdkInternalApi
final UpdateTrailResult executeUpdateTrail(UpdateTrailRequest updateTrailRequest) {
ExecutionContext executionContext = createExecutionContext(updateTrailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateTrailRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateTrailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudTrail");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateTrail");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateTrailResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}