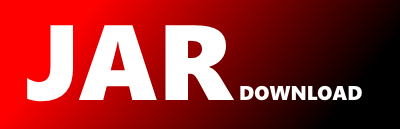
com.amazonaws.services.cloudtrail.model.ListInsightsMetricDataRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListInsightsMetricDataRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
*
*/
private String eventSource;
/**
*
* The name of the event, typically the Amazon Web Services API on which unusual levels of activity were recorded.
*
*/
private String eventName;
/**
*
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
*
*/
private String insightType;
/**
*
* Conditionally required if the InsightType
parameter is set to ApiErrorRateInsight
.
*
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to retrieve data
* for. For example, AccessDenied
.
*
*/
private String errorCode;
/**
*
* Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include data
* points with the specified time stamp.
*
*
* The default is 90 days before the time of request.
*
*/
private java.util.Date startTime;
/**
*
* Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include data
* points up to the specified time stamp.
*
*
* The default is the time of request.
*
*/
private java.util.Date endTime;
/**
*
* Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
*
*/
private Integer period;
/**
*
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
. The
* default is NonZeroData
.
*
*/
private String dataType;
/**
*
* The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value is
* 21600.
*
*/
private Integer maxResults;
/**
*
* Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
*
*/
private String nextToken;
/**
*
* The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
*
*
* @param eventSource
* The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
*/
public void setEventSource(String eventSource) {
this.eventSource = eventSource;
}
/**
*
* The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
*
*
* @return The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
*/
public String getEventSource() {
return this.eventSource;
}
/**
*
* The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
*
*
* @param eventSource
* The Amazon Web Services service to which the request was made, such as iam.amazonaws.com
or
* s3.amazonaws.com
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withEventSource(String eventSource) {
setEventSource(eventSource);
return this;
}
/**
*
* The name of the event, typically the Amazon Web Services API on which unusual levels of activity were recorded.
*
*
* @param eventName
* The name of the event, typically the Amazon Web Services API on which unusual levels of activity were
* recorded.
*/
public void setEventName(String eventName) {
this.eventName = eventName;
}
/**
*
* The name of the event, typically the Amazon Web Services API on which unusual levels of activity were recorded.
*
*
* @return The name of the event, typically the Amazon Web Services API on which unusual levels of activity were
* recorded.
*/
public String getEventName() {
return this.eventName;
}
/**
*
* The name of the event, typically the Amazon Web Services API on which unusual levels of activity were recorded.
*
*
* @param eventName
* The name of the event, typically the Amazon Web Services API on which unusual levels of activity were
* recorded.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withEventName(String eventName) {
setEventName(eventName);
return this;
}
/**
*
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
*
*
* @param insightType
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
* @see InsightType
*/
public void setInsightType(String insightType) {
this.insightType = insightType;
}
/**
*
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
*
*
* @return The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
* @see InsightType
*/
public String getInsightType() {
return this.insightType;
}
/**
*
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
*
*
* @param insightType
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InsightType
*/
public ListInsightsMetricDataRequest withInsightType(String insightType) {
setInsightType(insightType);
return this;
}
/**
*
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
*
*
* @param insightType
* The type of CloudTrail Insights event, which is either ApiCallRateInsight
or
* ApiErrorRateInsight
. The ApiCallRateInsight
Insights type analyzes write-only
* management API calls that are aggregated per minute against a baseline API call volume. The
* ApiErrorRateInsight
Insights type analyzes management API calls that result in error codes.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InsightType
*/
public ListInsightsMetricDataRequest withInsightType(InsightType insightType) {
this.insightType = insightType.toString();
return this;
}
/**
*
* Conditionally required if the InsightType
parameter is set to ApiErrorRateInsight
.
*
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to retrieve data
* for. For example, AccessDenied
.
*
*
* @param errorCode
* Conditionally required if the InsightType
parameter is set to
* ApiErrorRateInsight
.
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to retrieve
* data for. For example, AccessDenied
.
*/
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
/**
*
* Conditionally required if the InsightType
parameter is set to ApiErrorRateInsight
.
*
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to retrieve data
* for. For example, AccessDenied
.
*
*
* @return Conditionally required if the InsightType
parameter is set to
* ApiErrorRateInsight
.
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to
* retrieve data for. For example, AccessDenied
.
*/
public String getErrorCode() {
return this.errorCode;
}
/**
*
* Conditionally required if the InsightType
parameter is set to ApiErrorRateInsight
.
*
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to retrieve data
* for. For example, AccessDenied
.
*
*
* @param errorCode
* Conditionally required if the InsightType
parameter is set to
* ApiErrorRateInsight
.
*
* If returning metrics for the ApiErrorRateInsight
Insights type, this is the error to retrieve
* data for. For example, AccessDenied
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withErrorCode(String errorCode) {
setErrorCode(errorCode);
return this;
}
/**
*
* Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include data
* points with the specified time stamp.
*
*
* The default is 90 days before the time of request.
*
*
* @param startTime
* Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include
* data points with the specified time stamp.
*
* The default is 90 days before the time of request.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include data
* points with the specified time stamp.
*
*
* The default is 90 days before the time of request.
*
*
* @return Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include
* data points with the specified time stamp.
*
* The default is 90 days before the time of request.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include data
* points with the specified time stamp.
*
*
* The default is 90 days before the time of request.
*
*
* @param startTime
* Specifies, in UTC, the start time for time-series data. The value specified is inclusive; results include
* data points with the specified time stamp.
*
* The default is 90 days before the time of request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include data
* points up to the specified time stamp.
*
*
* The default is the time of request.
*
*
* @param endTime
* Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include
* data points up to the specified time stamp.
*
* The default is the time of request.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include data
* points up to the specified time stamp.
*
*
* The default is the time of request.
*
*
* @return Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include
* data points up to the specified time stamp.
*
* The default is the time of request.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include data
* points up to the specified time stamp.
*
*
* The default is the time of request.
*
*
* @param endTime
* Specifies, in UTC, the end time for time-series data. The value specified is exclusive; results include
* data points up to the specified time stamp.
*
* The default is the time of request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
*
*
* @param period
* Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
*/
public void setPeriod(Integer period) {
this.period = period;
}
/**
*
* Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
*
*
* @return Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
*/
public Integer getPeriod() {
return this.period;
}
/**
*
* Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
*
*
* @param period
* Granularity of data to retrieve, in seconds. Valid values are 60
, 300
, and
* 3600
. If you specify any other value, you will get an error. The default is 3600 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withPeriod(Integer period) {
setPeriod(period);
return this;
}
/**
*
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
. The
* default is NonZeroData
.
*
*
* @param dataType
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
.
* The default is NonZeroData
.
* @see InsightsMetricDataType
*/
public void setDataType(String dataType) {
this.dataType = dataType;
}
/**
*
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
. The
* default is NonZeroData
.
*
*
* @return Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
.
* The default is NonZeroData
.
* @see InsightsMetricDataType
*/
public String getDataType() {
return this.dataType;
}
/**
*
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
. The
* default is NonZeroData
.
*
*
* @param dataType
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
.
* The default is NonZeroData
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InsightsMetricDataType
*/
public ListInsightsMetricDataRequest withDataType(String dataType) {
setDataType(dataType);
return this;
}
/**
*
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
. The
* default is NonZeroData
.
*
*
* @param dataType
* Type of datapoints to return. Valid values are NonZeroData
and FillWithZeros
.
* The default is NonZeroData
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InsightsMetricDataType
*/
public ListInsightsMetricDataRequest withDataType(InsightsMetricDataType dataType) {
this.dataType = dataType.toString();
return this;
}
/**
*
* The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value is
* 21600.
*
*
* @param maxResults
* The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value
* is 21600.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value is
* 21600.
*
*
* @return The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value
* is 21600.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value is
* 21600.
*
*
* @param maxResults
* The maximum number of datapoints to return. Valid values are integers from 1 to 21600. The default value
* is 21600.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
*
*
* @param nextToken
* Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
*
*
* @return Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
*
*
* @param nextToken
* Returned if all datapoints can't be returned in a single call. For example, due to reaching
* MaxResults
.
*
* Add this parameter to the request to continue retrieving results starting from the last evaluated point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInsightsMetricDataRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEventSource() != null)
sb.append("EventSource: ").append(getEventSource()).append(",");
if (getEventName() != null)
sb.append("EventName: ").append(getEventName()).append(",");
if (getInsightType() != null)
sb.append("InsightType: ").append(getInsightType()).append(",");
if (getErrorCode() != null)
sb.append("ErrorCode: ").append(getErrorCode()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getPeriod() != null)
sb.append("Period: ").append(getPeriod()).append(",");
if (getDataType() != null)
sb.append("DataType: ").append(getDataType()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListInsightsMetricDataRequest == false)
return false;
ListInsightsMetricDataRequest other = (ListInsightsMetricDataRequest) obj;
if (other.getEventSource() == null ^ this.getEventSource() == null)
return false;
if (other.getEventSource() != null && other.getEventSource().equals(this.getEventSource()) == false)
return false;
if (other.getEventName() == null ^ this.getEventName() == null)
return false;
if (other.getEventName() != null && other.getEventName().equals(this.getEventName()) == false)
return false;
if (other.getInsightType() == null ^ this.getInsightType() == null)
return false;
if (other.getInsightType() != null && other.getInsightType().equals(this.getInsightType()) == false)
return false;
if (other.getErrorCode() == null ^ this.getErrorCode() == null)
return false;
if (other.getErrorCode() != null && other.getErrorCode().equals(this.getErrorCode()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getPeriod() == null ^ this.getPeriod() == null)
return false;
if (other.getPeriod() != null && other.getPeriod().equals(this.getPeriod()) == false)
return false;
if (other.getDataType() == null ^ this.getDataType() == null)
return false;
if (other.getDataType() != null && other.getDataType().equals(this.getDataType()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEventSource() == null) ? 0 : getEventSource().hashCode());
hashCode = prime * hashCode + ((getEventName() == null) ? 0 : getEventName().hashCode());
hashCode = prime * hashCode + ((getInsightType() == null) ? 0 : getInsightType().hashCode());
hashCode = prime * hashCode + ((getErrorCode() == null) ? 0 : getErrorCode().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getPeriod() == null) ? 0 : getPeriod().hashCode());
hashCode = prime * hashCode + ((getDataType() == null) ? 0 : getDataType().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public ListInsightsMetricDataRequest clone() {
return (ListInsightsMetricDataRequest) super.clone();
}
}