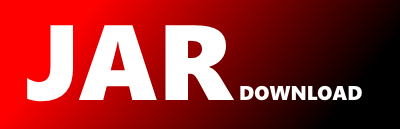
com.amazonaws.services.cloudtrail.model.StopImportResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudtrail Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudtrail.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StopImportResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The ID for the import.
*
*/
private String importId;
/**
*
* The source S3 bucket for the import.
*
*/
private ImportSource importSource;
/**
*
* The ARN of the destination event data store.
*
*/
private com.amazonaws.internal.SdkInternalList destinations;
/**
*
* The status of the import.
*
*/
private String importStatus;
/**
*
* The timestamp of the import's creation.
*
*/
private java.util.Date createdTimestamp;
/**
*
* The timestamp of the import's last update.
*
*/
private java.util.Date updatedTimestamp;
/**
*
* Used with EndEventTime
to bound a StartImport
request, and limit imported trail events
* to only those events logged within a specified time period.
*
*/
private java.util.Date startEventTime;
/**
*
* Used with StartEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
*
*/
private java.util.Date endEventTime;
/**
*
* Returns information on the stopped import.
*
*/
private ImportStatistics importStatistics;
/**
*
* The ID for the import.
*
*
* @param importId
* The ID for the import.
*/
public void setImportId(String importId) {
this.importId = importId;
}
/**
*
* The ID for the import.
*
*
* @return The ID for the import.
*/
public String getImportId() {
return this.importId;
}
/**
*
* The ID for the import.
*
*
* @param importId
* The ID for the import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withImportId(String importId) {
setImportId(importId);
return this;
}
/**
*
* The source S3 bucket for the import.
*
*
* @param importSource
* The source S3 bucket for the import.
*/
public void setImportSource(ImportSource importSource) {
this.importSource = importSource;
}
/**
*
* The source S3 bucket for the import.
*
*
* @return The source S3 bucket for the import.
*/
public ImportSource getImportSource() {
return this.importSource;
}
/**
*
* The source S3 bucket for the import.
*
*
* @param importSource
* The source S3 bucket for the import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withImportSource(ImportSource importSource) {
setImportSource(importSource);
return this;
}
/**
*
* The ARN of the destination event data store.
*
*
* @return The ARN of the destination event data store.
*/
public java.util.List getDestinations() {
if (destinations == null) {
destinations = new com.amazonaws.internal.SdkInternalList();
}
return destinations;
}
/**
*
* The ARN of the destination event data store.
*
*
* @param destinations
* The ARN of the destination event data store.
*/
public void setDestinations(java.util.Collection destinations) {
if (destinations == null) {
this.destinations = null;
return;
}
this.destinations = new com.amazonaws.internal.SdkInternalList(destinations);
}
/**
*
* The ARN of the destination event data store.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDestinations(java.util.Collection)} or {@link #withDestinations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param destinations
* The ARN of the destination event data store.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withDestinations(String... destinations) {
if (this.destinations == null) {
setDestinations(new com.amazonaws.internal.SdkInternalList(destinations.length));
}
for (String ele : destinations) {
this.destinations.add(ele);
}
return this;
}
/**
*
* The ARN of the destination event data store.
*
*
* @param destinations
* The ARN of the destination event data store.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withDestinations(java.util.Collection destinations) {
setDestinations(destinations);
return this;
}
/**
*
* The status of the import.
*
*
* @param importStatus
* The status of the import.
* @see ImportStatus
*/
public void setImportStatus(String importStatus) {
this.importStatus = importStatus;
}
/**
*
* The status of the import.
*
*
* @return The status of the import.
* @see ImportStatus
*/
public String getImportStatus() {
return this.importStatus;
}
/**
*
* The status of the import.
*
*
* @param importStatus
* The status of the import.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImportStatus
*/
public StopImportResult withImportStatus(String importStatus) {
setImportStatus(importStatus);
return this;
}
/**
*
* The status of the import.
*
*
* @param importStatus
* The status of the import.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImportStatus
*/
public StopImportResult withImportStatus(ImportStatus importStatus) {
this.importStatus = importStatus.toString();
return this;
}
/**
*
* The timestamp of the import's creation.
*
*
* @param createdTimestamp
* The timestamp of the import's creation.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The timestamp of the import's creation.
*
*
* @return The timestamp of the import's creation.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The timestamp of the import's creation.
*
*
* @param createdTimestamp
* The timestamp of the import's creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
*
* The timestamp of the import's last update.
*
*
* @param updatedTimestamp
* The timestamp of the import's last update.
*/
public void setUpdatedTimestamp(java.util.Date updatedTimestamp) {
this.updatedTimestamp = updatedTimestamp;
}
/**
*
* The timestamp of the import's last update.
*
*
* @return The timestamp of the import's last update.
*/
public java.util.Date getUpdatedTimestamp() {
return this.updatedTimestamp;
}
/**
*
* The timestamp of the import's last update.
*
*
* @param updatedTimestamp
* The timestamp of the import's last update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withUpdatedTimestamp(java.util.Date updatedTimestamp) {
setUpdatedTimestamp(updatedTimestamp);
return this;
}
/**
*
* Used with EndEventTime
to bound a StartImport
request, and limit imported trail events
* to only those events logged within a specified time period.
*
*
* @param startEventTime
* Used with EndEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
*/
public void setStartEventTime(java.util.Date startEventTime) {
this.startEventTime = startEventTime;
}
/**
*
* Used with EndEventTime
to bound a StartImport
request, and limit imported trail events
* to only those events logged within a specified time period.
*
*
* @return Used with EndEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
*/
public java.util.Date getStartEventTime() {
return this.startEventTime;
}
/**
*
* Used with EndEventTime
to bound a StartImport
request, and limit imported trail events
* to only those events logged within a specified time period.
*
*
* @param startEventTime
* Used with EndEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withStartEventTime(java.util.Date startEventTime) {
setStartEventTime(startEventTime);
return this;
}
/**
*
* Used with StartEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
*
*
* @param endEventTime
* Used with StartEventTime
to bound a StartImport
request, and limit imported
* trail events to only those events logged within a specified time period.
*/
public void setEndEventTime(java.util.Date endEventTime) {
this.endEventTime = endEventTime;
}
/**
*
* Used with StartEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
*
*
* @return Used with StartEventTime
to bound a StartImport
request, and limit imported
* trail events to only those events logged within a specified time period.
*/
public java.util.Date getEndEventTime() {
return this.endEventTime;
}
/**
*
* Used with StartEventTime
to bound a StartImport
request, and limit imported trail
* events to only those events logged within a specified time period.
*
*
* @param endEventTime
* Used with StartEventTime
to bound a StartImport
request, and limit imported
* trail events to only those events logged within a specified time period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withEndEventTime(java.util.Date endEventTime) {
setEndEventTime(endEventTime);
return this;
}
/**
*
* Returns information on the stopped import.
*
*
* @param importStatistics
* Returns information on the stopped import.
*/
public void setImportStatistics(ImportStatistics importStatistics) {
this.importStatistics = importStatistics;
}
/**
*
* Returns information on the stopped import.
*
*
* @return Returns information on the stopped import.
*/
public ImportStatistics getImportStatistics() {
return this.importStatistics;
}
/**
*
* Returns information on the stopped import.
*
*
* @param importStatistics
* Returns information on the stopped import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StopImportResult withImportStatistics(ImportStatistics importStatistics) {
setImportStatistics(importStatistics);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getImportId() != null)
sb.append("ImportId: ").append(getImportId()).append(",");
if (getImportSource() != null)
sb.append("ImportSource: ").append(getImportSource()).append(",");
if (getDestinations() != null)
sb.append("Destinations: ").append(getDestinations()).append(",");
if (getImportStatus() != null)
sb.append("ImportStatus: ").append(getImportStatus()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp()).append(",");
if (getUpdatedTimestamp() != null)
sb.append("UpdatedTimestamp: ").append(getUpdatedTimestamp()).append(",");
if (getStartEventTime() != null)
sb.append("StartEventTime: ").append(getStartEventTime()).append(",");
if (getEndEventTime() != null)
sb.append("EndEventTime: ").append(getEndEventTime()).append(",");
if (getImportStatistics() != null)
sb.append("ImportStatistics: ").append(getImportStatistics());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StopImportResult == false)
return false;
StopImportResult other = (StopImportResult) obj;
if (other.getImportId() == null ^ this.getImportId() == null)
return false;
if (other.getImportId() != null && other.getImportId().equals(this.getImportId()) == false)
return false;
if (other.getImportSource() == null ^ this.getImportSource() == null)
return false;
if (other.getImportSource() != null && other.getImportSource().equals(this.getImportSource()) == false)
return false;
if (other.getDestinations() == null ^ this.getDestinations() == null)
return false;
if (other.getDestinations() != null && other.getDestinations().equals(this.getDestinations()) == false)
return false;
if (other.getImportStatus() == null ^ this.getImportStatus() == null)
return false;
if (other.getImportStatus() != null && other.getImportStatus().equals(this.getImportStatus()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
if (other.getUpdatedTimestamp() == null ^ this.getUpdatedTimestamp() == null)
return false;
if (other.getUpdatedTimestamp() != null && other.getUpdatedTimestamp().equals(this.getUpdatedTimestamp()) == false)
return false;
if (other.getStartEventTime() == null ^ this.getStartEventTime() == null)
return false;
if (other.getStartEventTime() != null && other.getStartEventTime().equals(this.getStartEventTime()) == false)
return false;
if (other.getEndEventTime() == null ^ this.getEndEventTime() == null)
return false;
if (other.getEndEventTime() != null && other.getEndEventTime().equals(this.getEndEventTime()) == false)
return false;
if (other.getImportStatistics() == null ^ this.getImportStatistics() == null)
return false;
if (other.getImportStatistics() != null && other.getImportStatistics().equals(this.getImportStatistics()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getImportId() == null) ? 0 : getImportId().hashCode());
hashCode = prime * hashCode + ((getImportSource() == null) ? 0 : getImportSource().hashCode());
hashCode = prime * hashCode + ((getDestinations() == null) ? 0 : getDestinations().hashCode());
hashCode = prime * hashCode + ((getImportStatus() == null) ? 0 : getImportStatus().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
hashCode = prime * hashCode + ((getUpdatedTimestamp() == null) ? 0 : getUpdatedTimestamp().hashCode());
hashCode = prime * hashCode + ((getStartEventTime() == null) ? 0 : getStartEventTime().hashCode());
hashCode = prime * hashCode + ((getEndEventTime() == null) ? 0 : getEndEventTime().hashCode());
hashCode = prime * hashCode + ((getImportStatistics() == null) ? 0 : getImportStatistics().hashCode());
return hashCode;
}
@Override
public StopImportResult clone() {
try {
return (StopImportResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}