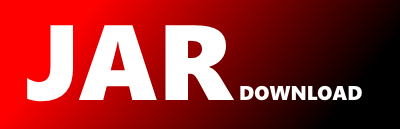
com.amazonaws.services.cloudwatch.AmazonCloudWatchAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudwatch Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudwatch;
import javax.annotation.Generated;
import com.amazonaws.services.cloudwatch.model.*;
/**
* Interface for accessing CloudWatch asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudwatch.AbstractAmazonCloudWatchAsync} instead.
*
*
*
* Amazon CloudWatch monitors your Amazon Web Services (AWS) resources and the applications you run on AWS in real time.
* You can use CloudWatch to collect and track metrics, which are the variables you want to measure for your resources
* and applications.
*
*
* CloudWatch alarms send notifications or automatically change the resources you are monitoring based on rules that you
* define. For example, you can monitor the CPU usage and disk reads and writes of your Amazon EC2 instances. Then, use
* this data to determine whether you should launch additional instances to handle increased load. You can also use this
* data to stop under-used instances to save money.
*
*
* In addition to monitoring the built-in metrics that come with AWS, you can monitor your own custom metrics. With
* CloudWatch, you gain system-wide visibility into resource utilization, application performance, and operational
* health.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCloudWatchAsync extends AmazonCloudWatch {
/**
*
* Deletes the specified alarms. In the event of an error, no alarms are deleted.
*
*
* @param deleteAlarmsRequest
* @return A Java Future containing the result of the DeleteAlarms operation returned by the service.
* @sample AmazonCloudWatchAsync.DeleteAlarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAlarmsAsync(DeleteAlarmsRequest deleteAlarmsRequest);
/**
*
* Deletes the specified alarms. In the event of an error, no alarms are deleted.
*
*
* @param deleteAlarmsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAlarms operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.DeleteAlarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAlarmsAsync(DeleteAlarmsRequest deleteAlarmsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all dashboards that you specify. You may specify up to 100 dashboards to delete. If there is an error
* during this call, no dashboards are deleted.
*
*
* @param deleteDashboardsRequest
* @return A Java Future containing the result of the DeleteDashboards operation returned by the service.
* @sample AmazonCloudWatchAsync.DeleteDashboards
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDashboardsAsync(DeleteDashboardsRequest deleteDashboardsRequest);
/**
*
* Deletes all dashboards that you specify. You may specify up to 100 dashboards to delete. If there is an error
* during this call, no dashboards are deleted.
*
*
* @param deleteDashboardsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDashboards operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.DeleteDashboards
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDashboardsAsync(DeleteDashboardsRequest deleteDashboardsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the history for the specified alarm. You can filter the results by date range or item type. If an alarm
* name is not specified, the histories for all alarms are returned.
*
*
* CloudWatch retains the history of an alarm even if you delete the alarm.
*
*
* @param describeAlarmHistoryRequest
* @return A Java Future containing the result of the DescribeAlarmHistory operation returned by the service.
* @sample AmazonCloudWatchAsync.DescribeAlarmHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAlarmHistoryAsync(DescribeAlarmHistoryRequest describeAlarmHistoryRequest);
/**
*
* Retrieves the history for the specified alarm. You can filter the results by date range or item type. If an alarm
* name is not specified, the histories for all alarms are returned.
*
*
* CloudWatch retains the history of an alarm even if you delete the alarm.
*
*
* @param describeAlarmHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAlarmHistory operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.DescribeAlarmHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAlarmHistoryAsync(DescribeAlarmHistoryRequest describeAlarmHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeAlarmHistory operation.
*
* @see #describeAlarmHistoryAsync(DescribeAlarmHistoryRequest)
*/
java.util.concurrent.Future describeAlarmHistoryAsync();
/**
* Simplified method form for invoking the DescribeAlarmHistory operation with an AsyncHandler.
*
* @see #describeAlarmHistoryAsync(DescribeAlarmHistoryRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeAlarmHistoryAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified alarms. If no alarms are specified, all alarms are returned. Alarms can be retrieved by
* using only a prefix for the alarm name, the alarm state, or a prefix for any action.
*
*
* @param describeAlarmsRequest
* @return A Java Future containing the result of the DescribeAlarms operation returned by the service.
* @sample AmazonCloudWatchAsync.DescribeAlarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAlarmsAsync(DescribeAlarmsRequest describeAlarmsRequest);
/**
*
* Retrieves the specified alarms. If no alarms are specified, all alarms are returned. Alarms can be retrieved by
* using only a prefix for the alarm name, the alarm state, or a prefix for any action.
*
*
* @param describeAlarmsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAlarms operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.DescribeAlarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAlarmsAsync(DescribeAlarmsRequest describeAlarmsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeAlarms operation.
*
* @see #describeAlarmsAsync(DescribeAlarmsRequest)
*/
java.util.concurrent.Future describeAlarmsAsync();
/**
* Simplified method form for invoking the DescribeAlarms operation with an AsyncHandler.
*
* @see #describeAlarmsAsync(DescribeAlarmsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeAlarmsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the alarms for the specified metric. To filter the results, specify a statistic, period, or unit.
*
*
* @param describeAlarmsForMetricRequest
* @return A Java Future containing the result of the DescribeAlarmsForMetric operation returned by the service.
* @sample AmazonCloudWatchAsync.DescribeAlarmsForMetric
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAlarmsForMetricAsync(DescribeAlarmsForMetricRequest describeAlarmsForMetricRequest);
/**
*
* Retrieves the alarms for the specified metric. To filter the results, specify a statistic, period, or unit.
*
*
* @param describeAlarmsForMetricRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAlarmsForMetric operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.DescribeAlarmsForMetric
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAlarmsForMetricAsync(DescribeAlarmsForMetricRequest describeAlarmsForMetricRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables the actions for the specified alarms. When an alarm's actions are disabled, the alarm actions do not
* execute when the alarm state changes.
*
*
* @param disableAlarmActionsRequest
* @return A Java Future containing the result of the DisableAlarmActions operation returned by the service.
* @sample AmazonCloudWatchAsync.DisableAlarmActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disableAlarmActionsAsync(DisableAlarmActionsRequest disableAlarmActionsRequest);
/**
*
* Disables the actions for the specified alarms. When an alarm's actions are disabled, the alarm actions do not
* execute when the alarm state changes.
*
*
* @param disableAlarmActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableAlarmActions operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.DisableAlarmActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disableAlarmActionsAsync(DisableAlarmActionsRequest disableAlarmActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables the actions for the specified alarms.
*
*
* @param enableAlarmActionsRequest
* @return A Java Future containing the result of the EnableAlarmActions operation returned by the service.
* @sample AmazonCloudWatchAsync.EnableAlarmActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future enableAlarmActionsAsync(EnableAlarmActionsRequest enableAlarmActionsRequest);
/**
*
* Enables the actions for the specified alarms.
*
*
* @param enableAlarmActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableAlarmActions operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.EnableAlarmActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future enableAlarmActionsAsync(EnableAlarmActionsRequest enableAlarmActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the details of the dashboard that you specify.
*
*
* To copy an existing dashboard, use GetDashboard
, and then use the data returned within
* DashboardBody
as the template for the new dashboard when you call PutDashboard
to
* create the copy.
*
*
* @param getDashboardRequest
* @return A Java Future containing the result of the GetDashboard operation returned by the service.
* @sample AmazonCloudWatchAsync.GetDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDashboardAsync(GetDashboardRequest getDashboardRequest);
/**
*
* Displays the details of the dashboard that you specify.
*
*
* To copy an existing dashboard, use GetDashboard
, and then use the data returned within
* DashboardBody
as the template for the new dashboard when you call PutDashboard
to
* create the copy.
*
*
* @param getDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDashboard operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.GetDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDashboardAsync(GetDashboardRequest getDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets statistics for the specified metric.
*
*
* Amazon CloudWatch retains metric data as follows:
*
*
* -
*
* Data points with a period of 60 seconds (1-minute) are available for 15 days
*
*
* -
*
* Data points with a period of 300 seconds (5-minute) are available for 63 days
*
*
* -
*
* Data points with a period of 3600 seconds (1 hour) are available for 455 days (15 months)
*
*
*
*
* CloudWatch started retaining 5-minute and 1-hour metric data as of July 9, 2016.
*
*
* The maximum number of data points returned from a single call is 1,440. If you request more than 1,440 data
* points, CloudWatch returns an error. To reduce the number of data points, you can narrow the specified time range
* and make multiple requests across adjacent time ranges, or you can increase the specified period. A period can be
* as short as one minute (60 seconds). Data points are not returned in chronological order.
*
*
* CloudWatch aggregates data points based on the length of the period that you specify. For example, if you request
* statistics with a one-hour period, CloudWatch aggregates all data points with time stamps that fall within each
* one-hour period. Therefore, the number of values aggregated by CloudWatch is larger than the number of data
* points returned.
*
*
* CloudWatch needs raw data points to calculate percentile statistics. If you publish data using a statistic set
* instead, you can only retrieve percentile statistics for this data if one of the following conditions is true:
*
*
* -
*
* The SampleCount value of the statistic set is 1.
*
*
* -
*
* The Min and the Max values of the statistic set are equal.
*
*
*
*
* For a list of metrics and dimensions supported by AWS services, see the Amazon CloudWatch
* Metrics and Dimensions Reference in the Amazon CloudWatch User Guide.
*
*
* @param getMetricStatisticsRequest
* @return A Java Future containing the result of the GetMetricStatistics operation returned by the service.
* @sample AmazonCloudWatchAsync.GetMetricStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMetricStatisticsAsync(GetMetricStatisticsRequest getMetricStatisticsRequest);
/**
*
* Gets statistics for the specified metric.
*
*
* Amazon CloudWatch retains metric data as follows:
*
*
* -
*
* Data points with a period of 60 seconds (1-minute) are available for 15 days
*
*
* -
*
* Data points with a period of 300 seconds (5-minute) are available for 63 days
*
*
* -
*
* Data points with a period of 3600 seconds (1 hour) are available for 455 days (15 months)
*
*
*
*
* CloudWatch started retaining 5-minute and 1-hour metric data as of July 9, 2016.
*
*
* The maximum number of data points returned from a single call is 1,440. If you request more than 1,440 data
* points, CloudWatch returns an error. To reduce the number of data points, you can narrow the specified time range
* and make multiple requests across adjacent time ranges, or you can increase the specified period. A period can be
* as short as one minute (60 seconds). Data points are not returned in chronological order.
*
*
* CloudWatch aggregates data points based on the length of the period that you specify. For example, if you request
* statistics with a one-hour period, CloudWatch aggregates all data points with time stamps that fall within each
* one-hour period. Therefore, the number of values aggregated by CloudWatch is larger than the number of data
* points returned.
*
*
* CloudWatch needs raw data points to calculate percentile statistics. If you publish data using a statistic set
* instead, you can only retrieve percentile statistics for this data if one of the following conditions is true:
*
*
* -
*
* The SampleCount value of the statistic set is 1.
*
*
* -
*
* The Min and the Max values of the statistic set are equal.
*
*
*
*
* For a list of metrics and dimensions supported by AWS services, see the Amazon CloudWatch
* Metrics and Dimensions Reference in the Amazon CloudWatch User Guide.
*
*
* @param getMetricStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMetricStatistics operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.GetMetricStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMetricStatisticsAsync(GetMetricStatisticsRequest getMetricStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the dashboards for your account. If you include DashboardNamePrefix
, only those
* dashboards with names starting with the prefix are listed. Otherwise, all dashboards in your account are listed.
*
*
* @param listDashboardsRequest
* @return A Java Future containing the result of the ListDashboards operation returned by the service.
* @sample AmazonCloudWatchAsync.ListDashboards
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDashboardsAsync(ListDashboardsRequest listDashboardsRequest);
/**
*
* Returns a list of the dashboards for your account. If you include DashboardNamePrefix
, only those
* dashboards with names starting with the prefix are listed. Otherwise, all dashboards in your account are listed.
*
*
* @param listDashboardsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDashboards operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.ListDashboards
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDashboardsAsync(ListDashboardsRequest listDashboardsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the specified metrics. You can use the returned metrics with GetMetricStatistics to obtain
* statistical data.
*
*
* Up to 500 results are returned for any one call. To retrieve additional results, use the returned token with
* subsequent calls.
*
*
* After you create a metric, allow up to fifteen minutes before the metric appears. Statistics about the metric,
* however, are available sooner using GetMetricStatistics.
*
*
* @param listMetricsRequest
* @return A Java Future containing the result of the ListMetrics operation returned by the service.
* @sample AmazonCloudWatchAsync.ListMetrics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMetricsAsync(ListMetricsRequest listMetricsRequest);
/**
*
* List the specified metrics. You can use the returned metrics with GetMetricStatistics to obtain
* statistical data.
*
*
* Up to 500 results are returned for any one call. To retrieve additional results, use the returned token with
* subsequent calls.
*
*
* After you create a metric, allow up to fifteen minutes before the metric appears. Statistics about the metric,
* however, are available sooner using GetMetricStatistics.
*
*
* @param listMetricsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMetrics operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.ListMetrics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMetricsAsync(ListMetricsRequest listMetricsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListMetrics operation.
*
* @see #listMetricsAsync(ListMetricsRequest)
*/
java.util.concurrent.Future listMetricsAsync();
/**
* Simplified method form for invoking the ListMetrics operation with an AsyncHandler.
*
* @see #listMetricsAsync(ListMetricsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listMetricsAsync(com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a dashboard if it does not already exist, or updates an existing dashboard. If you update a dashboard,
* the entire contents are replaced with what you specify here.
*
*
* You can have up to 500 dashboards per account. All dashboards in your account are global, not region-specific.
*
*
* To copy an existing dashboard, use GetDashboard
, and then use the data returned within
* DashboardBody
as the template for the new dashboard when you call PutDashboard
to
* create the copy.
*
*
* @param putDashboardRequest
* @return A Java Future containing the result of the PutDashboard operation returned by the service.
* @sample AmazonCloudWatchAsync.PutDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putDashboardAsync(PutDashboardRequest putDashboardRequest);
/**
*
* Creates a dashboard if it does not already exist, or updates an existing dashboard. If you update a dashboard,
* the entire contents are replaced with what you specify here.
*
*
* You can have up to 500 dashboards per account. All dashboards in your account are global, not region-specific.
*
*
* To copy an existing dashboard, use GetDashboard
, and then use the data returned within
* DashboardBody
as the template for the new dashboard when you call PutDashboard
to
* create the copy.
*
*
* @param putDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDashboard operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.PutDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putDashboardAsync(PutDashboardRequest putDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an alarm and associates it with the specified metric. Optionally, this operation can associate
* one or more Amazon SNS resources with the alarm.
*
*
* When this operation creates an alarm, the alarm state is immediately set to INSUFFICIENT_DATA
. The
* alarm is evaluated and its state is set appropriately. Any actions associated with the state are then executed.
*
*
* When you update an existing alarm, its state is left unchanged, but the update completely overwrites the previous
* configuration of the alarm.
*
*
* If you are an IAM user, you must have Amazon EC2 permissions for some operations:
*
*
* -
*
* ec2:DescribeInstanceStatus
and ec2:DescribeInstances
for all alarms on EC2 instance
* status metrics
*
*
* -
*
* ec2:StopInstances
for alarms with stop actions
*
*
* -
*
* ec2:TerminateInstances
for alarms with terminate actions
*
*
* -
*
* ec2:DescribeInstanceRecoveryAttribute
and ec2:RecoverInstances
for alarms with recover
* actions
*
*
*
*
* If you have read/write permissions for Amazon CloudWatch but not for Amazon EC2, you can still create an alarm,
* but the stop or terminate actions are not performed. However, if you are later granted the required permissions,
* the alarm actions that you created earlier are performed.
*
*
* If you are using an IAM role (for example, an EC2 instance profile), you cannot stop or terminate the instance
* using alarm actions. However, you can still see the alarm state and perform any other actions such as Amazon SNS
* notifications or Auto Scaling policies.
*
*
* If you are using temporary security credentials granted using AWS STS, you cannot stop or terminate an EC2
* instance using alarm actions.
*
*
* You must create at least one stop, terminate, or reboot alarm using either the Amazon EC2 or CloudWatch consoles
* to create the EC2ActionsAccess IAM role. After this IAM role is created, you can create stop, terminate,
* or reboot alarms using a command-line interface or API.
*
*
* @param putMetricAlarmRequest
* @return A Java Future containing the result of the PutMetricAlarm operation returned by the service.
* @sample AmazonCloudWatchAsync.PutMetricAlarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putMetricAlarmAsync(PutMetricAlarmRequest putMetricAlarmRequest);
/**
*
* Creates or updates an alarm and associates it with the specified metric. Optionally, this operation can associate
* one or more Amazon SNS resources with the alarm.
*
*
* When this operation creates an alarm, the alarm state is immediately set to INSUFFICIENT_DATA
. The
* alarm is evaluated and its state is set appropriately. Any actions associated with the state are then executed.
*
*
* When you update an existing alarm, its state is left unchanged, but the update completely overwrites the previous
* configuration of the alarm.
*
*
* If you are an IAM user, you must have Amazon EC2 permissions for some operations:
*
*
* -
*
* ec2:DescribeInstanceStatus
and ec2:DescribeInstances
for all alarms on EC2 instance
* status metrics
*
*
* -
*
* ec2:StopInstances
for alarms with stop actions
*
*
* -
*
* ec2:TerminateInstances
for alarms with terminate actions
*
*
* -
*
* ec2:DescribeInstanceRecoveryAttribute
and ec2:RecoverInstances
for alarms with recover
* actions
*
*
*
*
* If you have read/write permissions for Amazon CloudWatch but not for Amazon EC2, you can still create an alarm,
* but the stop or terminate actions are not performed. However, if you are later granted the required permissions,
* the alarm actions that you created earlier are performed.
*
*
* If you are using an IAM role (for example, an EC2 instance profile), you cannot stop or terminate the instance
* using alarm actions. However, you can still see the alarm state and perform any other actions such as Amazon SNS
* notifications or Auto Scaling policies.
*
*
* If you are using temporary security credentials granted using AWS STS, you cannot stop or terminate an EC2
* instance using alarm actions.
*
*
* You must create at least one stop, terminate, or reboot alarm using either the Amazon EC2 or CloudWatch consoles
* to create the EC2ActionsAccess IAM role. After this IAM role is created, you can create stop, terminate,
* or reboot alarms using a command-line interface or API.
*
*
* @param putMetricAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutMetricAlarm operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.PutMetricAlarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putMetricAlarmAsync(PutMetricAlarmRequest putMetricAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Publishes metric data points to Amazon CloudWatch. CloudWatch associates the data points with the specified
* metric. If the specified metric does not exist, CloudWatch creates the metric. When CloudWatch creates a metric,
* it can take up to fifteen minutes for the metric to appear in calls to ListMetrics.
*
*
* Each PutMetricData
request is limited to 40 KB in size for HTTP POST requests.
*
*
* Although the Value
parameter accepts numbers of type Double
, CloudWatch rejects values
* that are either too small or too large. Values must be in the range of 8.515920e-109 to 1.174271e+108 (Base 10)
* or 2e-360 to 2e360 (Base 2). In addition, special values (for example, NaN, +Infinity, -Infinity) are not
* supported.
*
*
* You can use up to 10 dimensions per metric to further clarify what data the metric collects. For more information
* about specifying dimensions, see Publishing
* Metrics in the Amazon CloudWatch User Guide.
*
*
* Data points with time stamps from 24 hours ago or longer can take at least 48 hours to become available for
* GetMetricStatistics from the time they are submitted.
*
*
* CloudWatch needs raw data points to calculate percentile statistics. If you publish data using a statistic set
* instead, you can only retrieve percentile statistics for this data if one of the following conditions is true:
*
*
* -
*
* The SampleCount value of the statistic set is 1
*
*
* -
*
* The Min and the Max values of the statistic set are equal
*
*
*
*
* @param putMetricDataRequest
* @return A Java Future containing the result of the PutMetricData operation returned by the service.
* @sample AmazonCloudWatchAsync.PutMetricData
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putMetricDataAsync(PutMetricDataRequest putMetricDataRequest);
/**
*
* Publishes metric data points to Amazon CloudWatch. CloudWatch associates the data points with the specified
* metric. If the specified metric does not exist, CloudWatch creates the metric. When CloudWatch creates a metric,
* it can take up to fifteen minutes for the metric to appear in calls to ListMetrics.
*
*
* Each PutMetricData
request is limited to 40 KB in size for HTTP POST requests.
*
*
* Although the Value
parameter accepts numbers of type Double
, CloudWatch rejects values
* that are either too small or too large. Values must be in the range of 8.515920e-109 to 1.174271e+108 (Base 10)
* or 2e-360 to 2e360 (Base 2). In addition, special values (for example, NaN, +Infinity, -Infinity) are not
* supported.
*
*
* You can use up to 10 dimensions per metric to further clarify what data the metric collects. For more information
* about specifying dimensions, see Publishing
* Metrics in the Amazon CloudWatch User Guide.
*
*
* Data points with time stamps from 24 hours ago or longer can take at least 48 hours to become available for
* GetMetricStatistics from the time they are submitted.
*
*
* CloudWatch needs raw data points to calculate percentile statistics. If you publish data using a statistic set
* instead, you can only retrieve percentile statistics for this data if one of the following conditions is true:
*
*
* -
*
* The SampleCount value of the statistic set is 1
*
*
* -
*
* The Min and the Max values of the statistic set are equal
*
*
*
*
* @param putMetricDataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutMetricData operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.PutMetricData
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putMetricDataAsync(PutMetricDataRequest putMetricDataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Temporarily sets the state of an alarm for testing purposes. When the updated state differs from the previous
* value, the action configured for the appropriate state is invoked. For example, if your alarm is configured to
* send an Amazon SNS message when an alarm is triggered, temporarily changing the alarm state to ALARM
* sends an SNS message. The alarm returns to its actual state (often within seconds). Because the alarm state
* change happens quickly, it is typically only visible in the alarm's History tab in the Amazon CloudWatch
* console or through DescribeAlarmHistory.
*
*
* @param setAlarmStateRequest
* @return A Java Future containing the result of the SetAlarmState operation returned by the service.
* @sample AmazonCloudWatchAsync.SetAlarmState
* @see AWS API
* Documentation
*/
java.util.concurrent.Future setAlarmStateAsync(SetAlarmStateRequest setAlarmStateRequest);
/**
*
* Temporarily sets the state of an alarm for testing purposes. When the updated state differs from the previous
* value, the action configured for the appropriate state is invoked. For example, if your alarm is configured to
* send an Amazon SNS message when an alarm is triggered, temporarily changing the alarm state to ALARM
* sends an SNS message. The alarm returns to its actual state (often within seconds). Because the alarm state
* change happens quickly, it is typically only visible in the alarm's History tab in the Amazon CloudWatch
* console or through DescribeAlarmHistory.
*
*
* @param setAlarmStateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetAlarmState operation returned by the service.
* @sample AmazonCloudWatchAsyncHandler.SetAlarmState
* @see AWS API
* Documentation
*/
java.util.concurrent.Future setAlarmStateAsync(SetAlarmStateRequest setAlarmStateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}