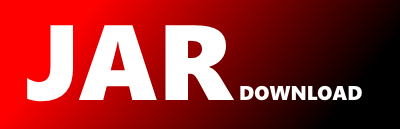
com.amazonaws.services.cloudwatchrum.AWSCloudWatchRUMAsync Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudwatchrum;
import javax.annotation.Generated;
import com.amazonaws.services.cloudwatchrum.model.*;
/**
* Interface for accessing CloudWatch RUM asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudwatchrum.AbstractAWSCloudWatchRUMAsync} instead.
*
*
*
* With Amazon CloudWatch RUM, you can perform real-user monitoring to collect client-side data about your web
* application performance from actual user sessions in real time. The data collected includes page load times,
* client-side errors, and user behavior. When you view this data, you can see it all aggregated together and also see
* breakdowns by the browsers and devices that your customers use.
*
*
*
* <p>You can use the collected data to quickly identify and debug client-side performance issues. CloudWatch RUM helps you visualize anomalies in your application performance and find relevant debugging data such as error messages, stack traces, and user sessions. You can also use RUM to understand the range of end-user impact including the number of users, geolocations, and browsers used.</p>
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSCloudWatchRUMAsync extends AWSCloudWatchRUM {
/**
*
* Creates a Amazon CloudWatch RUM app monitor, which collects telemetry data from your application and sends that
* data to RUM. The data includes performance and reliability information such as page load time, client-side
* errors, and user behavior.
*
*
* You use this operation only to create a new app monitor. To update an existing app monitor, use UpdateAppMonitor instead.
*
*
* After you create an app monitor, sign in to the CloudWatch RUM console to get the JavaScript code snippet to add
* to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param createAppMonitorRequest
* @return A Java Future containing the result of the CreateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.CreateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppMonitorAsync(CreateAppMonitorRequest createAppMonitorRequest);
/**
*
* Creates a Amazon CloudWatch RUM app monitor, which collects telemetry data from your application and sends that
* data to RUM. The data includes performance and reliability information such as page load time, client-side
* errors, and user behavior.
*
*
* You use this operation only to create a new app monitor. To update an existing app monitor, use UpdateAppMonitor instead.
*
*
* After you create an app monitor, sign in to the CloudWatch RUM console to get the JavaScript code snippet to add
* to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param createAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.CreateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppMonitorAsync(CreateAppMonitorRequest createAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing app monitor. This immediately stops the collection of data.
*
*
* @param deleteAppMonitorRequest
* @return A Java Future containing the result of the DeleteAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.DeleteAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppMonitorAsync(DeleteAppMonitorRequest deleteAppMonitorRequest);
/**
*
* Deletes an existing app monitor. This immediately stops the collection of data.
*
*
* @param deleteAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.DeleteAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppMonitorAsync(DeleteAppMonitorRequest deleteAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the complete configuration information for one app monitor.
*
*
* @param getAppMonitorRequest
* @return A Java Future containing the result of the GetAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.GetAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorAsync(GetAppMonitorRequest getAppMonitorRequest);
/**
*
* Retrieves the complete configuration information for one app monitor.
*
*
* @param getAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.GetAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorAsync(GetAppMonitorRequest getAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the raw performance events that RUM has collected from your web application, so that you can do your
* own processing or analysis of this data.
*
*
* @param getAppMonitorDataRequest
* @return A Java Future containing the result of the GetAppMonitorData operation returned by the service.
* @sample AWSCloudWatchRUMAsync.GetAppMonitorData
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorDataAsync(GetAppMonitorDataRequest getAppMonitorDataRequest);
/**
*
* Retrieves the raw performance events that RUM has collected from your web application, so that you can do your
* own processing or analysis of this data.
*
*
* @param getAppMonitorDataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppMonitorData operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.GetAppMonitorData
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorDataAsync(GetAppMonitorDataRequest getAppMonitorDataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the Amazon CloudWatch RUM app monitors in the account.
*
*
* @param listAppMonitorsRequest
* @return A Java Future containing the result of the ListAppMonitors operation returned by the service.
* @sample AWSCloudWatchRUMAsync.ListAppMonitors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppMonitorsAsync(ListAppMonitorsRequest listAppMonitorsRequest);
/**
*
* Returns a list of the Amazon CloudWatch RUM app monitors in the account.
*
*
* @param listAppMonitorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppMonitors operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.ListAppMonitors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppMonitorsAsync(ListAppMonitorsRequest listAppMonitorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the tags associated with a CloudWatch RUM resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSCloudWatchRUMAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Displays the tags associated with a CloudWatch RUM resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends telemetry events about your application performance and user behavior to CloudWatch RUM. The code snippet
* that RUM generates for you to add to your application includes PutRumEvents
operations to send this
* data to RUM.
*
*
* Each PutRumEvents
operation can send a batch of events from one user session.
*
*
* @param putRumEventsRequest
* @return A Java Future containing the result of the PutRumEvents operation returned by the service.
* @sample AWSCloudWatchRUMAsync.PutRumEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putRumEventsAsync(PutRumEventsRequest putRumEventsRequest);
/**
*
* Sends telemetry events about your application performance and user behavior to CloudWatch RUM. The code snippet
* that RUM generates for you to add to your application includes PutRumEvents
operations to send this
* data to RUM.
*
*
* Each PutRumEvents
operation can send a batch of events from one user session.
*
*
* @param putRumEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRumEvents operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.PutRumEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putRumEventsAsync(PutRumEventsRequest putRumEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch RUM resource. Currently, the only
* resources that can be tagged app monitors.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with a resource that already has tags. If you specify a new tag
* key for the resource, this tag is appended to the list of tags associated with the alarm. If you specify a tag
* key that is already associated with the resource, the new tag value that you specify replaces the previous value
* for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* For more information, see Tagging Amazon
* Web Services resources.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch RUM resource. Currently, the only
* resources that can be tagged app monitors.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with a resource that already has tags. If you specify a new tag
* key for the resource, this tag is appended to the list of tags associated with the alarm. If you specify a tag
* key that is already associated with the resource, the new tag value that you specify replaces the previous value
* for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* For more information, see Tagging Amazon
* Web Services resources.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing app monitor. When you use this operation, only the parts of the app
* monitor configuration that you specify in this operation are changed. For any parameters that you omit, the
* existing values are kept.
*
*
* You can't use this operation to change the tags of an existing app monitor. To change the tags of an existing app
* monitor, use TagResource.
*
*
* To create a new app monitor, use CreateAppMonitor.
*
*
* After you update an app monitor, sign in to the CloudWatch RUM console to get the updated JavaScript code snippet
* to add to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param updateAppMonitorRequest
* @return A Java Future containing the result of the UpdateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.UpdateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAppMonitorAsync(UpdateAppMonitorRequest updateAppMonitorRequest);
/**
*
* Updates the configuration of an existing app monitor. When you use this operation, only the parts of the app
* monitor configuration that you specify in this operation are changed. For any parameters that you omit, the
* existing values are kept.
*
*
* You can't use this operation to change the tags of an existing app monitor. To change the tags of an existing app
* monitor, use TagResource.
*
*
* To create a new app monitor, use CreateAppMonitor.
*
*
* After you update an app monitor, sign in to the CloudWatch RUM console to get the updated JavaScript code snippet
* to add to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param updateAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.UpdateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAppMonitorAsync(UpdateAppMonitorRequest updateAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}