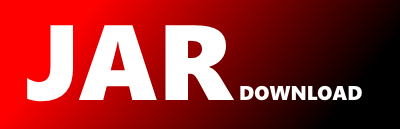
com.amazonaws.services.cloudwatchrum.AWSCloudWatchRUMAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudwatchrum Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudwatchrum;
import javax.annotation.Generated;
import com.amazonaws.services.cloudwatchrum.model.*;
/**
* Interface for accessing CloudWatch RUM asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudwatchrum.AbstractAWSCloudWatchRUMAsync} instead.
*
*
*
* With Amazon CloudWatch RUM, you can perform real-user monitoring to collect client-side data about your web
* application performance from actual user sessions in real time. The data collected includes page load times,
* client-side errors, and user behavior. When you view this data, you can see it all aggregated together and also see
* breakdowns by the browsers and devices that your customers use.
*
*
* You can use the collected data to quickly identify and debug client-side performance issues. CloudWatch RUM helps you
* visualize anomalies in your application performance and find relevant debugging data such as error messages, stack
* traces, and user sessions. You can also use RUM to understand the range of end-user impact including the number of
* users, geolocations, and browsers used.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSCloudWatchRUMAsync extends AWSCloudWatchRUM {
/**
*
* Specifies the extended metrics and custom metrics that you want a CloudWatch RUM app monitor to send to a
* destination. Valid destinations include CloudWatch and Evidently.
*
*
* By default, RUM app monitors send some metrics to CloudWatch. These default metrics are listed in CloudWatch
* metrics that you can collect with CloudWatch RUM.
*
*
* In addition to these default metrics, you can choose to send extended metrics, custom metrics, or both.
*
*
* -
*
* Extended metrics let you send metrics with additional dimensions that aren't included in the default metrics. You
* can also send extended metrics to both Evidently and CloudWatch. The valid dimension names for the additional
* dimensions for extended metrics are BrowserName
, CountryCode
, DeviceType
,
* FileType
, OSName
, and PageId
. For more information, see
* Extended metrics that you can send to CloudWatch and CloudWatch Evidently.
*
*
* -
*
* Custom metrics are metrics that you define. You can send custom metrics to CloudWatch. CloudWatch Evidently, or
* both. With custom metrics, you can use any metric name and namespace. To derive the metrics, you can use any
* custom events, built-in events, custom attributes, or default attributes.
*
*
* You can't send custom metrics to the AWS/RUM
namespace. You must send custom metrics to a custom
* namespace that you define. The namespace that you use can't start with AWS/
. CloudWatch RUM prepends
* RUM/CustomMetrics/
to the custom namespace that you define, so the final namespace for your metrics
* in CloudWatch is RUM/CustomMetrics/your-custom-namespace
.
*
*
*
*
* The maximum number of metric definitions that you can specify in one BatchCreateRumMetricDefinitions
* operation is 200.
*
*
* The maximum number of metric definitions that one destination can contain is 2000.
*
*
* Extended metrics sent to CloudWatch and RUM custom metrics are charged as CloudWatch custom metrics. Each
* combination of additional dimension name and dimension value counts as a custom metric. For more information, see
* Amazon CloudWatch Pricing.
*
*
* You must have already created a destination for the metrics before you send them. For more information, see
* PutRumMetricsDestination.
*
*
* If some metric definitions specified in a BatchCreateRumMetricDefinitions
operations are not valid,
* those metric definitions fail and return errors, but all valid metric definitions in the same operation still
* succeed.
*
*
* @param batchCreateRumMetricDefinitionsRequest
* @return A Java Future containing the result of the BatchCreateRumMetricDefinitions operation returned by the
* service.
* @sample AWSCloudWatchRUMAsync.BatchCreateRumMetricDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateRumMetricDefinitionsAsync(
BatchCreateRumMetricDefinitionsRequest batchCreateRumMetricDefinitionsRequest);
/**
*
* Specifies the extended metrics and custom metrics that you want a CloudWatch RUM app monitor to send to a
* destination. Valid destinations include CloudWatch and Evidently.
*
*
* By default, RUM app monitors send some metrics to CloudWatch. These default metrics are listed in CloudWatch
* metrics that you can collect with CloudWatch RUM.
*
*
* In addition to these default metrics, you can choose to send extended metrics, custom metrics, or both.
*
*
* -
*
* Extended metrics let you send metrics with additional dimensions that aren't included in the default metrics. You
* can also send extended metrics to both Evidently and CloudWatch. The valid dimension names for the additional
* dimensions for extended metrics are BrowserName
, CountryCode
, DeviceType
,
* FileType
, OSName
, and PageId
. For more information, see
* Extended metrics that you can send to CloudWatch and CloudWatch Evidently.
*
*
* -
*
* Custom metrics are metrics that you define. You can send custom metrics to CloudWatch. CloudWatch Evidently, or
* both. With custom metrics, you can use any metric name and namespace. To derive the metrics, you can use any
* custom events, built-in events, custom attributes, or default attributes.
*
*
* You can't send custom metrics to the AWS/RUM
namespace. You must send custom metrics to a custom
* namespace that you define. The namespace that you use can't start with AWS/
. CloudWatch RUM prepends
* RUM/CustomMetrics/
to the custom namespace that you define, so the final namespace for your metrics
* in CloudWatch is RUM/CustomMetrics/your-custom-namespace
.
*
*
*
*
* The maximum number of metric definitions that you can specify in one BatchCreateRumMetricDefinitions
* operation is 200.
*
*
* The maximum number of metric definitions that one destination can contain is 2000.
*
*
* Extended metrics sent to CloudWatch and RUM custom metrics are charged as CloudWatch custom metrics. Each
* combination of additional dimension name and dimension value counts as a custom metric. For more information, see
* Amazon CloudWatch Pricing.
*
*
* You must have already created a destination for the metrics before you send them. For more information, see
* PutRumMetricsDestination.
*
*
* If some metric definitions specified in a BatchCreateRumMetricDefinitions
operations are not valid,
* those metric definitions fail and return errors, but all valid metric definitions in the same operation still
* succeed.
*
*
* @param batchCreateRumMetricDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateRumMetricDefinitions operation returned by the
* service.
* @sample AWSCloudWatchRUMAsyncHandler.BatchCreateRumMetricDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateRumMetricDefinitionsAsync(
BatchCreateRumMetricDefinitionsRequest batchCreateRumMetricDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified metrics from being sent to an extended metrics destination.
*
*
* If some metric definition IDs specified in a BatchDeleteRumMetricDefinitions
operations are not
* valid, those metric definitions fail and return errors, but all valid metric definition IDs in the same operation
* are still deleted.
*
*
* The maximum number of metric definitions that you can specify in one BatchDeleteRumMetricDefinitions
* operation is 200.
*
*
* @param batchDeleteRumMetricDefinitionsRequest
* @return A Java Future containing the result of the BatchDeleteRumMetricDefinitions operation returned by the
* service.
* @sample AWSCloudWatchRUMAsync.BatchDeleteRumMetricDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteRumMetricDefinitionsAsync(
BatchDeleteRumMetricDefinitionsRequest batchDeleteRumMetricDefinitionsRequest);
/**
*
* Removes the specified metrics from being sent to an extended metrics destination.
*
*
* If some metric definition IDs specified in a BatchDeleteRumMetricDefinitions
operations are not
* valid, those metric definitions fail and return errors, but all valid metric definition IDs in the same operation
* are still deleted.
*
*
* The maximum number of metric definitions that you can specify in one BatchDeleteRumMetricDefinitions
* operation is 200.
*
*
* @param batchDeleteRumMetricDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteRumMetricDefinitions operation returned by the
* service.
* @sample AWSCloudWatchRUMAsyncHandler.BatchDeleteRumMetricDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteRumMetricDefinitionsAsync(
BatchDeleteRumMetricDefinitionsRequest batchDeleteRumMetricDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of metrics and dimensions that a RUM app monitor is sending to a single destination.
*
*
* @param batchGetRumMetricDefinitionsRequest
* @return A Java Future containing the result of the BatchGetRumMetricDefinitions operation returned by the
* service.
* @sample AWSCloudWatchRUMAsync.BatchGetRumMetricDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetRumMetricDefinitionsAsync(
BatchGetRumMetricDefinitionsRequest batchGetRumMetricDefinitionsRequest);
/**
*
* Retrieves the list of metrics and dimensions that a RUM app monitor is sending to a single destination.
*
*
* @param batchGetRumMetricDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetRumMetricDefinitions operation returned by the
* service.
* @sample AWSCloudWatchRUMAsyncHandler.BatchGetRumMetricDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetRumMetricDefinitionsAsync(
BatchGetRumMetricDefinitionsRequest batchGetRumMetricDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Amazon CloudWatch RUM app monitor, which collects telemetry data from your application and sends that
* data to RUM. The data includes performance and reliability information such as page load time, client-side
* errors, and user behavior.
*
*
* You use this operation only to create a new app monitor. To update an existing app monitor, use UpdateAppMonitor instead.
*
*
* After you create an app monitor, sign in to the CloudWatch RUM console to get the JavaScript code snippet to add
* to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param createAppMonitorRequest
* @return A Java Future containing the result of the CreateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.CreateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppMonitorAsync(CreateAppMonitorRequest createAppMonitorRequest);
/**
*
* Creates a Amazon CloudWatch RUM app monitor, which collects telemetry data from your application and sends that
* data to RUM. The data includes performance and reliability information such as page load time, client-side
* errors, and user behavior.
*
*
* You use this operation only to create a new app monitor. To update an existing app monitor, use UpdateAppMonitor instead.
*
*
* After you create an app monitor, sign in to the CloudWatch RUM console to get the JavaScript code snippet to add
* to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param createAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.CreateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppMonitorAsync(CreateAppMonitorRequest createAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing app monitor. This immediately stops the collection of data.
*
*
* @param deleteAppMonitorRequest
* @return A Java Future containing the result of the DeleteAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.DeleteAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppMonitorAsync(DeleteAppMonitorRequest deleteAppMonitorRequest);
/**
*
* Deletes an existing app monitor. This immediately stops the collection of data.
*
*
* @param deleteAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.DeleteAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppMonitorAsync(DeleteAppMonitorRequest deleteAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a destination for CloudWatch RUM extended metrics, so that the specified app monitor stops sending
* extended metrics to that destination.
*
*
* @param deleteRumMetricsDestinationRequest
* @return A Java Future containing the result of the DeleteRumMetricsDestination operation returned by the service.
* @sample AWSCloudWatchRUMAsync.DeleteRumMetricsDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRumMetricsDestinationAsync(
DeleteRumMetricsDestinationRequest deleteRumMetricsDestinationRequest);
/**
*
* Deletes a destination for CloudWatch RUM extended metrics, so that the specified app monitor stops sending
* extended metrics to that destination.
*
*
* @param deleteRumMetricsDestinationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRumMetricsDestination operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.DeleteRumMetricsDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRumMetricsDestinationAsync(
DeleteRumMetricsDestinationRequest deleteRumMetricsDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the complete configuration information for one app monitor.
*
*
* @param getAppMonitorRequest
* @return A Java Future containing the result of the GetAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.GetAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorAsync(GetAppMonitorRequest getAppMonitorRequest);
/**
*
* Retrieves the complete configuration information for one app monitor.
*
*
* @param getAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.GetAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorAsync(GetAppMonitorRequest getAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the raw performance events that RUM has collected from your web application, so that you can do your
* own processing or analysis of this data.
*
*
* @param getAppMonitorDataRequest
* @return A Java Future containing the result of the GetAppMonitorData operation returned by the service.
* @sample AWSCloudWatchRUMAsync.GetAppMonitorData
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorDataAsync(GetAppMonitorDataRequest getAppMonitorDataRequest);
/**
*
* Retrieves the raw performance events that RUM has collected from your web application, so that you can do your
* own processing or analysis of this data.
*
*
* @param getAppMonitorDataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppMonitorData operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.GetAppMonitorData
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppMonitorDataAsync(GetAppMonitorDataRequest getAppMonitorDataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the Amazon CloudWatch RUM app monitors in the account.
*
*
* @param listAppMonitorsRequest
* @return A Java Future containing the result of the ListAppMonitors operation returned by the service.
* @sample AWSCloudWatchRUMAsync.ListAppMonitors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppMonitorsAsync(ListAppMonitorsRequest listAppMonitorsRequest);
/**
*
* Returns a list of the Amazon CloudWatch RUM app monitors in the account.
*
*
* @param listAppMonitorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppMonitors operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.ListAppMonitors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppMonitorsAsync(ListAppMonitorsRequest listAppMonitorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of destinations that you have created to receive RUM extended metrics, for the specified app
* monitor.
*
*
* For more information about extended metrics, see AddRumMetrics.
*
*
* @param listRumMetricsDestinationsRequest
* @return A Java Future containing the result of the ListRumMetricsDestinations operation returned by the service.
* @sample AWSCloudWatchRUMAsync.ListRumMetricsDestinations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRumMetricsDestinationsAsync(
ListRumMetricsDestinationsRequest listRumMetricsDestinationsRequest);
/**
*
* Returns a list of destinations that you have created to receive RUM extended metrics, for the specified app
* monitor.
*
*
* For more information about extended metrics, see AddRumMetrics.
*
*
* @param listRumMetricsDestinationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRumMetricsDestinations operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.ListRumMetricsDestinations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRumMetricsDestinationsAsync(
ListRumMetricsDestinationsRequest listRumMetricsDestinationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the tags associated with a CloudWatch RUM resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSCloudWatchRUMAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Displays the tags associated with a CloudWatch RUM resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends telemetry events about your application performance and user behavior to CloudWatch RUM. The code snippet
* that RUM generates for you to add to your application includes PutRumEvents
operations to send this
* data to RUM.
*
*
* Each PutRumEvents
operation can send a batch of events from one user session.
*
*
* @param putRumEventsRequest
* @return A Java Future containing the result of the PutRumEvents operation returned by the service.
* @sample AWSCloudWatchRUMAsync.PutRumEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putRumEventsAsync(PutRumEventsRequest putRumEventsRequest);
/**
*
* Sends telemetry events about your application performance and user behavior to CloudWatch RUM. The code snippet
* that RUM generates for you to add to your application includes PutRumEvents
operations to send this
* data to RUM.
*
*
* Each PutRumEvents
operation can send a batch of events from one user session.
*
*
* @param putRumEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRumEvents operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.PutRumEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putRumEventsAsync(PutRumEventsRequest putRumEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a destination to receive extended metrics from CloudWatch RUM. You can send extended metrics
* to CloudWatch or to a CloudWatch Evidently experiment.
*
*
* For more information about extended metrics, see BatchCreateRumMetricDefinitions.
*
*
* @param putRumMetricsDestinationRequest
* @return A Java Future containing the result of the PutRumMetricsDestination operation returned by the service.
* @sample AWSCloudWatchRUMAsync.PutRumMetricsDestination
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putRumMetricsDestinationAsync(PutRumMetricsDestinationRequest putRumMetricsDestinationRequest);
/**
*
* Creates or updates a destination to receive extended metrics from CloudWatch RUM. You can send extended metrics
* to CloudWatch or to a CloudWatch Evidently experiment.
*
*
* For more information about extended metrics, see BatchCreateRumMetricDefinitions.
*
*
* @param putRumMetricsDestinationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRumMetricsDestination operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.PutRumMetricsDestination
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putRumMetricsDestinationAsync(PutRumMetricsDestinationRequest putRumMetricsDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch RUM resource. Currently, the only
* resources that can be tagged app monitors.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with a resource that already has tags. If you specify a new tag
* key for the resource, this tag is appended to the list of tags associated with the alarm. If you specify a tag
* key that is already associated with the resource, the new tag value that you specify replaces the previous value
* for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* For more information, see Tagging Amazon
* Web Services resources.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch RUM resource. Currently, the only
* resources that can be tagged app monitors.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with a resource that already has tags. If you specify a new tag
* key for the resource, this tag is appended to the list of tags associated with the alarm. If you specify a tag
* key that is already associated with the resource, the new tag value that you specify replaces the previous value
* for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* For more information, see Tagging Amazon
* Web Services resources.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing app monitor. When you use this operation, only the parts of the app
* monitor configuration that you specify in this operation are changed. For any parameters that you omit, the
* existing values are kept.
*
*
* You can't use this operation to change the tags of an existing app monitor. To change the tags of an existing app
* monitor, use TagResource.
*
*
* To create a new app monitor, use CreateAppMonitor.
*
*
* After you update an app monitor, sign in to the CloudWatch RUM console to get the updated JavaScript code snippet
* to add to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param updateAppMonitorRequest
* @return A Java Future containing the result of the UpdateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsync.UpdateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAppMonitorAsync(UpdateAppMonitorRequest updateAppMonitorRequest);
/**
*
* Updates the configuration of an existing app monitor. When you use this operation, only the parts of the app
* monitor configuration that you specify in this operation are changed. For any parameters that you omit, the
* existing values are kept.
*
*
* You can't use this operation to change the tags of an existing app monitor. To change the tags of an existing app
* monitor, use TagResource.
*
*
* To create a new app monitor, use CreateAppMonitor.
*
*
* After you update an app monitor, sign in to the CloudWatch RUM console to get the updated JavaScript code snippet
* to add to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param updateAppMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppMonitor operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.UpdateAppMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAppMonitorAsync(UpdateAppMonitorRequest updateAppMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies one existing metric definition for CloudWatch RUM extended metrics. For more information about extended
* metrics, see BatchCreateRumMetricsDefinitions.
*
*
* @param updateRumMetricDefinitionRequest
* @return A Java Future containing the result of the UpdateRumMetricDefinition operation returned by the service.
* @sample AWSCloudWatchRUMAsync.UpdateRumMetricDefinition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateRumMetricDefinitionAsync(
UpdateRumMetricDefinitionRequest updateRumMetricDefinitionRequest);
/**
*
* Modifies one existing metric definition for CloudWatch RUM extended metrics. For more information about extended
* metrics, see BatchCreateRumMetricsDefinitions.
*
*
* @param updateRumMetricDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRumMetricDefinition operation returned by the service.
* @sample AWSCloudWatchRUMAsyncHandler.UpdateRumMetricDefinition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateRumMetricDefinitionAsync(
UpdateRumMetricDefinitionRequest updateRumMetricDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}