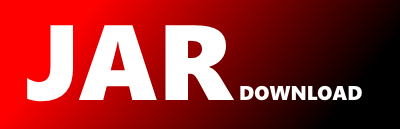
com.amazonaws.services.cloudwatchrum.AWSCloudWatchRUMClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudwatchrum Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudwatchrum;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.cloudwatchrum.AWSCloudWatchRUMClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.cloudwatchrum.model.*;
import com.amazonaws.services.cloudwatchrum.model.transform.*;
/**
* Client for accessing CloudWatch RUM. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* With Amazon CloudWatch RUM, you can perform real-user monitoring to collect client-side data about your web
* application performance from actual user sessions in real time. The data collected includes page load times,
* client-side errors, and user behavior. When you view this data, you can see it all aggregated together and also see
* breakdowns by the browsers and devices that your customers use.
*
*
* You can use the collected data to quickly identify and debug client-side performance issues. CloudWatch RUM helps you
* visualize anomalies in your application performance and find relevant debugging data such as error messages, stack
* traces, and user sessions. You can also use RUM to understand the range of end-user impact including the number of
* users, geolocations, and browsers used.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSCloudWatchRUMClient extends AmazonWebServiceClient implements AWSCloudWatchRUM {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSCloudWatchRUM.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "rum";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.cloudwatchrum.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.cloudwatchrum.model.AWSCloudWatchRUMException.class));
public static AWSCloudWatchRUMClientBuilder builder() {
return AWSCloudWatchRUMClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on CloudWatch RUM using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSCloudWatchRUMClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on CloudWatch RUM using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSCloudWatchRUMClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("rum.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/cloudwatchrum/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/cloudwatchrum/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Specifies the extended metrics and custom metrics that you want a CloudWatch RUM app monitor to send to a
* destination. Valid destinations include CloudWatch and Evidently.
*
*
* By default, RUM app monitors send some metrics to CloudWatch. These default metrics are listed in CloudWatch
* metrics that you can collect with CloudWatch RUM.
*
*
* In addition to these default metrics, you can choose to send extended metrics, custom metrics, or both.
*
*
* -
*
* Extended metrics let you send metrics with additional dimensions that aren't included in the default metrics. You
* can also send extended metrics to both Evidently and CloudWatch. The valid dimension names for the additional
* dimensions for extended metrics are BrowserName
, CountryCode
, DeviceType
,
* FileType
, OSName
, and PageId
. For more information, see
* Extended metrics that you can send to CloudWatch and CloudWatch Evidently.
*
*
* -
*
* Custom metrics are metrics that you define. You can send custom metrics to CloudWatch. CloudWatch Evidently, or
* both. With custom metrics, you can use any metric name and namespace. To derive the metrics, you can use any
* custom events, built-in events, custom attributes, or default attributes.
*
*
* You can't send custom metrics to the AWS/RUM
namespace. You must send custom metrics to a custom
* namespace that you define. The namespace that you use can't start with AWS/
. CloudWatch RUM prepends
* RUM/CustomMetrics/
to the custom namespace that you define, so the final namespace for your metrics
* in CloudWatch is RUM/CustomMetrics/your-custom-namespace
.
*
*
*
*
* The maximum number of metric definitions that you can specify in one BatchCreateRumMetricDefinitions
* operation is 200.
*
*
* The maximum number of metric definitions that one destination can contain is 2000.
*
*
* Extended metrics sent to CloudWatch and RUM custom metrics are charged as CloudWatch custom metrics. Each
* combination of additional dimension name and dimension value counts as a custom metric. For more information, see
* Amazon CloudWatch Pricing.
*
*
* You must have already created a destination for the metrics before you send them. For more information, see
* PutRumMetricsDestination.
*
*
* If some metric definitions specified in a BatchCreateRumMetricDefinitions
operations are not valid,
* those metric definitions fail and return errors, but all valid metric definitions in the same operation still
* succeed.
*
*
* @param batchCreateRumMetricDefinitionsRequest
* @return Result of the BatchCreateRumMetricDefinitions operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ServiceQuotaExceededException
* This request exceeds a service quota.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.BatchCreateRumMetricDefinitions
* @see AWS API Documentation
*/
@Override
public BatchCreateRumMetricDefinitionsResult batchCreateRumMetricDefinitions(BatchCreateRumMetricDefinitionsRequest request) {
request = beforeClientExecution(request);
return executeBatchCreateRumMetricDefinitions(request);
}
@SdkInternalApi
final BatchCreateRumMetricDefinitionsResult executeBatchCreateRumMetricDefinitions(
BatchCreateRumMetricDefinitionsRequest batchCreateRumMetricDefinitionsRequest) {
ExecutionContext executionContext = createExecutionContext(batchCreateRumMetricDefinitionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchCreateRumMetricDefinitionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchCreateRumMetricDefinitionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchCreateRumMetricDefinitions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchCreateRumMetricDefinitionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified metrics from being sent to an extended metrics destination.
*
*
* If some metric definition IDs specified in a BatchDeleteRumMetricDefinitions
operations are not
* valid, those metric definitions fail and return errors, but all valid metric definition IDs in the same operation
* are still deleted.
*
*
* The maximum number of metric definitions that you can specify in one BatchDeleteRumMetricDefinitions
* operation is 200.
*
*
* @param batchDeleteRumMetricDefinitionsRequest
* @return Result of the BatchDeleteRumMetricDefinitions operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.BatchDeleteRumMetricDefinitions
* @see AWS API Documentation
*/
@Override
public BatchDeleteRumMetricDefinitionsResult batchDeleteRumMetricDefinitions(BatchDeleteRumMetricDefinitionsRequest request) {
request = beforeClientExecution(request);
return executeBatchDeleteRumMetricDefinitions(request);
}
@SdkInternalApi
final BatchDeleteRumMetricDefinitionsResult executeBatchDeleteRumMetricDefinitions(
BatchDeleteRumMetricDefinitionsRequest batchDeleteRumMetricDefinitionsRequest) {
ExecutionContext executionContext = createExecutionContext(batchDeleteRumMetricDefinitionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDeleteRumMetricDefinitionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchDeleteRumMetricDefinitionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDeleteRumMetricDefinitions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchDeleteRumMetricDefinitionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the list of metrics and dimensions that a RUM app monitor is sending to a single destination.
*
*
* @param batchGetRumMetricDefinitionsRequest
* @return Result of the BatchGetRumMetricDefinitions operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.BatchGetRumMetricDefinitions
* @see AWS API Documentation
*/
@Override
public BatchGetRumMetricDefinitionsResult batchGetRumMetricDefinitions(BatchGetRumMetricDefinitionsRequest request) {
request = beforeClientExecution(request);
return executeBatchGetRumMetricDefinitions(request);
}
@SdkInternalApi
final BatchGetRumMetricDefinitionsResult executeBatchGetRumMetricDefinitions(BatchGetRumMetricDefinitionsRequest batchGetRumMetricDefinitionsRequest) {
ExecutionContext executionContext = createExecutionContext(batchGetRumMetricDefinitionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchGetRumMetricDefinitionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchGetRumMetricDefinitionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchGetRumMetricDefinitions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchGetRumMetricDefinitionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Amazon CloudWatch RUM app monitor, which collects telemetry data from your application and sends that
* data to RUM. The data includes performance and reliability information such as page load time, client-side
* errors, and user behavior.
*
*
* You use this operation only to create a new app monitor. To update an existing app monitor, use UpdateAppMonitor instead.
*
*
* After you create an app monitor, sign in to the CloudWatch RUM console to get the JavaScript code snippet to add
* to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param createAppMonitorRequest
* @return Result of the CreateAppMonitor operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ServiceQuotaExceededException
* This request exceeds a service quota.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.CreateAppMonitor
* @see AWS API
* Documentation
*/
@Override
public CreateAppMonitorResult createAppMonitor(CreateAppMonitorRequest request) {
request = beforeClientExecution(request);
return executeCreateAppMonitor(request);
}
@SdkInternalApi
final CreateAppMonitorResult executeCreateAppMonitor(CreateAppMonitorRequest createAppMonitorRequest) {
ExecutionContext executionContext = createExecutionContext(createAppMonitorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppMonitorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAppMonitorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAppMonitor");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAppMonitorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing app monitor. This immediately stops the collection of data.
*
*
* @param deleteAppMonitorRequest
* @return Result of the DeleteAppMonitor operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.DeleteAppMonitor
* @see AWS API
* Documentation
*/
@Override
public DeleteAppMonitorResult deleteAppMonitor(DeleteAppMonitorRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppMonitor(request);
}
@SdkInternalApi
final DeleteAppMonitorResult executeDeleteAppMonitor(DeleteAppMonitorRequest deleteAppMonitorRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppMonitorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppMonitorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppMonitorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppMonitor");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAppMonitorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a destination for CloudWatch RUM extended metrics, so that the specified app monitor stops sending
* extended metrics to that destination.
*
*
* @param deleteRumMetricsDestinationRequest
* @return Result of the DeleteRumMetricsDestination operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.DeleteRumMetricsDestination
* @see AWS API Documentation
*/
@Override
public DeleteRumMetricsDestinationResult deleteRumMetricsDestination(DeleteRumMetricsDestinationRequest request) {
request = beforeClientExecution(request);
return executeDeleteRumMetricsDestination(request);
}
@SdkInternalApi
final DeleteRumMetricsDestinationResult executeDeleteRumMetricsDestination(DeleteRumMetricsDestinationRequest deleteRumMetricsDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRumMetricsDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRumMetricsDestinationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteRumMetricsDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRumMetricsDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteRumMetricsDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the complete configuration information for one app monitor.
*
*
* @param getAppMonitorRequest
* @return Result of the GetAppMonitor operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.GetAppMonitor
* @see AWS API
* Documentation
*/
@Override
public GetAppMonitorResult getAppMonitor(GetAppMonitorRequest request) {
request = beforeClientExecution(request);
return executeGetAppMonitor(request);
}
@SdkInternalApi
final GetAppMonitorResult executeGetAppMonitor(GetAppMonitorRequest getAppMonitorRequest) {
ExecutionContext executionContext = createExecutionContext(getAppMonitorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAppMonitorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAppMonitorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAppMonitor");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAppMonitorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the raw performance events that RUM has collected from your web application, so that you can do your
* own processing or analysis of this data.
*
*
* @param getAppMonitorDataRequest
* @return Result of the GetAppMonitorData operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.GetAppMonitorData
* @see AWS API
* Documentation
*/
@Override
public GetAppMonitorDataResult getAppMonitorData(GetAppMonitorDataRequest request) {
request = beforeClientExecution(request);
return executeGetAppMonitorData(request);
}
@SdkInternalApi
final GetAppMonitorDataResult executeGetAppMonitorData(GetAppMonitorDataRequest getAppMonitorDataRequest) {
ExecutionContext executionContext = createExecutionContext(getAppMonitorDataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAppMonitorDataRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAppMonitorDataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAppMonitorData");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAppMonitorDataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the Amazon CloudWatch RUM app monitors in the account.
*
*
* @param listAppMonitorsRequest
* @return Result of the ListAppMonitors operation returned by the service.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.ListAppMonitors
* @see AWS API
* Documentation
*/
@Override
public ListAppMonitorsResult listAppMonitors(ListAppMonitorsRequest request) {
request = beforeClientExecution(request);
return executeListAppMonitors(request);
}
@SdkInternalApi
final ListAppMonitorsResult executeListAppMonitors(ListAppMonitorsRequest listAppMonitorsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppMonitorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppMonitorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAppMonitorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppMonitors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAppMonitorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of destinations that you have created to receive RUM extended metrics, for the specified app
* monitor.
*
*
* For more information about extended metrics, see AddRumMetrics.
*
*
* @param listRumMetricsDestinationsRequest
* @return Result of the ListRumMetricsDestinations operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.ListRumMetricsDestinations
* @see AWS
* API Documentation
*/
@Override
public ListRumMetricsDestinationsResult listRumMetricsDestinations(ListRumMetricsDestinationsRequest request) {
request = beforeClientExecution(request);
return executeListRumMetricsDestinations(request);
}
@SdkInternalApi
final ListRumMetricsDestinationsResult executeListRumMetricsDestinations(ListRumMetricsDestinationsRequest listRumMetricsDestinationsRequest) {
ExecutionContext executionContext = createExecutionContext(listRumMetricsDestinationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRumMetricsDestinationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRumMetricsDestinationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRumMetricsDestinations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRumMetricsDestinationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays the tags associated with a CloudWatch RUM resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @sample AWSCloudWatchRUM.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends telemetry events about your application performance and user behavior to CloudWatch RUM. The code snippet
* that RUM generates for you to add to your application includes PutRumEvents
operations to send this
* data to RUM.
*
*
* Each PutRumEvents
operation can send a batch of events from one user session.
*
*
* @param putRumEventsRequest
* @return Result of the PutRumEvents operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.PutRumEvents
* @see AWS API
* Documentation
*/
@Override
public PutRumEventsResult putRumEvents(PutRumEventsRequest request) {
request = beforeClientExecution(request);
return executePutRumEvents(request);
}
@SdkInternalApi
final PutRumEventsResult executePutRumEvents(PutRumEventsRequest putRumEventsRequest) {
ExecutionContext executionContext = createExecutionContext(putRumEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutRumEventsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putRumEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutRumEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "dataplane.";
String resolvedHostPrefix = String.format("dataplane.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutRumEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or updates a destination to receive extended metrics from CloudWatch RUM. You can send extended metrics
* to CloudWatch or to a CloudWatch Evidently experiment.
*
*
* For more information about extended metrics, see BatchCreateRumMetricDefinitions.
*
*
* @param putRumMetricsDestinationRequest
* @return Result of the PutRumMetricsDestination operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.PutRumMetricsDestination
* @see AWS
* API Documentation
*/
@Override
public PutRumMetricsDestinationResult putRumMetricsDestination(PutRumMetricsDestinationRequest request) {
request = beforeClientExecution(request);
return executePutRumMetricsDestination(request);
}
@SdkInternalApi
final PutRumMetricsDestinationResult executePutRumMetricsDestination(PutRumMetricsDestinationRequest putRumMetricsDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(putRumMetricsDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutRumMetricsDestinationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putRumMetricsDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutRumMetricsDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutRumMetricsDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch RUM resource. Currently, the only
* resources that can be tagged app monitors.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with a resource that already has tags. If you specify a new tag
* key for the resource, this tag is appended to the list of tags associated with the alarm. If you specify a tag
* key that is already associated with the resource, the new tag value that you specify replaces the previous value
* for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* For more information, see Tagging Amazon
* Web Services resources.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @sample AWSCloudWatchRUM.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @sample AWSCloudWatchRUM.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the configuration of an existing app monitor. When you use this operation, only the parts of the app
* monitor configuration that you specify in this operation are changed. For any parameters that you omit, the
* existing values are kept.
*
*
* You can't use this operation to change the tags of an existing app monitor. To change the tags of an existing app
* monitor, use TagResource.
*
*
* To create a new app monitor, use CreateAppMonitor.
*
*
* After you update an app monitor, sign in to the CloudWatch RUM console to get the updated JavaScript code snippet
* to add to your web application. For more information, see How
* do I find a code snippet that I've already generated?
*
*
* @param updateAppMonitorRequest
* @return Result of the UpdateAppMonitor operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.UpdateAppMonitor
* @see AWS API
* Documentation
*/
@Override
public UpdateAppMonitorResult updateAppMonitor(UpdateAppMonitorRequest request) {
request = beforeClientExecution(request);
return executeUpdateAppMonitor(request);
}
@SdkInternalApi
final UpdateAppMonitorResult executeUpdateAppMonitor(UpdateAppMonitorRequest updateAppMonitorRequest) {
ExecutionContext executionContext = createExecutionContext(updateAppMonitorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAppMonitorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAppMonitorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAppMonitor");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateAppMonitorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies one existing metric definition for CloudWatch RUM extended metrics. For more information about extended
* metrics, see BatchCreateRumMetricsDefinitions.
*
*
* @param updateRumMetricDefinitionRequest
* @return Result of the UpdateRumMetricDefinition operation returned by the service.
* @throws ConflictException
* This operation attempted to create a resource that already exists.
* @throws ServiceQuotaExceededException
* This request exceeds a service quota.
* @throws ResourceNotFoundException
* Resource not found.
* @throws InternalServerException
* Internal service exception.
* @throws ValidationException
* One of the arguments for the request is not valid.
* @throws ThrottlingException
* The request was throttled because of quota limits.
* @throws AccessDeniedException
* You don't have sufficient permissions to perform this action.
* @sample AWSCloudWatchRUM.UpdateRumMetricDefinition
* @see AWS
* API Documentation
*/
@Override
public UpdateRumMetricDefinitionResult updateRumMetricDefinition(UpdateRumMetricDefinitionRequest request) {
request = beforeClientExecution(request);
return executeUpdateRumMetricDefinition(request);
}
@SdkInternalApi
final UpdateRumMetricDefinitionResult executeUpdateRumMetricDefinition(UpdateRumMetricDefinitionRequest updateRumMetricDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(updateRumMetricDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRumMetricDefinitionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateRumMetricDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RUM");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRumMetricDefinition");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateRumMetricDefinitionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}