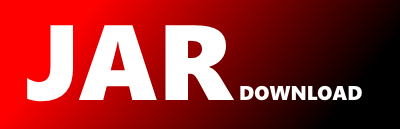
com.amazonaws.services.cloudwatchrum.model.AppMonitorConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudwatchrum Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudwatchrum.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* This structure contains much of the configuration data for the app monitor.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AppMonitorConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user cookie.
* The cookies allow the RUM web client to collect data relating to the number of users an application has and the
* behavior of the application across a sequence of events. Cookies are stored in the top-level domain of the
* current page.
*
*/
private Boolean allowCookies;
/**
*
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples. RUM adds
* an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP requests. You
* can see traces and segments from these user sessions in the X-Ray console and the CloudWatch ServiceLens console.
* For more information, see What is
* X-Ray?
*
*/
private Boolean enableXRay;
/**
*
* A list of URLs in your website or application to exclude from RUM data collection.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*/
private java.util.List excludedPages;
/**
*
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*
*/
private java.util.List favoritePages;
/**
*
* The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to authorize the
* sending of data to RUM.
*
*
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this can
* happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new identity
* pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor
* response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that for your
* app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
*
*/
private String guestRoleArn;
/**
*
* The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
*
*/
private String identityPoolId;
/**
*
* If this app monitor is to collect data from only certain pages in your application, this structure lists those
* pages.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*/
private java.util.List includedPages;
/**
*
* Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives you more
* data but also incurs more costs.
*
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are sampled, and
* setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
*
*/
private Double sessionSampleRate;
/**
*
* An array that lists the types of telemetry data that this app monitor is to collect.
*
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
*
*/
private java.util.List telemetries;
/**
*
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user cookie.
* The cookies allow the RUM web client to collect data relating to the number of users an application has and the
* behavior of the application across a sequence of events. Cookies are stored in the top-level domain of the
* current page.
*
*
* @param allowCookies
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user
* cookie. The cookies allow the RUM web client to collect data relating to the number of users an
* application has and the behavior of the application across a sequence of events. Cookies are stored in the
* top-level domain of the current page.
*/
public void setAllowCookies(Boolean allowCookies) {
this.allowCookies = allowCookies;
}
/**
*
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user cookie.
* The cookies allow the RUM web client to collect data relating to the number of users an application has and the
* behavior of the application across a sequence of events. Cookies are stored in the top-level domain of the
* current page.
*
*
* @return If you set this to true
, the RUM web client sets two cookies, a session cookie and a user
* cookie. The cookies allow the RUM web client to collect data relating to the number of users an
* application has and the behavior of the application across a sequence of events. Cookies are stored in
* the top-level domain of the current page.
*/
public Boolean getAllowCookies() {
return this.allowCookies;
}
/**
*
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user cookie.
* The cookies allow the RUM web client to collect data relating to the number of users an application has and the
* behavior of the application across a sequence of events. Cookies are stored in the top-level domain of the
* current page.
*
*
* @param allowCookies
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user
* cookie. The cookies allow the RUM web client to collect data relating to the number of users an
* application has and the behavior of the application across a sequence of events. Cookies are stored in the
* top-level domain of the current page.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withAllowCookies(Boolean allowCookies) {
setAllowCookies(allowCookies);
return this;
}
/**
*
* If you set this to true
, the RUM web client sets two cookies, a session cookie and a user cookie.
* The cookies allow the RUM web client to collect data relating to the number of users an application has and the
* behavior of the application across a sequence of events. Cookies are stored in the top-level domain of the
* current page.
*
*
* @return If you set this to true
, the RUM web client sets two cookies, a session cookie and a user
* cookie. The cookies allow the RUM web client to collect data relating to the number of users an
* application has and the behavior of the application across a sequence of events. Cookies are stored in
* the top-level domain of the current page.
*/
public Boolean isAllowCookies() {
return this.allowCookies;
}
/**
*
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples. RUM adds
* an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP requests. You
* can see traces and segments from these user sessions in the X-Ray console and the CloudWatch ServiceLens console.
* For more information, see What is
* X-Ray?
*
*
* @param enableXRay
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples.
* RUM adds an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP
* requests. You can see traces and segments from these user sessions in the X-Ray console and the CloudWatch
* ServiceLens console. For more information, see What is X-Ray?
*/
public void setEnableXRay(Boolean enableXRay) {
this.enableXRay = enableXRay;
}
/**
*
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples. RUM adds
* an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP requests. You
* can see traces and segments from these user sessions in the X-Ray console and the CloudWatch ServiceLens console.
* For more information, see What is
* X-Ray?
*
*
* @return If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples.
* RUM adds an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed
* HTTP requests. You can see traces and segments from these user sessions in the X-Ray console and the
* CloudWatch ServiceLens console. For more information, see What is X-Ray?
*/
public Boolean getEnableXRay() {
return this.enableXRay;
}
/**
*
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples. RUM adds
* an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP requests. You
* can see traces and segments from these user sessions in the X-Ray console and the CloudWatch ServiceLens console.
* For more information, see What is
* X-Ray?
*
*
* @param enableXRay
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples.
* RUM adds an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP
* requests. You can see traces and segments from these user sessions in the X-Ray console and the CloudWatch
* ServiceLens console. For more information, see What is X-Ray?
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withEnableXRay(Boolean enableXRay) {
setEnableXRay(enableXRay);
return this;
}
/**
*
* If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples. RUM adds
* an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed HTTP requests. You
* can see traces and segments from these user sessions in the X-Ray console and the CloudWatch ServiceLens console.
* For more information, see What is
* X-Ray?
*
*
* @return If you set this to true
, RUM enables X-Ray tracing for the user sessions that RUM samples.
* RUM adds an X-Ray trace header to allowed HTTP requests. It also records an X-Ray segment for allowed
* HTTP requests. You can see traces and segments from these user sessions in the X-Ray console and the
* CloudWatch ServiceLens console. For more information, see What is X-Ray?
*/
public Boolean isEnableXRay() {
return this.enableXRay;
}
/**
*
* A list of URLs in your website or application to exclude from RUM data collection.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* @return A list of URLs in your website or application to exclude from RUM data collection.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*/
public java.util.List getExcludedPages() {
return excludedPages;
}
/**
*
* A list of URLs in your website or application to exclude from RUM data collection.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* @param excludedPages
* A list of URLs in your website or application to exclude from RUM data collection.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*/
public void setExcludedPages(java.util.Collection excludedPages) {
if (excludedPages == null) {
this.excludedPages = null;
return;
}
this.excludedPages = new java.util.ArrayList(excludedPages);
}
/**
*
* A list of URLs in your website or application to exclude from RUM data collection.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExcludedPages(java.util.Collection)} or {@link #withExcludedPages(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param excludedPages
* A list of URLs in your website or application to exclude from RUM data collection.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withExcludedPages(String... excludedPages) {
if (this.excludedPages == null) {
setExcludedPages(new java.util.ArrayList(excludedPages.length));
}
for (String ele : excludedPages) {
this.excludedPages.add(ele);
}
return this;
}
/**
*
* A list of URLs in your website or application to exclude from RUM data collection.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* @param excludedPages
* A list of URLs in your website or application to exclude from RUM data collection.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withExcludedPages(java.util.Collection excludedPages) {
setExcludedPages(excludedPages);
return this;
}
/**
*
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*
*
* @return A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*/
public java.util.List getFavoritePages() {
return favoritePages;
}
/**
*
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*
*
* @param favoritePages
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*/
public void setFavoritePages(java.util.Collection favoritePages) {
if (favoritePages == null) {
this.favoritePages = null;
return;
}
this.favoritePages = new java.util.ArrayList(favoritePages);
}
/**
*
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFavoritePages(java.util.Collection)} or {@link #withFavoritePages(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param favoritePages
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withFavoritePages(String... favoritePages) {
if (this.favoritePages == null) {
setFavoritePages(new java.util.ArrayList(favoritePages.length));
}
for (String ele : favoritePages) {
this.favoritePages.add(ele);
}
return this;
}
/**
*
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
*
*
* @param favoritePages
* A list of pages in your application that are to be displayed with a "favorite" icon in the CloudWatch RUM
* console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withFavoritePages(java.util.Collection favoritePages) {
setFavoritePages(favoritePages);
return this;
}
/**
*
* The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to authorize the
* sending of data to RUM.
*
*
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this can
* happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new identity
* pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor
* response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that for your
* app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
*
*
* @param guestRoleArn
* The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to
* authorize the sending of data to RUM.
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this
* can happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new
* identity pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that
* for your app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
*/
public void setGuestRoleArn(String guestRoleArn) {
this.guestRoleArn = guestRoleArn;
}
/**
*
* The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to authorize the
* sending of data to RUM.
*
*
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this can
* happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new identity
* pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor
* response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that for your
* app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
*
*
* @return The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to
* authorize the sending of data to RUM.
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this
* can happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new
* identity pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that
* for your app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
*/
public String getGuestRoleArn() {
return this.guestRoleArn;
}
/**
*
* The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to authorize the
* sending of data to RUM.
*
*
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this can
* happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new identity
* pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor
* response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that for your
* app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
*
*
* @param guestRoleArn
* The ARN of the guest IAM role that is attached to the Amazon Cognito identity pool that is used to
* authorize the sending of data to RUM.
*
* It is possible that an app monitor does not have a value for GuestRoleArn
. For example, this
* can happen when you use the console to create an app monitor and you allow CloudWatch RUM to create a new
* identity pool for Authorization. In this case, GuestRoleArn
is not present in the GetAppMonitor response because it is not stored by the service.
*
*
* If this issue affects you, you can take one of the following steps:
*
*
* -
*
* Use the Cloud Development Kit (CDK) to create an identity pool and the associated IAM role, and use that
* for your app monitor.
*
*
* -
*
* Make a separate GetIdentityPoolRoles call to Amazon Cognito to retrieve the GuestRoleArn
.
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withGuestRoleArn(String guestRoleArn) {
setGuestRoleArn(guestRoleArn);
return this;
}
/**
*
* The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
*
*
* @param identityPoolId
* The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
*/
public void setIdentityPoolId(String identityPoolId) {
this.identityPoolId = identityPoolId;
}
/**
*
* The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
*
*
* @return The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
*/
public String getIdentityPoolId() {
return this.identityPoolId;
}
/**
*
* The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
*
*
* @param identityPoolId
* The ID of the Amazon Cognito identity pool that is used to authorize the sending of data to RUM.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withIdentityPoolId(String identityPoolId) {
setIdentityPoolId(identityPoolId);
return this;
}
/**
*
* If this app monitor is to collect data from only certain pages in your application, this structure lists those
* pages.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* @return If this app monitor is to collect data from only certain pages in your application, this structure lists
* those pages.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*/
public java.util.List getIncludedPages() {
return includedPages;
}
/**
*
* If this app monitor is to collect data from only certain pages in your application, this structure lists those
* pages.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* @param includedPages
* If this app monitor is to collect data from only certain pages in your application, this structure lists
* those pages.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*/
public void setIncludedPages(java.util.Collection includedPages) {
if (includedPages == null) {
this.includedPages = null;
return;
}
this.includedPages = new java.util.ArrayList(includedPages);
}
/**
*
* If this app monitor is to collect data from only certain pages in your application, this structure lists those
* pages.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludedPages(java.util.Collection)} or {@link #withIncludedPages(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param includedPages
* If this app monitor is to collect data from only certain pages in your application, this structure lists
* those pages.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withIncludedPages(String... includedPages) {
if (this.includedPages == null) {
setIncludedPages(new java.util.ArrayList(includedPages.length));
}
for (String ele : includedPages) {
this.includedPages.add(ele);
}
return this;
}
/**
*
* If this app monitor is to collect data from only certain pages in your application, this structure lists those
* pages.
*
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
*
*
* @param includedPages
* If this app monitor is to collect data from only certain pages in your application, this structure lists
* those pages.
*
* You can't include both ExcludedPages
and IncludedPages
in the same operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withIncludedPages(java.util.Collection includedPages) {
setIncludedPages(includedPages);
return this;
}
/**
*
* Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives you more
* data but also incurs more costs.
*
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are sampled, and
* setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
*
*
* @param sessionSampleRate
* Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives you
* more data but also incurs more costs.
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are
* sampled, and setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
*/
public void setSessionSampleRate(Double sessionSampleRate) {
this.sessionSampleRate = sessionSampleRate;
}
/**
*
* Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives you more
* data but also incurs more costs.
*
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are sampled, and
* setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
*
*
* @return Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives
* you more data but also incurs more costs.
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are
* sampled, and setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
*/
public Double getSessionSampleRate() {
return this.sessionSampleRate;
}
/**
*
* Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives you more
* data but also incurs more costs.
*
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are sampled, and
* setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
*
*
* @param sessionSampleRate
* Specifies the portion of user sessions to use for RUM data collection. Choosing a higher portion gives you
* more data but also incurs more costs.
*
* The range for this value is 0 to 1 inclusive. Setting this to 1 means that 100% of user sessions are
* sampled, and setting it to 0.1 means that 10% of user sessions are sampled.
*
*
* If you omit this parameter, the default of 0.1 is used, and 10% of sessions will be sampled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppMonitorConfiguration withSessionSampleRate(Double sessionSampleRate) {
setSessionSampleRate(sessionSampleRate);
return this;
}
/**
*
* An array that lists the types of telemetry data that this app monitor is to collect.
*
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
*
*
* @return An array that lists the types of telemetry data that this app monitor is to collect.
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
* @see Telemetry
*/
public java.util.List getTelemetries() {
return telemetries;
}
/**
*
* An array that lists the types of telemetry data that this app monitor is to collect.
*
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
*
*
* @param telemetries
* An array that lists the types of telemetry data that this app monitor is to collect.
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
* @see Telemetry
*/
public void setTelemetries(java.util.Collection telemetries) {
if (telemetries == null) {
this.telemetries = null;
return;
}
this.telemetries = new java.util.ArrayList(telemetries);
}
/**
*
* An array that lists the types of telemetry data that this app monitor is to collect.
*
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTelemetries(java.util.Collection)} or {@link #withTelemetries(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param telemetries
* An array that lists the types of telemetry data that this app monitor is to collect.
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Telemetry
*/
public AppMonitorConfiguration withTelemetries(String... telemetries) {
if (this.telemetries == null) {
setTelemetries(new java.util.ArrayList(telemetries.length));
}
for (String ele : telemetries) {
this.telemetries.add(ele);
}
return this;
}
/**
*
* An array that lists the types of telemetry data that this app monitor is to collect.
*
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
*
*
* @param telemetries
* An array that lists the types of telemetry data that this app monitor is to collect.
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Telemetry
*/
public AppMonitorConfiguration withTelemetries(java.util.Collection telemetries) {
setTelemetries(telemetries);
return this;
}
/**
*
* An array that lists the types of telemetry data that this app monitor is to collect.
*
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
*
*
* @param telemetries
* An array that lists the types of telemetry data that this app monitor is to collect.
*
* -
*
* errors
indicates that RUM collects data about unhandled JavaScript errors raised by your
* application.
*
*
* -
*
* performance
indicates that RUM collects performance data about how your application and its
* resources are loaded and rendered. This includes Core Web Vitals.
*
*
* -
*
* http
indicates that RUM collects data about HTTP errors thrown by your application.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Telemetry
*/
public AppMonitorConfiguration withTelemetries(Telemetry... telemetries) {
java.util.ArrayList telemetriesCopy = new java.util.ArrayList(telemetries.length);
for (Telemetry value : telemetries) {
telemetriesCopy.add(value.toString());
}
if (getTelemetries() == null) {
setTelemetries(telemetriesCopy);
} else {
getTelemetries().addAll(telemetriesCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAllowCookies() != null)
sb.append("AllowCookies: ").append(getAllowCookies()).append(",");
if (getEnableXRay() != null)
sb.append("EnableXRay: ").append(getEnableXRay()).append(",");
if (getExcludedPages() != null)
sb.append("ExcludedPages: ").append(getExcludedPages()).append(",");
if (getFavoritePages() != null)
sb.append("FavoritePages: ").append(getFavoritePages()).append(",");
if (getGuestRoleArn() != null)
sb.append("GuestRoleArn: ").append(getGuestRoleArn()).append(",");
if (getIdentityPoolId() != null)
sb.append("IdentityPoolId: ").append(getIdentityPoolId()).append(",");
if (getIncludedPages() != null)
sb.append("IncludedPages: ").append(getIncludedPages()).append(",");
if (getSessionSampleRate() != null)
sb.append("SessionSampleRate: ").append(getSessionSampleRate()).append(",");
if (getTelemetries() != null)
sb.append("Telemetries: ").append(getTelemetries());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AppMonitorConfiguration == false)
return false;
AppMonitorConfiguration other = (AppMonitorConfiguration) obj;
if (other.getAllowCookies() == null ^ this.getAllowCookies() == null)
return false;
if (other.getAllowCookies() != null && other.getAllowCookies().equals(this.getAllowCookies()) == false)
return false;
if (other.getEnableXRay() == null ^ this.getEnableXRay() == null)
return false;
if (other.getEnableXRay() != null && other.getEnableXRay().equals(this.getEnableXRay()) == false)
return false;
if (other.getExcludedPages() == null ^ this.getExcludedPages() == null)
return false;
if (other.getExcludedPages() != null && other.getExcludedPages().equals(this.getExcludedPages()) == false)
return false;
if (other.getFavoritePages() == null ^ this.getFavoritePages() == null)
return false;
if (other.getFavoritePages() != null && other.getFavoritePages().equals(this.getFavoritePages()) == false)
return false;
if (other.getGuestRoleArn() == null ^ this.getGuestRoleArn() == null)
return false;
if (other.getGuestRoleArn() != null && other.getGuestRoleArn().equals(this.getGuestRoleArn()) == false)
return false;
if (other.getIdentityPoolId() == null ^ this.getIdentityPoolId() == null)
return false;
if (other.getIdentityPoolId() != null && other.getIdentityPoolId().equals(this.getIdentityPoolId()) == false)
return false;
if (other.getIncludedPages() == null ^ this.getIncludedPages() == null)
return false;
if (other.getIncludedPages() != null && other.getIncludedPages().equals(this.getIncludedPages()) == false)
return false;
if (other.getSessionSampleRate() == null ^ this.getSessionSampleRate() == null)
return false;
if (other.getSessionSampleRate() != null && other.getSessionSampleRate().equals(this.getSessionSampleRate()) == false)
return false;
if (other.getTelemetries() == null ^ this.getTelemetries() == null)
return false;
if (other.getTelemetries() != null && other.getTelemetries().equals(this.getTelemetries()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAllowCookies() == null) ? 0 : getAllowCookies().hashCode());
hashCode = prime * hashCode + ((getEnableXRay() == null) ? 0 : getEnableXRay().hashCode());
hashCode = prime * hashCode + ((getExcludedPages() == null) ? 0 : getExcludedPages().hashCode());
hashCode = prime * hashCode + ((getFavoritePages() == null) ? 0 : getFavoritePages().hashCode());
hashCode = prime * hashCode + ((getGuestRoleArn() == null) ? 0 : getGuestRoleArn().hashCode());
hashCode = prime * hashCode + ((getIdentityPoolId() == null) ? 0 : getIdentityPoolId().hashCode());
hashCode = prime * hashCode + ((getIncludedPages() == null) ? 0 : getIncludedPages().hashCode());
hashCode = prime * hashCode + ((getSessionSampleRate() == null) ? 0 : getSessionSampleRate().hashCode());
hashCode = prime * hashCode + ((getTelemetries() == null) ? 0 : getTelemetries().hashCode());
return hashCode;
}
@Override
public AppMonitorConfiguration clone() {
try {
return (AppMonitorConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.cloudwatchrum.model.transform.AppMonitorConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}