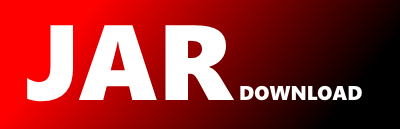
com.amazonaws.services.codebuild.AWSCodeBuild Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.codebuild;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.codebuild.model.*;
/**
* Interface for accessing AWS CodeBuild.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.codebuild.AbstractAWSCodeBuild} instead.
*
*
* CodeBuild
*
* CodeBuild is a fully managed build service in the cloud. CodeBuild compiles your source code, runs unit tests, and
* produces artifacts that are ready to deploy. CodeBuild eliminates the need to provision, manage, and scale your own
* build servers. It provides prepackaged build environments for the most popular programming languages and build tools,
* such as Apache Maven, Gradle, and more. You can also fully customize build environments in CodeBuild to use your own
* build tools. CodeBuild scales automatically to meet peak build requests. You pay only for the build time you consume.
* For more information about CodeBuild, see the CodeBuild User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSCodeBuild {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "codebuild";
/**
* Overrides the default endpoint for this client ("codebuild.us-east-1.amazonaws.com"). Callers can use this method
* to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "codebuild.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "codebuild.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol
* from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "codebuild.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "codebuild.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate
* with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSCodeBuild#setEndpoint(String)}, sets the regional endpoint for this client's service
* calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Deletes one or more builds.
*
*
* @param batchDeleteBuildsRequest
* @return Result of the BatchDeleteBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchDeleteBuilds
* @see AWS
* API Documentation
*/
BatchDeleteBuildsResult batchDeleteBuilds(BatchDeleteBuildsRequest batchDeleteBuildsRequest);
/**
*
* Retrieves information about one or more batch builds.
*
*
* @param batchGetBuildBatchesRequest
* @return Result of the BatchGetBuildBatches operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchGetBuildBatches
* @see AWS
* API Documentation
*/
BatchGetBuildBatchesResult batchGetBuildBatches(BatchGetBuildBatchesRequest batchGetBuildBatchesRequest);
/**
*
* Gets information about one or more builds.
*
*
* @param batchGetBuildsRequest
* @return Result of the BatchGetBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchGetBuilds
* @see AWS API
* Documentation
*/
BatchGetBuildsResult batchGetBuilds(BatchGetBuildsRequest batchGetBuildsRequest);
/**
*
* Gets information about one or more compute fleets.
*
*
* @param batchGetFleetsRequest
* @return Result of the BatchGetFleets operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchGetFleets
* @see AWS API
* Documentation
*/
BatchGetFleetsResult batchGetFleets(BatchGetFleetsRequest batchGetFleetsRequest);
/**
*
* Gets information about one or more build projects.
*
*
* @param batchGetProjectsRequest
* @return Result of the BatchGetProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchGetProjects
* @see AWS API
* Documentation
*/
BatchGetProjectsResult batchGetProjects(BatchGetProjectsRequest batchGetProjectsRequest);
/**
*
* Returns an array of report groups.
*
*
* @param batchGetReportGroupsRequest
* @return Result of the BatchGetReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchGetReportGroups
* @see AWS
* API Documentation
*/
BatchGetReportGroupsResult batchGetReportGroups(BatchGetReportGroupsRequest batchGetReportGroupsRequest);
/**
*
* Returns an array of reports.
*
*
* @param batchGetReportsRequest
* @return Result of the BatchGetReports operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.BatchGetReports
* @see AWS API
* Documentation
*/
BatchGetReportsResult batchGetReports(BatchGetReportsRequest batchGetReportsRequest);
/**
*
* Creates a compute fleet.
*
*
* @param createFleetRequest
* @return Result of the CreateFleet operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified Amazon Web Services resource cannot be created, because an Amazon Web Services resource
* with the same settings already exists.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @sample AWSCodeBuild.CreateFleet
* @see AWS API
* Documentation
*/
CreateFleetResult createFleet(CreateFleetRequest createFleetRequest);
/**
*
* Creates a build project.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified Amazon Web Services resource cannot be created, because an Amazon Web Services resource
* with the same settings already exists.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @sample AWSCodeBuild.CreateProject
* @see AWS API
* Documentation
*/
CreateProjectResult createProject(CreateProjectRequest createProjectRequest);
/**
*
* Creates a report group. A report group contains a collection of reports.
*
*
* @param createReportGroupRequest
* @return Result of the CreateReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceAlreadyExistsException
* The specified Amazon Web Services resource cannot be created, because an Amazon Web Services resource
* with the same settings already exists.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @sample AWSCodeBuild.CreateReportGroup
* @see AWS
* API Documentation
*/
CreateReportGroupResult createReportGroup(CreateReportGroupRequest createReportGroupRequest);
/**
*
* For an existing CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* enables CodeBuild to start rebuilding the source code every time a code change is pushed to the repository.
*
*
*
* If you enable webhooks for an CodeBuild project, and the project is used as a build step in CodePipeline, then
* two identical builds are created for each commit. One build is triggered through webhooks, and one through
* CodePipeline. Because billing is on a per-build basis, you are billed for both builds. Therefore, if you are
* using CodePipeline, we recommend that you disable webhooks in CodeBuild. In the CodeBuild console, clear the
* Webhook box. For more information, see step 5 in Change a
* Build Project's Settings.
*
*
*
* @param createWebhookRequest
* @return Result of the CreateWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @throws ResourceAlreadyExistsException
* The specified Amazon Web Services resource cannot be created, because an Amazon Web Services resource
* with the same settings already exists.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.CreateWebhook
* @see AWS API
* Documentation
*/
CreateWebhookResult createWebhook(CreateWebhookRequest createWebhookRequest);
/**
*
* Deletes a batch build.
*
*
* @param deleteBuildBatchRequest
* @return Result of the DeleteBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DeleteBuildBatch
* @see AWS API
* Documentation
*/
DeleteBuildBatchResult deleteBuildBatch(DeleteBuildBatchRequest deleteBuildBatchRequest);
/**
*
* Deletes a compute fleet. When you delete a compute fleet, its builds are not deleted.
*
*
* @param deleteFleetRequest
* @return Result of the DeleteFleet operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DeleteFleet
* @see AWS API
* Documentation
*/
DeleteFleetResult deleteFleet(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes a build project. When you delete a project, its builds are not deleted.
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DeleteProject
* @see AWS API
* Documentation
*/
DeleteProjectResult deleteProject(DeleteProjectRequest deleteProjectRequest);
/**
*
* Deletes a report.
*
*
* @param deleteReportRequest
* @return Result of the DeleteReport operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DeleteReport
* @see AWS API
* Documentation
*/
DeleteReportResult deleteReport(DeleteReportRequest deleteReportRequest);
/**
*
* Deletes a report group. Before you delete a report group, you must delete its reports.
*
*
* @param deleteReportGroupRequest
* @return Result of the DeleteReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DeleteReportGroup
* @see AWS
* API Documentation
*/
DeleteReportGroupResult deleteReportGroup(DeleteReportGroupRequest deleteReportGroupRequest);
/**
*
* Deletes a resource policy that is identified by its resource ARN.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes a set of GitHub, GitHub Enterprise, or Bitbucket source credentials.
*
*
* @param deleteSourceCredentialsRequest
* @return Result of the DeleteSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.DeleteSourceCredentials
* @see AWS API Documentation
*/
DeleteSourceCredentialsResult deleteSourceCredentials(DeleteSourceCredentialsRequest deleteSourceCredentialsRequest);
/**
*
* For an existing CodeBuild build project that has its source code stored in a GitHub or Bitbucket repository,
* stops CodeBuild from rebuilding the source code every time a code change is pushed to the repository.
*
*
* @param deleteWebhookRequest
* @return Result of the DeleteWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @sample AWSCodeBuild.DeleteWebhook
* @see AWS API
* Documentation
*/
DeleteWebhookResult deleteWebhook(DeleteWebhookRequest deleteWebhookRequest);
/**
*
* Retrieves one or more code coverage reports.
*
*
* @param describeCodeCoveragesRequest
* @return Result of the DescribeCodeCoverages operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.DescribeCodeCoverages
* @see AWS API Documentation
*/
DescribeCodeCoveragesResult describeCodeCoverages(DescribeCodeCoveragesRequest describeCodeCoveragesRequest);
/**
*
* Returns a list of details about test cases for a report.
*
*
* @param describeTestCasesRequest
* @return Result of the DescribeTestCases operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.DescribeTestCases
* @see AWS
* API Documentation
*/
DescribeTestCasesResult describeTestCases(DescribeTestCasesRequest describeTestCasesRequest);
/**
*
* Analyzes and accumulates test report values for the specified test reports.
*
*
* @param getReportGroupTrendRequest
* @return Result of the GetReportGroupTrend operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.GetReportGroupTrend
* @see AWS
* API Documentation
*/
GetReportGroupTrendResult getReportGroupTrend(GetReportGroupTrendRequest getReportGroupTrendRequest);
/**
*
* Gets a resource policy that is identified by its resource ARN.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.GetResourcePolicy
* @see AWS
* API Documentation
*/
GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest);
/**
*
* Imports the source repository credentials for an CodeBuild project that has its source code stored in a GitHub,
* GitHub Enterprise, or Bitbucket repository.
*
*
* @param importSourceCredentialsRequest
* @return Result of the ImportSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @throws ResourceAlreadyExistsException
* The specified Amazon Web Services resource cannot be created, because an Amazon Web Services resource
* with the same settings already exists.
* @sample AWSCodeBuild.ImportSourceCredentials
* @see AWS API Documentation
*/
ImportSourceCredentialsResult importSourceCredentials(ImportSourceCredentialsRequest importSourceCredentialsRequest);
/**
*
* Resets the cache for a project.
*
*
* @param invalidateProjectCacheRequest
* @return Result of the InvalidateProjectCache operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.InvalidateProjectCache
* @see AWS API Documentation
*/
InvalidateProjectCacheResult invalidateProjectCache(InvalidateProjectCacheRequest invalidateProjectCacheRequest);
/**
*
* Retrieves the identifiers of your build batches in the current region.
*
*
* @param listBuildBatchesRequest
* @return Result of the ListBuildBatches operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListBuildBatches
* @see AWS API
* Documentation
*/
ListBuildBatchesResult listBuildBatches(ListBuildBatchesRequest listBuildBatchesRequest);
/**
*
* Retrieves the identifiers of the build batches for a specific project.
*
*
* @param listBuildBatchesForProjectRequest
* @return Result of the ListBuildBatchesForProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.ListBuildBatchesForProject
* @see AWS API Documentation
*/
ListBuildBatchesForProjectResult listBuildBatchesForProject(ListBuildBatchesForProjectRequest listBuildBatchesForProjectRequest);
/**
*
* Gets a list of build IDs, with each build ID representing a single build.
*
*
* @param listBuildsRequest
* @return Result of the ListBuilds operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListBuilds
* @see AWS API
* Documentation
*/
ListBuildsResult listBuilds(ListBuildsRequest listBuildsRequest);
/**
*
* Gets a list of build identifiers for the specified build project, with each build identifier representing a
* single build.
*
*
* @param listBuildsForProjectRequest
* @return Result of the ListBuildsForProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.ListBuildsForProject
* @see AWS
* API Documentation
*/
ListBuildsForProjectResult listBuildsForProject(ListBuildsForProjectRequest listBuildsForProjectRequest);
/**
*
* Gets information about Docker images that are managed by CodeBuild.
*
*
* @param listCuratedEnvironmentImagesRequest
* @return Result of the ListCuratedEnvironmentImages operation returned by the service.
* @sample AWSCodeBuild.ListCuratedEnvironmentImages
* @see AWS API Documentation
*/
ListCuratedEnvironmentImagesResult listCuratedEnvironmentImages(ListCuratedEnvironmentImagesRequest listCuratedEnvironmentImagesRequest);
/**
*
* Gets a list of compute fleet names with each compute fleet name representing a single compute fleet.
*
*
* @param listFleetsRequest
* @return Result of the ListFleets operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListFleets
* @see AWS API
* Documentation
*/
ListFleetsResult listFleets(ListFleetsRequest listFleetsRequest);
/**
*
* Gets a list of build project names, with each build project name representing a single build project.
*
*
* @param listProjectsRequest
* @return Result of the ListProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListProjects
* @see AWS API
* Documentation
*/
ListProjectsResult listProjects(ListProjectsRequest listProjectsRequest);
/**
*
* Gets a list ARNs for the report groups in the current Amazon Web Services account.
*
*
* @param listReportGroupsRequest
* @return Result of the ListReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListReportGroups
* @see AWS API
* Documentation
*/
ListReportGroupsResult listReportGroups(ListReportGroupsRequest listReportGroupsRequest);
/**
*
* Returns a list of ARNs for the reports in the current Amazon Web Services account.
*
*
* @param listReportsRequest
* @return Result of the ListReports operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListReports
* @see AWS API
* Documentation
*/
ListReportsResult listReports(ListReportsRequest listReportsRequest);
/**
*
* Returns a list of ARNs for the reports that belong to a ReportGroup
.
*
*
* @param listReportsForReportGroupRequest
* @return Result of the ListReportsForReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.ListReportsForReportGroup
* @see AWS API Documentation
*/
ListReportsForReportGroupResult listReportsForReportGroup(ListReportsForReportGroupRequest listReportsForReportGroupRequest);
/**
*
* Gets a list of projects that are shared with other Amazon Web Services accounts or users.
*
*
* @param listSharedProjectsRequest
* @return Result of the ListSharedProjects operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListSharedProjects
* @see AWS
* API Documentation
*/
ListSharedProjectsResult listSharedProjects(ListSharedProjectsRequest listSharedProjectsRequest);
/**
*
* Gets a list of report groups that are shared with other Amazon Web Services accounts or users.
*
*
* @param listSharedReportGroupsRequest
* @return Result of the ListSharedReportGroups operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListSharedReportGroups
* @see AWS API Documentation
*/
ListSharedReportGroupsResult listSharedReportGroups(ListSharedReportGroupsRequest listSharedReportGroupsRequest);
/**
*
* Returns a list of SourceCredentialsInfo
objects.
*
*
* @param listSourceCredentialsRequest
* @return Result of the ListSourceCredentials operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.ListSourceCredentials
* @see AWS API Documentation
*/
ListSourceCredentialsResult listSourceCredentials(ListSourceCredentialsRequest listSourceCredentialsRequest);
/**
*
* Stores a resource policy for the ARN of a Project
or ReportGroup
object.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @sample AWSCodeBuild.PutResourcePolicy
* @see AWS
* API Documentation
*/
PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Restarts a build.
*
*
* @param retryBuildRequest
* @return Result of the RetryBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @sample AWSCodeBuild.RetryBuild
* @see AWS API
* Documentation
*/
RetryBuildResult retryBuild(RetryBuildRequest retryBuildRequest);
/**
*
* Restarts a failed batch build. Only batch builds that have failed can be retried.
*
*
* @param retryBuildBatchRequest
* @return Result of the RetryBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.RetryBuildBatch
* @see AWS API
* Documentation
*/
RetryBuildBatchResult retryBuildBatch(RetryBuildBatchRequest retryBuildBatchRequest);
/**
*
* Starts running a build with the settings defined in the project. These setting include: how to run a build, where
* to get the source code, which build environment to use, which build commands to run, and where to store the build
* output.
*
*
* You can also start a build run by overriding some of the build settings in the project. The overrides only apply
* for that specific start build request. The settings in the project are unaltered.
*
*
* @param startBuildRequest
* @return Result of the StartBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @sample AWSCodeBuild.StartBuild
* @see AWS API
* Documentation
*/
StartBuildResult startBuild(StartBuildRequest startBuildRequest);
/**
*
* Starts a batch build for a project.
*
*
* @param startBuildBatchRequest
* @return Result of the StartBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.StartBuildBatch
* @see AWS API
* Documentation
*/
StartBuildBatchResult startBuildBatch(StartBuildBatchRequest startBuildBatchRequest);
/**
*
* Attempts to stop running a build.
*
*
* @param stopBuildRequest
* @return Result of the StopBuild operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.StopBuild
* @see AWS API
* Documentation
*/
StopBuildResult stopBuild(StopBuildRequest stopBuildRequest);
/**
*
* Stops a running batch build.
*
*
* @param stopBuildBatchRequest
* @return Result of the StopBuildBatch operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.StopBuildBatch
* @see AWS API
* Documentation
*/
StopBuildBatchResult stopBuildBatch(StopBuildBatchRequest stopBuildBatchRequest);
/**
*
* Updates a compute fleet.
*
*
* @param updateFleetRequest
* @return Result of the UpdateFleet operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws AccountLimitExceededException
* An Amazon Web Services service limit was exceeded for the calling Amazon Web Services account.
* @sample AWSCodeBuild.UpdateFleet
* @see AWS API
* Documentation
*/
UpdateFleetResult updateFleet(UpdateFleetRequest updateFleetRequest);
/**
*
* Changes the settings of a build project.
*
*
* @param updateProjectRequest
* @return Result of the UpdateProject operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.UpdateProject
* @see AWS API
* Documentation
*/
UpdateProjectResult updateProject(UpdateProjectRequest updateProjectRequest);
/**
*
* Changes the public visibility for a project. The project's build results, logs, and artifacts are available to
* the general public. For more information, see Public build projects in the
* CodeBuild User Guide.
*
*
*
* The following should be kept in mind when making your projects public:
*
*
* -
*
* All of a project's build results, logs, and artifacts, including builds that were run when the project was
* private, are available to the general public.
*
*
* -
*
* All build logs and artifacts are available to the public. Environment variables, source code, and other sensitive
* information may have been output to the build logs and artifacts. You must be careful about what information is
* output to the build logs. Some best practice are:
*
*
* -
*
* Do not store sensitive values in environment variables. We recommend that you use an Amazon EC2 Systems Manager
* Parameter Store or Secrets Manager to store sensitive values.
*
*
* -
*
* Follow Best
* practices for using webhooks in the CodeBuild User Guide to limit which entities can trigger a build,
* and do not store the buildspec in the project itself, to ensure that your webhooks are as secure as possible.
*
*
*
*
* -
*
* A malicious user can use public builds to distribute malicious artifacts. We recommend that you review all pull
* requests to verify that the pull request is a legitimate change. We also recommend that you validate any
* artifacts with their checksums to make sure that the correct artifacts are being downloaded.
*
*
*
*
*
* @param updateProjectVisibilityRequest
* @return Result of the UpdateProjectVisibility operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.UpdateProjectVisibility
* @see AWS API Documentation
*/
UpdateProjectVisibilityResult updateProjectVisibility(UpdateProjectVisibilityRequest updateProjectVisibilityRequest);
/**
*
* Updates a report group.
*
*
* @param updateReportGroupRequest
* @return Result of the UpdateReportGroup operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @sample AWSCodeBuild.UpdateReportGroup
* @see AWS
* API Documentation
*/
UpdateReportGroupResult updateReportGroup(UpdateReportGroupRequest updateReportGroupRequest);
/**
*
* Updates the webhook associated with an CodeBuild build project.
*
*
*
* If you use Bitbucket for your repository, rotateSecret
is ignored.
*
*
*
* @param updateWebhookRequest
* @return Result of the UpdateWebhook operation returned by the service.
* @throws InvalidInputException
* The input value that was provided is not valid.
* @throws ResourceNotFoundException
* The specified Amazon Web Services resource cannot be found.
* @throws OAuthProviderException
* There was a problem with the underlying OAuth provider.
* @sample AWSCodeBuild.UpdateWebhook
* @see AWS API
* Documentation
*/
UpdateWebhookResult updateWebhook(UpdateWebhookRequest updateWebhookRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}