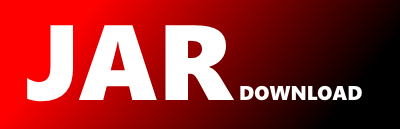
com.amazonaws.services.codecommit.AWSCodeCommitAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codecommit Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.codecommit;
import com.amazonaws.services.codecommit.model.*;
/**
* Interface for accessing CodeCommit asynchronously. Each asynchronous method
* will return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS CodeCommit
*
* This is the AWS CodeCommit API Reference. This reference provides
* descriptions of the operations and data types for AWS CodeCommit API.
*
*
* You can use the AWS CodeCommit API to work with the following objects:
*
*
* - Repositories, by calling the following:
*
* - BatchGetRepositories, which returns information about one or more
* repositories associated with your AWS account
* - CreateRepository, which creates an AWS CodeCommit repository
* - DeleteRepository, which deletes an AWS CodeCommit repository
* - GetRepository, which returns information about a specified
* repository
* - ListRepositories, which lists all AWS CodeCommit repositories
* associated with your AWS account
* - UpdateRepositoryDescription, which sets or updates the description
* of the repository
* - UpdateRepositoryName, which changes the name of the repository. If
* you change the name of a repository, no other users of that repository will
* be able to access it until you send them the new HTTPS or SSH URL to use.
*
*
* - Branches, by calling the following:
*
* - CreateBranch, which creates a new branch in a specified repository
*
* - GetBranch, which returns information about a specified branch
* - ListBranches, which lists all branches for a specified repository
* - UpdateDefaultBranch, which changes the default branch for a
* repository
*
*
* - Information about committed code in a repository, by calling the
* following:
*
* - GetCommit, which returns information about a commit, including
* commit messages and committer information.
*
*
* - Triggers, by calling the following:
*
* - GetRepositoryTriggers, which returns information about triggers
* configured for a repository
* - PutRepositoryTriggers, which replaces all triggers for a
* repository and can be used to create or delete triggers
* - TestRepositoryTriggers, which tests the functionality of a
* repository trigger by sending data to the trigger target
*
*
*
*
* For information about how to use AWS CodeCommit, see the AWS CodeCommit User Guide.
*
*/
public interface AWSCodeCommitAsync extends AWSCodeCommit {
/**
*
* Returns information about one or more repositories.
*
*
*
* The description field for a repository accepts all HTML characters and
* all valid Unicode characters. Applications that do not HTML-encode the
* description and display it in a web page could expose users to
* potentially malicious code. Make sure that you HTML-encode the
* description field in any application that uses this API to display the
* repository description on a web page.
*
*
*
* @param batchGetRepositoriesRequest
* Represents the input of a batch get repositories operation.
* @return A Java Future containing the result of the BatchGetRepositories
* operation returned by the service.
* @sample AWSCodeCommitAsync.BatchGetRepositories
*/
java.util.concurrent.Future batchGetRepositoriesAsync(
BatchGetRepositoriesRequest batchGetRepositoriesRequest);
/**
*
* Returns information about one or more repositories.
*
*
*
* The description field for a repository accepts all HTML characters and
* all valid Unicode characters. Applications that do not HTML-encode the
* description and display it in a web page could expose users to
* potentially malicious code. Make sure that you HTML-encode the
* description field in any application that uses this API to display the
* repository description on a web page.
*
*
*
* @param batchGetRepositoriesRequest
* Represents the input of a batch get repositories operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetRepositories
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.BatchGetRepositories
*/
java.util.concurrent.Future batchGetRepositoriesAsync(
BatchGetRepositoriesRequest batchGetRepositoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new branch in a repository and points the branch to a commit.
*
*
*
* Calling the create branch operation does not set a repository's default
* branch. To do this, call the update default branch operation.
*
*
*
* @param createBranchRequest
* Represents the input of a create branch operation.
* @return A Java Future containing the result of the CreateBranch operation
* returned by the service.
* @sample AWSCodeCommitAsync.CreateBranch
*/
java.util.concurrent.Future createBranchAsync(
CreateBranchRequest createBranchRequest);
/**
*
* Creates a new branch in a repository and points the branch to a commit.
*
*
*
* Calling the create branch operation does not set a repository's default
* branch. To do this, call the update default branch operation.
*
*
*
* @param createBranchRequest
* Represents the input of a create branch operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBranch operation
* returned by the service.
* @sample AWSCodeCommitAsyncHandler.CreateBranch
*/
java.util.concurrent.Future createBranchAsync(
CreateBranchRequest createBranchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new, empty repository.
*
*
* @param createRepositoryRequest
* Represents the input of a create repository operation.
* @return A Java Future containing the result of the CreateRepository
* operation returned by the service.
* @sample AWSCodeCommitAsync.CreateRepository
*/
java.util.concurrent.Future createRepositoryAsync(
CreateRepositoryRequest createRepositoryRequest);
/**
*
* Creates a new, empty repository.
*
*
* @param createRepositoryRequest
* Represents the input of a create repository operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRepository
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.CreateRepository
*/
java.util.concurrent.Future createRepositoryAsync(
CreateRepositoryRequest createRepositoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a repository. If a specified repository was already deleted, a
* null repository ID will be returned.
*
* Deleting a repository also deletes all associated objects and
* metadata. After a repository is deleted, all future push calls to the
* deleted repository will fail.
*
* @param deleteRepositoryRequest
* Represents the input of a delete repository operation.
* @return A Java Future containing the result of the DeleteRepository
* operation returned by the service.
* @sample AWSCodeCommitAsync.DeleteRepository
*/
java.util.concurrent.Future deleteRepositoryAsync(
DeleteRepositoryRequest deleteRepositoryRequest);
/**
*
* Deletes a repository. If a specified repository was already deleted, a
* null repository ID will be returned.
*
* Deleting a repository also deletes all associated objects and
* metadata. After a repository is deleted, all future push calls to the
* deleted repository will fail.
*
* @param deleteRepositoryRequest
* Represents the input of a delete repository operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRepository
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.DeleteRepository
*/
java.util.concurrent.Future deleteRepositoryAsync(
DeleteRepositoryRequest deleteRepositoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a repository branch, including its name and the
* last commit ID.
*
*
* @param getBranchRequest
* Represents the input of a get branch operation.
* @return A Java Future containing the result of the GetBranch operation
* returned by the service.
* @sample AWSCodeCommitAsync.GetBranch
*/
java.util.concurrent.Future getBranchAsync(
GetBranchRequest getBranchRequest);
/**
*
* Returns information about a repository branch, including its name and the
* last commit ID.
*
*
* @param getBranchRequest
* Represents the input of a get branch operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBranch operation
* returned by the service.
* @sample AWSCodeCommitAsyncHandler.GetBranch
*/
java.util.concurrent.Future getBranchAsync(
GetBranchRequest getBranchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a commit, including commit message and
* committer information.
*
*
* @param getCommitRequest
* Represents the input of a get commit operation.
* @return A Java Future containing the result of the GetCommit operation
* returned by the service.
* @sample AWSCodeCommitAsync.GetCommit
*/
java.util.concurrent.Future getCommitAsync(
GetCommitRequest getCommitRequest);
/**
*
* Returns information about a commit, including commit message and
* committer information.
*
*
* @param getCommitRequest
* Represents the input of a get commit operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCommit operation
* returned by the service.
* @sample AWSCodeCommitAsyncHandler.GetCommit
*/
java.util.concurrent.Future getCommitAsync(
GetCommitRequest getCommitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a repository.
*
*
*
* The description field for a repository accepts all HTML characters and
* all valid Unicode characters. Applications that do not HTML-encode the
* description and display it in a web page could expose users to
* potentially malicious code. Make sure that you HTML-encode the
* description field in any application that uses this API to display the
* repository description on a web page.
*
*
*
* @param getRepositoryRequest
* Represents the input of a get repository operation.
* @return A Java Future containing the result of the GetRepository
* operation returned by the service.
* @sample AWSCodeCommitAsync.GetRepository
*/
java.util.concurrent.Future getRepositoryAsync(
GetRepositoryRequest getRepositoryRequest);
/**
*
* Returns information about a repository.
*
*
*
* The description field for a repository accepts all HTML characters and
* all valid Unicode characters. Applications that do not HTML-encode the
* description and display it in a web page could expose users to
* potentially malicious code. Make sure that you HTML-encode the
* description field in any application that uses this API to display the
* repository description on a web page.
*
*
*
* @param getRepositoryRequest
* Represents the input of a get repository operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRepository
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.GetRepository
*/
java.util.concurrent.Future getRepositoryAsync(
GetRepositoryRequest getRepositoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about triggers configured for a repository.
*
*
* @param getRepositoryTriggersRequest
* Represents the input of a get repository triggers operation.
* @return A Java Future containing the result of the GetRepositoryTriggers
* operation returned by the service.
* @sample AWSCodeCommitAsync.GetRepositoryTriggers
*/
java.util.concurrent.Future getRepositoryTriggersAsync(
GetRepositoryTriggersRequest getRepositoryTriggersRequest);
/**
*
* Gets information about triggers configured for a repository.
*
*
* @param getRepositoryTriggersRequest
* Represents the input of a get repository triggers operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRepositoryTriggers
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.GetRepositoryTriggers
*/
java.util.concurrent.Future getRepositoryTriggersAsync(
GetRepositoryTriggersRequest getRepositoryTriggersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more branches in a repository.
*
*
* @param listBranchesRequest
* Represents the input of a list branches operation.
* @return A Java Future containing the result of the ListBranches operation
* returned by the service.
* @sample AWSCodeCommitAsync.ListBranches
*/
java.util.concurrent.Future listBranchesAsync(
ListBranchesRequest listBranchesRequest);
/**
*
* Gets information about one or more branches in a repository.
*
*
* @param listBranchesRequest
* Represents the input of a list branches operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBranches operation
* returned by the service.
* @sample AWSCodeCommitAsyncHandler.ListBranches
*/
java.util.concurrent.Future listBranchesAsync(
ListBranchesRequest listBranchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more repositories.
*
*
* @param listRepositoriesRequest
* Represents the input of a list repositories operation.
* @return A Java Future containing the result of the ListRepositories
* operation returned by the service.
* @sample AWSCodeCommitAsync.ListRepositories
*/
java.util.concurrent.Future listRepositoriesAsync(
ListRepositoriesRequest listRepositoriesRequest);
/**
*
* Gets information about one or more repositories.
*
*
* @param listRepositoriesRequest
* Represents the input of a list repositories operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRepositories
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.ListRepositories
*/
java.util.concurrent.Future listRepositoriesAsync(
ListRepositoriesRequest listRepositoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Replaces all triggers for a repository. This can be used to create or
* delete triggers.
*
*
* @param putRepositoryTriggersRequest
* Represents the input ofa put repository triggers operation.
* @return A Java Future containing the result of the PutRepositoryTriggers
* operation returned by the service.
* @sample AWSCodeCommitAsync.PutRepositoryTriggers
*/
java.util.concurrent.Future putRepositoryTriggersAsync(
PutRepositoryTriggersRequest putRepositoryTriggersRequest);
/**
*
* Replaces all triggers for a repository. This can be used to create or
* delete triggers.
*
*
* @param putRepositoryTriggersRequest
* Represents the input ofa put repository triggers operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRepositoryTriggers
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.PutRepositoryTriggers
*/
java.util.concurrent.Future putRepositoryTriggersAsync(
PutRepositoryTriggersRequest putRepositoryTriggersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tests the functionality of repository triggers by sending information to
* the trigger target. If real data is available in the repository, the test
* will send data from the last commit. If no data is available, sample data
* will be generated.
*
*
* @param testRepositoryTriggersRequest
* Represents the input of a test repository triggers operation.
* @return A Java Future containing the result of the TestRepositoryTriggers
* operation returned by the service.
* @sample AWSCodeCommitAsync.TestRepositoryTriggers
*/
java.util.concurrent.Future testRepositoryTriggersAsync(
TestRepositoryTriggersRequest testRepositoryTriggersRequest);
/**
*
* Tests the functionality of repository triggers by sending information to
* the trigger target. If real data is available in the repository, the test
* will send data from the last commit. If no data is available, sample data
* will be generated.
*
*
* @param testRepositoryTriggersRequest
* Represents the input of a test repository triggers operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TestRepositoryTriggers
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.TestRepositoryTriggers
*/
java.util.concurrent.Future testRepositoryTriggersAsync(
TestRepositoryTriggersRequest testRepositoryTriggersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets or changes the default branch name for the specified repository.
*
*
*
* If you use this operation to change the default branch name to the
* current default branch name, a success message is returned even though
* the default branch did not change.
*
*
*
* @param updateDefaultBranchRequest
* Represents the input of an update default branch operation.
* @return A Java Future containing the result of the UpdateDefaultBranch
* operation returned by the service.
* @sample AWSCodeCommitAsync.UpdateDefaultBranch
*/
java.util.concurrent.Future updateDefaultBranchAsync(
UpdateDefaultBranchRequest updateDefaultBranchRequest);
/**
*
* Sets or changes the default branch name for the specified repository.
*
*
*
* If you use this operation to change the default branch name to the
* current default branch name, a success message is returned even though
* the default branch did not change.
*
*
*
* @param updateDefaultBranchRequest
* Represents the input of an update default branch operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDefaultBranch
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.UpdateDefaultBranch
*/
java.util.concurrent.Future updateDefaultBranchAsync(
UpdateDefaultBranchRequest updateDefaultBranchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets or changes the comment or description for a repository.
*
*
*
* The description field for a repository accepts all HTML characters and
* all valid Unicode characters. Applications that do not HTML-encode the
* description and display it in a web page could expose users to
* potentially malicious code. Make sure that you HTML-encode the
* description field in any application that uses this API to display the
* repository description on a web page.
*
*
*
* @param updateRepositoryDescriptionRequest
* Represents the input of an update repository description
* operation.
* @return A Java Future containing the result of the
* UpdateRepositoryDescription operation returned by the service.
* @sample AWSCodeCommitAsync.UpdateRepositoryDescription
*/
java.util.concurrent.Future updateRepositoryDescriptionAsync(
UpdateRepositoryDescriptionRequest updateRepositoryDescriptionRequest);
/**
*
* Sets or changes the comment or description for a repository.
*
*
*
* The description field for a repository accepts all HTML characters and
* all valid Unicode characters. Applications that do not HTML-encode the
* description and display it in a web page could expose users to
* potentially malicious code. Make sure that you HTML-encode the
* description field in any application that uses this API to display the
* repository description on a web page.
*
*
*
* @param updateRepositoryDescriptionRequest
* Represents the input of an update repository description
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* UpdateRepositoryDescription operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.UpdateRepositoryDescription
*/
java.util.concurrent.Future updateRepositoryDescriptionAsync(
UpdateRepositoryDescriptionRequest updateRepositoryDescriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Renames a repository. The repository name must be unique across the
* calling AWS account. In addition, repository names are limited to 100
* alphanumeric, dash, and underscore characters, and cannot include certain
* characters. The suffix ".git" is prohibited. For a full description of
* the limits on repository names, see Limits in the AWS CodeCommit User Guide.
*
*
* @param updateRepositoryNameRequest
* Represents the input of an update repository description
* operation.
* @return A Java Future containing the result of the UpdateRepositoryName
* operation returned by the service.
* @sample AWSCodeCommitAsync.UpdateRepositoryName
*/
java.util.concurrent.Future updateRepositoryNameAsync(
UpdateRepositoryNameRequest updateRepositoryNameRequest);
/**
*
* Renames a repository. The repository name must be unique across the
* calling AWS account. In addition, repository names are limited to 100
* alphanumeric, dash, and underscore characters, and cannot include certain
* characters. The suffix ".git" is prohibited. For a full description of
* the limits on repository names, see Limits in the AWS CodeCommit User Guide.
*
*
* @param updateRepositoryNameRequest
* Represents the input of an update repository description
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRepositoryName
* operation returned by the service.
* @sample AWSCodeCommitAsyncHandler.UpdateRepositoryName
*/
java.util.concurrent.Future updateRepositoryNameAsync(
UpdateRepositoryNameRequest updateRepositoryNameRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}