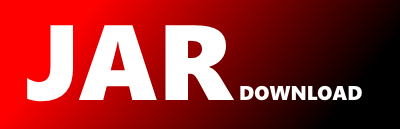
com.amazonaws.services.codecommit.model.RepositoryMetadata Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codecommit Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.codecommit.model;
import java.io.Serializable;
/**
*
* Information about a repository.
*
*/
public class RepositoryMetadata implements Serializable, Cloneable {
/**
*
* The ID of the AWS account associated with the repository.
*
*/
private String accountId;
/**
*
* The ID of the repository.
*
*/
private String repositoryId;
/**
*
* The repository's name.
*
*/
private String repositoryName;
/**
*
* A comment or description about the repository.
*
*/
private String repositoryDescription;
/**
*
* The repository's default branch name.
*
*/
private String defaultBranch;
/**
*
* The date and time the repository was last modified, in timestamp format.
*
*/
private java.util.Date lastModifiedDate;
/**
*
* The date and time the repository was created, in timestamp format.
*
*/
private java.util.Date creationDate;
/**
*
* The URL to use for cloning the repository over HTTPS.
*
*/
private String cloneUrlHttp;
/**
*
* The URL to use for cloning the repository over SSH.
*
*/
private String cloneUrlSsh;
/**
*
* The Amazon Resource Name (ARN) of the repository.
*
*/
private String arn;
/**
*
* The ID of the AWS account associated with the repository.
*
*
* @param accountId
* The ID of the AWS account associated with the repository.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The ID of the AWS account associated with the repository.
*
*
* @return The ID of the AWS account associated with the repository.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The ID of the AWS account associated with the repository.
*
*
* @param accountId
* The ID of the AWS account associated with the repository.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The ID of the repository.
*
*
* @param repositoryId
* The ID of the repository.
*/
public void setRepositoryId(String repositoryId) {
this.repositoryId = repositoryId;
}
/**
*
* The ID of the repository.
*
*
* @return The ID of the repository.
*/
public String getRepositoryId() {
return this.repositoryId;
}
/**
*
* The ID of the repository.
*
*
* @param repositoryId
* The ID of the repository.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withRepositoryId(String repositoryId) {
setRepositoryId(repositoryId);
return this;
}
/**
*
* The repository's name.
*
*
* @param repositoryName
* The repository's name.
*/
public void setRepositoryName(String repositoryName) {
this.repositoryName = repositoryName;
}
/**
*
* The repository's name.
*
*
* @return The repository's name.
*/
public String getRepositoryName() {
return this.repositoryName;
}
/**
*
* The repository's name.
*
*
* @param repositoryName
* The repository's name.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withRepositoryName(String repositoryName) {
setRepositoryName(repositoryName);
return this;
}
/**
*
* A comment or description about the repository.
*
*
* @param repositoryDescription
* A comment or description about the repository.
*/
public void setRepositoryDescription(String repositoryDescription) {
this.repositoryDescription = repositoryDescription;
}
/**
*
* A comment or description about the repository.
*
*
* @return A comment or description about the repository.
*/
public String getRepositoryDescription() {
return this.repositoryDescription;
}
/**
*
* A comment or description about the repository.
*
*
* @param repositoryDescription
* A comment or description about the repository.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withRepositoryDescription(
String repositoryDescription) {
setRepositoryDescription(repositoryDescription);
return this;
}
/**
*
* The repository's default branch name.
*
*
* @param defaultBranch
* The repository's default branch name.
*/
public void setDefaultBranch(String defaultBranch) {
this.defaultBranch = defaultBranch;
}
/**
*
* The repository's default branch name.
*
*
* @return The repository's default branch name.
*/
public String getDefaultBranch() {
return this.defaultBranch;
}
/**
*
* The repository's default branch name.
*
*
* @param defaultBranch
* The repository's default branch name.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withDefaultBranch(String defaultBranch) {
setDefaultBranch(defaultBranch);
return this;
}
/**
*
* The date and time the repository was last modified, in timestamp format.
*
*
* @param lastModifiedDate
* The date and time the repository was last modified, in timestamp
* format.
*/
public void setLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
*
* The date and time the repository was last modified, in timestamp format.
*
*
* @return The date and time the repository was last modified, in timestamp
* format.
*/
public java.util.Date getLastModifiedDate() {
return this.lastModifiedDate;
}
/**
*
* The date and time the repository was last modified, in timestamp format.
*
*
* @param lastModifiedDate
* The date and time the repository was last modified, in timestamp
* format.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withLastModifiedDate(
java.util.Date lastModifiedDate) {
setLastModifiedDate(lastModifiedDate);
return this;
}
/**
*
* The date and time the repository was created, in timestamp format.
*
*
* @param creationDate
* The date and time the repository was created, in timestamp format.
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The date and time the repository was created, in timestamp format.
*
*
* @return The date and time the repository was created, in timestamp
* format.
*/
public java.util.Date getCreationDate() {
return this.creationDate;
}
/**
*
* The date and time the repository was created, in timestamp format.
*
*
* @param creationDate
* The date and time the repository was created, in timestamp format.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withCreationDate(java.util.Date creationDate) {
setCreationDate(creationDate);
return this;
}
/**
*
* The URL to use for cloning the repository over HTTPS.
*
*
* @param cloneUrlHttp
* The URL to use for cloning the repository over HTTPS.
*/
public void setCloneUrlHttp(String cloneUrlHttp) {
this.cloneUrlHttp = cloneUrlHttp;
}
/**
*
* The URL to use for cloning the repository over HTTPS.
*
*
* @return The URL to use for cloning the repository over HTTPS.
*/
public String getCloneUrlHttp() {
return this.cloneUrlHttp;
}
/**
*
* The URL to use for cloning the repository over HTTPS.
*
*
* @param cloneUrlHttp
* The URL to use for cloning the repository over HTTPS.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withCloneUrlHttp(String cloneUrlHttp) {
setCloneUrlHttp(cloneUrlHttp);
return this;
}
/**
*
* The URL to use for cloning the repository over SSH.
*
*
* @param cloneUrlSsh
* The URL to use for cloning the repository over SSH.
*/
public void setCloneUrlSsh(String cloneUrlSsh) {
this.cloneUrlSsh = cloneUrlSsh;
}
/**
*
* The URL to use for cloning the repository over SSH.
*
*
* @return The URL to use for cloning the repository over SSH.
*/
public String getCloneUrlSsh() {
return this.cloneUrlSsh;
}
/**
*
* The URL to use for cloning the repository over SSH.
*
*
* @param cloneUrlSsh
* The URL to use for cloning the repository over SSH.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withCloneUrlSsh(String cloneUrlSsh) {
setCloneUrlSsh(cloneUrlSsh);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the repository.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the repository.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the repository.
*
*
* @return The Amazon Resource Name (ARN) of the repository.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the repository.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the repository.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RepositoryMetadata withArn(String arn) {
setArn(arn);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountId() != null)
sb.append("AccountId: " + getAccountId() + ",");
if (getRepositoryId() != null)
sb.append("RepositoryId: " + getRepositoryId() + ",");
if (getRepositoryName() != null)
sb.append("RepositoryName: " + getRepositoryName() + ",");
if (getRepositoryDescription() != null)
sb.append("RepositoryDescription: " + getRepositoryDescription()
+ ",");
if (getDefaultBranch() != null)
sb.append("DefaultBranch: " + getDefaultBranch() + ",");
if (getLastModifiedDate() != null)
sb.append("LastModifiedDate: " + getLastModifiedDate() + ",");
if (getCreationDate() != null)
sb.append("CreationDate: " + getCreationDate() + ",");
if (getCloneUrlHttp() != null)
sb.append("CloneUrlHttp: " + getCloneUrlHttp() + ",");
if (getCloneUrlSsh() != null)
sb.append("CloneUrlSsh: " + getCloneUrlSsh() + ",");
if (getArn() != null)
sb.append("Arn: " + getArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RepositoryMetadata == false)
return false;
RepositoryMetadata other = (RepositoryMetadata) obj;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null
&& other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getRepositoryId() == null ^ this.getRepositoryId() == null)
return false;
if (other.getRepositoryId() != null
&& other.getRepositoryId().equals(this.getRepositoryId()) == false)
return false;
if (other.getRepositoryName() == null
^ this.getRepositoryName() == null)
return false;
if (other.getRepositoryName() != null
&& other.getRepositoryName().equals(this.getRepositoryName()) == false)
return false;
if (other.getRepositoryDescription() == null
^ this.getRepositoryDescription() == null)
return false;
if (other.getRepositoryDescription() != null
&& other.getRepositoryDescription().equals(
this.getRepositoryDescription()) == false)
return false;
if (other.getDefaultBranch() == null ^ this.getDefaultBranch() == null)
return false;
if (other.getDefaultBranch() != null
&& other.getDefaultBranch().equals(this.getDefaultBranch()) == false)
return false;
if (other.getLastModifiedDate() == null
^ this.getLastModifiedDate() == null)
return false;
if (other.getLastModifiedDate() != null
&& other.getLastModifiedDate().equals(
this.getLastModifiedDate()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null
&& other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getCloneUrlHttp() == null ^ this.getCloneUrlHttp() == null)
return false;
if (other.getCloneUrlHttp() != null
&& other.getCloneUrlHttp().equals(this.getCloneUrlHttp()) == false)
return false;
if (other.getCloneUrlSsh() == null ^ this.getCloneUrlSsh() == null)
return false;
if (other.getCloneUrlSsh() != null
&& other.getCloneUrlSsh().equals(this.getCloneUrlSsh()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null
&& other.getArn().equals(this.getArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime
* hashCode
+ ((getRepositoryId() == null) ? 0 : getRepositoryId()
.hashCode());
hashCode = prime
* hashCode
+ ((getRepositoryName() == null) ? 0 : getRepositoryName()
.hashCode());
hashCode = prime
* hashCode
+ ((getRepositoryDescription() == null) ? 0
: getRepositoryDescription().hashCode());
hashCode = prime
* hashCode
+ ((getDefaultBranch() == null) ? 0 : getDefaultBranch()
.hashCode());
hashCode = prime
* hashCode
+ ((getLastModifiedDate() == null) ? 0 : getLastModifiedDate()
.hashCode());
hashCode = prime
* hashCode
+ ((getCreationDate() == null) ? 0 : getCreationDate()
.hashCode());
hashCode = prime
* hashCode
+ ((getCloneUrlHttp() == null) ? 0 : getCloneUrlHttp()
.hashCode());
hashCode = prime
* hashCode
+ ((getCloneUrlSsh() == null) ? 0 : getCloneUrlSsh().hashCode());
hashCode = prime * hashCode
+ ((getArn() == null) ? 0 : getArn().hashCode());
return hashCode;
}
@Override
public RepositoryMetadata clone() {
try {
return (RepositoryMetadata) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}