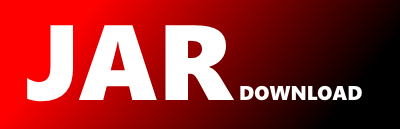
com.amazonaws.services.codedeploy.AmazonCodeDeployAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.codedeploy;
import javax.annotation.Generated;
import com.amazonaws.services.codedeploy.model.*;
/**
* Interface for accessing CodeDeploy asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.codedeploy.AbstractAmazonCodeDeployAsync} instead.
*
*
* AWS CodeDeploy
*
* AWS CodeDeploy is a deployment service that automates application deployments to Amazon EC2 instances or on-premises
* instances running in your own facility.
*
*
* You can deploy a nearly unlimited variety of application content, such as code, web and configuration files,
* executables, packages, scripts, multimedia files, and so on. AWS CodeDeploy can deploy application content stored in
* Amazon S3 buckets, GitHub repositories, or Bitbucket repositories. You do not need to make changes to your existing
* code before you can use AWS CodeDeploy.
*
*
* AWS CodeDeploy makes it easier for you to rapidly release new features, helps you avoid downtime during application
* deployment, and handles the complexity of updating your applications, without many of the risks associated with
* error-prone manual deployments.
*
*
* AWS CodeDeploy Components
*
*
* Use the information in this guide to help you work with the following AWS CodeDeploy components:
*
*
* -
*
* Application: A name that uniquely identifies the application you want to deploy. AWS CodeDeploy uses this
* name, which functions as a container, to ensure the correct combination of revision, deployment configuration, and
* deployment group are referenced during a deployment.
*
*
* -
*
* Deployment group: A set of individual instances. A deployment group contains individually tagged instances,
* Amazon EC2 instances in Auto Scaling groups, or both.
*
*
* -
*
* Deployment configuration: A set of deployment rules and deployment success and failure conditions used by AWS
* CodeDeploy during a deployment.
*
*
* -
*
* Deployment: The process, and the components involved in the process, of installing content on one or more
* instances.
*
*
* -
*
* Application revisions: An archive file containing source content—source code, web pages, executable files, and
* deployment scripts—along with an application specification file (AppSpec file). Revisions are stored in Amazon S3
* buckets or GitHub repositories. For Amazon S3, a revision is uniquely identified by its Amazon S3 object key and its
* ETag, version, or both. For GitHub, a revision is uniquely identified by its commit ID.
*
*
*
*
* This guide also contains information to help you get details about the instances in your deployments and to make
* on-premises instances available for AWS CodeDeploy deployments.
*
*
* AWS CodeDeploy Information Resources
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCodeDeployAsync extends AmazonCodeDeploy {
/**
*
* Adds tags to on-premises instances.
*
*
* @param addTagsToOnPremisesInstancesRequest
* Represents the input of, and adds tags to, an on-premises instance operation.
* @return A Java Future containing the result of the AddTagsToOnPremisesInstances operation returned by the
* service.
* @sample AmazonCodeDeployAsync.AddTagsToOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future addTagsToOnPremisesInstancesAsync(
AddTagsToOnPremisesInstancesRequest addTagsToOnPremisesInstancesRequest);
/**
*
* Adds tags to on-premises instances.
*
*
* @param addTagsToOnPremisesInstancesRequest
* Represents the input of, and adds tags to, an on-premises instance operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToOnPremisesInstances operation returned by the
* service.
* @sample AmazonCodeDeployAsyncHandler.AddTagsToOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future addTagsToOnPremisesInstancesAsync(
AddTagsToOnPremisesInstancesRequest addTagsToOnPremisesInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more application revisions.
*
*
* @param batchGetApplicationRevisionsRequest
* Represents the input of a BatchGetApplicationRevisions operation.
* @return A Java Future containing the result of the BatchGetApplicationRevisions operation returned by the
* service.
* @sample AmazonCodeDeployAsync.BatchGetApplicationRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetApplicationRevisionsAsync(
BatchGetApplicationRevisionsRequest batchGetApplicationRevisionsRequest);
/**
*
* Gets information about one or more application revisions.
*
*
* @param batchGetApplicationRevisionsRequest
* Represents the input of a BatchGetApplicationRevisions operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetApplicationRevisions operation returned by the
* service.
* @sample AmazonCodeDeployAsyncHandler.BatchGetApplicationRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetApplicationRevisionsAsync(
BatchGetApplicationRevisionsRequest batchGetApplicationRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more applications.
*
*
* @param batchGetApplicationsRequest
* Represents the input of a BatchGetApplications operation.
* @return A Java Future containing the result of the BatchGetApplications operation returned by the service.
* @sample AmazonCodeDeployAsync.BatchGetApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetApplicationsAsync(BatchGetApplicationsRequest batchGetApplicationsRequest);
/**
*
* Gets information about one or more applications.
*
*
* @param batchGetApplicationsRequest
* Represents the input of a BatchGetApplications operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetApplications operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.BatchGetApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetApplicationsAsync(BatchGetApplicationsRequest batchGetApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the BatchGetApplications operation.
*
* @see #batchGetApplicationsAsync(BatchGetApplicationsRequest)
*/
java.util.concurrent.Future batchGetApplicationsAsync();
/**
* Simplified method form for invoking the BatchGetApplications operation with an AsyncHandler.
*
* @see #batchGetApplicationsAsync(BatchGetApplicationsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future batchGetApplicationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more deployment groups.
*
*
* @param batchGetDeploymentGroupsRequest
* Represents the input of a BatchGetDeploymentGroups operation.
* @return A Java Future containing the result of the BatchGetDeploymentGroups operation returned by the service.
* @sample AmazonCodeDeployAsync.BatchGetDeploymentGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetDeploymentGroupsAsync(BatchGetDeploymentGroupsRequest batchGetDeploymentGroupsRequest);
/**
*
* Gets information about one or more deployment groups.
*
*
* @param batchGetDeploymentGroupsRequest
* Represents the input of a BatchGetDeploymentGroups operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetDeploymentGroups operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.BatchGetDeploymentGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetDeploymentGroupsAsync(BatchGetDeploymentGroupsRequest batchGetDeploymentGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more instance that are part of a deployment group.
*
*
* @param batchGetDeploymentInstancesRequest
* Represents the input of a BatchGetDeploymentInstances operation.
* @return A Java Future containing the result of the BatchGetDeploymentInstances operation returned by the service.
* @sample AmazonCodeDeployAsync.BatchGetDeploymentInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetDeploymentInstancesAsync(
BatchGetDeploymentInstancesRequest batchGetDeploymentInstancesRequest);
/**
*
* Gets information about one or more instance that are part of a deployment group.
*
*
* @param batchGetDeploymentInstancesRequest
* Represents the input of a BatchGetDeploymentInstances operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetDeploymentInstances operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.BatchGetDeploymentInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetDeploymentInstancesAsync(
BatchGetDeploymentInstancesRequest batchGetDeploymentInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more deployments.
*
*
* @param batchGetDeploymentsRequest
* Represents the input of a BatchGetDeployments operation.
* @return A Java Future containing the result of the BatchGetDeployments operation returned by the service.
* @sample AmazonCodeDeployAsync.BatchGetDeployments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchGetDeploymentsAsync(BatchGetDeploymentsRequest batchGetDeploymentsRequest);
/**
*
* Gets information about one or more deployments.
*
*
* @param batchGetDeploymentsRequest
* Represents the input of a BatchGetDeployments operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetDeployments operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.BatchGetDeployments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchGetDeploymentsAsync(BatchGetDeploymentsRequest batchGetDeploymentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the BatchGetDeployments operation.
*
* @see #batchGetDeploymentsAsync(BatchGetDeploymentsRequest)
*/
java.util.concurrent.Future batchGetDeploymentsAsync();
/**
* Simplified method form for invoking the BatchGetDeployments operation with an AsyncHandler.
*
* @see #batchGetDeploymentsAsync(BatchGetDeploymentsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future batchGetDeploymentsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about one or more on-premises instances.
*
*
* @param batchGetOnPremisesInstancesRequest
* Represents the input of a BatchGetOnPremisesInstances operation.
* @return A Java Future containing the result of the BatchGetOnPremisesInstances operation returned by the service.
* @sample AmazonCodeDeployAsync.BatchGetOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetOnPremisesInstancesAsync(
BatchGetOnPremisesInstancesRequest batchGetOnPremisesInstancesRequest);
/**
*
* Gets information about one or more on-premises instances.
*
*
* @param batchGetOnPremisesInstancesRequest
* Represents the input of a BatchGetOnPremisesInstances operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetOnPremisesInstances operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.BatchGetOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetOnPremisesInstancesAsync(
BatchGetOnPremisesInstancesRequest batchGetOnPremisesInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the BatchGetOnPremisesInstances operation.
*
* @see #batchGetOnPremisesInstancesAsync(BatchGetOnPremisesInstancesRequest)
*/
java.util.concurrent.Future batchGetOnPremisesInstancesAsync();
/**
* Simplified method form for invoking the BatchGetOnPremisesInstances operation with an AsyncHandler.
*
* @see #batchGetOnPremisesInstancesAsync(BatchGetOnPremisesInstancesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future batchGetOnPremisesInstancesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For a blue/green deployment, starts the process of rerouting traffic from instances in the original environment
* to instances in the replacement environment without waiting for a specified wait time to elapse. (Traffic
* rerouting, which is achieved by registering instances in the replacement environment with the load balancer, can
* start as soon as all instances have a status of Ready.)
*
*
* @param continueDeploymentRequest
* @return A Java Future containing the result of the ContinueDeployment operation returned by the service.
* @sample AmazonCodeDeployAsync.ContinueDeployment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future continueDeploymentAsync(ContinueDeploymentRequest continueDeploymentRequest);
/**
*
* For a blue/green deployment, starts the process of rerouting traffic from instances in the original environment
* to instances in the replacement environment without waiting for a specified wait time to elapse. (Traffic
* rerouting, which is achieved by registering instances in the replacement environment with the load balancer, can
* start as soon as all instances have a status of Ready.)
*
*
* @param continueDeploymentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ContinueDeployment operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ContinueDeployment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future continueDeploymentAsync(ContinueDeploymentRequest continueDeploymentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* Represents the input of a CreateApplication operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AmazonCodeDeployAsync.CreateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* Represents the input of a CreateApplication operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.CreateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deploys an application revision through the specified deployment group.
*
*
* @param createDeploymentRequest
* Represents the input of a CreateDeployment operation.
* @return A Java Future containing the result of the CreateDeployment operation returned by the service.
* @sample AmazonCodeDeployAsync.CreateDeployment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDeploymentAsync(CreateDeploymentRequest createDeploymentRequest);
/**
*
* Deploys an application revision through the specified deployment group.
*
*
* @param createDeploymentRequest
* Represents the input of a CreateDeployment operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDeployment operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.CreateDeployment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDeploymentAsync(CreateDeploymentRequest createDeploymentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a deployment configuration.
*
*
* @param createDeploymentConfigRequest
* Represents the input of a CreateDeploymentConfig operation.
* @return A Java Future containing the result of the CreateDeploymentConfig operation returned by the service.
* @sample AmazonCodeDeployAsync.CreateDeploymentConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeploymentConfigAsync(CreateDeploymentConfigRequest createDeploymentConfigRequest);
/**
*
* Creates a deployment configuration.
*
*
* @param createDeploymentConfigRequest
* Represents the input of a CreateDeploymentConfig operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDeploymentConfig operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.CreateDeploymentConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeploymentConfigAsync(CreateDeploymentConfigRequest createDeploymentConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a deployment group to which application revisions will be deployed.
*
*
* @param createDeploymentGroupRequest
* Represents the input of a CreateDeploymentGroup operation.
* @return A Java Future containing the result of the CreateDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsync.CreateDeploymentGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeploymentGroupAsync(CreateDeploymentGroupRequest createDeploymentGroupRequest);
/**
*
* Creates a deployment group to which application revisions will be deployed.
*
*
* @param createDeploymentGroupRequest
* Represents the input of a CreateDeploymentGroup operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.CreateDeploymentGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeploymentGroupAsync(CreateDeploymentGroupRequest createDeploymentGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* Represents the input of a DeleteApplication operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AmazonCodeDeployAsync.DeleteApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* Represents the input of a DeleteApplication operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.DeleteApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a deployment configuration.
*
*
*
* A deployment configuration cannot be deleted if it is currently in use. Predefined configurations cannot be
* deleted.
*
*
*
* @param deleteDeploymentConfigRequest
* Represents the input of a DeleteDeploymentConfig operation.
* @return A Java Future containing the result of the DeleteDeploymentConfig operation returned by the service.
* @sample AmazonCodeDeployAsync.DeleteDeploymentConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDeploymentConfigAsync(DeleteDeploymentConfigRequest deleteDeploymentConfigRequest);
/**
*
* Deletes a deployment configuration.
*
*
*
* A deployment configuration cannot be deleted if it is currently in use. Predefined configurations cannot be
* deleted.
*
*
*
* @param deleteDeploymentConfigRequest
* Represents the input of a DeleteDeploymentConfig operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDeploymentConfig operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.DeleteDeploymentConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDeploymentConfigAsync(DeleteDeploymentConfigRequest deleteDeploymentConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a deployment group.
*
*
* @param deleteDeploymentGroupRequest
* Represents the input of a DeleteDeploymentGroup operation.
* @return A Java Future containing the result of the DeleteDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsync.DeleteDeploymentGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDeploymentGroupAsync(DeleteDeploymentGroupRequest deleteDeploymentGroupRequest);
/**
*
* Deletes a deployment group.
*
*
* @param deleteDeploymentGroupRequest
* Represents the input of a DeleteDeploymentGroup operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.DeleteDeploymentGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDeploymentGroupAsync(DeleteDeploymentGroupRequest deleteDeploymentGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters an on-premises instance.
*
*
* @param deregisterOnPremisesInstanceRequest
* Represents the input of a DeregisterOnPremisesInstance operation.
* @return A Java Future containing the result of the DeregisterOnPremisesInstance operation returned by the
* service.
* @sample AmazonCodeDeployAsync.DeregisterOnPremisesInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterOnPremisesInstanceAsync(
DeregisterOnPremisesInstanceRequest deregisterOnPremisesInstanceRequest);
/**
*
* Deregisters an on-premises instance.
*
*
* @param deregisterOnPremisesInstanceRequest
* Represents the input of a DeregisterOnPremisesInstance operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterOnPremisesInstance operation returned by the
* service.
* @sample AmazonCodeDeployAsyncHandler.DeregisterOnPremisesInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterOnPremisesInstanceAsync(
DeregisterOnPremisesInstanceRequest deregisterOnPremisesInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an application.
*
*
* @param getApplicationRequest
* Represents the input of a GetApplication operation.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AmazonCodeDeployAsync.GetApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest);
/**
*
* Gets information about an application.
*
*
* @param getApplicationRequest
* Represents the input of a GetApplication operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetApplication
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an application revision.
*
*
* @param getApplicationRevisionRequest
* Represents the input of a GetApplicationRevision operation.
* @return A Java Future containing the result of the GetApplicationRevision operation returned by the service.
* @sample AmazonCodeDeployAsync.GetApplicationRevision
* @see AWS API Documentation
*/
java.util.concurrent.Future getApplicationRevisionAsync(GetApplicationRevisionRequest getApplicationRevisionRequest);
/**
*
* Gets information about an application revision.
*
*
* @param getApplicationRevisionRequest
* Represents the input of a GetApplicationRevision operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApplicationRevision operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetApplicationRevision
* @see AWS API Documentation
*/
java.util.concurrent.Future getApplicationRevisionAsync(GetApplicationRevisionRequest getApplicationRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a deployment.
*
*
* @param getDeploymentRequest
* Represents the input of a GetDeployment operation.
* @return A Java Future containing the result of the GetDeployment operation returned by the service.
* @sample AmazonCodeDeployAsync.GetDeployment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDeploymentAsync(GetDeploymentRequest getDeploymentRequest);
/**
*
* Gets information about a deployment.
*
*
* @param getDeploymentRequest
* Represents the input of a GetDeployment operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeployment operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetDeployment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDeploymentAsync(GetDeploymentRequest getDeploymentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a deployment configuration.
*
*
* @param getDeploymentConfigRequest
* Represents the input of a GetDeploymentConfig operation.
* @return A Java Future containing the result of the GetDeploymentConfig operation returned by the service.
* @sample AmazonCodeDeployAsync.GetDeploymentConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDeploymentConfigAsync(GetDeploymentConfigRequest getDeploymentConfigRequest);
/**
*
* Gets information about a deployment configuration.
*
*
* @param getDeploymentConfigRequest
* Represents the input of a GetDeploymentConfig operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeploymentConfig operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetDeploymentConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDeploymentConfigAsync(GetDeploymentConfigRequest getDeploymentConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a deployment group.
*
*
* @param getDeploymentGroupRequest
* Represents the input of a GetDeploymentGroup operation.
* @return A Java Future containing the result of the GetDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsync.GetDeploymentGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDeploymentGroupAsync(GetDeploymentGroupRequest getDeploymentGroupRequest);
/**
*
* Gets information about a deployment group.
*
*
* @param getDeploymentGroupRequest
* Represents the input of a GetDeploymentGroup operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetDeploymentGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDeploymentGroupAsync(GetDeploymentGroupRequest getDeploymentGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an instance as part of a deployment.
*
*
* @param getDeploymentInstanceRequest
* Represents the input of a GetDeploymentInstance operation.
* @return A Java Future containing the result of the GetDeploymentInstance operation returned by the service.
* @sample AmazonCodeDeployAsync.GetDeploymentInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeploymentInstanceAsync(GetDeploymentInstanceRequest getDeploymentInstanceRequest);
/**
*
* Gets information about an instance as part of a deployment.
*
*
* @param getDeploymentInstanceRequest
* Represents the input of a GetDeploymentInstance operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeploymentInstance operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetDeploymentInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeploymentInstanceAsync(GetDeploymentInstanceRequest getDeploymentInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an on-premises instance.
*
*
* @param getOnPremisesInstanceRequest
* Represents the input of a GetOnPremisesInstance operation.
* @return A Java Future containing the result of the GetOnPremisesInstance operation returned by the service.
* @sample AmazonCodeDeployAsync.GetOnPremisesInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getOnPremisesInstanceAsync(GetOnPremisesInstanceRequest getOnPremisesInstanceRequest);
/**
*
* Gets information about an on-premises instance.
*
*
* @param getOnPremisesInstanceRequest
* Represents the input of a GetOnPremisesInstance operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOnPremisesInstance operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.GetOnPremisesInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getOnPremisesInstanceAsync(GetOnPremisesInstanceRequest getOnPremisesInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists information about revisions for an application.
*
*
* @param listApplicationRevisionsRequest
* Represents the input of a ListApplicationRevisions operation.
* @return A Java Future containing the result of the ListApplicationRevisions operation returned by the service.
* @sample AmazonCodeDeployAsync.ListApplicationRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationRevisionsAsync(ListApplicationRevisionsRequest listApplicationRevisionsRequest);
/**
*
* Lists information about revisions for an application.
*
*
* @param listApplicationRevisionsRequest
* Represents the input of a ListApplicationRevisions operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplicationRevisions operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListApplicationRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationRevisionsAsync(ListApplicationRevisionsRequest listApplicationRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the applications registered with the applicable IAM user or AWS account.
*
*
* @param listApplicationsRequest
* Represents the input of a ListApplications operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AmazonCodeDeployAsync.ListApplications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists the applications registered with the applicable IAM user or AWS account.
*
*
* @param listApplicationsRequest
* Represents the input of a ListApplications operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListApplications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListApplications operation.
*
* @see #listApplicationsAsync(ListApplicationsRequest)
*/
java.util.concurrent.Future listApplicationsAsync();
/**
* Simplified method form for invoking the ListApplications operation with an AsyncHandler.
*
* @see #listApplicationsAsync(ListApplicationsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listApplicationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the deployment configurations with the applicable IAM user or AWS account.
*
*
* @param listDeploymentConfigsRequest
* Represents the input of a ListDeploymentConfigs operation.
* @return A Java Future containing the result of the ListDeploymentConfigs operation returned by the service.
* @sample AmazonCodeDeployAsync.ListDeploymentConfigs
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeploymentConfigsAsync(ListDeploymentConfigsRequest listDeploymentConfigsRequest);
/**
*
* Lists the deployment configurations with the applicable IAM user or AWS account.
*
*
* @param listDeploymentConfigsRequest
* Represents the input of a ListDeploymentConfigs operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeploymentConfigs operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListDeploymentConfigs
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeploymentConfigsAsync(ListDeploymentConfigsRequest listDeploymentConfigsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListDeploymentConfigs operation.
*
* @see #listDeploymentConfigsAsync(ListDeploymentConfigsRequest)
*/
java.util.concurrent.Future listDeploymentConfigsAsync();
/**
* Simplified method form for invoking the ListDeploymentConfigs operation with an AsyncHandler.
*
* @see #listDeploymentConfigsAsync(ListDeploymentConfigsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listDeploymentConfigsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the deployment groups for an application registered with the applicable IAM user or AWS account.
*
*
* @param listDeploymentGroupsRequest
* Represents the input of a ListDeploymentGroups operation.
* @return A Java Future containing the result of the ListDeploymentGroups operation returned by the service.
* @sample AmazonCodeDeployAsync.ListDeploymentGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeploymentGroupsAsync(ListDeploymentGroupsRequest listDeploymentGroupsRequest);
/**
*
* Lists the deployment groups for an application registered with the applicable IAM user or AWS account.
*
*
* @param listDeploymentGroupsRequest
* Represents the input of a ListDeploymentGroups operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeploymentGroups operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListDeploymentGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeploymentGroupsAsync(ListDeploymentGroupsRequest listDeploymentGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the instance for a deployment associated with the applicable IAM user or AWS account.
*
*
* @param listDeploymentInstancesRequest
* Represents the input of a ListDeploymentInstances operation.
* @return A Java Future containing the result of the ListDeploymentInstances operation returned by the service.
* @sample AmazonCodeDeployAsync.ListDeploymentInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeploymentInstancesAsync(ListDeploymentInstancesRequest listDeploymentInstancesRequest);
/**
*
* Lists the instance for a deployment associated with the applicable IAM user or AWS account.
*
*
* @param listDeploymentInstancesRequest
* Represents the input of a ListDeploymentInstances operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeploymentInstances operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListDeploymentInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeploymentInstancesAsync(ListDeploymentInstancesRequest listDeploymentInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the deployments in a deployment group for an application registered with the applicable IAM user or AWS
* account.
*
*
* @param listDeploymentsRequest
* Represents the input of a ListDeployments operation.
* @return A Java Future containing the result of the ListDeployments operation returned by the service.
* @sample AmazonCodeDeployAsync.ListDeployments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDeploymentsAsync(ListDeploymentsRequest listDeploymentsRequest);
/**
*
* Lists the deployments in a deployment group for an application registered with the applicable IAM user or AWS
* account.
*
*
* @param listDeploymentsRequest
* Represents the input of a ListDeployments operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeployments operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListDeployments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDeploymentsAsync(ListDeploymentsRequest listDeploymentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListDeployments operation.
*
* @see #listDeploymentsAsync(ListDeploymentsRequest)
*/
java.util.concurrent.Future listDeploymentsAsync();
/**
* Simplified method form for invoking the ListDeployments operation with an AsyncHandler.
*
* @see #listDeploymentsAsync(ListDeploymentsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listDeploymentsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the names of stored connections to GitHub accounts.
*
*
* @param listGitHubAccountTokenNamesRequest
* Represents the input of a ListGitHubAccountTokenNames operation.
* @return A Java Future containing the result of the ListGitHubAccountTokenNames operation returned by the service.
* @sample AmazonCodeDeployAsync.ListGitHubAccountTokenNames
* @see AWS API Documentation
*/
java.util.concurrent.Future listGitHubAccountTokenNamesAsync(
ListGitHubAccountTokenNamesRequest listGitHubAccountTokenNamesRequest);
/**
*
* Lists the names of stored connections to GitHub accounts.
*
*
* @param listGitHubAccountTokenNamesRequest
* Represents the input of a ListGitHubAccountTokenNames operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGitHubAccountTokenNames operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListGitHubAccountTokenNames
* @see AWS API Documentation
*/
java.util.concurrent.Future listGitHubAccountTokenNamesAsync(
ListGitHubAccountTokenNamesRequest listGitHubAccountTokenNamesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of names for one or more on-premises instances.
*
*
* Unless otherwise specified, both registered and deregistered on-premises instance names will be listed. To list
* only registered or deregistered on-premises instance names, use the registration status parameter.
*
*
* @param listOnPremisesInstancesRequest
* Represents the input of a ListOnPremisesInstances operation.
* @return A Java Future containing the result of the ListOnPremisesInstances operation returned by the service.
* @sample AmazonCodeDeployAsync.ListOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listOnPremisesInstancesAsync(ListOnPremisesInstancesRequest listOnPremisesInstancesRequest);
/**
*
* Gets a list of names for one or more on-premises instances.
*
*
* Unless otherwise specified, both registered and deregistered on-premises instance names will be listed. To list
* only registered or deregistered on-premises instance names, use the registration status parameter.
*
*
* @param listOnPremisesInstancesRequest
* Represents the input of a ListOnPremisesInstances operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOnPremisesInstances operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.ListOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listOnPremisesInstancesAsync(ListOnPremisesInstancesRequest listOnPremisesInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListOnPremisesInstances operation.
*
* @see #listOnPremisesInstancesAsync(ListOnPremisesInstancesRequest)
*/
java.util.concurrent.Future listOnPremisesInstancesAsync();
/**
* Simplified method form for invoking the ListOnPremisesInstances operation with an AsyncHandler.
*
* @see #listOnPremisesInstancesAsync(ListOnPremisesInstancesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listOnPremisesInstancesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers with AWS CodeDeploy a revision for the specified application.
*
*
* @param registerApplicationRevisionRequest
* Represents the input of a RegisterApplicationRevision operation.
* @return A Java Future containing the result of the RegisterApplicationRevision operation returned by the service.
* @sample AmazonCodeDeployAsync.RegisterApplicationRevision
* @see AWS API Documentation
*/
java.util.concurrent.Future registerApplicationRevisionAsync(
RegisterApplicationRevisionRequest registerApplicationRevisionRequest);
/**
*
* Registers with AWS CodeDeploy a revision for the specified application.
*
*
* @param registerApplicationRevisionRequest
* Represents the input of a RegisterApplicationRevision operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterApplicationRevision operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.RegisterApplicationRevision
* @see AWS API Documentation
*/
java.util.concurrent.Future registerApplicationRevisionAsync(
RegisterApplicationRevisionRequest registerApplicationRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers an on-premises instance.
*
*
*
* Only one IAM ARN (an IAM session ARN or IAM user ARN) is supported in the request. You cannot use both.
*
*
*
* @param registerOnPremisesInstanceRequest
* Represents the input of the register on-premises instance operation.
* @return A Java Future containing the result of the RegisterOnPremisesInstance operation returned by the service.
* @sample AmazonCodeDeployAsync.RegisterOnPremisesInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future registerOnPremisesInstanceAsync(
RegisterOnPremisesInstanceRequest registerOnPremisesInstanceRequest);
/**
*
* Registers an on-premises instance.
*
*
*
* Only one IAM ARN (an IAM session ARN or IAM user ARN) is supported in the request. You cannot use both.
*
*
*
* @param registerOnPremisesInstanceRequest
* Represents the input of the register on-premises instance operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterOnPremisesInstance operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.RegisterOnPremisesInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future registerOnPremisesInstanceAsync(
RegisterOnPremisesInstanceRequest registerOnPremisesInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from one or more on-premises instances.
*
*
* @param removeTagsFromOnPremisesInstancesRequest
* Represents the input of a RemoveTagsFromOnPremisesInstances operation.
* @return A Java Future containing the result of the RemoveTagsFromOnPremisesInstances operation returned by the
* service.
* @sample AmazonCodeDeployAsync.RemoveTagsFromOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future removeTagsFromOnPremisesInstancesAsync(
RemoveTagsFromOnPremisesInstancesRequest removeTagsFromOnPremisesInstancesRequest);
/**
*
* Removes one or more tags from one or more on-premises instances.
*
*
* @param removeTagsFromOnPremisesInstancesRequest
* Represents the input of a RemoveTagsFromOnPremisesInstances operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromOnPremisesInstances operation returned by the
* service.
* @sample AmazonCodeDeployAsyncHandler.RemoveTagsFromOnPremisesInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future removeTagsFromOnPremisesInstancesAsync(
RemoveTagsFromOnPremisesInstancesRequest removeTagsFromOnPremisesInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* In a blue/green deployment, overrides any specified wait time and starts terminating instances immediately after
* the traffic routing is completed.
*
*
* @param skipWaitTimeForInstanceTerminationRequest
* @return A Java Future containing the result of the SkipWaitTimeForInstanceTermination operation returned by the
* service.
* @sample AmazonCodeDeployAsync.SkipWaitTimeForInstanceTermination
* @see AWS API Documentation
*/
java.util.concurrent.Future skipWaitTimeForInstanceTerminationAsync(
SkipWaitTimeForInstanceTerminationRequest skipWaitTimeForInstanceTerminationRequest);
/**
*
* In a blue/green deployment, overrides any specified wait time and starts terminating instances immediately after
* the traffic routing is completed.
*
*
* @param skipWaitTimeForInstanceTerminationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SkipWaitTimeForInstanceTermination operation returned by the
* service.
* @sample AmazonCodeDeployAsyncHandler.SkipWaitTimeForInstanceTermination
* @see AWS API Documentation
*/
java.util.concurrent.Future skipWaitTimeForInstanceTerminationAsync(
SkipWaitTimeForInstanceTerminationRequest skipWaitTimeForInstanceTerminationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attempts to stop an ongoing deployment.
*
*
* @param stopDeploymentRequest
* Represents the input of a StopDeployment operation.
* @return A Java Future containing the result of the StopDeployment operation returned by the service.
* @sample AmazonCodeDeployAsync.StopDeployment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopDeploymentAsync(StopDeploymentRequest stopDeploymentRequest);
/**
*
* Attempts to stop an ongoing deployment.
*
*
* @param stopDeploymentRequest
* Represents the input of a StopDeployment operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopDeployment operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.StopDeployment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopDeploymentAsync(StopDeploymentRequest stopDeploymentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes the name of an application.
*
*
* @param updateApplicationRequest
* Represents the input of an UpdateApplication operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AmazonCodeDeployAsync.UpdateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Changes the name of an application.
*
*
* @param updateApplicationRequest
* Represents the input of an UpdateApplication operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.UpdateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the UpdateApplication operation.
*
* @see #updateApplicationAsync(UpdateApplicationRequest)
*/
java.util.concurrent.Future updateApplicationAsync();
/**
* Simplified method form for invoking the UpdateApplication operation with an AsyncHandler.
*
* @see #updateApplicationAsync(UpdateApplicationRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future updateApplicationAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes information about a deployment group.
*
*
* @param updateDeploymentGroupRequest
* Represents the input of an UpdateDeploymentGroup operation.
* @return A Java Future containing the result of the UpdateDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsync.UpdateDeploymentGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDeploymentGroupAsync(UpdateDeploymentGroupRequest updateDeploymentGroupRequest);
/**
*
* Changes information about a deployment group.
*
*
* @param updateDeploymentGroupRequest
* Represents the input of an UpdateDeploymentGroup operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDeploymentGroup operation returned by the service.
* @sample AmazonCodeDeployAsyncHandler.UpdateDeploymentGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDeploymentGroupAsync(UpdateDeploymentGroupRequest updateDeploymentGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}