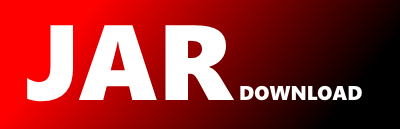
com.amazonaws.services.codedeploy.model.CreateDeploymentGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.codedeploy.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input of a CreateDeploymentGroup operation.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDeploymentGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
*
*/
private String applicationName;
/**
*
* The name of a new deployment group for the specified application.
*
*/
private String deploymentGroupName;
/**
*
* If specified, the deployment configuration name can be either one of the predefined configurations provided with
* AWS CodeDeploy or a custom deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
*
*/
private String deploymentConfigName;
/**
*
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the specified
* tags. Cannot be used in the same call as ec2TagSet.
*
*/
private com.amazonaws.internal.SdkInternalList ec2TagFilters;
/**
*
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances with
* any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*
*/
private com.amazonaws.internal.SdkInternalList onPremisesInstanceTagFilters;
/**
*
* A list of associated Auto Scaling groups.
*
*/
private com.amazonaws.internal.SdkInternalList autoScalingGroups;
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS services.
*
*/
private String serviceRoleArn;
/**
*
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for an AWS
* CodeDeploy Event in the AWS CodeDeploy User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList triggerConfigurations;
/**
*
* Information to add about Amazon CloudWatch alarms when the deployment group is created.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* Configuration information for an automatic rollback that is added when a deployment group is created.
*
*/
private AutoRollbackConfiguration autoRollbackConfiguration;
/**
*
* Information about the type of deployment, in-place or blue/green, that you want to run and whether to route
* deployment traffic behind a load balancer.
*
*/
private DeploymentStyle deploymentStyle;
/**
*
* Information about blue/green deployment options for a deployment group.
*
*/
private BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration;
/**
*
* Information about the load balancer used in a deployment.
*
*/
private LoadBalancerInfo loadBalancerInfo;
/**
*
* Information about groups of tags applied to EC2 instances. The deployment group will include only EC2 instances
* identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
*
*/
private EC2TagSet ec2TagSet;
/**
*
* Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*
*/
private OnPremisesTagSet onPremisesTagSet;
/**
*
* The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
*
*
* @param applicationName
* The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
*/
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
/**
*
* The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
*
*
* @return The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
*/
public String getApplicationName() {
return this.applicationName;
}
/**
*
* The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
*
*
* @param applicationName
* The name of an AWS CodeDeploy application associated with the applicable IAM user or AWS account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withApplicationName(String applicationName) {
setApplicationName(applicationName);
return this;
}
/**
*
* The name of a new deployment group for the specified application.
*
*
* @param deploymentGroupName
* The name of a new deployment group for the specified application.
*/
public void setDeploymentGroupName(String deploymentGroupName) {
this.deploymentGroupName = deploymentGroupName;
}
/**
*
* The name of a new deployment group for the specified application.
*
*
* @return The name of a new deployment group for the specified application.
*/
public String getDeploymentGroupName() {
return this.deploymentGroupName;
}
/**
*
* The name of a new deployment group for the specified application.
*
*
* @param deploymentGroupName
* The name of a new deployment group for the specified application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withDeploymentGroupName(String deploymentGroupName) {
setDeploymentGroupName(deploymentGroupName);
return this;
}
/**
*
* If specified, the deployment configuration name can be either one of the predefined configurations provided with
* AWS CodeDeploy or a custom deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
*
*
* @param deploymentConfigName
* If specified, the deployment configuration name can be either one of the predefined configurations
* provided with AWS CodeDeploy or a custom deployment configuration that you create by calling the create
* deployment configuration operation.
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
*/
public void setDeploymentConfigName(String deploymentConfigName) {
this.deploymentConfigName = deploymentConfigName;
}
/**
*
* If specified, the deployment configuration name can be either one of the predefined configurations provided with
* AWS CodeDeploy or a custom deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
*
*
* @return If specified, the deployment configuration name can be either one of the predefined configurations
* provided with AWS CodeDeploy or a custom deployment configuration that you create by calling the create
* deployment configuration operation.
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
*/
public String getDeploymentConfigName() {
return this.deploymentConfigName;
}
/**
*
* If specified, the deployment configuration name can be either one of the predefined configurations provided with
* AWS CodeDeploy or a custom deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
*
*
* @param deploymentConfigName
* If specified, the deployment configuration name can be either one of the predefined configurations
* provided with AWS CodeDeploy or a custom deployment configuration that you create by calling the create
* deployment configuration operation.
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It is used if a configuration isn't
* specified for the deployment or the deployment group.
*
*
* For more information about the predefined deployment configurations in AWS CodeDeploy, see Working with
* Deployment Groups in AWS CodeDeploy in the AWS CodeDeploy User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withDeploymentConfigName(String deploymentConfigName) {
setDeploymentConfigName(deploymentConfigName);
return this;
}
/**
*
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the specified
* tags. Cannot be used in the same call as ec2TagSet.
*
*
* @return The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the
* specified tags. Cannot be used in the same call as ec2TagSet.
*/
public java.util.List getEc2TagFilters() {
if (ec2TagFilters == null) {
ec2TagFilters = new com.amazonaws.internal.SdkInternalList();
}
return ec2TagFilters;
}
/**
*
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the specified
* tags. Cannot be used in the same call as ec2TagSet.
*
*
* @param ec2TagFilters
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the
* specified tags. Cannot be used in the same call as ec2TagSet.
*/
public void setEc2TagFilters(java.util.Collection ec2TagFilters) {
if (ec2TagFilters == null) {
this.ec2TagFilters = null;
return;
}
this.ec2TagFilters = new com.amazonaws.internal.SdkInternalList(ec2TagFilters);
}
/**
*
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the specified
* tags. Cannot be used in the same call as ec2TagSet.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEc2TagFilters(java.util.Collection)} or {@link #withEc2TagFilters(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param ec2TagFilters
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the
* specified tags. Cannot be used in the same call as ec2TagSet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withEc2TagFilters(EC2TagFilter... ec2TagFilters) {
if (this.ec2TagFilters == null) {
setEc2TagFilters(new com.amazonaws.internal.SdkInternalList(ec2TagFilters.length));
}
for (EC2TagFilter ele : ec2TagFilters) {
this.ec2TagFilters.add(ele);
}
return this;
}
/**
*
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the specified
* tags. Cannot be used in the same call as ec2TagSet.
*
*
* @param ec2TagFilters
* The Amazon EC2 tags on which to filter. The deployment group will include EC2 instances with any of the
* specified tags. Cannot be used in the same call as ec2TagSet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withEc2TagFilters(java.util.Collection ec2TagFilters) {
setEc2TagFilters(ec2TagFilters);
return this;
}
/**
*
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances with
* any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*
*
* @return The on-premises instance tags on which to filter. The deployment group will include on-premises instances
* with any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*/
public java.util.List getOnPremisesInstanceTagFilters() {
if (onPremisesInstanceTagFilters == null) {
onPremisesInstanceTagFilters = new com.amazonaws.internal.SdkInternalList();
}
return onPremisesInstanceTagFilters;
}
/**
*
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances with
* any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*
*
* @param onPremisesInstanceTagFilters
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances
* with any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*/
public void setOnPremisesInstanceTagFilters(java.util.Collection onPremisesInstanceTagFilters) {
if (onPremisesInstanceTagFilters == null) {
this.onPremisesInstanceTagFilters = null;
return;
}
this.onPremisesInstanceTagFilters = new com.amazonaws.internal.SdkInternalList(onPremisesInstanceTagFilters);
}
/**
*
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances with
* any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOnPremisesInstanceTagFilters(java.util.Collection)} or
* {@link #withOnPremisesInstanceTagFilters(java.util.Collection)} if you want to override the existing values.
*
*
* @param onPremisesInstanceTagFilters
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances
* with any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withOnPremisesInstanceTagFilters(TagFilter... onPremisesInstanceTagFilters) {
if (this.onPremisesInstanceTagFilters == null) {
setOnPremisesInstanceTagFilters(new com.amazonaws.internal.SdkInternalList(onPremisesInstanceTagFilters.length));
}
for (TagFilter ele : onPremisesInstanceTagFilters) {
this.onPremisesInstanceTagFilters.add(ele);
}
return this;
}
/**
*
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances with
* any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
*
*
* @param onPremisesInstanceTagFilters
* The on-premises instance tags on which to filter. The deployment group will include on-premises instances
* with any of the specified tags. Cannot be used in the same call as OnPremisesTagSet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withOnPremisesInstanceTagFilters(java.util.Collection onPremisesInstanceTagFilters) {
setOnPremisesInstanceTagFilters(onPremisesInstanceTagFilters);
return this;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* @return A list of associated Auto Scaling groups.
*/
public java.util.List getAutoScalingGroups() {
if (autoScalingGroups == null) {
autoScalingGroups = new com.amazonaws.internal.SdkInternalList();
}
return autoScalingGroups;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* @param autoScalingGroups
* A list of associated Auto Scaling groups.
*/
public void setAutoScalingGroups(java.util.Collection autoScalingGroups) {
if (autoScalingGroups == null) {
this.autoScalingGroups = null;
return;
}
this.autoScalingGroups = new com.amazonaws.internal.SdkInternalList(autoScalingGroups);
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAutoScalingGroups(java.util.Collection)} or {@link #withAutoScalingGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param autoScalingGroups
* A list of associated Auto Scaling groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withAutoScalingGroups(String... autoScalingGroups) {
if (this.autoScalingGroups == null) {
setAutoScalingGroups(new com.amazonaws.internal.SdkInternalList(autoScalingGroups.length));
}
for (String ele : autoScalingGroups) {
this.autoScalingGroups.add(ele);
}
return this;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* @param autoScalingGroups
* A list of associated Auto Scaling groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withAutoScalingGroups(java.util.Collection autoScalingGroups) {
setAutoScalingGroups(autoScalingGroups);
return this;
}
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS services.
*
*
* @param serviceRoleArn
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS
* services.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS services.
*
*
* @return A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS
* services.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS services.
*
*
* @param serviceRoleArn
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf when interacting with AWS
* services.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for an AWS
* CodeDeploy Event in the AWS CodeDeploy User Guide.
*
*
* @return Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for
* an AWS CodeDeploy Event in the AWS CodeDeploy User Guide.
*/
public java.util.List getTriggerConfigurations() {
if (triggerConfigurations == null) {
triggerConfigurations = new com.amazonaws.internal.SdkInternalList();
}
return triggerConfigurations;
}
/**
*
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for an AWS
* CodeDeploy Event in the AWS CodeDeploy User Guide.
*
*
* @param triggerConfigurations
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for
* an AWS CodeDeploy Event in the AWS CodeDeploy User Guide.
*/
public void setTriggerConfigurations(java.util.Collection triggerConfigurations) {
if (triggerConfigurations == null) {
this.triggerConfigurations = null;
return;
}
this.triggerConfigurations = new com.amazonaws.internal.SdkInternalList(triggerConfigurations);
}
/**
*
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for an AWS
* CodeDeploy Event in the AWS CodeDeploy User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggerConfigurations(java.util.Collection)} or
* {@link #withTriggerConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param triggerConfigurations
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for
* an AWS CodeDeploy Event in the AWS CodeDeploy User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withTriggerConfigurations(TriggerConfig... triggerConfigurations) {
if (this.triggerConfigurations == null) {
setTriggerConfigurations(new com.amazonaws.internal.SdkInternalList(triggerConfigurations.length));
}
for (TriggerConfig ele : triggerConfigurations) {
this.triggerConfigurations.add(ele);
}
return this;
}
/**
*
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for an AWS
* CodeDeploy Event in the AWS CodeDeploy User Guide.
*
*
* @param triggerConfigurations
* Information about triggers to create when the deployment group is created. For examples, see Create a Trigger for
* an AWS CodeDeploy Event in the AWS CodeDeploy User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withTriggerConfigurations(java.util.Collection triggerConfigurations) {
setTriggerConfigurations(triggerConfigurations);
return this;
}
/**
*
* Information to add about Amazon CloudWatch alarms when the deployment group is created.
*
*
* @param alarmConfiguration
* Information to add about Amazon CloudWatch alarms when the deployment group is created.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* Information to add about Amazon CloudWatch alarms when the deployment group is created.
*
*
* @return Information to add about Amazon CloudWatch alarms when the deployment group is created.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* Information to add about Amazon CloudWatch alarms when the deployment group is created.
*
*
* @param alarmConfiguration
* Information to add about Amazon CloudWatch alarms when the deployment group is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* Configuration information for an automatic rollback that is added when a deployment group is created.
*
*
* @param autoRollbackConfiguration
* Configuration information for an automatic rollback that is added when a deployment group is created.
*/
public void setAutoRollbackConfiguration(AutoRollbackConfiguration autoRollbackConfiguration) {
this.autoRollbackConfiguration = autoRollbackConfiguration;
}
/**
*
* Configuration information for an automatic rollback that is added when a deployment group is created.
*
*
* @return Configuration information for an automatic rollback that is added when a deployment group is created.
*/
public AutoRollbackConfiguration getAutoRollbackConfiguration() {
return this.autoRollbackConfiguration;
}
/**
*
* Configuration information for an automatic rollback that is added when a deployment group is created.
*
*
* @param autoRollbackConfiguration
* Configuration information for an automatic rollback that is added when a deployment group is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withAutoRollbackConfiguration(AutoRollbackConfiguration autoRollbackConfiguration) {
setAutoRollbackConfiguration(autoRollbackConfiguration);
return this;
}
/**
*
* Information about the type of deployment, in-place or blue/green, that you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @param deploymentStyle
* Information about the type of deployment, in-place or blue/green, that you want to run and whether to
* route deployment traffic behind a load balancer.
*/
public void setDeploymentStyle(DeploymentStyle deploymentStyle) {
this.deploymentStyle = deploymentStyle;
}
/**
*
* Information about the type of deployment, in-place or blue/green, that you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @return Information about the type of deployment, in-place or blue/green, that you want to run and whether to
* route deployment traffic behind a load balancer.
*/
public DeploymentStyle getDeploymentStyle() {
return this.deploymentStyle;
}
/**
*
* Information about the type of deployment, in-place or blue/green, that you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @param deploymentStyle
* Information about the type of deployment, in-place or blue/green, that you want to run and whether to
* route deployment traffic behind a load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withDeploymentStyle(DeploymentStyle deploymentStyle) {
setDeploymentStyle(deploymentStyle);
return this;
}
/**
*
* Information about blue/green deployment options for a deployment group.
*
*
* @param blueGreenDeploymentConfiguration
* Information about blue/green deployment options for a deployment group.
*/
public void setBlueGreenDeploymentConfiguration(BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration) {
this.blueGreenDeploymentConfiguration = blueGreenDeploymentConfiguration;
}
/**
*
* Information about blue/green deployment options for a deployment group.
*
*
* @return Information about blue/green deployment options for a deployment group.
*/
public BlueGreenDeploymentConfiguration getBlueGreenDeploymentConfiguration() {
return this.blueGreenDeploymentConfiguration;
}
/**
*
* Information about blue/green deployment options for a deployment group.
*
*
* @param blueGreenDeploymentConfiguration
* Information about blue/green deployment options for a deployment group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withBlueGreenDeploymentConfiguration(BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration) {
setBlueGreenDeploymentConfiguration(blueGreenDeploymentConfiguration);
return this;
}
/**
*
* Information about the load balancer used in a deployment.
*
*
* @param loadBalancerInfo
* Information about the load balancer used in a deployment.
*/
public void setLoadBalancerInfo(LoadBalancerInfo loadBalancerInfo) {
this.loadBalancerInfo = loadBalancerInfo;
}
/**
*
* Information about the load balancer used in a deployment.
*
*
* @return Information about the load balancer used in a deployment.
*/
public LoadBalancerInfo getLoadBalancerInfo() {
return this.loadBalancerInfo;
}
/**
*
* Information about the load balancer used in a deployment.
*
*
* @param loadBalancerInfo
* Information about the load balancer used in a deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withLoadBalancerInfo(LoadBalancerInfo loadBalancerInfo) {
setLoadBalancerInfo(loadBalancerInfo);
return this;
}
/**
*
* Information about groups of tags applied to EC2 instances. The deployment group will include only EC2 instances
* identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
*
*
* @param ec2TagSet
* Information about groups of tags applied to EC2 instances. The deployment group will include only EC2
* instances identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
*/
public void setEc2TagSet(EC2TagSet ec2TagSet) {
this.ec2TagSet = ec2TagSet;
}
/**
*
* Information about groups of tags applied to EC2 instances. The deployment group will include only EC2 instances
* identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
*
*
* @return Information about groups of tags applied to EC2 instances. The deployment group will include only EC2
* instances identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
*/
public EC2TagSet getEc2TagSet() {
return this.ec2TagSet;
}
/**
*
* Information about groups of tags applied to EC2 instances. The deployment group will include only EC2 instances
* identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
*
*
* @param ec2TagSet
* Information about groups of tags applied to EC2 instances. The deployment group will include only EC2
* instances identified by all the tag groups. Cannot be used in the same call as ec2TagFilters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withEc2TagSet(EC2TagSet ec2TagSet) {
setEc2TagSet(ec2TagSet);
return this;
}
/**
*
* Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*
*
* @param onPremisesTagSet
* Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*/
public void setOnPremisesTagSet(OnPremisesTagSet onPremisesTagSet) {
this.onPremisesTagSet = onPremisesTagSet;
}
/**
*
* Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*
*
* @return Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*/
public OnPremisesTagSet getOnPremisesTagSet() {
return this.onPremisesTagSet;
}
/**
*
* Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
*
*
* @param onPremisesTagSet
* Information about groups of tags applied to on-premises instances. The deployment group will include only
* on-premises instances identified by all the tag groups. Cannot be used in the same call as
* onPremisesInstanceTagFilters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDeploymentGroupRequest withOnPremisesTagSet(OnPremisesTagSet onPremisesTagSet) {
setOnPremisesTagSet(onPremisesTagSet);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationName() != null)
sb.append("ApplicationName: ").append(getApplicationName()).append(",");
if (getDeploymentGroupName() != null)
sb.append("DeploymentGroupName: ").append(getDeploymentGroupName()).append(",");
if (getDeploymentConfigName() != null)
sb.append("DeploymentConfigName: ").append(getDeploymentConfigName()).append(",");
if (getEc2TagFilters() != null)
sb.append("Ec2TagFilters: ").append(getEc2TagFilters()).append(",");
if (getOnPremisesInstanceTagFilters() != null)
sb.append("OnPremisesInstanceTagFilters: ").append(getOnPremisesInstanceTagFilters()).append(",");
if (getAutoScalingGroups() != null)
sb.append("AutoScalingGroups: ").append(getAutoScalingGroups()).append(",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: ").append(getServiceRoleArn()).append(",");
if (getTriggerConfigurations() != null)
sb.append("TriggerConfigurations: ").append(getTriggerConfigurations()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getAutoRollbackConfiguration() != null)
sb.append("AutoRollbackConfiguration: ").append(getAutoRollbackConfiguration()).append(",");
if (getDeploymentStyle() != null)
sb.append("DeploymentStyle: ").append(getDeploymentStyle()).append(",");
if (getBlueGreenDeploymentConfiguration() != null)
sb.append("BlueGreenDeploymentConfiguration: ").append(getBlueGreenDeploymentConfiguration()).append(",");
if (getLoadBalancerInfo() != null)
sb.append("LoadBalancerInfo: ").append(getLoadBalancerInfo()).append(",");
if (getEc2TagSet() != null)
sb.append("Ec2TagSet: ").append(getEc2TagSet()).append(",");
if (getOnPremisesTagSet() != null)
sb.append("OnPremisesTagSet: ").append(getOnPremisesTagSet());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDeploymentGroupRequest == false)
return false;
CreateDeploymentGroupRequest other = (CreateDeploymentGroupRequest) obj;
if (other.getApplicationName() == null ^ this.getApplicationName() == null)
return false;
if (other.getApplicationName() != null && other.getApplicationName().equals(this.getApplicationName()) == false)
return false;
if (other.getDeploymentGroupName() == null ^ this.getDeploymentGroupName() == null)
return false;
if (other.getDeploymentGroupName() != null && other.getDeploymentGroupName().equals(this.getDeploymentGroupName()) == false)
return false;
if (other.getDeploymentConfigName() == null ^ this.getDeploymentConfigName() == null)
return false;
if (other.getDeploymentConfigName() != null && other.getDeploymentConfigName().equals(this.getDeploymentConfigName()) == false)
return false;
if (other.getEc2TagFilters() == null ^ this.getEc2TagFilters() == null)
return false;
if (other.getEc2TagFilters() != null && other.getEc2TagFilters().equals(this.getEc2TagFilters()) == false)
return false;
if (other.getOnPremisesInstanceTagFilters() == null ^ this.getOnPremisesInstanceTagFilters() == null)
return false;
if (other.getOnPremisesInstanceTagFilters() != null && other.getOnPremisesInstanceTagFilters().equals(this.getOnPremisesInstanceTagFilters()) == false)
return false;
if (other.getAutoScalingGroups() == null ^ this.getAutoScalingGroups() == null)
return false;
if (other.getAutoScalingGroups() != null && other.getAutoScalingGroups().equals(this.getAutoScalingGroups()) == false)
return false;
if (other.getServiceRoleArn() == null ^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null && other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getTriggerConfigurations() == null ^ this.getTriggerConfigurations() == null)
return false;
if (other.getTriggerConfigurations() != null && other.getTriggerConfigurations().equals(this.getTriggerConfigurations()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getAutoRollbackConfiguration() == null ^ this.getAutoRollbackConfiguration() == null)
return false;
if (other.getAutoRollbackConfiguration() != null && other.getAutoRollbackConfiguration().equals(this.getAutoRollbackConfiguration()) == false)
return false;
if (other.getDeploymentStyle() == null ^ this.getDeploymentStyle() == null)
return false;
if (other.getDeploymentStyle() != null && other.getDeploymentStyle().equals(this.getDeploymentStyle()) == false)
return false;
if (other.getBlueGreenDeploymentConfiguration() == null ^ this.getBlueGreenDeploymentConfiguration() == null)
return false;
if (other.getBlueGreenDeploymentConfiguration() != null
&& other.getBlueGreenDeploymentConfiguration().equals(this.getBlueGreenDeploymentConfiguration()) == false)
return false;
if (other.getLoadBalancerInfo() == null ^ this.getLoadBalancerInfo() == null)
return false;
if (other.getLoadBalancerInfo() != null && other.getLoadBalancerInfo().equals(this.getLoadBalancerInfo()) == false)
return false;
if (other.getEc2TagSet() == null ^ this.getEc2TagSet() == null)
return false;
if (other.getEc2TagSet() != null && other.getEc2TagSet().equals(this.getEc2TagSet()) == false)
return false;
if (other.getOnPremisesTagSet() == null ^ this.getOnPremisesTagSet() == null)
return false;
if (other.getOnPremisesTagSet() != null && other.getOnPremisesTagSet().equals(this.getOnPremisesTagSet()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationName() == null) ? 0 : getApplicationName().hashCode());
hashCode = prime * hashCode + ((getDeploymentGroupName() == null) ? 0 : getDeploymentGroupName().hashCode());
hashCode = prime * hashCode + ((getDeploymentConfigName() == null) ? 0 : getDeploymentConfigName().hashCode());
hashCode = prime * hashCode + ((getEc2TagFilters() == null) ? 0 : getEc2TagFilters().hashCode());
hashCode = prime * hashCode + ((getOnPremisesInstanceTagFilters() == null) ? 0 : getOnPremisesInstanceTagFilters().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroups() == null) ? 0 : getAutoScalingGroups().hashCode());
hashCode = prime * hashCode + ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn().hashCode());
hashCode = prime * hashCode + ((getTriggerConfigurations() == null) ? 0 : getTriggerConfigurations().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getAutoRollbackConfiguration() == null) ? 0 : getAutoRollbackConfiguration().hashCode());
hashCode = prime * hashCode + ((getDeploymentStyle() == null) ? 0 : getDeploymentStyle().hashCode());
hashCode = prime * hashCode + ((getBlueGreenDeploymentConfiguration() == null) ? 0 : getBlueGreenDeploymentConfiguration().hashCode());
hashCode = prime * hashCode + ((getLoadBalancerInfo() == null) ? 0 : getLoadBalancerInfo().hashCode());
hashCode = prime * hashCode + ((getEc2TagSet() == null) ? 0 : getEc2TagSet().hashCode());
hashCode = prime * hashCode + ((getOnPremisesTagSet() == null) ? 0 : getOnPremisesTagSet().hashCode());
return hashCode;
}
@Override
public CreateDeploymentGroupRequest clone() {
return (CreateDeploymentGroupRequest) super.clone();
}
}