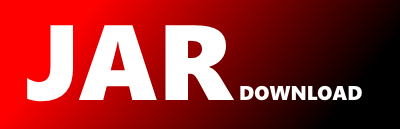
com.amazonaws.services.codedeploy.AmazonCodeDeployAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.codedeploy;
import com.amazonaws.services.codedeploy.model.*;
import com.amazonaws.annotation.ThreadSafe;
/**
* Interface for accessing CodeDeploy asynchronously. Each asynchronous method
* will return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS CodeDeploy Overview
*
* This reference guide provides descriptions of the AWS CodeDeploy APIs. For
* more information about AWS CodeDeploy, see the AWS CodeDeploy User
* Guide.
*
* Using the APIs
*
* You can use the AWS CodeDeploy APIs to work with the following:
*
*
* -
*
* Applications are unique identifiers used by AWS CodeDeploy to ensure the
* correct combinations of revisions, deployment configurations, and deployment
* groups are being referenced during deployments.
*
*
* You can use the AWS CodeDeploy APIs to create, delete, get, list, and update
* applications.
*
*
* -
*
* Deployment configurations are sets of deployment rules and success and
* failure conditions used by AWS CodeDeploy during deployments.
*
*
* You can use the AWS CodeDeploy APIs to create, delete, get, and list
* deployment configurations.
*
*
* -
*
* Deployment groups are groups of instances to which application revisions can
* be deployed.
*
*
* You can use the AWS CodeDeploy APIs to create, delete, get, list, and update
* deployment groups.
*
*
* -
*
* Instances represent Amazon EC2 instances to which application revisions are
* deployed. Instances are identified by their Amazon EC2 tags or Auto Scaling
* group names. Instances belong to deployment groups.
*
*
* You can use the AWS CodeDeploy APIs to get and list instance.
*
*
* -
*
* Deployments represent the process of deploying revisions to instances.
*
*
* You can use the AWS CodeDeploy APIs to create, get, list, and stop
* deployments.
*
*
* -
*
* Application revisions are archive files stored in Amazon S3 buckets or GitHub
* repositories. These revisions contain source content (such as source code,
* web pages, executable files, and deployment scripts) along with an
* application specification (AppSpec) file. (The AppSpec file is unique to AWS
* CodeDeploy; it defines the deployment actions you want AWS CodeDeploy to
* execute.) Ffor application revisions stored in Amazon S3 buckets, an
* application revision is uniquely identified by its Amazon S3 object key and
* its ETag, version, or both. For application revisions stored in GitHub
* repositories, an application revision is uniquely identified by its
* repository name and commit ID. Application revisions are deployed through
* deployment groups.
*
*
* You can use the AWS CodeDeploy APIs to get, list, and register application
* revisions.
*
*
*
*/
@ThreadSafe
public class AmazonCodeDeployAsyncClient extends AmazonCodeDeployClient
implements AmazonCodeDeployAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy. A credentials provider chain will be used that searches for
* credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCodeDeployAsyncClient() {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy. A credentials provider chain will be used that searches for
* credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to CodeDeploy (ex: proxy settings, retry counts, etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.ClientConfiguration clientConfiguration) {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain(),
clientConfiguration, java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the specified AWS account credentials.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials) {
this(awsCredentials, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the specified AWS account credentials and executor
* service. Default client settings will be used.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentials, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the specified AWS account credentials, executor service,
* and client configuration options.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the specified AWS account credentials provider. Default
* client settings will be used.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the provided AWS account credentials provider and client
* configuration options.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration,
java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the specified AWS account credentials provider and
* executor service. Default client settings will be used.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentialsProvider, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on
* CodeDeploy using the specified AWS account credentials provider, executor
* service, and client configuration options.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCodeDeployAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this client to execute async
* requests.
*
* @return The executor service used by this client to execute async
* requests.
*/
public java.util.concurrent.ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future addTagsToOnPremisesInstancesAsync(
AddTagsToOnPremisesInstancesRequest request) {
return addTagsToOnPremisesInstancesAsync(request, null);
}
@Override
public java.util.concurrent.Future addTagsToOnPremisesInstancesAsync(
final AddTagsToOnPremisesInstancesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public AddTagsToOnPremisesInstancesResult call()
throws Exception {
AddTagsToOnPremisesInstancesResult result;
try {
result = addTagsToOnPremisesInstances(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future batchGetApplicationRevisionsAsync(
BatchGetApplicationRevisionsRequest request) {
return batchGetApplicationRevisionsAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetApplicationRevisionsAsync(
final BatchGetApplicationRevisionsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public BatchGetApplicationRevisionsResult call()
throws Exception {
BatchGetApplicationRevisionsResult result;
try {
result = batchGetApplicationRevisions(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future batchGetApplicationsAsync(
BatchGetApplicationsRequest request) {
return batchGetApplicationsAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetApplicationsAsync(
final BatchGetApplicationsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public BatchGetApplicationsResult call() throws Exception {
BatchGetApplicationsResult result;
try {
result = batchGetApplications(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the BatchGetApplications operation.
*
* @see #batchGetApplicationsAsync(BatchGetApplicationsRequest)
*/
@Override
public java.util.concurrent.Future batchGetApplicationsAsync() {
return batchGetApplicationsAsync(new BatchGetApplicationsRequest());
}
/**
* Simplified method form for invoking the BatchGetApplications operation
* with an AsyncHandler.
*
* @see #batchGetApplicationsAsync(BatchGetApplicationsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future batchGetApplicationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return batchGetApplicationsAsync(new BatchGetApplicationsRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future batchGetDeploymentGroupsAsync(
BatchGetDeploymentGroupsRequest request) {
return batchGetDeploymentGroupsAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetDeploymentGroupsAsync(
final BatchGetDeploymentGroupsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public BatchGetDeploymentGroupsResult call()
throws Exception {
BatchGetDeploymentGroupsResult result;
try {
result = batchGetDeploymentGroups(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future batchGetDeploymentInstancesAsync(
BatchGetDeploymentInstancesRequest request) {
return batchGetDeploymentInstancesAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetDeploymentInstancesAsync(
final BatchGetDeploymentInstancesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public BatchGetDeploymentInstancesResult call()
throws Exception {
BatchGetDeploymentInstancesResult result;
try {
result = batchGetDeploymentInstances(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future batchGetDeploymentsAsync(
BatchGetDeploymentsRequest request) {
return batchGetDeploymentsAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetDeploymentsAsync(
final BatchGetDeploymentsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public BatchGetDeploymentsResult call() throws Exception {
BatchGetDeploymentsResult result;
try {
result = batchGetDeployments(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the BatchGetDeployments operation.
*
* @see #batchGetDeploymentsAsync(BatchGetDeploymentsRequest)
*/
@Override
public java.util.concurrent.Future batchGetDeploymentsAsync() {
return batchGetDeploymentsAsync(new BatchGetDeploymentsRequest());
}
/**
* Simplified method form for invoking the BatchGetDeployments operation
* with an AsyncHandler.
*
* @see #batchGetDeploymentsAsync(BatchGetDeploymentsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future batchGetDeploymentsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return batchGetDeploymentsAsync(new BatchGetDeploymentsRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future batchGetOnPremisesInstancesAsync(
BatchGetOnPremisesInstancesRequest request) {
return batchGetOnPremisesInstancesAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetOnPremisesInstancesAsync(
final BatchGetOnPremisesInstancesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public BatchGetOnPremisesInstancesResult call()
throws Exception {
BatchGetOnPremisesInstancesResult result;
try {
result = batchGetOnPremisesInstances(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the BatchGetOnPremisesInstances
* operation.
*
* @see #batchGetOnPremisesInstancesAsync(BatchGetOnPremisesInstancesRequest)
*/
@Override
public java.util.concurrent.Future batchGetOnPremisesInstancesAsync() {
return batchGetOnPremisesInstancesAsync(new BatchGetOnPremisesInstancesRequest());
}
/**
* Simplified method form for invoking the BatchGetOnPremisesInstances
* operation with an AsyncHandler.
*
* @see #batchGetOnPremisesInstancesAsync(BatchGetOnPremisesInstancesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future batchGetOnPremisesInstancesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return batchGetOnPremisesInstancesAsync(
new BatchGetOnPremisesInstancesRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future createApplicationAsync(
CreateApplicationRequest request) {
return createApplicationAsync(request, null);
}
@Override
public java.util.concurrent.Future createApplicationAsync(
final CreateApplicationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateApplicationResult call() throws Exception {
CreateApplicationResult result;
try {
result = createApplication(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createDeploymentAsync(
CreateDeploymentRequest request) {
return createDeploymentAsync(request, null);
}
@Override
public java.util.concurrent.Future createDeploymentAsync(
final CreateDeploymentRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateDeploymentResult call() throws Exception {
CreateDeploymentResult result;
try {
result = createDeployment(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createDeploymentConfigAsync(
CreateDeploymentConfigRequest request) {
return createDeploymentConfigAsync(request, null);
}
@Override
public java.util.concurrent.Future createDeploymentConfigAsync(
final CreateDeploymentConfigRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateDeploymentConfigResult call() throws Exception {
CreateDeploymentConfigResult result;
try {
result = createDeploymentConfig(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createDeploymentGroupAsync(
CreateDeploymentGroupRequest request) {
return createDeploymentGroupAsync(request, null);
}
@Override
public java.util.concurrent.Future createDeploymentGroupAsync(
final CreateDeploymentGroupRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateDeploymentGroupResult call() throws Exception {
CreateDeploymentGroupResult result;
try {
result = createDeploymentGroup(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteApplicationAsync(
DeleteApplicationRequest request) {
return deleteApplicationAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteApplicationAsync(
final DeleteApplicationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteApplicationResult call() throws Exception {
DeleteApplicationResult result;
try {
result = deleteApplication(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteDeploymentConfigAsync(
DeleteDeploymentConfigRequest request) {
return deleteDeploymentConfigAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteDeploymentConfigAsync(
final DeleteDeploymentConfigRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteDeploymentConfigResult call() throws Exception {
DeleteDeploymentConfigResult result;
try {
result = deleteDeploymentConfig(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteDeploymentGroupAsync(
DeleteDeploymentGroupRequest request) {
return deleteDeploymentGroupAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteDeploymentGroupAsync(
final DeleteDeploymentGroupRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteDeploymentGroupResult call() throws Exception {
DeleteDeploymentGroupResult result;
try {
result = deleteDeploymentGroup(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deregisterOnPremisesInstanceAsync(
DeregisterOnPremisesInstanceRequest request) {
return deregisterOnPremisesInstanceAsync(request, null);
}
@Override
public java.util.concurrent.Future deregisterOnPremisesInstanceAsync(
final DeregisterOnPremisesInstanceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeregisterOnPremisesInstanceResult call()
throws Exception {
DeregisterOnPremisesInstanceResult result;
try {
result = deregisterOnPremisesInstance(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getApplicationAsync(
GetApplicationRequest request) {
return getApplicationAsync(request, null);
}
@Override
public java.util.concurrent.Future getApplicationAsync(
final GetApplicationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetApplicationResult call() throws Exception {
GetApplicationResult result;
try {
result = getApplication(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getApplicationRevisionAsync(
GetApplicationRevisionRequest request) {
return getApplicationRevisionAsync(request, null);
}
@Override
public java.util.concurrent.Future getApplicationRevisionAsync(
final GetApplicationRevisionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetApplicationRevisionResult call() throws Exception {
GetApplicationRevisionResult result;
try {
result = getApplicationRevision(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getDeploymentAsync(
GetDeploymentRequest request) {
return getDeploymentAsync(request, null);
}
@Override
public java.util.concurrent.Future getDeploymentAsync(
final GetDeploymentRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetDeploymentResult call() throws Exception {
GetDeploymentResult result;
try {
result = getDeployment(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getDeploymentConfigAsync(
GetDeploymentConfigRequest request) {
return getDeploymentConfigAsync(request, null);
}
@Override
public java.util.concurrent.Future getDeploymentConfigAsync(
final GetDeploymentConfigRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetDeploymentConfigResult call() throws Exception {
GetDeploymentConfigResult result;
try {
result = getDeploymentConfig(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getDeploymentGroupAsync(
GetDeploymentGroupRequest request) {
return getDeploymentGroupAsync(request, null);
}
@Override
public java.util.concurrent.Future getDeploymentGroupAsync(
final GetDeploymentGroupRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetDeploymentGroupResult call() throws Exception {
GetDeploymentGroupResult result;
try {
result = getDeploymentGroup(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getDeploymentInstanceAsync(
GetDeploymentInstanceRequest request) {
return getDeploymentInstanceAsync(request, null);
}
@Override
public java.util.concurrent.Future getDeploymentInstanceAsync(
final GetDeploymentInstanceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetDeploymentInstanceResult call() throws Exception {
GetDeploymentInstanceResult result;
try {
result = getDeploymentInstance(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getOnPremisesInstanceAsync(
GetOnPremisesInstanceRequest request) {
return getOnPremisesInstanceAsync(request, null);
}
@Override
public java.util.concurrent.Future getOnPremisesInstanceAsync(
final GetOnPremisesInstanceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetOnPremisesInstanceResult call() throws Exception {
GetOnPremisesInstanceResult result;
try {
result = getOnPremisesInstance(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listApplicationRevisionsAsync(
ListApplicationRevisionsRequest request) {
return listApplicationRevisionsAsync(request, null);
}
@Override
public java.util.concurrent.Future listApplicationRevisionsAsync(
final ListApplicationRevisionsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListApplicationRevisionsResult call()
throws Exception {
ListApplicationRevisionsResult result;
try {
result = listApplicationRevisions(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listApplicationsAsync(
ListApplicationsRequest request) {
return listApplicationsAsync(request, null);
}
@Override
public java.util.concurrent.Future listApplicationsAsync(
final ListApplicationsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListApplicationsResult call() throws Exception {
ListApplicationsResult result;
try {
result = listApplications(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListApplications operation.
*
* @see #listApplicationsAsync(ListApplicationsRequest)
*/
@Override
public java.util.concurrent.Future listApplicationsAsync() {
return listApplicationsAsync(new ListApplicationsRequest());
}
/**
* Simplified method form for invoking the ListApplications operation with
* an AsyncHandler.
*
* @see #listApplicationsAsync(ListApplicationsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listApplicationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listApplicationsAsync(new ListApplicationsRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future listDeploymentConfigsAsync(
ListDeploymentConfigsRequest request) {
return listDeploymentConfigsAsync(request, null);
}
@Override
public java.util.concurrent.Future listDeploymentConfigsAsync(
final ListDeploymentConfigsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListDeploymentConfigsResult call() throws Exception {
ListDeploymentConfigsResult result;
try {
result = listDeploymentConfigs(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListDeploymentConfigs operation.
*
* @see #listDeploymentConfigsAsync(ListDeploymentConfigsRequest)
*/
@Override
public java.util.concurrent.Future listDeploymentConfigsAsync() {
return listDeploymentConfigsAsync(new ListDeploymentConfigsRequest());
}
/**
* Simplified method form for invoking the ListDeploymentConfigs operation
* with an AsyncHandler.
*
* @see #listDeploymentConfigsAsync(ListDeploymentConfigsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listDeploymentConfigsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listDeploymentConfigsAsync(new ListDeploymentConfigsRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future listDeploymentGroupsAsync(
ListDeploymentGroupsRequest request) {
return listDeploymentGroupsAsync(request, null);
}
@Override
public java.util.concurrent.Future listDeploymentGroupsAsync(
final ListDeploymentGroupsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListDeploymentGroupsResult call() throws Exception {
ListDeploymentGroupsResult result;
try {
result = listDeploymentGroups(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listDeploymentInstancesAsync(
ListDeploymentInstancesRequest request) {
return listDeploymentInstancesAsync(request, null);
}
@Override
public java.util.concurrent.Future listDeploymentInstancesAsync(
final ListDeploymentInstancesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListDeploymentInstancesResult call()
throws Exception {
ListDeploymentInstancesResult result;
try {
result = listDeploymentInstances(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listDeploymentsAsync(
ListDeploymentsRequest request) {
return listDeploymentsAsync(request, null);
}
@Override
public java.util.concurrent.Future listDeploymentsAsync(
final ListDeploymentsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListDeploymentsResult call() throws Exception {
ListDeploymentsResult result;
try {
result = listDeployments(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListDeployments operation.
*
* @see #listDeploymentsAsync(ListDeploymentsRequest)
*/
@Override
public java.util.concurrent.Future listDeploymentsAsync() {
return listDeploymentsAsync(new ListDeploymentsRequest());
}
/**
* Simplified method form for invoking the ListDeployments operation with an
* AsyncHandler.
*
* @see #listDeploymentsAsync(ListDeploymentsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listDeploymentsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listDeploymentsAsync(new ListDeploymentsRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future listOnPremisesInstancesAsync(
ListOnPremisesInstancesRequest request) {
return listOnPremisesInstancesAsync(request, null);
}
@Override
public java.util.concurrent.Future listOnPremisesInstancesAsync(
final ListOnPremisesInstancesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListOnPremisesInstancesResult call()
throws Exception {
ListOnPremisesInstancesResult result;
try {
result = listOnPremisesInstances(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListOnPremisesInstances
* operation.
*
* @see #listOnPremisesInstancesAsync(ListOnPremisesInstancesRequest)
*/
@Override
public java.util.concurrent.Future listOnPremisesInstancesAsync() {
return listOnPremisesInstancesAsync(new ListOnPremisesInstancesRequest());
}
/**
* Simplified method form for invoking the ListOnPremisesInstances operation
* with an AsyncHandler.
*
* @see #listOnPremisesInstancesAsync(ListOnPremisesInstancesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listOnPremisesInstancesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listOnPremisesInstancesAsync(
new ListOnPremisesInstancesRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future registerApplicationRevisionAsync(
RegisterApplicationRevisionRequest request) {
return registerApplicationRevisionAsync(request, null);
}
@Override
public java.util.concurrent.Future registerApplicationRevisionAsync(
final RegisterApplicationRevisionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public RegisterApplicationRevisionResult call()
throws Exception {
RegisterApplicationRevisionResult result;
try {
result = registerApplicationRevision(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future registerOnPremisesInstanceAsync(
RegisterOnPremisesInstanceRequest request) {
return registerOnPremisesInstanceAsync(request, null);
}
@Override
public java.util.concurrent.Future registerOnPremisesInstanceAsync(
final RegisterOnPremisesInstanceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public RegisterOnPremisesInstanceResult call()
throws Exception {
RegisterOnPremisesInstanceResult result;
try {
result = registerOnPremisesInstance(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future removeTagsFromOnPremisesInstancesAsync(
RemoveTagsFromOnPremisesInstancesRequest request) {
return removeTagsFromOnPremisesInstancesAsync(request, null);
}
@Override
public java.util.concurrent.Future removeTagsFromOnPremisesInstancesAsync(
final RemoveTagsFromOnPremisesInstancesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public RemoveTagsFromOnPremisesInstancesResult call()
throws Exception {
RemoveTagsFromOnPremisesInstancesResult result;
try {
result = removeTagsFromOnPremisesInstances(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future stopDeploymentAsync(
StopDeploymentRequest request) {
return stopDeploymentAsync(request, null);
}
@Override
public java.util.concurrent.Future stopDeploymentAsync(
final StopDeploymentRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public StopDeploymentResult call() throws Exception {
StopDeploymentResult result;
try {
result = stopDeployment(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateApplicationAsync(
UpdateApplicationRequest request) {
return updateApplicationAsync(request, null);
}
@Override
public java.util.concurrent.Future updateApplicationAsync(
final UpdateApplicationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateApplicationResult call() throws Exception {
UpdateApplicationResult result;
try {
result = updateApplication(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the UpdateApplication operation.
*
* @see #updateApplicationAsync(UpdateApplicationRequest)
*/
@Override
public java.util.concurrent.Future updateApplicationAsync() {
return updateApplicationAsync(new UpdateApplicationRequest());
}
/**
* Simplified method form for invoking the UpdateApplication operation with
* an AsyncHandler.
*
* @see #updateApplicationAsync(UpdateApplicationRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future updateApplicationAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return updateApplicationAsync(new UpdateApplicationRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future updateDeploymentGroupAsync(
UpdateDeploymentGroupRequest request) {
return updateDeploymentGroupAsync(request, null);
}
@Override
public java.util.concurrent.Future updateDeploymentGroupAsync(
final UpdateDeploymentGroupRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateDeploymentGroupResult call() throws Exception {
UpdateDeploymentGroupResult result;
try {
result = updateDeploymentGroup(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by
* {@code getExecutorService().awaitTermination()} prior to calling this
* method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}