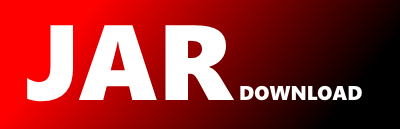
com.amazonaws.services.codedeploy.model.CreateDeploymentGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.codedeploy.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input of a create deployment group operation.
*
*/
public class CreateDeploymentGroupRequest extends AmazonWebServiceRequest
implements Serializable, Cloneable {
/**
*
* The name of an AWS CodeDeploy application associated with the applicable
* IAM user or AWS account.
*
*/
private String applicationName;
/**
*
* The name of a new deployment group for the specified application.
*
*/
private String deploymentGroupName;
/**
*
* If specified, the deployment configuration name can be either one of the
* predefined configurations provided with AWS CodeDeploy or a custom
* deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It
* is used if a configuration isn't specified for the deployment or the
* deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an application
* revision to as many instance as possible at once. The status of the
* overall deployment will be displayed as Succeeded if the
* application revision is deployed to one or more of the instances. The
* status of the overall deployment will be displayed as Failed if
* the application revision is not deployed to any of the instances. Using
* an example of nine instance, CodeDeployDefault.AllAtOnce will attempt to
* deploy to all nine instance at once. The overall deployment will succeed
* if deployment to even a single instance is successful; it will fail only
* if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall deployment
* succeeds if the application revision is deployed to at least half of the
* instances (with fractions rounded up); otherwise, the deployment fails.
* In the example of nine instances, it will deploy to up to four instance
* at a time. The overall deployment succeeds if deployment to five or more
* instances succeed; otherwise, the deployment fails. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application revision to
* only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is deployed
* to all of the instances. The exception to this rule is if deployment to
* the last instance fails, the overall deployment still succeeds. This is
* because AWS CodeDeploy allows only one instance at a time to be taken
* offline with the CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision fails to
* be deployed to any but the last instance. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance at a
* time. The overall deployment succeeds if deployment to the first eight
* instance is successful; the overall deployment fails if deployment to any
* of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single instance is
* successful
*
*
*
*/
private String deploymentConfigName;
/**
*
* The Amazon EC2 tags on which to filter.
*
*/
private com.amazonaws.internal.SdkInternalList ec2TagFilters;
/**
*
* The on-premises instance tags on which to filter.
*
*/
private com.amazonaws.internal.SdkInternalList onPremisesInstanceTagFilters;
/**
*
* A list of associated Auto Scaling groups.
*
*/
private com.amazonaws.internal.SdkInternalList autoScalingGroups;
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf
* when interacting with AWS services.
*
*/
private String serviceRoleArn;
/**
*
* Information about triggers to create when the deployment group is
* created.
*
*/
private com.amazonaws.internal.SdkInternalList triggerConfigurations;
/**
*
* The name of an AWS CodeDeploy application associated with the applicable
* IAM user or AWS account.
*
*
* @param applicationName
* The name of an AWS CodeDeploy application associated with the
* applicable IAM user or AWS account.
*/
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
/**
*
* The name of an AWS CodeDeploy application associated with the applicable
* IAM user or AWS account.
*
*
* @return The name of an AWS CodeDeploy application associated with the
* applicable IAM user or AWS account.
*/
public String getApplicationName() {
return this.applicationName;
}
/**
*
* The name of an AWS CodeDeploy application associated with the applicable
* IAM user or AWS account.
*
*
* @param applicationName
* The name of an AWS CodeDeploy application associated with the
* applicable IAM user or AWS account.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withApplicationName(
String applicationName) {
setApplicationName(applicationName);
return this;
}
/**
*
* The name of a new deployment group for the specified application.
*
*
* @param deploymentGroupName
* The name of a new deployment group for the specified application.
*/
public void setDeploymentGroupName(String deploymentGroupName) {
this.deploymentGroupName = deploymentGroupName;
}
/**
*
* The name of a new deployment group for the specified application.
*
*
* @return The name of a new deployment group for the specified application.
*/
public String getDeploymentGroupName() {
return this.deploymentGroupName;
}
/**
*
* The name of a new deployment group for the specified application.
*
*
* @param deploymentGroupName
* The name of a new deployment group for the specified application.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withDeploymentGroupName(
String deploymentGroupName) {
setDeploymentGroupName(deploymentGroupName);
return this;
}
/**
*
* If specified, the deployment configuration name can be either one of the
* predefined configurations provided with AWS CodeDeploy or a custom
* deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It
* is used if a configuration isn't specified for the deployment or the
* deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an application
* revision to as many instance as possible at once. The status of the
* overall deployment will be displayed as Succeeded if the
* application revision is deployed to one or more of the instances. The
* status of the overall deployment will be displayed as Failed if
* the application revision is not deployed to any of the instances. Using
* an example of nine instance, CodeDeployDefault.AllAtOnce will attempt to
* deploy to all nine instance at once. The overall deployment will succeed
* if deployment to even a single instance is successful; it will fail only
* if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall deployment
* succeeds if the application revision is deployed to at least half of the
* instances (with fractions rounded up); otherwise, the deployment fails.
* In the example of nine instances, it will deploy to up to four instance
* at a time. The overall deployment succeeds if deployment to five or more
* instances succeed; otherwise, the deployment fails. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application revision to
* only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is deployed
* to all of the instances. The exception to this rule is if deployment to
* the last instance fails, the overall deployment still succeeds. This is
* because AWS CodeDeploy allows only one instance at a time to be taken
* offline with the CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision fails to
* be deployed to any but the last instance. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance at a
* time. The overall deployment succeeds if deployment to the first eight
* instance is successful; the overall deployment fails if deployment to any
* of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single instance is
* successful
*
*
*
*
* @param deploymentConfigName
* If specified, the deployment configuration name can be either one
* of the predefined configurations provided with AWS CodeDeploy or a
* custom deployment configuration that you create by calling the
* create deployment configuration operation.
*
* CodeDeployDefault.OneAtATime is the default deployment
* configuration. It is used if a configuration isn't specified for
* the deployment or the deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an
* application revision to as many instance as possible at once. The
* status of the overall deployment will be displayed as
* Succeeded if the application revision is deployed to one or
* more of the instances. The status of the overall deployment will
* be displayed as Failed if the application revision is not
* deployed to any of the instances. Using an example of nine
* instance, CodeDeployDefault.AllAtOnce will attempt to deploy to
* all nine instance at once. The overall deployment will succeed if
* deployment to even a single instance is successful; it will fail
* only if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall
* deployment succeeds if the application revision is deployed to at
* least half of the instances (with fractions rounded up);
* otherwise, the deployment fails. In the example of nine instances,
* it will deploy to up to four instance at a time. The overall
* deployment succeeds if deployment to five or more instances
* succeed; otherwise, the deployment fails. The deployment may be
* successfully deployed to some instances even if the overall
* deployment fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application
* revision to only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is
* deployed to all of the instances. The exception to this rule is if
* deployment to the last instance fails, the overall deployment
* still succeeds. This is because AWS CodeDeploy allows only one
* instance at a time to be taken offline with the
* CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision
* fails to be deployed to any but the last instance. The deployment
* may be successfully deployed to some instances even if the overall
* deployment fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance
* at a time. The overall deployment succeeds if deployment to the
* first eight instance is successful; the overall deployment fails
* if deployment to any of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single instance
* is successful
*
*
*/
public void setDeploymentConfigName(String deploymentConfigName) {
this.deploymentConfigName = deploymentConfigName;
}
/**
*
* If specified, the deployment configuration name can be either one of the
* predefined configurations provided with AWS CodeDeploy or a custom
* deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It
* is used if a configuration isn't specified for the deployment or the
* deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an application
* revision to as many instance as possible at once. The status of the
* overall deployment will be displayed as Succeeded if the
* application revision is deployed to one or more of the instances. The
* status of the overall deployment will be displayed as Failed if
* the application revision is not deployed to any of the instances. Using
* an example of nine instance, CodeDeployDefault.AllAtOnce will attempt to
* deploy to all nine instance at once. The overall deployment will succeed
* if deployment to even a single instance is successful; it will fail only
* if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall deployment
* succeeds if the application revision is deployed to at least half of the
* instances (with fractions rounded up); otherwise, the deployment fails.
* In the example of nine instances, it will deploy to up to four instance
* at a time. The overall deployment succeeds if deployment to five or more
* instances succeed; otherwise, the deployment fails. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application revision to
* only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is deployed
* to all of the instances. The exception to this rule is if deployment to
* the last instance fails, the overall deployment still succeeds. This is
* because AWS CodeDeploy allows only one instance at a time to be taken
* offline with the CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision fails to
* be deployed to any but the last instance. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance at a
* time. The overall deployment succeeds if deployment to the first eight
* instance is successful; the overall deployment fails if deployment to any
* of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single instance is
* successful
*
*
*
*
* @return If specified, the deployment configuration name can be either one
* of the predefined configurations provided with AWS CodeDeploy or
* a custom deployment configuration that you create by calling the
* create deployment configuration operation.
*
* CodeDeployDefault.OneAtATime is the default deployment
* configuration. It is used if a configuration isn't specified for
* the deployment or the deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an
* application revision to as many instance as possible at once. The
* status of the overall deployment will be displayed as
* Succeeded if the application revision is deployed to one
* or more of the instances. The status of the overall deployment
* will be displayed as Failed if the application revision is
* not deployed to any of the instances. Using an example of nine
* instance, CodeDeployDefault.AllAtOnce will attempt to deploy to
* all nine instance at once. The overall deployment will succeed if
* deployment to even a single instance is successful; it will fail
* only if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall
* deployment succeeds if the application revision is deployed to at
* least half of the instances (with fractions rounded up);
* otherwise, the deployment fails. In the example of nine
* instances, it will deploy to up to four instance at a time. The
* overall deployment succeeds if deployment to five or more
* instances succeed; otherwise, the deployment fails. The
* deployment may be successfully deployed to some instances even if
* the overall deployment fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application
* revision to only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is
* deployed to all of the instances. The exception to this rule is
* if deployment to the last instance fails, the overall deployment
* still succeeds. This is because AWS CodeDeploy allows only one
* instance at a time to be taken offline with the
* CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision
* fails to be deployed to any but the last instance. The deployment
* may be successfully deployed to some instances even if the
* overall deployment fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance
* at a time. The overall deployment succeeds if deployment to the
* first eight instance is successful; the overall deployment fails
* if deployment to any of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single
* instance is successful
*
*
*/
public String getDeploymentConfigName() {
return this.deploymentConfigName;
}
/**
*
* If specified, the deployment configuration name can be either one of the
* predefined configurations provided with AWS CodeDeploy or a custom
* deployment configuration that you create by calling the create deployment
* configuration operation.
*
*
*
* CodeDeployDefault.OneAtATime is the default deployment configuration. It
* is used if a configuration isn't specified for the deployment or the
* deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an application
* revision to as many instance as possible at once. The status of the
* overall deployment will be displayed as Succeeded if the
* application revision is deployed to one or more of the instances. The
* status of the overall deployment will be displayed as Failed if
* the application revision is not deployed to any of the instances. Using
* an example of nine instance, CodeDeployDefault.AllAtOnce will attempt to
* deploy to all nine instance at once. The overall deployment will succeed
* if deployment to even a single instance is successful; it will fail only
* if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall deployment
* succeeds if the application revision is deployed to at least half of the
* instances (with fractions rounded up); otherwise, the deployment fails.
* In the example of nine instances, it will deploy to up to four instance
* at a time. The overall deployment succeeds if deployment to five or more
* instances succeed; otherwise, the deployment fails. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application revision to
* only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is deployed
* to all of the instances. The exception to this rule is if deployment to
* the last instance fails, the overall deployment still succeeds. This is
* because AWS CodeDeploy allows only one instance at a time to be taken
* offline with the CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision fails to
* be deployed to any but the last instance. The deployment may be
* successfully deployed to some instances even if the overall deployment
* fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance at a
* time. The overall deployment succeeds if deployment to the first eight
* instance is successful; the overall deployment fails if deployment to any
* of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single instance is
* successful
*
*
*
*
* @param deploymentConfigName
* If specified, the deployment configuration name can be either one
* of the predefined configurations provided with AWS CodeDeploy or a
* custom deployment configuration that you create by calling the
* create deployment configuration operation.
*
* CodeDeployDefault.OneAtATime is the default deployment
* configuration. It is used if a configuration isn't specified for
* the deployment or the deployment group.
*
*
*
* The predefined deployment configurations include the following:
*
*
* -
*
* CodeDeployDefault.AllAtOnce attempts to deploy an
* application revision to as many instance as possible at once. The
* status of the overall deployment will be displayed as
* Succeeded if the application revision is deployed to one or
* more of the instances. The status of the overall deployment will
* be displayed as Failed if the application revision is not
* deployed to any of the instances. Using an example of nine
* instance, CodeDeployDefault.AllAtOnce will attempt to deploy to
* all nine instance at once. The overall deployment will succeed if
* deployment to even a single instance is successful; it will fail
* only if deployments to all nine instance fail.
*
*
* -
*
* CodeDeployDefault.HalfAtATime deploys to up to half of the
* instances at a time (with fractions rounded down). The overall
* deployment succeeds if the application revision is deployed to at
* least half of the instances (with fractions rounded up);
* otherwise, the deployment fails. In the example of nine instances,
* it will deploy to up to four instance at a time. The overall
* deployment succeeds if deployment to five or more instances
* succeed; otherwise, the deployment fails. The deployment may be
* successfully deployed to some instances even if the overall
* deployment fails.
*
*
* -
*
* CodeDeployDefault.OneAtATime deploys the application
* revision to only one instance at a time.
*
*
* For deployment groups that contain more than one instance:
*
*
* -
*
* The overall deployment succeeds if the application revision is
* deployed to all of the instances. The exception to this rule is if
* deployment to the last instance fails, the overall deployment
* still succeeds. This is because AWS CodeDeploy allows only one
* instance at a time to be taken offline with the
* CodeDeployDefault.OneAtATime configuration.
*
*
* -
*
* The overall deployment fails as soon as the application revision
* fails to be deployed to any but the last instance. The deployment
* may be successfully deployed to some instances even if the overall
* deployment fails.
*
*
* -
*
* In an example using nine instance, it will deploy to one instance
* at a time. The overall deployment succeeds if deployment to the
* first eight instance is successful; the overall deployment fails
* if deployment to any of the first eight instance fails.
*
*
*
*
* For deployment groups that contain only one instance, the overall
* deployment is successful only if deployment to the single instance
* is successful
*
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withDeploymentConfigName(
String deploymentConfigName) {
setDeploymentConfigName(deploymentConfigName);
return this;
}
/**
*
* The Amazon EC2 tags on which to filter.
*
*
* @return The Amazon EC2 tags on which to filter.
*/
public java.util.List getEc2TagFilters() {
if (ec2TagFilters == null) {
ec2TagFilters = new com.amazonaws.internal.SdkInternalList();
}
return ec2TagFilters;
}
/**
*
* The Amazon EC2 tags on which to filter.
*
*
* @param ec2TagFilters
* The Amazon EC2 tags on which to filter.
*/
public void setEc2TagFilters(
java.util.Collection ec2TagFilters) {
if (ec2TagFilters == null) {
this.ec2TagFilters = null;
return;
}
this.ec2TagFilters = new com.amazonaws.internal.SdkInternalList(
ec2TagFilters);
}
/**
*
* The Amazon EC2 tags on which to filter.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setEc2TagFilters(java.util.Collection)} or
* {@link #withEc2TagFilters(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param ec2TagFilters
* The Amazon EC2 tags on which to filter.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withEc2TagFilters(
EC2TagFilter... ec2TagFilters) {
if (this.ec2TagFilters == null) {
setEc2TagFilters(new com.amazonaws.internal.SdkInternalList(
ec2TagFilters.length));
}
for (EC2TagFilter ele : ec2TagFilters) {
this.ec2TagFilters.add(ele);
}
return this;
}
/**
*
* The Amazon EC2 tags on which to filter.
*
*
* @param ec2TagFilters
* The Amazon EC2 tags on which to filter.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withEc2TagFilters(
java.util.Collection ec2TagFilters) {
setEc2TagFilters(ec2TagFilters);
return this;
}
/**
*
* The on-premises instance tags on which to filter.
*
*
* @return The on-premises instance tags on which to filter.
*/
public java.util.List getOnPremisesInstanceTagFilters() {
if (onPremisesInstanceTagFilters == null) {
onPremisesInstanceTagFilters = new com.amazonaws.internal.SdkInternalList();
}
return onPremisesInstanceTagFilters;
}
/**
*
* The on-premises instance tags on which to filter.
*
*
* @param onPremisesInstanceTagFilters
* The on-premises instance tags on which to filter.
*/
public void setOnPremisesInstanceTagFilters(
java.util.Collection onPremisesInstanceTagFilters) {
if (onPremisesInstanceTagFilters == null) {
this.onPremisesInstanceTagFilters = null;
return;
}
this.onPremisesInstanceTagFilters = new com.amazonaws.internal.SdkInternalList(
onPremisesInstanceTagFilters);
}
/**
*
* The on-premises instance tags on which to filter.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setOnPremisesInstanceTagFilters(java.util.Collection)}
* or {@link #withOnPremisesInstanceTagFilters(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param onPremisesInstanceTagFilters
* The on-premises instance tags on which to filter.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withOnPremisesInstanceTagFilters(
TagFilter... onPremisesInstanceTagFilters) {
if (this.onPremisesInstanceTagFilters == null) {
setOnPremisesInstanceTagFilters(new com.amazonaws.internal.SdkInternalList(
onPremisesInstanceTagFilters.length));
}
for (TagFilter ele : onPremisesInstanceTagFilters) {
this.onPremisesInstanceTagFilters.add(ele);
}
return this;
}
/**
*
* The on-premises instance tags on which to filter.
*
*
* @param onPremisesInstanceTagFilters
* The on-premises instance tags on which to filter.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withOnPremisesInstanceTagFilters(
java.util.Collection onPremisesInstanceTagFilters) {
setOnPremisesInstanceTagFilters(onPremisesInstanceTagFilters);
return this;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* @return A list of associated Auto Scaling groups.
*/
public java.util.List getAutoScalingGroups() {
if (autoScalingGroups == null) {
autoScalingGroups = new com.amazonaws.internal.SdkInternalList();
}
return autoScalingGroups;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* @param autoScalingGroups
* A list of associated Auto Scaling groups.
*/
public void setAutoScalingGroups(
java.util.Collection autoScalingGroups) {
if (autoScalingGroups == null) {
this.autoScalingGroups = null;
return;
}
this.autoScalingGroups = new com.amazonaws.internal.SdkInternalList(
autoScalingGroups);
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setAutoScalingGroups(java.util.Collection)} or
* {@link #withAutoScalingGroups(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param autoScalingGroups
* A list of associated Auto Scaling groups.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withAutoScalingGroups(
String... autoScalingGroups) {
if (this.autoScalingGroups == null) {
setAutoScalingGroups(new com.amazonaws.internal.SdkInternalList(
autoScalingGroups.length));
}
for (String ele : autoScalingGroups) {
this.autoScalingGroups.add(ele);
}
return this;
}
/**
*
* A list of associated Auto Scaling groups.
*
*
* @param autoScalingGroups
* A list of associated Auto Scaling groups.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withAutoScalingGroups(
java.util.Collection autoScalingGroups) {
setAutoScalingGroups(autoScalingGroups);
return this;
}
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf
* when interacting with AWS services.
*
*
* @param serviceRoleArn
* A service role ARN that allows AWS CodeDeploy to act on the user's
* behalf when interacting with AWS services.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf
* when interacting with AWS services.
*
*
* @return A service role ARN that allows AWS CodeDeploy to act on the
* user's behalf when interacting with AWS services.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* A service role ARN that allows AWS CodeDeploy to act on the user's behalf
* when interacting with AWS services.
*
*
* @param serviceRoleArn
* A service role ARN that allows AWS CodeDeploy to act on the user's
* behalf when interacting with AWS services.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* Information about triggers to create when the deployment group is
* created.
*
*
* @return Information about triggers to create when the deployment group is
* created.
*/
public java.util.List getTriggerConfigurations() {
if (triggerConfigurations == null) {
triggerConfigurations = new com.amazonaws.internal.SdkInternalList();
}
return triggerConfigurations;
}
/**
*
* Information about triggers to create when the deployment group is
* created.
*
*
* @param triggerConfigurations
* Information about triggers to create when the deployment group is
* created.
*/
public void setTriggerConfigurations(
java.util.Collection triggerConfigurations) {
if (triggerConfigurations == null) {
this.triggerConfigurations = null;
return;
}
this.triggerConfigurations = new com.amazonaws.internal.SdkInternalList(
triggerConfigurations);
}
/**
*
* Information about triggers to create when the deployment group is
* created.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setTriggerConfigurations(java.util.Collection)} or
* {@link #withTriggerConfigurations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param triggerConfigurations
* Information about triggers to create when the deployment group is
* created.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withTriggerConfigurations(
TriggerConfig... triggerConfigurations) {
if (this.triggerConfigurations == null) {
setTriggerConfigurations(new com.amazonaws.internal.SdkInternalList(
triggerConfigurations.length));
}
for (TriggerConfig ele : triggerConfigurations) {
this.triggerConfigurations.add(ele);
}
return this;
}
/**
*
* Information about triggers to create when the deployment group is
* created.
*
*
* @param triggerConfigurations
* Information about triggers to create when the deployment group is
* created.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateDeploymentGroupRequest withTriggerConfigurations(
java.util.Collection triggerConfigurations) {
setTriggerConfigurations(triggerConfigurations);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationName() != null)
sb.append("ApplicationName: " + getApplicationName() + ",");
if (getDeploymentGroupName() != null)
sb.append("DeploymentGroupName: " + getDeploymentGroupName() + ",");
if (getDeploymentConfigName() != null)
sb.append("DeploymentConfigName: " + getDeploymentConfigName()
+ ",");
if (getEc2TagFilters() != null)
sb.append("Ec2TagFilters: " + getEc2TagFilters() + ",");
if (getOnPremisesInstanceTagFilters() != null)
sb.append("OnPremisesInstanceTagFilters: "
+ getOnPremisesInstanceTagFilters() + ",");
if (getAutoScalingGroups() != null)
sb.append("AutoScalingGroups: " + getAutoScalingGroups() + ",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: " + getServiceRoleArn() + ",");
if (getTriggerConfigurations() != null)
sb.append("TriggerConfigurations: " + getTriggerConfigurations());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDeploymentGroupRequest == false)
return false;
CreateDeploymentGroupRequest other = (CreateDeploymentGroupRequest) obj;
if (other.getApplicationName() == null
^ this.getApplicationName() == null)
return false;
if (other.getApplicationName() != null
&& other.getApplicationName().equals(this.getApplicationName()) == false)
return false;
if (other.getDeploymentGroupName() == null
^ this.getDeploymentGroupName() == null)
return false;
if (other.getDeploymentGroupName() != null
&& other.getDeploymentGroupName().equals(
this.getDeploymentGroupName()) == false)
return false;
if (other.getDeploymentConfigName() == null
^ this.getDeploymentConfigName() == null)
return false;
if (other.getDeploymentConfigName() != null
&& other.getDeploymentConfigName().equals(
this.getDeploymentConfigName()) == false)
return false;
if (other.getEc2TagFilters() == null ^ this.getEc2TagFilters() == null)
return false;
if (other.getEc2TagFilters() != null
&& other.getEc2TagFilters().equals(this.getEc2TagFilters()) == false)
return false;
if (other.getOnPremisesInstanceTagFilters() == null
^ this.getOnPremisesInstanceTagFilters() == null)
return false;
if (other.getOnPremisesInstanceTagFilters() != null
&& other.getOnPremisesInstanceTagFilters().equals(
this.getOnPremisesInstanceTagFilters()) == false)
return false;
if (other.getAutoScalingGroups() == null
^ this.getAutoScalingGroups() == null)
return false;
if (other.getAutoScalingGroups() != null
&& other.getAutoScalingGroups().equals(
this.getAutoScalingGroups()) == false)
return false;
if (other.getServiceRoleArn() == null
^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null
&& other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getTriggerConfigurations() == null
^ this.getTriggerConfigurations() == null)
return false;
if (other.getTriggerConfigurations() != null
&& other.getTriggerConfigurations().equals(
this.getTriggerConfigurations()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getApplicationName() == null) ? 0 : getApplicationName()
.hashCode());
hashCode = prime
* hashCode
+ ((getDeploymentGroupName() == null) ? 0
: getDeploymentGroupName().hashCode());
hashCode = prime
* hashCode
+ ((getDeploymentConfigName() == null) ? 0
: getDeploymentConfigName().hashCode());
hashCode = prime
* hashCode
+ ((getEc2TagFilters() == null) ? 0 : getEc2TagFilters()
.hashCode());
hashCode = prime
* hashCode
+ ((getOnPremisesInstanceTagFilters() == null) ? 0
: getOnPremisesInstanceTagFilters().hashCode());
hashCode = prime
* hashCode
+ ((getAutoScalingGroups() == null) ? 0
: getAutoScalingGroups().hashCode());
hashCode = prime
* hashCode
+ ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn()
.hashCode());
hashCode = prime
* hashCode
+ ((getTriggerConfigurations() == null) ? 0
: getTriggerConfigurations().hashCode());
return hashCode;
}
@Override
public CreateDeploymentGroupRequest clone() {
return (CreateDeploymentGroupRequest) super.clone();
}
}