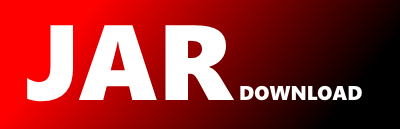
com.amazonaws.services.codedeploy.AmazonCodeDeploy Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.codedeploy;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.codedeploy.model.*;
import com.amazonaws.services.codedeploy.waiters.AmazonCodeDeployWaiters;
/**
* Interface for accessing CodeDeploy.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.codedeploy.AbstractAmazonCodeDeploy} instead.
*
*
*
* CodeDeploy is a deployment service that automates application deployments to Amazon EC2 instances, on-premises
* instances running in your own facility, serverless Lambda functions, or applications in an Amazon ECS service.
*
*
* You can deploy a nearly unlimited variety of application content, such as an updated Lambda function, updated
* applications in an Amazon ECS service, code, web and configuration files, executables, packages, scripts, multimedia
* files, and so on. CodeDeploy can deploy application content stored in Amazon S3 buckets, GitHub repositories, or
* Bitbucket repositories. You do not need to make changes to your existing code before you can use CodeDeploy.
*
*
* CodeDeploy makes it easier for you to rapidly release new features, helps you avoid downtime during application
* deployment, and handles the complexity of updating your applications, without many of the risks associated with
* error-prone manual deployments.
*
*
* CodeDeploy Components
*
*
* Use the information in this guide to help you work with the following CodeDeploy components:
*
*
* -
*
* Application: A name that uniquely identifies the application you want to deploy. CodeDeploy uses this name,
* which functions as a container, to ensure the correct combination of revision, deployment configuration, and
* deployment group are referenced during a deployment.
*
*
* -
*
* Deployment group: A set of individual instances, CodeDeploy Lambda deployment configuration settings, or an
* Amazon ECS service and network details. A Lambda deployment group specifies how to route traffic to a new version of
* a Lambda function. An Amazon ECS deployment group specifies the service created in Amazon ECS to deploy, a load
* balancer, and a listener to reroute production traffic to an updated containerized application. An Amazon
* EC2/On-premises deployment group contains individually tagged instances, Amazon EC2 instances in Amazon EC2 Auto
* Scaling groups, or both. All deployment groups can specify optional trigger, alarm, and rollback settings.
*
*
* -
*
* Deployment configuration: A set of deployment rules and deployment success and failure conditions used by
* CodeDeploy during a deployment.
*
*
* -
*
* Deployment: The process and the components used when updating a Lambda function, a containerized application
* in an Amazon ECS service, or of installing content on one or more instances.
*
*
* -
*
* Application revisions: For an Lambda deployment, this is an AppSpec file that specifies the Lambda function to
* be updated and one or more functions to validate deployment lifecycle events. For an Amazon ECS deployment, this is
* an AppSpec file that specifies the Amazon ECS task definition, container, and port where production traffic is
* rerouted. For an EC2/On-premises deployment, this is an archive file that contains source content—source code,
* webpages, executable files, and deployment scripts—along with an AppSpec file. Revisions are stored in Amazon S3
* buckets or GitHub repositories. For Amazon S3, a revision is uniquely identified by its Amazon S3 object key and its
* ETag, version, or both. For GitHub, a revision is uniquely identified by its commit ID.
*
*
*
*
* This guide also contains information to help you get details about the instances in your deployments, to make
* on-premises instances available for CodeDeploy deployments, to get details about a Lambda function deployment, and to
* get details about Amazon ECS service deployments.
*
*
* CodeDeploy Information Resources
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCodeDeploy {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "codedeploy";
/**
* Overrides the default endpoint for this client ("https://codedeploy.us-east-1.amazonaws.com"). Callers can use
* this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "codedeploy.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "https://codedeploy.us-east-1.amazonaws.com"). If the protocol is not specified here, the default
* protocol from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "codedeploy.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://codedeploy.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonCodeDeploy#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Adds tags to on-premises instances.
*
*
* @param addTagsToOnPremisesInstancesRequest
* Represents the input of, and adds tags to, an on-premises instance operation.
* @return Result of the AddTagsToOnPremisesInstances operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws TagLimitExceededException
* The maximum allowed number of tags was exceeded.
* @throws InstanceLimitExceededException
* The maximum number of allowed on-premises instances in a single call was exceeded.
* @throws InstanceNotRegisteredException
* The specified on-premises instance is not registered.
* @sample AmazonCodeDeploy.AddTagsToOnPremisesInstances
* @see AWS API Documentation
*/
AddTagsToOnPremisesInstancesResult addTagsToOnPremisesInstances(AddTagsToOnPremisesInstancesRequest addTagsToOnPremisesInstancesRequest);
/**
*
* Gets information about one or more application revisions. The maximum number of application revisions that can be
* returned is 25.
*
*
* @param batchGetApplicationRevisionsRequest
* Represents the input of a BatchGetApplicationRevisions
operation.
* @return Result of the BatchGetApplicationRevisions operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @sample AmazonCodeDeploy.BatchGetApplicationRevisions
* @see AWS API Documentation
*/
BatchGetApplicationRevisionsResult batchGetApplicationRevisions(BatchGetApplicationRevisionsRequest batchGetApplicationRevisionsRequest);
/**
*
* Gets information about one or more applications. The maximum number of applications that can be returned is 100.
*
*
* @param batchGetApplicationsRequest
* Represents the input of a BatchGetApplications
operation.
* @return Result of the BatchGetApplications operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @sample AmazonCodeDeploy.BatchGetApplications
* @see AWS API Documentation
*/
BatchGetApplicationsResult batchGetApplications(BatchGetApplicationsRequest batchGetApplicationsRequest);
/**
* Simplified method form for invoking the BatchGetApplications operation.
*
* @see #batchGetApplications(BatchGetApplicationsRequest)
*/
BatchGetApplicationsResult batchGetApplications();
/**
*
* Gets information about one or more deployment groups.
*
*
* @param batchGetDeploymentGroupsRequest
* Represents the input of a BatchGetDeploymentGroups
operation.
* @return Result of the BatchGetDeploymentGroups operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @sample AmazonCodeDeploy.BatchGetDeploymentGroups
* @see AWS API Documentation
*/
BatchGetDeploymentGroupsResult batchGetDeploymentGroups(BatchGetDeploymentGroupsRequest batchGetDeploymentGroupsRequest);
/**
*
*
* This method works, but is deprecated. Use BatchGetDeploymentTargets
instead.
*
*
*
* Returns an array of one or more instances associated with a deployment. This method works with EC2/On-premises
* and Lambda compute platforms. The newer BatchGetDeploymentTargets
works with all compute platforms.
* The maximum number of instances that can be returned is 25.
*
*
* @param batchGetDeploymentInstancesRequest
* Represents the input of a BatchGetDeploymentInstances
operation.
* @return Result of the BatchGetDeploymentInstances operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws InstanceIdRequiredException
* The instance ID was not specified.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @sample AmazonCodeDeploy.BatchGetDeploymentInstances
* @see AWS API Documentation
*/
@Deprecated
BatchGetDeploymentInstancesResult batchGetDeploymentInstances(BatchGetDeploymentInstancesRequest batchGetDeploymentInstancesRequest);
/**
*
* Returns an array of one or more targets associated with a deployment. This method works with all compute types
* and should be used instead of the deprecated BatchGetDeploymentInstances
. The maximum number of
* targets that can be returned is 25.
*
*
* The type of targets returned depends on the deployment's compute platform or deployment method:
*
*
* -
*
* EC2/On-premises: Information about Amazon EC2 instance targets.
*
*
* -
*
* Lambda: Information about Lambda functions targets.
*
*
* -
*
* Amazon ECS: Information about Amazon ECS service targets.
*
*
* -
*
* CloudFormation: Information about targets of blue/green deployments initiated by a CloudFormation stack
* update.
*
*
*
*
* @param batchGetDeploymentTargetsRequest
* @return Result of the BatchGetDeploymentTargets operation returned by the service.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws DeploymentTargetIdRequiredException
* A deployment target ID was not provided.
* @throws InvalidDeploymentTargetIdException
* The target ID provided was not valid.
* @throws DeploymentTargetDoesNotExistException
* The provided target ID does not belong to the attempted deployment.
* @throws DeploymentTargetListSizeExceededException
* The maximum number of targets that can be associated with an Amazon ECS or Lambda deployment was
* exceeded. The target list of both types of deployments must have exactly one item. This exception does
* not apply to EC2/On-premises deployments.
* @throws InstanceDoesNotExistException
* The specified instance does not exist in the deployment group.
* @sample AmazonCodeDeploy.BatchGetDeploymentTargets
* @see AWS API Documentation
*/
BatchGetDeploymentTargetsResult batchGetDeploymentTargets(BatchGetDeploymentTargetsRequest batchGetDeploymentTargetsRequest);
/**
*
* Gets information about one or more deployments. The maximum number of deployments that can be returned is 25.
*
*
* @param batchGetDeploymentsRequest
* Represents the input of a BatchGetDeployments
operation.
* @return Result of the BatchGetDeployments operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @sample AmazonCodeDeploy.BatchGetDeployments
* @see AWS
* API Documentation
*/
BatchGetDeploymentsResult batchGetDeployments(BatchGetDeploymentsRequest batchGetDeploymentsRequest);
/**
* Simplified method form for invoking the BatchGetDeployments operation.
*
* @see #batchGetDeployments(BatchGetDeploymentsRequest)
*/
BatchGetDeploymentsResult batchGetDeployments();
/**
*
* Gets information about one or more on-premises instances. The maximum number of on-premises instances that can be
* returned is 25.
*
*
* @param batchGetOnPremisesInstancesRequest
* Represents the input of a BatchGetOnPremisesInstances
operation.
* @return Result of the BatchGetOnPremisesInstances operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws BatchLimitExceededException
* The maximum number of names or IDs allowed for this request (100) was exceeded.
* @sample AmazonCodeDeploy.BatchGetOnPremisesInstances
* @see AWS API Documentation
*/
BatchGetOnPremisesInstancesResult batchGetOnPremisesInstances(BatchGetOnPremisesInstancesRequest batchGetOnPremisesInstancesRequest);
/**
* Simplified method form for invoking the BatchGetOnPremisesInstances operation.
*
* @see #batchGetOnPremisesInstances(BatchGetOnPremisesInstancesRequest)
*/
BatchGetOnPremisesInstancesResult batchGetOnPremisesInstances();
/**
*
* For a blue/green deployment, starts the process of rerouting traffic from instances in the original environment
* to instances in the replacement environment without waiting for a specified wait time to elapse. (Traffic
* rerouting, which is achieved by registering instances in the replacement environment with the load balancer, can
* start as soon as all instances have a status of Ready.)
*
*
* @param continueDeploymentRequest
* @return Result of the ContinueDeployment operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentAlreadyCompletedException
* The deployment is already complete.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentIsNotInReadyStateException
* The deployment does not have a status of Ready and can't continue yet.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @throws InvalidDeploymentWaitTypeException
* The wait type is invalid.
* @throws InvalidDeploymentStatusException
* The specified deployment status doesn't exist or cannot be determined.
* @sample AmazonCodeDeploy.ContinueDeployment
* @see AWS
* API Documentation
*/
ContinueDeploymentResult continueDeployment(ContinueDeploymentRequest continueDeploymentRequest);
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* Represents the input of a CreateApplication
operation.
* @return Result of the CreateApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationAlreadyExistsException
* An application with the specified name with the user or Amazon Web Services account already exists.
* @throws ApplicationLimitExceededException
* More applications were attempted to be created than are allowed.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @sample AmazonCodeDeploy.CreateApplication
* @see AWS
* API Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Deploys an application revision through the specified deployment group.
*
*
* @param createDeploymentRequest
* Represents the input of a CreateDeployment
operation.
* @return Result of the CreateDeployment operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws RevisionDoesNotExistException
* The named revision does not exist with the user or Amazon Web Services account.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws DescriptionTooLongException
* The description is too long.
* @throws DeploymentLimitExceededException
* The number of allowed deployments was exceeded.
* @throws InvalidTargetInstancesException
* The target instance configuration is invalid. Possible causes include:
*
* -
*
* Configuration data for target instances was entered for an in-place deployment.
*
*
* -
*
* The limit of 10 tags for a tag type was exceeded.
*
*
* -
*
* The combined length of the tag names exceeded the limit.
*
*
* -
*
* A specified tag is not currently applied to any instances.
*
*
* @throws InvalidAlarmConfigException
* The format of the alarm configuration is invalid. Possible causes include:
*
* -
*
* The alarm list is null.
*
*
* -
*
* The alarm object is null.
*
*
* -
*
* The alarm name is empty or null or exceeds the limit of 255 characters.
*
*
* -
*
* Two alarms with the same name have been specified.
*
*
* -
*
* The alarm configuration is enabled, but the alarm list is empty.
*
*
* @throws AlarmsLimitExceededException
* The maximum number of alarms for a deployment group (10) was exceeded.
* @throws InvalidAutoRollbackConfigException
* The automatic rollback configuration was specified in an invalid format. For example, automatic rollback
* is enabled, but an invalid triggering event type or no event types were listed.
* @throws InvalidLoadBalancerInfoException
* An invalid load balancer name, or no load balancer name, was specified.
* @throws InvalidFileExistsBehaviorException
* An invalid fileExistsBehavior option was specified to determine how CodeDeploy handles files or
* directories that already exist in a deployment target location, but weren't part of the previous
* successful deployment. Valid values include "DISALLOW," "OVERWRITE," and "RETAIN."
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws InvalidAutoScalingGroupException
* The Auto Scaling group was specified in an invalid format or does not exist.
* @throws ThrottlingException
* An API function was called too frequently.
* @throws InvalidUpdateOutdatedInstancesOnlyValueException
* The UpdateOutdatedInstancesOnly value is invalid. For Lambda deployments, false
is expected.
* For EC2/On-premises deployments, true
or false
is expected.
* @throws InvalidIgnoreApplicationStopFailuresValueException
* The IgnoreApplicationStopFailures value is invalid. For Lambda deployments, false
is
* expected. For EC2/On-premises deployments, true
or false
is expected.
* @throws InvalidGitHubAccountTokenException
* The GitHub token is not valid.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @sample AmazonCodeDeploy.CreateDeployment
* @see AWS
* API Documentation
*/
CreateDeploymentResult createDeployment(CreateDeploymentRequest createDeploymentRequest);
/**
*
* Creates a deployment configuration.
*
*
* @param createDeploymentConfigRequest
* Represents the input of a CreateDeploymentConfig
operation.
* @return Result of the CreateDeploymentConfig operation returned by the service.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigNameRequiredException
* The deployment configuration name was not specified.
* @throws DeploymentConfigAlreadyExistsException
* A deployment configuration with the specified name with the user or Amazon Web Services account already
* exists.
* @throws InvalidMinimumHealthyHostValueException
* The minimum healthy instance value was specified in an invalid format.
* @throws DeploymentConfigLimitExceededException
* The deployment configurations limit was exceeded.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @throws InvalidZonalDeploymentConfigurationException
* The ZonalConfig
object is not valid.
* @sample AmazonCodeDeploy.CreateDeploymentConfig
* @see AWS API Documentation
*/
CreateDeploymentConfigResult createDeploymentConfig(CreateDeploymentConfigRequest createDeploymentConfigRequest);
/**
*
* Creates a deployment group to which application revisions are deployed.
*
*
* @param createDeploymentGroupRequest
* Represents the input of a CreateDeploymentGroup
operation.
* @return Result of the CreateDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupAlreadyExistsException
* A deployment group with the specified name with the user or Amazon Web Services account already exists.
* @throws InvalidEC2TagException
* The tag was specified in an invalid format.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws InvalidAutoScalingGroupException
* The Auto Scaling group was specified in an invalid format or does not exist.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws RoleRequiredException
* The role ID was not specified.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws DeploymentGroupLimitExceededException
* The deployment groups limit was exceeded.
* @throws LifecycleHookLimitExceededException
* The limit for lifecycle hooks was exceeded.
* @throws InvalidTriggerConfigException
* The trigger was specified in an invalid format.
* @throws TriggerTargetsLimitExceededException
* The maximum allowed number of triggers was exceeded.
* @throws InvalidAlarmConfigException
* The format of the alarm configuration is invalid. Possible causes include:
*
* -
*
* The alarm list is null.
*
*
* -
*
* The alarm object is null.
*
*
* -
*
* The alarm name is empty or null or exceeds the limit of 255 characters.
*
*
* -
*
* Two alarms with the same name have been specified.
*
*
* -
*
* The alarm configuration is enabled, but the alarm list is empty.
*
*
* @throws AlarmsLimitExceededException
* The maximum number of alarms for a deployment group (10) was exceeded.
* @throws InvalidAutoRollbackConfigException
* The automatic rollback configuration was specified in an invalid format. For example, automatic rollback
* is enabled, but an invalid triggering event type or no event types were listed.
* @throws InvalidLoadBalancerInfoException
* An invalid load balancer name, or no load balancer name, was specified.
* @throws InvalidDeploymentStyleException
* An invalid deployment style was specified. Valid deployment types include "IN_PLACE" and "BLUE_GREEN."
* Valid deployment options include "WITH_TRAFFIC_CONTROL" and "WITHOUT_TRAFFIC_CONTROL."
* @throws InvalidBlueGreenDeploymentConfigurationException
* The configuration for the blue/green deployment group was provided in an invalid format. For information
* about deployment configuration format, see CreateDeploymentConfig.
* @throws InvalidEC2TagCombinationException
* A call was submitted that specified both Ec2TagFilters and Ec2TagSet, but only one of these data types
* can be used in a single call.
* @throws InvalidOnPremisesTagCombinationException
* A call was submitted that specified both OnPremisesTagFilters and OnPremisesTagSet, but only one of these
* data types can be used in a single call.
* @throws TagSetListLimitExceededException
* The number of tag groups included in the tag set list exceeded the maximum allowed limit of 3.
* @throws InvalidInputException
* The input was specified in an invalid format.
* @throws ThrottlingException
* An API function was called too frequently.
* @throws InvalidECSServiceException
* The Amazon ECS service identifier is not valid.
* @throws InvalidTargetGroupPairException
* A target group pair associated with this deployment is not valid.
* @throws ECSServiceMappingLimitExceededException
* The Amazon ECS service is associated with more than one deployment groups. An Amazon ECS service can be
* associated with only one deployment group.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @sample AmazonCodeDeploy.CreateDeploymentGroup
* @see AWS API Documentation
*/
CreateDeploymentGroupResult createDeploymentGroup(CreateDeploymentGroupRequest createDeploymentGroupRequest);
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* Represents the input of a DeleteApplication
operation.
* @return Result of the DeleteApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @sample AmazonCodeDeploy.DeleteApplication
* @see AWS
* API Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes a deployment configuration.
*
*
*
* A deployment configuration cannot be deleted if it is currently in use. Predefined configurations cannot be
* deleted.
*
*
*
* @param deleteDeploymentConfigRequest
* Represents the input of a DeleteDeploymentConfig
operation.
* @return Result of the DeleteDeploymentConfig operation returned by the service.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigNameRequiredException
* The deployment configuration name was not specified.
* @throws DeploymentConfigInUseException
* The deployment configuration is still in use.
* @throws InvalidOperationException
* An invalid operation was detected.
* @sample AmazonCodeDeploy.DeleteDeploymentConfig
* @see AWS API Documentation
*/
DeleteDeploymentConfigResult deleteDeploymentConfig(DeleteDeploymentConfigRequest deleteDeploymentConfigRequest);
/**
*
* Deletes a deployment group.
*
*
* @param deleteDeploymentGroupRequest
* Represents the input of a DeleteDeploymentGroup
operation.
* @return Result of the DeleteDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @sample AmazonCodeDeploy.DeleteDeploymentGroup
* @see AWS API Documentation
*/
DeleteDeploymentGroupResult deleteDeploymentGroup(DeleteDeploymentGroupRequest deleteDeploymentGroupRequest);
/**
*
* Deletes a GitHub account connection.
*
*
* @param deleteGitHubAccountTokenRequest
* Represents the input of a DeleteGitHubAccount
operation.
* @return Result of the DeleteGitHubAccountToken operation returned by the service.
* @throws GitHubAccountTokenNameRequiredException
* The call is missing a required GitHub account connection name.
* @throws GitHubAccountTokenDoesNotExistException
* No GitHub account connection exists with the named specified in the call.
* @throws InvalidGitHubAccountTokenNameException
* The format of the specified GitHub account connection name is invalid.
* @throws ResourceValidationException
* The specified resource could not be validated.
* @throws OperationNotSupportedException
* The API used does not support the deployment.
* @sample AmazonCodeDeploy.DeleteGitHubAccountToken
* @see AWS API Documentation
*/
DeleteGitHubAccountTokenResult deleteGitHubAccountToken(DeleteGitHubAccountTokenRequest deleteGitHubAccountTokenRequest);
/**
*
* Deletes resources linked to an external ID. This action only applies if you have configured blue/green
* deployments through CloudFormation.
*
*
*
* It is not necessary to call this action directly. CloudFormation calls it on your behalf when it needs to delete
* stack resources. This action is offered publicly in case you need to delete resources to comply with General Data
* Protection Regulation (GDPR) requirements.
*
*
*
* @param deleteResourcesByExternalIdRequest
* @return Result of the DeleteResourcesByExternalId operation returned by the service.
* @sample AmazonCodeDeploy.DeleteResourcesByExternalId
* @see AWS API Documentation
*/
DeleteResourcesByExternalIdResult deleteResourcesByExternalId(DeleteResourcesByExternalIdRequest deleteResourcesByExternalIdRequest);
/**
*
* Deregisters an on-premises instance.
*
*
* @param deregisterOnPremisesInstanceRequest
* Represents the input of a DeregisterOnPremisesInstance
operation.
* @return Result of the DeregisterOnPremisesInstance operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @sample AmazonCodeDeploy.DeregisterOnPremisesInstance
* @see AWS API Documentation
*/
DeregisterOnPremisesInstanceResult deregisterOnPremisesInstance(DeregisterOnPremisesInstanceRequest deregisterOnPremisesInstanceRequest);
/**
*
* Gets information about an application.
*
*
* @param getApplicationRequest
* Represents the input of a GetApplication
operation.
* @return Result of the GetApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @sample AmazonCodeDeploy.GetApplication
* @see AWS API
* Documentation
*/
GetApplicationResult getApplication(GetApplicationRequest getApplicationRequest);
/**
*
* Gets information about an application revision.
*
*
* @param getApplicationRevisionRequest
* Represents the input of a GetApplicationRevision
operation.
* @return Result of the GetApplicationRevision operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws RevisionDoesNotExistException
* The named revision does not exist with the user or Amazon Web Services account.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @sample AmazonCodeDeploy.GetApplicationRevision
* @see AWS API Documentation
*/
GetApplicationRevisionResult getApplicationRevision(GetApplicationRevisionRequest getApplicationRevisionRequest);
/**
*
* Gets information about a deployment.
*
*
*
* The content
property of the appSpecContent
object in the returned revision is always
* null. Use GetApplicationRevision
and the sha256
property of the returned
* appSpecContent
object to get the content of the deployment’s AppSpec file.
*
*
*
* @param getDeploymentRequest
* Represents the input of a GetDeployment
operation.
* @return Result of the GetDeployment operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @sample AmazonCodeDeploy.GetDeployment
* @see AWS API
* Documentation
*/
GetDeploymentResult getDeployment(GetDeploymentRequest getDeploymentRequest);
/**
*
* Gets information about a deployment configuration.
*
*
* @param getDeploymentConfigRequest
* Represents the input of a GetDeploymentConfig
operation.
* @return Result of the GetDeploymentConfig operation returned by the service.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigNameRequiredException
* The deployment configuration name was not specified.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @sample AmazonCodeDeploy.GetDeploymentConfig
* @see AWS
* API Documentation
*/
GetDeploymentConfigResult getDeploymentConfig(GetDeploymentConfigRequest getDeploymentConfigRequest);
/**
*
* Gets information about a deployment group.
*
*
* @param getDeploymentGroupRequest
* Represents the input of a GetDeploymentGroup
operation.
* @return Result of the GetDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @sample AmazonCodeDeploy.GetDeploymentGroup
* @see AWS
* API Documentation
*/
GetDeploymentGroupResult getDeploymentGroup(GetDeploymentGroupRequest getDeploymentGroupRequest);
/**
*
* Gets information about an instance as part of a deployment.
*
*
* @param getDeploymentInstanceRequest
* Represents the input of a GetDeploymentInstance
operation.
* @return Result of the GetDeploymentInstance operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws InstanceIdRequiredException
* The instance ID was not specified.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws InstanceDoesNotExistException
* The specified instance does not exist in the deployment group.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @sample AmazonCodeDeploy.GetDeploymentInstance
* @see AWS API Documentation
*/
@Deprecated
GetDeploymentInstanceResult getDeploymentInstance(GetDeploymentInstanceRequest getDeploymentInstanceRequest);
/**
*
* Returns information about a deployment target.
*
*
* @param getDeploymentTargetRequest
* @return Result of the GetDeploymentTarget operation returned by the service.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws DeploymentTargetIdRequiredException
* A deployment target ID was not provided.
* @throws InvalidDeploymentTargetIdException
* The target ID provided was not valid.
* @throws DeploymentTargetDoesNotExistException
* The provided target ID does not belong to the attempted deployment.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @sample AmazonCodeDeploy.GetDeploymentTarget
* @see AWS
* API Documentation
*/
GetDeploymentTargetResult getDeploymentTarget(GetDeploymentTargetRequest getDeploymentTargetRequest);
/**
*
* Gets information about an on-premises instance.
*
*
* @param getOnPremisesInstanceRequest
* Represents the input of a GetOnPremisesInstance
operation.
* @return Result of the GetOnPremisesInstance operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InstanceNotRegisteredException
* The specified on-premises instance is not registered.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @sample AmazonCodeDeploy.GetOnPremisesInstance
* @see AWS API Documentation
*/
GetOnPremisesInstanceResult getOnPremisesInstance(GetOnPremisesInstanceRequest getOnPremisesInstanceRequest);
/**
*
* Lists information about revisions for an application.
*
*
* @param listApplicationRevisionsRequest
* Represents the input of a ListApplicationRevisions
operation.
* @return Result of the ListApplicationRevisions operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws InvalidSortByException
* The column name to sort by is either not present or was specified in an invalid format.
* @throws InvalidSortOrderException
* The sort order was specified in an invalid format.
* @throws InvalidBucketNameFilterException
* The bucket name either doesn't exist or was specified in an invalid format.
* @throws InvalidKeyPrefixFilterException
* The specified key prefix filter was specified in an invalid format.
* @throws BucketNameFilterRequiredException
* A bucket name is required, but was not provided.
* @throws InvalidDeployedStateFilterException
* The deployed state filter was specified in an invalid format.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @sample AmazonCodeDeploy.ListApplicationRevisions
* @see AWS API Documentation
*/
ListApplicationRevisionsResult listApplicationRevisions(ListApplicationRevisionsRequest listApplicationRevisionsRequest);
/**
*
* Lists the applications registered with the user or Amazon Web Services account.
*
*
* @param listApplicationsRequest
* Represents the input of a ListApplications
operation.
* @return Result of the ListApplications operation returned by the service.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @sample AmazonCodeDeploy.ListApplications
* @see AWS
* API Documentation
*/
ListApplicationsResult listApplications(ListApplicationsRequest listApplicationsRequest);
/**
* Simplified method form for invoking the ListApplications operation.
*
* @see #listApplications(ListApplicationsRequest)
*/
ListApplicationsResult listApplications();
/**
*
* Lists the deployment configurations with the user or Amazon Web Services account.
*
*
* @param listDeploymentConfigsRequest
* Represents the input of a ListDeploymentConfigs
operation.
* @return Result of the ListDeploymentConfigs operation returned by the service.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @sample AmazonCodeDeploy.ListDeploymentConfigs
* @see AWS API Documentation
*/
ListDeploymentConfigsResult listDeploymentConfigs(ListDeploymentConfigsRequest listDeploymentConfigsRequest);
/**
* Simplified method form for invoking the ListDeploymentConfigs operation.
*
* @see #listDeploymentConfigs(ListDeploymentConfigsRequest)
*/
ListDeploymentConfigsResult listDeploymentConfigs();
/**
*
* Lists the deployment groups for an application registered with the Amazon Web Services user or Amazon Web
* Services account.
*
*
* @param listDeploymentGroupsRequest
* Represents the input of a ListDeploymentGroups
operation.
* @return Result of the ListDeploymentGroups operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @sample AmazonCodeDeploy.ListDeploymentGroups
* @see AWS API Documentation
*/
ListDeploymentGroupsResult listDeploymentGroups(ListDeploymentGroupsRequest listDeploymentGroupsRequest);
/**
*
*
* The newer BatchGetDeploymentTargets
should be used instead because it works with all compute types.
* ListDeploymentInstances
throws an exception if it is used with a compute platform other than
* EC2/On-premises or Lambda.
*
*
*
* Lists the instance for a deployment associated with the user or Amazon Web Services account.
*
*
* @param listDeploymentInstancesRequest
* Represents the input of a ListDeploymentInstances
operation.
* @return Result of the ListDeploymentInstances operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws InvalidInstanceStatusException
* The specified instance status does not exist.
* @throws InvalidInstanceTypeException
* An invalid instance type was specified for instances in a blue/green deployment. Valid values include
* "Blue" for an original environment and "Green" for a replacement environment.
* @throws InvalidDeploymentInstanceTypeException
* An instance type was specified for an in-place deployment. Instance types are supported for blue/green
* deployments only.
* @throws InvalidTargetFilterNameException
* The target filter name is invalid.
* @throws InvalidComputePlatformException
* The computePlatform is invalid. The computePlatform should be Lambda
, Server
,
* or ECS
.
* @sample AmazonCodeDeploy.ListDeploymentInstances
* @see AWS API Documentation
*/
@Deprecated
ListDeploymentInstancesResult listDeploymentInstances(ListDeploymentInstancesRequest listDeploymentInstancesRequest);
/**
*
* Returns an array of target IDs that are associated a deployment.
*
*
* @param listDeploymentTargetsRequest
* @return Result of the ListDeploymentTargets operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws InvalidInstanceStatusException
* The specified instance status does not exist.
* @throws InvalidInstanceTypeException
* An invalid instance type was specified for instances in a blue/green deployment. Valid values include
* "Blue" for an original environment and "Green" for a replacement environment.
* @throws InvalidDeploymentInstanceTypeException
* An instance type was specified for an in-place deployment. Instance types are supported for blue/green
* deployments only.
* @throws InvalidTargetFilterNameException
* The target filter name is invalid.
* @sample AmazonCodeDeploy.ListDeploymentTargets
* @see AWS API Documentation
*/
ListDeploymentTargetsResult listDeploymentTargets(ListDeploymentTargetsRequest listDeploymentTargetsRequest);
/**
*
* Lists the deployments in a deployment group for an application registered with the user or Amazon Web Services
* account.
*
*
* @param listDeploymentsRequest
* Represents the input of a ListDeployments
operation.
* @return Result of the ListDeployments operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws InvalidTimeRangeException
* The specified time range was specified in an invalid format.
* @throws InvalidDeploymentStatusException
* The specified deployment status doesn't exist or cannot be determined.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws InvalidExternalIdException
* The external ID was specified in an invalid format.
* @throws InvalidInputException
* The input was specified in an invalid format.
* @sample AmazonCodeDeploy.ListDeployments
* @see AWS API
* Documentation
*/
ListDeploymentsResult listDeployments(ListDeploymentsRequest listDeploymentsRequest);
/**
* Simplified method form for invoking the ListDeployments operation.
*
* @see #listDeployments(ListDeploymentsRequest)
*/
ListDeploymentsResult listDeployments();
/**
*
* Lists the names of stored connections to GitHub accounts.
*
*
* @param listGitHubAccountTokenNamesRequest
* Represents the input of a ListGitHubAccountTokenNames
operation.
* @return Result of the ListGitHubAccountTokenNames operation returned by the service.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @throws ResourceValidationException
* The specified resource could not be validated.
* @throws OperationNotSupportedException
* The API used does not support the deployment.
* @sample AmazonCodeDeploy.ListGitHubAccountTokenNames
* @see AWS API Documentation
*/
ListGitHubAccountTokenNamesResult listGitHubAccountTokenNames(ListGitHubAccountTokenNamesRequest listGitHubAccountTokenNamesRequest);
/**
*
* Gets a list of names for one or more on-premises instances.
*
*
* Unless otherwise specified, both registered and deregistered on-premises instance names are listed. To list only
* registered or deregistered on-premises instance names, use the registration status parameter.
*
*
* @param listOnPremisesInstancesRequest
* Represents the input of a ListOnPremisesInstances
operation.
* @return Result of the ListOnPremisesInstances operation returned by the service.
* @throws InvalidRegistrationStatusException
* The registration status was specified in an invalid format.
* @throws InvalidTagFilterException
* The tag filter was specified in an invalid format.
* @throws InvalidNextTokenException
* The next token was specified in an invalid format.
* @sample AmazonCodeDeploy.ListOnPremisesInstances
* @see AWS API Documentation
*/
ListOnPremisesInstancesResult listOnPremisesInstances(ListOnPremisesInstancesRequest listOnPremisesInstancesRequest);
/**
* Simplified method form for invoking the ListOnPremisesInstances operation.
*
* @see #listOnPremisesInstances(ListOnPremisesInstancesRequest)
*/
ListOnPremisesInstancesResult listOnPremisesInstances();
/**
*
* Returns a list of tags for the resource identified by a specified Amazon Resource Name (ARN). Tags are used to
* organize and categorize your CodeDeploy resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ArnNotSupportedException
* The specified ARN is not supported. For example, it might be an ARN for a resource that is not expected.
* @throws InvalidArnException
* The specified ARN is not in a valid format.
* @throws ResourceArnRequiredException
* The ARN of a resource is required, but was not found.
* @sample AmazonCodeDeploy.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Sets the result of a Lambda validation function. The function validates lifecycle hooks during a deployment that
* uses the Lambda or Amazon ECS compute platform. For Lambda deployments, the available lifecycle hooks are
* BeforeAllowTraffic
and AfterAllowTraffic
. For Amazon ECS deployments, the available
* lifecycle hooks are BeforeInstall
, AfterInstall
, AfterAllowTestTraffic
,
* BeforeAllowTraffic
, and AfterAllowTraffic
. Lambda validation functions return
* Succeeded
or Failed
. For more information, see AppSpec 'hooks' Section for an Lambda Deployment and AppSpec 'hooks' Section for an Amazon ECS Deployment.
*
*
* @param putLifecycleEventHookExecutionStatusRequest
* @return Result of the PutLifecycleEventHookExecutionStatus operation returned by the service.
* @throws InvalidLifecycleEventHookExecutionStatusException
* The result of a Lambda validation function that verifies a lifecycle event is invalid. It should return
* Succeeded
or Failed
.
* @throws InvalidLifecycleEventHookExecutionIdException
* A lifecycle event hook is invalid. Review the hooks
section in your AppSpec file to ensure
* the lifecycle events and hooks
functions are valid.
* @throws LifecycleEventAlreadyCompletedException
* An attempt to return the status of an already completed lifecycle event occurred.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @sample AmazonCodeDeploy.PutLifecycleEventHookExecutionStatus
* @see AWS API Documentation
*/
PutLifecycleEventHookExecutionStatusResult putLifecycleEventHookExecutionStatus(
PutLifecycleEventHookExecutionStatusRequest putLifecycleEventHookExecutionStatusRequest);
/**
*
* Registers with CodeDeploy a revision for the specified application.
*
*
* @param registerApplicationRevisionRequest
* Represents the input of a RegisterApplicationRevision operation.
* @return Result of the RegisterApplicationRevision operation returned by the service.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws DescriptionTooLongException
* The description is too long.
* @throws RevisionRequiredException
* The revision ID was not specified.
* @throws InvalidRevisionException
* The revision was specified in an invalid format.
* @sample AmazonCodeDeploy.RegisterApplicationRevision
* @see AWS API Documentation
*/
RegisterApplicationRevisionResult registerApplicationRevision(RegisterApplicationRevisionRequest registerApplicationRevisionRequest);
/**
*
* Registers an on-premises instance.
*
*
*
* Only one IAM ARN (an IAM session ARN or IAM user ARN) is supported in the request. You cannot use both.
*
*
*
* @param registerOnPremisesInstanceRequest
* Represents the input of the register on-premises instance operation.
* @return Result of the RegisterOnPremisesInstance operation returned by the service.
* @throws InstanceNameAlreadyRegisteredException
* The specified on-premises instance name is already registered.
* @throws IamArnRequiredException
* No IAM ARN was included in the request. You must use an IAM session ARN or user ARN in the request.
* @throws IamSessionArnAlreadyRegisteredException
* The request included an IAM session ARN that has already been used to register a different instance.
* @throws IamUserArnAlreadyRegisteredException
* The specified user ARN is already registered with an on-premises instance.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws IamUserArnRequiredException
* An user ARN was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws InvalidIamSessionArnException
* The IAM session ARN was specified in an invalid format.
* @throws InvalidIamUserArnException
* The user ARN was specified in an invalid format.
* @throws MultipleIamArnsProvidedException
* Both an user ARN and an IAM session ARN were included in the request. Use only one ARN type.
* @sample AmazonCodeDeploy.RegisterOnPremisesInstance
* @see AWS API Documentation
*/
RegisterOnPremisesInstanceResult registerOnPremisesInstance(RegisterOnPremisesInstanceRequest registerOnPremisesInstanceRequest);
/**
*
* Removes one or more tags from one or more on-premises instances.
*
*
* @param removeTagsFromOnPremisesInstancesRequest
* Represents the input of a RemoveTagsFromOnPremisesInstances
operation.
* @return Result of the RemoveTagsFromOnPremisesInstances operation returned by the service.
* @throws InstanceNameRequiredException
* An on-premises instance name was not specified.
* @throws InvalidInstanceNameException
* The on-premises instance name was specified in an invalid format.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws TagLimitExceededException
* The maximum allowed number of tags was exceeded.
* @throws InstanceLimitExceededException
* The maximum number of allowed on-premises instances in a single call was exceeded.
* @throws InstanceNotRegisteredException
* The specified on-premises instance is not registered.
* @sample AmazonCodeDeploy.RemoveTagsFromOnPremisesInstances
* @see AWS API Documentation
*/
RemoveTagsFromOnPremisesInstancesResult removeTagsFromOnPremisesInstances(RemoveTagsFromOnPremisesInstancesRequest removeTagsFromOnPremisesInstancesRequest);
/**
*
* In a blue/green deployment, overrides any specified wait time and starts terminating instances immediately after
* the traffic routing is complete.
*
*
* @param skipWaitTimeForInstanceTerminationRequest
* @return Result of the SkipWaitTimeForInstanceTermination operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentAlreadyCompletedException
* The deployment is already complete.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws DeploymentNotStartedException
* The specified deployment has not started.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @sample AmazonCodeDeploy.SkipWaitTimeForInstanceTermination
* @see AWS API Documentation
*/
@Deprecated
SkipWaitTimeForInstanceTerminationResult skipWaitTimeForInstanceTermination(
SkipWaitTimeForInstanceTerminationRequest skipWaitTimeForInstanceTerminationRequest);
/**
*
* Attempts to stop an ongoing deployment.
*
*
* @param stopDeploymentRequest
* Represents the input of a StopDeployment
operation.
* @return Result of the StopDeployment operation returned by the service.
* @throws DeploymentIdRequiredException
* At least one deployment ID must be specified.
* @throws DeploymentDoesNotExistException
* The deployment with the user or Amazon Web Services account does not exist.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentAlreadyCompletedException
* The deployment is already complete.
* @throws InvalidDeploymentIdException
* At least one of the deployment IDs was specified in an invalid format.
* @throws UnsupportedActionForDeploymentTypeException
* A call was submitted that is not supported for the specified deployment type.
* @sample AmazonCodeDeploy.StopDeployment
* @see AWS API
* Documentation
*/
StopDeploymentResult stopDeployment(StopDeploymentRequest stopDeploymentRequest);
/**
*
* Associates the list of tags in the input Tags
parameter with the resource identified by the
* ResourceArn
input parameter.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceArnRequiredException
* The ARN of a resource is required, but was not found.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws ArnNotSupportedException
* The specified ARN is not supported. For example, it might be an ARN for a resource that is not expected.
* @throws InvalidArnException
* The specified ARN is not in a valid format.
* @sample AmazonCodeDeploy.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Disassociates a resource from a list of tags. The resource is identified by the ResourceArn
input
* parameter. The tags are identified by the list of keys in the TagKeys
input parameter.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceArnRequiredException
* The ARN of a resource is required, but was not found.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws TagRequiredException
* A tag was not specified.
* @throws InvalidTagsToAddException
* The specified tags are not valid.
* @throws ArnNotSupportedException
* The specified ARN is not supported. For example, it might be an ARN for a resource that is not expected.
* @throws InvalidArnException
* The specified ARN is not in a valid format.
* @sample AmazonCodeDeploy.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Changes the name of an application.
*
*
* @param updateApplicationRequest
* Represents the input of an UpdateApplication
operation.
* @return Result of the UpdateApplication operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationAlreadyExistsException
* An application with the specified name with the user or Amazon Web Services account already exists.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @sample AmazonCodeDeploy.UpdateApplication
* @see AWS
* API Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
* Simplified method form for invoking the UpdateApplication operation.
*
* @see #updateApplication(UpdateApplicationRequest)
*/
UpdateApplicationResult updateApplication();
/**
*
* Changes information about a deployment group.
*
*
* @param updateDeploymentGroupRequest
* Represents the input of an UpdateDeploymentGroup
operation.
* @return Result of the UpdateDeploymentGroup operation returned by the service.
* @throws ApplicationNameRequiredException
* The minimum number of required application names was not specified.
* @throws InvalidApplicationNameException
* The application name was specified in an invalid format.
* @throws ApplicationDoesNotExistException
* The application does not exist with the user or Amazon Web Services account.
* @throws InvalidDeploymentGroupNameException
* The deployment group name was specified in an invalid format.
* @throws DeploymentGroupAlreadyExistsException
* A deployment group with the specified name with the user or Amazon Web Services account already exists.
* @throws DeploymentGroupNameRequiredException
* The deployment group name was not specified.
* @throws DeploymentGroupDoesNotExistException
* The named deployment group with the user or Amazon Web Services account does not exist.
* @throws InvalidEC2TagException
* The tag was specified in an invalid format.
* @throws InvalidTagException
* The tag was specified in an invalid format.
* @throws InvalidAutoScalingGroupException
* The Auto Scaling group was specified in an invalid format or does not exist.
* @throws InvalidDeploymentConfigNameException
* The deployment configuration name was specified in an invalid format.
* @throws DeploymentConfigDoesNotExistException
* The deployment configuration does not exist with the user or Amazon Web Services account.
* @throws InvalidRoleException
* The service role ARN was specified in an invalid format. Or, if an Auto Scaling group was specified, the
* specified service role does not grant the appropriate permissions to Amazon EC2 Auto Scaling.
* @throws LifecycleHookLimitExceededException
* The limit for lifecycle hooks was exceeded.
* @throws InvalidTriggerConfigException
* The trigger was specified in an invalid format.
* @throws TriggerTargetsLimitExceededException
* The maximum allowed number of triggers was exceeded.
* @throws InvalidAlarmConfigException
* The format of the alarm configuration is invalid. Possible causes include:
*
* -
*
* The alarm list is null.
*
*
* -
*
* The alarm object is null.
*
*
* -
*
* The alarm name is empty or null or exceeds the limit of 255 characters.
*
*
* -
*
* Two alarms with the same name have been specified.
*
*
* -
*
* The alarm configuration is enabled, but the alarm list is empty.
*
*
* @throws AlarmsLimitExceededException
* The maximum number of alarms for a deployment group (10) was exceeded.
* @throws InvalidAutoRollbackConfigException
* The automatic rollback configuration was specified in an invalid format. For example, automatic rollback
* is enabled, but an invalid triggering event type or no event types were listed.
* @throws InvalidLoadBalancerInfoException
* An invalid load balancer name, or no load balancer name, was specified.
* @throws InvalidDeploymentStyleException
* An invalid deployment style was specified. Valid deployment types include "IN_PLACE" and "BLUE_GREEN."
* Valid deployment options include "WITH_TRAFFIC_CONTROL" and "WITHOUT_TRAFFIC_CONTROL."
* @throws InvalidBlueGreenDeploymentConfigurationException
* The configuration for the blue/green deployment group was provided in an invalid format. For information
* about deployment configuration format, see CreateDeploymentConfig.
* @throws InvalidEC2TagCombinationException
* A call was submitted that specified both Ec2TagFilters and Ec2TagSet, but only one of these data types
* can be used in a single call.
* @throws InvalidOnPremisesTagCombinationException
* A call was submitted that specified both OnPremisesTagFilters and OnPremisesTagSet, but only one of these
* data types can be used in a single call.
* @throws TagSetListLimitExceededException
* The number of tag groups included in the tag set list exceeded the maximum allowed limit of 3.
* @throws InvalidInputException
* The input was specified in an invalid format.
* @throws ThrottlingException
* An API function was called too frequently.
* @throws InvalidECSServiceException
* The Amazon ECS service identifier is not valid.
* @throws InvalidTargetGroupPairException
* A target group pair associated with this deployment is not valid.
* @throws ECSServiceMappingLimitExceededException
* The Amazon ECS service is associated with more than one deployment groups. An Amazon ECS service can be
* associated with only one deployment group.
* @throws InvalidTrafficRoutingConfigurationException
* The configuration that specifies how traffic is routed during a deployment is invalid.
* @sample AmazonCodeDeploy.UpdateDeploymentGroup
* @see AWS API Documentation
*/
UpdateDeploymentGroupResult updateDeploymentGroup(UpdateDeploymentGroupRequest updateDeploymentGroupRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonCodeDeployWaiters waiters();
}