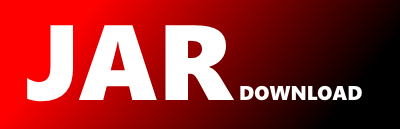
com.amazonaws.services.codedeploy.model.DeploymentConfigInfo Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.codedeploy.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a deployment configuration.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DeploymentConfigInfo implements Serializable, Cloneable, StructuredPojo {
/**
*
* The deployment configuration ID.
*
*/
private String deploymentConfigId;
/**
*
* The deployment configuration name.
*
*/
private String deploymentConfigName;
/**
*
* Information about the number or percentage of minimum healthy instances.
*
*/
private MinimumHealthyHosts minimumHealthyHosts;
/**
*
* The time at which the deployment configuration was created.
*
*/
private java.util.Date createTime;
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*/
private String computePlatform;
/**
*
* The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda or
* Amazon ECS compute platform only.
*
*/
private TrafficRoutingConfig trafficRoutingConfig;
/**
*
* Information about a zonal configuration.
*
*/
private ZonalConfig zonalConfig;
/**
*
* The deployment configuration ID.
*
*
* @param deploymentConfigId
* The deployment configuration ID.
*/
public void setDeploymentConfigId(String deploymentConfigId) {
this.deploymentConfigId = deploymentConfigId;
}
/**
*
* The deployment configuration ID.
*
*
* @return The deployment configuration ID.
*/
public String getDeploymentConfigId() {
return this.deploymentConfigId;
}
/**
*
* The deployment configuration ID.
*
*
* @param deploymentConfigId
* The deployment configuration ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentConfigInfo withDeploymentConfigId(String deploymentConfigId) {
setDeploymentConfigId(deploymentConfigId);
return this;
}
/**
*
* The deployment configuration name.
*
*
* @param deploymentConfigName
* The deployment configuration name.
*/
public void setDeploymentConfigName(String deploymentConfigName) {
this.deploymentConfigName = deploymentConfigName;
}
/**
*
* The deployment configuration name.
*
*
* @return The deployment configuration name.
*/
public String getDeploymentConfigName() {
return this.deploymentConfigName;
}
/**
*
* The deployment configuration name.
*
*
* @param deploymentConfigName
* The deployment configuration name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentConfigInfo withDeploymentConfigName(String deploymentConfigName) {
setDeploymentConfigName(deploymentConfigName);
return this;
}
/**
*
* Information about the number or percentage of minimum healthy instances.
*
*
* @param minimumHealthyHosts
* Information about the number or percentage of minimum healthy instances.
*/
public void setMinimumHealthyHosts(MinimumHealthyHosts minimumHealthyHosts) {
this.minimumHealthyHosts = minimumHealthyHosts;
}
/**
*
* Information about the number or percentage of minimum healthy instances.
*
*
* @return Information about the number or percentage of minimum healthy instances.
*/
public MinimumHealthyHosts getMinimumHealthyHosts() {
return this.minimumHealthyHosts;
}
/**
*
* Information about the number or percentage of minimum healthy instances.
*
*
* @param minimumHealthyHosts
* Information about the number or percentage of minimum healthy instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentConfigInfo withMinimumHealthyHosts(MinimumHealthyHosts minimumHealthyHosts) {
setMinimumHealthyHosts(minimumHealthyHosts);
return this;
}
/**
*
* The time at which the deployment configuration was created.
*
*
* @param createTime
* The time at which the deployment configuration was created.
*/
public void setCreateTime(java.util.Date createTime) {
this.createTime = createTime;
}
/**
*
* The time at which the deployment configuration was created.
*
*
* @return The time at which the deployment configuration was created.
*/
public java.util.Date getCreateTime() {
return this.createTime;
}
/**
*
* The time at which the deployment configuration was created.
*
*
* @param createTime
* The time at which the deployment configuration was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentConfigInfo withCreateTime(java.util.Date createTime) {
setCreateTime(createTime);
return this;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @see ComputePlatform
*/
public void setComputePlatform(String computePlatform) {
this.computePlatform = computePlatform;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @return The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @see ComputePlatform
*/
public String getComputePlatform() {
return this.computePlatform;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputePlatform
*/
public DeploymentConfigInfo withComputePlatform(String computePlatform) {
setComputePlatform(computePlatform);
return this;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputePlatform
*/
public DeploymentConfigInfo withComputePlatform(ComputePlatform computePlatform) {
this.computePlatform = computePlatform.toString();
return this;
}
/**
*
* The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda or
* Amazon ECS compute platform only.
*
*
* @param trafficRoutingConfig
* The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda
* or Amazon ECS compute platform only.
*/
public void setTrafficRoutingConfig(TrafficRoutingConfig trafficRoutingConfig) {
this.trafficRoutingConfig = trafficRoutingConfig;
}
/**
*
* The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda or
* Amazon ECS compute platform only.
*
*
* @return The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda
* or Amazon ECS compute platform only.
*/
public TrafficRoutingConfig getTrafficRoutingConfig() {
return this.trafficRoutingConfig;
}
/**
*
* The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda or
* Amazon ECS compute platform only.
*
*
* @param trafficRoutingConfig
* The configuration that specifies how the deployment traffic is routed. Used for deployments with a Lambda
* or Amazon ECS compute platform only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentConfigInfo withTrafficRoutingConfig(TrafficRoutingConfig trafficRoutingConfig) {
setTrafficRoutingConfig(trafficRoutingConfig);
return this;
}
/**
*
* Information about a zonal configuration.
*
*
* @param zonalConfig
* Information about a zonal configuration.
*/
public void setZonalConfig(ZonalConfig zonalConfig) {
this.zonalConfig = zonalConfig;
}
/**
*
* Information about a zonal configuration.
*
*
* @return Information about a zonal configuration.
*/
public ZonalConfig getZonalConfig() {
return this.zonalConfig;
}
/**
*
* Information about a zonal configuration.
*
*
* @param zonalConfig
* Information about a zonal configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentConfigInfo withZonalConfig(ZonalConfig zonalConfig) {
setZonalConfig(zonalConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDeploymentConfigId() != null)
sb.append("DeploymentConfigId: ").append(getDeploymentConfigId()).append(",");
if (getDeploymentConfigName() != null)
sb.append("DeploymentConfigName: ").append(getDeploymentConfigName()).append(",");
if (getMinimumHealthyHosts() != null)
sb.append("MinimumHealthyHosts: ").append(getMinimumHealthyHosts()).append(",");
if (getCreateTime() != null)
sb.append("CreateTime: ").append(getCreateTime()).append(",");
if (getComputePlatform() != null)
sb.append("ComputePlatform: ").append(getComputePlatform()).append(",");
if (getTrafficRoutingConfig() != null)
sb.append("TrafficRoutingConfig: ").append(getTrafficRoutingConfig()).append(",");
if (getZonalConfig() != null)
sb.append("ZonalConfig: ").append(getZonalConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DeploymentConfigInfo == false)
return false;
DeploymentConfigInfo other = (DeploymentConfigInfo) obj;
if (other.getDeploymentConfigId() == null ^ this.getDeploymentConfigId() == null)
return false;
if (other.getDeploymentConfigId() != null && other.getDeploymentConfigId().equals(this.getDeploymentConfigId()) == false)
return false;
if (other.getDeploymentConfigName() == null ^ this.getDeploymentConfigName() == null)
return false;
if (other.getDeploymentConfigName() != null && other.getDeploymentConfigName().equals(this.getDeploymentConfigName()) == false)
return false;
if (other.getMinimumHealthyHosts() == null ^ this.getMinimumHealthyHosts() == null)
return false;
if (other.getMinimumHealthyHosts() != null && other.getMinimumHealthyHosts().equals(this.getMinimumHealthyHosts()) == false)
return false;
if (other.getCreateTime() == null ^ this.getCreateTime() == null)
return false;
if (other.getCreateTime() != null && other.getCreateTime().equals(this.getCreateTime()) == false)
return false;
if (other.getComputePlatform() == null ^ this.getComputePlatform() == null)
return false;
if (other.getComputePlatform() != null && other.getComputePlatform().equals(this.getComputePlatform()) == false)
return false;
if (other.getTrafficRoutingConfig() == null ^ this.getTrafficRoutingConfig() == null)
return false;
if (other.getTrafficRoutingConfig() != null && other.getTrafficRoutingConfig().equals(this.getTrafficRoutingConfig()) == false)
return false;
if (other.getZonalConfig() == null ^ this.getZonalConfig() == null)
return false;
if (other.getZonalConfig() != null && other.getZonalConfig().equals(this.getZonalConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDeploymentConfigId() == null) ? 0 : getDeploymentConfigId().hashCode());
hashCode = prime * hashCode + ((getDeploymentConfigName() == null) ? 0 : getDeploymentConfigName().hashCode());
hashCode = prime * hashCode + ((getMinimumHealthyHosts() == null) ? 0 : getMinimumHealthyHosts().hashCode());
hashCode = prime * hashCode + ((getCreateTime() == null) ? 0 : getCreateTime().hashCode());
hashCode = prime * hashCode + ((getComputePlatform() == null) ? 0 : getComputePlatform().hashCode());
hashCode = prime * hashCode + ((getTrafficRoutingConfig() == null) ? 0 : getTrafficRoutingConfig().hashCode());
hashCode = prime * hashCode + ((getZonalConfig() == null) ? 0 : getZonalConfig().hashCode());
return hashCode;
}
@Override
public DeploymentConfigInfo clone() {
try {
return (DeploymentConfigInfo) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.codedeploy.model.transform.DeploymentConfigInfoMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}