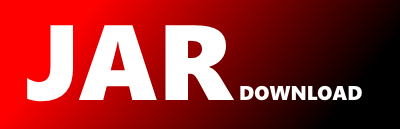
com.amazonaws.services.codedeploy.model.DeploymentInfo Maven / Gradle / Ivy
Show all versions of aws-java-sdk-codedeploy Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.codedeploy.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a deployment.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DeploymentInfo implements Serializable, Cloneable, StructuredPojo {
/**
*
* The application name.
*
*/
private String applicationName;
/**
*
* The deployment group name.
*
*/
private String deploymentGroupName;
/**
*
* The deployment configuration name.
*
*/
private String deploymentConfigName;
/**
*
* The unique ID of a deployment.
*
*/
private String deploymentId;
/**
*
* Information about the application revision that was deployed to the deployment group before the most recent
* successful deployment.
*
*/
private RevisionLocation previousRevision;
/**
*
* Information about the location of stored application artifacts and the service from which to retrieve them.
*
*/
private RevisionLocation revision;
/**
*
* The current state of the deployment as a whole.
*
*/
private String status;
/**
*
* Information about any error associated with this deployment.
*
*/
private ErrorInformation errorInformation;
/**
*
* A timestamp that indicates when the deployment was created.
*
*/
private java.util.Date createTime;
/**
*
* A timestamp that indicates when the deployment was deployed to the deployment group.
*
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
*
*/
private java.util.Date startTime;
/**
*
* A timestamp that indicates when the deployment was complete.
*
*/
private java.util.Date completeTime;
/**
*
* A summary of the deployment status of the instances in the deployment.
*
*/
private DeploymentOverview deploymentOverview;
/**
*
* A comment about the deployment.
*
*/
private String description;
/**
*
* The means by which the deployment was created:
*
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated Amazon
* EC2 instances.
*
*
*
*/
private String creator;
/**
*
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment continues to
* the next deployment lifecycle event. For example, if ApplicationStop
fails, the deployment continues
* with DownloadBundle. If BeforeBlockTraffic
fails, the deployment continues with
* BlockTraffic
. If AfterBlockTraffic
fails, the deployment continues with
* ApplicationStop
.
*
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that deployment
* fails. If deployment to that instance is part of an overall deployment and the number of healthy hosts is not
* less than the minimum number of healthy hosts, then a deployment to the next instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one of
* these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run successfully,
* create a new deployment and use ignoreApplicationStopFailures
to specify that the
* ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
failures should
* be ignored.
*
*/
private Boolean ignoreApplicationStopFailures;
/**
*
* Information about the automatic rollback configuration associated with the deployment.
*
*/
private AutoRollbackConfiguration autoRollbackConfiguration;
/**
*
* Indicates whether only instances that are not running the latest application revision are to be deployed to.
*
*/
private Boolean updateOutdatedInstancesOnly;
/**
*
* Information about a deployment rollback.
*
*/
private RollbackInfo rollbackInfo;
/**
*
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to route
* deployment traffic behind a load balancer.
*
*/
private DeploymentStyle deploymentStyle;
/**
*
* Information about the instances that belong to the replacement environment in a blue/green deployment.
*
*/
private TargetInstances targetInstances;
/**
*
* Indicates whether the wait period set for the termination of instances in the original environment has started.
* Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the termination wait period
* starts.
*
*/
private Boolean instanceTerminationWaitTimeStarted;
/**
*
* Information about blue/green deployment options for this deployment.
*
*/
private BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration;
/**
*
* Information about the load balancer used in the deployment.
*
*/
private LoadBalancerInfo loadBalancerInfo;
/**
*
* Provides information about the results of a deployment, such as whether instances in the original environment in
* a blue/green deployment were not terminated.
*
*/
private String additionalDeploymentStatusInfo;
/**
*
* Information about how CodeDeploy handles files that already exist in a deployment target location but weren't
* part of the previous successful deployment.
*
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed replaces
* the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
*
*/
private String fileExistsBehavior;
/**
*
* Messages that contain information about the status of a deployment.
*
*/
private com.amazonaws.internal.SdkInternalList deploymentStatusMessages;
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*/
private String computePlatform;
/**
*
* The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
*
*/
private String externalId;
private RelatedDeployments relatedDeployments;
private AlarmConfiguration overrideAlarmConfiguration;
/**
*
* The application name.
*
*
* @param applicationName
* The application name.
*/
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
/**
*
* The application name.
*
*
* @return The application name.
*/
public String getApplicationName() {
return this.applicationName;
}
/**
*
* The application name.
*
*
* @param applicationName
* The application name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withApplicationName(String applicationName) {
setApplicationName(applicationName);
return this;
}
/**
*
* The deployment group name.
*
*
* @param deploymentGroupName
* The deployment group name.
*/
public void setDeploymentGroupName(String deploymentGroupName) {
this.deploymentGroupName = deploymentGroupName;
}
/**
*
* The deployment group name.
*
*
* @return The deployment group name.
*/
public String getDeploymentGroupName() {
return this.deploymentGroupName;
}
/**
*
* The deployment group name.
*
*
* @param deploymentGroupName
* The deployment group name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentGroupName(String deploymentGroupName) {
setDeploymentGroupName(deploymentGroupName);
return this;
}
/**
*
* The deployment configuration name.
*
*
* @param deploymentConfigName
* The deployment configuration name.
*/
public void setDeploymentConfigName(String deploymentConfigName) {
this.deploymentConfigName = deploymentConfigName;
}
/**
*
* The deployment configuration name.
*
*
* @return The deployment configuration name.
*/
public String getDeploymentConfigName() {
return this.deploymentConfigName;
}
/**
*
* The deployment configuration name.
*
*
* @param deploymentConfigName
* The deployment configuration name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentConfigName(String deploymentConfigName) {
setDeploymentConfigName(deploymentConfigName);
return this;
}
/**
*
* The unique ID of a deployment.
*
*
* @param deploymentId
* The unique ID of a deployment.
*/
public void setDeploymentId(String deploymentId) {
this.deploymentId = deploymentId;
}
/**
*
* The unique ID of a deployment.
*
*
* @return The unique ID of a deployment.
*/
public String getDeploymentId() {
return this.deploymentId;
}
/**
*
* The unique ID of a deployment.
*
*
* @param deploymentId
* The unique ID of a deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentId(String deploymentId) {
setDeploymentId(deploymentId);
return this;
}
/**
*
* Information about the application revision that was deployed to the deployment group before the most recent
* successful deployment.
*
*
* @param previousRevision
* Information about the application revision that was deployed to the deployment group before the most
* recent successful deployment.
*/
public void setPreviousRevision(RevisionLocation previousRevision) {
this.previousRevision = previousRevision;
}
/**
*
* Information about the application revision that was deployed to the deployment group before the most recent
* successful deployment.
*
*
* @return Information about the application revision that was deployed to the deployment group before the most
* recent successful deployment.
*/
public RevisionLocation getPreviousRevision() {
return this.previousRevision;
}
/**
*
* Information about the application revision that was deployed to the deployment group before the most recent
* successful deployment.
*
*
* @param previousRevision
* Information about the application revision that was deployed to the deployment group before the most
* recent successful deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withPreviousRevision(RevisionLocation previousRevision) {
setPreviousRevision(previousRevision);
return this;
}
/**
*
* Information about the location of stored application artifacts and the service from which to retrieve them.
*
*
* @param revision
* Information about the location of stored application artifacts and the service from which to retrieve
* them.
*/
public void setRevision(RevisionLocation revision) {
this.revision = revision;
}
/**
*
* Information about the location of stored application artifacts and the service from which to retrieve them.
*
*
* @return Information about the location of stored application artifacts and the service from which to retrieve
* them.
*/
public RevisionLocation getRevision() {
return this.revision;
}
/**
*
* Information about the location of stored application artifacts and the service from which to retrieve them.
*
*
* @param revision
* Information about the location of stored application artifacts and the service from which to retrieve
* them.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withRevision(RevisionLocation revision) {
setRevision(revision);
return this;
}
/**
*
* The current state of the deployment as a whole.
*
*
* @param status
* The current state of the deployment as a whole.
* @see DeploymentStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current state of the deployment as a whole.
*
*
* @return The current state of the deployment as a whole.
* @see DeploymentStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current state of the deployment as a whole.
*
*
* @param status
* The current state of the deployment as a whole.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeploymentStatus
*/
public DeploymentInfo withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current state of the deployment as a whole.
*
*
* @param status
* The current state of the deployment as a whole.
* @see DeploymentStatus
*/
public void setStatus(DeploymentStatus status) {
withStatus(status);
}
/**
*
* The current state of the deployment as a whole.
*
*
* @param status
* The current state of the deployment as a whole.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeploymentStatus
*/
public DeploymentInfo withStatus(DeploymentStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Information about any error associated with this deployment.
*
*
* @param errorInformation
* Information about any error associated with this deployment.
*/
public void setErrorInformation(ErrorInformation errorInformation) {
this.errorInformation = errorInformation;
}
/**
*
* Information about any error associated with this deployment.
*
*
* @return Information about any error associated with this deployment.
*/
public ErrorInformation getErrorInformation() {
return this.errorInformation;
}
/**
*
* Information about any error associated with this deployment.
*
*
* @param errorInformation
* Information about any error associated with this deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withErrorInformation(ErrorInformation errorInformation) {
setErrorInformation(errorInformation);
return this;
}
/**
*
* A timestamp that indicates when the deployment was created.
*
*
* @param createTime
* A timestamp that indicates when the deployment was created.
*/
public void setCreateTime(java.util.Date createTime) {
this.createTime = createTime;
}
/**
*
* A timestamp that indicates when the deployment was created.
*
*
* @return A timestamp that indicates when the deployment was created.
*/
public java.util.Date getCreateTime() {
return this.createTime;
}
/**
*
* A timestamp that indicates when the deployment was created.
*
*
* @param createTime
* A timestamp that indicates when the deployment was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withCreateTime(java.util.Date createTime) {
setCreateTime(createTime);
return this;
}
/**
*
* A timestamp that indicates when the deployment was deployed to the deployment group.
*
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
*
*
* @param startTime
* A timestamp that indicates when the deployment was deployed to the deployment group.
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* A timestamp that indicates when the deployment was deployed to the deployment group.
*
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
*
*
* @return A timestamp that indicates when the deployment was deployed to the deployment group.
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* A timestamp that indicates when the deployment was deployed to the deployment group.
*
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
*
*
* @param startTime
* A timestamp that indicates when the deployment was deployed to the deployment group.
*
* In some cases, the reported value of the start time might be later than the complete time. This is due to
* differences in the clock settings of backend servers that participate in the deployment process.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* A timestamp that indicates when the deployment was complete.
*
*
* @param completeTime
* A timestamp that indicates when the deployment was complete.
*/
public void setCompleteTime(java.util.Date completeTime) {
this.completeTime = completeTime;
}
/**
*
* A timestamp that indicates when the deployment was complete.
*
*
* @return A timestamp that indicates when the deployment was complete.
*/
public java.util.Date getCompleteTime() {
return this.completeTime;
}
/**
*
* A timestamp that indicates when the deployment was complete.
*
*
* @param completeTime
* A timestamp that indicates when the deployment was complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withCompleteTime(java.util.Date completeTime) {
setCompleteTime(completeTime);
return this;
}
/**
*
* A summary of the deployment status of the instances in the deployment.
*
*
* @param deploymentOverview
* A summary of the deployment status of the instances in the deployment.
*/
public void setDeploymentOverview(DeploymentOverview deploymentOverview) {
this.deploymentOverview = deploymentOverview;
}
/**
*
* A summary of the deployment status of the instances in the deployment.
*
*
* @return A summary of the deployment status of the instances in the deployment.
*/
public DeploymentOverview getDeploymentOverview() {
return this.deploymentOverview;
}
/**
*
* A summary of the deployment status of the instances in the deployment.
*
*
* @param deploymentOverview
* A summary of the deployment status of the instances in the deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentOverview(DeploymentOverview deploymentOverview) {
setDeploymentOverview(deploymentOverview);
return this;
}
/**
*
* A comment about the deployment.
*
*
* @param description
* A comment about the deployment.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A comment about the deployment.
*
*
* @return A comment about the deployment.
*/
public String getDescription() {
return this.description;
}
/**
*
* A comment about the deployment.
*
*
* @param description
* A comment about the deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The means by which the deployment was created:
*
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated Amazon
* EC2 instances.
*
*
*
*
* @param creator
* The means by which the deployment was created:
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated
* Amazon EC2 instances.
*
*
* @see DeploymentCreator
*/
public void setCreator(String creator) {
this.creator = creator;
}
/**
*
* The means by which the deployment was created:
*
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated Amazon
* EC2 instances.
*
*
*
*
* @return The means by which the deployment was created:
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected
* outdated Amazon EC2 instances.
*
*
* @see DeploymentCreator
*/
public String getCreator() {
return this.creator;
}
/**
*
* The means by which the deployment was created:
*
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated Amazon
* EC2 instances.
*
*
*
*
* @param creator
* The means by which the deployment was created:
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated
* Amazon EC2 instances.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeploymentCreator
*/
public DeploymentInfo withCreator(String creator) {
setCreator(creator);
return this;
}
/**
*
* The means by which the deployment was created:
*
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated Amazon
* EC2 instances.
*
*
*
*
* @param creator
* The means by which the deployment was created:
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated
* Amazon EC2 instances.
*
*
* @see DeploymentCreator
*/
public void setCreator(DeploymentCreator creator) {
withCreator(creator);
}
/**
*
* The means by which the deployment was created:
*
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated Amazon
* EC2 instances.
*
*
*
*
* @param creator
* The means by which the deployment was created:
*
* -
*
* user
: A user created the deployment.
*
*
* -
*
* autoscaling
: Amazon EC2 Auto Scaling created the deployment.
*
*
* -
*
* codeDeployRollback
: A rollback process created the deployment.
*
*
* -
*
* CodeDeployAutoUpdate
: An auto-update process created the deployment when it detected outdated
* Amazon EC2 instances.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeploymentCreator
*/
public DeploymentInfo withCreator(DeploymentCreator creator) {
this.creator = creator.toString();
return this;
}
/**
*
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment continues to
* the next deployment lifecycle event. For example, if ApplicationStop
fails, the deployment continues
* with DownloadBundle. If BeforeBlockTraffic
fails, the deployment continues with
* BlockTraffic
. If AfterBlockTraffic
fails, the deployment continues with
* ApplicationStop
.
*
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that deployment
* fails. If deployment to that instance is part of an overall deployment and the number of healthy hosts is not
* less than the minimum number of healthy hosts, then a deployment to the next instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one of
* these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run successfully,
* create a new deployment and use ignoreApplicationStopFailures
to specify that the
* ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
failures should
* be ignored.
*
*
* @param ignoreApplicationStopFailures
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment
* continues to the next deployment lifecycle event. For example, if ApplicationStop
fails, the
* deployment continues with DownloadBundle. If BeforeBlockTraffic
fails, the deployment
* continues with BlockTraffic
. If AfterBlockTraffic
fails, the deployment
* continues with ApplicationStop
.
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that
* deployment fails. If deployment to that instance is part of an overall deployment and the number of
* healthy hosts is not less than the minimum number of healthy hosts, then a deployment to the next instance
* is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one
* of these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run
* successfully, create a new deployment and use ignoreApplicationStopFailures
to specify that
* the ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
* failures should be ignored.
*/
public void setIgnoreApplicationStopFailures(Boolean ignoreApplicationStopFailures) {
this.ignoreApplicationStopFailures = ignoreApplicationStopFailures;
}
/**
*
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment continues to
* the next deployment lifecycle event. For example, if ApplicationStop
fails, the deployment continues
* with DownloadBundle. If BeforeBlockTraffic
fails, the deployment continues with
* BlockTraffic
. If AfterBlockTraffic
fails, the deployment continues with
* ApplicationStop
.
*
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that deployment
* fails. If deployment to that instance is part of an overall deployment and the number of healthy hosts is not
* less than the minimum number of healthy hosts, then a deployment to the next instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one of
* these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run successfully,
* create a new deployment and use ignoreApplicationStopFailures
to specify that the
* ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
failures should
* be ignored.
*
*
* @return If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment
* continues to the next deployment lifecycle event. For example, if ApplicationStop
fails, the
* deployment continues with DownloadBundle. If BeforeBlockTraffic
fails, the deployment
* continues with BlockTraffic
. If AfterBlockTraffic
fails, the deployment
* continues with ApplicationStop
.
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that
* deployment fails. If deployment to that instance is part of an overall deployment and the number of
* healthy hosts is not less than the minimum number of healthy hosts, then a deployment to the next
* instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If
* one of these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run
* successfully, create a new deployment and use ignoreApplicationStopFailures
to specify that
* the ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
* failures should be ignored.
*/
public Boolean getIgnoreApplicationStopFailures() {
return this.ignoreApplicationStopFailures;
}
/**
*
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment continues to
* the next deployment lifecycle event. For example, if ApplicationStop
fails, the deployment continues
* with DownloadBundle. If BeforeBlockTraffic
fails, the deployment continues with
* BlockTraffic
. If AfterBlockTraffic
fails, the deployment continues with
* ApplicationStop
.
*
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that deployment
* fails. If deployment to that instance is part of an overall deployment and the number of healthy hosts is not
* less than the minimum number of healthy hosts, then a deployment to the next instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one of
* these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run successfully,
* create a new deployment and use ignoreApplicationStopFailures
to specify that the
* ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
failures should
* be ignored.
*
*
* @param ignoreApplicationStopFailures
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment
* continues to the next deployment lifecycle event. For example, if ApplicationStop
fails, the
* deployment continues with DownloadBundle. If BeforeBlockTraffic
fails, the deployment
* continues with BlockTraffic
. If AfterBlockTraffic
fails, the deployment
* continues with ApplicationStop
.
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that
* deployment fails. If deployment to that instance is part of an overall deployment and the number of
* healthy hosts is not less than the minimum number of healthy hosts, then a deployment to the next instance
* is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one
* of these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run
* successfully, create a new deployment and use ignoreApplicationStopFailures
to specify that
* the ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
* failures should be ignored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withIgnoreApplicationStopFailures(Boolean ignoreApplicationStopFailures) {
setIgnoreApplicationStopFailures(ignoreApplicationStopFailures);
return this;
}
/**
*
* If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment continues to
* the next deployment lifecycle event. For example, if ApplicationStop
fails, the deployment continues
* with DownloadBundle. If BeforeBlockTraffic
fails, the deployment continues with
* BlockTraffic
. If AfterBlockTraffic
fails, the deployment continues with
* ApplicationStop
.
*
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that deployment
* fails. If deployment to that instance is part of an overall deployment and the number of healthy hosts is not
* less than the minimum number of healthy hosts, then a deployment to the next instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If one of
* these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run successfully,
* create a new deployment and use ignoreApplicationStopFailures
to specify that the
* ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
failures should
* be ignored.
*
*
* @return If true, then if an ApplicationStop
, BeforeBlockTraffic
, or
* AfterBlockTraffic
deployment lifecycle event to an instance fails, then the deployment
* continues to the next deployment lifecycle event. For example, if ApplicationStop
fails, the
* deployment continues with DownloadBundle. If BeforeBlockTraffic
fails, the deployment
* continues with BlockTraffic
. If AfterBlockTraffic
fails, the deployment
* continues with ApplicationStop
.
*
* If false or not specified, then if a lifecycle event fails during a deployment to an instance, that
* deployment fails. If deployment to that instance is part of an overall deployment and the number of
* healthy hosts is not less than the minimum number of healthy hosts, then a deployment to the next
* instance is attempted.
*
*
* During a deployment, the CodeDeploy agent runs the scripts specified for ApplicationStop
,
* BeforeBlockTraffic
, and AfterBlockTraffic
in the AppSpec file from the previous
* successful deployment. (All other scripts are run from the AppSpec file in the current deployment.) If
* one of these scripts contains an error and does not run successfully, the deployment can fail.
*
*
* If the cause of the failure is a script from the last successful deployment that will never run
* successfully, create a new deployment and use ignoreApplicationStopFailures
to specify that
* the ApplicationStop
, BeforeBlockTraffic
, and AfterBlockTraffic
* failures should be ignored.
*/
public Boolean isIgnoreApplicationStopFailures() {
return this.ignoreApplicationStopFailures;
}
/**
*
* Information about the automatic rollback configuration associated with the deployment.
*
*
* @param autoRollbackConfiguration
* Information about the automatic rollback configuration associated with the deployment.
*/
public void setAutoRollbackConfiguration(AutoRollbackConfiguration autoRollbackConfiguration) {
this.autoRollbackConfiguration = autoRollbackConfiguration;
}
/**
*
* Information about the automatic rollback configuration associated with the deployment.
*
*
* @return Information about the automatic rollback configuration associated with the deployment.
*/
public AutoRollbackConfiguration getAutoRollbackConfiguration() {
return this.autoRollbackConfiguration;
}
/**
*
* Information about the automatic rollback configuration associated with the deployment.
*
*
* @param autoRollbackConfiguration
* Information about the automatic rollback configuration associated with the deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withAutoRollbackConfiguration(AutoRollbackConfiguration autoRollbackConfiguration) {
setAutoRollbackConfiguration(autoRollbackConfiguration);
return this;
}
/**
*
* Indicates whether only instances that are not running the latest application revision are to be deployed to.
*
*
* @param updateOutdatedInstancesOnly
* Indicates whether only instances that are not running the latest application revision are to be deployed
* to.
*/
public void setUpdateOutdatedInstancesOnly(Boolean updateOutdatedInstancesOnly) {
this.updateOutdatedInstancesOnly = updateOutdatedInstancesOnly;
}
/**
*
* Indicates whether only instances that are not running the latest application revision are to be deployed to.
*
*
* @return Indicates whether only instances that are not running the latest application revision are to be deployed
* to.
*/
public Boolean getUpdateOutdatedInstancesOnly() {
return this.updateOutdatedInstancesOnly;
}
/**
*
* Indicates whether only instances that are not running the latest application revision are to be deployed to.
*
*
* @param updateOutdatedInstancesOnly
* Indicates whether only instances that are not running the latest application revision are to be deployed
* to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withUpdateOutdatedInstancesOnly(Boolean updateOutdatedInstancesOnly) {
setUpdateOutdatedInstancesOnly(updateOutdatedInstancesOnly);
return this;
}
/**
*
* Indicates whether only instances that are not running the latest application revision are to be deployed to.
*
*
* @return Indicates whether only instances that are not running the latest application revision are to be deployed
* to.
*/
public Boolean isUpdateOutdatedInstancesOnly() {
return this.updateOutdatedInstancesOnly;
}
/**
*
* Information about a deployment rollback.
*
*
* @param rollbackInfo
* Information about a deployment rollback.
*/
public void setRollbackInfo(RollbackInfo rollbackInfo) {
this.rollbackInfo = rollbackInfo;
}
/**
*
* Information about a deployment rollback.
*
*
* @return Information about a deployment rollback.
*/
public RollbackInfo getRollbackInfo() {
return this.rollbackInfo;
}
/**
*
* Information about a deployment rollback.
*
*
* @param rollbackInfo
* Information about a deployment rollback.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withRollbackInfo(RollbackInfo rollbackInfo) {
setRollbackInfo(rollbackInfo);
return this;
}
/**
*
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @param deploymentStyle
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to
* route deployment traffic behind a load balancer.
*/
public void setDeploymentStyle(DeploymentStyle deploymentStyle) {
this.deploymentStyle = deploymentStyle;
}
/**
*
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @return Information about the type of deployment, either in-place or blue/green, you want to run and whether to
* route deployment traffic behind a load balancer.
*/
public DeploymentStyle getDeploymentStyle() {
return this.deploymentStyle;
}
/**
*
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to route
* deployment traffic behind a load balancer.
*
*
* @param deploymentStyle
* Information about the type of deployment, either in-place or blue/green, you want to run and whether to
* route deployment traffic behind a load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentStyle(DeploymentStyle deploymentStyle) {
setDeploymentStyle(deploymentStyle);
return this;
}
/**
*
* Information about the instances that belong to the replacement environment in a blue/green deployment.
*
*
* @param targetInstances
* Information about the instances that belong to the replacement environment in a blue/green deployment.
*/
public void setTargetInstances(TargetInstances targetInstances) {
this.targetInstances = targetInstances;
}
/**
*
* Information about the instances that belong to the replacement environment in a blue/green deployment.
*
*
* @return Information about the instances that belong to the replacement environment in a blue/green deployment.
*/
public TargetInstances getTargetInstances() {
return this.targetInstances;
}
/**
*
* Information about the instances that belong to the replacement environment in a blue/green deployment.
*
*
* @param targetInstances
* Information about the instances that belong to the replacement environment in a blue/green deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withTargetInstances(TargetInstances targetInstances) {
setTargetInstances(targetInstances);
return this;
}
/**
*
* Indicates whether the wait period set for the termination of instances in the original environment has started.
* Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the termination wait period
* starts.
*
*
* @param instanceTerminationWaitTimeStarted
* Indicates whether the wait period set for the termination of instances in the original environment has
* started. Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the
* termination wait period starts.
*/
public void setInstanceTerminationWaitTimeStarted(Boolean instanceTerminationWaitTimeStarted) {
this.instanceTerminationWaitTimeStarted = instanceTerminationWaitTimeStarted;
}
/**
*
* Indicates whether the wait period set for the termination of instances in the original environment has started.
* Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the termination wait period
* starts.
*
*
* @return Indicates whether the wait period set for the termination of instances in the original environment has
* started. Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the
* termination wait period starts.
*/
public Boolean getInstanceTerminationWaitTimeStarted() {
return this.instanceTerminationWaitTimeStarted;
}
/**
*
* Indicates whether the wait period set for the termination of instances in the original environment has started.
* Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the termination wait period
* starts.
*
*
* @param instanceTerminationWaitTimeStarted
* Indicates whether the wait period set for the termination of instances in the original environment has
* started. Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the
* termination wait period starts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withInstanceTerminationWaitTimeStarted(Boolean instanceTerminationWaitTimeStarted) {
setInstanceTerminationWaitTimeStarted(instanceTerminationWaitTimeStarted);
return this;
}
/**
*
* Indicates whether the wait period set for the termination of instances in the original environment has started.
* Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the termination wait period
* starts.
*
*
* @return Indicates whether the wait period set for the termination of instances in the original environment has
* started. Status is 'false' if the KEEP_ALIVE option is specified. Otherwise, 'true' as soon as the
* termination wait period starts.
*/
public Boolean isInstanceTerminationWaitTimeStarted() {
return this.instanceTerminationWaitTimeStarted;
}
/**
*
* Information about blue/green deployment options for this deployment.
*
*
* @param blueGreenDeploymentConfiguration
* Information about blue/green deployment options for this deployment.
*/
public void setBlueGreenDeploymentConfiguration(BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration) {
this.blueGreenDeploymentConfiguration = blueGreenDeploymentConfiguration;
}
/**
*
* Information about blue/green deployment options for this deployment.
*
*
* @return Information about blue/green deployment options for this deployment.
*/
public BlueGreenDeploymentConfiguration getBlueGreenDeploymentConfiguration() {
return this.blueGreenDeploymentConfiguration;
}
/**
*
* Information about blue/green deployment options for this deployment.
*
*
* @param blueGreenDeploymentConfiguration
* Information about blue/green deployment options for this deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withBlueGreenDeploymentConfiguration(BlueGreenDeploymentConfiguration blueGreenDeploymentConfiguration) {
setBlueGreenDeploymentConfiguration(blueGreenDeploymentConfiguration);
return this;
}
/**
*
* Information about the load balancer used in the deployment.
*
*
* @param loadBalancerInfo
* Information about the load balancer used in the deployment.
*/
public void setLoadBalancerInfo(LoadBalancerInfo loadBalancerInfo) {
this.loadBalancerInfo = loadBalancerInfo;
}
/**
*
* Information about the load balancer used in the deployment.
*
*
* @return Information about the load balancer used in the deployment.
*/
public LoadBalancerInfo getLoadBalancerInfo() {
return this.loadBalancerInfo;
}
/**
*
* Information about the load balancer used in the deployment.
*
*
* @param loadBalancerInfo
* Information about the load balancer used in the deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withLoadBalancerInfo(LoadBalancerInfo loadBalancerInfo) {
setLoadBalancerInfo(loadBalancerInfo);
return this;
}
/**
*
* Provides information about the results of a deployment, such as whether instances in the original environment in
* a blue/green deployment were not terminated.
*
*
* @param additionalDeploymentStatusInfo
* Provides information about the results of a deployment, such as whether instances in the original
* environment in a blue/green deployment were not terminated.
*/
public void setAdditionalDeploymentStatusInfo(String additionalDeploymentStatusInfo) {
this.additionalDeploymentStatusInfo = additionalDeploymentStatusInfo;
}
/**
*
* Provides information about the results of a deployment, such as whether instances in the original environment in
* a blue/green deployment were not terminated.
*
*
* @return Provides information about the results of a deployment, such as whether instances in the original
* environment in a blue/green deployment were not terminated.
*/
public String getAdditionalDeploymentStatusInfo() {
return this.additionalDeploymentStatusInfo;
}
/**
*
* Provides information about the results of a deployment, such as whether instances in the original environment in
* a blue/green deployment were not terminated.
*
*
* @param additionalDeploymentStatusInfo
* Provides information about the results of a deployment, such as whether instances in the original
* environment in a blue/green deployment were not terminated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withAdditionalDeploymentStatusInfo(String additionalDeploymentStatusInfo) {
setAdditionalDeploymentStatusInfo(additionalDeploymentStatusInfo);
return this;
}
/**
*
* Information about how CodeDeploy handles files that already exist in a deployment target location but weren't
* part of the previous successful deployment.
*
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed replaces
* the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
*
*
* @param fileExistsBehavior
* Information about how CodeDeploy handles files that already exist in a deployment target location but
* weren't part of the previous successful deployment.
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed
* replaces the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
* @see FileExistsBehavior
*/
public void setFileExistsBehavior(String fileExistsBehavior) {
this.fileExistsBehavior = fileExistsBehavior;
}
/**
*
* Information about how CodeDeploy handles files that already exist in a deployment target location but weren't
* part of the previous successful deployment.
*
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed replaces
* the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
*
*
* @return Information about how CodeDeploy handles files that already exist in a deployment target location but
* weren't part of the previous successful deployment.
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed
* replaces the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
* @see FileExistsBehavior
*/
public String getFileExistsBehavior() {
return this.fileExistsBehavior;
}
/**
*
* Information about how CodeDeploy handles files that already exist in a deployment target location but weren't
* part of the previous successful deployment.
*
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed replaces
* the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
*
*
* @param fileExistsBehavior
* Information about how CodeDeploy handles files that already exist in a deployment target location but
* weren't part of the previous successful deployment.
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed
* replaces the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FileExistsBehavior
*/
public DeploymentInfo withFileExistsBehavior(String fileExistsBehavior) {
setFileExistsBehavior(fileExistsBehavior);
return this;
}
/**
*
* Information about how CodeDeploy handles files that already exist in a deployment target location but weren't
* part of the previous successful deployment.
*
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed replaces
* the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
*
*
* @param fileExistsBehavior
* Information about how CodeDeploy handles files that already exist in a deployment target location but
* weren't part of the previous successful deployment.
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed
* replaces the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
* @see FileExistsBehavior
*/
public void setFileExistsBehavior(FileExistsBehavior fileExistsBehavior) {
withFileExistsBehavior(fileExistsBehavior);
}
/**
*
* Information about how CodeDeploy handles files that already exist in a deployment target location but weren't
* part of the previous successful deployment.
*
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed replaces
* the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
*
*
* @param fileExistsBehavior
* Information about how CodeDeploy handles files that already exist in a deployment target location but
* weren't part of the previous successful deployment.
*
* -
*
* DISALLOW
: The deployment fails. This is also the default behavior if no option is specified.
*
*
* -
*
* OVERWRITE
: The version of the file from the application revision currently being deployed
* replaces the version already on the instance.
*
*
* -
*
* RETAIN
: The version of the file already on the instance is kept and used as part of the new
* deployment.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FileExistsBehavior
*/
public DeploymentInfo withFileExistsBehavior(FileExistsBehavior fileExistsBehavior) {
this.fileExistsBehavior = fileExistsBehavior.toString();
return this;
}
/**
*
* Messages that contain information about the status of a deployment.
*
*
* @return Messages that contain information about the status of a deployment.
*/
public java.util.List getDeploymentStatusMessages() {
if (deploymentStatusMessages == null) {
deploymentStatusMessages = new com.amazonaws.internal.SdkInternalList();
}
return deploymentStatusMessages;
}
/**
*
* Messages that contain information about the status of a deployment.
*
*
* @param deploymentStatusMessages
* Messages that contain information about the status of a deployment.
*/
public void setDeploymentStatusMessages(java.util.Collection deploymentStatusMessages) {
if (deploymentStatusMessages == null) {
this.deploymentStatusMessages = null;
return;
}
this.deploymentStatusMessages = new com.amazonaws.internal.SdkInternalList(deploymentStatusMessages);
}
/**
*
* Messages that contain information about the status of a deployment.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDeploymentStatusMessages(java.util.Collection)} or
* {@link #withDeploymentStatusMessages(java.util.Collection)} if you want to override the existing values.
*
*
* @param deploymentStatusMessages
* Messages that contain information about the status of a deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentStatusMessages(String... deploymentStatusMessages) {
if (this.deploymentStatusMessages == null) {
setDeploymentStatusMessages(new com.amazonaws.internal.SdkInternalList(deploymentStatusMessages.length));
}
for (String ele : deploymentStatusMessages) {
this.deploymentStatusMessages.add(ele);
}
return this;
}
/**
*
* Messages that contain information about the status of a deployment.
*
*
* @param deploymentStatusMessages
* Messages that contain information about the status of a deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withDeploymentStatusMessages(java.util.Collection deploymentStatusMessages) {
setDeploymentStatusMessages(deploymentStatusMessages);
return this;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @see ComputePlatform
*/
public void setComputePlatform(String computePlatform) {
this.computePlatform = computePlatform;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @return The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @see ComputePlatform
*/
public String getComputePlatform() {
return this.computePlatform;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputePlatform
*/
public DeploymentInfo withComputePlatform(String computePlatform) {
setComputePlatform(computePlatform);
return this;
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @see ComputePlatform
*/
public void setComputePlatform(ComputePlatform computePlatform) {
withComputePlatform(computePlatform);
}
/**
*
* The destination platform type for the deployment (Lambda
, Server
, or ECS
).
*
*
* @param computePlatform
* The destination platform type for the deployment (Lambda
, Server
, or
* ECS
).
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputePlatform
*/
public DeploymentInfo withComputePlatform(ComputePlatform computePlatform) {
this.computePlatform = computePlatform.toString();
return this;
}
/**
*
* The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
*
*
* @param externalId
* The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
*/
public void setExternalId(String externalId) {
this.externalId = externalId;
}
/**
*
* The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
*
*
* @return The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
*/
public String getExternalId() {
return this.externalId;
}
/**
*
* The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
*
*
* @param externalId
* The unique ID for an external resource (for example, a CloudFormation stack ID) that is linked to this
* deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withExternalId(String externalId) {
setExternalId(externalId);
return this;
}
/**
* @param relatedDeployments
*/
public void setRelatedDeployments(RelatedDeployments relatedDeployments) {
this.relatedDeployments = relatedDeployments;
}
/**
* @return
*/
public RelatedDeployments getRelatedDeployments() {
return this.relatedDeployments;
}
/**
* @param relatedDeployments
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withRelatedDeployments(RelatedDeployments relatedDeployments) {
setRelatedDeployments(relatedDeployments);
return this;
}
/**
* @param overrideAlarmConfiguration
*/
public void setOverrideAlarmConfiguration(AlarmConfiguration overrideAlarmConfiguration) {
this.overrideAlarmConfiguration = overrideAlarmConfiguration;
}
/**
* @return
*/
public AlarmConfiguration getOverrideAlarmConfiguration() {
return this.overrideAlarmConfiguration;
}
/**
* @param overrideAlarmConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentInfo withOverrideAlarmConfiguration(AlarmConfiguration overrideAlarmConfiguration) {
setOverrideAlarmConfiguration(overrideAlarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationName() != null)
sb.append("ApplicationName: ").append(getApplicationName()).append(",");
if (getDeploymentGroupName() != null)
sb.append("DeploymentGroupName: ").append(getDeploymentGroupName()).append(",");
if (getDeploymentConfigName() != null)
sb.append("DeploymentConfigName: ").append(getDeploymentConfigName()).append(",");
if (getDeploymentId() != null)
sb.append("DeploymentId: ").append(getDeploymentId()).append(",");
if (getPreviousRevision() != null)
sb.append("PreviousRevision: ").append(getPreviousRevision()).append(",");
if (getRevision() != null)
sb.append("Revision: ").append(getRevision()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getErrorInformation() != null)
sb.append("ErrorInformation: ").append(getErrorInformation()).append(",");
if (getCreateTime() != null)
sb.append("CreateTime: ").append(getCreateTime()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getCompleteTime() != null)
sb.append("CompleteTime: ").append(getCompleteTime()).append(",");
if (getDeploymentOverview() != null)
sb.append("DeploymentOverview: ").append(getDeploymentOverview()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getCreator() != null)
sb.append("Creator: ").append(getCreator()).append(",");
if (getIgnoreApplicationStopFailures() != null)
sb.append("IgnoreApplicationStopFailures: ").append(getIgnoreApplicationStopFailures()).append(",");
if (getAutoRollbackConfiguration() != null)
sb.append("AutoRollbackConfiguration: ").append(getAutoRollbackConfiguration()).append(",");
if (getUpdateOutdatedInstancesOnly() != null)
sb.append("UpdateOutdatedInstancesOnly: ").append(getUpdateOutdatedInstancesOnly()).append(",");
if (getRollbackInfo() != null)
sb.append("RollbackInfo: ").append(getRollbackInfo()).append(",");
if (getDeploymentStyle() != null)
sb.append("DeploymentStyle: ").append(getDeploymentStyle()).append(",");
if (getTargetInstances() != null)
sb.append("TargetInstances: ").append(getTargetInstances()).append(",");
if (getInstanceTerminationWaitTimeStarted() != null)
sb.append("InstanceTerminationWaitTimeStarted: ").append(getInstanceTerminationWaitTimeStarted()).append(",");
if (getBlueGreenDeploymentConfiguration() != null)
sb.append("BlueGreenDeploymentConfiguration: ").append(getBlueGreenDeploymentConfiguration()).append(",");
if (getLoadBalancerInfo() != null)
sb.append("LoadBalancerInfo: ").append(getLoadBalancerInfo()).append(",");
if (getAdditionalDeploymentStatusInfo() != null)
sb.append("AdditionalDeploymentStatusInfo: ").append(getAdditionalDeploymentStatusInfo()).append(",");
if (getFileExistsBehavior() != null)
sb.append("FileExistsBehavior: ").append(getFileExistsBehavior()).append(",");
if (getDeploymentStatusMessages() != null)
sb.append("DeploymentStatusMessages: ").append(getDeploymentStatusMessages()).append(",");
if (getComputePlatform() != null)
sb.append("ComputePlatform: ").append(getComputePlatform()).append(",");
if (getExternalId() != null)
sb.append("ExternalId: ").append(getExternalId()).append(",");
if (getRelatedDeployments() != null)
sb.append("RelatedDeployments: ").append(getRelatedDeployments()).append(",");
if (getOverrideAlarmConfiguration() != null)
sb.append("OverrideAlarmConfiguration: ").append(getOverrideAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DeploymentInfo == false)
return false;
DeploymentInfo other = (DeploymentInfo) obj;
if (other.getApplicationName() == null ^ this.getApplicationName() == null)
return false;
if (other.getApplicationName() != null && other.getApplicationName().equals(this.getApplicationName()) == false)
return false;
if (other.getDeploymentGroupName() == null ^ this.getDeploymentGroupName() == null)
return false;
if (other.getDeploymentGroupName() != null && other.getDeploymentGroupName().equals(this.getDeploymentGroupName()) == false)
return false;
if (other.getDeploymentConfigName() == null ^ this.getDeploymentConfigName() == null)
return false;
if (other.getDeploymentConfigName() != null && other.getDeploymentConfigName().equals(this.getDeploymentConfigName()) == false)
return false;
if (other.getDeploymentId() == null ^ this.getDeploymentId() == null)
return false;
if (other.getDeploymentId() != null && other.getDeploymentId().equals(this.getDeploymentId()) == false)
return false;
if (other.getPreviousRevision() == null ^ this.getPreviousRevision() == null)
return false;
if (other.getPreviousRevision() != null && other.getPreviousRevision().equals(this.getPreviousRevision()) == false)
return false;
if (other.getRevision() == null ^ this.getRevision() == null)
return false;
if (other.getRevision() != null && other.getRevision().equals(this.getRevision()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getErrorInformation() == null ^ this.getErrorInformation() == null)
return false;
if (other.getErrorInformation() != null && other.getErrorInformation().equals(this.getErrorInformation()) == false)
return false;
if (other.getCreateTime() == null ^ this.getCreateTime() == null)
return false;
if (other.getCreateTime() != null && other.getCreateTime().equals(this.getCreateTime()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getCompleteTime() == null ^ this.getCompleteTime() == null)
return false;
if (other.getCompleteTime() != null && other.getCompleteTime().equals(this.getCompleteTime()) == false)
return false;
if (other.getDeploymentOverview() == null ^ this.getDeploymentOverview() == null)
return false;
if (other.getDeploymentOverview() != null && other.getDeploymentOverview().equals(this.getDeploymentOverview()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCreator() == null ^ this.getCreator() == null)
return false;
if (other.getCreator() != null && other.getCreator().equals(this.getCreator()) == false)
return false;
if (other.getIgnoreApplicationStopFailures() == null ^ this.getIgnoreApplicationStopFailures() == null)
return false;
if (other.getIgnoreApplicationStopFailures() != null
&& other.getIgnoreApplicationStopFailures().equals(this.getIgnoreApplicationStopFailures()) == false)
return false;
if (other.getAutoRollbackConfiguration() == null ^ this.getAutoRollbackConfiguration() == null)
return false;
if (other.getAutoRollbackConfiguration() != null && other.getAutoRollbackConfiguration().equals(this.getAutoRollbackConfiguration()) == false)
return false;
if (other.getUpdateOutdatedInstancesOnly() == null ^ this.getUpdateOutdatedInstancesOnly() == null)
return false;
if (other.getUpdateOutdatedInstancesOnly() != null && other.getUpdateOutdatedInstancesOnly().equals(this.getUpdateOutdatedInstancesOnly()) == false)
return false;
if (other.getRollbackInfo() == null ^ this.getRollbackInfo() == null)
return false;
if (other.getRollbackInfo() != null && other.getRollbackInfo().equals(this.getRollbackInfo()) == false)
return false;
if (other.getDeploymentStyle() == null ^ this.getDeploymentStyle() == null)
return false;
if (other.getDeploymentStyle() != null && other.getDeploymentStyle().equals(this.getDeploymentStyle()) == false)
return false;
if (other.getTargetInstances() == null ^ this.getTargetInstances() == null)
return false;
if (other.getTargetInstances() != null && other.getTargetInstances().equals(this.getTargetInstances()) == false)
return false;
if (other.getInstanceTerminationWaitTimeStarted() == null ^ this.getInstanceTerminationWaitTimeStarted() == null)
return false;
if (other.getInstanceTerminationWaitTimeStarted() != null
&& other.getInstanceTerminationWaitTimeStarted().equals(this.getInstanceTerminationWaitTimeStarted()) == false)
return false;
if (other.getBlueGreenDeploymentConfiguration() == null ^ this.getBlueGreenDeploymentConfiguration() == null)
return false;
if (other.getBlueGreenDeploymentConfiguration() != null
&& other.getBlueGreenDeploymentConfiguration().equals(this.getBlueGreenDeploymentConfiguration()) == false)
return false;
if (other.getLoadBalancerInfo() == null ^ this.getLoadBalancerInfo() == null)
return false;
if (other.getLoadBalancerInfo() != null && other.getLoadBalancerInfo().equals(this.getLoadBalancerInfo()) == false)
return false;
if (other.getAdditionalDeploymentStatusInfo() == null ^ this.getAdditionalDeploymentStatusInfo() == null)
return false;
if (other.getAdditionalDeploymentStatusInfo() != null
&& other.getAdditionalDeploymentStatusInfo().equals(this.getAdditionalDeploymentStatusInfo()) == false)
return false;
if (other.getFileExistsBehavior() == null ^ this.getFileExistsBehavior() == null)
return false;
if (other.getFileExistsBehavior() != null && other.getFileExistsBehavior().equals(this.getFileExistsBehavior()) == false)
return false;
if (other.getDeploymentStatusMessages() == null ^ this.getDeploymentStatusMessages() == null)
return false;
if (other.getDeploymentStatusMessages() != null && other.getDeploymentStatusMessages().equals(this.getDeploymentStatusMessages()) == false)
return false;
if (other.getComputePlatform() == null ^ this.getComputePlatform() == null)
return false;
if (other.getComputePlatform() != null && other.getComputePlatform().equals(this.getComputePlatform()) == false)
return false;
if (other.getExternalId() == null ^ this.getExternalId() == null)
return false;
if (other.getExternalId() != null && other.getExternalId().equals(this.getExternalId()) == false)
return false;
if (other.getRelatedDeployments() == null ^ this.getRelatedDeployments() == null)
return false;
if (other.getRelatedDeployments() != null && other.getRelatedDeployments().equals(this.getRelatedDeployments()) == false)
return false;
if (other.getOverrideAlarmConfiguration() == null ^ this.getOverrideAlarmConfiguration() == null)
return false;
if (other.getOverrideAlarmConfiguration() != null && other.getOverrideAlarmConfiguration().equals(this.getOverrideAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationName() == null) ? 0 : getApplicationName().hashCode());
hashCode = prime * hashCode + ((getDeploymentGroupName() == null) ? 0 : getDeploymentGroupName().hashCode());
hashCode = prime * hashCode + ((getDeploymentConfigName() == null) ? 0 : getDeploymentConfigName().hashCode());
hashCode = prime * hashCode + ((getDeploymentId() == null) ? 0 : getDeploymentId().hashCode());
hashCode = prime * hashCode + ((getPreviousRevision() == null) ? 0 : getPreviousRevision().hashCode());
hashCode = prime * hashCode + ((getRevision() == null) ? 0 : getRevision().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getErrorInformation() == null) ? 0 : getErrorInformation().hashCode());
hashCode = prime * hashCode + ((getCreateTime() == null) ? 0 : getCreateTime().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getCompleteTime() == null) ? 0 : getCompleteTime().hashCode());
hashCode = prime * hashCode + ((getDeploymentOverview() == null) ? 0 : getDeploymentOverview().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCreator() == null) ? 0 : getCreator().hashCode());
hashCode = prime * hashCode + ((getIgnoreApplicationStopFailures() == null) ? 0 : getIgnoreApplicationStopFailures().hashCode());
hashCode = prime * hashCode + ((getAutoRollbackConfiguration() == null) ? 0 : getAutoRollbackConfiguration().hashCode());
hashCode = prime * hashCode + ((getUpdateOutdatedInstancesOnly() == null) ? 0 : getUpdateOutdatedInstancesOnly().hashCode());
hashCode = prime * hashCode + ((getRollbackInfo() == null) ? 0 : getRollbackInfo().hashCode());
hashCode = prime * hashCode + ((getDeploymentStyle() == null) ? 0 : getDeploymentStyle().hashCode());
hashCode = prime * hashCode + ((getTargetInstances() == null) ? 0 : getTargetInstances().hashCode());
hashCode = prime * hashCode + ((getInstanceTerminationWaitTimeStarted() == null) ? 0 : getInstanceTerminationWaitTimeStarted().hashCode());
hashCode = prime * hashCode + ((getBlueGreenDeploymentConfiguration() == null) ? 0 : getBlueGreenDeploymentConfiguration().hashCode());
hashCode = prime * hashCode + ((getLoadBalancerInfo() == null) ? 0 : getLoadBalancerInfo().hashCode());
hashCode = prime * hashCode + ((getAdditionalDeploymentStatusInfo() == null) ? 0 : getAdditionalDeploymentStatusInfo().hashCode());
hashCode = prime * hashCode + ((getFileExistsBehavior() == null) ? 0 : getFileExistsBehavior().hashCode());
hashCode = prime * hashCode + ((getDeploymentStatusMessages() == null) ? 0 : getDeploymentStatusMessages().hashCode());
hashCode = prime * hashCode + ((getComputePlatform() == null) ? 0 : getComputePlatform().hashCode());
hashCode = prime * hashCode + ((getExternalId() == null) ? 0 : getExternalId().hashCode());
hashCode = prime * hashCode + ((getRelatedDeployments() == null) ? 0 : getRelatedDeployments().hashCode());
hashCode = prime * hashCode + ((getOverrideAlarmConfiguration() == null) ? 0 : getOverrideAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public DeploymentInfo clone() {
try {
return (DeploymentInfo) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.codedeploy.model.transform.DeploymentInfoMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}