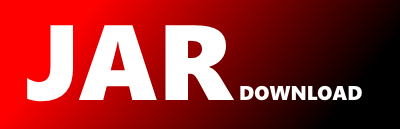
com.amazonaws.services.codedeploy.AmazonCodeDeployAsyncClient Maven / Gradle / Ivy
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.codedeploy;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.services.codedeploy.model.*;
/**
* Asynchronous client for accessing AmazonCodeDeploy.
* All asynchronous calls made using this client are non-blocking. Callers could either
* process the result and handle the exceptions in the worker thread by providing a callback handler
* when making the call, or use the returned Future object to check the result of the call in the calling thread.
* AWS CodeDeploy Overview
* This is the AWS CodeDeploy API Reference. This guide provides
* descriptions of the AWS CodeDeploy APIs. For additional information,
* see the
* AWS CodeDeploy User Guide
* .
*
* Using the APIs
* You can use the AWS CodeDeploy APIs to work with the following items:
*
*
*
* -
* Applications , which are unique identifiers that AWS
* CodeDeploy uses to ensure that the correct combinations of revisions,
* deployment configurations, and deployment groups are being referenced
* during deployments.
*
*
* You can work with applications by calling CreateApplication,
* DeleteApplication, GetApplication, ListApplications,
* BatchGetApplications, and UpdateApplication to create, delete, and get
* information about applications, and to change information about an
* application, respectively.
*
*
* -
* Deployment configurations , which are sets of deployment rules
* and deployment success and failure conditions that AWS CodeDeploy uses
* during deployments.
*
*
* You can work with deployment configurations by calling
* CreateDeploymentConfig, DeleteDeploymentConfig, GetDeploymentConfig,
* and ListDeploymentConfigs to create, delete, and get information about
* deployment configurations, respectively.
*
*
* -
* Deployment groups , which represent groups of Amazon EC2
* instances to which application revisions can be deployed.
*
*
* You can work with deployment groups by calling CreateDeploymentGroup,
* DeleteDeploymentGroup, GetDeploymentGroup, ListDeploymentGroups, and
* UpdateDeploymentGroup to create, delete, and get information about
* single and multiple deployment groups, and to change information about
* a deployment group, respectively.
*
*
* -
* Deployment instances (also known simply as instances ),
* which represent Amazon EC2 instances to which application revisions
* are deployed. Deployment instances are identified by their Amazon EC2
* tags or Auto Scaling group names. Deployment instances belong to
* deployment groups.
*
*
* You can work with deployment instances by calling
* GetDeploymentInstance and ListDeploymentInstances to get information
* about single and multiple deployment instances, respectively.
*
*
* -
* Deployments , which represent the process of deploying
* revisions to deployment groups.
*
*
* You can work with deployments by calling CreateDeployment,
* GetDeployment, ListDeployments, BatchGetDeployments, and
* StopDeployment to create and get information about deployments, and to
* stop a deployment, respectively.
*
*
* -
* Application revisions (also known simply as revisions
* ), which are archive files that are stored in Amazon S3 buckets or
* GitHub repositories. These revisions contain source content (such as
* source code, web pages, executable files, any deployment scripts, and
* similar) along with an Application Specification file (AppSpec file).
* (The AppSpec file is unique to AWS CodeDeploy; it defines a series of
* deployment actions that you want AWS CodeDeploy to execute.) An
* application revision is uniquely identified by its Amazon S3 object
* key and its ETag, version, or both. Application revisions are deployed
* to deployment groups.
*
*
* You can work with application revisions by calling
* GetApplicationRevision, ListApplicationRevisions, and
* RegisterApplicationRevision to get information about application
* revisions and to inform AWS CodeDeploy about an application revision,
* respectively.
*
*
*
*
*/
public class AmazonCodeDeployAsyncClient extends AmazonCodeDeployClient
implements AmazonCodeDeployAsync {
/**
* Executor service for executing asynchronous requests.
*/
private ExecutorService executorService;
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonCodeDeployAsyncClient() {
this(new DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonCodeDeploy
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonCodeDeployAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonCodeDeployAsyncClient(AWSCredentials awsCredentials) {
this(awsCredentials, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonCodeDeployAsyncClient(AWSCredentials awsCredentials, ExecutorService executorService) {
super(awsCredentials);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials,
* executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonCodeDeployAsyncClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials provider.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonCodeDeployAsyncClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials provider
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonCodeDeployAsyncClient(AWSCredentialsProvider awsCredentialsProvider, ExecutorService executorService) {
this(awsCredentialsProvider, new ClientConfiguration(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials
* provider and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
*/
public AmazonCodeDeployAsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonCodeDeploy using the specified AWS account credentials
* provider, executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonCodeDeployAsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this async client to execute
* requests.
*
* @return The executor service used by this async client to execute
* requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call getExecutorService().shutdown() followed by
* getExecutorService().awaitTermination() prior to calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
/**
*
* Gets information about a deployment group.
*
*
* @param getDeploymentGroupRequest Container for the necessary
* parameters to execute the GetDeploymentGroup operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* GetDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentGroupAsync(final GetDeploymentGroupRequest getDeploymentGroupRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentGroupResult call() throws Exception {
return getDeploymentGroup(getDeploymentGroupRequest);
}
});
}
/**
*
* Gets information about a deployment group.
*
*
* @param getDeploymentGroupRequest Container for the necessary
* parameters to execute the GetDeploymentGroup operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentGroupAsync(
final GetDeploymentGroupRequest getDeploymentGroupRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentGroupResult call() throws Exception {
GetDeploymentGroupResult result;
try {
result = getDeploymentGroup(getDeploymentGroupRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getDeploymentGroupRequest, result);
return result;
}
});
}
/**
*
* Changes an existing application's name.
*
*
* @param updateApplicationRequest Container for the necessary parameters
* to execute the UpdateApplication operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* UpdateApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateApplicationAsync(final UpdateApplicationRequest updateApplicationRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateApplication(updateApplicationRequest);
return null;
}
});
}
/**
*
* Changes an existing application's name.
*
*
* @param updateApplicationRequest Container for the necessary parameters
* to execute the UpdateApplication operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateApplicationAsync(
final UpdateApplicationRequest updateApplicationRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateApplication(updateApplicationRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateApplicationRequest, null);
return null;
}
});
}
/**
*
* Creates a new deployment configuration.
*
*
* @param createDeploymentConfigRequest Container for the necessary
* parameters to execute the CreateDeploymentConfig operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* CreateDeploymentConfig service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDeploymentConfigAsync(final CreateDeploymentConfigRequest createDeploymentConfigRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDeploymentConfigResult call() throws Exception {
return createDeploymentConfig(createDeploymentConfigRequest);
}
});
}
/**
*
* Creates a new deployment configuration.
*
*
* @param createDeploymentConfigRequest Container for the necessary
* parameters to execute the CreateDeploymentConfig operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateDeploymentConfig service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDeploymentConfigAsync(
final CreateDeploymentConfigRequest createDeploymentConfigRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDeploymentConfigResult call() throws Exception {
CreateDeploymentConfigResult result;
try {
result = createDeploymentConfig(createDeploymentConfigRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createDeploymentConfigRequest, result);
return result;
}
});
}
/**
*
* Lists the Amazon EC2 instances for a deployment within the AWS user
* account.
*
*
* @param listDeploymentInstancesRequest Container for the necessary
* parameters to execute the ListDeploymentInstances operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* ListDeploymentInstances service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentInstancesAsync(final ListDeploymentInstancesRequest listDeploymentInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentInstancesResult call() throws Exception {
return listDeploymentInstances(listDeploymentInstancesRequest);
}
});
}
/**
*
* Lists the Amazon EC2 instances for a deployment within the AWS user
* account.
*
*
* @param listDeploymentInstancesRequest Container for the necessary
* parameters to execute the ListDeploymentInstances operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListDeploymentInstances service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentInstancesAsync(
final ListDeploymentInstancesRequest listDeploymentInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentInstancesResult call() throws Exception {
ListDeploymentInstancesResult result;
try {
result = listDeploymentInstances(listDeploymentInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listDeploymentInstancesRequest, result);
return result;
}
});
}
/**
*
* Lists the applications registered within the AWS user account.
*
*
* @param listApplicationsRequest Container for the necessary parameters
* to execute the ListApplications operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* ListApplications service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listApplicationsAsync(final ListApplicationsRequest listApplicationsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListApplicationsResult call() throws Exception {
return listApplications(listApplicationsRequest);
}
});
}
/**
*
* Lists the applications registered within the AWS user account.
*
*
* @param listApplicationsRequest Container for the necessary parameters
* to execute the ListApplications operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListApplications service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listApplicationsAsync(
final ListApplicationsRequest listApplicationsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListApplicationsResult call() throws Exception {
ListApplicationsResult result;
try {
result = listApplications(listApplicationsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listApplicationsRequest, result);
return result;
}
});
}
/**
*
* Registers with AWS CodeDeploy a revision for the specified
* application.
*
*
* @param registerApplicationRevisionRequest Container for the necessary
* parameters to execute the RegisterApplicationRevision operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* RegisterApplicationRevision service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerApplicationRevisionAsync(final RegisterApplicationRevisionRequest registerApplicationRevisionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
registerApplicationRevision(registerApplicationRevisionRequest);
return null;
}
});
}
/**
*
* Registers with AWS CodeDeploy a revision for the specified
* application.
*
*
* @param registerApplicationRevisionRequest Container for the necessary
* parameters to execute the RegisterApplicationRevision operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RegisterApplicationRevision service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerApplicationRevisionAsync(
final RegisterApplicationRevisionRequest registerApplicationRevisionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
registerApplicationRevision(registerApplicationRevisionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(registerApplicationRevisionRequest, null);
return null;
}
});
}
/**
*
* Gets information about a deployment configuration.
*
*
* @param getDeploymentConfigRequest Container for the necessary
* parameters to execute the GetDeploymentConfig operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* GetDeploymentConfig service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentConfigAsync(final GetDeploymentConfigRequest getDeploymentConfigRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentConfigResult call() throws Exception {
return getDeploymentConfig(getDeploymentConfigRequest);
}
});
}
/**
*
* Gets information about a deployment configuration.
*
*
* @param getDeploymentConfigRequest Container for the necessary
* parameters to execute the GetDeploymentConfig operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetDeploymentConfig service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentConfigAsync(
final GetDeploymentConfigRequest getDeploymentConfigRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentConfigResult call() throws Exception {
GetDeploymentConfigResult result;
try {
result = getDeploymentConfig(getDeploymentConfigRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getDeploymentConfigRequest, result);
return result;
}
});
}
/**
*
* Lists the deployment groups for an application registered within the
* AWS user account.
*
*
* @param listDeploymentGroupsRequest Container for the necessary
* parameters to execute the ListDeploymentGroups operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* ListDeploymentGroups service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentGroupsAsync(final ListDeploymentGroupsRequest listDeploymentGroupsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentGroupsResult call() throws Exception {
return listDeploymentGroups(listDeploymentGroupsRequest);
}
});
}
/**
*
* Lists the deployment groups for an application registered within the
* AWS user account.
*
*
* @param listDeploymentGroupsRequest Container for the necessary
* parameters to execute the ListDeploymentGroups operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListDeploymentGroups service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentGroupsAsync(
final ListDeploymentGroupsRequest listDeploymentGroupsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentGroupsResult call() throws Exception {
ListDeploymentGroupsResult result;
try {
result = listDeploymentGroups(listDeploymentGroupsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listDeploymentGroupsRequest, result);
return result;
}
});
}
/**
*
* Gets information about one or more applications.
*
*
* @param batchGetApplicationsRequest Container for the necessary
* parameters to execute the BatchGetApplications operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* BatchGetApplications service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future batchGetApplicationsAsync(final BatchGetApplicationsRequest batchGetApplicationsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public BatchGetApplicationsResult call() throws Exception {
return batchGetApplications(batchGetApplicationsRequest);
}
});
}
/**
*
* Gets information about one or more applications.
*
*
* @param batchGetApplicationsRequest Container for the necessary
* parameters to execute the BatchGetApplications operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* BatchGetApplications service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future batchGetApplicationsAsync(
final BatchGetApplicationsRequest batchGetApplicationsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public BatchGetApplicationsResult call() throws Exception {
BatchGetApplicationsResult result;
try {
result = batchGetApplications(batchGetApplicationsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(batchGetApplicationsRequest, result);
return result;
}
});
}
/**
*
* Deletes a deployment group.
*
*
* @param deleteDeploymentGroupRequest Container for the necessary
* parameters to execute the DeleteDeploymentGroup operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* DeleteDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteDeploymentGroupAsync(final DeleteDeploymentGroupRequest deleteDeploymentGroupRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DeleteDeploymentGroupResult call() throws Exception {
return deleteDeploymentGroup(deleteDeploymentGroupRequest);
}
});
}
/**
*
* Deletes a deployment group.
*
*
* @param deleteDeploymentGroupRequest Container for the necessary
* parameters to execute the DeleteDeploymentGroup operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteDeploymentGroupAsync(
final DeleteDeploymentGroupRequest deleteDeploymentGroupRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DeleteDeploymentGroupResult call() throws Exception {
DeleteDeploymentGroupResult result;
try {
result = deleteDeploymentGroup(deleteDeploymentGroupRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteDeploymentGroupRequest, result);
return result;
}
});
}
/**
*
* Lists information about revisions for an application.
*
*
* @param listApplicationRevisionsRequest Container for the necessary
* parameters to execute the ListApplicationRevisions operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* ListApplicationRevisions service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listApplicationRevisionsAsync(final ListApplicationRevisionsRequest listApplicationRevisionsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListApplicationRevisionsResult call() throws Exception {
return listApplicationRevisions(listApplicationRevisionsRequest);
}
});
}
/**
*
* Lists information about revisions for an application.
*
*
* @param listApplicationRevisionsRequest Container for the necessary
* parameters to execute the ListApplicationRevisions operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListApplicationRevisions service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listApplicationRevisionsAsync(
final ListApplicationRevisionsRequest listApplicationRevisionsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListApplicationRevisionsResult call() throws Exception {
ListApplicationRevisionsResult result;
try {
result = listApplicationRevisions(listApplicationRevisionsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listApplicationRevisionsRequest, result);
return result;
}
});
}
/**
*
* Changes information about an existing deployment group.
*
*
* @param updateDeploymentGroupRequest Container for the necessary
* parameters to execute the UpdateDeploymentGroup operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* UpdateDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateDeploymentGroupAsync(final UpdateDeploymentGroupRequest updateDeploymentGroupRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public UpdateDeploymentGroupResult call() throws Exception {
return updateDeploymentGroup(updateDeploymentGroupRequest);
}
});
}
/**
*
* Changes information about an existing deployment group.
*
*
* @param updateDeploymentGroupRequest Container for the necessary
* parameters to execute the UpdateDeploymentGroup operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateDeploymentGroupAsync(
final UpdateDeploymentGroupRequest updateDeploymentGroupRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public UpdateDeploymentGroupResult call() throws Exception {
UpdateDeploymentGroupResult result;
try {
result = updateDeploymentGroup(updateDeploymentGroupRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateDeploymentGroupRequest, result);
return result;
}
});
}
/**
*
* Lists the deployments under a deployment group for an application
* registered within the AWS user account.
*
*
* @param listDeploymentsRequest Container for the necessary parameters
* to execute the ListDeployments operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* ListDeployments service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentsAsync(final ListDeploymentsRequest listDeploymentsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentsResult call() throws Exception {
return listDeployments(listDeploymentsRequest);
}
});
}
/**
*
* Lists the deployments under a deployment group for an application
* registered within the AWS user account.
*
*
* @param listDeploymentsRequest Container for the necessary parameters
* to execute the ListDeployments operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListDeployments service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentsAsync(
final ListDeploymentsRequest listDeploymentsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentsResult call() throws Exception {
ListDeploymentsResult result;
try {
result = listDeployments(listDeploymentsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listDeploymentsRequest, result);
return result;
}
});
}
/**
*
* Gets information about an application.
*
*
* @param getApplicationRequest Container for the necessary parameters to
* execute the GetApplication operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* GetApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getApplicationAsync(final GetApplicationRequest getApplicationRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetApplicationResult call() throws Exception {
return getApplication(getApplicationRequest);
}
});
}
/**
*
* Gets information about an application.
*
*
* @param getApplicationRequest Container for the necessary parameters to
* execute the GetApplication operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getApplicationAsync(
final GetApplicationRequest getApplicationRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetApplicationResult call() throws Exception {
GetApplicationResult result;
try {
result = getApplication(getApplicationRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getApplicationRequest, result);
return result;
}
});
}
/**
*
* Creates a new application.
*
*
* @param createApplicationRequest Container for the necessary parameters
* to execute the CreateApplication operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* CreateApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createApplicationAsync(final CreateApplicationRequest createApplicationRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateApplicationResult call() throws Exception {
return createApplication(createApplicationRequest);
}
});
}
/**
*
* Creates a new application.
*
*
* @param createApplicationRequest Container for the necessary parameters
* to execute the CreateApplication operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createApplicationAsync(
final CreateApplicationRequest createApplicationRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateApplicationResult call() throws Exception {
CreateApplicationResult result;
try {
result = createApplication(createApplicationRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createApplicationRequest, result);
return result;
}
});
}
/**
*
* Attempts to stop an ongoing deployment.
*
*
* @param stopDeploymentRequest Container for the necessary parameters to
* execute the StopDeployment operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* StopDeployment service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future stopDeploymentAsync(final StopDeploymentRequest stopDeploymentRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public StopDeploymentResult call() throws Exception {
return stopDeployment(stopDeploymentRequest);
}
});
}
/**
*
* Attempts to stop an ongoing deployment.
*
*
* @param stopDeploymentRequest Container for the necessary parameters to
* execute the StopDeployment operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* StopDeployment service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future stopDeploymentAsync(
final StopDeploymentRequest stopDeploymentRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public StopDeploymentResult call() throws Exception {
StopDeploymentResult result;
try {
result = stopDeployment(stopDeploymentRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(stopDeploymentRequest, result);
return result;
}
});
}
/**
*
* Creates a new deployment group for application revisions to be
* deployed to.
*
*
* @param createDeploymentGroupRequest Container for the necessary
* parameters to execute the CreateDeploymentGroup operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* CreateDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDeploymentGroupAsync(final CreateDeploymentGroupRequest createDeploymentGroupRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDeploymentGroupResult call() throws Exception {
return createDeploymentGroup(createDeploymentGroupRequest);
}
});
}
/**
*
* Creates a new deployment group for application revisions to be
* deployed to.
*
*
* @param createDeploymentGroupRequest Container for the necessary
* parameters to execute the CreateDeploymentGroup operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateDeploymentGroup service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDeploymentGroupAsync(
final CreateDeploymentGroupRequest createDeploymentGroupRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDeploymentGroupResult call() throws Exception {
CreateDeploymentGroupResult result;
try {
result = createDeploymentGroup(createDeploymentGroupRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createDeploymentGroupRequest, result);
return result;
}
});
}
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest Container for the necessary parameters
* to execute the DeleteApplication operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* DeleteApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteApplicationAsync(final DeleteApplicationRequest deleteApplicationRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteApplication(deleteApplicationRequest);
return null;
}
});
}
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest Container for the necessary parameters
* to execute the DeleteApplication operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteApplication service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteApplicationAsync(
final DeleteApplicationRequest deleteApplicationRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteApplication(deleteApplicationRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteApplicationRequest, null);
return null;
}
});
}
/**
*
* Gets information about one or more deployments.
*
*
* @param batchGetDeploymentsRequest Container for the necessary
* parameters to execute the BatchGetDeployments operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* BatchGetDeployments service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future batchGetDeploymentsAsync(final BatchGetDeploymentsRequest batchGetDeploymentsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public BatchGetDeploymentsResult call() throws Exception {
return batchGetDeployments(batchGetDeploymentsRequest);
}
});
}
/**
*
* Gets information about one or more deployments.
*
*
* @param batchGetDeploymentsRequest Container for the necessary
* parameters to execute the BatchGetDeployments operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* BatchGetDeployments service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future batchGetDeploymentsAsync(
final BatchGetDeploymentsRequest batchGetDeploymentsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public BatchGetDeploymentsResult call() throws Exception {
BatchGetDeploymentsResult result;
try {
result = batchGetDeployments(batchGetDeploymentsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(batchGetDeploymentsRequest, result);
return result;
}
});
}
/**
*
* Deletes a deployment configuration.
*
*
* NOTE:A deployment configuration cannot be deleted if it is
* currently in use. Also, predefined configurations cannot be deleted.
*
*
* @param deleteDeploymentConfigRequest Container for the necessary
* parameters to execute the DeleteDeploymentConfig operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* DeleteDeploymentConfig service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteDeploymentConfigAsync(final DeleteDeploymentConfigRequest deleteDeploymentConfigRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteDeploymentConfig(deleteDeploymentConfigRequest);
return null;
}
});
}
/**
*
* Deletes a deployment configuration.
*
*
* NOTE:A deployment configuration cannot be deleted if it is
* currently in use. Also, predefined configurations cannot be deleted.
*
*
* @param deleteDeploymentConfigRequest Container for the necessary
* parameters to execute the DeleteDeploymentConfig operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteDeploymentConfig service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteDeploymentConfigAsync(
final DeleteDeploymentConfigRequest deleteDeploymentConfigRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteDeploymentConfig(deleteDeploymentConfigRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteDeploymentConfigRequest, null);
return null;
}
});
}
/**
*
* Gets information about a deployment.
*
*
* @param getDeploymentRequest Container for the necessary parameters to
* execute the GetDeployment operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* GetDeployment service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentAsync(final GetDeploymentRequest getDeploymentRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentResult call() throws Exception {
return getDeployment(getDeploymentRequest);
}
});
}
/**
*
* Gets information about a deployment.
*
*
* @param getDeploymentRequest Container for the necessary parameters to
* execute the GetDeployment operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetDeployment service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentAsync(
final GetDeploymentRequest getDeploymentRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentResult call() throws Exception {
GetDeploymentResult result;
try {
result = getDeployment(getDeploymentRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getDeploymentRequest, result);
return result;
}
});
}
/**
*
* Deploys an application revision to the specified deployment group.
*
*
* @param createDeploymentRequest Container for the necessary parameters
* to execute the CreateDeployment operation on AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* CreateDeployment service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDeploymentAsync(final CreateDeploymentRequest createDeploymentRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDeploymentResult call() throws Exception {
return createDeployment(createDeploymentRequest);
}
});
}
/**
*
* Deploys an application revision to the specified deployment group.
*
*
* @param createDeploymentRequest Container for the necessary parameters
* to execute the CreateDeployment operation on AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateDeployment service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDeploymentAsync(
final CreateDeploymentRequest createDeploymentRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDeploymentResult call() throws Exception {
CreateDeploymentResult result;
try {
result = createDeployment(createDeploymentRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createDeploymentRequest, result);
return result;
}
});
}
/**
*
* Gets information about an Amazon EC2 instance as part of a
* deployment.
*
*
* @param getDeploymentInstanceRequest Container for the necessary
* parameters to execute the GetDeploymentInstance operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* GetDeploymentInstance service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentInstanceAsync(final GetDeploymentInstanceRequest getDeploymentInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentInstanceResult call() throws Exception {
return getDeploymentInstance(getDeploymentInstanceRequest);
}
});
}
/**
*
* Gets information about an Amazon EC2 instance as part of a
* deployment.
*
*
* @param getDeploymentInstanceRequest Container for the necessary
* parameters to execute the GetDeploymentInstance operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetDeploymentInstance service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getDeploymentInstanceAsync(
final GetDeploymentInstanceRequest getDeploymentInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetDeploymentInstanceResult call() throws Exception {
GetDeploymentInstanceResult result;
try {
result = getDeploymentInstance(getDeploymentInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getDeploymentInstanceRequest, result);
return result;
}
});
}
/**
*
* Lists the deployment configurations within the AWS user account.
*
*
* @param listDeploymentConfigsRequest Container for the necessary
* parameters to execute the ListDeploymentConfigs operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* ListDeploymentConfigs service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentConfigsAsync(final ListDeploymentConfigsRequest listDeploymentConfigsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentConfigsResult call() throws Exception {
return listDeploymentConfigs(listDeploymentConfigsRequest);
}
});
}
/**
*
* Lists the deployment configurations within the AWS user account.
*
*
* @param listDeploymentConfigsRequest Container for the necessary
* parameters to execute the ListDeploymentConfigs operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListDeploymentConfigs service method, as returned by AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDeploymentConfigsAsync(
final ListDeploymentConfigsRequest listDeploymentConfigsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListDeploymentConfigsResult call() throws Exception {
ListDeploymentConfigsResult result;
try {
result = listDeploymentConfigs(listDeploymentConfigsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listDeploymentConfigsRequest, result);
return result;
}
});
}
/**
*
* Gets information about an application revision.
*
*
* @param getApplicationRevisionRequest Container for the necessary
* parameters to execute the GetApplicationRevision operation on
* AmazonCodeDeploy.
*
* @return A Java Future object containing the response from the
* GetApplicationRevision service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getApplicationRevisionAsync(final GetApplicationRevisionRequest getApplicationRevisionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetApplicationRevisionResult call() throws Exception {
return getApplicationRevision(getApplicationRevisionRequest);
}
});
}
/**
*
* Gets information about an application revision.
*
*
* @param getApplicationRevisionRequest Container for the necessary
* parameters to execute the GetApplicationRevision operation on
* AmazonCodeDeploy.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetApplicationRevision service method, as returned by
* AmazonCodeDeploy.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCodeDeploy indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getApplicationRevisionAsync(
final GetApplicationRevisionRequest getApplicationRevisionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetApplicationRevisionResult call() throws Exception {
GetApplicationRevisionResult result;
try {
result = getApplicationRevision(getApplicationRevisionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getApplicationRevisionRequest, result);
return result;
}
});
}
}