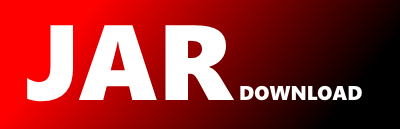
com.amazonaws.services.cognitoidentity.AbstractAmazonCognitoIdentityAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-cognitoidentity Show documentation
Show all versions of aws-java-sdk-cognitoidentity Show documentation
The AWS Java SDK for Amazon Cognito Identity module holds the client classes that are used for communicating with Amazon Cognito Identity Service
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidentity;
import javax.annotation.Generated;
import com.amazonaws.services.cognitoidentity.model.*;
/**
* Abstract implementation of {@code AmazonCognitoIdentityAsync}. Convenient method forms pass through to the
* corresponding overload that takes a request object and an {@code AsyncHandler}, which throws an
* {@code UnsupportedOperationException}.
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AbstractAmazonCognitoIdentityAsync extends AbstractAmazonCognitoIdentity implements AmazonCognitoIdentityAsync {
protected AbstractAmazonCognitoIdentityAsync() {
}
@Override
public java.util.concurrent.Future createIdentityPoolAsync(CreateIdentityPoolRequest request) {
return createIdentityPoolAsync(request, null);
}
@Override
public java.util.concurrent.Future createIdentityPoolAsync(CreateIdentityPoolRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future deleteIdentitiesAsync(DeleteIdentitiesRequest request) {
return deleteIdentitiesAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteIdentitiesAsync(DeleteIdentitiesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future deleteIdentityPoolAsync(DeleteIdentityPoolRequest request) {
return deleteIdentityPoolAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteIdentityPoolAsync(DeleteIdentityPoolRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future describeIdentityAsync(DescribeIdentityRequest request) {
return describeIdentityAsync(request, null);
}
@Override
public java.util.concurrent.Future describeIdentityAsync(DescribeIdentityRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future describeIdentityPoolAsync(DescribeIdentityPoolRequest request) {
return describeIdentityPoolAsync(request, null);
}
@Override
public java.util.concurrent.Future describeIdentityPoolAsync(DescribeIdentityPoolRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getCredentialsForIdentityAsync(GetCredentialsForIdentityRequest request) {
return getCredentialsForIdentityAsync(request, null);
}
@Override
public java.util.concurrent.Future getCredentialsForIdentityAsync(GetCredentialsForIdentityRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getIdAsync(GetIdRequest request) {
return getIdAsync(request, null);
}
@Override
public java.util.concurrent.Future getIdAsync(GetIdRequest request, com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getIdentityPoolRolesAsync(GetIdentityPoolRolesRequest request) {
return getIdentityPoolRolesAsync(request, null);
}
@Override
public java.util.concurrent.Future getIdentityPoolRolesAsync(GetIdentityPoolRolesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getOpenIdTokenAsync(GetOpenIdTokenRequest request) {
return getOpenIdTokenAsync(request, null);
}
@Override
public java.util.concurrent.Future getOpenIdTokenAsync(GetOpenIdTokenRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getOpenIdTokenForDeveloperIdentityAsync(
GetOpenIdTokenForDeveloperIdentityRequest request) {
return getOpenIdTokenForDeveloperIdentityAsync(request, null);
}
@Override
public java.util.concurrent.Future getOpenIdTokenForDeveloperIdentityAsync(
GetOpenIdTokenForDeveloperIdentityRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getPrincipalTagAttributeMapAsync(GetPrincipalTagAttributeMapRequest request) {
return getPrincipalTagAttributeMapAsync(request, null);
}
@Override
public java.util.concurrent.Future getPrincipalTagAttributeMapAsync(GetPrincipalTagAttributeMapRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listIdentitiesAsync(ListIdentitiesRequest request) {
return listIdentitiesAsync(request, null);
}
@Override
public java.util.concurrent.Future listIdentitiesAsync(ListIdentitiesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listIdentityPoolsAsync(ListIdentityPoolsRequest request) {
return listIdentityPoolsAsync(request, null);
}
@Override
public java.util.concurrent.Future listIdentityPoolsAsync(ListIdentityPoolsRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest request) {
return listTagsForResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future lookupDeveloperIdentityAsync(LookupDeveloperIdentityRequest request) {
return lookupDeveloperIdentityAsync(request, null);
}
@Override
public java.util.concurrent.Future lookupDeveloperIdentityAsync(LookupDeveloperIdentityRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future mergeDeveloperIdentitiesAsync(MergeDeveloperIdentitiesRequest request) {
return mergeDeveloperIdentitiesAsync(request, null);
}
@Override
public java.util.concurrent.Future mergeDeveloperIdentitiesAsync(MergeDeveloperIdentitiesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future setIdentityPoolRolesAsync(SetIdentityPoolRolesRequest request) {
return setIdentityPoolRolesAsync(request, null);
}
@Override
public java.util.concurrent.Future setIdentityPoolRolesAsync(SetIdentityPoolRolesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future setPrincipalTagAttributeMapAsync(SetPrincipalTagAttributeMapRequest request) {
return setPrincipalTagAttributeMapAsync(request, null);
}
@Override
public java.util.concurrent.Future setPrincipalTagAttributeMapAsync(SetPrincipalTagAttributeMapRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future tagResourceAsync(TagResourceRequest request) {
return tagResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future tagResourceAsync(TagResourceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future unlinkDeveloperIdentityAsync(UnlinkDeveloperIdentityRequest request) {
return unlinkDeveloperIdentityAsync(request, null);
}
@Override
public java.util.concurrent.Future unlinkDeveloperIdentityAsync(UnlinkDeveloperIdentityRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future unlinkIdentityAsync(UnlinkIdentityRequest request) {
return unlinkIdentityAsync(request, null);
}
@Override
public java.util.concurrent.Future unlinkIdentityAsync(UnlinkIdentityRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future untagResourceAsync(UntagResourceRequest request) {
return untagResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future untagResourceAsync(UntagResourceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future updateIdentityPoolAsync(UpdateIdentityPoolRequest request) {
return updateIdentityPoolAsync(request, null);
}
@Override
public java.util.concurrent.Future updateIdentityPoolAsync(UpdateIdentityPoolRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy