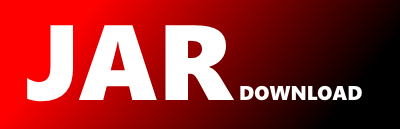
com.amazonaws.services.cognitoidentity.AmazonCognitoIdentity Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cognitoidentity Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidentity;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.cognitoidentity.model.*;
/**
* Interface for accessing Amazon Cognito Identity.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cognitoidentity.AbstractAmazonCognitoIdentity} instead.
*
*
* Amazon Cognito Federated Identities
*
* Amazon Cognito Federated Identities is a web service that delivers scoped temporary credentials to mobile devices and
* other untrusted environments. It uniquely identifies a device and supplies the user with a consistent identity over
* the lifetime of an application.
*
*
* Using Amazon Cognito Federated Identities, you can enable authentication with one or more third-party identity
* providers (Facebook, Google, or Login with Amazon) or an Amazon Cognito user pool, and you can also choose to support
* unauthenticated access from your app. Cognito delivers a unique identifier for each user and acts as an OpenID token
* provider trusted by AWS Security Token Service (STS) to access temporary, limited-privilege AWS credentials.
*
*
* For a description of the authentication flow from the Amazon Cognito Developer Guide see Authentication Flow.
*
*
* For more information see Amazon Cognito Federated
* Identities.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCognitoIdentity {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "cognito-identity";
/**
* Overrides the default endpoint for this client ("https://cognito-identity.us-east-1.amazonaws.com"). Callers can
* use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "cognito-identity.us-east-1.amazonaws.com") or a full URL, including
* the protocol (ex: "https://cognito-identity.us-east-1.amazonaws.com"). If the protocol is not specified here, the
* default protocol from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "cognito-identity.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://cognito-identity.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonCognitoIdentity#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Creates a new identity pool. The identity pool is a store of user identity information that is specific to your
* AWS account. The keys for SupportedLoginProviders
are as follows:
*
*
* -
*
* Facebook: graph.facebook.com
*
*
* -
*
* Google: accounts.google.com
*
*
* -
*
* Amazon: www.amazon.com
*
*
* -
*
* Twitter: api.twitter.com
*
*
* -
*
* Digits: www.digits.com
*
*
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param createIdentityPoolRequest
* Input to the CreateIdentityPool action.
* @return Result of the CreateIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws LimitExceededException
* Thrown when the total number of user pools has exceeded a preset limit.
* @sample AmazonCognitoIdentity.CreateIdentityPool
* @see AWS API Documentation
*/
CreateIdentityPoolResult createIdentityPool(CreateIdentityPoolRequest createIdentityPoolRequest);
/**
*
* Deletes identities from an identity pool. You can specify a list of 1-60 identities that you want to delete.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param deleteIdentitiesRequest
* Input to the DeleteIdentities
action.
* @return Result of the DeleteIdentities operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.DeleteIdentities
* @see AWS API Documentation
*/
DeleteIdentitiesResult deleteIdentities(DeleteIdentitiesRequest deleteIdentitiesRequest);
/**
*
* Deletes an identity pool. Once a pool is deleted, users will not be able to authenticate with the pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param deleteIdentityPoolRequest
* Input to the DeleteIdentityPool action.
* @return Result of the DeleteIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.DeleteIdentityPool
* @see AWS API Documentation
*/
DeleteIdentityPoolResult deleteIdentityPool(DeleteIdentityPoolRequest deleteIdentityPoolRequest);
/**
*
* Returns metadata related to the given identity, including when the identity was created and any associated linked
* logins.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param describeIdentityRequest
* Input to the DescribeIdentity
action.
* @return Result of the DescribeIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.DescribeIdentity
* @see AWS API Documentation
*/
DescribeIdentityResult describeIdentity(DescribeIdentityRequest describeIdentityRequest);
/**
*
* Gets details about a particular identity pool, including the pool name, ID description, creation date, and
* current number of users.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param describeIdentityPoolRequest
* Input to the DescribeIdentityPool action.
* @return Result of the DescribeIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.DescribeIdentityPool
* @see AWS API Documentation
*/
DescribeIdentityPoolResult describeIdentityPool(DescribeIdentityPoolRequest describeIdentityPoolRequest);
/**
*
* Returns credentials for the provided identity ID. Any provided logins will be validated against supported login
* providers. If the token is for cognito-identity.amazonaws.com, it will be passed through to AWS Security Token
* Service with the appropriate role for the token.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getCredentialsForIdentityRequest
* Input to the GetCredentialsForIdentity
action.
* @return Result of the GetCredentialsForIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InvalidIdentityPoolConfigurationException
* Thrown if the identity pool has no role associated for the given auth type (auth/unauth) or if the
* AssumeRole fails.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @sample AmazonCognitoIdentity.GetCredentialsForIdentity
* @see AWS API Documentation
*/
GetCredentialsForIdentityResult getCredentialsForIdentity(GetCredentialsForIdentityRequest getCredentialsForIdentityRequest);
/**
*
* Generates (or retrieves) a Cognito ID. Supplying multiple logins will create an implicit linked account.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getIdRequest
* Input to the GetId action.
* @return Result of the GetId operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws LimitExceededException
* Thrown when the total number of user pools has exceeded a preset limit.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @sample AmazonCognitoIdentity.GetId
* @see AWS API
* Documentation
*/
GetIdResult getId(GetIdRequest getIdRequest);
/**
*
* Gets the roles for an identity pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param getIdentityPoolRolesRequest
* Input to the GetIdentityPoolRoles
action.
* @return Result of the GetIdentityPoolRoles operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.GetIdentityPoolRoles
* @see AWS API Documentation
*/
GetIdentityPoolRolesResult getIdentityPoolRoles(GetIdentityPoolRolesRequest getIdentityPoolRolesRequest);
/**
*
* Gets an OpenID token, using a known Cognito ID. This known Cognito ID is returned by GetId. You can
* optionally add additional logins for the identity. Supplying multiple logins creates an implicit link.
*
*
* The OpenID token is valid for 10 minutes.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getOpenIdTokenRequest
* Input to the GetOpenIdToken action.
* @return Result of the GetOpenIdToken operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @sample AmazonCognitoIdentity.GetOpenIdToken
* @see AWS API Documentation
*/
GetOpenIdTokenResult getOpenIdToken(GetOpenIdTokenRequest getOpenIdTokenRequest);
/**
*
* Registers (or retrieves) a Cognito IdentityId
and an OpenID Connect token for a user authenticated
* by your backend authentication process. Supplying multiple logins will create an implicit linked account. You can
* only specify one developer provider as part of the Logins
map, which is linked to the identity pool.
* The developer provider is the "domain" by which Cognito will refer to your users.
*
*
* You can use GetOpenIdTokenForDeveloperIdentity
to create a new identity and to link new logins (that
* is, user credentials issued by a public provider or developer provider) to an existing identity. When you want to
* create a new identity, the IdentityId
should be null. When you want to associate a new login with an
* existing authenticated/unauthenticated identity, you can do so by providing the existing IdentityId
.
* This API will create the identity in the specified IdentityPoolId
.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param getOpenIdTokenForDeveloperIdentityRequest
* Input to the GetOpenIdTokenForDeveloperIdentity
action.
* @return Result of the GetOpenIdTokenForDeveloperIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws DeveloperUserAlreadyRegisteredException
* The provided developer user identifier is already registered with Cognito under a different identity ID.
* @sample AmazonCognitoIdentity.GetOpenIdTokenForDeveloperIdentity
* @see AWS API Documentation
*/
GetOpenIdTokenForDeveloperIdentityResult getOpenIdTokenForDeveloperIdentity(
GetOpenIdTokenForDeveloperIdentityRequest getOpenIdTokenForDeveloperIdentityRequest);
/**
*
* Use GetPrincipalTagAttributeMap
to list all mappings between PrincipalTags
and user
* attributes.
*
*
* @param getPrincipalTagAttributeMapRequest
* @return Result of the GetPrincipalTagAttributeMap operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.GetPrincipalTagAttributeMap
* @see AWS API Documentation
*/
GetPrincipalTagAttributeMapResult getPrincipalTagAttributeMap(GetPrincipalTagAttributeMapRequest getPrincipalTagAttributeMapRequest);
/**
*
* Lists the identities in an identity pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param listIdentitiesRequest
* Input to the ListIdentities action.
* @return Result of the ListIdentities operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.ListIdentities
* @see AWS API Documentation
*/
ListIdentitiesResult listIdentities(ListIdentitiesRequest listIdentitiesRequest);
/**
*
* Lists all of the Cognito identity pools registered for your account.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param listIdentityPoolsRequest
* Input to the ListIdentityPools action.
* @return Result of the ListIdentityPools operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.ListIdentityPools
* @see AWS API Documentation
*/
ListIdentityPoolsResult listIdentityPools(ListIdentityPoolsRequest listIdentityPoolsRequest);
/**
*
* Lists the tags that are assigned to an Amazon Cognito identity pool.
*
*
* A tag is a label that you can apply to identity pools to categorize and manage them in different ways, such as by
* purpose, owner, environment, or other criteria.
*
*
* You can use this action up to 10 times per second, per account.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves the IdentityID
associated with a DeveloperUserIdentifier
or the list of
* DeveloperUserIdentifier
values associated with an IdentityId
for an existing identity.
* Either IdentityID
or DeveloperUserIdentifier
must not be null. If you supply only one
* of these values, the other value will be searched in the database and returned as a part of the response. If you
* supply both, DeveloperUserIdentifier
will be matched against IdentityID
. If the values
* are verified against the database, the response returns both values and is the same as the request. Otherwise a
* ResourceConflictException
is thrown.
*
*
* LookupDeveloperIdentity
is intended for low-throughput control plane operations: for example, to
* enable customer service to locate an identity ID by username. If you are using it for higher-volume operations
* such as user authentication, your requests are likely to be throttled. GetOpenIdTokenForDeveloperIdentity
* is a better option for higher-volume operations for user authentication.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param lookupDeveloperIdentityRequest
* Input to the LookupDeveloperIdentityInput
action.
* @return Result of the LookupDeveloperIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.LookupDeveloperIdentity
* @see AWS API Documentation
*/
LookupDeveloperIdentityResult lookupDeveloperIdentity(LookupDeveloperIdentityRequest lookupDeveloperIdentityRequest);
/**
*
* Merges two users having different IdentityId
s, existing in the same identity pool, and identified by
* the same developer provider. You can use this action to request that discrete users be merged and identified as a
* single user in the Cognito environment. Cognito associates the given source user (
* SourceUserIdentifier
) with the IdentityId
of the DestinationUserIdentifier
* . Only developer-authenticated users can be merged. If the users to be merged are associated with the same public
* provider, but as two different users, an exception will be thrown.
*
*
* The number of linked logins is limited to 20. So, the number of linked logins for the source user,
* SourceUserIdentifier
, and the destination user, DestinationUserIdentifier
, together
* should not be larger than 20. Otherwise, an exception will be thrown.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param mergeDeveloperIdentitiesRequest
* Input to the MergeDeveloperIdentities
action.
* @return Result of the MergeDeveloperIdentities operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.MergeDeveloperIdentities
* @see AWS API Documentation
*/
MergeDeveloperIdentitiesResult mergeDeveloperIdentities(MergeDeveloperIdentitiesRequest mergeDeveloperIdentitiesRequest);
/**
*
* Sets the roles for an identity pool. These roles are used when making calls to GetCredentialsForIdentity
* action.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param setIdentityPoolRolesRequest
* Input to the SetIdentityPoolRoles
action.
* @return Result of the SetIdentityPoolRoles operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ConcurrentModificationException
* Thrown if there are parallel requests to modify a resource.
* @sample AmazonCognitoIdentity.SetIdentityPoolRoles
* @see AWS API Documentation
*/
SetIdentityPoolRolesResult setIdentityPoolRoles(SetIdentityPoolRolesRequest setIdentityPoolRolesRequest);
/**
*
* You can use this operation to use default (username and clientID) attribute or custom attribute mappings.
*
*
* @param setPrincipalTagAttributeMapRequest
* @return Result of the SetPrincipalTagAttributeMap operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.SetPrincipalTagAttributeMap
* @see AWS API Documentation
*/
SetPrincipalTagAttributeMapResult setPrincipalTagAttributeMap(SetPrincipalTagAttributeMapRequest setPrincipalTagAttributeMapRequest);
/**
*
* Assigns a set of tags to the specified Amazon Cognito identity pool. A tag is a label that you can use to
* categorize and manage identity pools in different ways, such as by purpose, owner, environment, or other
* criteria.
*
*
* Each tag consists of a key and value, both of which you define. A key is a general category for more specific
* values. For example, if you have two versions of an identity pool, one for testing and another for production,
* you might assign an Environment
tag key to both identity pools. The value of this key might be
* Test
for one identity pool and Production
for the other.
*
*
* Tags are useful for cost tracking and access control. You can activate your tags so that they appear on the
* Billing and Cost Management console, where you can track the costs associated with your identity pools. In an IAM
* policy, you can constrain permissions for identity pools based on specific tags or tag values.
*
*
* You can use this action up to 5 times per second, per account. An identity pool can have as many as 50 tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Unlinks a DeveloperUserIdentifier
from an existing identity. Unlinked developer users will be
* considered new identities next time they are seen. If, for a given Cognito identity, you remove all federated
* identities as well as the developer user identifier, the Cognito identity becomes inaccessible.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param unlinkDeveloperIdentityRequest
* Input to the UnlinkDeveloperIdentity
action.
* @return Result of the UnlinkDeveloperIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.UnlinkDeveloperIdentity
* @see AWS API Documentation
*/
UnlinkDeveloperIdentityResult unlinkDeveloperIdentity(UnlinkDeveloperIdentityRequest unlinkDeveloperIdentityRequest);
/**
*
* Unlinks a federated identity from an existing account. Unlinked logins will be considered new identities next
* time they are seen. Removing the last linked login will make this identity inaccessible.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param unlinkIdentityRequest
* Input to the UnlinkIdentity action.
* @return Result of the UnlinkIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @sample AmazonCognitoIdentity.UnlinkIdentity
* @see AWS API Documentation
*/
UnlinkIdentityResult unlinkIdentity(UnlinkIdentityRequest unlinkIdentityRequest);
/**
*
* Removes the specified tags from the specified Amazon Cognito identity pool. You can use this action up to 5 times
* per second, per account
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @sample AmazonCognitoIdentity.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an identity pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param updateIdentityPoolRequest
* An object representing an Amazon Cognito identity pool.
* @return Result of the UpdateIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ConcurrentModificationException
* Thrown if there are parallel requests to modify a resource.
* @throws LimitExceededException
* Thrown when the total number of user pools has exceeded a preset limit.
* @sample AmazonCognitoIdentity.UpdateIdentityPool
* @see AWS API Documentation
*/
UpdateIdentityPoolResult updateIdentityPool(UpdateIdentityPoolRequest updateIdentityPoolRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}