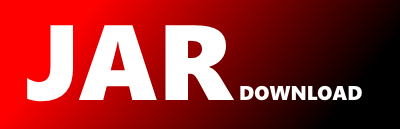
com.amazonaws.services.cognitoidentity.model.UpdateIdentityPoolRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cognitoidentity Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidentity.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* An object representing an Amazon Cognito identity pool.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateIdentityPoolRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* An identity pool ID in the format REGION:GUID.
*
*/
private String identityPoolId;
/**
*
* A string that you provide.
*
*/
private String identityPoolName;
/**
*
* TRUE if the identity pool supports unauthenticated logins.
*
*/
private Boolean allowUnauthenticatedIdentities;
/**
*
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*
*/
private Boolean allowClassicFlow;
/**
*
* Optional key:value pairs mapping provider names to provider app IDs.
*
*/
private java.util.Map supportedLoginProviders;
/**
*
* The "domain" by which Cognito will refer to your users.
*
*/
private String developerProviderName;
/**
*
* The ARNs of the OpenID Connect providers.
*
*/
private java.util.List openIdConnectProviderARNs;
/**
*
* A list representing an Amazon Cognito user pool and its client ID.
*
*/
private java.util.List cognitoIdentityProviders;
/**
*
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*
*/
private java.util.List samlProviderARNs;
/**
*
* The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
*
*/
private java.util.Map identityPoolTags;
/**
*
* An identity pool ID in the format REGION:GUID.
*
*
* @param identityPoolId
* An identity pool ID in the format REGION:GUID.
*/
public void setIdentityPoolId(String identityPoolId) {
this.identityPoolId = identityPoolId;
}
/**
*
* An identity pool ID in the format REGION:GUID.
*
*
* @return An identity pool ID in the format REGION:GUID.
*/
public String getIdentityPoolId() {
return this.identityPoolId;
}
/**
*
* An identity pool ID in the format REGION:GUID.
*
*
* @param identityPoolId
* An identity pool ID in the format REGION:GUID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withIdentityPoolId(String identityPoolId) {
setIdentityPoolId(identityPoolId);
return this;
}
/**
*
* A string that you provide.
*
*
* @param identityPoolName
* A string that you provide.
*/
public void setIdentityPoolName(String identityPoolName) {
this.identityPoolName = identityPoolName;
}
/**
*
* A string that you provide.
*
*
* @return A string that you provide.
*/
public String getIdentityPoolName() {
return this.identityPoolName;
}
/**
*
* A string that you provide.
*
*
* @param identityPoolName
* A string that you provide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withIdentityPoolName(String identityPoolName) {
setIdentityPoolName(identityPoolName);
return this;
}
/**
*
* TRUE if the identity pool supports unauthenticated logins.
*
*
* @param allowUnauthenticatedIdentities
* TRUE if the identity pool supports unauthenticated logins.
*/
public void setAllowUnauthenticatedIdentities(Boolean allowUnauthenticatedIdentities) {
this.allowUnauthenticatedIdentities = allowUnauthenticatedIdentities;
}
/**
*
* TRUE if the identity pool supports unauthenticated logins.
*
*
* @return TRUE if the identity pool supports unauthenticated logins.
*/
public Boolean getAllowUnauthenticatedIdentities() {
return this.allowUnauthenticatedIdentities;
}
/**
*
* TRUE if the identity pool supports unauthenticated logins.
*
*
* @param allowUnauthenticatedIdentities
* TRUE if the identity pool supports unauthenticated logins.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withAllowUnauthenticatedIdentities(Boolean allowUnauthenticatedIdentities) {
setAllowUnauthenticatedIdentities(allowUnauthenticatedIdentities);
return this;
}
/**
*
* TRUE if the identity pool supports unauthenticated logins.
*
*
* @return TRUE if the identity pool supports unauthenticated logins.
*/
public Boolean isAllowUnauthenticatedIdentities() {
return this.allowUnauthenticatedIdentities;
}
/**
*
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*
*
* @param allowClassicFlow
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*/
public void setAllowClassicFlow(Boolean allowClassicFlow) {
this.allowClassicFlow = allowClassicFlow;
}
/**
*
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*
*
* @return Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*/
public Boolean getAllowClassicFlow() {
return this.allowClassicFlow;
}
/**
*
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*
*
* @param allowClassicFlow
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withAllowClassicFlow(Boolean allowClassicFlow) {
setAllowClassicFlow(allowClassicFlow);
return this;
}
/**
*
* Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*
*
* @return Enables or disables the Basic (Classic) authentication flow. For more information, see Identity Pools
* (Federated Identities) Authentication Flow in the Amazon Cognito Developer Guide.
*/
public Boolean isAllowClassicFlow() {
return this.allowClassicFlow;
}
/**
*
* Optional key:value pairs mapping provider names to provider app IDs.
*
*
* @return Optional key:value pairs mapping provider names to provider app IDs.
*/
public java.util.Map getSupportedLoginProviders() {
return supportedLoginProviders;
}
/**
*
* Optional key:value pairs mapping provider names to provider app IDs.
*
*
* @param supportedLoginProviders
* Optional key:value pairs mapping provider names to provider app IDs.
*/
public void setSupportedLoginProviders(java.util.Map supportedLoginProviders) {
this.supportedLoginProviders = supportedLoginProviders;
}
/**
*
* Optional key:value pairs mapping provider names to provider app IDs.
*
*
* @param supportedLoginProviders
* Optional key:value pairs mapping provider names to provider app IDs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withSupportedLoginProviders(java.util.Map supportedLoginProviders) {
setSupportedLoginProviders(supportedLoginProviders);
return this;
}
/**
* Add a single SupportedLoginProviders entry
*
* @see UpdateIdentityPoolRequest#withSupportedLoginProviders
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest addSupportedLoginProvidersEntry(String key, String value) {
if (null == this.supportedLoginProviders) {
this.supportedLoginProviders = new java.util.HashMap();
}
if (this.supportedLoginProviders.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.supportedLoginProviders.put(key, value);
return this;
}
/**
* Removes all the entries added into SupportedLoginProviders.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest clearSupportedLoginProvidersEntries() {
this.supportedLoginProviders = null;
return this;
}
/**
*
* The "domain" by which Cognito will refer to your users.
*
*
* @param developerProviderName
* The "domain" by which Cognito will refer to your users.
*/
public void setDeveloperProviderName(String developerProviderName) {
this.developerProviderName = developerProviderName;
}
/**
*
* The "domain" by which Cognito will refer to your users.
*
*
* @return The "domain" by which Cognito will refer to your users.
*/
public String getDeveloperProviderName() {
return this.developerProviderName;
}
/**
*
* The "domain" by which Cognito will refer to your users.
*
*
* @param developerProviderName
* The "domain" by which Cognito will refer to your users.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withDeveloperProviderName(String developerProviderName) {
setDeveloperProviderName(developerProviderName);
return this;
}
/**
*
* The ARNs of the OpenID Connect providers.
*
*
* @return The ARNs of the OpenID Connect providers.
*/
public java.util.List getOpenIdConnectProviderARNs() {
return openIdConnectProviderARNs;
}
/**
*
* The ARNs of the OpenID Connect providers.
*
*
* @param openIdConnectProviderARNs
* The ARNs of the OpenID Connect providers.
*/
public void setOpenIdConnectProviderARNs(java.util.Collection openIdConnectProviderARNs) {
if (openIdConnectProviderARNs == null) {
this.openIdConnectProviderARNs = null;
return;
}
this.openIdConnectProviderARNs = new java.util.ArrayList(openIdConnectProviderARNs);
}
/**
*
* The ARNs of the OpenID Connect providers.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOpenIdConnectProviderARNs(java.util.Collection)} or
* {@link #withOpenIdConnectProviderARNs(java.util.Collection)} if you want to override the existing values.
*
*
* @param openIdConnectProviderARNs
* The ARNs of the OpenID Connect providers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withOpenIdConnectProviderARNs(String... openIdConnectProviderARNs) {
if (this.openIdConnectProviderARNs == null) {
setOpenIdConnectProviderARNs(new java.util.ArrayList(openIdConnectProviderARNs.length));
}
for (String ele : openIdConnectProviderARNs) {
this.openIdConnectProviderARNs.add(ele);
}
return this;
}
/**
*
* The ARNs of the OpenID Connect providers.
*
*
* @param openIdConnectProviderARNs
* The ARNs of the OpenID Connect providers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withOpenIdConnectProviderARNs(java.util.Collection openIdConnectProviderARNs) {
setOpenIdConnectProviderARNs(openIdConnectProviderARNs);
return this;
}
/**
*
* A list representing an Amazon Cognito user pool and its client ID.
*
*
* @return A list representing an Amazon Cognito user pool and its client ID.
*/
public java.util.List getCognitoIdentityProviders() {
return cognitoIdentityProviders;
}
/**
*
* A list representing an Amazon Cognito user pool and its client ID.
*
*
* @param cognitoIdentityProviders
* A list representing an Amazon Cognito user pool and its client ID.
*/
public void setCognitoIdentityProviders(java.util.Collection cognitoIdentityProviders) {
if (cognitoIdentityProviders == null) {
this.cognitoIdentityProviders = null;
return;
}
this.cognitoIdentityProviders = new java.util.ArrayList(cognitoIdentityProviders);
}
/**
*
* A list representing an Amazon Cognito user pool and its client ID.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCognitoIdentityProviders(java.util.Collection)} or
* {@link #withCognitoIdentityProviders(java.util.Collection)} if you want to override the existing values.
*
*
* @param cognitoIdentityProviders
* A list representing an Amazon Cognito user pool and its client ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withCognitoIdentityProviders(CognitoIdentityProvider... cognitoIdentityProviders) {
if (this.cognitoIdentityProviders == null) {
setCognitoIdentityProviders(new java.util.ArrayList(cognitoIdentityProviders.length));
}
for (CognitoIdentityProvider ele : cognitoIdentityProviders) {
this.cognitoIdentityProviders.add(ele);
}
return this;
}
/**
*
* A list representing an Amazon Cognito user pool and its client ID.
*
*
* @param cognitoIdentityProviders
* A list representing an Amazon Cognito user pool and its client ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withCognitoIdentityProviders(java.util.Collection cognitoIdentityProviders) {
setCognitoIdentityProviders(cognitoIdentityProviders);
return this;
}
/**
*
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*
*
* @return An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*/
public java.util.List getSamlProviderARNs() {
return samlProviderARNs;
}
/**
*
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*
*
* @param samlProviderARNs
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*/
public void setSamlProviderARNs(java.util.Collection samlProviderARNs) {
if (samlProviderARNs == null) {
this.samlProviderARNs = null;
return;
}
this.samlProviderARNs = new java.util.ArrayList(samlProviderARNs);
}
/**
*
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSamlProviderARNs(java.util.Collection)} or {@link #withSamlProviderARNs(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param samlProviderARNs
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withSamlProviderARNs(String... samlProviderARNs) {
if (this.samlProviderARNs == null) {
setSamlProviderARNs(new java.util.ArrayList(samlProviderARNs.length));
}
for (String ele : samlProviderARNs) {
this.samlProviderARNs.add(ele);
}
return this;
}
/**
*
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
*
*
* @param samlProviderARNs
* An array of Amazon Resource Names (ARNs) of the SAML provider for your identity pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withSamlProviderARNs(java.util.Collection samlProviderARNs) {
setSamlProviderARNs(samlProviderARNs);
return this;
}
/**
*
* The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
*
*
* @return The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
*/
public java.util.Map getIdentityPoolTags() {
return identityPoolTags;
}
/**
*
* The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
*
*
* @param identityPoolTags
* The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
*/
public void setIdentityPoolTags(java.util.Map identityPoolTags) {
this.identityPoolTags = identityPoolTags;
}
/**
*
* The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
*
*
* @param identityPoolTags
* The tags that are assigned to the identity pool. A tag is a label that you can apply to identity pools to
* categorize and manage them in different ways, such as by purpose, owner, environment, or other criteria.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest withIdentityPoolTags(java.util.Map identityPoolTags) {
setIdentityPoolTags(identityPoolTags);
return this;
}
/**
* Add a single IdentityPoolTags entry
*
* @see UpdateIdentityPoolRequest#withIdentityPoolTags
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest addIdentityPoolTagsEntry(String key, String value) {
if (null == this.identityPoolTags) {
this.identityPoolTags = new java.util.HashMap();
}
if (this.identityPoolTags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.identityPoolTags.put(key, value);
return this;
}
/**
* Removes all the entries added into IdentityPoolTags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateIdentityPoolRequest clearIdentityPoolTagsEntries() {
this.identityPoolTags = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIdentityPoolId() != null)
sb.append("IdentityPoolId: ").append(getIdentityPoolId()).append(",");
if (getIdentityPoolName() != null)
sb.append("IdentityPoolName: ").append(getIdentityPoolName()).append(",");
if (getAllowUnauthenticatedIdentities() != null)
sb.append("AllowUnauthenticatedIdentities: ").append(getAllowUnauthenticatedIdentities()).append(",");
if (getAllowClassicFlow() != null)
sb.append("AllowClassicFlow: ").append(getAllowClassicFlow()).append(",");
if (getSupportedLoginProviders() != null)
sb.append("SupportedLoginProviders: ").append(getSupportedLoginProviders()).append(",");
if (getDeveloperProviderName() != null)
sb.append("DeveloperProviderName: ").append(getDeveloperProviderName()).append(",");
if (getOpenIdConnectProviderARNs() != null)
sb.append("OpenIdConnectProviderARNs: ").append(getOpenIdConnectProviderARNs()).append(",");
if (getCognitoIdentityProviders() != null)
sb.append("CognitoIdentityProviders: ").append(getCognitoIdentityProviders()).append(",");
if (getSamlProviderARNs() != null)
sb.append("SamlProviderARNs: ").append(getSamlProviderARNs()).append(",");
if (getIdentityPoolTags() != null)
sb.append("IdentityPoolTags: ").append(getIdentityPoolTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateIdentityPoolRequest == false)
return false;
UpdateIdentityPoolRequest other = (UpdateIdentityPoolRequest) obj;
if (other.getIdentityPoolId() == null ^ this.getIdentityPoolId() == null)
return false;
if (other.getIdentityPoolId() != null && other.getIdentityPoolId().equals(this.getIdentityPoolId()) == false)
return false;
if (other.getIdentityPoolName() == null ^ this.getIdentityPoolName() == null)
return false;
if (other.getIdentityPoolName() != null && other.getIdentityPoolName().equals(this.getIdentityPoolName()) == false)
return false;
if (other.getAllowUnauthenticatedIdentities() == null ^ this.getAllowUnauthenticatedIdentities() == null)
return false;
if (other.getAllowUnauthenticatedIdentities() != null
&& other.getAllowUnauthenticatedIdentities().equals(this.getAllowUnauthenticatedIdentities()) == false)
return false;
if (other.getAllowClassicFlow() == null ^ this.getAllowClassicFlow() == null)
return false;
if (other.getAllowClassicFlow() != null && other.getAllowClassicFlow().equals(this.getAllowClassicFlow()) == false)
return false;
if (other.getSupportedLoginProviders() == null ^ this.getSupportedLoginProviders() == null)
return false;
if (other.getSupportedLoginProviders() != null && other.getSupportedLoginProviders().equals(this.getSupportedLoginProviders()) == false)
return false;
if (other.getDeveloperProviderName() == null ^ this.getDeveloperProviderName() == null)
return false;
if (other.getDeveloperProviderName() != null && other.getDeveloperProviderName().equals(this.getDeveloperProviderName()) == false)
return false;
if (other.getOpenIdConnectProviderARNs() == null ^ this.getOpenIdConnectProviderARNs() == null)
return false;
if (other.getOpenIdConnectProviderARNs() != null && other.getOpenIdConnectProviderARNs().equals(this.getOpenIdConnectProviderARNs()) == false)
return false;
if (other.getCognitoIdentityProviders() == null ^ this.getCognitoIdentityProviders() == null)
return false;
if (other.getCognitoIdentityProviders() != null && other.getCognitoIdentityProviders().equals(this.getCognitoIdentityProviders()) == false)
return false;
if (other.getSamlProviderARNs() == null ^ this.getSamlProviderARNs() == null)
return false;
if (other.getSamlProviderARNs() != null && other.getSamlProviderARNs().equals(this.getSamlProviderARNs()) == false)
return false;
if (other.getIdentityPoolTags() == null ^ this.getIdentityPoolTags() == null)
return false;
if (other.getIdentityPoolTags() != null && other.getIdentityPoolTags().equals(this.getIdentityPoolTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIdentityPoolId() == null) ? 0 : getIdentityPoolId().hashCode());
hashCode = prime * hashCode + ((getIdentityPoolName() == null) ? 0 : getIdentityPoolName().hashCode());
hashCode = prime * hashCode + ((getAllowUnauthenticatedIdentities() == null) ? 0 : getAllowUnauthenticatedIdentities().hashCode());
hashCode = prime * hashCode + ((getAllowClassicFlow() == null) ? 0 : getAllowClassicFlow().hashCode());
hashCode = prime * hashCode + ((getSupportedLoginProviders() == null) ? 0 : getSupportedLoginProviders().hashCode());
hashCode = prime * hashCode + ((getDeveloperProviderName() == null) ? 0 : getDeveloperProviderName().hashCode());
hashCode = prime * hashCode + ((getOpenIdConnectProviderARNs() == null) ? 0 : getOpenIdConnectProviderARNs().hashCode());
hashCode = prime * hashCode + ((getCognitoIdentityProviders() == null) ? 0 : getCognitoIdentityProviders().hashCode());
hashCode = prime * hashCode + ((getSamlProviderARNs() == null) ? 0 : getSamlProviderARNs().hashCode());
hashCode = prime * hashCode + ((getIdentityPoolTags() == null) ? 0 : getIdentityPoolTags().hashCode());
return hashCode;
}
@Override
public UpdateIdentityPoolRequest clone() {
return (UpdateIdentityPoolRequest) super.clone();
}
}