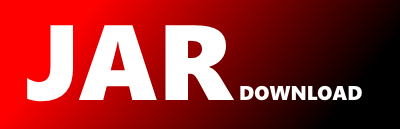
com.amazonaws.services.cognitoidp.model.CreateUserPoolRequest Maven / Gradle / Ivy
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cognitoidp.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the request to create a user pool.
*
*/
public class CreateUserPoolRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* A string used to name the user pool.
*
*/
private String poolName;
/**
*
* The policies associated with the new user pool.
*
*/
private UserPoolPolicyType policies;
/**
*
* The Lambda trigger configuration information for the new user pool.
*
*/
private LambdaConfigType lambdaConfig;
/**
*
* The attributes to be auto-verified. Possible values: email,
* phone_number.
*
*/
private java.util.List autoVerifiedAttributes;
/**
*
* Attributes supported as an alias for this user pool. Possible values:
* phone_number, email, or preferred_username.
*
*/
private java.util.List aliasAttributes;
/**
*
* A string representing the SMS verification message.
*
*/
private String smsVerificationMessage;
/**
*
* A string representing the email verification message.
*
*/
private String emailVerificationMessage;
/**
*
* A string representing the email verification subject.
*
*/
private String emailVerificationSubject;
/**
*
* A string representing the SMS authentication message.
*
*/
private String smsAuthenticationMessage;
/**
*
* Specifies MFA configuration details.
*
*/
private String mfaConfiguration;
/**
*
* A string used to name the user pool.
*
*
* @param poolName
* A string used to name the user pool.
*/
public void setPoolName(String poolName) {
this.poolName = poolName;
}
/**
*
* A string used to name the user pool.
*
*
* @return A string used to name the user pool.
*/
public String getPoolName() {
return this.poolName;
}
/**
*
* A string used to name the user pool.
*
*
* @param poolName
* A string used to name the user pool.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withPoolName(String poolName) {
setPoolName(poolName);
return this;
}
/**
*
* The policies associated with the new user pool.
*
*
* @param policies
* The policies associated with the new user pool.
*/
public void setPolicies(UserPoolPolicyType policies) {
this.policies = policies;
}
/**
*
* The policies associated with the new user pool.
*
*
* @return The policies associated with the new user pool.
*/
public UserPoolPolicyType getPolicies() {
return this.policies;
}
/**
*
* The policies associated with the new user pool.
*
*
* @param policies
* The policies associated with the new user pool.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withPolicies(UserPoolPolicyType policies) {
setPolicies(policies);
return this;
}
/**
*
* The Lambda trigger configuration information for the new user pool.
*
*
* @param lambdaConfig
* The Lambda trigger configuration information for the new user
* pool.
*/
public void setLambdaConfig(LambdaConfigType lambdaConfig) {
this.lambdaConfig = lambdaConfig;
}
/**
*
* The Lambda trigger configuration information for the new user pool.
*
*
* @return The Lambda trigger configuration information for the new user
* pool.
*/
public LambdaConfigType getLambdaConfig() {
return this.lambdaConfig;
}
/**
*
* The Lambda trigger configuration information for the new user pool.
*
*
* @param lambdaConfig
* The Lambda trigger configuration information for the new user
* pool.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withLambdaConfig(LambdaConfigType lambdaConfig) {
setLambdaConfig(lambdaConfig);
return this;
}
/**
*
* The attributes to be auto-verified. Possible values: email,
* phone_number.
*
*
* @return The attributes to be auto-verified. Possible values:
* email, phone_number.
* @see VerifiedAttributeType
*/
public java.util.List getAutoVerifiedAttributes() {
return autoVerifiedAttributes;
}
/**
*
* The attributes to be auto-verified. Possible values: email,
* phone_number.
*
*
* @param autoVerifiedAttributes
* The attributes to be auto-verified. Possible values: email,
* phone_number.
* @see VerifiedAttributeType
*/
public void setAutoVerifiedAttributes(
java.util.Collection autoVerifiedAttributes) {
if (autoVerifiedAttributes == null) {
this.autoVerifiedAttributes = null;
return;
}
this.autoVerifiedAttributes = new java.util.ArrayList(
autoVerifiedAttributes);
}
/**
*
* The attributes to be auto-verified. Possible values: email,
* phone_number.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setAutoVerifiedAttributes(java.util.Collection)} or
* {@link #withAutoVerifiedAttributes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param autoVerifiedAttributes
* The attributes to be auto-verified. Possible values: email,
* phone_number.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see VerifiedAttributeType
*/
public CreateUserPoolRequest withAutoVerifiedAttributes(
String... autoVerifiedAttributes) {
if (this.autoVerifiedAttributes == null) {
setAutoVerifiedAttributes(new java.util.ArrayList(
autoVerifiedAttributes.length));
}
for (String ele : autoVerifiedAttributes) {
this.autoVerifiedAttributes.add(ele);
}
return this;
}
/**
*
* The attributes to be auto-verified. Possible values: email,
* phone_number.
*
*
* @param autoVerifiedAttributes
* The attributes to be auto-verified. Possible values: email,
* phone_number.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see VerifiedAttributeType
*/
public CreateUserPoolRequest withAutoVerifiedAttributes(
java.util.Collection autoVerifiedAttributes) {
setAutoVerifiedAttributes(autoVerifiedAttributes);
return this;
}
/**
*
* The attributes to be auto-verified. Possible values: email,
* phone_number.
*
*
* @param autoVerifiedAttributes
* The attributes to be auto-verified. Possible values: email,
* phone_number.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see VerifiedAttributeType
*/
public CreateUserPoolRequest withAutoVerifiedAttributes(
VerifiedAttributeType... autoVerifiedAttributes) {
java.util.ArrayList autoVerifiedAttributesCopy = new java.util.ArrayList(
autoVerifiedAttributes.length);
for (VerifiedAttributeType value : autoVerifiedAttributes) {
autoVerifiedAttributesCopy.add(value.toString());
}
if (getAutoVerifiedAttributes() == null) {
setAutoVerifiedAttributes(autoVerifiedAttributesCopy);
} else {
getAutoVerifiedAttributes().addAll(autoVerifiedAttributesCopy);
}
return this;
}
/**
*
* Attributes supported as an alias for this user pool. Possible values:
* phone_number, email, or preferred_username.
*
*
* @return Attributes supported as an alias for this user pool. Possible
* values: phone_number, email, or
* preferred_username.
* @see AliasAttributeType
*/
public java.util.List getAliasAttributes() {
return aliasAttributes;
}
/**
*
* Attributes supported as an alias for this user pool. Possible values:
* phone_number, email, or preferred_username.
*
*
* @param aliasAttributes
* Attributes supported as an alias for this user pool. Possible
* values: phone_number, email, or
* preferred_username.
* @see AliasAttributeType
*/
public void setAliasAttributes(java.util.Collection aliasAttributes) {
if (aliasAttributes == null) {
this.aliasAttributes = null;
return;
}
this.aliasAttributes = new java.util.ArrayList(aliasAttributes);
}
/**
*
* Attributes supported as an alias for this user pool. Possible values:
* phone_number, email, or preferred_username.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setAliasAttributes(java.util.Collection)} or
* {@link #withAliasAttributes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param aliasAttributes
* Attributes supported as an alias for this user pool. Possible
* values: phone_number, email, or
* preferred_username.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see AliasAttributeType
*/
public CreateUserPoolRequest withAliasAttributes(String... aliasAttributes) {
if (this.aliasAttributes == null) {
setAliasAttributes(new java.util.ArrayList(
aliasAttributes.length));
}
for (String ele : aliasAttributes) {
this.aliasAttributes.add(ele);
}
return this;
}
/**
*
* Attributes supported as an alias for this user pool. Possible values:
* phone_number, email, or preferred_username.
*
*
* @param aliasAttributes
* Attributes supported as an alias for this user pool. Possible
* values: phone_number, email, or
* preferred_username.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see AliasAttributeType
*/
public CreateUserPoolRequest withAliasAttributes(
java.util.Collection aliasAttributes) {
setAliasAttributes(aliasAttributes);
return this;
}
/**
*
* Attributes supported as an alias for this user pool. Possible values:
* phone_number, email, or preferred_username.
*
*
* @param aliasAttributes
* Attributes supported as an alias for this user pool. Possible
* values: phone_number, email, or
* preferred_username.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see AliasAttributeType
*/
public CreateUserPoolRequest withAliasAttributes(
AliasAttributeType... aliasAttributes) {
java.util.ArrayList aliasAttributesCopy = new java.util.ArrayList(
aliasAttributes.length);
for (AliasAttributeType value : aliasAttributes) {
aliasAttributesCopy.add(value.toString());
}
if (getAliasAttributes() == null) {
setAliasAttributes(aliasAttributesCopy);
} else {
getAliasAttributes().addAll(aliasAttributesCopy);
}
return this;
}
/**
*
* A string representing the SMS verification message.
*
*
* @param smsVerificationMessage
* A string representing the SMS verification message.
*/
public void setSmsVerificationMessage(String smsVerificationMessage) {
this.smsVerificationMessage = smsVerificationMessage;
}
/**
*
* A string representing the SMS verification message.
*
*
* @return A string representing the SMS verification message.
*/
public String getSmsVerificationMessage() {
return this.smsVerificationMessage;
}
/**
*
* A string representing the SMS verification message.
*
*
* @param smsVerificationMessage
* A string representing the SMS verification message.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withSmsVerificationMessage(
String smsVerificationMessage) {
setSmsVerificationMessage(smsVerificationMessage);
return this;
}
/**
*
* A string representing the email verification message.
*
*
* @param emailVerificationMessage
* A string representing the email verification message.
*/
public void setEmailVerificationMessage(String emailVerificationMessage) {
this.emailVerificationMessage = emailVerificationMessage;
}
/**
*
* A string representing the email verification message.
*
*
* @return A string representing the email verification message.
*/
public String getEmailVerificationMessage() {
return this.emailVerificationMessage;
}
/**
*
* A string representing the email verification message.
*
*
* @param emailVerificationMessage
* A string representing the email verification message.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withEmailVerificationMessage(
String emailVerificationMessage) {
setEmailVerificationMessage(emailVerificationMessage);
return this;
}
/**
*
* A string representing the email verification subject.
*
*
* @param emailVerificationSubject
* A string representing the email verification subject.
*/
public void setEmailVerificationSubject(String emailVerificationSubject) {
this.emailVerificationSubject = emailVerificationSubject;
}
/**
*
* A string representing the email verification subject.
*
*
* @return A string representing the email verification subject.
*/
public String getEmailVerificationSubject() {
return this.emailVerificationSubject;
}
/**
*
* A string representing the email verification subject.
*
*
* @param emailVerificationSubject
* A string representing the email verification subject.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withEmailVerificationSubject(
String emailVerificationSubject) {
setEmailVerificationSubject(emailVerificationSubject);
return this;
}
/**
*
* A string representing the SMS authentication message.
*
*
* @param smsAuthenticationMessage
* A string representing the SMS authentication message.
*/
public void setSmsAuthenticationMessage(String smsAuthenticationMessage) {
this.smsAuthenticationMessage = smsAuthenticationMessage;
}
/**
*
* A string representing the SMS authentication message.
*
*
* @return A string representing the SMS authentication message.
*/
public String getSmsAuthenticationMessage() {
return this.smsAuthenticationMessage;
}
/**
*
* A string representing the SMS authentication message.
*
*
* @param smsAuthenticationMessage
* A string representing the SMS authentication message.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateUserPoolRequest withSmsAuthenticationMessage(
String smsAuthenticationMessage) {
setSmsAuthenticationMessage(smsAuthenticationMessage);
return this;
}
/**
*
* Specifies MFA configuration details.
*
*
* @param mfaConfiguration
* Specifies MFA configuration details.
* @see UserPoolMfaType
*/
public void setMfaConfiguration(String mfaConfiguration) {
this.mfaConfiguration = mfaConfiguration;
}
/**
*
* Specifies MFA configuration details.
*
*
* @return Specifies MFA configuration details.
* @see UserPoolMfaType
*/
public String getMfaConfiguration() {
return this.mfaConfiguration;
}
/**
*
* Specifies MFA configuration details.
*
*
* @param mfaConfiguration
* Specifies MFA configuration details.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see UserPoolMfaType
*/
public CreateUserPoolRequest withMfaConfiguration(String mfaConfiguration) {
setMfaConfiguration(mfaConfiguration);
return this;
}
/**
*
* Specifies MFA configuration details.
*
*
* @param mfaConfiguration
* Specifies MFA configuration details.
* @see UserPoolMfaType
*/
public void setMfaConfiguration(UserPoolMfaType mfaConfiguration) {
this.mfaConfiguration = mfaConfiguration.toString();
}
/**
*
* Specifies MFA configuration details.
*
*
* @param mfaConfiguration
* Specifies MFA configuration details.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see UserPoolMfaType
*/
public CreateUserPoolRequest withMfaConfiguration(
UserPoolMfaType mfaConfiguration) {
setMfaConfiguration(mfaConfiguration);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPoolName() != null)
sb.append("PoolName: " + getPoolName() + ",");
if (getPolicies() != null)
sb.append("Policies: " + getPolicies() + ",");
if (getLambdaConfig() != null)
sb.append("LambdaConfig: " + getLambdaConfig() + ",");
if (getAutoVerifiedAttributes() != null)
sb.append("AutoVerifiedAttributes: " + getAutoVerifiedAttributes()
+ ",");
if (getAliasAttributes() != null)
sb.append("AliasAttributes: " + getAliasAttributes() + ",");
if (getSmsVerificationMessage() != null)
sb.append("SmsVerificationMessage: " + getSmsVerificationMessage()
+ ",");
if (getEmailVerificationMessage() != null)
sb.append("EmailVerificationMessage: "
+ getEmailVerificationMessage() + ",");
if (getEmailVerificationSubject() != null)
sb.append("EmailVerificationSubject: "
+ getEmailVerificationSubject() + ",");
if (getSmsAuthenticationMessage() != null)
sb.append("SmsAuthenticationMessage: "
+ getSmsAuthenticationMessage() + ",");
if (getMfaConfiguration() != null)
sb.append("MfaConfiguration: " + getMfaConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateUserPoolRequest == false)
return false;
CreateUserPoolRequest other = (CreateUserPoolRequest) obj;
if (other.getPoolName() == null ^ this.getPoolName() == null)
return false;
if (other.getPoolName() != null
&& other.getPoolName().equals(this.getPoolName()) == false)
return false;
if (other.getPolicies() == null ^ this.getPolicies() == null)
return false;
if (other.getPolicies() != null
&& other.getPolicies().equals(this.getPolicies()) == false)
return false;
if (other.getLambdaConfig() == null ^ this.getLambdaConfig() == null)
return false;
if (other.getLambdaConfig() != null
&& other.getLambdaConfig().equals(this.getLambdaConfig()) == false)
return false;
if (other.getAutoVerifiedAttributes() == null
^ this.getAutoVerifiedAttributes() == null)
return false;
if (other.getAutoVerifiedAttributes() != null
&& other.getAutoVerifiedAttributes().equals(
this.getAutoVerifiedAttributes()) == false)
return false;
if (other.getAliasAttributes() == null
^ this.getAliasAttributes() == null)
return false;
if (other.getAliasAttributes() != null
&& other.getAliasAttributes().equals(this.getAliasAttributes()) == false)
return false;
if (other.getSmsVerificationMessage() == null
^ this.getSmsVerificationMessage() == null)
return false;
if (other.getSmsVerificationMessage() != null
&& other.getSmsVerificationMessage().equals(
this.getSmsVerificationMessage()) == false)
return false;
if (other.getEmailVerificationMessage() == null
^ this.getEmailVerificationMessage() == null)
return false;
if (other.getEmailVerificationMessage() != null
&& other.getEmailVerificationMessage().equals(
this.getEmailVerificationMessage()) == false)
return false;
if (other.getEmailVerificationSubject() == null
^ this.getEmailVerificationSubject() == null)
return false;
if (other.getEmailVerificationSubject() != null
&& other.getEmailVerificationSubject().equals(
this.getEmailVerificationSubject()) == false)
return false;
if (other.getSmsAuthenticationMessage() == null
^ this.getSmsAuthenticationMessage() == null)
return false;
if (other.getSmsAuthenticationMessage() != null
&& other.getSmsAuthenticationMessage().equals(
this.getSmsAuthenticationMessage()) == false)
return false;
if (other.getMfaConfiguration() == null
^ this.getMfaConfiguration() == null)
return false;
if (other.getMfaConfiguration() != null
&& other.getMfaConfiguration().equals(
this.getMfaConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getPoolName() == null) ? 0 : getPoolName().hashCode());
hashCode = prime * hashCode
+ ((getPolicies() == null) ? 0 : getPolicies().hashCode());
hashCode = prime
* hashCode
+ ((getLambdaConfig() == null) ? 0 : getLambdaConfig()
.hashCode());
hashCode = prime
* hashCode
+ ((getAutoVerifiedAttributes() == null) ? 0
: getAutoVerifiedAttributes().hashCode());
hashCode = prime
* hashCode
+ ((getAliasAttributes() == null) ? 0 : getAliasAttributes()
.hashCode());
hashCode = prime
* hashCode
+ ((getSmsVerificationMessage() == null) ? 0
: getSmsVerificationMessage().hashCode());
hashCode = prime
* hashCode
+ ((getEmailVerificationMessage() == null) ? 0
: getEmailVerificationMessage().hashCode());
hashCode = prime
* hashCode
+ ((getEmailVerificationSubject() == null) ? 0
: getEmailVerificationSubject().hashCode());
hashCode = prime
* hashCode
+ ((getSmsAuthenticationMessage() == null) ? 0
: getSmsAuthenticationMessage().hashCode());
hashCode = prime
* hashCode
+ ((getMfaConfiguration() == null) ? 0 : getMfaConfiguration()
.hashCode());
return hashCode;
}
@Override
public CreateUserPoolRequest clone() {
return (CreateUserPoolRequest) super.clone();
}
}