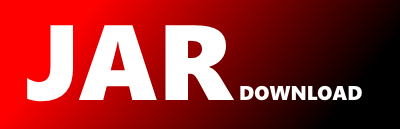
com.amazonaws.services.cognitoidp.model.ListUsersRequest Maven / Gradle / Ivy
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cognitoidp.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the request to list users.
*
*/
public class ListUsersRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* The user pool ID for which you want to list users.
*
*/
private String userPoolId;
/**
*
* The attributes to get from the request to list users.
*
*/
private java.util.List attributesToGet;
/**
*
* The limit of the request to list users.
*
*/
private Integer limit;
/**
*
* An identifier that was returned from the previous call to this operation,
* which can be used to return the next set of items in the list.
*
*/
private String paginationToken;
/**
*
* The user status. Can be one of the following:
*
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security threat.
* - UNKNOWN - User status is not known.
*
*/
private String userStatus;
/**
*
* The user pool ID for which you want to list users.
*
*
* @param userPoolId
* The user pool ID for which you want to list users.
*/
public void setUserPoolId(String userPoolId) {
this.userPoolId = userPoolId;
}
/**
*
* The user pool ID for which you want to list users.
*
*
* @return The user pool ID for which you want to list users.
*/
public String getUserPoolId() {
return this.userPoolId;
}
/**
*
* The user pool ID for which you want to list users.
*
*
* @param userPoolId
* The user pool ID for which you want to list users.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ListUsersRequest withUserPoolId(String userPoolId) {
setUserPoolId(userPoolId);
return this;
}
/**
*
* The attributes to get from the request to list users.
*
*
* @return The attributes to get from the request to list users.
*/
public java.util.List getAttributesToGet() {
return attributesToGet;
}
/**
*
* The attributes to get from the request to list users.
*
*
* @param attributesToGet
* The attributes to get from the request to list users.
*/
public void setAttributesToGet(java.util.Collection attributesToGet) {
if (attributesToGet == null) {
this.attributesToGet = null;
return;
}
this.attributesToGet = new java.util.ArrayList(attributesToGet);
}
/**
*
* The attributes to get from the request to list users.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setAttributesToGet(java.util.Collection)} or
* {@link #withAttributesToGet(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param attributesToGet
* The attributes to get from the request to list users.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ListUsersRequest withAttributesToGet(String... attributesToGet) {
if (this.attributesToGet == null) {
setAttributesToGet(new java.util.ArrayList(
attributesToGet.length));
}
for (String ele : attributesToGet) {
this.attributesToGet.add(ele);
}
return this;
}
/**
*
* The attributes to get from the request to list users.
*
*
* @param attributesToGet
* The attributes to get from the request to list users.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ListUsersRequest withAttributesToGet(
java.util.Collection attributesToGet) {
setAttributesToGet(attributesToGet);
return this;
}
/**
*
* The limit of the request to list users.
*
*
* @param limit
* The limit of the request to list users.
*/
public void setLimit(Integer limit) {
this.limit = limit;
}
/**
*
* The limit of the request to list users.
*
*
* @return The limit of the request to list users.
*/
public Integer getLimit() {
return this.limit;
}
/**
*
* The limit of the request to list users.
*
*
* @param limit
* The limit of the request to list users.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ListUsersRequest withLimit(Integer limit) {
setLimit(limit);
return this;
}
/**
*
* An identifier that was returned from the previous call to this operation,
* which can be used to return the next set of items in the list.
*
*
* @param paginationToken
* An identifier that was returned from the previous call to this
* operation, which can be used to return the next set of items in
* the list.
*/
public void setPaginationToken(String paginationToken) {
this.paginationToken = paginationToken;
}
/**
*
* An identifier that was returned from the previous call to this operation,
* which can be used to return the next set of items in the list.
*
*
* @return An identifier that was returned from the previous call to this
* operation, which can be used to return the next set of items in
* the list.
*/
public String getPaginationToken() {
return this.paginationToken;
}
/**
*
* An identifier that was returned from the previous call to this operation,
* which can be used to return the next set of items in the list.
*
*
* @param paginationToken
* An identifier that was returned from the previous call to this
* operation, which can be used to return the next set of items in
* the list.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ListUsersRequest withPaginationToken(String paginationToken) {
setPaginationToken(paginationToken);
return this;
}
/**
*
* The user status. Can be one of the following:
*
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security threat.
* - UNKNOWN - User status is not known.
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security
* threat.
* - UNKNOWN - User status is not known.
* @see UserStatusType
*/
public void setUserStatus(String userStatus) {
this.userStatus = userStatus;
}
/**
*
* The user status. Can be one of the following:
*
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security threat.
* - UNKNOWN - User status is not known.
*
*
* @return The user status. Can be one of the following:
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security
* threat.
* - UNKNOWN - User status is not known.
* @see UserStatusType
*/
public String getUserStatus() {
return this.userStatus;
}
/**
*
* The user status. Can be one of the following:
*
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security threat.
* - UNKNOWN - User status is not known.
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security
* threat.
* - UNKNOWN - User status is not known.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see UserStatusType
*/
public ListUsersRequest withUserStatus(String userStatus) {
setUserStatus(userStatus);
return this;
}
/**
*
* The user status. Can be one of the following:
*
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security threat.
* - UNKNOWN - User status is not known.
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security
* threat.
* - UNKNOWN - User status is not known.
* @see UserStatusType
*/
public void setUserStatus(UserStatusType userStatus) {
this.userStatus = userStatus.toString();
}
/**
*
* The user status. Can be one of the following:
*
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security threat.
* - UNKNOWN - User status is not known.
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* - UNCONFIRMED - User has been created but not confirmed.
* - CONFIRMED - User has been confirmed.
* - ARCHIVED - User is no longer active.
* - COMPROMISED - User is disabled due to a potential security
* threat.
* - UNKNOWN - User status is not known.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see UserStatusType
*/
public ListUsersRequest withUserStatus(UserStatusType userStatus) {
setUserStatus(userStatus);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUserPoolId() != null)
sb.append("UserPoolId: " + getUserPoolId() + ",");
if (getAttributesToGet() != null)
sb.append("AttributesToGet: " + getAttributesToGet() + ",");
if (getLimit() != null)
sb.append("Limit: " + getLimit() + ",");
if (getPaginationToken() != null)
sb.append("PaginationToken: " + getPaginationToken() + ",");
if (getUserStatus() != null)
sb.append("UserStatus: " + getUserStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListUsersRequest == false)
return false;
ListUsersRequest other = (ListUsersRequest) obj;
if (other.getUserPoolId() == null ^ this.getUserPoolId() == null)
return false;
if (other.getUserPoolId() != null
&& other.getUserPoolId().equals(this.getUserPoolId()) == false)
return false;
if (other.getAttributesToGet() == null
^ this.getAttributesToGet() == null)
return false;
if (other.getAttributesToGet() != null
&& other.getAttributesToGet().equals(this.getAttributesToGet()) == false)
return false;
if (other.getLimit() == null ^ this.getLimit() == null)
return false;
if (other.getLimit() != null
&& other.getLimit().equals(this.getLimit()) == false)
return false;
if (other.getPaginationToken() == null
^ this.getPaginationToken() == null)
return false;
if (other.getPaginationToken() != null
&& other.getPaginationToken().equals(this.getPaginationToken()) == false)
return false;
if (other.getUserStatus() == null ^ this.getUserStatus() == null)
return false;
if (other.getUserStatus() != null
&& other.getUserStatus().equals(this.getUserStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getUserPoolId() == null) ? 0 : getUserPoolId().hashCode());
hashCode = prime
* hashCode
+ ((getAttributesToGet() == null) ? 0 : getAttributesToGet()
.hashCode());
hashCode = prime * hashCode
+ ((getLimit() == null) ? 0 : getLimit().hashCode());
hashCode = prime
* hashCode
+ ((getPaginationToken() == null) ? 0 : getPaginationToken()
.hashCode());
hashCode = prime * hashCode
+ ((getUserStatus() == null) ? 0 : getUserStatus().hashCode());
return hashCode;
}
@Override
public ListUsersRequest clone() {
return (ListUsersRequest) super.clone();
}
}