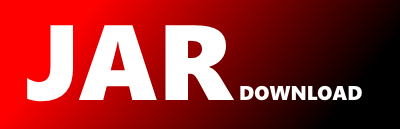
com.amazonaws.services.cognitoidp.model.AdminInitiateAuthResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cognitoidp Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidp.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Initiates the authentication response, as an administrator.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AdminInitiateAuthResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up are
* presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to continue to
* authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text SMS MFA,
* and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the user
* should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous challenges were
* passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful first
* login. Respond to this challenge with NEW_PASSWORD
and any required attributes that Amazon Cognito
* returned in the requiredAttributes
parameter. You can also set values for attributes that aren't
* required by your user pool and that your app client can write. For more information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that already
* has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon Cognito returned
* in the requiredAttributes
parameter, then use the AdminUpdateUserAttributes
API
* operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The MFA types
* activated for the user pool will be listed in the challenge parameters MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as an input
* to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete sign-in. To set up
* SMS MFA, users will need help from an administrator to add a phone number to their account and then call
* InitiateAuth
again to restart sign-in.
*
*
*
*/
private String challengeName;
/**
*
* The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the caller
* must pass another challenge, they return a session with other challenge parameters. This session should be passed
* as it is to the next AdminRespondToAuthChallenge
API call.
*
*/
private String session;
/**
*
* The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you must
* pass another challenge. The responses in this parameter should be used to compute inputs to the next call (
* AdminRespondToAuthChallenge
).
*
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such as
* email address or phone number), even if you specified an alias in your call to AdminInitiateAuth
.
* This happens because, in the AdminRespondToAuthChallenge
API ChallengeResponses
, the
* USERNAME
attribute can't be an alias.
*
*/
private java.util.Map challengeParameters;
/**
*
* The result of the authentication response. This is only returned if the caller doesn't need to pass another
* challenge. If the caller does need to pass another challenge before it gets tokens, ChallengeName
,
* ChallengeParameters
, and Session
are returned.
*
*/
private AuthenticationResultType authenticationResult;
/**
*
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up are
* presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to continue to
* authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text SMS MFA,
* and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the user
* should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous challenges were
* passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful first
* login. Respond to this challenge with NEW_PASSWORD
and any required attributes that Amazon Cognito
* returned in the requiredAttributes
parameter. You can also set values for attributes that aren't
* required by your user pool and that your app client can write. For more information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that already
* has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon Cognito returned
* in the requiredAttributes
parameter, then use the AdminUpdateUserAttributes
API
* operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The MFA types
* activated for the user pool will be listed in the challenge parameters MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as an input
* to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete sign-in. To set up
* SMS MFA, users will need help from an administrator to add a phone number to their account and then call
* InitiateAuth
again to restart sign-in.
*
*
*
*
* @param challengeName
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up
* are presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to
* continue to authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text
* SMS MFA, and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP
* calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the
* user should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous
* challenges were passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful
* first login. Respond to this challenge with NEW_PASSWORD
and any required attributes that
* Amazon Cognito returned in the requiredAttributes
parameter. You can also set values for
* attributes that aren't required by your user pool and that your app client can write. For more
* information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that
* already has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon
* Cognito returned in the requiredAttributes
parameter, then use the
* AdminUpdateUserAttributes
API operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The
* MFA types activated for the user pool will be listed in the challenge parameters
* MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as
* an input to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete
* sign-in. To set up SMS MFA, users will need help from an administrator to add a phone number to their
* account and then call InitiateAuth
again to restart sign-in.
*
*
* @see ChallengeNameType
*/
public void setChallengeName(String challengeName) {
this.challengeName = challengeName;
}
/**
*
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up are
* presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to continue to
* authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text SMS MFA,
* and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the user
* should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous challenges were
* passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful first
* login. Respond to this challenge with NEW_PASSWORD
and any required attributes that Amazon Cognito
* returned in the requiredAttributes
parameter. You can also set values for attributes that aren't
* required by your user pool and that your app client can write. For more information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that already
* has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon Cognito returned
* in the requiredAttributes
parameter, then use the AdminUpdateUserAttributes
API
* operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The MFA types
* activated for the user pool will be listed in the challenge parameters MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as an input
* to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete sign-in. To set up
* SMS MFA, users will need help from an administrator to add a phone number to their account and then call
* InitiateAuth
again to restart sign-in.
*
*
*
*
* @return The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up
* are presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to
* continue to authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text
* SMS MFA, and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP
* calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the
* user should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous
* challenges were passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful
* first login. Respond to this challenge with NEW_PASSWORD
and any required attributes that
* Amazon Cognito returned in the requiredAttributes
parameter. You can also set values for
* attributes that aren't required by your user pool and that your app client can write. For more
* information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that
* already has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon
* Cognito returned in the requiredAttributes
parameter, then use the
* AdminUpdateUserAttributes
API operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The
* MFA types activated for the user pool will be listed in the challenge parameters
* MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as
* an input to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete
* sign-in. To set up SMS MFA, users will need help from an administrator to add a phone number to their
* account and then call InitiateAuth
again to restart sign-in.
*
*
* @see ChallengeNameType
*/
public String getChallengeName() {
return this.challengeName;
}
/**
*
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up are
* presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to continue to
* authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text SMS MFA,
* and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the user
* should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous challenges were
* passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful first
* login. Respond to this challenge with NEW_PASSWORD
and any required attributes that Amazon Cognito
* returned in the requiredAttributes
parameter. You can also set values for attributes that aren't
* required by your user pool and that your app client can write. For more information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that already
* has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon Cognito returned
* in the requiredAttributes
parameter, then use the AdminUpdateUserAttributes
API
* operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The MFA types
* activated for the user pool will be listed in the challenge parameters MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as an input
* to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete sign-in. To set up
* SMS MFA, users will need help from an administrator to add a phone number to their account and then call
* InitiateAuth
again to restart sign-in.
*
*
*
*
* @param challengeName
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up
* are presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to
* continue to authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text
* SMS MFA, and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP
* calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the
* user should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous
* challenges were passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful
* first login. Respond to this challenge with NEW_PASSWORD
and any required attributes that
* Amazon Cognito returned in the requiredAttributes
parameter. You can also set values for
* attributes that aren't required by your user pool and that your app client can write. For more
* information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that
* already has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon
* Cognito returned in the requiredAttributes
parameter, then use the
* AdminUpdateUserAttributes
API operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The
* MFA types activated for the user pool will be listed in the challenge parameters
* MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as
* an input to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete
* sign-in. To set up SMS MFA, users will need help from an administrator to add a phone number to their
* account and then call InitiateAuth
again to restart sign-in.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChallengeNameType
*/
public AdminInitiateAuthResult withChallengeName(String challengeName) {
setChallengeName(challengeName);
return this;
}
/**
*
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up are
* presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to continue to
* authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text SMS MFA,
* and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the user
* should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous challenges were
* passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful first
* login. Respond to this challenge with NEW_PASSWORD
and any required attributes that Amazon Cognito
* returned in the requiredAttributes
parameter. You can also set values for attributes that aren't
* required by your user pool and that your app client can write. For more information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that already
* has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon Cognito returned
* in the requiredAttributes
parameter, then use the AdminUpdateUserAttributes
API
* operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The MFA types
* activated for the user pool will be listed in the challenge parameters MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as an input
* to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete sign-in. To set up
* SMS MFA, users will need help from an administrator to add a phone number to their account and then call
* InitiateAuth
again to restart sign-in.
*
*
*
*
* @param challengeName
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up
* are presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to
* continue to authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text
* SMS MFA, and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP
* calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the
* user should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous
* challenges were passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful
* first login. Respond to this challenge with NEW_PASSWORD
and any required attributes that
* Amazon Cognito returned in the requiredAttributes
parameter. You can also set values for
* attributes that aren't required by your user pool and that your app client can write. For more
* information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that
* already has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon
* Cognito returned in the requiredAttributes
parameter, then use the
* AdminUpdateUserAttributes
API operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The
* MFA types activated for the user pool will be listed in the challenge parameters
* MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as
* an input to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete
* sign-in. To set up SMS MFA, users will need help from an administrator to add a phone number to their
* account and then call InitiateAuth
again to restart sign-in.
*
*
* @see ChallengeNameType
*/
public void setChallengeName(ChallengeNameType challengeName) {
withChallengeName(challengeName);
}
/**
*
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up are
* presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to continue to
* authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text SMS MFA,
* and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the user
* should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous challenges were
* passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful first
* login. Respond to this challenge with NEW_PASSWORD
and any required attributes that Amazon Cognito
* returned in the requiredAttributes
parameter. You can also set values for attributes that aren't
* required by your user pool and that your app client can write. For more information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that already
* has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon Cognito returned
* in the requiredAttributes
parameter, then use the AdminUpdateUserAttributes
API
* operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The MFA types
* activated for the user pool will be listed in the challenge parameters MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as an input
* to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete sign-in. To set up
* SMS MFA, users will need help from an administrator to add a phone number to their account and then call
* InitiateAuth
again to restart sign-in.
*
*
*
*
* @param challengeName
* The name of the challenge that you're responding to with this call. This is returned in the
* AdminInitiateAuth
response if you must pass another challenge.
*
* -
*
* MFA_SETUP
: If MFA is required, users who don't have at least one of the MFA methods set up
* are presented with an MFA_SETUP
challenge. The user must set up at least one MFA type to
* continue to authenticate.
*
*
* -
*
* SELECT_MFA_TYPE
: Selects the MFA type. Valid MFA options are SMS_MFA
for text
* SMS MFA, and SOFTWARE_TOKEN_MFA
for time-based one-time password (TOTP) software token MFA.
*
*
* -
*
* SMS_MFA
: Next challenge is to supply an SMS_MFA_CODE
, delivered via SMS.
*
*
* -
*
* PASSWORD_VERIFIER
: Next challenge is to supply PASSWORD_CLAIM_SIGNATURE
,
* PASSWORD_CLAIM_SECRET_BLOCK
, and TIMESTAMP
after the client-side SRP
* calculations.
*
*
* -
*
* CUSTOM_CHALLENGE
: This is returned if your custom authentication flow determines that the
* user should pass another challenge before tokens are issued.
*
*
* -
*
* DEVICE_SRP_AUTH
: If device tracking was activated in your user pool and the previous
* challenges were passed, this challenge is returned so that Amazon Cognito can start tracking this device.
*
*
* -
*
* DEVICE_PASSWORD_VERIFIER
: Similar to PASSWORD_VERIFIER
, but for devices only.
*
*
* -
*
* ADMIN_NO_SRP_AUTH
: This is returned if you must authenticate with USERNAME
and
* PASSWORD
directly. An app client must be enabled to use this flow.
*
*
* -
*
* NEW_PASSWORD_REQUIRED
: For users who are required to change their passwords after successful
* first login. Respond to this challenge with NEW_PASSWORD
and any required attributes that
* Amazon Cognito returned in the requiredAttributes
parameter. You can also set values for
* attributes that aren't required by your user pool and that your app client can write. For more
* information, see AdminRespondToAuthChallenge.
*
*
*
* In a NEW_PASSWORD_REQUIRED
challenge response, you can't modify a required attribute that
* already has a value. In AdminRespondToAuthChallenge
, set a value for any keys that Amazon
* Cognito returned in the requiredAttributes
parameter, then use the
* AdminUpdateUserAttributes
API operation to modify the value of any additional attributes.
*
*
* -
*
* MFA_SETUP
: For users who are required to set up an MFA factor before they can sign in. The
* MFA types activated for the user pool will be listed in the challenge parameters
* MFAS_CAN_SETUP
value.
*
*
* To set up software token MFA, use the session returned here from InitiateAuth
as an input to
* AssociateSoftwareToken
, and use the session returned by VerifySoftwareToken
as
* an input to RespondToAuthChallenge
with challenge name MFA_SETUP
to complete
* sign-in. To set up SMS MFA, users will need help from an administrator to add a phone number to their
* account and then call InitiateAuth
again to restart sign-in.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChallengeNameType
*/
public AdminInitiateAuthResult withChallengeName(ChallengeNameType challengeName) {
this.challengeName = challengeName.toString();
return this;
}
/**
*
* The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the caller
* must pass another challenge, they return a session with other challenge parameters. This session should be passed
* as it is to the next AdminRespondToAuthChallenge
API call.
*
*
* @param session
* The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the
* caller must pass another challenge, they return a session with other challenge parameters. This session
* should be passed as it is to the next AdminRespondToAuthChallenge
API call.
*/
public void setSession(String session) {
this.session = session;
}
/**
*
* The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the caller
* must pass another challenge, they return a session with other challenge parameters. This session should be passed
* as it is to the next AdminRespondToAuthChallenge
API call.
*
*
* @return The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the
* caller must pass another challenge, they return a session with other challenge parameters. This session
* should be passed as it is to the next AdminRespondToAuthChallenge
API call.
*/
public String getSession() {
return this.session;
}
/**
*
* The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the caller
* must pass another challenge, they return a session with other challenge parameters. This session should be passed
* as it is to the next AdminRespondToAuthChallenge
API call.
*
*
* @param session
* The session that should be passed both ways in challenge-response calls to the service. If
* AdminInitiateAuth
or AdminRespondToAuthChallenge
API call determines that the
* caller must pass another challenge, they return a session with other challenge parameters. This session
* should be passed as it is to the next AdminRespondToAuthChallenge
API call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminInitiateAuthResult withSession(String session) {
setSession(session);
return this;
}
/**
*
* The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you must
* pass another challenge. The responses in this parameter should be used to compute inputs to the next call (
* AdminRespondToAuthChallenge
).
*
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such as
* email address or phone number), even if you specified an alias in your call to AdminInitiateAuth
.
* This happens because, in the AdminRespondToAuthChallenge
API ChallengeResponses
, the
* USERNAME
attribute can't be an alias.
*
*
* @return The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you
* must pass another challenge. The responses in this parameter should be used to compute inputs to the next
* call (AdminRespondToAuthChallenge
).
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such
* as email address or phone number), even if you specified an alias in your call to
* AdminInitiateAuth
. This happens because, in the AdminRespondToAuthChallenge
API
* ChallengeResponses
, the USERNAME
attribute can't be an alias.
*/
public java.util.Map getChallengeParameters() {
return challengeParameters;
}
/**
*
* The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you must
* pass another challenge. The responses in this parameter should be used to compute inputs to the next call (
* AdminRespondToAuthChallenge
).
*
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such as
* email address or phone number), even if you specified an alias in your call to AdminInitiateAuth
.
* This happens because, in the AdminRespondToAuthChallenge
API ChallengeResponses
, the
* USERNAME
attribute can't be an alias.
*
*
* @param challengeParameters
* The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you
* must pass another challenge. The responses in this parameter should be used to compute inputs to the next
* call (AdminRespondToAuthChallenge
).
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such
* as email address or phone number), even if you specified an alias in your call to
* AdminInitiateAuth
. This happens because, in the AdminRespondToAuthChallenge
API
* ChallengeResponses
, the USERNAME
attribute can't be an alias.
*/
public void setChallengeParameters(java.util.Map challengeParameters) {
this.challengeParameters = challengeParameters;
}
/**
*
* The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you must
* pass another challenge. The responses in this parameter should be used to compute inputs to the next call (
* AdminRespondToAuthChallenge
).
*
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such as
* email address or phone number), even if you specified an alias in your call to AdminInitiateAuth
.
* This happens because, in the AdminRespondToAuthChallenge
API ChallengeResponses
, the
* USERNAME
attribute can't be an alias.
*
*
* @param challengeParameters
* The challenge parameters. These are returned to you in the AdminInitiateAuth
response if you
* must pass another challenge. The responses in this parameter should be used to compute inputs to the next
* call (AdminRespondToAuthChallenge
).
*
* All challenges require USERNAME
and SECRET_HASH
(if applicable).
*
*
* The value of the USER_ID_FOR_SRP
attribute is the user's actual username, not an alias (such
* as email address or phone number), even if you specified an alias in your call to
* AdminInitiateAuth
. This happens because, in the AdminRespondToAuthChallenge
API
* ChallengeResponses
, the USERNAME
attribute can't be an alias.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminInitiateAuthResult withChallengeParameters(java.util.Map challengeParameters) {
setChallengeParameters(challengeParameters);
return this;
}
/**
* Add a single ChallengeParameters entry
*
* @see AdminInitiateAuthResult#withChallengeParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public AdminInitiateAuthResult addChallengeParametersEntry(String key, String value) {
if (null == this.challengeParameters) {
this.challengeParameters = new java.util.HashMap();
}
if (this.challengeParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.challengeParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into ChallengeParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminInitiateAuthResult clearChallengeParametersEntries() {
this.challengeParameters = null;
return this;
}
/**
*
* The result of the authentication response. This is only returned if the caller doesn't need to pass another
* challenge. If the caller does need to pass another challenge before it gets tokens, ChallengeName
,
* ChallengeParameters
, and Session
are returned.
*
*
* @param authenticationResult
* The result of the authentication response. This is only returned if the caller doesn't need to pass
* another challenge. If the caller does need to pass another challenge before it gets tokens,
* ChallengeName
, ChallengeParameters
, and Session
are returned.
*/
public void setAuthenticationResult(AuthenticationResultType authenticationResult) {
this.authenticationResult = authenticationResult;
}
/**
*
* The result of the authentication response. This is only returned if the caller doesn't need to pass another
* challenge. If the caller does need to pass another challenge before it gets tokens, ChallengeName
,
* ChallengeParameters
, and Session
are returned.
*
*
* @return The result of the authentication response. This is only returned if the caller doesn't need to pass
* another challenge. If the caller does need to pass another challenge before it gets tokens,
* ChallengeName
, ChallengeParameters
, and Session
are returned.
*/
public AuthenticationResultType getAuthenticationResult() {
return this.authenticationResult;
}
/**
*
* The result of the authentication response. This is only returned if the caller doesn't need to pass another
* challenge. If the caller does need to pass another challenge before it gets tokens, ChallengeName
,
* ChallengeParameters
, and Session
are returned.
*
*
* @param authenticationResult
* The result of the authentication response. This is only returned if the caller doesn't need to pass
* another challenge. If the caller does need to pass another challenge before it gets tokens,
* ChallengeName
, ChallengeParameters
, and Session
are returned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminInitiateAuthResult withAuthenticationResult(AuthenticationResultType authenticationResult) {
setAuthenticationResult(authenticationResult);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getChallengeName() != null)
sb.append("ChallengeName: ").append(getChallengeName()).append(",");
if (getSession() != null)
sb.append("Session: ").append("***Sensitive Data Redacted***").append(",");
if (getChallengeParameters() != null)
sb.append("ChallengeParameters: ").append(getChallengeParameters()).append(",");
if (getAuthenticationResult() != null)
sb.append("AuthenticationResult: ").append(getAuthenticationResult());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AdminInitiateAuthResult == false)
return false;
AdminInitiateAuthResult other = (AdminInitiateAuthResult) obj;
if (other.getChallengeName() == null ^ this.getChallengeName() == null)
return false;
if (other.getChallengeName() != null && other.getChallengeName().equals(this.getChallengeName()) == false)
return false;
if (other.getSession() == null ^ this.getSession() == null)
return false;
if (other.getSession() != null && other.getSession().equals(this.getSession()) == false)
return false;
if (other.getChallengeParameters() == null ^ this.getChallengeParameters() == null)
return false;
if (other.getChallengeParameters() != null && other.getChallengeParameters().equals(this.getChallengeParameters()) == false)
return false;
if (other.getAuthenticationResult() == null ^ this.getAuthenticationResult() == null)
return false;
if (other.getAuthenticationResult() != null && other.getAuthenticationResult().equals(this.getAuthenticationResult()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getChallengeName() == null) ? 0 : getChallengeName().hashCode());
hashCode = prime * hashCode + ((getSession() == null) ? 0 : getSession().hashCode());
hashCode = prime * hashCode + ((getChallengeParameters() == null) ? 0 : getChallengeParameters().hashCode());
hashCode = prime * hashCode + ((getAuthenticationResult() == null) ? 0 : getAuthenticationResult().hashCode());
return hashCode;
}
@Override
public AdminInitiateAuthResult clone() {
try {
return (AdminInitiateAuthResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}