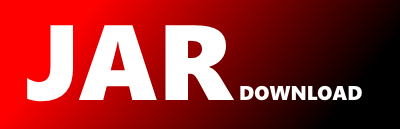
com.amazonaws.services.cognitoidp.model.InitiateAuthRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cognitoidp Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidp.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Initiates the authentication request.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InitiateAuthRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP variables to
* be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the next
* challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access token
* and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly. If a
* user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't find the user
* name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
*
*/
private String authFlow;
/**
*
* The authentication parameters. These are inputs corresponding to the AuthFlow
that you're invoking.
* The required values depend on the value of AuthFlow
:
*
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client is
* configured with client secret), DEVICE_KEY
. To start the authentication flow with password
* verification, include ChallengeName: SRP_A
and SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
*
*/
private java.util.Map authParameters;
/**
*
* A map of custom key-value pairs that you can provide as input for certain custom workflows that this action
* triggers.
*
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the InitiateAuth
* API action, Amazon Cognito invokes the Lambda functions that are specified for various triggers. The
* ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the function
* receives as input. This payload contains a validationData
attribute, which provides the data that
* you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function code in Lambda, you
* can process the validationData
value to enhance your workflow for your specific needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following triggers,
* but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a user pool
* to support custom workflows. If your user pool configuration doesn't include triggers, the ClientMetadata
* parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
*
*/
private java.util.Map clientMetadata;
/**
*
* The app client ID.
*
*/
private String clientId;
/**
*
* The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
calls.
*
*/
private AnalyticsMetadataType analyticsMetadata;
/**
*
* Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon Cognito
* advanced security evaluates the risk of an authentication event based on the context that your app generates and
* passes to Amazon Cognito when it makes API requests.
*
*/
private UserContextDataType userContextData;
/**
*
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP variables to
* be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the next
* challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access token
* and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly. If a
* user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't find the user
* name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
*
*
* @param authFlow
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP
* variables to be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the
* next challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access
* token and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly.
* If a user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't
* find the user name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
* @see AuthFlowType
*/
public void setAuthFlow(String authFlow) {
this.authFlow = authFlow;
}
/**
*
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP variables to
* be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the next
* challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access token
* and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly. If a
* user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't find the user
* name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
*
*
* @return The authentication flow for this call to run. The API action will depend on this value. For example:
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP
* variables to be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the
* next challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access
* token and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly.
* If a user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't
* find the user name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
* @see AuthFlowType
*/
public String getAuthFlow() {
return this.authFlow;
}
/**
*
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP variables to
* be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the next
* challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access token
* and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly. If a
* user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't find the user
* name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
*
*
* @param authFlow
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP
* variables to be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the
* next challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access
* token and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly.
* If a user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't
* find the user name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthFlowType
*/
public InitiateAuthRequest withAuthFlow(String authFlow) {
setAuthFlow(authFlow);
return this;
}
/**
*
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP variables to
* be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the next
* challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access token
* and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly. If a
* user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't find the user
* name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
*
*
* @param authFlow
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP
* variables to be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the
* next challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access
* token and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly.
* If a user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't
* find the user name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
* @see AuthFlowType
*/
public void setAuthFlow(AuthFlowType authFlow) {
withAuthFlow(authFlow);
}
/**
*
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP variables to
* be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the next
* challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access token
* and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly. If a
* user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't find the user
* name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
*
*
* @param authFlow
* The authentication flow for this call to run. The API action will depend on this value. For example:
*
* -
*
* REFRESH_TOKEN_AUTH
takes in a valid refresh token and returns new tokens.
*
*
* -
*
* USER_SRP_AUTH
takes in USERNAME
and SRP_A
and returns the SRP
* variables to be used for next challenge execution.
*
*
* -
*
* USER_PASSWORD_AUTH
takes in USERNAME
and PASSWORD
and returns the
* next challenge or tokens.
*
*
*
*
* Valid values include:
*
*
* -
*
* USER_SRP_AUTH
: Authentication flow for the Secure Remote Password (SRP) protocol.
*
*
* -
*
* REFRESH_TOKEN_AUTH
/REFRESH_TOKEN
: Authentication flow for refreshing the access
* token and ID token by supplying a valid refresh token.
*
*
* -
*
* CUSTOM_AUTH
: Custom authentication flow.
*
*
* -
*
* USER_PASSWORD_AUTH
: Non-SRP authentication flow; user name and password are passed directly.
* If a user migration Lambda trigger is set, this flow will invoke the user migration Lambda if it doesn't
* find the user name in the user pool.
*
*
*
*
* ADMIN_NO_SRP_AUTH
isn't a valid value.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthFlowType
*/
public InitiateAuthRequest withAuthFlow(AuthFlowType authFlow) {
this.authFlow = authFlow.toString();
return this;
}
/**
*
* The authentication parameters. These are inputs corresponding to the AuthFlow
that you're invoking.
* The required values depend on the value of AuthFlow
:
*
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client is
* configured with client secret), DEVICE_KEY
. To start the authentication flow with password
* verification, include ChallengeName: SRP_A
and SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
*
*
* @return The authentication parameters. These are inputs corresponding to the AuthFlow
that you're
* invoking. The required values depend on the value of AuthFlow
:
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client
* is configured with client secret), DEVICE_KEY
. To start the authentication flow with
* password verification, include ChallengeName: SRP_A
and
* SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
*/
public java.util.Map getAuthParameters() {
return authParameters;
}
/**
*
* The authentication parameters. These are inputs corresponding to the AuthFlow
that you're invoking.
* The required values depend on the value of AuthFlow
:
*
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client is
* configured with client secret), DEVICE_KEY
. To start the authentication flow with password
* verification, include ChallengeName: SRP_A
and SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
*
*
* @param authParameters
* The authentication parameters. These are inputs corresponding to the AuthFlow
that you're
* invoking. The required values depend on the value of AuthFlow
:
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client is
* configured with client secret), DEVICE_KEY
. To start the authentication flow with password
* verification, include ChallengeName: SRP_A
and SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
*/
public void setAuthParameters(java.util.Map authParameters) {
this.authParameters = authParameters;
}
/**
*
* The authentication parameters. These are inputs corresponding to the AuthFlow
that you're invoking.
* The required values depend on the value of AuthFlow
:
*
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret), DEVICE_KEY
* .
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client is
* configured with client secret), DEVICE_KEY
. To start the authentication flow with password
* verification, include ChallengeName: SRP_A
and SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
*
*
* @param authParameters
* The authentication parameters. These are inputs corresponding to the AuthFlow
that you're
* invoking. The required values depend on the value of AuthFlow
:
*
* -
*
* For USER_SRP_AUTH
: USERNAME
(required), SRP_A
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For USER_PASSWORD_AUTH
: USERNAME
(required), PASSWORD
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For REFRESH_TOKEN_AUTH/REFRESH_TOKEN
: REFRESH_TOKEN
(required),
* SECRET_HASH
(required if the app client is configured with a client secret),
* DEVICE_KEY
.
*
*
* -
*
* For CUSTOM_AUTH
: USERNAME
(required), SECRET_HASH
(if app client is
* configured with client secret), DEVICE_KEY
. To start the authentication flow with password
* verification, include ChallengeName: SRP_A
and SRP_A: (The SRP_A Value)
.
*
*
*
*
* For more information about SECRET_HASH
, see Computing secret hash values. For information about DEVICE_KEY
, see Working with user devices in your user pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest withAuthParameters(java.util.Map authParameters) {
setAuthParameters(authParameters);
return this;
}
/**
* Add a single AuthParameters entry
*
* @see InitiateAuthRequest#withAuthParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest addAuthParametersEntry(String key, String value) {
if (null == this.authParameters) {
this.authParameters = new java.util.HashMap();
}
if (this.authParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.authParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into AuthParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest clearAuthParametersEntries() {
this.authParameters = null;
return this;
}
/**
*
* A map of custom key-value pairs that you can provide as input for certain custom workflows that this action
* triggers.
*
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the InitiateAuth
* API action, Amazon Cognito invokes the Lambda functions that are specified for various triggers. The
* ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the function
* receives as input. This payload contains a validationData
attribute, which provides the data that
* you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function code in Lambda, you
* can process the validationData
value to enhance your workflow for your specific needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following triggers,
* but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a user pool
* to support custom workflows. If your user pool configuration doesn't include triggers, the ClientMetadata
* parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
*
*
* @return A map of custom key-value pairs that you can provide as input for certain custom workflows that this
* action triggers.
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the
* InitiateAuth API action, Amazon Cognito invokes the Lambda functions that are specified for various
* triggers. The ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the
* function receives as input. This payload contains a validationData
attribute, which provides
* the data that you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function
* code in Lambda, you can process the validationData
value to enhance your workflow for your
* specific needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following
* triggers, but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a
* user pool to support custom workflows. If your user pool configuration doesn't include triggers, the
* ClientMetadata parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
*/
public java.util.Map getClientMetadata() {
return clientMetadata;
}
/**
*
* A map of custom key-value pairs that you can provide as input for certain custom workflows that this action
* triggers.
*
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the InitiateAuth
* API action, Amazon Cognito invokes the Lambda functions that are specified for various triggers. The
* ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the function
* receives as input. This payload contains a validationData
attribute, which provides the data that
* you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function code in Lambda, you
* can process the validationData
value to enhance your workflow for your specific needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following triggers,
* but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a user pool
* to support custom workflows. If your user pool configuration doesn't include triggers, the ClientMetadata
* parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
*
*
* @param clientMetadata
* A map of custom key-value pairs that you can provide as input for certain custom workflows that this
* action triggers.
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the
* InitiateAuth API action, Amazon Cognito invokes the Lambda functions that are specified for various
* triggers. The ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the function
* receives as input. This payload contains a validationData
attribute, which provides the data
* that you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function code in
* Lambda, you can process the validationData
value to enhance your workflow for your specific
* needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following
* triggers, but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a user
* pool to support custom workflows. If your user pool configuration doesn't include triggers, the
* ClientMetadata parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
*/
public void setClientMetadata(java.util.Map clientMetadata) {
this.clientMetadata = clientMetadata;
}
/**
*
* A map of custom key-value pairs that you can provide as input for certain custom workflows that this action
* triggers.
*
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the InitiateAuth
* API action, Amazon Cognito invokes the Lambda functions that are specified for various triggers. The
* ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the function
* receives as input. This payload contains a validationData
attribute, which provides the data that
* you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function code in Lambda, you
* can process the validationData
value to enhance your workflow for your specific needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following triggers,
* but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a user pool
* to support custom workflows. If your user pool configuration doesn't include triggers, the ClientMetadata
* parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
*
*
* @param clientMetadata
* A map of custom key-value pairs that you can provide as input for certain custom workflows that this
* action triggers.
*
* You create custom workflows by assigning Lambda functions to user pool triggers. When you use the
* InitiateAuth API action, Amazon Cognito invokes the Lambda functions that are specified for various
* triggers. The ClientMetadata value is passed as input to the functions for only the following triggers:
*
*
* -
*
* Pre signup
*
*
* -
*
* Pre authentication
*
*
* -
*
* User migration
*
*
*
*
* When Amazon Cognito invokes the functions for these triggers, it passes a JSON payload, which the function
* receives as input. This payload contains a validationData
attribute, which provides the data
* that you assigned to the ClientMetadata parameter in your InitiateAuth request. In your function code in
* Lambda, you can process the validationData
value to enhance your workflow for your specific
* needs.
*
*
* When you use the InitiateAuth API action, Amazon Cognito also invokes the functions for the following
* triggers, but it doesn't provide the ClientMetadata value as input:
*
*
* -
*
* Post authentication
*
*
* -
*
* Custom message
*
*
* -
*
* Pre token generation
*
*
* -
*
* Create auth challenge
*
*
* -
*
* Define auth challenge
*
*
*
*
* For more information, see Customizing user pool Workflows with Lambda Triggers in the Amazon Cognito Developer Guide.
*
*
*
* When you use the ClientMetadata parameter, remember that Amazon Cognito won't do the following:
*
*
* -
*
* Store the ClientMetadata value. This data is available only to Lambda triggers that are assigned to a user
* pool to support custom workflows. If your user pool configuration doesn't include triggers, the
* ClientMetadata parameter serves no purpose.
*
*
* -
*
* Validate the ClientMetadata value.
*
*
* -
*
* Encrypt the ClientMetadata value. Don't use Amazon Cognito to provide sensitive information.
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest withClientMetadata(java.util.Map clientMetadata) {
setClientMetadata(clientMetadata);
return this;
}
/**
* Add a single ClientMetadata entry
*
* @see InitiateAuthRequest#withClientMetadata
* @returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest addClientMetadataEntry(String key, String value) {
if (null == this.clientMetadata) {
this.clientMetadata = new java.util.HashMap();
}
if (this.clientMetadata.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.clientMetadata.put(key, value);
return this;
}
/**
* Removes all the entries added into ClientMetadata.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest clearClientMetadataEntries() {
this.clientMetadata = null;
return this;
}
/**
*
* The app client ID.
*
*
* @param clientId
* The app client ID.
*/
public void setClientId(String clientId) {
this.clientId = clientId;
}
/**
*
* The app client ID.
*
*
* @return The app client ID.
*/
public String getClientId() {
return this.clientId;
}
/**
*
* The app client ID.
*
*
* @param clientId
* The app client ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest withClientId(String clientId) {
setClientId(clientId);
return this;
}
/**
*
* The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
calls.
*
*
* @param analyticsMetadata
* The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
* calls.
*/
public void setAnalyticsMetadata(AnalyticsMetadataType analyticsMetadata) {
this.analyticsMetadata = analyticsMetadata;
}
/**
*
* The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
calls.
*
*
* @return The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
* calls.
*/
public AnalyticsMetadataType getAnalyticsMetadata() {
return this.analyticsMetadata;
}
/**
*
* The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
calls.
*
*
* @param analyticsMetadata
* The Amazon Pinpoint analytics metadata that contributes to your metrics for InitiateAuth
* calls.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest withAnalyticsMetadata(AnalyticsMetadataType analyticsMetadata) {
setAnalyticsMetadata(analyticsMetadata);
return this;
}
/**
*
* Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon Cognito
* advanced security evaluates the risk of an authentication event based on the context that your app generates and
* passes to Amazon Cognito when it makes API requests.
*
*
* @param userContextData
* Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon
* Cognito advanced security evaluates the risk of an authentication event based on the context that your app
* generates and passes to Amazon Cognito when it makes API requests.
*/
public void setUserContextData(UserContextDataType userContextData) {
this.userContextData = userContextData;
}
/**
*
* Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon Cognito
* advanced security evaluates the risk of an authentication event based on the context that your app generates and
* passes to Amazon Cognito when it makes API requests.
*
*
* @return Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon
* Cognito advanced security evaluates the risk of an authentication event based on the context that your
* app generates and passes to Amazon Cognito when it makes API requests.
*/
public UserContextDataType getUserContextData() {
return this.userContextData;
}
/**
*
* Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon Cognito
* advanced security evaluates the risk of an authentication event based on the context that your app generates and
* passes to Amazon Cognito when it makes API requests.
*
*
* @param userContextData
* Contextual data about your user session, such as the device fingerprint, IP address, or location. Amazon
* Cognito advanced security evaluates the risk of an authentication event based on the context that your app
* generates and passes to Amazon Cognito when it makes API requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InitiateAuthRequest withUserContextData(UserContextDataType userContextData) {
setUserContextData(userContextData);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAuthFlow() != null)
sb.append("AuthFlow: ").append(getAuthFlow()).append(",");
if (getAuthParameters() != null)
sb.append("AuthParameters: ").append("***Sensitive Data Redacted***").append(",");
if (getClientMetadata() != null)
sb.append("ClientMetadata: ").append(getClientMetadata()).append(",");
if (getClientId() != null)
sb.append("ClientId: ").append("***Sensitive Data Redacted***").append(",");
if (getAnalyticsMetadata() != null)
sb.append("AnalyticsMetadata: ").append(getAnalyticsMetadata()).append(",");
if (getUserContextData() != null)
sb.append("UserContextData: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InitiateAuthRequest == false)
return false;
InitiateAuthRequest other = (InitiateAuthRequest) obj;
if (other.getAuthFlow() == null ^ this.getAuthFlow() == null)
return false;
if (other.getAuthFlow() != null && other.getAuthFlow().equals(this.getAuthFlow()) == false)
return false;
if (other.getAuthParameters() == null ^ this.getAuthParameters() == null)
return false;
if (other.getAuthParameters() != null && other.getAuthParameters().equals(this.getAuthParameters()) == false)
return false;
if (other.getClientMetadata() == null ^ this.getClientMetadata() == null)
return false;
if (other.getClientMetadata() != null && other.getClientMetadata().equals(this.getClientMetadata()) == false)
return false;
if (other.getClientId() == null ^ this.getClientId() == null)
return false;
if (other.getClientId() != null && other.getClientId().equals(this.getClientId()) == false)
return false;
if (other.getAnalyticsMetadata() == null ^ this.getAnalyticsMetadata() == null)
return false;
if (other.getAnalyticsMetadata() != null && other.getAnalyticsMetadata().equals(this.getAnalyticsMetadata()) == false)
return false;
if (other.getUserContextData() == null ^ this.getUserContextData() == null)
return false;
if (other.getUserContextData() != null && other.getUserContextData().equals(this.getUserContextData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAuthFlow() == null) ? 0 : getAuthFlow().hashCode());
hashCode = prime * hashCode + ((getAuthParameters() == null) ? 0 : getAuthParameters().hashCode());
hashCode = prime * hashCode + ((getClientMetadata() == null) ? 0 : getClientMetadata().hashCode());
hashCode = prime * hashCode + ((getClientId() == null) ? 0 : getClientId().hashCode());
hashCode = prime * hashCode + ((getAnalyticsMetadata() == null) ? 0 : getAnalyticsMetadata().hashCode());
hashCode = prime * hashCode + ((getUserContextData() == null) ? 0 : getUserContextData().hashCode());
return hashCode;
}
@Override
public InitiateAuthRequest clone() {
return (InitiateAuthRequest) super.clone();
}
}