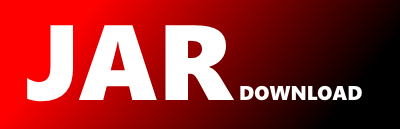
com.amazonaws.services.cognitoidp.model.ListUsersRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cognitoidp Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidp.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the request to list users.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListUsersRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The user pool ID for the user pool on which the search should be performed.
*
*/
private String userPoolId;
/**
*
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter, Amazon
* Cognito returns all attributes for each user.
*
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for the
* attribute you request. Attributes that you can't filter on, including custom attributes, must have a value set in
* every user profile before an AttributesToGet
parameter returns results.
*
*/
private java.util.List attributesToGet;
/**
*
* Maximum number of users to be returned.
*
*/
private Integer limit;
/**
*
* This API operation returns a limited number of results. The pagination token is an identifier that you can
* present in an additional API request with the same parameters. When you include the pagination token, Amazon
* Cognito returns the next set of items after the current list. Subsequent requests return a new pagination token.
* By use of this token, you can paginate through the full list of items.
*
*/
private String paginationToken;
/**
*
* A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation marks
* within the filter string must be escaped using the backslash (\
) character. For example,
* "family_name = \"Reddy\""
.
*
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "given_name = \"Jon\"
* ". For a prefix ("starts with") match, use ^=
, for example, "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one attribute. For
* an advanced search, use a client-side filter with the --query
parameter of the
* list-users
action in the CLI. When you use a client-side filter, ListUsers returns a paginated list
* of zero or more users. You can receive multiple pages in a row with zero results. Repeat the query with each
* pagination token that is returned until you receive a null pagination token value, and then review the combined
* result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in the Command Line Interface User
* Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
*
*/
private String filter;
/**
*
* The user pool ID for the user pool on which the search should be performed.
*
*
* @param userPoolId
* The user pool ID for the user pool on which the search should be performed.
*/
public void setUserPoolId(String userPoolId) {
this.userPoolId = userPoolId;
}
/**
*
* The user pool ID for the user pool on which the search should be performed.
*
*
* @return The user pool ID for the user pool on which the search should be performed.
*/
public String getUserPoolId() {
return this.userPoolId;
}
/**
*
* The user pool ID for the user pool on which the search should be performed.
*
*
* @param userPoolId
* The user pool ID for the user pool on which the search should be performed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListUsersRequest withUserPoolId(String userPoolId) {
setUserPoolId(userPoolId);
return this;
}
/**
*
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter, Amazon
* Cognito returns all attributes for each user.
*
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for the
* attribute you request. Attributes that you can't filter on, including custom attributes, must have a value set in
* every user profile before an AttributesToGet
parameter returns results.
*
*
* @return A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito
* to include in the response for each user. When you don't provide an AttributesToGet
* parameter, Amazon Cognito returns all attributes for each user.
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for
* the attribute you request. Attributes that you can't filter on, including custom attributes, must have a
* value set in every user profile before an AttributesToGet
parameter returns results.
*/
public java.util.List getAttributesToGet() {
return attributesToGet;
}
/**
*
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter, Amazon
* Cognito returns all attributes for each user.
*
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for the
* attribute you request. Attributes that you can't filter on, including custom attributes, must have a value set in
* every user profile before an AttributesToGet
parameter returns results.
*
*
* @param attributesToGet
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter,
* Amazon Cognito returns all attributes for each user.
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for
* the attribute you request. Attributes that you can't filter on, including custom attributes, must have a
* value set in every user profile before an AttributesToGet
parameter returns results.
*/
public void setAttributesToGet(java.util.Collection attributesToGet) {
if (attributesToGet == null) {
this.attributesToGet = null;
return;
}
this.attributesToGet = new java.util.ArrayList(attributesToGet);
}
/**
*
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter, Amazon
* Cognito returns all attributes for each user.
*
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for the
* attribute you request. Attributes that you can't filter on, including custom attributes, must have a value set in
* every user profile before an AttributesToGet
parameter returns results.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttributesToGet(java.util.Collection)} or {@link #withAttributesToGet(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param attributesToGet
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter,
* Amazon Cognito returns all attributes for each user.
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for
* the attribute you request. Attributes that you can't filter on, including custom attributes, must have a
* value set in every user profile before an AttributesToGet
parameter returns results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListUsersRequest withAttributesToGet(String... attributesToGet) {
if (this.attributesToGet == null) {
setAttributesToGet(new java.util.ArrayList(attributesToGet.length));
}
for (String ele : attributesToGet) {
this.attributesToGet.add(ele);
}
return this;
}
/**
*
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter, Amazon
* Cognito returns all attributes for each user.
*
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for the
* attribute you request. Attributes that you can't filter on, including custom attributes, must have a value set in
* every user profile before an AttributesToGet
parameter returns results.
*
*
* @param attributesToGet
* A JSON array of user attribute names, for example given_name
, that you want Amazon Cognito to
* include in the response for each user. When you don't provide an AttributesToGet
parameter,
* Amazon Cognito returns all attributes for each user.
*
* Use AttributesToGet
with required attributes in your user pool, or in conjunction with
* Filter
. Amazon Cognito returns an error if not all users in the results have set a value for
* the attribute you request. Attributes that you can't filter on, including custom attributes, must have a
* value set in every user profile before an AttributesToGet
parameter returns results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListUsersRequest withAttributesToGet(java.util.Collection attributesToGet) {
setAttributesToGet(attributesToGet);
return this;
}
/**
*
* Maximum number of users to be returned.
*
*
* @param limit
* Maximum number of users to be returned.
*/
public void setLimit(Integer limit) {
this.limit = limit;
}
/**
*
* Maximum number of users to be returned.
*
*
* @return Maximum number of users to be returned.
*/
public Integer getLimit() {
return this.limit;
}
/**
*
* Maximum number of users to be returned.
*
*
* @param limit
* Maximum number of users to be returned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListUsersRequest withLimit(Integer limit) {
setLimit(limit);
return this;
}
/**
*
* This API operation returns a limited number of results. The pagination token is an identifier that you can
* present in an additional API request with the same parameters. When you include the pagination token, Amazon
* Cognito returns the next set of items after the current list. Subsequent requests return a new pagination token.
* By use of this token, you can paginate through the full list of items.
*
*
* @param paginationToken
* This API operation returns a limited number of results. The pagination token is an identifier that you can
* present in an additional API request with the same parameters. When you include the pagination token,
* Amazon Cognito returns the next set of items after the current list. Subsequent requests return a new
* pagination token. By use of this token, you can paginate through the full list of items.
*/
public void setPaginationToken(String paginationToken) {
this.paginationToken = paginationToken;
}
/**
*
* This API operation returns a limited number of results. The pagination token is an identifier that you can
* present in an additional API request with the same parameters. When you include the pagination token, Amazon
* Cognito returns the next set of items after the current list. Subsequent requests return a new pagination token.
* By use of this token, you can paginate through the full list of items.
*
*
* @return This API operation returns a limited number of results. The pagination token is an identifier that you
* can present in an additional API request with the same parameters. When you include the pagination token,
* Amazon Cognito returns the next set of items after the current list. Subsequent requests return a new
* pagination token. By use of this token, you can paginate through the full list of items.
*/
public String getPaginationToken() {
return this.paginationToken;
}
/**
*
* This API operation returns a limited number of results. The pagination token is an identifier that you can
* present in an additional API request with the same parameters. When you include the pagination token, Amazon
* Cognito returns the next set of items after the current list. Subsequent requests return a new pagination token.
* By use of this token, you can paginate through the full list of items.
*
*
* @param paginationToken
* This API operation returns a limited number of results. The pagination token is an identifier that you can
* present in an additional API request with the same parameters. When you include the pagination token,
* Amazon Cognito returns the next set of items after the current list. Subsequent requests return a new
* pagination token. By use of this token, you can paginate through the full list of items.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListUsersRequest withPaginationToken(String paginationToken) {
setPaginationToken(paginationToken);
return this;
}
/**
*
* A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation marks
* within the filter string must be escaped using the backslash (\
) character. For example,
* "family_name = \"Reddy\""
.
*
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "given_name = \"Jon\"
* ". For a prefix ("starts with") match, use ^=
, for example, "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one attribute. For
* an advanced search, use a client-side filter with the --query
parameter of the
* list-users
action in the CLI. When you use a client-side filter, ListUsers returns a paginated list
* of zero or more users. You can receive multiple pages in a row with zero results. Repeat the query with each
* pagination token that is returned until you receive a null pagination token value, and then review the combined
* result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in the Command Line Interface User
* Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
*
*
* @param filter
* A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation
* marks within the filter string must be escaped using the backslash (\
) character. For
* example, "family_name = \"Reddy\""
.
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a
* time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "
* given_name = \"Jon\"
". For a prefix ("starts with") match, use ^=
, for example,
* "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one
* attribute. For an advanced search, use a client-side filter with the --query
parameter of the
* list-users
action in the CLI. When you use a client-side filter, ListUsers returns a
* paginated list of zero or more users. You can receive multiple pages in a row with zero results. Repeat
* the query with each pagination token that is returned until you receive a null pagination token value, and
* then review the combined result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in
* the Command Line
* Interface User Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
*/
public void setFilter(String filter) {
this.filter = filter;
}
/**
*
* A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation marks
* within the filter string must be escaped using the backslash (\
) character. For example,
* "family_name = \"Reddy\""
.
*
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "given_name = \"Jon\"
* ". For a prefix ("starts with") match, use ^=
, for example, "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one attribute. For
* an advanced search, use a client-side filter with the --query
parameter of the
* list-users
action in the CLI. When you use a client-side filter, ListUsers returns a paginated list
* of zero or more users. You can receive multiple pages in a row with zero results. Repeat the query with each
* pagination token that is returned until you receive a null pagination token value, and then review the combined
* result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in the Command Line Interface User
* Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
*
*
* @return A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation
* marks within the filter string must be escaped using the backslash (\
) character. For
* example, "family_name = \"Reddy\""
.
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a
* time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "
* given_name = \"Jon\"
". For a prefix ("starts with") match, use ^=
, for example,
* "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one
* attribute. For an advanced search, use a client-side filter with the --query
parameter of
* the list-users
action in the CLI. When you use a client-side filter, ListUsers returns a
* paginated list of zero or more users. You can receive multiple pages in a row with zero results. Repeat
* the query with each pagination token that is returned until you receive a null pagination token value,
* and then review the combined result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in
* the Command Line
* Interface User Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
*/
public String getFilter() {
return this.filter;
}
/**
*
* A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation marks
* within the filter string must be escaped using the backslash (\
) character. For example,
* "family_name = \"Reddy\""
.
*
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "given_name = \"Jon\"
* ". For a prefix ("starts with") match, use ^=
, for example, "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one attribute. For
* an advanced search, use a client-side filter with the --query
parameter of the
* list-users
action in the CLI. When you use a client-side filter, ListUsers returns a paginated list
* of zero or more users. You can receive multiple pages in a row with zero results. Repeat the query with each
* pagination token that is returned until you receive a null pagination token value, and then review the combined
* result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in the Command Line Interface User
* Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
*
*
* @param filter
* A filter string of the form "AttributeName Filter-Type "AttributeValue"". Quotation
* marks within the filter string must be escaped using the backslash (\
) character. For
* example, "family_name = \"Reddy\""
.
*
* -
*
* AttributeName: The name of the attribute to search for. You can only search for one attribute at a
* time.
*
*
* -
*
* Filter-Type: For an exact match, use =
, for example, "
* given_name = \"Jon\"
". For a prefix ("starts with") match, use ^=
, for example,
* "given_name ^= \"Jon\"
".
*
*
* -
*
* AttributeValue: The attribute value that must be matched for each user.
*
*
*
*
* If the filter string is empty, ListUsers
returns all users in the user pool.
*
*
* You can only search for the following standard attributes:
*
*
* -
*
* username
(case-sensitive)
*
*
* -
*
* email
*
*
* -
*
* phone_number
*
*
* -
*
* name
*
*
* -
*
* given_name
*
*
* -
*
* family_name
*
*
* -
*
* preferred_username
*
*
* -
*
* cognito:user_status
(called Status in the Console) (case-insensitive)
*
*
* -
*
* status (called Enabled in the Console) (case-sensitive)
*
*
* -
*
* sub
*
*
*
*
* Custom attributes aren't searchable.
*
*
*
* You can also list users with a client-side filter. The server-side filter matches no more than one
* attribute. For an advanced search, use a client-side filter with the --query
parameter of the
* list-users
action in the CLI. When you use a client-side filter, ListUsers returns a
* paginated list of zero or more users. You can receive multiple pages in a row with zero results. Repeat
* the query with each pagination token that is returned until you receive a null pagination token value, and
* then review the combined result.
*
*
* For more information about server-side and client-side filtering, see FilteringCLI output in
* the Command Line
* Interface User Guide.
*
*
*
* For more information, see Searching for Users Using the ListUsers API and Examples of Using the ListUsers API in the Amazon Cognito Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListUsersRequest withFilter(String filter) {
setFilter(filter);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUserPoolId() != null)
sb.append("UserPoolId: ").append(getUserPoolId()).append(",");
if (getAttributesToGet() != null)
sb.append("AttributesToGet: ").append(getAttributesToGet()).append(",");
if (getLimit() != null)
sb.append("Limit: ").append(getLimit()).append(",");
if (getPaginationToken() != null)
sb.append("PaginationToken: ").append(getPaginationToken()).append(",");
if (getFilter() != null)
sb.append("Filter: ").append(getFilter());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListUsersRequest == false)
return false;
ListUsersRequest other = (ListUsersRequest) obj;
if (other.getUserPoolId() == null ^ this.getUserPoolId() == null)
return false;
if (other.getUserPoolId() != null && other.getUserPoolId().equals(this.getUserPoolId()) == false)
return false;
if (other.getAttributesToGet() == null ^ this.getAttributesToGet() == null)
return false;
if (other.getAttributesToGet() != null && other.getAttributesToGet().equals(this.getAttributesToGet()) == false)
return false;
if (other.getLimit() == null ^ this.getLimit() == null)
return false;
if (other.getLimit() != null && other.getLimit().equals(this.getLimit()) == false)
return false;
if (other.getPaginationToken() == null ^ this.getPaginationToken() == null)
return false;
if (other.getPaginationToken() != null && other.getPaginationToken().equals(this.getPaginationToken()) == false)
return false;
if (other.getFilter() == null ^ this.getFilter() == null)
return false;
if (other.getFilter() != null && other.getFilter().equals(this.getFilter()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUserPoolId() == null) ? 0 : getUserPoolId().hashCode());
hashCode = prime * hashCode + ((getAttributesToGet() == null) ? 0 : getAttributesToGet().hashCode());
hashCode = prime * hashCode + ((getLimit() == null) ? 0 : getLimit().hashCode());
hashCode = prime * hashCode + ((getPaginationToken() == null) ? 0 : getPaginationToken().hashCode());
hashCode = prime * hashCode + ((getFilter() == null) ? 0 : getFilter().hashCode());
return hashCode;
}
@Override
public ListUsersRequest clone() {
return (ListUsersRequest) super.clone();
}
}