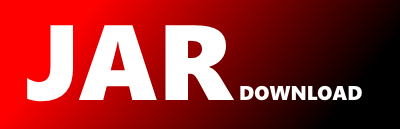
com.amazonaws.services.cognitoidp.model.AdminGetUserResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cognitoidp Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cognitoidp.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Represents the response from the server from the request to get the specified user as an administrator.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AdminGetUserResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The username of the user that you requested.
*
*/
private String username;
/**
*
* An array of name-value pairs representing user attributes.
*
*/
private java.util.List userAttributes;
/**
*
* The date the user was created.
*
*/
private java.util.Date userCreateDate;
/**
*
* The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time format.
* Your SDK might render the output in a human-readable format like ISO 8601 or a Java Date
object.
*
*/
private java.util.Date userLastModifiedDate;
/**
*
* Indicates that the status is enabled
.
*
*/
private Boolean enabled;
/**
*
* The user status. Can be one of the following:
*
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they can
* sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on first
* sign-in, the user must change their password to a new value before doing anything else.
*
*
*
*/
private String userStatus;
/**
*
* This response parameter is no longer supported. It provides information only about SMS MFA configurations.
* It doesn't provide information about time-based one-time password (TOTP) software token MFA configurations. To
* look up information about either type of MFA configuration, use UserMFASettingList instead.
*
*/
private java.util.List mFAOptions;
/**
*
* The user's preferred MFA setting.
*
*/
private String preferredMfaSetting;
/**
*
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
and
* SOFTWARE_TOKEN_MFA
.
*
*/
private java.util.List userMFASettingList;
/**
*
* The username of the user that you requested.
*
*
* @param username
* The username of the user that you requested.
*/
public void setUsername(String username) {
this.username = username;
}
/**
*
* The username of the user that you requested.
*
*
* @return The username of the user that you requested.
*/
public String getUsername() {
return this.username;
}
/**
*
* The username of the user that you requested.
*
*
* @param username
* The username of the user that you requested.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUsername(String username) {
setUsername(username);
return this;
}
/**
*
* An array of name-value pairs representing user attributes.
*
*
* @return An array of name-value pairs representing user attributes.
*/
public java.util.List getUserAttributes() {
return userAttributes;
}
/**
*
* An array of name-value pairs representing user attributes.
*
*
* @param userAttributes
* An array of name-value pairs representing user attributes.
*/
public void setUserAttributes(java.util.Collection userAttributes) {
if (userAttributes == null) {
this.userAttributes = null;
return;
}
this.userAttributes = new java.util.ArrayList(userAttributes);
}
/**
*
* An array of name-value pairs representing user attributes.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUserAttributes(java.util.Collection)} or {@link #withUserAttributes(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param userAttributes
* An array of name-value pairs representing user attributes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUserAttributes(AttributeType... userAttributes) {
if (this.userAttributes == null) {
setUserAttributes(new java.util.ArrayList(userAttributes.length));
}
for (AttributeType ele : userAttributes) {
this.userAttributes.add(ele);
}
return this;
}
/**
*
* An array of name-value pairs representing user attributes.
*
*
* @param userAttributes
* An array of name-value pairs representing user attributes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUserAttributes(java.util.Collection userAttributes) {
setUserAttributes(userAttributes);
return this;
}
/**
*
* The date the user was created.
*
*
* @param userCreateDate
* The date the user was created.
*/
public void setUserCreateDate(java.util.Date userCreateDate) {
this.userCreateDate = userCreateDate;
}
/**
*
* The date the user was created.
*
*
* @return The date the user was created.
*/
public java.util.Date getUserCreateDate() {
return this.userCreateDate;
}
/**
*
* The date the user was created.
*
*
* @param userCreateDate
* The date the user was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUserCreateDate(java.util.Date userCreateDate) {
setUserCreateDate(userCreateDate);
return this;
}
/**
*
* The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time format.
* Your SDK might render the output in a human-readable format like ISO 8601 or a Java Date
object.
*
*
* @param userLastModifiedDate
* The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time
* format. Your SDK might render the output in a human-readable format like ISO 8601 or a Java
* Date
object.
*/
public void setUserLastModifiedDate(java.util.Date userLastModifiedDate) {
this.userLastModifiedDate = userLastModifiedDate;
}
/**
*
* The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time format.
* Your SDK might render the output in a human-readable format like ISO 8601 or a Java Date
object.
*
*
* @return The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time
* format. Your SDK might render the output in a human-readable format like ISO 8601 or a Java
* Date
object.
*/
public java.util.Date getUserLastModifiedDate() {
return this.userLastModifiedDate;
}
/**
*
* The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time format.
* Your SDK might render the output in a human-readable format like ISO 8601 or a Java Date
object.
*
*
* @param userLastModifiedDate
* The date and time when the item was modified. Amazon Cognito returns this timestamp in UNIX epoch time
* format. Your SDK might render the output in a human-readable format like ISO 8601 or a Java
* Date
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUserLastModifiedDate(java.util.Date userLastModifiedDate) {
setUserLastModifiedDate(userLastModifiedDate);
return this;
}
/**
*
* Indicates that the status is enabled
.
*
*
* @param enabled
* Indicates that the status is enabled
.
*/
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
/**
*
* Indicates that the status is enabled
.
*
*
* @return Indicates that the status is enabled
.
*/
public Boolean getEnabled() {
return this.enabled;
}
/**
*
* Indicates that the status is enabled
.
*
*
* @param enabled
* Indicates that the status is enabled
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withEnabled(Boolean enabled) {
setEnabled(enabled);
return this;
}
/**
*
* Indicates that the status is enabled
.
*
*
* @return Indicates that the status is enabled
.
*/
public Boolean isEnabled() {
return this.enabled;
}
/**
*
* The user status. Can be one of the following:
*
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they can
* sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on first
* sign-in, the user must change their password to a new value before doing anything else.
*
*
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they
* can sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on
* first sign-in, the user must change their password to a new value before doing anything else.
*
*
* @see UserStatusType
*/
public void setUserStatus(String userStatus) {
this.userStatus = userStatus;
}
/**
*
* The user status. Can be one of the following:
*
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they can
* sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on first
* sign-in, the user must change their password to a new value before doing anything else.
*
*
*
*
* @return The user status. Can be one of the following:
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they
* can sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on
* first sign-in, the user must change their password to a new value before doing anything else.
*
*
* @see UserStatusType
*/
public String getUserStatus() {
return this.userStatus;
}
/**
*
* The user status. Can be one of the following:
*
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they can
* sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on first
* sign-in, the user must change their password to a new value before doing anything else.
*
*
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they
* can sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on
* first sign-in, the user must change their password to a new value before doing anything else.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see UserStatusType
*/
public AdminGetUserResult withUserStatus(String userStatus) {
setUserStatus(userStatus);
return this;
}
/**
*
* The user status. Can be one of the following:
*
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they can
* sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on first
* sign-in, the user must change their password to a new value before doing anything else.
*
*
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they
* can sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on
* first sign-in, the user must change their password to a new value before doing anything else.
*
*
* @see UserStatusType
*/
public void setUserStatus(UserStatusType userStatus) {
withUserStatus(userStatus);
}
/**
*
* The user status. Can be one of the following:
*
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they can
* sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on first
* sign-in, the user must change their password to a new value before doing anything else.
*
*
*
*
* @param userStatus
* The user status. Can be one of the following:
*
* -
*
* UNCONFIRMED - User has been created but not confirmed.
*
*
* -
*
* CONFIRMED - User has been confirmed.
*
*
* -
*
* UNKNOWN - User status isn't known.
*
*
* -
*
* RESET_REQUIRED - User is confirmed, but the user must request a code and reset their password before they
* can sign in.
*
*
* -
*
* FORCE_CHANGE_PASSWORD - The user is confirmed and the user can sign in using a temporary password, but on
* first sign-in, the user must change their password to a new value before doing anything else.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see UserStatusType
*/
public AdminGetUserResult withUserStatus(UserStatusType userStatus) {
this.userStatus = userStatus.toString();
return this;
}
/**
*
* This response parameter is no longer supported. It provides information only about SMS MFA configurations.
* It doesn't provide information about time-based one-time password (TOTP) software token MFA configurations. To
* look up information about either type of MFA configuration, use UserMFASettingList instead.
*
*
* @return This response parameter is no longer supported. It provides information only about SMS MFA
* configurations. It doesn't provide information about time-based one-time password (TOTP) software token
* MFA configurations. To look up information about either type of MFA configuration, use UserMFASettingList
* instead.
*/
public java.util.List getMFAOptions() {
return mFAOptions;
}
/**
*
* This response parameter is no longer supported. It provides information only about SMS MFA configurations.
* It doesn't provide information about time-based one-time password (TOTP) software token MFA configurations. To
* look up information about either type of MFA configuration, use UserMFASettingList instead.
*
*
* @param mFAOptions
* This response parameter is no longer supported. It provides information only about SMS MFA
* configurations. It doesn't provide information about time-based one-time password (TOTP) software token
* MFA configurations. To look up information about either type of MFA configuration, use UserMFASettingList
* instead.
*/
public void setMFAOptions(java.util.Collection mFAOptions) {
if (mFAOptions == null) {
this.mFAOptions = null;
return;
}
this.mFAOptions = new java.util.ArrayList(mFAOptions);
}
/**
*
* This response parameter is no longer supported. It provides information only about SMS MFA configurations.
* It doesn't provide information about time-based one-time password (TOTP) software token MFA configurations. To
* look up information about either type of MFA configuration, use UserMFASettingList instead.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMFAOptions(java.util.Collection)} or {@link #withMFAOptions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param mFAOptions
* This response parameter is no longer supported. It provides information only about SMS MFA
* configurations. It doesn't provide information about time-based one-time password (TOTP) software token
* MFA configurations. To look up information about either type of MFA configuration, use UserMFASettingList
* instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withMFAOptions(MFAOptionType... mFAOptions) {
if (this.mFAOptions == null) {
setMFAOptions(new java.util.ArrayList(mFAOptions.length));
}
for (MFAOptionType ele : mFAOptions) {
this.mFAOptions.add(ele);
}
return this;
}
/**
*
* This response parameter is no longer supported. It provides information only about SMS MFA configurations.
* It doesn't provide information about time-based one-time password (TOTP) software token MFA configurations. To
* look up information about either type of MFA configuration, use UserMFASettingList instead.
*
*
* @param mFAOptions
* This response parameter is no longer supported. It provides information only about SMS MFA
* configurations. It doesn't provide information about time-based one-time password (TOTP) software token
* MFA configurations. To look up information about either type of MFA configuration, use UserMFASettingList
* instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withMFAOptions(java.util.Collection mFAOptions) {
setMFAOptions(mFAOptions);
return this;
}
/**
*
* The user's preferred MFA setting.
*
*
* @param preferredMfaSetting
* The user's preferred MFA setting.
*/
public void setPreferredMfaSetting(String preferredMfaSetting) {
this.preferredMfaSetting = preferredMfaSetting;
}
/**
*
* The user's preferred MFA setting.
*
*
* @return The user's preferred MFA setting.
*/
public String getPreferredMfaSetting() {
return this.preferredMfaSetting;
}
/**
*
* The user's preferred MFA setting.
*
*
* @param preferredMfaSetting
* The user's preferred MFA setting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withPreferredMfaSetting(String preferredMfaSetting) {
setPreferredMfaSetting(preferredMfaSetting);
return this;
}
/**
*
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
and
* SOFTWARE_TOKEN_MFA
.
*
*
* @return The MFA options that are activated for the user. The possible values in this list are
* SMS_MFA
and SOFTWARE_TOKEN_MFA
.
*/
public java.util.List getUserMFASettingList() {
return userMFASettingList;
}
/**
*
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
and
* SOFTWARE_TOKEN_MFA
.
*
*
* @param userMFASettingList
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
* and SOFTWARE_TOKEN_MFA
.
*/
public void setUserMFASettingList(java.util.Collection userMFASettingList) {
if (userMFASettingList == null) {
this.userMFASettingList = null;
return;
}
this.userMFASettingList = new java.util.ArrayList(userMFASettingList);
}
/**
*
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
and
* SOFTWARE_TOKEN_MFA
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUserMFASettingList(java.util.Collection)} or {@link #withUserMFASettingList(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param userMFASettingList
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
* and SOFTWARE_TOKEN_MFA
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUserMFASettingList(String... userMFASettingList) {
if (this.userMFASettingList == null) {
setUserMFASettingList(new java.util.ArrayList(userMFASettingList.length));
}
for (String ele : userMFASettingList) {
this.userMFASettingList.add(ele);
}
return this;
}
/**
*
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
and
* SOFTWARE_TOKEN_MFA
.
*
*
* @param userMFASettingList
* The MFA options that are activated for the user. The possible values in this list are SMS_MFA
* and SOFTWARE_TOKEN_MFA
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdminGetUserResult withUserMFASettingList(java.util.Collection userMFASettingList) {
setUserMFASettingList(userMFASettingList);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUsername() != null)
sb.append("Username: ").append("***Sensitive Data Redacted***").append(",");
if (getUserAttributes() != null)
sb.append("UserAttributes: ").append(getUserAttributes()).append(",");
if (getUserCreateDate() != null)
sb.append("UserCreateDate: ").append(getUserCreateDate()).append(",");
if (getUserLastModifiedDate() != null)
sb.append("UserLastModifiedDate: ").append(getUserLastModifiedDate()).append(",");
if (getEnabled() != null)
sb.append("Enabled: ").append(getEnabled()).append(",");
if (getUserStatus() != null)
sb.append("UserStatus: ").append(getUserStatus()).append(",");
if (getMFAOptions() != null)
sb.append("MFAOptions: ").append(getMFAOptions()).append(",");
if (getPreferredMfaSetting() != null)
sb.append("PreferredMfaSetting: ").append(getPreferredMfaSetting()).append(",");
if (getUserMFASettingList() != null)
sb.append("UserMFASettingList: ").append(getUserMFASettingList());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AdminGetUserResult == false)
return false;
AdminGetUserResult other = (AdminGetUserResult) obj;
if (other.getUsername() == null ^ this.getUsername() == null)
return false;
if (other.getUsername() != null && other.getUsername().equals(this.getUsername()) == false)
return false;
if (other.getUserAttributes() == null ^ this.getUserAttributes() == null)
return false;
if (other.getUserAttributes() != null && other.getUserAttributes().equals(this.getUserAttributes()) == false)
return false;
if (other.getUserCreateDate() == null ^ this.getUserCreateDate() == null)
return false;
if (other.getUserCreateDate() != null && other.getUserCreateDate().equals(this.getUserCreateDate()) == false)
return false;
if (other.getUserLastModifiedDate() == null ^ this.getUserLastModifiedDate() == null)
return false;
if (other.getUserLastModifiedDate() != null && other.getUserLastModifiedDate().equals(this.getUserLastModifiedDate()) == false)
return false;
if (other.getEnabled() == null ^ this.getEnabled() == null)
return false;
if (other.getEnabled() != null && other.getEnabled().equals(this.getEnabled()) == false)
return false;
if (other.getUserStatus() == null ^ this.getUserStatus() == null)
return false;
if (other.getUserStatus() != null && other.getUserStatus().equals(this.getUserStatus()) == false)
return false;
if (other.getMFAOptions() == null ^ this.getMFAOptions() == null)
return false;
if (other.getMFAOptions() != null && other.getMFAOptions().equals(this.getMFAOptions()) == false)
return false;
if (other.getPreferredMfaSetting() == null ^ this.getPreferredMfaSetting() == null)
return false;
if (other.getPreferredMfaSetting() != null && other.getPreferredMfaSetting().equals(this.getPreferredMfaSetting()) == false)
return false;
if (other.getUserMFASettingList() == null ^ this.getUserMFASettingList() == null)
return false;
if (other.getUserMFASettingList() != null && other.getUserMFASettingList().equals(this.getUserMFASettingList()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUsername() == null) ? 0 : getUsername().hashCode());
hashCode = prime * hashCode + ((getUserAttributes() == null) ? 0 : getUserAttributes().hashCode());
hashCode = prime * hashCode + ((getUserCreateDate() == null) ? 0 : getUserCreateDate().hashCode());
hashCode = prime * hashCode + ((getUserLastModifiedDate() == null) ? 0 : getUserLastModifiedDate().hashCode());
hashCode = prime * hashCode + ((getEnabled() == null) ? 0 : getEnabled().hashCode());
hashCode = prime * hashCode + ((getUserStatus() == null) ? 0 : getUserStatus().hashCode());
hashCode = prime * hashCode + ((getMFAOptions() == null) ? 0 : getMFAOptions().hashCode());
hashCode = prime * hashCode + ((getPreferredMfaSetting() == null) ? 0 : getPreferredMfaSetting().hashCode());
hashCode = prime * hashCode + ((getUserMFASettingList() == null) ? 0 : getUserMFASettingList().hashCode());
return hashCode;
}
@Override
public AdminGetUserResult clone() {
try {
return (AdminGetUserResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}