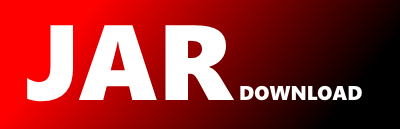
com.amazonaws.services.comprehend.model.CreateEntityRecognizerRequest Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.comprehend.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateEntityRecognizerRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters. Alphanumeric
* characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the account/region.
*
*/
private String recognizerName;
/**
*
* The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique among all
* models with the same recognizer name in the account/ AWS Region.
*
*/
private String versionName;
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon Comprehend read
* access to your input data.
*
*/
private String dataAccessRoleArn;
/**
*
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a metadata
* to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be added to a resource
* to indicate its use by the sales department.
*
*/
private java.util.List tags;
/**
*
* Specifies the format and location of the input data. The S3 bucket containing the input data must be located in
* the same region as the entity recognizer being created.
*
*/
private EntityRecognizerInputDataConfig inputDataConfig;
/**
*
* A unique identifier for the request. If you don't set the client request token, Amazon Comprehend generates one.
*
*/
private String clientRequestToken;
/**
*
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish ("es"),
* French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the same language.
*
*/
private String languageCode;
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the storage volume
* attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId can be either of the
* following formats:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*/
private String volumeKmsKeyId;
/**
*
* Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you are
* using for your custom entity recognizer. For more information, see Amazon VPC.
*
*/
private VpcConfig vpcConfig;
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom models. The
* ModelKmsKeyId can be either of the following formats
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*/
private String modelKmsKeyId;
/**
*
* The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy to allow
* another AWS account to import your custom model.
*
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy, enclose
* the attribute names and values in double quotes. If the JSON body is also enclosed in double quotes, then you
* must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose the JSON
* names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
*
*/
private String modelPolicy;
/**
*
* The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters. Alphanumeric
* characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the account/region.
*
*
* @param recognizerName
* The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the
* account/region.
*/
public void setRecognizerName(String recognizerName) {
this.recognizerName = recognizerName;
}
/**
*
* The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters. Alphanumeric
* characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the account/region.
*
*
* @return The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the
* account/region.
*/
public String getRecognizerName() {
return this.recognizerName;
}
/**
*
* The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters. Alphanumeric
* characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the account/region.
*
*
* @param recognizerName
* The name given to the newly created recognizer. Recognizer names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The name must be unique in the
* account/region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withRecognizerName(String recognizerName) {
setRecognizerName(recognizerName);
return this;
}
/**
*
* The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique among all
* models with the same recognizer name in the account/ AWS Region.
*
*
* @param versionName
* The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique
* among all models with the same recognizer name in the account/ AWS Region.
*/
public void setVersionName(String versionName) {
this.versionName = versionName;
}
/**
*
* The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique among all
* models with the same recognizer name in the account/ AWS Region.
*
*
* @return The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique
* among all models with the same recognizer name in the account/ AWS Region.
*/
public String getVersionName() {
return this.versionName;
}
/**
*
* The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique among all
* models with the same recognizer name in the account/ AWS Region.
*
*
* @param versionName
* The version name given to the newly created recognizer. Version names can be a maximum of 256 characters.
* Alphanumeric characters, hyphens (-) and underscores (_) are allowed. The version name must be unique
* among all models with the same recognizer name in the account/ AWS Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withVersionName(String versionName) {
setVersionName(versionName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon Comprehend read
* access to your input data.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon Comprehend
* read access to your input data.
*/
public void setDataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon Comprehend read
* access to your input data.
*
*
* @return The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon
* Comprehend read access to your input data.
*/
public String getDataAccessRoleArn() {
return this.dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon Comprehend read
* access to your input data.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of the AWS Identity and Management (IAM) role that grants Amazon Comprehend
* read access to your input data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withDataAccessRoleArn(String dataAccessRoleArn) {
setDataAccessRoleArn(dataAccessRoleArn);
return this;
}
/**
*
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a metadata
* to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be added to a resource
* to indicate its use by the sales department.
*
*
* @return Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a
* metadata to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be
* added to a resource to indicate its use by the sales department.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a metadata
* to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be added to a resource
* to indicate its use by the sales department.
*
*
* @param tags
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a
* metadata to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be
* added to a resource to indicate its use by the sales department.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a metadata
* to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be added to a resource
* to indicate its use by the sales department.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a
* metadata to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be
* added to a resource to indicate its use by the sales department.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a metadata
* to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be added to a resource
* to indicate its use by the sales department.
*
*
* @param tags
* Tags to be associated with the entity recognizer being created. A tag is a key-value pair that adds as a
* metadata to a resource used by Amazon Comprehend. For example, a tag with "Sales" as the key might be
* added to a resource to indicate its use by the sales department.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Specifies the format and location of the input data. The S3 bucket containing the input data must be located in
* the same region as the entity recognizer being created.
*
*
* @param inputDataConfig
* Specifies the format and location of the input data. The S3 bucket containing the input data must be
* located in the same region as the entity recognizer being created.
*/
public void setInputDataConfig(EntityRecognizerInputDataConfig inputDataConfig) {
this.inputDataConfig = inputDataConfig;
}
/**
*
* Specifies the format and location of the input data. The S3 bucket containing the input data must be located in
* the same region as the entity recognizer being created.
*
*
* @return Specifies the format and location of the input data. The S3 bucket containing the input data must be
* located in the same region as the entity recognizer being created.
*/
public EntityRecognizerInputDataConfig getInputDataConfig() {
return this.inputDataConfig;
}
/**
*
* Specifies the format and location of the input data. The S3 bucket containing the input data must be located in
* the same region as the entity recognizer being created.
*
*
* @param inputDataConfig
* Specifies the format and location of the input data. The S3 bucket containing the input data must be
* located in the same region as the entity recognizer being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withInputDataConfig(EntityRecognizerInputDataConfig inputDataConfig) {
setInputDataConfig(inputDataConfig);
return this;
}
/**
*
* A unique identifier for the request. If you don't set the client request token, Amazon Comprehend generates one.
*
*
* @param clientRequestToken
* A unique identifier for the request. If you don't set the client request token, Amazon Comprehend
* generates one.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* A unique identifier for the request. If you don't set the client request token, Amazon Comprehend generates one.
*
*
* @return A unique identifier for the request. If you don't set the client request token, Amazon Comprehend
* generates one.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* A unique identifier for the request. If you don't set the client request token, Amazon Comprehend generates one.
*
*
* @param clientRequestToken
* A unique identifier for the request. If you don't set the client request token, Amazon Comprehend
* generates one.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish ("es"),
* French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the same language.
*
*
* @param languageCode
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish
* ("es"), French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the
* same language.
* @see LanguageCode
*/
public void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
/**
*
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish ("es"),
* French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the same language.
*
*
* @return You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish
* ("es"), French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the
* same language.
* @see LanguageCode
*/
public String getLanguageCode() {
return this.languageCode;
}
/**
*
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish ("es"),
* French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the same language.
*
*
* @param languageCode
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish
* ("es"), French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the
* same language.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public CreateEntityRecognizerRequest withLanguageCode(String languageCode) {
setLanguageCode(languageCode);
return this;
}
/**
*
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish ("es"),
* French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the same language.
*
*
* @param languageCode
* You can specify any of the following languages supported by Amazon Comprehend: English ("en"), Spanish
* ("es"), French ("fr"), Italian ("it"), German ("de"), or Portuguese ("pt"). All documents must be in the
* same language.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public CreateEntityRecognizerRequest withLanguageCode(LanguageCode languageCode) {
this.languageCode = languageCode.toString();
return this;
}
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the storage volume
* attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId can be either of the
* following formats:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @param volumeKmsKeyId
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the storage
* volume attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId can be
* either of the following formats:
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*/
public void setVolumeKmsKeyId(String volumeKmsKeyId) {
this.volumeKmsKeyId = volumeKmsKeyId;
}
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the storage volume
* attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId can be either of the
* following formats:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @return ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the
* storage volume attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId
* can be either of the following formats:
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*/
public String getVolumeKmsKeyId() {
return this.volumeKmsKeyId;
}
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the storage volume
* attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId can be either of the
* following formats:
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @param volumeKmsKeyId
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt data on the storage
* volume attached to the ML compute instance(s) that process the analysis job. The VolumeKmsKeyId can be
* either of the following formats:
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withVolumeKmsKeyId(String volumeKmsKeyId) {
setVolumeKmsKeyId(volumeKmsKeyId);
return this;
}
/**
*
* Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you are
* using for your custom entity recognizer. For more information, see Amazon VPC.
*
*
* @param vpcConfig
* Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you
* are using for your custom entity recognizer. For more information, see Amazon VPC.
*/
public void setVpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you are
* using for your custom entity recognizer. For more information, see Amazon VPC.
*
*
* @return Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you
* are using for your custom entity recognizer. For more information, see Amazon VPC.
*/
public VpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you are
* using for your custom entity recognizer. For more information, see Amazon VPC.
*
*
* @param vpcConfig
* Configuration parameters for an optional private Virtual Private Cloud (VPC) containing the resources you
* are using for your custom entity recognizer. For more information, see Amazon VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withVpcConfig(VpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom models. The
* ModelKmsKeyId can be either of the following formats
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @param modelKmsKeyId
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom
* models. The ModelKmsKeyId can be either of the following formats
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*/
public void setModelKmsKeyId(String modelKmsKeyId) {
this.modelKmsKeyId = modelKmsKeyId;
}
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom models. The
* ModelKmsKeyId can be either of the following formats
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @return ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom
* models. The ModelKmsKeyId can be either of the following formats
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*/
public String getModelKmsKeyId() {
return this.modelKmsKeyId;
}
/**
*
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom models. The
* ModelKmsKeyId can be either of the following formats
*
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
*
*
* @param modelKmsKeyId
* ID for the AWS Key Management Service (KMS) key that Amazon Comprehend uses to encrypt trained custom
* models. The ModelKmsKeyId can be either of the following formats
*
* -
*
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* Amazon Resource Name (ARN) of a KMS Key:
* "arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withModelKmsKeyId(String modelKmsKeyId) {
setModelKmsKeyId(modelKmsKeyId);
return this;
}
/**
*
* The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy to allow
* another AWS account to import your custom model.
*
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy, enclose
* the attribute names and values in double quotes. If the JSON body is also enclosed in double quotes, then you
* must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose the JSON
* names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
*
*
* @param modelPolicy
* The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy
* to allow another AWS account to import your custom model.
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy,
* enclose the attribute names and values in double quotes. If the JSON body is also enclosed in double
* quotes, then you must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose the
* JSON names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
*/
public void setModelPolicy(String modelPolicy) {
this.modelPolicy = modelPolicy;
}
/**
*
* The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy to allow
* another AWS account to import your custom model.
*
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy, enclose
* the attribute names and values in double quotes. If the JSON body is also enclosed in double quotes, then you
* must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose the JSON
* names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
*
*
* @return The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy
* to allow another AWS account to import your custom model.
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy,
* enclose the attribute names and values in double quotes. If the JSON body is also enclosed in double
* quotes, then you must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose
* the JSON names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
*/
public String getModelPolicy() {
return this.modelPolicy;
}
/**
*
* The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy to allow
* another AWS account to import your custom model.
*
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy, enclose
* the attribute names and values in double quotes. If the JSON body is also enclosed in double quotes, then you
* must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose the JSON
* names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
*
*
* @param modelPolicy
* The JSON resource-based policy to attach to your custom entity recognizer model. You can use this policy
* to allow another AWS account to import your custom model.
*
* Provide your JSON as a UTF-8 encoded string without line breaks. To provide valid JSON for your policy,
* enclose the attribute names and values in double quotes. If the JSON body is also enclosed in double
* quotes, then you must escape the double quotes that are inside the policy:
*
*
* "{\"attribute\": \"value\", \"attribute\": [\"value\"]}"
*
*
* To avoid escaping quotes, you can use single quotes to enclose the policy and double quotes to enclose the
* JSON names and values:
*
*
* '{"attribute": "value", "attribute": ["value"]}'
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEntityRecognizerRequest withModelPolicy(String modelPolicy) {
setModelPolicy(modelPolicy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRecognizerName() != null)
sb.append("RecognizerName: ").append(getRecognizerName()).append(",");
if (getVersionName() != null)
sb.append("VersionName: ").append(getVersionName()).append(",");
if (getDataAccessRoleArn() != null)
sb.append("DataAccessRoleArn: ").append(getDataAccessRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getInputDataConfig() != null)
sb.append("InputDataConfig: ").append(getInputDataConfig()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getLanguageCode() != null)
sb.append("LanguageCode: ").append(getLanguageCode()).append(",");
if (getVolumeKmsKeyId() != null)
sb.append("VolumeKmsKeyId: ").append(getVolumeKmsKeyId()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getModelKmsKeyId() != null)
sb.append("ModelKmsKeyId: ").append(getModelKmsKeyId()).append(",");
if (getModelPolicy() != null)
sb.append("ModelPolicy: ").append(getModelPolicy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateEntityRecognizerRequest == false)
return false;
CreateEntityRecognizerRequest other = (CreateEntityRecognizerRequest) obj;
if (other.getRecognizerName() == null ^ this.getRecognizerName() == null)
return false;
if (other.getRecognizerName() != null && other.getRecognizerName().equals(this.getRecognizerName()) == false)
return false;
if (other.getVersionName() == null ^ this.getVersionName() == null)
return false;
if (other.getVersionName() != null && other.getVersionName().equals(this.getVersionName()) == false)
return false;
if (other.getDataAccessRoleArn() == null ^ this.getDataAccessRoleArn() == null)
return false;
if (other.getDataAccessRoleArn() != null && other.getDataAccessRoleArn().equals(this.getDataAccessRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getInputDataConfig() == null ^ this.getInputDataConfig() == null)
return false;
if (other.getInputDataConfig() != null && other.getInputDataConfig().equals(this.getInputDataConfig()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getLanguageCode() == null ^ this.getLanguageCode() == null)
return false;
if (other.getLanguageCode() != null && other.getLanguageCode().equals(this.getLanguageCode()) == false)
return false;
if (other.getVolumeKmsKeyId() == null ^ this.getVolumeKmsKeyId() == null)
return false;
if (other.getVolumeKmsKeyId() != null && other.getVolumeKmsKeyId().equals(this.getVolumeKmsKeyId()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getModelKmsKeyId() == null ^ this.getModelKmsKeyId() == null)
return false;
if (other.getModelKmsKeyId() != null && other.getModelKmsKeyId().equals(this.getModelKmsKeyId()) == false)
return false;
if (other.getModelPolicy() == null ^ this.getModelPolicy() == null)
return false;
if (other.getModelPolicy() != null && other.getModelPolicy().equals(this.getModelPolicy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRecognizerName() == null) ? 0 : getRecognizerName().hashCode());
hashCode = prime * hashCode + ((getVersionName() == null) ? 0 : getVersionName().hashCode());
hashCode = prime * hashCode + ((getDataAccessRoleArn() == null) ? 0 : getDataAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getInputDataConfig() == null) ? 0 : getInputDataConfig().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getLanguageCode() == null) ? 0 : getLanguageCode().hashCode());
hashCode = prime * hashCode + ((getVolumeKmsKeyId() == null) ? 0 : getVolumeKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getModelKmsKeyId() == null) ? 0 : getModelKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getModelPolicy() == null) ? 0 : getModelPolicy().hashCode());
return hashCode;
}
@Override
public CreateEntityRecognizerRequest clone() {
return (CreateEntityRecognizerRequest) super.clone();
}
}