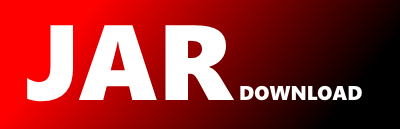
com.amazonaws.services.computeoptimizer.model.AutoScalingGroupRecommendation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-computeoptimizer Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.computeoptimizer.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes an Auto Scaling group recommendation.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AutoScalingGroupRecommendation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Web Services account ID of the Auto Scaling group.
*
*/
private String accountId;
/**
*
* The Amazon Resource Name (ARN) of the Auto Scaling group.
*
*/
private String autoScalingGroupArn;
/**
*
* The name of the Auto Scaling group.
*
*/
private String autoScalingGroupName;
/**
*
* The finding classification of the Auto Scaling group.
*
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute Optimizer
* identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer determines
* that the group is correctly provisioned to run your workload based on the chosen instance type. For optimized
* resources, Compute Optimizer might recommend a new generation instance type.
*
*
*
*/
private String finding;
/**
*
* An array of objects that describe the utilization metrics of the Auto Scaling group.
*
*/
private java.util.List utilizationMetrics;
/**
*
* The number of days for which utilization metrics were analyzed for the Auto Scaling group.
*
*/
private Double lookBackPeriodInDays;
/**
*
* An array of objects that describe the current configuration of the Auto Scaling group.
*
*/
private AutoScalingGroupConfiguration currentConfiguration;
/**
*
* Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
*
*/
private GpuInfo currentInstanceGpuInfo;
/**
*
* An array of objects that describe the recommendation options for the Auto Scaling group.
*
*/
private java.util.List recommendationOptions;
/**
*
* The timestamp of when the Auto Scaling group recommendation was last generated.
*
*/
private java.util.Date lastRefreshTimestamp;
/**
*
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current Auto Scaling group configuration has insufficient capacity and cannot meet
* workload requirements.
*
*/
private String currentPerformanceRisk;
/**
*
* An object that describes the effective recommendation preferences for the Auto Scaling group.
*
*/
private EffectiveRecommendationPreferences effectiveRecommendationPreferences;
/**
*
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
*
*/
private java.util.List inferredWorkloadTypes;
/**
*
* The Amazon Web Services account ID of the Auto Scaling group.
*
*
* @param accountId
* The Amazon Web Services account ID of the Auto Scaling group.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The Amazon Web Services account ID of the Auto Scaling group.
*
*
* @return The Amazon Web Services account ID of the Auto Scaling group.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The Amazon Web Services account ID of the Auto Scaling group.
*
*
* @param accountId
* The Amazon Web Services account ID of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Auto Scaling group.
*
*
* @param autoScalingGroupArn
* The Amazon Resource Name (ARN) of the Auto Scaling group.
*/
public void setAutoScalingGroupArn(String autoScalingGroupArn) {
this.autoScalingGroupArn = autoScalingGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Auto Scaling group.
*
*
* @return The Amazon Resource Name (ARN) of the Auto Scaling group.
*/
public String getAutoScalingGroupArn() {
return this.autoScalingGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Auto Scaling group.
*
*
* @param autoScalingGroupArn
* The Amazon Resource Name (ARN) of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withAutoScalingGroupArn(String autoScalingGroupArn) {
setAutoScalingGroupArn(autoScalingGroupArn);
return this;
}
/**
*
* The name of the Auto Scaling group.
*
*
* @param autoScalingGroupName
* The name of the Auto Scaling group.
*/
public void setAutoScalingGroupName(String autoScalingGroupName) {
this.autoScalingGroupName = autoScalingGroupName;
}
/**
*
* The name of the Auto Scaling group.
*
*
* @return The name of the Auto Scaling group.
*/
public String getAutoScalingGroupName() {
return this.autoScalingGroupName;
}
/**
*
* The name of the Auto Scaling group.
*
*
* @param autoScalingGroupName
* The name of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withAutoScalingGroupName(String autoScalingGroupName) {
setAutoScalingGroupName(autoScalingGroupName);
return this;
}
/**
*
* The finding classification of the Auto Scaling group.
*
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute Optimizer
* identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer determines
* that the group is correctly provisioned to run your workload based on the chosen instance type. For optimized
* resources, Compute Optimizer might recommend a new generation instance type.
*
*
*
*
* @param finding
* The finding classification of the Auto Scaling group.
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute
* Optimizer identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer
* determines that the group is correctly provisioned to run your workload based on the chosen instance type.
* For optimized resources, Compute Optimizer might recommend a new generation instance type.
*
*
* @see Finding
*/
public void setFinding(String finding) {
this.finding = finding;
}
/**
*
* The finding classification of the Auto Scaling group.
*
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute Optimizer
* identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer determines
* that the group is correctly provisioned to run your workload based on the chosen instance type. For optimized
* resources, Compute Optimizer might recommend a new generation instance type.
*
*
*
*
* @return The finding classification of the Auto Scaling group.
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute
* Optimizer identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer
* determines that the group is correctly provisioned to run your workload based on the chosen instance
* type. For optimized resources, Compute Optimizer might recommend a new generation instance type.
*
*
* @see Finding
*/
public String getFinding() {
return this.finding;
}
/**
*
* The finding classification of the Auto Scaling group.
*
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute Optimizer
* identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer determines
* that the group is correctly provisioned to run your workload based on the chosen instance type. For optimized
* resources, Compute Optimizer might recommend a new generation instance type.
*
*
*
*
* @param finding
* The finding classification of the Auto Scaling group.
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute
* Optimizer identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer
* determines that the group is correctly provisioned to run your workload based on the chosen instance type.
* For optimized resources, Compute Optimizer might recommend a new generation instance type.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Finding
*/
public AutoScalingGroupRecommendation withFinding(String finding) {
setFinding(finding);
return this;
}
/**
*
* The finding classification of the Auto Scaling group.
*
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute Optimizer
* identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer determines
* that the group is correctly provisioned to run your workload based on the chosen instance type. For optimized
* resources, Compute Optimizer might recommend a new generation instance type.
*
*
*
*
* @param finding
* The finding classification of the Auto Scaling group.
*
* Findings for Auto Scaling groups include:
*
*
* -
*
* NotOptimized
—An Auto Scaling group is considered not optimized when Compute
* Optimizer identifies a recommendation that can provide better performance for your workload.
*
*
* -
*
* Optimized
—An Auto Scaling group is considered optimized when Compute Optimizer
* determines that the group is correctly provisioned to run your workload based on the chosen instance type.
* For optimized resources, Compute Optimizer might recommend a new generation instance type.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Finding
*/
public AutoScalingGroupRecommendation withFinding(Finding finding) {
this.finding = finding.toString();
return this;
}
/**
*
* An array of objects that describe the utilization metrics of the Auto Scaling group.
*
*
* @return An array of objects that describe the utilization metrics of the Auto Scaling group.
*/
public java.util.List getUtilizationMetrics() {
return utilizationMetrics;
}
/**
*
* An array of objects that describe the utilization metrics of the Auto Scaling group.
*
*
* @param utilizationMetrics
* An array of objects that describe the utilization metrics of the Auto Scaling group.
*/
public void setUtilizationMetrics(java.util.Collection utilizationMetrics) {
if (utilizationMetrics == null) {
this.utilizationMetrics = null;
return;
}
this.utilizationMetrics = new java.util.ArrayList(utilizationMetrics);
}
/**
*
* An array of objects that describe the utilization metrics of the Auto Scaling group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUtilizationMetrics(java.util.Collection)} or {@link #withUtilizationMetrics(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param utilizationMetrics
* An array of objects that describe the utilization metrics of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withUtilizationMetrics(UtilizationMetric... utilizationMetrics) {
if (this.utilizationMetrics == null) {
setUtilizationMetrics(new java.util.ArrayList(utilizationMetrics.length));
}
for (UtilizationMetric ele : utilizationMetrics) {
this.utilizationMetrics.add(ele);
}
return this;
}
/**
*
* An array of objects that describe the utilization metrics of the Auto Scaling group.
*
*
* @param utilizationMetrics
* An array of objects that describe the utilization metrics of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withUtilizationMetrics(java.util.Collection utilizationMetrics) {
setUtilizationMetrics(utilizationMetrics);
return this;
}
/**
*
* The number of days for which utilization metrics were analyzed for the Auto Scaling group.
*
*
* @param lookBackPeriodInDays
* The number of days for which utilization metrics were analyzed for the Auto Scaling group.
*/
public void setLookBackPeriodInDays(Double lookBackPeriodInDays) {
this.lookBackPeriodInDays = lookBackPeriodInDays;
}
/**
*
* The number of days for which utilization metrics were analyzed for the Auto Scaling group.
*
*
* @return The number of days for which utilization metrics were analyzed for the Auto Scaling group.
*/
public Double getLookBackPeriodInDays() {
return this.lookBackPeriodInDays;
}
/**
*
* The number of days for which utilization metrics were analyzed for the Auto Scaling group.
*
*
* @param lookBackPeriodInDays
* The number of days for which utilization metrics were analyzed for the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withLookBackPeriodInDays(Double lookBackPeriodInDays) {
setLookBackPeriodInDays(lookBackPeriodInDays);
return this;
}
/**
*
* An array of objects that describe the current configuration of the Auto Scaling group.
*
*
* @param currentConfiguration
* An array of objects that describe the current configuration of the Auto Scaling group.
*/
public void setCurrentConfiguration(AutoScalingGroupConfiguration currentConfiguration) {
this.currentConfiguration = currentConfiguration;
}
/**
*
* An array of objects that describe the current configuration of the Auto Scaling group.
*
*
* @return An array of objects that describe the current configuration of the Auto Scaling group.
*/
public AutoScalingGroupConfiguration getCurrentConfiguration() {
return this.currentConfiguration;
}
/**
*
* An array of objects that describe the current configuration of the Auto Scaling group.
*
*
* @param currentConfiguration
* An array of objects that describe the current configuration of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withCurrentConfiguration(AutoScalingGroupConfiguration currentConfiguration) {
setCurrentConfiguration(currentConfiguration);
return this;
}
/**
*
* Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
*
*
* @param currentInstanceGpuInfo
* Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
*/
public void setCurrentInstanceGpuInfo(GpuInfo currentInstanceGpuInfo) {
this.currentInstanceGpuInfo = currentInstanceGpuInfo;
}
/**
*
* Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
*
*
* @return Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
*/
public GpuInfo getCurrentInstanceGpuInfo() {
return this.currentInstanceGpuInfo;
}
/**
*
* Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
*
*
* @param currentInstanceGpuInfo
* Describes the GPU accelerator settings for the current instance type of the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withCurrentInstanceGpuInfo(GpuInfo currentInstanceGpuInfo) {
setCurrentInstanceGpuInfo(currentInstanceGpuInfo);
return this;
}
/**
*
* An array of objects that describe the recommendation options for the Auto Scaling group.
*
*
* @return An array of objects that describe the recommendation options for the Auto Scaling group.
*/
public java.util.List getRecommendationOptions() {
return recommendationOptions;
}
/**
*
* An array of objects that describe the recommendation options for the Auto Scaling group.
*
*
* @param recommendationOptions
* An array of objects that describe the recommendation options for the Auto Scaling group.
*/
public void setRecommendationOptions(java.util.Collection recommendationOptions) {
if (recommendationOptions == null) {
this.recommendationOptions = null;
return;
}
this.recommendationOptions = new java.util.ArrayList(recommendationOptions);
}
/**
*
* An array of objects that describe the recommendation options for the Auto Scaling group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRecommendationOptions(java.util.Collection)} or
* {@link #withRecommendationOptions(java.util.Collection)} if you want to override the existing values.
*
*
* @param recommendationOptions
* An array of objects that describe the recommendation options for the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withRecommendationOptions(AutoScalingGroupRecommendationOption... recommendationOptions) {
if (this.recommendationOptions == null) {
setRecommendationOptions(new java.util.ArrayList(recommendationOptions.length));
}
for (AutoScalingGroupRecommendationOption ele : recommendationOptions) {
this.recommendationOptions.add(ele);
}
return this;
}
/**
*
* An array of objects that describe the recommendation options for the Auto Scaling group.
*
*
* @param recommendationOptions
* An array of objects that describe the recommendation options for the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withRecommendationOptions(java.util.Collection recommendationOptions) {
setRecommendationOptions(recommendationOptions);
return this;
}
/**
*
* The timestamp of when the Auto Scaling group recommendation was last generated.
*
*
* @param lastRefreshTimestamp
* The timestamp of when the Auto Scaling group recommendation was last generated.
*/
public void setLastRefreshTimestamp(java.util.Date lastRefreshTimestamp) {
this.lastRefreshTimestamp = lastRefreshTimestamp;
}
/**
*
* The timestamp of when the Auto Scaling group recommendation was last generated.
*
*
* @return The timestamp of when the Auto Scaling group recommendation was last generated.
*/
public java.util.Date getLastRefreshTimestamp() {
return this.lastRefreshTimestamp;
}
/**
*
* The timestamp of when the Auto Scaling group recommendation was last generated.
*
*
* @param lastRefreshTimestamp
* The timestamp of when the Auto Scaling group recommendation was last generated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withLastRefreshTimestamp(java.util.Date lastRefreshTimestamp) {
setLastRefreshTimestamp(lastRefreshTimestamp);
return this;
}
/**
*
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current Auto Scaling group configuration has insufficient capacity and cannot meet
* workload requirements.
*
*
* @param currentPerformanceRisk
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current Auto Scaling group configuration has insufficient capacity and
* cannot meet workload requirements.
* @see CurrentPerformanceRisk
*/
public void setCurrentPerformanceRisk(String currentPerformanceRisk) {
this.currentPerformanceRisk = currentPerformanceRisk;
}
/**
*
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current Auto Scaling group configuration has insufficient capacity and cannot meet
* workload requirements.
*
*
* @return The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current Auto Scaling group configuration has insufficient capacity and
* cannot meet workload requirements.
* @see CurrentPerformanceRisk
*/
public String getCurrentPerformanceRisk() {
return this.currentPerformanceRisk;
}
/**
*
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current Auto Scaling group configuration has insufficient capacity and cannot meet
* workload requirements.
*
*
* @param currentPerformanceRisk
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current Auto Scaling group configuration has insufficient capacity and
* cannot meet workload requirements.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrentPerformanceRisk
*/
public AutoScalingGroupRecommendation withCurrentPerformanceRisk(String currentPerformanceRisk) {
setCurrentPerformanceRisk(currentPerformanceRisk);
return this;
}
/**
*
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current Auto Scaling group configuration has insufficient capacity and cannot meet
* workload requirements.
*
*
* @param currentPerformanceRisk
* The risk of the current Auto Scaling group not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current Auto Scaling group configuration has insufficient capacity and
* cannot meet workload requirements.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrentPerformanceRisk
*/
public AutoScalingGroupRecommendation withCurrentPerformanceRisk(CurrentPerformanceRisk currentPerformanceRisk) {
this.currentPerformanceRisk = currentPerformanceRisk.toString();
return this;
}
/**
*
* An object that describes the effective recommendation preferences for the Auto Scaling group.
*
*
* @param effectiveRecommendationPreferences
* An object that describes the effective recommendation preferences for the Auto Scaling group.
*/
public void setEffectiveRecommendationPreferences(EffectiveRecommendationPreferences effectiveRecommendationPreferences) {
this.effectiveRecommendationPreferences = effectiveRecommendationPreferences;
}
/**
*
* An object that describes the effective recommendation preferences for the Auto Scaling group.
*
*
* @return An object that describes the effective recommendation preferences for the Auto Scaling group.
*/
public EffectiveRecommendationPreferences getEffectiveRecommendationPreferences() {
return this.effectiveRecommendationPreferences;
}
/**
*
* An object that describes the effective recommendation preferences for the Auto Scaling group.
*
*
* @param effectiveRecommendationPreferences
* An object that describes the effective recommendation preferences for the Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingGroupRecommendation withEffectiveRecommendationPreferences(EffectiveRecommendationPreferences effectiveRecommendationPreferences) {
setEffectiveRecommendationPreferences(effectiveRecommendationPreferences);
return this;
}
/**
*
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
*
*
* @return The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
* @see InferredWorkloadType
*/
public java.util.List getInferredWorkloadTypes() {
return inferredWorkloadTypes;
}
/**
*
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
*
*
* @param inferredWorkloadTypes
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
* @see InferredWorkloadType
*/
public void setInferredWorkloadTypes(java.util.Collection inferredWorkloadTypes) {
if (inferredWorkloadTypes == null) {
this.inferredWorkloadTypes = null;
return;
}
this.inferredWorkloadTypes = new java.util.ArrayList(inferredWorkloadTypes);
}
/**
*
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInferredWorkloadTypes(java.util.Collection)} or
* {@link #withInferredWorkloadTypes(java.util.Collection)} if you want to override the existing values.
*
*
* @param inferredWorkloadTypes
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadType
*/
public AutoScalingGroupRecommendation withInferredWorkloadTypes(String... inferredWorkloadTypes) {
if (this.inferredWorkloadTypes == null) {
setInferredWorkloadTypes(new java.util.ArrayList(inferredWorkloadTypes.length));
}
for (String ele : inferredWorkloadTypes) {
this.inferredWorkloadTypes.add(ele);
}
return this;
}
/**
*
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
*
*
* @param inferredWorkloadTypes
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadType
*/
public AutoScalingGroupRecommendation withInferredWorkloadTypes(java.util.Collection inferredWorkloadTypes) {
setInferredWorkloadTypes(inferredWorkloadTypes);
return this;
}
/**
*
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
*
*
* @param inferredWorkloadTypes
* The applications that might be running on the instances in the Auto Scaling group as inferred by Compute
* Optimizer.
*
* Compute Optimizer can infer if one of the following applications might be running on the instances:
*
*
* -
*
* AmazonEmr
- Infers that Amazon EMR might be running on the instances.
*
*
* -
*
* ApacheCassandra
- Infers that Apache Cassandra might be running on the instances.
*
*
* -
*
* ApacheHadoop
- Infers that Apache Hadoop might be running on the instances.
*
*
* -
*
* Memcached
- Infers that Memcached might be running on the instances.
*
*
* -
*
* NGINX
- Infers that NGINX might be running on the instances.
*
*
* -
*
* PostgreSql
- Infers that PostgreSQL might be running on the instances.
*
*
* -
*
* Redis
- Infers that Redis might be running on the instances.
*
*
* -
*
* Kafka
- Infers that Kafka might be running on the instance.
*
*
* -
*
* SQLServer
- Infers that SQLServer might be running on the instance.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadType
*/
public AutoScalingGroupRecommendation withInferredWorkloadTypes(InferredWorkloadType... inferredWorkloadTypes) {
java.util.ArrayList inferredWorkloadTypesCopy = new java.util.ArrayList(inferredWorkloadTypes.length);
for (InferredWorkloadType value : inferredWorkloadTypes) {
inferredWorkloadTypesCopy.add(value.toString());
}
if (getInferredWorkloadTypes() == null) {
setInferredWorkloadTypes(inferredWorkloadTypesCopy);
} else {
getInferredWorkloadTypes().addAll(inferredWorkloadTypesCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getAutoScalingGroupArn() != null)
sb.append("AutoScalingGroupArn: ").append(getAutoScalingGroupArn()).append(",");
if (getAutoScalingGroupName() != null)
sb.append("AutoScalingGroupName: ").append(getAutoScalingGroupName()).append(",");
if (getFinding() != null)
sb.append("Finding: ").append(getFinding()).append(",");
if (getUtilizationMetrics() != null)
sb.append("UtilizationMetrics: ").append(getUtilizationMetrics()).append(",");
if (getLookBackPeriodInDays() != null)
sb.append("LookBackPeriodInDays: ").append(getLookBackPeriodInDays()).append(",");
if (getCurrentConfiguration() != null)
sb.append("CurrentConfiguration: ").append(getCurrentConfiguration()).append(",");
if (getCurrentInstanceGpuInfo() != null)
sb.append("CurrentInstanceGpuInfo: ").append(getCurrentInstanceGpuInfo()).append(",");
if (getRecommendationOptions() != null)
sb.append("RecommendationOptions: ").append(getRecommendationOptions()).append(",");
if (getLastRefreshTimestamp() != null)
sb.append("LastRefreshTimestamp: ").append(getLastRefreshTimestamp()).append(",");
if (getCurrentPerformanceRisk() != null)
sb.append("CurrentPerformanceRisk: ").append(getCurrentPerformanceRisk()).append(",");
if (getEffectiveRecommendationPreferences() != null)
sb.append("EffectiveRecommendationPreferences: ").append(getEffectiveRecommendationPreferences()).append(",");
if (getInferredWorkloadTypes() != null)
sb.append("InferredWorkloadTypes: ").append(getInferredWorkloadTypes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AutoScalingGroupRecommendation == false)
return false;
AutoScalingGroupRecommendation other = (AutoScalingGroupRecommendation) obj;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getAutoScalingGroupArn() == null ^ this.getAutoScalingGroupArn() == null)
return false;
if (other.getAutoScalingGroupArn() != null && other.getAutoScalingGroupArn().equals(this.getAutoScalingGroupArn()) == false)
return false;
if (other.getAutoScalingGroupName() == null ^ this.getAutoScalingGroupName() == null)
return false;
if (other.getAutoScalingGroupName() != null && other.getAutoScalingGroupName().equals(this.getAutoScalingGroupName()) == false)
return false;
if (other.getFinding() == null ^ this.getFinding() == null)
return false;
if (other.getFinding() != null && other.getFinding().equals(this.getFinding()) == false)
return false;
if (other.getUtilizationMetrics() == null ^ this.getUtilizationMetrics() == null)
return false;
if (other.getUtilizationMetrics() != null && other.getUtilizationMetrics().equals(this.getUtilizationMetrics()) == false)
return false;
if (other.getLookBackPeriodInDays() == null ^ this.getLookBackPeriodInDays() == null)
return false;
if (other.getLookBackPeriodInDays() != null && other.getLookBackPeriodInDays().equals(this.getLookBackPeriodInDays()) == false)
return false;
if (other.getCurrentConfiguration() == null ^ this.getCurrentConfiguration() == null)
return false;
if (other.getCurrentConfiguration() != null && other.getCurrentConfiguration().equals(this.getCurrentConfiguration()) == false)
return false;
if (other.getCurrentInstanceGpuInfo() == null ^ this.getCurrentInstanceGpuInfo() == null)
return false;
if (other.getCurrentInstanceGpuInfo() != null && other.getCurrentInstanceGpuInfo().equals(this.getCurrentInstanceGpuInfo()) == false)
return false;
if (other.getRecommendationOptions() == null ^ this.getRecommendationOptions() == null)
return false;
if (other.getRecommendationOptions() != null && other.getRecommendationOptions().equals(this.getRecommendationOptions()) == false)
return false;
if (other.getLastRefreshTimestamp() == null ^ this.getLastRefreshTimestamp() == null)
return false;
if (other.getLastRefreshTimestamp() != null && other.getLastRefreshTimestamp().equals(this.getLastRefreshTimestamp()) == false)
return false;
if (other.getCurrentPerformanceRisk() == null ^ this.getCurrentPerformanceRisk() == null)
return false;
if (other.getCurrentPerformanceRisk() != null && other.getCurrentPerformanceRisk().equals(this.getCurrentPerformanceRisk()) == false)
return false;
if (other.getEffectiveRecommendationPreferences() == null ^ this.getEffectiveRecommendationPreferences() == null)
return false;
if (other.getEffectiveRecommendationPreferences() != null
&& other.getEffectiveRecommendationPreferences().equals(this.getEffectiveRecommendationPreferences()) == false)
return false;
if (other.getInferredWorkloadTypes() == null ^ this.getInferredWorkloadTypes() == null)
return false;
if (other.getInferredWorkloadTypes() != null && other.getInferredWorkloadTypes().equals(this.getInferredWorkloadTypes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroupArn() == null) ? 0 : getAutoScalingGroupArn().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroupName() == null) ? 0 : getAutoScalingGroupName().hashCode());
hashCode = prime * hashCode + ((getFinding() == null) ? 0 : getFinding().hashCode());
hashCode = prime * hashCode + ((getUtilizationMetrics() == null) ? 0 : getUtilizationMetrics().hashCode());
hashCode = prime * hashCode + ((getLookBackPeriodInDays() == null) ? 0 : getLookBackPeriodInDays().hashCode());
hashCode = prime * hashCode + ((getCurrentConfiguration() == null) ? 0 : getCurrentConfiguration().hashCode());
hashCode = prime * hashCode + ((getCurrentInstanceGpuInfo() == null) ? 0 : getCurrentInstanceGpuInfo().hashCode());
hashCode = prime * hashCode + ((getRecommendationOptions() == null) ? 0 : getRecommendationOptions().hashCode());
hashCode = prime * hashCode + ((getLastRefreshTimestamp() == null) ? 0 : getLastRefreshTimestamp().hashCode());
hashCode = prime * hashCode + ((getCurrentPerformanceRisk() == null) ? 0 : getCurrentPerformanceRisk().hashCode());
hashCode = prime * hashCode + ((getEffectiveRecommendationPreferences() == null) ? 0 : getEffectiveRecommendationPreferences().hashCode());
hashCode = prime * hashCode + ((getInferredWorkloadTypes() == null) ? 0 : getInferredWorkloadTypes().hashCode());
return hashCode;
}
@Override
public AutoScalingGroupRecommendation clone() {
try {
return (AutoScalingGroupRecommendation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.computeoptimizer.model.transform.AutoScalingGroupRecommendationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}